datomic-flare 1.0.0
Sign up to get free protection for your applications and to get access to all the features.
- checksums.yaml +7 -0
- data/.env.example +4 -0
- data/.gitignore +3 -0
- data/.rspec +1 -0
- data/.rubocop.yml +15 -0
- data/.ruby-version +1 -0
- data/Gemfile +19 -0
- data/Gemfile.lock +100 -0
- data/LICENSE +9 -0
- data/README.md +1042 -0
- data/components/errors.rb +22 -0
- data/components/http.rb +55 -0
- data/controllers/api.rb +48 -0
- data/controllers/client.rb +43 -0
- data/controllers/documentation/formatter.rb +37 -0
- data/controllers/documentation/generator.rb +390 -0
- data/controllers/dsl/querying.rb +39 -0
- data/controllers/dsl/schema.rb +37 -0
- data/controllers/dsl/transacting.rb +62 -0
- data/controllers/dsl.rb +48 -0
- data/datomic-flare.gemspec +39 -0
- data/docs/CURL.md +781 -0
- data/docs/README.md +360 -0
- data/docs/api.md +395 -0
- data/docs/dsl.md +257 -0
- data/docs/templates/.rubocop.yml +15 -0
- data/docs/templates/README.md +319 -0
- data/docs/templates/api.md +267 -0
- data/docs/templates/dsl.md +206 -0
- data/helpers/h.rb +17 -0
- data/logic/dangerous_override.rb +108 -0
- data/logic/querying.rb +34 -0
- data/logic/schema.rb +91 -0
- data/logic/transacting.rb +53 -0
- data/logic/types.rb +141 -0
- data/ports/cli.rb +26 -0
- data/ports/dsl/datomic-flare/errors.rb +5 -0
- data/ports/dsl/datomic-flare.rb +20 -0
- data/static/gem.rb +15 -0
- metadata +146 -0
data/docs/README.md
ADDED
@@ -0,0 +1,360 @@
|
|
1
|
+
# Datomic Flare for Ruby
|
2
|
+
|
3
|
+
A Ruby gem for interacting with [Datomic](https://www.datomic.com) through [Datomic Flare](https://github.com/gbaptista/datomic-flare).
|
4
|
+
|
5
|
+
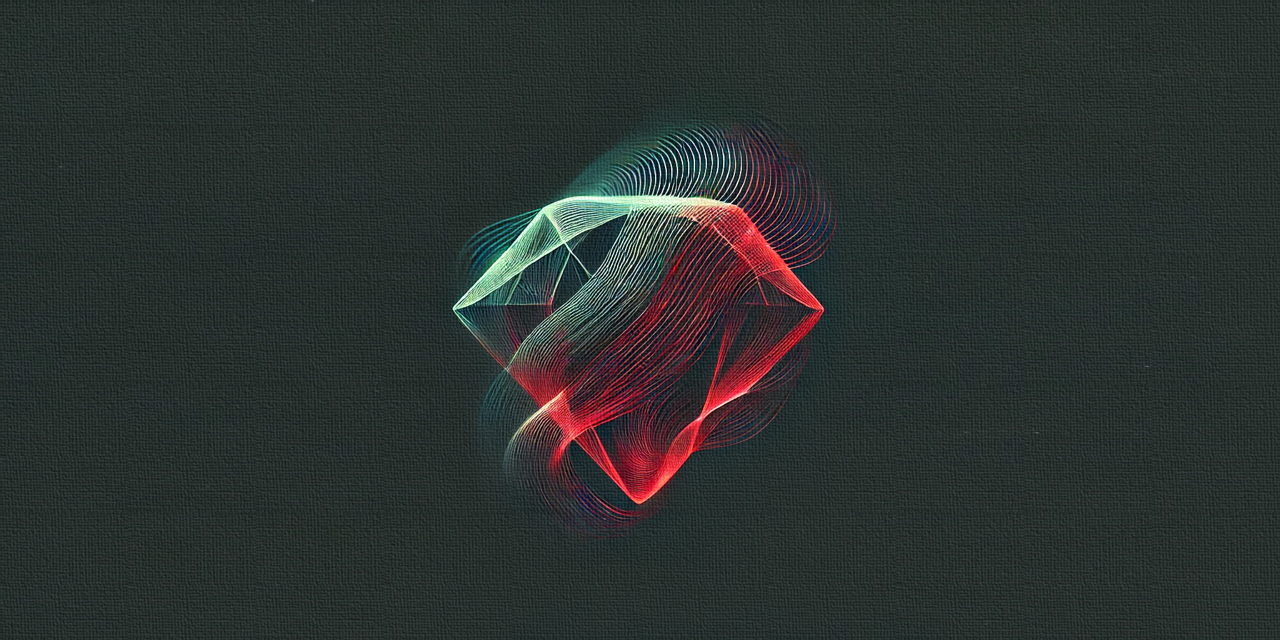
|
6
|
+
|
7
|
+
_This is not an official Datomic project or documentation and it is not affiliated with Datomic in any way._
|
8
|
+
|
9
|
+
## TL;DR and Quick Start
|
10
|
+
|
11
|
+
```ruby
|
12
|
+
gem '{{ gem.name }}', '~> {{ gem.version }}'
|
13
|
+
```
|
14
|
+
|
15
|
+
```ruby
|
16
|
+
require '{{ gem.name }}'
|
17
|
+
|
18
|
+
client = Flare.new(credentials: { address: 'http://localhost:3042' })
|
19
|
+
```
|
20
|
+
|
21
|
+
```ruby
|
22
|
+
client.dsl.transact_schema!(
|
23
|
+
{
|
24
|
+
book: {
|
25
|
+
title: { type: :string, doc: 'The title of the book.' },
|
26
|
+
genre: { type: :string, doc: 'The genre of the book.' }
|
27
|
+
}
|
28
|
+
}
|
29
|
+
)
|
30
|
+
|
31
|
+
client.dsl.assert_into!(
|
32
|
+
:book,
|
33
|
+
{
|
34
|
+
title: 'The Tell-Tale Heart',
|
35
|
+
genre: 'Horror'
|
36
|
+
}
|
37
|
+
)
|
38
|
+
|
39
|
+
client.dsl.query(
|
40
|
+
datalog: <<~EDN
|
41
|
+
[:find ?e ?title ?genre
|
42
|
+
:where [?e :book/title ?title]
|
43
|
+
[?e :book/genre ?genre]]
|
44
|
+
EDN
|
45
|
+
)
|
46
|
+
```
|
47
|
+
|
48
|
+
```ruby
|
49
|
+
[[4611681620380877802, 'The Tell-Tale Heart', 'Horror']]
|
50
|
+
```
|
51
|
+
|
52
|
+
{{ index }}
|
53
|
+
|
54
|
+
## Flare
|
55
|
+
|
56
|
+
### Creating a Client
|
57
|
+
|
58
|
+
```ruby
|
59
|
+
require '{{ gem.name }}'
|
60
|
+
|
61
|
+
client = Flare.new(credentials: { address: 'http://localhost:3042' })
|
62
|
+
```
|
63
|
+
|
64
|
+
### Meta
|
65
|
+
|
66
|
+
```ruby
|
67
|
+
client.meta
|
68
|
+
```
|
69
|
+
|
70
|
+
```ruby
|
71
|
+
{
|
72
|
+
'meta' =>
|
73
|
+
{
|
74
|
+
'at' => '2024-09-29T13:52:52.252882446Z',
|
75
|
+
'mode' => 'peer',
|
76
|
+
'took' => { 'milliseconds' => 0.377446 }
|
77
|
+
},
|
78
|
+
'data' =>
|
79
|
+
{
|
80
|
+
'mode' => 'peer',
|
81
|
+
'datomic-flare' => '1.0.0',
|
82
|
+
'org.clojure/clojure' => '1.12.0',
|
83
|
+
'com.datomic/peer' => '1.0.7187',
|
84
|
+
'com.datomic/client-pro' => '1.0.81'
|
85
|
+
}
|
86
|
+
}
|
87
|
+
```
|
88
|
+
|
89
|
+
{{ dsl }}
|
90
|
+
|
91
|
+
{{ api }}
|
92
|
+
|
93
|
+
## Development
|
94
|
+
|
95
|
+
```bash
|
96
|
+
bundle
|
97
|
+
rubocop -A
|
98
|
+
|
99
|
+
```
|
100
|
+
|
101
|
+
### Publish to RubyGems
|
102
|
+
|
103
|
+
```bash
|
104
|
+
gem build {{ gem.name }}.gemspec
|
105
|
+
|
106
|
+
gem signin
|
107
|
+
|
108
|
+
gem push {{ gem.name }}-{{ gem.version }}.gem
|
109
|
+
|
110
|
+
```
|
111
|
+
|
112
|
+
### Setup for Tests and Documentation
|
113
|
+
|
114
|
+
Tests run against real Datomic databases, and documentation (README) is generated by interacting with real Datomic databases.
|
115
|
+
|
116
|
+
To accomplish that, we need to have [Datomic](https://github.com/gbaptista/datomic-pro-docker) and [Flare](https://github.com/gbaptista/datomic-flare) running.
|
117
|
+
|
118
|
+
**TL;DR:**
|
119
|
+
|
120
|
+
```bash
|
121
|
+
git clone https://github.com/gbaptista/datomic-pro-docker.git
|
122
|
+
|
123
|
+
cd datomic-pro-docker
|
124
|
+
|
125
|
+
cp compose/flare-dev.yml docker-compose.yml
|
126
|
+
|
127
|
+
docker compose up -d datomic-storage
|
128
|
+
|
129
|
+
docker compose run datomic-tools psql \
|
130
|
+
-f bin/sql/postgres-table.sql \
|
131
|
+
-h datomic-storage \
|
132
|
+
-U datomic-user \
|
133
|
+
-d my-datomic-storage
|
134
|
+
|
135
|
+
docker compose up -d datomic-transactor
|
136
|
+
|
137
|
+
docker compose run datomic-tools clojure -M -e "$(cat <<'CLOJURE'
|
138
|
+
(require '[datomic.api :as d])
|
139
|
+
|
140
|
+
(d/create-database "datomic:sql://my-datomic-database?jdbc:postgresql://datomic-storage:5432/my-datomic-storage?user=datomic-user&password=unsafe")
|
141
|
+
|
142
|
+
(d/create-database "datomic:sql://my-datomic-database-test?jdbc:postgresql://datomic-storage:5432/my-datomic-storage?user=datomic-user&password=unsafe")
|
143
|
+
|
144
|
+
(d/create-database "datomic:sql://my-datomic-database-test-green?jdbc:postgresql://datomic-storage:5432/my-datomic-storage?user=datomic-user&password=unsafe")
|
145
|
+
|
146
|
+
(System/exit 0)
|
147
|
+
CLOJURE
|
148
|
+
)"
|
149
|
+
|
150
|
+
docker compose up -d datomic-peer-server
|
151
|
+
|
152
|
+
docker compose up -d datomic-flare-peer datomic-flare-client
|
153
|
+
|
154
|
+
```
|
155
|
+
|
156
|
+
```bash
|
157
|
+
curl -s http://localhost:3042/meta \
|
158
|
+
-X GET \
|
159
|
+
-H "Content-Type: application/json" \
|
160
|
+
| jq
|
161
|
+
|
162
|
+
```
|
163
|
+
|
164
|
+
```json
|
165
|
+
{
|
166
|
+
"data": {
|
167
|
+
"mode": "peer"
|
168
|
+
}
|
169
|
+
}
|
170
|
+
|
171
|
+
```
|
172
|
+
|
173
|
+
```bash
|
174
|
+
curl -s http://localhost:3043/meta \
|
175
|
+
-X GET \
|
176
|
+
-H "Content-Type: application/json" \
|
177
|
+
| jq
|
178
|
+
|
179
|
+
```
|
180
|
+
|
181
|
+
```json
|
182
|
+
{
|
183
|
+
"data": {
|
184
|
+
"mode": "client"
|
185
|
+
}
|
186
|
+
}
|
187
|
+
|
188
|
+
```
|
189
|
+
|
190
|
+
You are ready to run tests and generate documentation.
|
191
|
+
|
192
|
+
**Detailed instructions:**
|
193
|
+
|
194
|
+
Clone the [datomic-pro-docker](https://github.com/gbaptista/datomic-pro-docker) repository and copy the Docker Compose template:
|
195
|
+
|
196
|
+
```bash
|
197
|
+
git clone https://github.com/gbaptista/datomic-pro-docker.git
|
198
|
+
|
199
|
+
cd datomic-pro-docker
|
200
|
+
|
201
|
+
cp compose/flare-dev.yml docker-compose.yml
|
202
|
+
|
203
|
+
```
|
204
|
+
|
205
|
+
Start PostgreSQL as Datomic's storage service:
|
206
|
+
|
207
|
+
```bash
|
208
|
+
docker compose up -d datomic-storage
|
209
|
+
|
210
|
+
docker compose logs -f datomic-storage
|
211
|
+
|
212
|
+
```
|
213
|
+
|
214
|
+
Create the table for Datomic databases:
|
215
|
+
|
216
|
+
```bash
|
217
|
+
docker compose run datomic-tools psql \
|
218
|
+
-f bin/sql/postgres-table.sql \
|
219
|
+
-h datomic-storage \
|
220
|
+
-U datomic-user \
|
221
|
+
-d my-datomic-storage
|
222
|
+
|
223
|
+
```
|
224
|
+
|
225
|
+
You will be prompted for a password, which is `unsafe`.
|
226
|
+
|
227
|
+
Start the Datomic Transactor:
|
228
|
+
|
229
|
+
```bash
|
230
|
+
docker compose up -d datomic-transactor
|
231
|
+
|
232
|
+
docker compose logs -f datomic-transactor
|
233
|
+
|
234
|
+
```
|
235
|
+
|
236
|
+
Create the following databases:
|
237
|
+
|
238
|
+
- `my-datomic-database`
|
239
|
+
- `my-datomic-database-test`
|
240
|
+
- `my-datomic-database-test-green`
|
241
|
+
|
242
|
+
```bash
|
243
|
+
docker compose run datomic-tools clojure -M -e "$(cat <<'CLOJURE'
|
244
|
+
(require '[datomic.api :as d])
|
245
|
+
|
246
|
+
(d/create-database "datomic:sql://my-datomic-database?jdbc:postgresql://datomic-storage:5432/my-datomic-storage?user=datomic-user&password=unsafe")
|
247
|
+
|
248
|
+
(d/create-database "datomic:sql://my-datomic-database-test?jdbc:postgresql://datomic-storage:5432/my-datomic-storage?user=datomic-user&password=unsafe")
|
249
|
+
|
250
|
+
(d/create-database "datomic:sql://my-datomic-database-test-green?jdbc:postgresql://datomic-storage:5432/my-datomic-storage?user=datomic-user&password=unsafe")
|
251
|
+
|
252
|
+
(System/exit 0)
|
253
|
+
CLOJURE
|
254
|
+
)"
|
255
|
+
|
256
|
+
```
|
257
|
+
|
258
|
+
Start the Peer Server:
|
259
|
+
|
260
|
+
```bash
|
261
|
+
docker compose up -d datomic-peer-server
|
262
|
+
|
263
|
+
docker compose logs -f datomic-peer-server
|
264
|
+
|
265
|
+
```
|
266
|
+
|
267
|
+
Start 2 instances of Flare, one in Peer Mode and another in Client Mode:
|
268
|
+
|
269
|
+
```bash
|
270
|
+
docker compose up -d datomic-flare-peer datomic-flare-client
|
271
|
+
|
272
|
+
docker compose logs -f datomic-flare-peer
|
273
|
+
docker compose logs -f datomic-flare-client
|
274
|
+
|
275
|
+
```
|
276
|
+
|
277
|
+
You should be able to request both:
|
278
|
+
|
279
|
+
Datomic Flare in Peer Mode:
|
280
|
+
```bash
|
281
|
+
curl -s http://localhost:3042/meta \
|
282
|
+
-X GET \
|
283
|
+
-H "Content-Type: application/json" \
|
284
|
+
| jq
|
285
|
+
|
286
|
+
```
|
287
|
+
|
288
|
+
```json
|
289
|
+
{
|
290
|
+
"data": {
|
291
|
+
"mode": "peer"
|
292
|
+
}
|
293
|
+
}
|
294
|
+
|
295
|
+
```
|
296
|
+
|
297
|
+
Datomic Flare in Client Mode:
|
298
|
+
```bash
|
299
|
+
curl -s http://localhost:3043/meta \
|
300
|
+
-X GET \
|
301
|
+
-H "Content-Type: application/json" \
|
302
|
+
| jq
|
303
|
+
|
304
|
+
```
|
305
|
+
|
306
|
+
```json
|
307
|
+
{
|
308
|
+
"data": {
|
309
|
+
"mode": "client"
|
310
|
+
}
|
311
|
+
}
|
312
|
+
|
313
|
+
```
|
314
|
+
|
315
|
+
You are ready to run tests and generate documentation.
|
316
|
+
|
317
|
+
### Running Tests
|
318
|
+
|
319
|
+
Tests run against real Datomic databases, so complete the [Setup for Tests and Documentation](#setup-for-tests-and-documentation) first.
|
320
|
+
|
321
|
+
```bash
|
322
|
+
cp .env.example .env
|
323
|
+
|
324
|
+
bundle exec rspec
|
325
|
+
|
326
|
+
```
|
327
|
+
|
328
|
+
### Updating the README
|
329
|
+
|
330
|
+
Documentation (README) is generated by interacting with real Datomic databases, so complete the [Setup for Tests and Documentation](#setup-for-tests-and-documentation) first.
|
331
|
+
|
332
|
+
Update the `docs/templates/*.md` files, and then:
|
333
|
+
|
334
|
+
```sh
|
335
|
+
cp .env.example .env
|
336
|
+
|
337
|
+
bundle exec ruby ports/cli.rb docs:generate
|
338
|
+
|
339
|
+
```
|
340
|
+
|
341
|
+
Trick for automatically updating the `README.md` when `docs/templates/*.md` files change:
|
342
|
+
|
343
|
+
```sh
|
344
|
+
sudo pacman -S inotify-tools # Arch / Manjaro
|
345
|
+
sudo apt-get install inotify-tools # Debian / Ubuntu / Raspberry Pi OS
|
346
|
+
sudo dnf install inotify-tools # Fedora / CentOS / RHEL
|
347
|
+
|
348
|
+
while inotifywait -e modify docs/templates/*; \
|
349
|
+
do bundle exec ruby ports/cli.rb docs:generate; \
|
350
|
+
done
|
351
|
+
|
352
|
+
```
|
353
|
+
|
354
|
+
Trick for Markdown Live Preview:
|
355
|
+
```sh
|
356
|
+
pip install -U markdown_live_preview
|
357
|
+
|
358
|
+
mlp README.md -p 8042 --no-follow
|
359
|
+
|
360
|
+
```
|