metaflow 2.12.32__py2.py3-none-any.whl → 2.12.33__py2.py3-none-any.whl
This diff represents the content of publicly available package versions that have been released to one of the supported registries. The information contained in this diff is provided for informational purposes only and reflects changes between package versions as they appear in their respective public registries.
- metaflow/flowspec.py +1 -0
- metaflow/parameters.py +2 -8
- metaflow/plugins/argo/argo_workflows.py +4 -8
- metaflow/plugins/events_decorator.py +72 -253
- metaflow/version.py +1 -1
- {metaflow-2.12.32.dist-info → metaflow-2.12.33.dist-info}/METADATA +2 -2
- {metaflow-2.12.32.dist-info → metaflow-2.12.33.dist-info}/RECORD +11 -11
- {metaflow-2.12.32.dist-info → metaflow-2.12.33.dist-info}/LICENSE +0 -0
- {metaflow-2.12.32.dist-info → metaflow-2.12.33.dist-info}/WHEEL +0 -0
- {metaflow-2.12.32.dist-info → metaflow-2.12.33.dist-info}/entry_points.txt +0 -0
- {metaflow-2.12.32.dist-info → metaflow-2.12.33.dist-info}/top_level.txt +0 -0
metaflow/flowspec.py
CHANGED
metaflow/parameters.py
CHANGED
@@ -151,7 +151,6 @@ class DeployTimeField(object):
|
|
151
151
|
return self._check_type(val, deploy_time)
|
152
152
|
|
153
153
|
def _check_type(self, val, deploy_time):
|
154
|
-
|
155
154
|
# it is easy to introduce a deploy-time function that accidentally
|
156
155
|
# returns a value whose type is not compatible with what is defined
|
157
156
|
# in Parameter. Let's catch those mistakes early here, instead of
|
@@ -159,7 +158,7 @@ class DeployTimeField(object):
|
|
159
158
|
|
160
159
|
# note: this doesn't work with long in Python2 or types defined as
|
161
160
|
# click types, e.g. click.INT
|
162
|
-
TYPES = {bool: "bool", int: "int", float: "float", list: "list"
|
161
|
+
TYPES = {bool: "bool", int: "int", float: "float", list: "list"}
|
163
162
|
|
164
163
|
msg = (
|
165
164
|
"The value returned by the deploy-time function for "
|
@@ -167,12 +166,7 @@ class DeployTimeField(object):
|
|
167
166
|
% (self.parameter_name, self.field)
|
168
167
|
)
|
169
168
|
|
170
|
-
if
|
171
|
-
if not any(isinstance(val, x) for x in self.parameter_type):
|
172
|
-
msg += "Expected one of the following %s." % TYPES[self.parameter_type]
|
173
|
-
raise ParameterFieldTypeMismatch(msg)
|
174
|
-
return str(val) if self.return_str else val
|
175
|
-
elif self.parameter_type in TYPES:
|
169
|
+
if self.parameter_type in TYPES:
|
176
170
|
if type(val) != self.parameter_type:
|
177
171
|
msg += "Expected a %s." % TYPES[self.parameter_type]
|
178
172
|
raise ParameterFieldTypeMismatch(msg)
|
@@ -522,9 +522,7 @@ class ArgoWorkflows(object):
|
|
522
522
|
params = set(
|
523
523
|
[param.name.lower() for var, param in self.flow._get_parameters()]
|
524
524
|
)
|
525
|
-
|
526
|
-
trigger_deco.format_deploytime_value()
|
527
|
-
for event in trigger_deco.triggers:
|
525
|
+
for event in self.flow._flow_decorators.get("trigger")[0].triggers:
|
528
526
|
parameters = {}
|
529
527
|
# TODO: Add a check to guard against names starting with numerals(?)
|
530
528
|
if not re.match(r"^[A-Za-z0-9_.-]+$", event["name"]):
|
@@ -564,11 +562,9 @@ class ArgoWorkflows(object):
|
|
564
562
|
|
565
563
|
# @trigger_on_finish decorator
|
566
564
|
if self.flow._flow_decorators.get("trigger_on_finish"):
|
567
|
-
|
568
|
-
|
569
|
-
|
570
|
-
trigger_on_finish_deco.format_deploytime_value()
|
571
|
-
for event in trigger_on_finish_deco.triggers:
|
565
|
+
for event in self.flow._flow_decorators.get("trigger_on_finish")[
|
566
|
+
0
|
567
|
+
].triggers:
|
572
568
|
# Actual filters are deduced here since we don't have access to
|
573
569
|
# the current object in the @trigger_on_finish decorator.
|
574
570
|
triggers.append(
|
@@ -1,11 +1,9 @@
|
|
1
1
|
import re
|
2
|
-
import json
|
3
2
|
|
4
3
|
from metaflow import current
|
5
4
|
from metaflow.decorators import FlowDecorator
|
6
5
|
from metaflow.exception import MetaflowException
|
7
6
|
from metaflow.util import is_stringish
|
8
|
-
from metaflow.parameters import DeployTimeField, deploy_time_eval
|
9
7
|
|
10
8
|
# TODO: Support dynamic parameter mapping through a context object that exposes
|
11
9
|
# flow name and user name similar to parameter context
|
@@ -70,75 +68,6 @@ class TriggerDecorator(FlowDecorator):
|
|
70
68
|
"options": {},
|
71
69
|
}
|
72
70
|
|
73
|
-
def process_event_name(self, event):
|
74
|
-
if is_stringish(event):
|
75
|
-
return {"name": str(event)}
|
76
|
-
elif isinstance(event, dict):
|
77
|
-
if "name" not in event:
|
78
|
-
raise MetaflowException(
|
79
|
-
"The *event* attribute for *@trigger* is missing the *name* key."
|
80
|
-
)
|
81
|
-
if callable(event["name"]) and not isinstance(
|
82
|
-
event["name"], DeployTimeField
|
83
|
-
):
|
84
|
-
event["name"] = DeployTimeField(
|
85
|
-
"event_name", str, None, event["name"], False
|
86
|
-
)
|
87
|
-
event["parameters"] = self.process_parameters(event.get("parameters", {}))
|
88
|
-
return event
|
89
|
-
elif callable(event) and not isinstance(event, DeployTimeField):
|
90
|
-
return DeployTimeField("event", [str, dict], None, event, False)
|
91
|
-
else:
|
92
|
-
raise MetaflowException(
|
93
|
-
"Incorrect format for *event* attribute in *@trigger* decorator. "
|
94
|
-
"Supported formats are string and dictionary - \n"
|
95
|
-
"@trigger(event='foo') or @trigger(event={'name': 'foo', "
|
96
|
-
"'parameters': {'alpha': 'beta'}})"
|
97
|
-
)
|
98
|
-
|
99
|
-
def process_parameters(self, parameters):
|
100
|
-
new_param_values = {}
|
101
|
-
if isinstance(parameters, (list, tuple)):
|
102
|
-
for mapping in parameters:
|
103
|
-
if is_stringish(mapping):
|
104
|
-
new_param_values[mapping] = mapping
|
105
|
-
elif callable(mapping) and not isinstance(mapping, DeployTimeField):
|
106
|
-
mapping = DeployTimeField(
|
107
|
-
"parameter_val", str, None, mapping, False
|
108
|
-
)
|
109
|
-
new_param_values[mapping] = mapping
|
110
|
-
elif isinstance(mapping, (list, tuple)) and len(mapping) == 2:
|
111
|
-
if callable(mapping[0]) and not isinstance(
|
112
|
-
mapping[0], DeployTimeField
|
113
|
-
):
|
114
|
-
mapping[0] = DeployTimeField(
|
115
|
-
"parameter_val", str, None, mapping[0], False
|
116
|
-
)
|
117
|
-
if callable(mapping[1]) and not isinstance(
|
118
|
-
mapping[1], DeployTimeField
|
119
|
-
):
|
120
|
-
mapping[1] = DeployTimeField(
|
121
|
-
"parameter_val", str, None, mapping[1], False
|
122
|
-
)
|
123
|
-
new_param_values[mapping[0]] = mapping[1]
|
124
|
-
else:
|
125
|
-
raise MetaflowException(
|
126
|
-
"The *parameters* attribute for event is invalid. "
|
127
|
-
"It should be a list/tuple of strings and lists/tuples of size 2"
|
128
|
-
)
|
129
|
-
elif callable(parameters) and not isinstance(parameters, DeployTimeField):
|
130
|
-
return DeployTimeField(
|
131
|
-
"parameters", [list, dict, tuple], None, parameters, False
|
132
|
-
)
|
133
|
-
elif isinstance(parameters, dict):
|
134
|
-
for key, value in parameters.items():
|
135
|
-
if callable(key) and not isinstance(key, DeployTimeField):
|
136
|
-
key = DeployTimeField("flow_parameter", str, None, key, False)
|
137
|
-
if callable(value) and not isinstance(value, DeployTimeField):
|
138
|
-
value = DeployTimeField("signal_parameter", str, None, value, False)
|
139
|
-
new_param_values[key] = value
|
140
|
-
return new_param_values
|
141
|
-
|
142
71
|
def flow_init(
|
143
72
|
self,
|
144
73
|
flow_name,
|
@@ -157,9 +86,41 @@ class TriggerDecorator(FlowDecorator):
|
|
157
86
|
"attributes in *@trigger* decorator."
|
158
87
|
)
|
159
88
|
elif self.attributes["event"]:
|
160
|
-
event
|
161
|
-
|
162
|
-
|
89
|
+
# event attribute supports the following formats -
|
90
|
+
# 1. event='table.prod_db.members'
|
91
|
+
# 2. event={'name': 'table.prod_db.members',
|
92
|
+
# 'parameters': {'alpha': 'member_weight'}}
|
93
|
+
if is_stringish(self.attributes["event"]):
|
94
|
+
self.triggers.append({"name": str(self.attributes["event"])})
|
95
|
+
elif isinstance(self.attributes["event"], dict):
|
96
|
+
if "name" not in self.attributes["event"]:
|
97
|
+
raise MetaflowException(
|
98
|
+
"The *event* attribute for *@trigger* is missing the "
|
99
|
+
"*name* key."
|
100
|
+
)
|
101
|
+
param_value = self.attributes["event"].get("parameters", {})
|
102
|
+
if isinstance(param_value, (list, tuple)):
|
103
|
+
new_param_value = {}
|
104
|
+
for mapping in param_value:
|
105
|
+
if is_stringish(mapping):
|
106
|
+
new_param_value[mapping] = mapping
|
107
|
+
elif isinstance(mapping, (list, tuple)) and len(mapping) == 2:
|
108
|
+
new_param_value[mapping[0]] = mapping[1]
|
109
|
+
else:
|
110
|
+
raise MetaflowException(
|
111
|
+
"The *parameters* attribute for event '%s' is invalid. "
|
112
|
+
"It should be a list/tuple of strings and lists/tuples "
|
113
|
+
"of size 2" % self.attributes["event"]["name"]
|
114
|
+
)
|
115
|
+
self.attributes["event"]["parameters"] = new_param_value
|
116
|
+
self.triggers.append(self.attributes["event"])
|
117
|
+
else:
|
118
|
+
raise MetaflowException(
|
119
|
+
"Incorrect format for *event* attribute in *@trigger* decorator. "
|
120
|
+
"Supported formats are string and dictionary - \n"
|
121
|
+
"@trigger(event='foo') or @trigger(event={'name': 'foo', "
|
122
|
+
"'parameters': {'alpha': 'beta'}})"
|
123
|
+
)
|
163
124
|
elif self.attributes["events"]:
|
164
125
|
# events attribute supports the following formats -
|
165
126
|
# 1. events=[{'name': 'table.prod_db.members',
|
@@ -167,17 +128,43 @@ class TriggerDecorator(FlowDecorator):
|
|
167
128
|
# {'name': 'table.prod_db.metadata',
|
168
129
|
# 'parameters': {'beta': 'grade'}}]
|
169
130
|
if isinstance(self.attributes["events"], list):
|
170
|
-
# process every event in events
|
171
131
|
for event in self.attributes["events"]:
|
172
|
-
|
173
|
-
|
174
|
-
|
175
|
-
|
176
|
-
|
177
|
-
|
178
|
-
|
179
|
-
|
180
|
-
|
132
|
+
if is_stringish(event):
|
133
|
+
self.triggers.append({"name": str(event)})
|
134
|
+
elif isinstance(event, dict):
|
135
|
+
if "name" not in event:
|
136
|
+
raise MetaflowException(
|
137
|
+
"One or more events in *events* attribute for "
|
138
|
+
"*@trigger* are missing the *name* key."
|
139
|
+
)
|
140
|
+
param_value = event.get("parameters", {})
|
141
|
+
if isinstance(param_value, (list, tuple)):
|
142
|
+
new_param_value = {}
|
143
|
+
for mapping in param_value:
|
144
|
+
if is_stringish(mapping):
|
145
|
+
new_param_value[mapping] = mapping
|
146
|
+
elif (
|
147
|
+
isinstance(mapping, (list, tuple))
|
148
|
+
and len(mapping) == 2
|
149
|
+
):
|
150
|
+
new_param_value[mapping[0]] = mapping[1]
|
151
|
+
else:
|
152
|
+
raise MetaflowException(
|
153
|
+
"The *parameters* attribute for event '%s' is "
|
154
|
+
"invalid. It should be a list/tuple of strings "
|
155
|
+
"and lists/tuples of size 2" % event["name"]
|
156
|
+
)
|
157
|
+
event["parameters"] = new_param_value
|
158
|
+
self.triggers.append(event)
|
159
|
+
else:
|
160
|
+
raise MetaflowException(
|
161
|
+
"One or more events in *events* attribute in *@trigger* "
|
162
|
+
"decorator have an incorrect format. Supported format "
|
163
|
+
"is dictionary - \n"
|
164
|
+
"@trigger(events=[{'name': 'foo', 'parameters': {'alpha': "
|
165
|
+
"'beta'}}, {'name': 'bar', 'parameters': "
|
166
|
+
"{'gamma': 'kappa'}}])"
|
167
|
+
)
|
181
168
|
else:
|
182
169
|
raise MetaflowException(
|
183
170
|
"Incorrect format for *events* attribute in *@trigger* decorator. "
|
@@ -191,12 +178,7 @@ class TriggerDecorator(FlowDecorator):
|
|
191
178
|
raise MetaflowException("No event(s) specified in *@trigger* decorator.")
|
192
179
|
|
193
180
|
# same event shouldn't occur more than once
|
194
|
-
names = [
|
195
|
-
x["name"]
|
196
|
-
for x in self.triggers
|
197
|
-
if not isinstance(x, DeployTimeField)
|
198
|
-
and not isinstance(x["name"], DeployTimeField)
|
199
|
-
]
|
181
|
+
names = [x["name"] for x in self.triggers]
|
200
182
|
if len(names) != len(set(names)):
|
201
183
|
raise MetaflowException(
|
202
184
|
"Duplicate event names defined in *@trigger* decorator."
|
@@ -206,104 +188,6 @@ class TriggerDecorator(FlowDecorator):
|
|
206
188
|
|
207
189
|
# TODO: Handle scenario for local testing using --trigger.
|
208
190
|
|
209
|
-
def format_deploytime_value(self):
|
210
|
-
new_triggers = []
|
211
|
-
for trigger in self.triggers:
|
212
|
-
# Case where trigger is a function that returns a list of events
|
213
|
-
# Need to do this bc we need to iterate over list later
|
214
|
-
if isinstance(trigger, DeployTimeField):
|
215
|
-
evaluated_trigger = deploy_time_eval(trigger)
|
216
|
-
if isinstance(evaluated_trigger, dict):
|
217
|
-
trigger = evaluated_trigger
|
218
|
-
elif isinstance(evaluated_trigger, str):
|
219
|
-
trigger = {"name": evaluated_trigger}
|
220
|
-
if isinstance(evaluated_trigger, list):
|
221
|
-
for trig in evaluated_trigger:
|
222
|
-
if is_stringish(trig):
|
223
|
-
new_triggers.append({"name": trig})
|
224
|
-
else: # dict or another deploytimefield
|
225
|
-
new_triggers.append(trig)
|
226
|
-
else:
|
227
|
-
new_triggers.append(trigger)
|
228
|
-
else:
|
229
|
-
new_triggers.append(trigger)
|
230
|
-
|
231
|
-
self.triggers = new_triggers
|
232
|
-
for trigger in self.triggers:
|
233
|
-
old_trigger = trigger
|
234
|
-
trigger_params = trigger.get("parameters", {})
|
235
|
-
# Case where param is a function (can return list or dict)
|
236
|
-
if isinstance(trigger_params, DeployTimeField):
|
237
|
-
trigger_params = deploy_time_eval(trigger_params)
|
238
|
-
# If params is a list of strings, convert to dict with same key and value
|
239
|
-
if isinstance(trigger_params, (list, tuple)):
|
240
|
-
new_trigger_params = {}
|
241
|
-
for mapping in trigger_params:
|
242
|
-
if is_stringish(mapping) or callable(mapping):
|
243
|
-
new_trigger_params[mapping] = mapping
|
244
|
-
elif callable(mapping) and not isinstance(mapping, DeployTimeField):
|
245
|
-
mapping = DeployTimeField(
|
246
|
-
"parameter_val", str, None, mapping, False
|
247
|
-
)
|
248
|
-
new_trigger_params[mapping] = mapping
|
249
|
-
elif isinstance(mapping, (list, tuple)) and len(mapping) == 2:
|
250
|
-
if callable(mapping[0]) and not isinstance(
|
251
|
-
mapping[0], DeployTimeField
|
252
|
-
):
|
253
|
-
mapping[0] = DeployTimeField(
|
254
|
-
"parameter_val",
|
255
|
-
str,
|
256
|
-
None,
|
257
|
-
mapping[1],
|
258
|
-
False,
|
259
|
-
)
|
260
|
-
if callable(mapping[1]) and not isinstance(
|
261
|
-
mapping[1], DeployTimeField
|
262
|
-
):
|
263
|
-
mapping[1] = DeployTimeField(
|
264
|
-
"parameter_val",
|
265
|
-
str,
|
266
|
-
None,
|
267
|
-
mapping[1],
|
268
|
-
False,
|
269
|
-
)
|
270
|
-
|
271
|
-
new_trigger_params[mapping[0]] = mapping[1]
|
272
|
-
else:
|
273
|
-
raise MetaflowException(
|
274
|
-
"The *parameters* attribute for event '%s' is invalid. "
|
275
|
-
"It should be a list/tuple of strings and lists/tuples "
|
276
|
-
"of size 2" % self.attributes["event"]["name"]
|
277
|
-
)
|
278
|
-
trigger_params = new_trigger_params
|
279
|
-
trigger["parameters"] = trigger_params
|
280
|
-
|
281
|
-
trigger_name = trigger.get("name")
|
282
|
-
# Case where just the name is a function (always a str)
|
283
|
-
if isinstance(trigger_name, DeployTimeField):
|
284
|
-
trigger_name = deploy_time_eval(trigger_name)
|
285
|
-
trigger["name"] = trigger_name
|
286
|
-
|
287
|
-
# Third layer
|
288
|
-
# {name:, parameters:[func, ..., ...]}
|
289
|
-
# {name:, parameters:{func : func2}}
|
290
|
-
for trigger in self.triggers:
|
291
|
-
old_trigger = trigger
|
292
|
-
trigger_params = trigger.get("parameters", {})
|
293
|
-
new_trigger_params = {}
|
294
|
-
for key, value in trigger_params.items():
|
295
|
-
if isinstance(value, DeployTimeField) and key is value:
|
296
|
-
evaluated_param = deploy_time_eval(value)
|
297
|
-
new_trigger_params[evaluated_param] = evaluated_param
|
298
|
-
elif isinstance(value, DeployTimeField):
|
299
|
-
new_trigger_params[key] = deploy_time_eval(value)
|
300
|
-
elif isinstance(key, DeployTimeField):
|
301
|
-
new_trigger_params[deploy_time_eval(key)] = value
|
302
|
-
else:
|
303
|
-
new_trigger_params[key] = value
|
304
|
-
trigger["parameters"] = new_trigger_params
|
305
|
-
self.triggers[self.triggers.index(old_trigger)] = trigger
|
306
|
-
|
307
191
|
|
308
192
|
class TriggerOnFinishDecorator(FlowDecorator):
|
309
193
|
"""
|
@@ -428,13 +312,6 @@ class TriggerOnFinishDecorator(FlowDecorator):
|
|
428
312
|
"The *project_branch* attribute of the *flow* is not a string"
|
429
313
|
)
|
430
314
|
self.triggers.append(result)
|
431
|
-
elif callable(self.attributes["flow"]) and not isinstance(
|
432
|
-
self.attributes["flow"], DeployTimeField
|
433
|
-
):
|
434
|
-
trig = DeployTimeField(
|
435
|
-
"fq_name", [str, dict], None, self.attributes["flow"], False
|
436
|
-
)
|
437
|
-
self.triggers.append(trig)
|
438
315
|
else:
|
439
316
|
raise MetaflowException(
|
440
317
|
"Incorrect type for *flow* attribute in *@trigger_on_finish* "
|
@@ -492,13 +369,6 @@ class TriggerOnFinishDecorator(FlowDecorator):
|
|
492
369
|
"Supported type is string or Dict[str, str]- \n"
|
493
370
|
"@trigger_on_finish(flows=['FooFlow', 'BarFlow']"
|
494
371
|
)
|
495
|
-
elif callable(self.attributes["flows"]) and not isinstance(
|
496
|
-
self.attributes["flows"], DeployTimeField
|
497
|
-
):
|
498
|
-
trig = DeployTimeField(
|
499
|
-
"flows", list, None, self.attributes["flows"], False
|
500
|
-
)
|
501
|
-
self.triggers.append(trig)
|
502
372
|
else:
|
503
373
|
raise MetaflowException(
|
504
374
|
"Incorrect type for *flows* attribute in *@trigger_on_finish* "
|
@@ -513,8 +383,6 @@ class TriggerOnFinishDecorator(FlowDecorator):
|
|
513
383
|
|
514
384
|
# Make triggers @project aware
|
515
385
|
for trigger in self.triggers:
|
516
|
-
if isinstance(trigger, DeployTimeField):
|
517
|
-
continue
|
518
386
|
if trigger["fq_name"].count(".") == 0:
|
519
387
|
# fully qualified name is just the flow name
|
520
388
|
trigger["flow"] = trigger["fq_name"]
|
@@ -559,54 +427,5 @@ class TriggerOnFinishDecorator(FlowDecorator):
|
|
559
427
|
run_objs.append(run_obj)
|
560
428
|
current._update_env({"trigger": Trigger.from_runs(run_objs)})
|
561
429
|
|
562
|
-
def _parse_fq_name(self, trigger):
|
563
|
-
if isinstance(trigger, DeployTimeField):
|
564
|
-
trigger["fq_name"] = deploy_time_eval(trigger["fq_name"])
|
565
|
-
if trigger["fq_name"].count(".") == 0:
|
566
|
-
# fully qualified name is just the flow name
|
567
|
-
trigger["flow"] = trigger["fq_name"]
|
568
|
-
elif trigger["fq_name"].count(".") >= 2:
|
569
|
-
# fully qualified name is of the format - project.branch.flow_name
|
570
|
-
trigger["project"], tail = trigger["fq_name"].split(".", maxsplit=1)
|
571
|
-
trigger["branch"], trigger["flow"] = tail.rsplit(".", maxsplit=1)
|
572
|
-
else:
|
573
|
-
raise MetaflowException(
|
574
|
-
"Incorrect format for *flow* in *@trigger_on_finish* "
|
575
|
-
"decorator. Specify either just the *flow_name* or a fully "
|
576
|
-
"qualified name like *project_name.branch_name.flow_name*."
|
577
|
-
)
|
578
|
-
if not re.match(r"^[A-Za-z0-9_]+$", trigger["flow"]):
|
579
|
-
raise MetaflowException(
|
580
|
-
"Invalid flow name *%s* in *@trigger_on_finish* "
|
581
|
-
"decorator. Only alphanumeric characters and "
|
582
|
-
"underscores(_) are allowed." % trigger["flow"]
|
583
|
-
)
|
584
|
-
return trigger
|
585
|
-
|
586
|
-
def format_deploytime_value(self):
|
587
|
-
for trigger in self.triggers:
|
588
|
-
# Case were trigger is a function that returns a list
|
589
|
-
# Need to do this bc we need to iterate over list and process
|
590
|
-
if isinstance(trigger, DeployTimeField):
|
591
|
-
deploy_value = deploy_time_eval(trigger)
|
592
|
-
if isinstance(deploy_value, list):
|
593
|
-
self.triggers = deploy_value
|
594
|
-
else:
|
595
|
-
break
|
596
|
-
for trigger in self.triggers:
|
597
|
-
# Entire trigger is a function (returns either string or dict)
|
598
|
-
old_trig = trigger
|
599
|
-
if isinstance(trigger, DeployTimeField):
|
600
|
-
trigger = deploy_time_eval(trigger)
|
601
|
-
if isinstance(trigger, dict):
|
602
|
-
trigger["fq_name"] = trigger.get("name")
|
603
|
-
trigger["project"] = trigger.get("project")
|
604
|
-
trigger["branch"] = trigger.get("project_branch")
|
605
|
-
# We also added this bc it won't be formatted yet
|
606
|
-
if isinstance(trigger, str):
|
607
|
-
trigger = {"fq_name": trigger}
|
608
|
-
trigger = self._parse_fq_name(trigger)
|
609
|
-
self.triggers[self.triggers.index(old_trig)] = trigger
|
610
|
-
|
611
430
|
def get_top_level_options(self):
|
612
431
|
return list(self._option_values.items())
|
metaflow/version.py
CHANGED
@@ -1 +1 @@
|
|
1
|
-
metaflow_version = "2.12.
|
1
|
+
metaflow_version = "2.12.33"
|
@@ -1,6 +1,6 @@
|
|
1
1
|
Metadata-Version: 2.1
|
2
2
|
Name: metaflow
|
3
|
-
Version: 2.12.
|
3
|
+
Version: 2.12.33
|
4
4
|
Summary: Metaflow: More Data Science, Less Engineering
|
5
5
|
Author: Metaflow Developers
|
6
6
|
Author-email: help@metaflow.org
|
@@ -26,7 +26,7 @@ License-File: LICENSE
|
|
26
26
|
Requires-Dist: requests
|
27
27
|
Requires-Dist: boto3
|
28
28
|
Provides-Extra: stubs
|
29
|
-
Requires-Dist: metaflow-stubs==2.12.
|
29
|
+
Requires-Dist: metaflow-stubs==2.12.33; extra == "stubs"
|
30
30
|
|
31
31
|
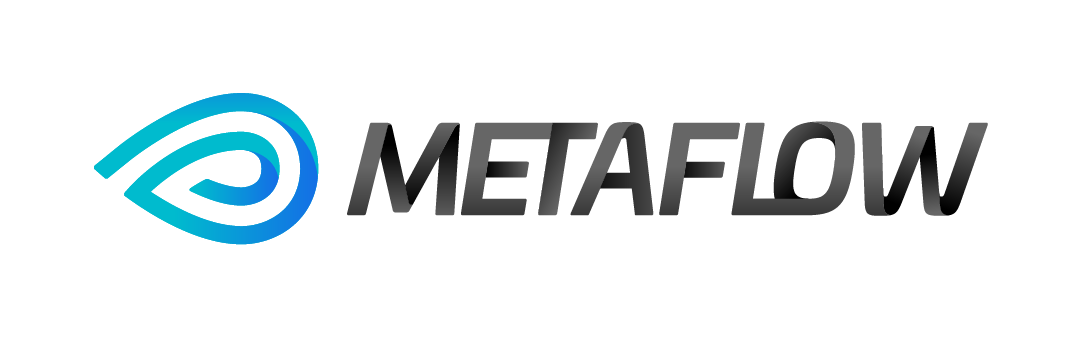
|
32
32
|
|
@@ -10,7 +10,7 @@ metaflow/decorators.py,sha256=hbJwRlZuLbl6t7fOsTiH7Sux4Lx6zgEEpldIXoM5TQc,21540
|
|
10
10
|
metaflow/event_logger.py,sha256=joTVRqZPL87nvah4ZOwtqWX8NeraM_CXKXXGVpKGD8o,780
|
11
11
|
metaflow/events.py,sha256=ahjzkSbSnRCK9RZ-9vTfUviz_6gMvSO9DGkJ86X80-k,5300
|
12
12
|
metaflow/exception.py,sha256=KC1LHJQzzYkWib0DeQ4l_A2r8VaudywsSqIQuq1RDZU,4954
|
13
|
-
metaflow/flowspec.py,sha256=
|
13
|
+
metaflow/flowspec.py,sha256=VEeaItArFwCJqRNq0e2i9gZrBSZAaq0XwmN_8dTcY1M,27298
|
14
14
|
metaflow/graph.py,sha256=HFJ7V_bPSht_NHIm8BejrSqOX2fyBQpVOczRCliRw08,11975
|
15
15
|
metaflow/includefile.py,sha256=rDJnxF0U7vD3cz9hhPkKlW_KS3ToaXnlOjhjNZ__Rx4,19628
|
16
16
|
metaflow/info_file.py,sha256=wtf2_F0M6dgiUu74AFImM8lfy5RrUw5Yj7Rgs2swKRY,686
|
@@ -25,7 +25,7 @@ metaflow/metaflow_version.py,sha256=duhIzfKZtcxMVMs2uiBqBvUarSHJqyWDwMhaBOQd_g0,
|
|
25
25
|
metaflow/monitor.py,sha256=T0NMaBPvXynlJAO_avKtk8OIIRMyEuMAyF8bIp79aZU,5323
|
26
26
|
metaflow/multicore_utils.py,sha256=vdTNgczVLODifscUbbveJbuSDOl3Y9pAxhr7sqYiNf4,4760
|
27
27
|
metaflow/package.py,sha256=QutDP6WzjwGk1UCKXqBfXa9F10Q--FlRr0J7fwlple0,7399
|
28
|
-
metaflow/parameters.py,sha256=
|
28
|
+
metaflow/parameters.py,sha256=U5G8CGbUurLmrj-YOlt18komEZ2eyFI_F1ymkAKYI-Y,15741
|
29
29
|
metaflow/procpoll.py,sha256=U2tE4iK_Mwj2WDyVTx_Uglh6xZ-jixQOo4wrM9OOhxg,2859
|
30
30
|
metaflow/py.typed,sha256=47DEQpj8HBSa-_TImW-5JCeuQeRkm5NMpJWZG3hSuFU,0
|
31
31
|
metaflow/pylint_wrapper.py,sha256=zzBY9YaSUZOGH-ypDKAv2B_7XcoyMZj-zCoCrmYqNRc,2865
|
@@ -36,7 +36,7 @@ metaflow/tuple_util.py,sha256=_G5YIEhuugwJ_f6rrZoelMFak3DqAR2tt_5CapS1XTY,830
|
|
36
36
|
metaflow/unbounded_foreach.py,sha256=p184WMbrMJ3xKYHwewj27ZhRUsSj_kw1jlye5gA9xJk,387
|
37
37
|
metaflow/util.py,sha256=olAvJK3y1it_k99MhLulTaAJo7OFVt5rnrD-ulIFLCU,13616
|
38
38
|
metaflow/vendor.py,sha256=FchtA9tH22JM-eEtJ2c9FpUdMn8sSb1VHuQS56EcdZk,5139
|
39
|
-
metaflow/version.py,sha256=
|
39
|
+
metaflow/version.py,sha256=MpOKzSbObVlxAXuFrnuHcHrkrHsL2fg2pDPIFhLPjVY,29
|
40
40
|
metaflow/_vendor/__init__.py,sha256=y_CiwUD3l4eAKvTVDZeqgVujMy31cAM1qjAB-HfI-9s,353
|
41
41
|
metaflow/_vendor/typing_extensions.py,sha256=0nUs5p1A_UrZigrAVBoOEM6TxU37zzPDUtiij1ZwpNc,110417
|
42
42
|
metaflow/_vendor/zipp.py,sha256=ajztOH-9I7KA_4wqDYygtHa6xUBVZgFpmZ8FE74HHHI,8425
|
@@ -148,7 +148,7 @@ metaflow/plugins/catch_decorator.py,sha256=UOM2taN_OL2RPpuJhwEOA9ZALm0-hHD0XS2Hn
|
|
148
148
|
metaflow/plugins/debug_logger.py,sha256=mcF5HYzJ0NQmqCMjyVUk3iAP-heroHRIiVWQC6Ha2-I,879
|
149
149
|
metaflow/plugins/debug_monitor.py,sha256=Md5X_sDOSssN9pt2D8YcaIjTK5JaQD55UAYTcF6xYF0,1099
|
150
150
|
metaflow/plugins/environment_decorator.py,sha256=6m9j2B77d-Ja_l_9CTJ__0O6aB2a8Qt_lAZu6UjAcUA,587
|
151
|
-
metaflow/plugins/events_decorator.py,sha256=
|
151
|
+
metaflow/plugins/events_decorator.py,sha256=c2GcH6Mspbey3wBkjM5lqxaNByFOzYDQdllLpXzRNv8,18283
|
152
152
|
metaflow/plugins/logs_cli.py,sha256=77W5UNagU2mOKSMMvrQxQmBLRzvmjK-c8dWxd-Ygbqs,11410
|
153
153
|
metaflow/plugins/package_cli.py,sha256=-J6D4cupHfWSZ4GEFo2yy9Je9oL3owRWm5pEJwaiqd4,1649
|
154
154
|
metaflow/plugins/parallel_decorator.py,sha256=GIjZZVTqkvtnMuGE8RNtObX6CAJavZTxttqRujGmnGs,8973
|
@@ -175,7 +175,7 @@ metaflow/plugins/airflow/sensors/s3_sensor.py,sha256=iDReG-7FKnumrtQg-HY6cCUAAqN
|
|
175
175
|
metaflow/plugins/argo/__init__.py,sha256=47DEQpj8HBSa-_TImW-5JCeuQeRkm5NMpJWZG3hSuFU,0
|
176
176
|
metaflow/plugins/argo/argo_client.py,sha256=Z_A1TO9yw4Y-a8VAlwrFS0BwunWzXpbtik-j_xjcuHE,16303
|
177
177
|
metaflow/plugins/argo/argo_events.py,sha256=_C1KWztVqgi3zuH57pInaE9OzABc2NnncC-zdwOMZ-w,5909
|
178
|
-
metaflow/plugins/argo/argo_workflows.py,sha256=
|
178
|
+
metaflow/plugins/argo/argo_workflows.py,sha256=9ygK_yp5YMCmcNo3TGwi562pRKXJtCw0OyRvKl-B9JQ,173669
|
179
179
|
metaflow/plugins/argo/argo_workflows_cli.py,sha256=NdLwzfBcTsR72qLycZBesR4Pwv48o3Z_v6OfYrZuVEY,36721
|
180
180
|
metaflow/plugins/argo/argo_workflows_decorator.py,sha256=QdM1rK9gM-lDhyZldK8WqvFqJDvfJ7i3JPR5Uzaq2as,7887
|
181
181
|
metaflow/plugins/argo/argo_workflows_deployer.py,sha256=6kHxEnYXJwzNCM9swI8-0AckxtPWqwhZLerYkX8fxUM,4444
|
@@ -348,9 +348,9 @@ metaflow/tutorials/07-worldview/README.md,sha256=5vQTrFqulJ7rWN6r20dhot9lI2sVj9W
|
|
348
348
|
metaflow/tutorials/07-worldview/worldview.ipynb,sha256=ztPZPI9BXxvW1QdS2Tfe7LBuVzvFvv0AToDnsDJhLdE,2237
|
349
349
|
metaflow/tutorials/08-autopilot/README.md,sha256=GnePFp_q76jPs991lMUqfIIh5zSorIeWznyiUxzeUVE,1039
|
350
350
|
metaflow/tutorials/08-autopilot/autopilot.ipynb,sha256=DQoJlILV7Mq9vfPBGW-QV_kNhWPjS5n6SJLqePjFYLY,3191
|
351
|
-
metaflow-2.12.
|
352
|
-
metaflow-2.12.
|
353
|
-
metaflow-2.12.
|
354
|
-
metaflow-2.12.
|
355
|
-
metaflow-2.12.
|
356
|
-
metaflow-2.12.
|
351
|
+
metaflow-2.12.33.dist-info/LICENSE,sha256=nl_Lt5v9VvJ-5lWJDT4ddKAG-VZ-2IaLmbzpgYDz2hU,11343
|
352
|
+
metaflow-2.12.33.dist-info/METADATA,sha256=AsBEmE49lfnJ8Ebyk7ReCICOnawskpwvjmXHy9GEP3M,5907
|
353
|
+
metaflow-2.12.33.dist-info/WHEEL,sha256=pxeNX5JdtCe58PUSYP9upmc7jdRPgvT0Gm9kb1SHlVw,109
|
354
|
+
metaflow-2.12.33.dist-info/entry_points.txt,sha256=IKwTN1T3I5eJL3uo_vnkyxVffcgnRdFbKwlghZfn27k,57
|
355
|
+
metaflow-2.12.33.dist-info/top_level.txt,sha256=v1pDHoWaSaKeuc5fKTRSfsXCKSdW1zvNVmvA-i0if3o,9
|
356
|
+
metaflow-2.12.33.dist-info/RECORD,,
|
File without changes
|
File without changes
|
File without changes
|
File without changes
|