metaflow 2.12.36__py2.py3-none-any.whl → 2.12.37__py2.py3-none-any.whl
This diff represents the content of publicly available package versions that have been released to one of the supported registries. The information contained in this diff is provided for informational purposes only and reflects changes between package versions as they appear in their respective public registries.
- metaflow/__init__.py +3 -0
- metaflow/cli.py +84 -697
- metaflow/cli_args.py +17 -0
- metaflow/cli_components/__init__.py +0 -0
- metaflow/cli_components/dump_cmd.py +96 -0
- metaflow/cli_components/init_cmd.py +51 -0
- metaflow/cli_components/run_cmds.py +358 -0
- metaflow/cli_components/step_cmd.py +189 -0
- metaflow/cli_components/utils.py +140 -0
- metaflow/cmd/develop/stub_generator.py +9 -2
- metaflow/decorators.py +54 -2
- metaflow/extension_support/plugins.py +41 -27
- metaflow/flowspec.py +156 -16
- metaflow/includefile.py +50 -22
- metaflow/metaflow_config.py +1 -1
- metaflow/package.py +17 -3
- metaflow/parameters.py +80 -23
- metaflow/plugins/__init__.py +4 -0
- metaflow/plugins/airflow/airflow_cli.py +1 -0
- metaflow/plugins/argo/argo_workflows.py +41 -1
- metaflow/plugins/argo/argo_workflows_cli.py +1 -0
- metaflow/plugins/aws/batch/batch_decorator.py +2 -2
- metaflow/plugins/aws/step_functions/step_functions.py +32 -0
- metaflow/plugins/aws/step_functions/step_functions_cli.py +1 -0
- metaflow/plugins/datatools/s3/s3op.py +3 -3
- metaflow/plugins/kubernetes/kubernetes_cli.py +1 -1
- metaflow/plugins/kubernetes/kubernetes_decorator.py +2 -2
- metaflow/plugins/pypi/conda_decorator.py +22 -0
- metaflow/plugins/pypi/pypi_decorator.py +1 -0
- metaflow/plugins/timeout_decorator.py +2 -2
- metaflow/runner/click_api.py +73 -19
- metaflow/runtime.py +111 -73
- metaflow/sidecar/sidecar_worker.py +1 -1
- metaflow/user_configs/__init__.py +0 -0
- metaflow/user_configs/config_decorators.py +563 -0
- metaflow/user_configs/config_options.py +495 -0
- metaflow/user_configs/config_parameters.py +386 -0
- metaflow/util.py +17 -0
- metaflow/version.py +1 -1
- {metaflow-2.12.36.dist-info → metaflow-2.12.37.dist-info}/METADATA +3 -2
- {metaflow-2.12.36.dist-info → metaflow-2.12.37.dist-info}/RECORD +45 -35
- {metaflow-2.12.36.dist-info → metaflow-2.12.37.dist-info}/LICENSE +0 -0
- {metaflow-2.12.36.dist-info → metaflow-2.12.37.dist-info}/WHEEL +0 -0
- {metaflow-2.12.36.dist-info → metaflow-2.12.37.dist-info}/entry_points.txt +0 -0
- {metaflow-2.12.36.dist-info → metaflow-2.12.37.dist-info}/top_level.txt +0 -0
@@ -0,0 +1,386 @@
|
|
1
|
+
import collections.abc
|
2
|
+
import json
|
3
|
+
import os
|
4
|
+
import re
|
5
|
+
|
6
|
+
from typing import Any, Callable, Dict, Optional, TYPE_CHECKING, Union
|
7
|
+
|
8
|
+
|
9
|
+
from ..exception import MetaflowException
|
10
|
+
|
11
|
+
from ..parameters import (
|
12
|
+
Parameter,
|
13
|
+
ParameterContext,
|
14
|
+
current_flow,
|
15
|
+
)
|
16
|
+
|
17
|
+
if TYPE_CHECKING:
|
18
|
+
from metaflow import FlowSpec
|
19
|
+
|
20
|
+
# _tracefunc_depth = 0
|
21
|
+
|
22
|
+
|
23
|
+
# def tracefunc(func):
|
24
|
+
# """Decorates a function to show its trace."""
|
25
|
+
|
26
|
+
# @functools.wraps(func)
|
27
|
+
# def tracefunc_closure(*args, **kwargs):
|
28
|
+
# global _tracefunc_depth
|
29
|
+
# """The closure."""
|
30
|
+
# print(f"{_tracefunc_depth}: {func.__name__}(args={args}, kwargs={kwargs})")
|
31
|
+
# _tracefunc_depth += 1
|
32
|
+
# result = func(*args, **kwargs)
|
33
|
+
# _tracefunc_depth -= 1
|
34
|
+
# print(f"{_tracefunc_depth} => {result}")
|
35
|
+
# return result
|
36
|
+
|
37
|
+
# return tracefunc_closure
|
38
|
+
|
39
|
+
CONFIG_FILE = os.path.join(
|
40
|
+
os.path.dirname(os.path.abspath(__file__)), "CONFIG_PARAMETERS"
|
41
|
+
)
|
42
|
+
|
43
|
+
ID_PATTERN = re.compile(r"^[a-zA-Z_][a-zA-Z0-9_]*$")
|
44
|
+
|
45
|
+
UNPACK_KEY = "_unpacked_delayed_"
|
46
|
+
|
47
|
+
|
48
|
+
def dump_config_values(flow: "FlowSpec"):
|
49
|
+
from ..flowspec import _FlowState # Prevent circular import
|
50
|
+
|
51
|
+
configs = flow._flow_state.get(_FlowState.CONFIGS)
|
52
|
+
if configs:
|
53
|
+
return {"user_configs": configs}
|
54
|
+
return {}
|
55
|
+
|
56
|
+
|
57
|
+
class ConfigValue(collections.abc.Mapping):
|
58
|
+
"""
|
59
|
+
ConfigValue is a thin wrapper around an arbitrarily nested dictionary-like
|
60
|
+
configuration object. It allows you to access elements of this nested structure
|
61
|
+
using either a "." notation or a [] notation. As an example, if your configuration
|
62
|
+
object is:
|
63
|
+
{"foo": {"bar": 42}}
|
64
|
+
you can access the value 42 using either config["foo"]["bar"] or config.foo.bar.
|
65
|
+
|
66
|
+
All "keys"" need to be valid Python identifiers
|
67
|
+
"""
|
68
|
+
|
69
|
+
# Thin wrapper to allow configuration values to be accessed using a "." notation
|
70
|
+
# as well as a [] notation.
|
71
|
+
|
72
|
+
def __init__(self, data: Dict[str, Any]):
|
73
|
+
if any(not ID_PATTERN.match(k) for k in data.keys()):
|
74
|
+
raise MetaflowException(
|
75
|
+
"All keys in the configuration must be valid Python identifiers"
|
76
|
+
)
|
77
|
+
self._data = data
|
78
|
+
|
79
|
+
def __getattr__(self, key: str) -> Any:
|
80
|
+
"""
|
81
|
+
Access an element of this configuration
|
82
|
+
|
83
|
+
Parameters
|
84
|
+
----------
|
85
|
+
key : str
|
86
|
+
Element to access
|
87
|
+
|
88
|
+
Returns
|
89
|
+
-------
|
90
|
+
Any
|
91
|
+
Element of the configuration
|
92
|
+
"""
|
93
|
+
if key == "_data":
|
94
|
+
# Called during unpickling. Special case to not run into infinite loop
|
95
|
+
# below.
|
96
|
+
raise AttributeError(key)
|
97
|
+
|
98
|
+
if key in self._data:
|
99
|
+
return self[key]
|
100
|
+
raise AttributeError(key)
|
101
|
+
|
102
|
+
def __setattr__(self, name: str, value: Any) -> None:
|
103
|
+
# Prevent configuration modification
|
104
|
+
if name == "_data":
|
105
|
+
return super().__setattr__(name, value)
|
106
|
+
raise TypeError("ConfigValue is immutable")
|
107
|
+
|
108
|
+
def __getitem__(self, key: Any) -> Any:
|
109
|
+
"""
|
110
|
+
Access an element of this configuration
|
111
|
+
|
112
|
+
Parameters
|
113
|
+
----------
|
114
|
+
key : Any
|
115
|
+
Element to access
|
116
|
+
|
117
|
+
Returns
|
118
|
+
-------
|
119
|
+
Any
|
120
|
+
Element of the configuration
|
121
|
+
"""
|
122
|
+
value = self._data[key]
|
123
|
+
if isinstance(value, dict):
|
124
|
+
value = ConfigValue(value)
|
125
|
+
return value
|
126
|
+
|
127
|
+
def __len__(self):
|
128
|
+
return len(self._data)
|
129
|
+
|
130
|
+
def __iter__(self):
|
131
|
+
return iter(self._data)
|
132
|
+
|
133
|
+
def __repr__(self):
|
134
|
+
return repr(self._data)
|
135
|
+
|
136
|
+
def __str__(self):
|
137
|
+
return json.dumps(self._data)
|
138
|
+
|
139
|
+
def to_dict(self) -> Dict[Any, Any]:
|
140
|
+
"""
|
141
|
+
Returns a dictionary representation of this configuration object.
|
142
|
+
|
143
|
+
Returns
|
144
|
+
-------
|
145
|
+
Dict[Any, Any]
|
146
|
+
Dictionary equivalent of this configuration object.
|
147
|
+
"""
|
148
|
+
return dict(self._data)
|
149
|
+
|
150
|
+
|
151
|
+
class DelayEvaluator(collections.abc.Mapping):
|
152
|
+
"""
|
153
|
+
Small wrapper that allows the evaluation of a Config() value in a delayed manner.
|
154
|
+
This is used when we want to use config.* values in decorators for example.
|
155
|
+
|
156
|
+
It also allows the following "delayed" access on an obj that is a DelayEvaluation
|
157
|
+
- obj.x.y.z (ie: accessing members of DelayEvaluator; accesses will be delayed until
|
158
|
+
the DelayEvaluator is evaluated)
|
159
|
+
- **obj (ie: unpacking the DelayEvaluator as a dictionary). Note that this requires
|
160
|
+
special handling in whatever this is being unpacked into, specifically the handling
|
161
|
+
of _unpacked_delayed_*
|
162
|
+
"""
|
163
|
+
|
164
|
+
def __init__(self, ex: str):
|
165
|
+
self._config_expr = ex
|
166
|
+
if ID_PATTERN.match(self._config_expr):
|
167
|
+
# This is a variable only so allow things like config_expr("config").var
|
168
|
+
self._is_var_only = True
|
169
|
+
self._access = []
|
170
|
+
else:
|
171
|
+
self._is_var_only = False
|
172
|
+
self._access = None
|
173
|
+
|
174
|
+
def __iter__(self):
|
175
|
+
yield "%s%d" % (UNPACK_KEY, id(self))
|
176
|
+
|
177
|
+
def __getitem__(self, key):
|
178
|
+
if key == "%s%d" % (UNPACK_KEY, id(self)):
|
179
|
+
return self
|
180
|
+
raise KeyError(key)
|
181
|
+
|
182
|
+
def __len__(self):
|
183
|
+
return 1
|
184
|
+
|
185
|
+
def __getattr__(self, name):
|
186
|
+
if self._access is None:
|
187
|
+
raise AttributeError()
|
188
|
+
self._access.append(name)
|
189
|
+
return self
|
190
|
+
|
191
|
+
def __call__(self, ctx=None, deploy_time=False):
|
192
|
+
from ..flowspec import _FlowState # Prevent circular import
|
193
|
+
|
194
|
+
# Two additional arguments are only used by DeployTimeField which will call
|
195
|
+
# this function with those two additional arguments. They are ignored.
|
196
|
+
flow_cls = getattr(current_flow, "flow_cls", None)
|
197
|
+
if flow_cls is None:
|
198
|
+
# We are not executing inside a flow (ie: not the CLI)
|
199
|
+
raise MetaflowException(
|
200
|
+
"Config object can only be used directly in the FlowSpec defining them. "
|
201
|
+
"If using outside of the FlowSpec, please use ConfigEval"
|
202
|
+
)
|
203
|
+
if self._access is not None:
|
204
|
+
# Build the final expression by adding all the fields in access as . fields
|
205
|
+
self._config_expr = ".".join([self._config_expr] + self._access)
|
206
|
+
# Evaluate the expression setting the config values as local variables
|
207
|
+
try:
|
208
|
+
return eval(
|
209
|
+
self._config_expr,
|
210
|
+
globals(),
|
211
|
+
{
|
212
|
+
k: ConfigValue(v)
|
213
|
+
for k, v in flow_cls._flow_state.get(_FlowState.CONFIGS, {}).items()
|
214
|
+
},
|
215
|
+
)
|
216
|
+
except NameError as e:
|
217
|
+
potential_config_name = self._config_expr.split(".")[0]
|
218
|
+
if potential_config_name not in flow_cls._flow_state.get(
|
219
|
+
_FlowState.CONFIGS, {}
|
220
|
+
):
|
221
|
+
raise MetaflowException(
|
222
|
+
"Config '%s' not found in the flow (maybe not required and not "
|
223
|
+
"provided?)" % potential_config_name
|
224
|
+
) from e
|
225
|
+
raise
|
226
|
+
|
227
|
+
|
228
|
+
def config_expr(expr: str) -> DelayEvaluator:
|
229
|
+
"""
|
230
|
+
Function to allow you to use an expression involving a config parameter in
|
231
|
+
places where it may not be directory accessible or if you want a more complicated
|
232
|
+
expression than just a single variable.
|
233
|
+
|
234
|
+
You can use it as follows:
|
235
|
+
- When the config is not directly accessible:
|
236
|
+
|
237
|
+
@project(name=config_expr("config").project.name)
|
238
|
+
class MyFlow(FlowSpec):
|
239
|
+
config = Config("config")
|
240
|
+
...
|
241
|
+
- When you want a more complex expression:
|
242
|
+
class MyFlow(FlowSpec):
|
243
|
+
config = Config("config")
|
244
|
+
|
245
|
+
@environment(vars={"foo": config_expr("config.bar.baz.lower()")})
|
246
|
+
@step
|
247
|
+
def start(self):
|
248
|
+
...
|
249
|
+
|
250
|
+
Parameters
|
251
|
+
----------
|
252
|
+
expr : str
|
253
|
+
Expression using the config values.
|
254
|
+
"""
|
255
|
+
return DelayEvaluator(expr)
|
256
|
+
|
257
|
+
|
258
|
+
class Config(Parameter, collections.abc.Mapping):
|
259
|
+
"""
|
260
|
+
Includes a configuration for this flow.
|
261
|
+
|
262
|
+
`Config` is a special type of `Parameter` but differs in a few key areas:
|
263
|
+
- it is immutable and determined at deploy time (or prior to running if not deploying
|
264
|
+
to a scheduler)
|
265
|
+
- as such, it can be used anywhere in your code including in Metaflow decorators
|
266
|
+
|
267
|
+
The value of the configuration is determines as follows:
|
268
|
+
- use the user-provided file path or value. It is an error to provide both
|
269
|
+
- if none are present:
|
270
|
+
- if a default file path (default) is provided, attempt to read this file
|
271
|
+
- if the file is present, use that value. Note that the file will be used
|
272
|
+
even if it has an invalid syntax
|
273
|
+
- if the file is not present, and a default value is present, use that
|
274
|
+
- if still None and is required, this is an error.
|
275
|
+
|
276
|
+
Parameters
|
277
|
+
----------
|
278
|
+
name : str
|
279
|
+
User-visible configuration name.
|
280
|
+
default : Union[str, Callable[[ParameterContext], str], optional, default None
|
281
|
+
Default path from where to read this configuration. A function implies that the
|
282
|
+
value will be computed using that function.
|
283
|
+
You can only specify default or default_value.
|
284
|
+
default_value : Union[str, Dict[str, Any], Callable[[ParameterContext, Union[str, Dict[str, Any]]], Any], optional, default None
|
285
|
+
Default value for the parameter. A function
|
286
|
+
implies that the value will be computed using that function.
|
287
|
+
You can only specify default or default_value.
|
288
|
+
help : str, optional, default None
|
289
|
+
Help text to show in `run --help`.
|
290
|
+
required : bool, optional, default None
|
291
|
+
Require that the user specified a value for the configuration. Note that if
|
292
|
+
a default is provided, the required flag is ignored. A value of None is
|
293
|
+
equivalent to False.
|
294
|
+
parser : Union[str, Callable[[str], Dict[Any, Any]]], optional, default None
|
295
|
+
If a callable, it is a function that can parse the configuration string
|
296
|
+
into an arbitrarily nested dictionary. If a string, the string should refer to
|
297
|
+
a function (like "my_parser_package.my_parser.my_parser_function") which should
|
298
|
+
be able to parse the configuration string into an arbitrarily nested dictionary.
|
299
|
+
If the name starts with a ".", it is assumed to be relative to "metaflow".
|
300
|
+
show_default : bool, default True
|
301
|
+
If True, show the default value in the help text.
|
302
|
+
"""
|
303
|
+
|
304
|
+
IS_CONFIG_PARAMETER = True
|
305
|
+
|
306
|
+
def __init__(
|
307
|
+
self,
|
308
|
+
name: str,
|
309
|
+
default: Optional[Union[str, Callable[[ParameterContext], str]]] = None,
|
310
|
+
default_value: Optional[
|
311
|
+
Union[
|
312
|
+
str,
|
313
|
+
Dict[str, Any],
|
314
|
+
Callable[[ParameterContext], Union[str, Dict[str, Any]]],
|
315
|
+
]
|
316
|
+
] = None,
|
317
|
+
help: Optional[str] = None,
|
318
|
+
required: Optional[bool] = None,
|
319
|
+
parser: Optional[Union[str, Callable[[str], Dict[Any, Any]]]] = None,
|
320
|
+
**kwargs: Dict[str, str]
|
321
|
+
):
|
322
|
+
|
323
|
+
if default and default_value:
|
324
|
+
raise MetaflowException(
|
325
|
+
"For config '%s', you can only specify default or default_value, not both"
|
326
|
+
% name
|
327
|
+
)
|
328
|
+
self._default_is_file = default is not None
|
329
|
+
kwargs["default"] = default or default_value
|
330
|
+
super(Config, self).__init__(
|
331
|
+
name, required=required, help=help, type=str, **kwargs
|
332
|
+
)
|
333
|
+
super(Config, self).init()
|
334
|
+
|
335
|
+
if isinstance(kwargs.get("default", None), str):
|
336
|
+
kwargs["default"] = json.dumps(kwargs["default"])
|
337
|
+
self.parser = parser
|
338
|
+
self._computed_value = None
|
339
|
+
|
340
|
+
def load_parameter(self, v):
|
341
|
+
return v
|
342
|
+
|
343
|
+
def _store_value(self, v: Any) -> None:
|
344
|
+
self._computed_value = v
|
345
|
+
|
346
|
+
# Support <config>.<var> syntax
|
347
|
+
def __getattr__(self, name):
|
348
|
+
return DelayEvaluator(self.name.lower()).__getattr__(name)
|
349
|
+
|
350
|
+
# Next three methods are to implement mapping to support **<config> syntax
|
351
|
+
def __iter__(self):
|
352
|
+
return iter(DelayEvaluator(self.name.lower()))
|
353
|
+
|
354
|
+
def __len__(self):
|
355
|
+
return len(DelayEvaluator(self.name.lower()))
|
356
|
+
|
357
|
+
def __getitem__(self, key):
|
358
|
+
return DelayEvaluator(self.name.lower())[key]
|
359
|
+
|
360
|
+
|
361
|
+
def resolve_delayed_evaluator(v: Any) -> Any:
|
362
|
+
if isinstance(v, DelayEvaluator):
|
363
|
+
return v()
|
364
|
+
if isinstance(v, dict):
|
365
|
+
return {
|
366
|
+
resolve_delayed_evaluator(k): resolve_delayed_evaluator(v)
|
367
|
+
for k, v in v.items()
|
368
|
+
}
|
369
|
+
if isinstance(v, list):
|
370
|
+
return [resolve_delayed_evaluator(x) for x in v]
|
371
|
+
if isinstance(v, tuple):
|
372
|
+
return tuple(resolve_delayed_evaluator(x) for x in v)
|
373
|
+
if isinstance(v, set):
|
374
|
+
return {resolve_delayed_evaluator(x) for x in v}
|
375
|
+
return v
|
376
|
+
|
377
|
+
|
378
|
+
def unpack_delayed_evaluator(to_unpack: Dict[str, Any]) -> Dict[str, Any]:
|
379
|
+
result = {}
|
380
|
+
for k, v in to_unpack.items():
|
381
|
+
if not isinstance(k, str) or not k.startswith(UNPACK_KEY):
|
382
|
+
result[k] = v
|
383
|
+
else:
|
384
|
+
# k.startswith(UNPACK_KEY)
|
385
|
+
result.update(resolve_delayed_evaluator(v[k]))
|
386
|
+
return result
|
metaflow/util.py
CHANGED
@@ -296,6 +296,9 @@ def get_metaflow_root():
|
|
296
296
|
|
297
297
|
|
298
298
|
def dict_to_cli_options(params):
|
299
|
+
# Prevent circular imports
|
300
|
+
from .user_configs.config_options import ConfigInput
|
301
|
+
|
299
302
|
for k, v in params.items():
|
300
303
|
# Omit boolean options set to false or None, but preserve options with an empty
|
301
304
|
# string argument.
|
@@ -304,6 +307,20 @@ def dict_to_cli_options(params):
|
|
304
307
|
# keyword in Python, so we call it 'decospecs' in click args
|
305
308
|
if k == "decospecs":
|
306
309
|
k = "with"
|
310
|
+
if k in ("config_file_options", "config_value_options"):
|
311
|
+
# Special handling here since we gather them all in one option but actually
|
312
|
+
# need to send them one at a time using --config-value <name> kv.<name>
|
313
|
+
# Note it can be either config_file_options or config_value_options depending
|
314
|
+
# on click processing order.
|
315
|
+
for config_name in v.keys():
|
316
|
+
yield "--config-value"
|
317
|
+
yield to_unicode(config_name)
|
318
|
+
yield to_unicode(ConfigInput.make_key_name(config_name))
|
319
|
+
continue
|
320
|
+
if k == "local_config_file":
|
321
|
+
# Skip this value -- it should only be used locally and never when
|
322
|
+
# forming another command line
|
323
|
+
continue
|
307
324
|
k = k.replace("_", "-")
|
308
325
|
v = v if isinstance(v, (list, tuple, set)) else [v]
|
309
326
|
for value in v:
|
metaflow/version.py
CHANGED
@@ -1 +1 @@
|
|
1
|
-
metaflow_version = "2.12.
|
1
|
+
metaflow_version = "2.12.37"
|
@@ -1,6 +1,6 @@
|
|
1
1
|
Metadata-Version: 2.1
|
2
2
|
Name: metaflow
|
3
|
-
Version: 2.12.
|
3
|
+
Version: 2.12.37
|
4
4
|
Summary: Metaflow: More Data Science, Less Engineering
|
5
5
|
Author: Metaflow Developers
|
6
6
|
Author-email: help@metaflow.org
|
@@ -26,7 +26,7 @@ License-File: LICENSE
|
|
26
26
|
Requires-Dist: requests
|
27
27
|
Requires-Dist: boto3
|
28
28
|
Provides-Extra: stubs
|
29
|
-
Requires-Dist: metaflow-stubs==2.12.
|
29
|
+
Requires-Dist: metaflow-stubs==2.12.37; extra == "stubs"
|
30
30
|
|
31
31
|
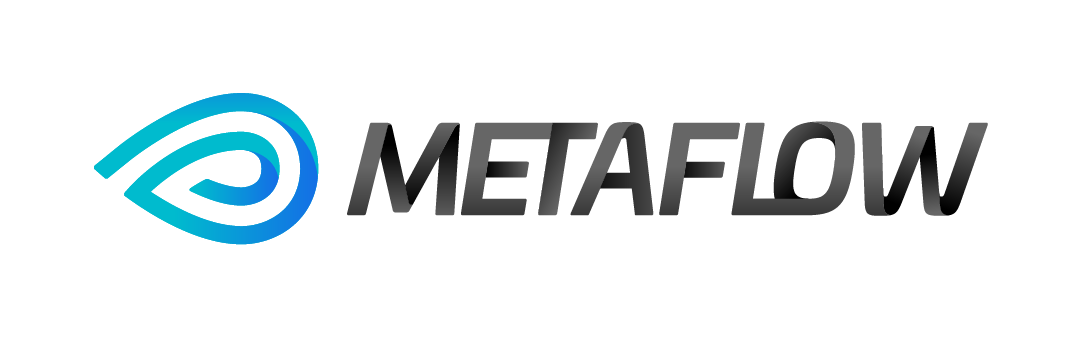
|
32
32
|
|
@@ -97,3 +97,4 @@ There are several ways to get in touch with us:
|
|
97
97
|
|
98
98
|
## Contributing
|
99
99
|
We welcome contributions to Metaflow. Please see our [contribution guide](https://docs.metaflow.org/introduction/contributing-to-metaflow) for more details.
|
100
|
+
|
@@ -1,22 +1,22 @@
|
|
1
1
|
metaflow/R.py,sha256=CqVfIatvmjciuICNnoyyNGrwE7Va9iXfLdFbQa52hwA,3958
|
2
|
-
metaflow/__init__.py,sha256=
|
2
|
+
metaflow/__init__.py,sha256=yeHIcwunlMyp_1Y6D9iuCbWi4atVMoEp2F_6-CagnDs,5819
|
3
3
|
metaflow/cards.py,sha256=tP1_RrtmqdFh741pqE4t98S7SA0MtGRlGvRICRZF1Mg,426
|
4
|
-
metaflow/cli.py,sha256=
|
5
|
-
metaflow/cli_args.py,sha256=
|
4
|
+
metaflow/cli.py,sha256=M7MRG0Zqyem1YIUa92Nqp7cO5JL2BG0EFwr-j8vzaTw,18451
|
5
|
+
metaflow/cli_args.py,sha256=nz6ZN-gv-NFTFrfHCBJRy_JtzV42iltXI4V4-sBTukE,3626
|
6
6
|
metaflow/clone_util.py,sha256=LSuVbFpPUh92UW32DBcnZbL0FFw-4w3CLa0tpEbCkzk,2066
|
7
7
|
metaflow/cmd_with_io.py,sha256=kl53HkAIyv0ecpItv08wZYczv7u3msD1VCcciqigqf0,588
|
8
8
|
metaflow/debug.py,sha256=HEmt_16tJtqHXQXsqD9pqOFe3CWR5GZ7VwpaYQgnRdU,1466
|
9
|
-
metaflow/decorators.py,sha256=
|
9
|
+
metaflow/decorators.py,sha256=IqpTOpuaNJZuPkDHGbDe9Q4AIaLlQvR_lG1jvfzA9jg,23559
|
10
10
|
metaflow/event_logger.py,sha256=joTVRqZPL87nvah4ZOwtqWX8NeraM_CXKXXGVpKGD8o,780
|
11
11
|
metaflow/events.py,sha256=ahjzkSbSnRCK9RZ-9vTfUviz_6gMvSO9DGkJ86X80-k,5300
|
12
12
|
metaflow/exception.py,sha256=KC1LHJQzzYkWib0DeQ4l_A2r8VaudywsSqIQuq1RDZU,4954
|
13
|
-
metaflow/flowspec.py,sha256=
|
13
|
+
metaflow/flowspec.py,sha256=ZJ8g5q41I0YaKp_5_pZczPT_H9coVHA3uwyVADWxgo8,33538
|
14
14
|
metaflow/graph.py,sha256=HFJ7V_bPSht_NHIm8BejrSqOX2fyBQpVOczRCliRw08,11975
|
15
|
-
metaflow/includefile.py,sha256=
|
15
|
+
metaflow/includefile.py,sha256=lMPJxxGJ-U1iqsJdUxFN5Sdcf6lb6FER39eVySJHfxg,20914
|
16
16
|
metaflow/info_file.py,sha256=wtf2_F0M6dgiUu74AFImM8lfy5RrUw5Yj7Rgs2swKRY,686
|
17
17
|
metaflow/integrations.py,sha256=LlsaoePRg03DjENnmLxZDYto3NwWc9z_PtU6nJxLldg,1480
|
18
18
|
metaflow/lint.py,sha256=5rj1MlpluxyPTSINjtMoJ7viotyNzfjtBJSAihlAwMU,10870
|
19
|
-
metaflow/metaflow_config.py,sha256=
|
19
|
+
metaflow/metaflow_config.py,sha256=f2lUcfkdUVVts9t05Wh6kzCcbQ_JUxnFAzUHjO-Cfsk,23051
|
20
20
|
metaflow/metaflow_config_funcs.py,sha256=5GlvoafV6SxykwfL8D12WXSfwjBN_NsyuKE_Q3gjGVE,6738
|
21
21
|
metaflow/metaflow_current.py,sha256=pfkXmkyHeMJhxIs6HBJNBEaBDpcl5kz9Wx5mW6F_3qo,7164
|
22
22
|
metaflow/metaflow_environment.py,sha256=rojFyGdyY56sN1HaEb1-0XX53Q3XPNnl0SaH-8xXZ8w,7987
|
@@ -24,19 +24,19 @@ metaflow/metaflow_profile.py,sha256=jKPEW-hmAQO-htSxb9hXaeloLacAh41A35rMZH6G8pA,
|
|
24
24
|
metaflow/metaflow_version.py,sha256=duhIzfKZtcxMVMs2uiBqBvUarSHJqyWDwMhaBOQd_g0,7491
|
25
25
|
metaflow/monitor.py,sha256=T0NMaBPvXynlJAO_avKtk8OIIRMyEuMAyF8bIp79aZU,5323
|
26
26
|
metaflow/multicore_utils.py,sha256=yEo5T6Gemn4_vl8b6IOz7fsTUYtEyqa3AaKZgJY96Wc,4974
|
27
|
-
metaflow/package.py,sha256=
|
28
|
-
metaflow/parameters.py,sha256=
|
27
|
+
metaflow/package.py,sha256=yfwVMVB1mD-Sw94KwXNK3N-26YHoKMn6btrcgd67Izs,7845
|
28
|
+
metaflow/parameters.py,sha256=cftYDVz8j7gQ9cdtRPx9VVhPNwKakHEecDrlagos7x4,18238
|
29
29
|
metaflow/procpoll.py,sha256=U2tE4iK_Mwj2WDyVTx_Uglh6xZ-jixQOo4wrM9OOhxg,2859
|
30
30
|
metaflow/py.typed,sha256=47DEQpj8HBSa-_TImW-5JCeuQeRkm5NMpJWZG3hSuFU,0
|
31
31
|
metaflow/pylint_wrapper.py,sha256=zzBY9YaSUZOGH-ypDKAv2B_7XcoyMZj-zCoCrmYqNRc,2865
|
32
|
-
metaflow/runtime.py,sha256=
|
32
|
+
metaflow/runtime.py,sha256=ISkkrjK4WXHaWywDEO_Po71ECLDCW4T0pqMFvgYkVrM,73857
|
33
33
|
metaflow/tagging_util.py,sha256=ctyf0Q1gBi0RyZX6J0e9DQGNkNHblV_CITfy66axXB4,2346
|
34
34
|
metaflow/task.py,sha256=xVVLWy8NH16OlLu2VoOb1OfiFzcOVVCdQldlmb1Zb_w,29691
|
35
35
|
metaflow/tuple_util.py,sha256=_G5YIEhuugwJ_f6rrZoelMFak3DqAR2tt_5CapS1XTY,830
|
36
36
|
metaflow/unbounded_foreach.py,sha256=p184WMbrMJ3xKYHwewj27ZhRUsSj_kw1jlye5gA9xJk,387
|
37
|
-
metaflow/util.py,sha256=
|
37
|
+
metaflow/util.py,sha256=wA25u2oXP-mHuDJM8Yx9gnPGZa9dP5tP1RWD9EULPow,14698
|
38
38
|
metaflow/vendor.py,sha256=FchtA9tH22JM-eEtJ2c9FpUdMn8sSb1VHuQS56EcdZk,5139
|
39
|
-
metaflow/version.py,sha256=
|
39
|
+
metaflow/version.py,sha256=rbXl9FIHxAycvo-PZLNF2WHWcZy_9dxHui5CINEj4NM,29
|
40
40
|
metaflow/_vendor/__init__.py,sha256=y_CiwUD3l4eAKvTVDZeqgVujMy31cAM1qjAB-HfI-9s,353
|
41
41
|
metaflow/_vendor/typing_extensions.py,sha256=0nUs5p1A_UrZigrAVBoOEM6TxU37zzPDUtiij1ZwpNc,110417
|
42
42
|
metaflow/_vendor/zipp.py,sha256=ajztOH-9I7KA_4wqDYygtHa6xUBVZgFpmZ8FE74HHHI,8425
|
@@ -110,6 +110,12 @@ metaflow/_vendor/v3_6/importlib_metadata/_itertools.py,sha256=cvr_2v8BRbxcIl5x5l
|
|
110
110
|
metaflow/_vendor/v3_6/importlib_metadata/_meta.py,sha256=_F48Hu_jFxkfKWz5wcYS8vO23qEygbVdF9r-6qh-hjE,1154
|
111
111
|
metaflow/_vendor/v3_6/importlib_metadata/_text.py,sha256=HCsFksZpJLeTP3NEk_ngrAeXVRRtTrtyh9eOABoRP4A,2166
|
112
112
|
metaflow/_vendor/v3_6/importlib_metadata/py.typed,sha256=47DEQpj8HBSa-_TImW-5JCeuQeRkm5NMpJWZG3hSuFU,0
|
113
|
+
metaflow/cli_components/__init__.py,sha256=47DEQpj8HBSa-_TImW-5JCeuQeRkm5NMpJWZG3hSuFU,0
|
114
|
+
metaflow/cli_components/dump_cmd.py,sha256=SZEX51BWNd1o3H2uHDkYA8KRvou5X8g5rTwpdu5vnNQ,2704
|
115
|
+
metaflow/cli_components/init_cmd.py,sha256=Er-BO59UEUV1HIsg81bRtZWT2D2IZNMp93l-AoZLCls,1519
|
116
|
+
metaflow/cli_components/run_cmds.py,sha256=vnjkvVt_-VwK22eRQ6arFQ4zxD9WQXmX2WZkusKrUwU,10374
|
117
|
+
metaflow/cli_components/step_cmd.py,sha256=ZnUTHTf8Gh2d5LcgMSo_-pAd7FFNEDighrp1tmzAZqo,5079
|
118
|
+
metaflow/cli_components/utils.py,sha256=gpoDociadjnJD7MuiJup_MDR02ZJjjleejr0jPBu29c,6057
|
113
119
|
metaflow/client/__init__.py,sha256=1GtQB4Y_CBkzaxg32L1syNQSlfj762wmLrfrDxGi1b8,226
|
114
120
|
metaflow/client/core.py,sha256=Ka6G12h6pS_046cej0c8npvaBGpiKyvJ1bGbRFZL6Kg,75437
|
115
121
|
metaflow/client/filecache.py,sha256=Wy0yhhCqC1JZgebqi7z52GCwXYnkAqMZHTtxThvwBgM,15229
|
@@ -119,7 +125,7 @@ metaflow/cmd/main_cli.py,sha256=E546zT_jYQKysmjwfpEgzZd5QMsyirs28M2s0OPU93E,2966
|
|
119
125
|
metaflow/cmd/tutorials_cmd.py,sha256=8FdlKkicTOhCIDKcBR5b0Oz6giDvS-EMY3o9skIrRqw,5156
|
120
126
|
metaflow/cmd/util.py,sha256=jS_0rUjOnGGzPT65fzRLdGjrYAOOLA4jU2S0HJLV0oc,406
|
121
127
|
metaflow/cmd/develop/__init__.py,sha256=p1Sy8yU1MEKSrH5ttOWOZvNcI1qYu6J6jghdTHwPgOw,689
|
122
|
-
metaflow/cmd/develop/stub_generator.py,sha256=
|
128
|
+
metaflow/cmd/develop/stub_generator.py,sha256=7xm36_sli0nlOwnOD4pPCIbqunaj1yrC_BS4YAD4zj0,64992
|
123
129
|
metaflow/cmd/develop/stubs.py,sha256=JX2qNZDvG0upvPueAcLhoR_zyLtRranZMwY05tLdpRQ,11884
|
124
130
|
metaflow/datastore/__init__.py,sha256=VxP6ddJt3rwiCkpiSfAhyVkUCOe1pgZZsytVEJzFmSQ,155
|
125
131
|
metaflow/datastore/content_addressed_store.py,sha256=6T7tNqL29kpmecyMLHF35RhoSBOb-OZcExnsB65AvnI,7641
|
@@ -133,7 +139,7 @@ metaflow/extension_support/__init__.py,sha256=2z0c4R8zsVmEFOMGT2Jujsl6xveDVa9KLl
|
|
133
139
|
metaflow/extension_support/_empty_file.py,sha256=HENjnM4uAfeNygxMB_feCCWORFoSat9n_QwzSx2oXPw,109
|
134
140
|
metaflow/extension_support/cmd.py,sha256=hk8iBUUINqvKCDxInKgWpum8ThiRZtHSJP7qBASHzl8,5711
|
135
141
|
metaflow/extension_support/integrations.py,sha256=AWAh-AZ-vo9IxuAVEjGw3s8p_NMm2DKHYx10oC51gPU,5506
|
136
|
-
metaflow/extension_support/plugins.py,sha256
|
142
|
+
metaflow/extension_support/plugins.py,sha256=4JbcXOWosSBqXlj1VINI5rSJGSnKx2_u3lN4KQxH-hs,11227
|
137
143
|
metaflow/metadata_provider/__init__.py,sha256=FZNSnz26VB_m18DQG8mup6-Gfl7r1U6lRMljJBp3VAM,64
|
138
144
|
metaflow/metadata_provider/heartbeat.py,sha256=9XJs4SV4R4taxUSMOKmRChnJCQ5a5o0dzRZ1iICWr9Y,2423
|
139
145
|
metaflow/metadata_provider/metadata.py,sha256=4tmySlgQaoV-p9bHig7BjsEsqFRZWEOuwSLpODuKxjA,26169
|
@@ -143,7 +149,7 @@ metaflow/mflog/mflog.py,sha256=VebXxqitOtNAs7VJixnNfziO_i_urG7bsJ5JiB5IXgY,4370
|
|
143
149
|
metaflow/mflog/save_logs.py,sha256=ZBAF4BMukw4FMAC7odpr9OI2BC_2petPtDX0ca6srC4,2352
|
144
150
|
metaflow/mflog/save_logs_periodically.py,sha256=2Uvk9hi-zlCqXxOQoXmmjH1SCugfw6eG6w70WgfI-ho,1256
|
145
151
|
metaflow/mflog/tee.py,sha256=wTER15qeHuiRpCkOqo-bd-r3Gj-EVlf3IvWRCA4beW4,887
|
146
|
-
metaflow/plugins/__init__.py,sha256=
|
152
|
+
metaflow/plugins/__init__.py,sha256=BWwrnLi0MX0LtgmtATGau_FFNKbmJ-BgCJcy-MYsmoQ,7600
|
147
153
|
metaflow/plugins/catch_decorator.py,sha256=UOM2taN_OL2RPpuJhwEOA9ZALm0-hHD0XS2Hn2GUev0,4061
|
148
154
|
metaflow/plugins/debug_logger.py,sha256=mcF5HYzJ0NQmqCMjyVUk3iAP-heroHRIiVWQC6Ha2-I,879
|
149
155
|
metaflow/plugins/debug_monitor.py,sha256=Md5X_sDOSssN9pt2D8YcaIjTK5JaQD55UAYTcF6xYF0,1099
|
@@ -158,10 +164,10 @@ metaflow/plugins/retry_decorator.py,sha256=tz_2Tq6GLg3vjDBZp0KKVTk3ADlCvqaWTSf7b
|
|
158
164
|
metaflow/plugins/storage_executor.py,sha256=FqAgR0-L9MuqN8fRtTe4jjUfJL9lqt6fQkYaglAjRbk,6137
|
159
165
|
metaflow/plugins/tag_cli.py,sha256=10039-0DUF0cmhudoDNrRGLWq8tCGQJ7tBsQAGAmkBQ,17549
|
160
166
|
metaflow/plugins/test_unbounded_foreach_decorator.py,sha256=33p5aCWnyk9MT5DmXcm4Q_Qnwfd4y4xvVTEfeqs4by0,5957
|
161
|
-
metaflow/plugins/timeout_decorator.py,sha256=
|
167
|
+
metaflow/plugins/timeout_decorator.py,sha256=ZOUmg5HIm_9kteMC7qbzj2tTVBESwRfp1hPJd8MJMBg,3586
|
162
168
|
metaflow/plugins/airflow/__init__.py,sha256=47DEQpj8HBSa-_TImW-5JCeuQeRkm5NMpJWZG3hSuFU,0
|
163
169
|
metaflow/plugins/airflow/airflow.py,sha256=GDaKLdzzySttJfhl_OiYjkK_ubIaRKR4YgcLaKRCQLk,32293
|
164
|
-
metaflow/plugins/airflow/airflow_cli.py,sha256=
|
170
|
+
metaflow/plugins/airflow/airflow_cli.py,sha256=6qoei1o4Eslf9EsOgBRIfl1TsX4YI8O56Siza37oGiw,14745
|
165
171
|
metaflow/plugins/airflow/airflow_decorator.py,sha256=IWT6M9gga8t65FR4Wi7pIZvOupk3hE75B5NRg9tMEps,1781
|
166
172
|
metaflow/plugins/airflow/airflow_utils.py,sha256=dvRllfQeOWfDUseFnOocIGaL3gRI_A7cEHnC1w01vfk,28905
|
167
173
|
metaflow/plugins/airflow/dag.py,sha256=zYV3QsyqGIOxgipbiEb4dX-r6aippNbXjuT6Jt2s4xI,129
|
@@ -175,8 +181,8 @@ metaflow/plugins/airflow/sensors/s3_sensor.py,sha256=iDReG-7FKnumrtQg-HY6cCUAAqN
|
|
175
181
|
metaflow/plugins/argo/__init__.py,sha256=47DEQpj8HBSa-_TImW-5JCeuQeRkm5NMpJWZG3hSuFU,0
|
176
182
|
metaflow/plugins/argo/argo_client.py,sha256=Z_A1TO9yw4Y-a8VAlwrFS0BwunWzXpbtik-j_xjcuHE,16303
|
177
183
|
metaflow/plugins/argo/argo_events.py,sha256=_C1KWztVqgi3zuH57pInaE9OzABc2NnncC-zdwOMZ-w,5909
|
178
|
-
metaflow/plugins/argo/argo_workflows.py,sha256=
|
179
|
-
metaflow/plugins/argo/argo_workflows_cli.py,sha256=
|
184
|
+
metaflow/plugins/argo/argo_workflows.py,sha256=lPPPKlHgz2cKYHM8mdq8Aywoi6vYytyCzxd5PrsWGFE,175733
|
185
|
+
metaflow/plugins/argo/argo_workflows_cli.py,sha256=PcvFDn_VOW2D6_fb19hhSzC_CNnXP1CINES5cugB6BU,36774
|
180
186
|
metaflow/plugins/argo/argo_workflows_decorator.py,sha256=QdM1rK9gM-lDhyZldK8WqvFqJDvfJ7i3JPR5Uzaq2as,7887
|
181
187
|
metaflow/plugins/argo/argo_workflows_deployer.py,sha256=6kHxEnYXJwzNCM9swI8-0AckxtPWqwhZLerYkX8fxUM,4444
|
182
188
|
metaflow/plugins/argo/argo_workflows_deployer_objects.py,sha256=GJ1Jsm5KHYaBbJjKRz82Cwhi_PN1XnMiSmL1C0LNLYQ,12318
|
@@ -190,7 +196,7 @@ metaflow/plugins/aws/batch/__init__.py,sha256=47DEQpj8HBSa-_TImW-5JCeuQeRkm5NMpJ
|
|
190
196
|
metaflow/plugins/aws/batch/batch.py,sha256=e9ssahWM18GnipPK2sqYB-ztx9w7Eoo7YtWyEtufYxs,17787
|
191
197
|
metaflow/plugins/aws/batch/batch_cli.py,sha256=gVQMWBLeuqO3U3PhVJSHLwa-CNHsmW0Cvmv-K0C-DoA,11758
|
192
198
|
metaflow/plugins/aws/batch/batch_client.py,sha256=ddlGG0Vk1mkO7tcvJjDvNAVsVLOlqddF7MA1kKfHSqM,28830
|
193
|
-
metaflow/plugins/aws/batch/batch_decorator.py,sha256=
|
199
|
+
metaflow/plugins/aws/batch/batch_decorator.py,sha256=1MAhri-A_CYN2op0LPZikWL30m5h0w05XA8PXqb-Gk8,17561
|
194
200
|
metaflow/plugins/aws/secrets_manager/__init__.py,sha256=47DEQpj8HBSa-_TImW-5JCeuQeRkm5NMpJWZG3hSuFU,0
|
195
201
|
metaflow/plugins/aws/secrets_manager/aws_secrets_manager_secrets_provider.py,sha256=JtFUVu00Cg0FzAizgrPLXmrMqsT7YeQMkQlgeivUxcE,7986
|
196
202
|
metaflow/plugins/aws/step_functions/__init__.py,sha256=47DEQpj8HBSa-_TImW-5JCeuQeRkm5NMpJWZG3hSuFU,0
|
@@ -199,8 +205,8 @@ metaflow/plugins/aws/step_functions/event_bridge_client.py,sha256=U9-tqKdih4KR-Z
|
|
199
205
|
metaflow/plugins/aws/step_functions/production_token.py,sha256=_o4emv3rozYZoWpaj1Y6UfKhTMlYpQc7GDDDBfZ2G7s,1898
|
200
206
|
metaflow/plugins/aws/step_functions/schedule_decorator.py,sha256=Ab1rW8O_no4HNZm4__iBmFDCDW0Z8-TgK4lnxHHA6HI,1940
|
201
207
|
metaflow/plugins/aws/step_functions/set_batch_environment.py,sha256=ibiGWFHDjKcLfprH3OsX-g2M9lUsh6J-bp7v2cdLhD4,1294
|
202
|
-
metaflow/plugins/aws/step_functions/step_functions.py,sha256=
|
203
|
-
metaflow/plugins/aws/step_functions/step_functions_cli.py,sha256=
|
208
|
+
metaflow/plugins/aws/step_functions/step_functions.py,sha256=ZQ1qKLieQ99lWm6RI0zagEW8eOKKxGBiGwnBgFJS1DQ,53146
|
209
|
+
metaflow/plugins/aws/step_functions/step_functions_cli.py,sha256=vxXhbOu9NibYXyrrT--Pkp1DF8YMfq0vqeyfahOncTI,26129
|
204
210
|
metaflow/plugins/aws/step_functions/step_functions_client.py,sha256=DKpNwAIWElvWjFANs5Ku3rgzjxFoqAD6k-EF8Xhkg3Q,4754
|
205
211
|
metaflow/plugins/aws/step_functions/step_functions_decorator.py,sha256=LoZC5BuQLqyFtfE-sGla26l2xXlCKN9aSvIlzPKV134,3800
|
206
212
|
metaflow/plugins/aws/step_functions/step_functions_deployer.py,sha256=JKYtDhKivtXUWPklprZFzkqezh14loGDmk8mNk6QtpI,3714
|
@@ -248,7 +254,7 @@ metaflow/plugins/datatools/__init__.py,sha256=ge4L16OBQLy2J_MMvoHg3lMfdm-MluQgRW
|
|
248
254
|
metaflow/plugins/datatools/local.py,sha256=FJvMOBcjdyhSPHmdLocBSiIT0rmKkKBmsaclxH75x08,4233
|
249
255
|
metaflow/plugins/datatools/s3/__init__.py,sha256=14tr9fPjN3ULW5IOfKHeG7Uhjmgm7LMtQHfz1SFv-h8,248
|
250
256
|
metaflow/plugins/datatools/s3/s3.py,sha256=dSUEf3v_BVyvYXlehWy04xcBtNDhKKR5Gnn7oXwagaw,67037
|
251
|
-
metaflow/plugins/datatools/s3/s3op.py,sha256=
|
257
|
+
metaflow/plugins/datatools/s3/s3op.py,sha256=P7c7s3GgAw8gVdLR3hOy1w4Z8TjsrNCMhs0stcn9JXU,43454
|
252
258
|
metaflow/plugins/datatools/s3/s3tail.py,sha256=boQjQGQMI-bvTqcMP2y7uSlSYLcvWOy7J3ZUaF78NAA,2597
|
253
259
|
metaflow/plugins/datatools/s3/s3util.py,sha256=FgRgaVmEq7-i2dV7q8XK5w5PfFt-xJjZa8WrK8IJfdI,3769
|
254
260
|
metaflow/plugins/env_escape/__init__.py,sha256=tGNUZnmPvk52eNs__VK443b3CZ7ogEFTT-s9_n_HF8Q,8837
|
@@ -283,9 +289,9 @@ metaflow/plugins/gcp/includefile_support.py,sha256=OQO0IVWv4ObboL0VqEZwcDOyj9ORL
|
|
283
289
|
metaflow/plugins/kubernetes/__init__.py,sha256=47DEQpj8HBSa-_TImW-5JCeuQeRkm5NMpJWZG3hSuFU,0
|
284
290
|
metaflow/plugins/kubernetes/kube_utils.py,sha256=CbJRMn-sQyGqG-hKMBBjA6xmw15_DyQmhU8TxNyWqcQ,2124
|
285
291
|
metaflow/plugins/kubernetes/kubernetes.py,sha256=FrIL2wRUzy4bJr6pNz3I-tNFH-OJWHJcrarJsBKRPLE,31728
|
286
|
-
metaflow/plugins/kubernetes/kubernetes_cli.py,sha256=
|
292
|
+
metaflow/plugins/kubernetes/kubernetes_cli.py,sha256=LbkhQRw6Wi262QHD_iH1NAk8uIiXT6jUBQIQpqvB2w4,13633
|
287
293
|
metaflow/plugins/kubernetes/kubernetes_client.py,sha256=tuvXP-QKpdeSmzVolB2R_TaacOr5DIb0j642eKcjsiM,6491
|
288
|
-
metaflow/plugins/kubernetes/kubernetes_decorator.py,sha256=
|
294
|
+
metaflow/plugins/kubernetes/kubernetes_decorator.py,sha256=wuPsh7ENZGngGFkYc2gKwX3aLGrhT8zQk-m-Xn9lrLo,28488
|
289
295
|
metaflow/plugins/kubernetes/kubernetes_job.py,sha256=CoDzG0eEcJezfMTmgYJ4Ea9G_o5INYm0w1DvjGwJT2A,31916
|
290
296
|
metaflow/plugins/kubernetes/kubernetes_jobsets.py,sha256=0SGOfStlh6orXVpF3s6Mu26OFR36eh4dj-sFYwg3HaA,42066
|
291
297
|
metaflow/plugins/metadata_providers/__init__.py,sha256=AbpHGcgLb-kRsJGnwFEktk7uzpZOCcBY74-YBdrKVGs,1
|
@@ -293,18 +299,18 @@ metaflow/plugins/metadata_providers/local.py,sha256=9UAxe9caN6kU1lkSlIoJbRGgTqsM
|
|
293
299
|
metaflow/plugins/metadata_providers/service.py,sha256=NKZfFMamx6upP6aFRJfXlfYIhySgFNzz6kbp1yPD7LA,20222
|
294
300
|
metaflow/plugins/pypi/__init__.py,sha256=0YFZpXvX7HCkyBFglatual7XGifdA1RwC3U4kcizyak,1037
|
295
301
|
metaflow/plugins/pypi/bootstrap.py,sha256=FI-itExqIz7DUzLnnkGwoB60rFBviygpIFThUtqk_4E,5227
|
296
|
-
metaflow/plugins/pypi/conda_decorator.py,sha256=
|
302
|
+
metaflow/plugins/pypi/conda_decorator.py,sha256=XSdY2jDMzJdEL-P0YYJYx4-IinMjB40aqDk8QNbkbNk,16388
|
297
303
|
metaflow/plugins/pypi/conda_environment.py,sha256=IGHIphHm1e8UEJX-PvyTesfKRCpxtJIc1pxJ5Wen-aU,19765
|
298
304
|
metaflow/plugins/pypi/micromamba.py,sha256=QaZYMy5w4esW2w_Lb9kZdWU07EtZD_Ky00MVlA4FJw0,14079
|
299
305
|
metaflow/plugins/pypi/pip.py,sha256=Uewmt6-meLyPhNLiAOAkDdfd1P4Go07bkQUD0uE5VIs,13827
|
300
|
-
metaflow/plugins/pypi/pypi_decorator.py,sha256=
|
306
|
+
metaflow/plugins/pypi/pypi_decorator.py,sha256=iTY3dU_W3SqI6XFtI9mQVAmmnL3l5avxkio6feW1gm8,7282
|
301
307
|
metaflow/plugins/pypi/pypi_environment.py,sha256=FYMg8kF3lXqcLfRYWD83a9zpVjcoo_TARqMGZ763rRk,230
|
302
308
|
metaflow/plugins/pypi/utils.py,sha256=ds1Mnv_DaxGnLAYp7ozg_K6oyguGyNhvHfE-75Ia1YA,2836
|
303
309
|
metaflow/plugins/secrets/__init__.py,sha256=mhJaN2eMS_ZZVewAMR2E-JdP5i0t3v9e6Dcwd-WpruE,310
|
304
310
|
metaflow/plugins/secrets/inline_secrets_provider.py,sha256=EChmoBGA1i7qM3jtYwPpLZDBybXLergiDlN63E0u3x8,294
|
305
311
|
metaflow/plugins/secrets/secrets_decorator.py,sha256=s-sFzPWOjahhpr5fMj-ZEaHkDYAPTO0isYXGvaUwlG8,11273
|
306
312
|
metaflow/runner/__init__.py,sha256=47DEQpj8HBSa-_TImW-5JCeuQeRkm5NMpJWZG3hSuFU,0
|
307
|
-
metaflow/runner/click_api.py,sha256=
|
313
|
+
metaflow/runner/click_api.py,sha256=BicwlQRGrLhy2xOYo89IV9pu5L8ZkGGTUI2FTsrq33I,15944
|
308
314
|
metaflow/runner/deployer.py,sha256=goCCDq9mRJIrH5-9HU6E6J55pYoju--CtM1zADTzHvY,8967
|
309
315
|
metaflow/runner/deployer_impl.py,sha256=QjMoTM1IviRK-dZ7WB3vGExpaScOCESlHxSR9ZoAkgA,5625
|
310
316
|
metaflow/runner/metaflow_runner.py,sha256=PrAEJhWqjbGuu4IsnxCSLLf8o4AqqRpeTM7rCMbOAvg,14827
|
@@ -316,7 +322,7 @@ metaflow/sidecar/__init__.py,sha256=1mmNpmQ5puZCpRmmYlCOeieZ4108Su9XQ4_EqF1FGOU,
|
|
316
322
|
metaflow/sidecar/sidecar.py,sha256=EspKXvPPNiyRToaUZ51PS5TT_PzrBNAurn_wbFnmGr0,1334
|
317
323
|
metaflow/sidecar/sidecar_messages.py,sha256=zPsCoYgDIcDkkvdC9MEpJTJ3y6TSGm2JWkRc4vxjbFA,1071
|
318
324
|
metaflow/sidecar/sidecar_subprocess.py,sha256=f72n5iJJAYfCIbz4D94-RxR37VvM7kVvE3c8E9dYHe8,9708
|
319
|
-
metaflow/sidecar/sidecar_worker.py,sha256=
|
325
|
+
metaflow/sidecar/sidecar_worker.py,sha256=J50QoeCBP9mk2romVU8mpI-Aq4QVPJc9tEAtWv5GTT4,2050
|
320
326
|
metaflow/system/__init__.py,sha256=SB9Py7Acecqi76MY9MonSHXFuDD1yIJEGJtEQH8cNq4,149
|
321
327
|
metaflow/system/system_logger.py,sha256=NwL40-cHnUud58WRW3v7hke5IWIpwDdTnM8USiu-uio,2726
|
322
328
|
metaflow/system/system_monitor.py,sha256=6h3EAisD762NzL7fMuc7RQGygnhp0qqD97kDHrTacU8,2939
|
@@ -348,9 +354,13 @@ metaflow/tutorials/07-worldview/README.md,sha256=5vQTrFqulJ7rWN6r20dhot9lI2sVj9W
|
|
348
354
|
metaflow/tutorials/07-worldview/worldview.ipynb,sha256=ztPZPI9BXxvW1QdS2Tfe7LBuVzvFvv0AToDnsDJhLdE,2237
|
349
355
|
metaflow/tutorials/08-autopilot/README.md,sha256=GnePFp_q76jPs991lMUqfIIh5zSorIeWznyiUxzeUVE,1039
|
350
356
|
metaflow/tutorials/08-autopilot/autopilot.ipynb,sha256=DQoJlILV7Mq9vfPBGW-QV_kNhWPjS5n6SJLqePjFYLY,3191
|
351
|
-
metaflow
|
352
|
-
metaflow
|
353
|
-
metaflow
|
354
|
-
metaflow
|
355
|
-
metaflow-2.12.
|
356
|
-
metaflow-2.12.
|
357
|
+
metaflow/user_configs/__init__.py,sha256=47DEQpj8HBSa-_TImW-5JCeuQeRkm5NMpJWZG3hSuFU,0
|
358
|
+
metaflow/user_configs/config_decorators.py,sha256=Tj0H88UT8Q6pylXxHXgiA6cqnNlw4d3mR7M8J9g3ZUg,20139
|
359
|
+
metaflow/user_configs/config_options.py,sha256=3l293IQHOE-DqNzDt7kc1vLWMLuevoJceYVQ43dEhQY,19165
|
360
|
+
metaflow/user_configs/config_parameters.py,sha256=cBlnhLoax-QbpD46oofuLUJhT3_8WHscLNDSpO8hWOU,13098
|
361
|
+
metaflow-2.12.37.dist-info/LICENSE,sha256=nl_Lt5v9VvJ-5lWJDT4ddKAG-VZ-2IaLmbzpgYDz2hU,11343
|
362
|
+
metaflow-2.12.37.dist-info/METADATA,sha256=dlMDdlS3IEuX5HrIvEWl8e-b4EdFL2G9zgMv4aDE7pE,5908
|
363
|
+
metaflow-2.12.37.dist-info/WHEEL,sha256=pxeNX5JdtCe58PUSYP9upmc7jdRPgvT0Gm9kb1SHlVw,109
|
364
|
+
metaflow-2.12.37.dist-info/entry_points.txt,sha256=IKwTN1T3I5eJL3uo_vnkyxVffcgnRdFbKwlghZfn27k,57
|
365
|
+
metaflow-2.12.37.dist-info/top_level.txt,sha256=v1pDHoWaSaKeuc5fKTRSfsXCKSdW1zvNVmvA-i0if3o,9
|
366
|
+
metaflow-2.12.37.dist-info/RECORD,,
|
File without changes
|
File without changes
|
File without changes
|