zrb 1.4.0__py3-none-any.whl → 1.4.2__py3-none-any.whl
This diff represents the content of publicly available package versions that have been released to one of the supported registries. The information contained in this diff is provided for informational purposes only and reflects changes between package versions as they appear in their respective public registries.
- zrb/__main__.py +25 -1
- zrb/builtin/llm/llm_chat.py +7 -6
- zrb/builtin/llm/tool/file.py +109 -33
- zrb/config.py +0 -9
- zrb/llm_config.py +55 -10
- zrb/runner/cli.py +0 -2
- zrb/task/llm_task.py +9 -7
- zrb/util/load.py +0 -16
- {zrb-1.4.0.dist-info → zrb-1.4.2.dist-info}/METADATA +78 -47
- {zrb-1.4.0.dist-info → zrb-1.4.2.dist-info}/RECORD +12 -12
- {zrb-1.4.0.dist-info → zrb-1.4.2.dist-info}/WHEEL +0 -0
- {zrb-1.4.0.dist-info → zrb-1.4.2.dist-info}/entry_points.txt +0 -0
zrb/__main__.py
CHANGED
@@ -1,3 +1,4 @@
|
|
1
|
+
import os
|
1
2
|
import sys
|
2
3
|
|
3
4
|
from zrb.config import INIT_MODULES, INIT_SCRIPTS
|
@@ -9,10 +10,19 @@ from zrb.util.load import load_file, load_module
|
|
9
10
|
|
10
11
|
def serve_cli():
|
11
12
|
try:
|
13
|
+
# load init modules
|
12
14
|
for init_module in INIT_MODULES:
|
13
15
|
load_module(init_module)
|
16
|
+
zrb_init_path_list = _get_zrb_init_path_list()
|
17
|
+
# load init scripts
|
14
18
|
for init_script in INIT_SCRIPTS:
|
15
|
-
|
19
|
+
abs_init_script = os.path.abspath(init_script)
|
20
|
+
if abs_init_script not in zrb_init_path_list:
|
21
|
+
load_file(abs_init_script, -1)
|
22
|
+
# load zrb init
|
23
|
+
for zrb_init_path in zrb_init_path_list:
|
24
|
+
load_file(zrb_init_path)
|
25
|
+
# run the CLI
|
16
26
|
cli.run(sys.argv[1:])
|
17
27
|
except KeyboardInterrupt:
|
18
28
|
print(stylize_warning("\nStopped"), file=sys.stderr)
|
@@ -24,3 +34,17 @@ def serve_cli():
|
|
24
34
|
except NodeNotFoundError as e:
|
25
35
|
print(stylize_error(f"{e}"), file=sys.stderr)
|
26
36
|
sys.exit(1)
|
37
|
+
|
38
|
+
|
39
|
+
def _get_zrb_init_path_list() -> list[str]:
|
40
|
+
current_path = os.path.abspath(os.getcwd())
|
41
|
+
dir_path_list = [current_path]
|
42
|
+
while current_path != os.path.dirname(current_path): # Stop at root
|
43
|
+
current_path = os.path.dirname(current_path)
|
44
|
+
dir_path_list.append(current_path)
|
45
|
+
zrb_init_path_list = []
|
46
|
+
for current_path in dir_path_list[::-1]:
|
47
|
+
zrb_init_path = os.path.join(current_path, "zrb_init.py")
|
48
|
+
if os.path.isfile(zrb_init_path):
|
49
|
+
zrb_init_path_list.append(zrb_init_path)
|
50
|
+
return zrb_init_path_list
|
zrb/builtin/llm/llm_chat.py
CHANGED
@@ -22,7 +22,6 @@ from zrb.config import (
|
|
22
22
|
LLM_ALLOW_ACCESS_LOCAL_FILE,
|
23
23
|
LLM_ALLOW_ACCESS_SHELL,
|
24
24
|
LLM_HISTORY_DIR,
|
25
|
-
LLM_SYSTEM_PROMPT,
|
26
25
|
SERP_API_KEY,
|
27
26
|
)
|
28
27
|
from zrb.context.any_shared_context import AnySharedContext
|
@@ -119,7 +118,7 @@ llm_chat: LLMTask = llm_group.add_task(
|
|
119
118
|
"system-prompt",
|
120
119
|
description="System prompt",
|
121
120
|
prompt="System prompt",
|
122
|
-
default=
|
121
|
+
default="",
|
123
122
|
allow_positional_parsing=False,
|
124
123
|
always_prompt=False,
|
125
124
|
),
|
@@ -141,17 +140,19 @@ llm_chat: LLMTask = llm_group.add_task(
|
|
141
140
|
always_prompt=False,
|
142
141
|
),
|
143
142
|
],
|
144
|
-
model=lambda ctx: None if ctx.input.model == "" else ctx.input.model,
|
143
|
+
model=lambda ctx: None if ctx.input.model.strip() == "" else ctx.input.model,
|
145
144
|
model_base_url=lambda ctx: (
|
146
|
-
None if ctx.input.base_url == "" else ctx.input.base_url
|
145
|
+
None if ctx.input.base_url.strip() == "" else ctx.input.base_url
|
147
146
|
),
|
148
147
|
model_api_key=lambda ctx: (
|
149
|
-
None if ctx.input.api_key == "" else ctx.input.api_key
|
148
|
+
None if ctx.input.api_key.strip() == "" else ctx.input.api_key
|
150
149
|
),
|
151
150
|
conversation_history_reader=_read_chat_conversation,
|
152
151
|
conversation_history_writer=_write_chat_conversation,
|
153
152
|
description="Chat with LLM",
|
154
|
-
system_prompt=
|
153
|
+
system_prompt=lambda ctx: (
|
154
|
+
None if ctx.input.system_prompt.strip() == "" else ctx.input.system_prompt
|
155
|
+
),
|
155
156
|
message="{ctx.input.message}",
|
156
157
|
retries=0,
|
157
158
|
),
|
zrb/builtin/llm/tool/file.py
CHANGED
@@ -3,25 +3,92 @@ import os
|
|
3
3
|
|
4
4
|
from zrb.util.file import read_file, write_file
|
5
5
|
|
6
|
+
_INCLUDED_PATTERNS: list[str] = [
|
7
|
+
"*.py", # Python
|
8
|
+
"*.go", # Go
|
9
|
+
"*.rs", # Rust
|
10
|
+
"*.js", # JavaScript
|
11
|
+
"*.ts", # TypeScript
|
12
|
+
"*.java", # Java
|
13
|
+
"*.c", # C
|
14
|
+
"*.cpp", # C++
|
15
|
+
"*.cc", # Alternative C++ extension
|
16
|
+
"*.cxx", # Alternative C++ extension
|
17
|
+
"*.rb", # Ruby
|
18
|
+
"*.swift", # Swift
|
19
|
+
"*.kt", # Kotlin
|
20
|
+
"*.php", # PHP
|
21
|
+
"*.pl", # Perl / Prolog
|
22
|
+
"*.pm", # Perl module
|
23
|
+
"*.sh", # Shell
|
24
|
+
"*.bat", # Batch
|
25
|
+
"*.ps1", # PowerShell
|
26
|
+
"*.R", # R (capital)
|
27
|
+
"*.r", # R (lowercase)
|
28
|
+
"*.scala", # Scala
|
29
|
+
"*.hs", # Haskell
|
30
|
+
"*.cs", # C#
|
31
|
+
"*.fs", # F#
|
32
|
+
"*.ex", # Elixir
|
33
|
+
"*.exs", # Elixir script
|
34
|
+
"*.erl", # Erlang
|
35
|
+
"*.hrl", # Erlang header
|
36
|
+
"*.dart", # Dart
|
37
|
+
"*.m", # Objective-C / Matlab (note: conflicts may arise)
|
38
|
+
"*.mm", # Objective-C++
|
39
|
+
"*.lua", # Lua
|
40
|
+
"*.jl", # Julia
|
41
|
+
"*.groovy", # Groovy
|
42
|
+
"*.clj", # Clojure
|
43
|
+
"*.cljs", # ClojureScript
|
44
|
+
"*.cljc", # Clojure common
|
45
|
+
"*.vb", # Visual Basic
|
46
|
+
"*.f90", # Fortran
|
47
|
+
"*.f95", # Fortran
|
48
|
+
"*.adb", # Ada
|
49
|
+
"*.ads", # Ada specification
|
50
|
+
"*.pas", # Pascal
|
51
|
+
"*.pp", # Pascal
|
52
|
+
"*.ml", # OCaml
|
53
|
+
"*.mli", # OCaml interface
|
54
|
+
"*.nim", # Nim
|
55
|
+
"*.rkt", # Racket
|
56
|
+
"*.d", # D
|
57
|
+
"*.lisp", # Common Lisp
|
58
|
+
"*.lsp", # Lisp variant
|
59
|
+
"*.cl", # Common Lisp
|
60
|
+
"*.scm", # Scheme
|
61
|
+
"*.st", # Smalltalk
|
62
|
+
"*.vhd", # VHDL
|
63
|
+
"*.vhdl", # VHDL
|
64
|
+
"*.v", # Verilog
|
65
|
+
"*.asm", # Assembly
|
66
|
+
"*.s", # Assembly (alternative)
|
67
|
+
"*.sql", # SQL (if desired)
|
68
|
+
]
|
69
|
+
|
70
|
+
# Extended list of directories and patterns to exclude.
|
71
|
+
_EXCLUDED_PATTERNS: list[str] = [
|
72
|
+
"venv", # Python virtual environments
|
73
|
+
".venv",
|
74
|
+
"node_modules", # Node.js dependencies
|
75
|
+
".git", # Git repositories
|
76
|
+
"__pycache__", # Python cache directories
|
77
|
+
"build", # Build directories
|
78
|
+
"dist", # Distribution directories
|
79
|
+
"target", # Build output directories (Java, Rust, etc.)
|
80
|
+
"bin", # Binary directories
|
81
|
+
"obj", # Object files directories
|
82
|
+
".idea", # JetBrains IDEs
|
83
|
+
".vscode", # VS Code settings
|
84
|
+
".eggs", # Python eggs
|
85
|
+
]
|
86
|
+
|
6
87
|
|
7
88
|
def list_files(
|
8
89
|
directory: str = ".",
|
9
|
-
included_patterns: list[str] =
|
10
|
-
|
11
|
-
"*.go",
|
12
|
-
"*.js",
|
13
|
-
"*.ts",
|
14
|
-
"*.java",
|
15
|
-
"*.c",
|
16
|
-
"*.cpp",
|
17
|
-
],
|
18
|
-
excluded_patterns: list[str] = [
|
19
|
-
"venv",
|
20
|
-
".venv",
|
21
|
-
"node_modules",
|
22
|
-
".git",
|
23
|
-
"__pycache__",
|
24
|
-
],
|
90
|
+
included_patterns: list[str] = _INCLUDED_PATTERNS,
|
91
|
+
excluded_patterns: list[str] = _EXCLUDED_PATTERNS,
|
25
92
|
) -> list[str]:
|
26
93
|
"""List all files in a directory that match any of the included glob patterns
|
27
94
|
and do not reside in any directory matching an excluded pattern.
|
@@ -32,38 +99,47 @@ def list_files(
|
|
32
99
|
for filename in files:
|
33
100
|
if any(fnmatch.fnmatch(filename, pat) for pat in included_patterns):
|
34
101
|
full_path = os.path.join(root, filename)
|
35
|
-
|
36
|
-
if any(
|
37
|
-
any(fnmatch.fnmatch(part, pat) for pat in excluded_patterns)
|
38
|
-
for part in os.path.normpath(full_path).split(os.sep)
|
39
|
-
):
|
102
|
+
if _should_exclude(full_path, excluded_patterns):
|
40
103
|
continue
|
41
104
|
all_files.append(full_path)
|
42
105
|
return all_files
|
43
106
|
|
44
107
|
|
108
|
+
def _should_exclude(full_path: str, excluded_patterns: list[str]) -> bool:
|
109
|
+
"""
|
110
|
+
Return True if the file at full_path should be excluded based on
|
111
|
+
the list of excluded_patterns. Patterns that include a path separator
|
112
|
+
are applied to the full normalized path; otherwise they are matched
|
113
|
+
against each individual component of the path.
|
114
|
+
"""
|
115
|
+
norm_path = os.path.normpath(full_path)
|
116
|
+
path_parts = norm_path.split(os.sep)
|
117
|
+
for pat in excluded_patterns:
|
118
|
+
# If the pattern seems intended for full path matching (contains a separator)
|
119
|
+
if os.sep in pat or "/" in pat:
|
120
|
+
if fnmatch.fnmatch(norm_path, pat):
|
121
|
+
return True
|
122
|
+
else:
|
123
|
+
# Otherwise check each part of the path
|
124
|
+
if any(fnmatch.fnmatch(part, pat) for part in path_parts):
|
125
|
+
return True
|
126
|
+
return False
|
127
|
+
|
128
|
+
|
45
129
|
def read_text_file(file: str) -> str:
|
46
|
-
"""Read a text file"""
|
130
|
+
"""Read a text file and return a string containing the file content."""
|
47
131
|
return read_file(os.path.abspath(file))
|
48
132
|
|
49
133
|
|
50
134
|
def write_text_file(file: str, content: str):
|
51
|
-
"""Write a text file"""
|
135
|
+
"""Write content to a text file"""
|
52
136
|
return write_file(os.path.abspath(file), content)
|
53
137
|
|
54
138
|
|
55
139
|
def read_all_files(
|
56
140
|
directory: str = ".",
|
57
|
-
included_patterns: list[str] =
|
58
|
-
|
59
|
-
"*.go",
|
60
|
-
"*.js",
|
61
|
-
"*.ts",
|
62
|
-
"*.java",
|
63
|
-
"*.c",
|
64
|
-
"*.cpp",
|
65
|
-
],
|
66
|
-
excluded_patterns: list[str] = [],
|
141
|
+
included_patterns: list[str] = _INCLUDED_PATTERNS,
|
142
|
+
excluded_patterns: list[str] = _EXCLUDED_PATTERNS,
|
67
143
|
) -> list[str]:
|
68
144
|
"""Read all files in a directory that match any of the included glob patterns
|
69
145
|
and do not match any of the excluded glob patterns.
|
zrb/config.py
CHANGED
@@ -76,15 +76,6 @@ WEB_AUTH_REFRESH_TOKEN_EXPIRE_MINUTES = int(
|
|
76
76
|
os.getenv("ZRB_WEB_REFRESH_TOKEN_EXPIRE_MINUTES", "60")
|
77
77
|
)
|
78
78
|
|
79
|
-
_DEFAULT_PROMPT = (
|
80
|
-
"You are a helpful AI assistant capable of using various tools to answer user queries. When solving a problem:\n"
|
81
|
-
"1. Carefully analyze the user's request and identify what information is needed to provide a complete answer.\n"
|
82
|
-
"2. Determine which available tools can help you gather the necessary information.\n"
|
83
|
-
"3. Call tools strategically and in a logical sequence to collect required data.\n"
|
84
|
-
"4. If a tool provides incomplete information, intelligently decide which additional tool or approach to use.\n"
|
85
|
-
"5. Always aim to provide the most accurate and helpful response possible."
|
86
|
-
)
|
87
|
-
LLM_SYSTEM_PROMPT = os.getenv("ZRB_LLM_SYSTEM_PROMPT", _DEFAULT_PROMPT)
|
88
79
|
LLM_HISTORY_DIR = os.getenv(
|
89
80
|
"ZRB_LLM_HISTORY_DIR", os.path.expanduser(os.path.join("~", ".zrb-llm-history"))
|
90
81
|
)
|
zrb/llm_config.py
CHANGED
@@ -4,23 +4,49 @@ from pydantic_ai.models import Model
|
|
4
4
|
from pydantic_ai.models.openai import OpenAIModel
|
5
5
|
from pydantic_ai.providers.openai import OpenAIProvider
|
6
6
|
|
7
|
+
DEFAULT_SYSTEM_PROMPT = """
|
8
|
+
You have access to tools.
|
9
|
+
Your goal to to answer user queries accurately.
|
10
|
+
Follow these instructions precisely:
|
11
|
+
1. ALWAYS use available tools to gather information BEFORE asking the user questions
|
12
|
+
2. For tools that require arguments: provide arguments in valid JSON format
|
13
|
+
3. For tools that require NO arguments: call with empty JSON object ({}) NOT empty string ('')
|
14
|
+
4. NEVER pass arguments to tools that don't accept parameters
|
15
|
+
5. NEVER ask users for information obtainable through tools
|
16
|
+
6. Use tools in logical sequence until you have sufficient information
|
17
|
+
7. If a tool call fails, check if you're passing arguments in the correct format
|
18
|
+
8. Only after exhausting relevant tools should you request clarification
|
19
|
+
""".strip()
|
20
|
+
|
7
21
|
|
8
22
|
class LLMConfig:
|
9
23
|
|
10
24
|
def __init__(
|
11
25
|
self,
|
12
|
-
|
13
|
-
|
14
|
-
|
26
|
+
default_model_name: str | None = None,
|
27
|
+
default_base_url: str | None = None,
|
28
|
+
default_api_key: str | None = None,
|
29
|
+
default_system_prompt: str | None = None,
|
15
30
|
):
|
16
31
|
self._model_name = (
|
17
|
-
|
32
|
+
default_model_name
|
33
|
+
if default_model_name is not None
|
34
|
+
else os.getenv("ZRB_LLM_MODEL", None)
|
35
|
+
)
|
36
|
+
self._model_base_url = (
|
37
|
+
default_base_url
|
38
|
+
if default_base_url is not None
|
39
|
+
else os.getenv("ZRB_LLM_BASE_URL", None)
|
18
40
|
)
|
19
|
-
self.
|
20
|
-
|
41
|
+
self._model_api_key = (
|
42
|
+
default_api_key
|
43
|
+
if default_api_key is not None
|
44
|
+
else os.getenv("ZRB_LLM_API_KEY", None)
|
21
45
|
)
|
22
|
-
self.
|
23
|
-
|
46
|
+
self._system_prompt = (
|
47
|
+
default_system_prompt
|
48
|
+
if default_system_prompt is not None
|
49
|
+
else os.getenv("ZRB_LLM_SYSTEM_PROMPT", None)
|
24
50
|
)
|
25
51
|
self._default_model = None
|
26
52
|
|
@@ -28,9 +54,16 @@ class LLMConfig:
|
|
28
54
|
return self._model_name if self._model_name is not None else None
|
29
55
|
|
30
56
|
def _get_model_provider(self) -> OpenAIProvider:
|
31
|
-
if self.
|
57
|
+
if self._model_base_url is None and self._model_api_key is None:
|
32
58
|
return "openai"
|
33
|
-
return OpenAIProvider(
|
59
|
+
return OpenAIProvider(
|
60
|
+
base_url=self._model_base_url, api_key=self._model_api_key
|
61
|
+
)
|
62
|
+
|
63
|
+
def get_default_system_prompt(self) -> str:
|
64
|
+
if self._system_prompt is not None:
|
65
|
+
return self._system_prompt
|
66
|
+
return DEFAULT_SYSTEM_PROMPT
|
34
67
|
|
35
68
|
def get_default_model(self) -> Model | str | None:
|
36
69
|
if self._default_model is not None:
|
@@ -43,6 +76,18 @@ class LLMConfig:
|
|
43
76
|
provider=self._get_model_provider(),
|
44
77
|
)
|
45
78
|
|
79
|
+
def set_default_system_prompt(self, system_prompt: str):
|
80
|
+
self._system_prompt = system_prompt
|
81
|
+
|
82
|
+
def set_default_model_name(self, model_name: str):
|
83
|
+
self._model_name = model_name
|
84
|
+
|
85
|
+
def set_default_model_api_key(self, model_api_key: str):
|
86
|
+
self._model_api_key = model_api_key
|
87
|
+
|
88
|
+
def set_default_model_base_url(self, model_base_url: str):
|
89
|
+
self._model_base_url = model_base_url
|
90
|
+
|
46
91
|
def set_default_model(self, model: Model | str | None):
|
47
92
|
self._default_model = model
|
48
93
|
|
zrb/runner/cli.py
CHANGED
@@ -18,13 +18,11 @@ from zrb.util.cli.style import (
|
|
18
18
|
stylize_section_header,
|
19
19
|
)
|
20
20
|
from zrb.util.group import extract_node_from_args, get_non_empty_subgroups, get_subtasks
|
21
|
-
from zrb.util.load import load_zrb_init
|
22
21
|
from zrb.util.string.conversion import double_quote
|
23
22
|
|
24
23
|
|
25
24
|
class Cli(Group):
|
26
25
|
def run(self, args: list[str] = []):
|
27
|
-
load_zrb_init()
|
28
26
|
kwargs, args = self._extract_kwargs_from_args(args)
|
29
27
|
node, node_path, args = extract_node_from_args(self, args)
|
30
28
|
if isinstance(node, Group):
|
zrb/task/llm_task.py
CHANGED
@@ -18,7 +18,6 @@ from pydantic_ai.models import Model
|
|
18
18
|
from pydantic_ai.settings import ModelSettings
|
19
19
|
|
20
20
|
from zrb.attr.type import StrAttr, fstring
|
21
|
-
from zrb.config import LLM_SYSTEM_PROMPT
|
22
21
|
from zrb.context.any_context import AnyContext
|
23
22
|
from zrb.context.any_shared_context import AnySharedContext
|
24
23
|
from zrb.env.any_env import AnyEnv
|
@@ -58,7 +57,7 @@ class LLMTask(BaseTask):
|
|
58
57
|
ModelSettings | Callable[[AnySharedContext], ModelSettings] | None
|
59
58
|
) = None,
|
60
59
|
agent: Agent | Callable[[AnySharedContext], Agent] | None = None,
|
61
|
-
system_prompt: StrAttr | None =
|
60
|
+
system_prompt: StrAttr | None = None,
|
62
61
|
render_system_prompt: bool = True,
|
63
62
|
message: StrAttr | None = None,
|
64
63
|
tools: (
|
@@ -258,14 +257,14 @@ class LLMTask(BaseTask):
|
|
258
257
|
return default_llm_config.get_default_model()
|
259
258
|
if isinstance(model, str):
|
260
259
|
llm_config = LLMConfig(
|
261
|
-
|
262
|
-
|
260
|
+
default_model_name=model,
|
261
|
+
default_base_url=get_attr(
|
263
262
|
ctx,
|
264
263
|
self._get_model_base_url(ctx),
|
265
264
|
None,
|
266
265
|
auto_render=self._render_model_base_url,
|
267
266
|
),
|
268
|
-
|
267
|
+
default_api_key=get_attr(
|
269
268
|
ctx,
|
270
269
|
self._get_model_api_key(ctx),
|
271
270
|
None,
|
@@ -292,12 +291,15 @@ class LLMTask(BaseTask):
|
|
292
291
|
raise ValueError(f"Invalid model base URL: {api_key}")
|
293
292
|
|
294
293
|
def _get_system_prompt(self, ctx: AnyContext) -> str:
|
295
|
-
|
294
|
+
system_prompt = get_attr(
|
296
295
|
ctx,
|
297
296
|
self._system_prompt,
|
298
|
-
|
297
|
+
None,
|
299
298
|
auto_render=self._render_system_prompt,
|
300
299
|
)
|
300
|
+
if system_prompt is not None:
|
301
|
+
return system_prompt
|
302
|
+
return default_llm_config.get_default_system_prompt()
|
301
303
|
|
302
304
|
def _get_message(self, ctx: AnyContext) -> str:
|
303
305
|
return get_str_attr(ctx, self._message, "How are you?", auto_render=True)
|
zrb/util/load.py
CHANGED
@@ -9,22 +9,6 @@ from typing import Any
|
|
9
9
|
pattern = re.compile("[^a-zA-Z0-9]")
|
10
10
|
|
11
11
|
|
12
|
-
def load_zrb_init(dir_path: str | None = None) -> Any | None:
|
13
|
-
if dir_path is None:
|
14
|
-
dir_path = os.getcwd()
|
15
|
-
# get path list from current path to the absolute root
|
16
|
-
current_path = os.path.abspath(dir_path)
|
17
|
-
path_list = [current_path]
|
18
|
-
while current_path != os.path.dirname(current_path): # Stop at root
|
19
|
-
current_path = os.path.dirname(current_path)
|
20
|
-
path_list.append(current_path)
|
21
|
-
# loop from root to current path to load zrb_init
|
22
|
-
for current_path in path_list[::-1]:
|
23
|
-
script_path = os.path.join(current_path, "zrb_init.py")
|
24
|
-
if os.path.isfile(script_path):
|
25
|
-
load_file(script_path)
|
26
|
-
|
27
|
-
|
28
12
|
@lru_cache
|
29
13
|
def load_file(script_path: str, sys_path_index: int = 0) -> Any | None:
|
30
14
|
if not os.path.isfile(script_path):
|
@@ -1,6 +1,6 @@
|
|
1
1
|
Metadata-Version: 2.1
|
2
2
|
Name: zrb
|
3
|
-
Version: 1.4.
|
3
|
+
Version: 1.4.2
|
4
4
|
Summary: Your Automation Powerhouse
|
5
5
|
Home-page: https://github.com/state-alchemists/zrb
|
6
6
|
License: AGPL-3.0-or-later
|
@@ -39,29 +39,58 @@ Description-Content-Type: text/markdown
|
|
39
39
|
|
40
40
|
# 🤖 Zrb: Your Automation Powerhouse
|
41
41
|
|
42
|
-
|
42
|
+
|
43
|
+
**Unlock the full potential of automation in your projects!**
|
44
|
+
|
45
|
+
Zrb streamlines repetitive tasks, integrates with powerful LLMs, and lets you create custom automation workflows effortlessly. Whether you’re building CI/CD pipelines, code generators, or unique automation scripts, Zrb is designed to simplify and supercharge your workflow.
|
46
|
+
|
47
|
+
|
48
|
+
## 🚀 Why Zrb?
|
49
|
+
|
50
|
+
- **Easy Automation with Python:** Write your tasks in Python and let Zrb handle the rest.
|
51
|
+
- **Seamless Integration:** Utilize built-in support for LLM tasks, command execution, and more.
|
52
|
+
- **Custom Workflows:** Chain tasks, set dependencies, and build robust automation pipelines.
|
53
|
+
- **Developer-Friendly:** Quick to install and get started, with clear documentation and examples.
|
54
|
+
- **Web Interface:** Run Zrb as a server to make tasks accessible even to non-technical team members.
|
55
|
+
|
56
|
+
|
57
|
+
## 🔥 Key Features
|
58
|
+
|
59
|
+
- **LLM Integration:** Leverage state-of-the-art language models to generate code, diagrams, and documentation.
|
60
|
+
- **Task Chaining:** Easily define dependencies between tasks to create complex workflows.
|
61
|
+
- **CLI & Server Mode:** Run tasks directly from the command line or through a user-friendly web UI.
|
62
|
+
- **Flexible Input Handling:** Defaults, prompts, and command-line parameters to suit any workflow.
|
63
|
+
- **Extensible & Open Source:** Contribute, customize, or extend Zrb to fit your unique needs.
|
64
|
+
|
65
|
+
|
66
|
+
# 🛠️ Installation
|
67
|
+
|
68
|
+
Install Zrb via pip:
|
69
|
+
|
70
|
+
```bash
|
71
|
+
pip install zrb
|
72
|
+
|
73
|
+
```
|
74
|
+
|
75
|
+
Or run our installation script to set up Zrb along with all prerequisites:
|
76
|
+
|
77
|
+
```bash
|
78
|
+
bash -c "$(curl -fsSL https://raw.githubusercontent.com/state-alchemists/zrb/main/install.sh)"
|
79
|
+
|
80
|
+
```
|
81
|
+
|
82
|
+
# 🍲 Quick Start
|
83
|
+
|
84
|
+
Create a file at `/home/<your-user-name>/zrb_init.py` with the following content:
|
43
85
|
|
44
86
|
|
45
87
|
```python
|
46
88
|
import os
|
47
89
|
from zrb import cli, llm_config, LLMTask, CmdTask, StrInput, Group
|
48
90
|
from zrb.builtin.llm.tool.file import read_all_files, write_text_file
|
49
|
-
from pydantic_ai.models.openai import OpenAIModel
|
50
|
-
from pydantic_ai.providers.openai import OpenAIProvider
|
51
91
|
|
52
92
|
CURRENT_DIR = os.getcwd()
|
53
93
|
|
54
|
-
# Setup default LLM Config
|
55
|
-
llm_config.set_default_model(
|
56
|
-
OpenAIModel(
|
57
|
-
model_name="gpt-4o",
|
58
|
-
provider=OpenAIProvider(
|
59
|
-
base_url="https://openrouter.ai/api/v1",
|
60
|
-
api_key=os.getenv("OPENROUTER_API_KEY", "")
|
61
|
-
)
|
62
|
-
)
|
63
|
-
)
|
64
|
-
|
65
94
|
# Make UML group
|
66
95
|
uml_group = cli.add_group(Group(name="uml", description="UML related tasks"))
|
67
96
|
|
@@ -98,27 +127,39 @@ make_uml_image = uml_group.add_task(
|
|
98
127
|
make_uml_script >> make_uml_image
|
99
128
|
```
|
100
129
|
|
101
|
-
|
130
|
+
You have just define two automation tasks.
|
131
|
+
|
132
|
+
The first one use LLM to read files in your current directory and create a `PlantUML script` on that directory.
|
133
|
+
|
134
|
+
The second task turn the PlantUML script into a `*.png` file. The second task depends on the first task and both of them are located under the same group.
|
135
|
+
|
136
|
+
You can run the tasks by invoking `zrb uml make-script` or `zrb uml make-image` respectively.
|
137
|
+
|
138
|
+
When you run zrb, it automatically searches for a file named `zrb_init.py` starting from your current directory and moving upward through its parent directories. This design lets you set up common automation tasks in a central location—like placing a `zrb_init.py` in your home directory (`/home/<your-user>/zrb_init.py`)—so that your tasks are available across all your projects.
|
139
|
+
|
140
|
+
Now, go to your project and create a state diagram:
|
102
141
|
|
103
142
|
```bash
|
143
|
+
git clone git@github.com:jjinux/gotetris.git
|
144
|
+
cd gotetris
|
104
145
|
zrb uml make-image --diagram "state diagram"
|
105
146
|
```
|
106
147
|
|
107
|
-
|
148
|
+
You can also invoke the task without specifying parameter.
|
108
149
|
|
109
150
|
```bash
|
110
151
|
zrb uml make-image
|
111
152
|
```
|
112
153
|
|
113
|
-
|
154
|
+
Once you do so, Zrb will ask you to provide the diagram type.
|
114
155
|
|
115
156
|
```
|
116
157
|
diagram [state diagram]:
|
117
158
|
```
|
118
159
|
|
119
|
-
You can just press enter if you want to use the default value.
|
160
|
+
You can just press enter if you want to use the default value (i.e., in this case `state diagram`).
|
120
161
|
|
121
|
-
Finally, you can
|
162
|
+
Finally, you can also serve the tasks via a Web UI interface by invoking the following command:
|
122
163
|
|
123
164
|
```bash
|
124
165
|
zrb server start
|
@@ -128,49 +169,39 @@ You will have a nice web interface running on `http://localhost:12123`
|
|
128
169
|
|
129
170
|

|
130
171
|
|
131
|
-
Now, let's see how
|
172
|
+
Now, let's see how things work in detail. First, Zrb generates a `state diagram.uml` in your current directory, it then transform the UML script into a PNG image `state diagram.png`.
|
132
173
|
|
133
174
|

|
134
175
|
|
135
|
-
See the [getting started guide](https://github.com/state-alchemists/zrb/blob/main/docs/recipes/getting-started/README.md) for more information. Or just watch the demo:
|
136
|
-
|
137
|
-
[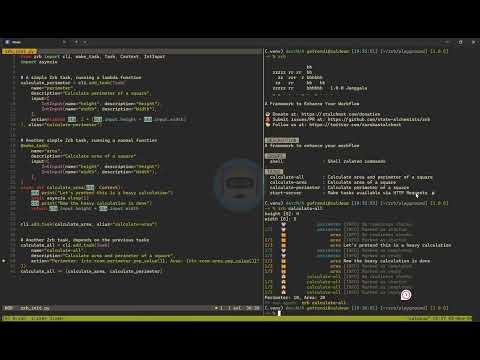](https://www.youtube.com/watch?v=W7dgk96l__o)
|
138
|
-
|
139
176
|
|
140
|
-
#
|
177
|
+
# 🎥 Demo & Documentation
|
141
178
|
|
142
|
-
|
179
|
+
- **Step by step guide:** [Getting started with Zrb](https://github.com/state-alchemists/zrb/blob/main/docs/recipes/getting-started/README.md).
|
180
|
+
- **Full documentation:** [Zrb Documentation](https://github.com/state-alchemists/zrb/blob/main/docs/README.md)
|
181
|
+
- **Video demo:**
|
182
|
+
[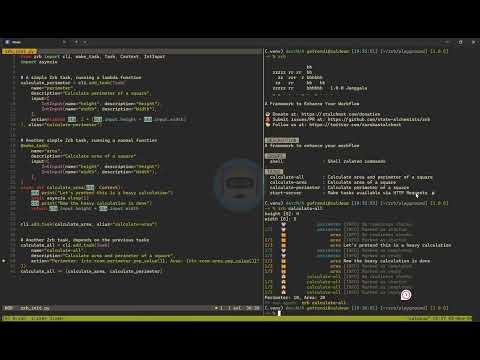](https://www.youtube.com/watch?v=W7dgk96l__o)
|
143
183
|
|
144
|
-
```bash
|
145
|
-
pip install --pre zrb
|
146
|
-
```
|
147
|
-
|
148
|
-
Alternatively, you can also use our installation script to install Zrb along with some prerequisites:
|
149
184
|
|
150
|
-
|
151
|
-
bash -c "$(curl -fsSL https://raw.githubusercontent.com/state-alchemists/zrb/main/install.sh)"
|
152
|
-
```
|
185
|
+
# 🤝 Join the Community
|
153
186
|
|
154
|
-
|
187
|
+
- **Bug Reports & Feature Requests:** Create an [issue](https://github.com/state-alchemists/zrb/issues) on Zrb's GitHub Repositories and include:
|
188
|
+
- Your Zrb version (i.e., `zrb version`).
|
189
|
+
- Steps you’ve taken and what you expected versus what happened
|
190
|
+
- **Contributions:** We welcome pull requests! Check out our [contribution guidelines](https://github.com/state-alchemists/zrb/pulls).
|
155
191
|
|
156
|
-
You can submit bug reports and feature requests by creating a new [issue](https://github.com/state-alchemists/zrb/issues) on Zrb's GitHub Repositories. When reporting a bug or requesting a feature, please be sure to:
|
157
192
|
|
158
|
-
|
159
|
-
- Tell us what you have tried
|
160
|
-
- Tell us what you expect
|
161
|
-
- Tell us what you get
|
193
|
+
# ☕ Support The Project
|
162
194
|
|
163
|
-
|
164
|
-
|
165
|
-
|
166
|
-
# ☕ Donation
|
167
|
-
|
168
|
-
Help Red Skull to click the donation button:
|
195
|
+
If you find Zrb valuable, please consider donating:
|
169
196
|
|
170
197
|
[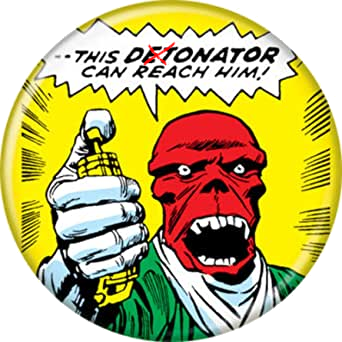](https://stalchmst.com/donation)
|
171
198
|
|
172
199
|
# 🎉 Fun Fact
|
173
200
|
|
201
|
+
Did you know?
|
202
|
+
|
203
|
+
Zrb is named after `Zaruba`, a powerful support tool from the Garo universe!
|
204
|
+
|
174
205
|
> Madou Ring Zaruba (魔導輪ザルバ, Madōrin Zaruba) is a Madougu which supports bearers of the Garo Armor. [(Garo Wiki | Fandom)](https://garo.fandom.com/wiki/Zaruba)
|
175
206
|
|
176
207
|
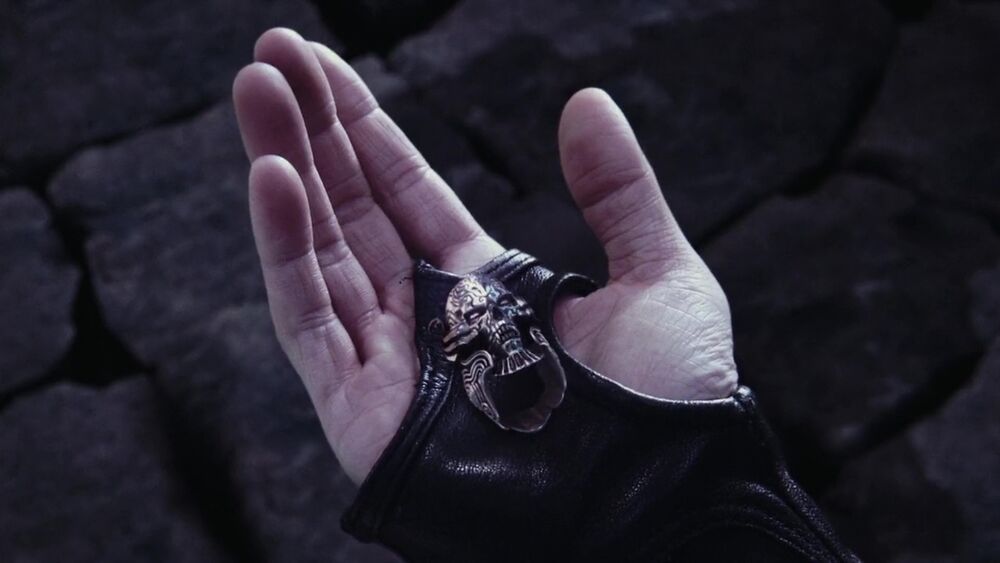
|
@@ -1,5 +1,5 @@
|
|
1
1
|
zrb/__init__.py,sha256=1waPjZcA3IHUEvIuVQso0YfNfW9i7SCJgEfzhiNTaCk,3020
|
2
|
-
zrb/__main__.py,sha256=
|
2
|
+
zrb/__main__.py,sha256=MvAGzoM3ElJZOPMKNqaTdnrT9PXi9Saq8CPa11RiLQk,1748
|
3
3
|
zrb/attr/__init__.py,sha256=47DEQpj8HBSa-_TImW-5JCeuQeRkm5NMpJWZG3hSuFU,0
|
4
4
|
zrb/attr/type.py,sha256=4TV5gPYMMrKh5V-yB6iRYKCbsXAH_AvGXMsjxKLHcUs,568
|
5
5
|
zrb/builtin/__init__.py,sha256=oXG4Zm_rIp3G81Y7hiSe38jeS2sGZAnADoP_yxxhYEc,1926
|
@@ -7,11 +7,11 @@ zrb/builtin/base64.py,sha256=1YnSwASp7OEAvQcsnHZGpJEvYoI1Z2zTIJ1bCDHfcPQ,921
|
|
7
7
|
zrb/builtin/git.py,sha256=8_qVE_2lVQEVXQ9vhiw8Tn4Prj1VZB78ZjEJJS5Ab3M,5461
|
8
8
|
zrb/builtin/git_subtree.py,sha256=7BKwOkVTWDrR0DXXQ4iJyHqeR6sV5VYRt8y_rEB0EHg,3505
|
9
9
|
zrb/builtin/group.py,sha256=-phJfVpTX3_gUwS1u8-RbZUHe-X41kxDBSmrVh4rq8E,1682
|
10
|
-
zrb/builtin/llm/llm_chat.py,sha256=
|
10
|
+
zrb/builtin/llm/llm_chat.py,sha256=OwbeXNaskyufYIhbhLmj9JRYB9bw5D8JfntAzOhmrP8,6140
|
11
11
|
zrb/builtin/llm/previous-session.js,sha256=xMKZvJoAbrwiyHS0OoPrWuaKxWYLoyR5sguePIoCjTY,816
|
12
12
|
zrb/builtin/llm/tool/api.py,sha256=bXFE7jihdhUscxJH8lu5imwlYH735AalbCyUTl28BaQ,826
|
13
13
|
zrb/builtin/llm/tool/cli.py,sha256=to_IjkfrMGs6eLfG0cpVN9oyADWYsJQCtyluUhUdBww,253
|
14
|
-
zrb/builtin/llm/tool/file.py,sha256=
|
14
|
+
zrb/builtin/llm/tool/file.py,sha256=YkJ5RGwsqlv3ZxAcQDKqjlcOdmHYRJlZ6M9P49uMJEY,4792
|
15
15
|
zrb/builtin/llm/tool/rag.py,sha256=vEIThEy0JGwXEiNRLOEJAHAE0l1Qie2qvU3ryioeYMk,6066
|
16
16
|
zrb/builtin/llm/tool/web.py,sha256=SDnCtYHZ0Q4DtLbIhc11a0UyyKbTTeW60UfeIKzK35k,3204
|
17
17
|
zrb/builtin/md5.py,sha256=0pNlrfZA0wlZlHvFHLgyqN0JZJWGKQIF5oXxO44_OJk,949
|
@@ -208,7 +208,7 @@ zrb/callback/callback.py,sha256=hKefB_Jd1XGjPSLQdMKDsGLHPzEGO2dqrIArLl_EmD0,848
|
|
208
208
|
zrb/cmd/__init__.py,sha256=47DEQpj8HBSa-_TImW-5JCeuQeRkm5NMpJWZG3hSuFU,0
|
209
209
|
zrb/cmd/cmd_result.py,sha256=L8bQJzWCpcYexIxHBNsXj2pT3BtLmWex0iJSMkvimOA,597
|
210
210
|
zrb/cmd/cmd_val.py,sha256=7Doowyg6BK3ISSGBLt-PmlhzaEkBjWWm51cED6fAUOQ,1014
|
211
|
-
zrb/config.py,sha256=
|
211
|
+
zrb/config.py,sha256=YBbpjH4Wnb8yQz0-982RCnbrdefdQy8SNZnJZJsfDvk,3985
|
212
212
|
zrb/content_transformer/__init__.py,sha256=47DEQpj8HBSa-_TImW-5JCeuQeRkm5NMpJWZG3hSuFU,0
|
213
213
|
zrb/content_transformer/any_content_transformer.py,sha256=v8ZUbcix1GGeDQwB6OKX_1TjpY__ksxWVeqibwa_iZA,850
|
214
214
|
zrb/content_transformer/content_transformer.py,sha256=STl77wW-I69QaGzCXjvkppngYFLufow8ybPLSyAvlHs,2404
|
@@ -237,9 +237,9 @@ zrb/input/option_input.py,sha256=TQB82ko5odgzkULEizBZi0e9TIHEbIgvdP0AR3RhA74,213
|
|
237
237
|
zrb/input/password_input.py,sha256=szBojWxSP9QJecgsgA87OIYwQrY2AQ3USIKdDZY6snU,1465
|
238
238
|
zrb/input/str_input.py,sha256=NevZHX9rf1g8eMatPyy-kUX3DglrVAQpzvVpKAzf7bA,81
|
239
239
|
zrb/input/text_input.py,sha256=shvVbc2U8Is36h23M5lcW8IEwKc9FR-4uEPZZroj3rU,3377
|
240
|
-
zrb/llm_config.py,sha256=
|
240
|
+
zrb/llm_config.py,sha256=zNr46IOm8lGQKSp9yzWLfa4KOx5Yn_7xFoReyk2Cp9Y,3328
|
241
241
|
zrb/runner/__init__.py,sha256=47DEQpj8HBSa-_TImW-5JCeuQeRkm5NMpJWZG3hSuFU,0
|
242
|
-
zrb/runner/cli.py,sha256=
|
242
|
+
zrb/runner/cli.py,sha256=0mT0oO_yEhc8N4nYCJNujhgLjVykZ0B-kAOFXyAvAqM,6672
|
243
243
|
zrb/runner/common_util.py,sha256=0zhZn1Jdmr194_nsL5_L-Kn9-_NDpMTI2z6_LXUQJ-U,1369
|
244
244
|
zrb/runner/web_app.py,sha256=Ji2AWeFpJu5guXmur7mAAbjMToyjgmPDdfYu8047FFI,2616
|
245
245
|
zrb/runner/web_config/config.py,sha256=0wR58KreAmawGGfamm0GLZY344HaXs7qfDgHLavBDwo,3125
|
@@ -300,7 +300,7 @@ zrb/task/base_task.py,sha256=SQRf37bylS586KwyW0eYDe9JZ5Hl18FP8kScHae6y3A,21251
|
|
300
300
|
zrb/task/base_trigger.py,sha256=jC722rDvodaBLeNaFghkTyv1u0QXrK6BLZUUqcmBJ7Q,4581
|
301
301
|
zrb/task/cmd_task.py,sha256=pUKRSR4DZKjbmluB6vi7cxqyhxOLfJ2czSpYeQbiDvo,10705
|
302
302
|
zrb/task/http_check.py,sha256=Gf5rOB2Se2EdizuN9rp65HpGmfZkGc-clIAlHmPVehs,2565
|
303
|
-
zrb/task/llm_task.py,sha256=
|
303
|
+
zrb/task/llm_task.py,sha256=m8B0dXsRZOo5h0FPEm3KJTEj378iAVpwxMMUfbZwTW0,13578
|
304
304
|
zrb/task/make_task.py,sha256=PD3b_aYazthS8LHeJsLAhwKDEgdurQZpymJDKeN60u0,2265
|
305
305
|
zrb/task/rsync_task.py,sha256=GSL9144bmp6F0EckT6m-2a1xG25AzrrWYzH4k3SVUKM,6370
|
306
306
|
zrb/task/scaffolder.py,sha256=rME18w1HJUHXgi9eTYXx_T2G4JdqDYzBoNOkdOOo5-o,6806
|
@@ -332,7 +332,7 @@ zrb/util/file.py,sha256=mgNobIKCr0eIQUlg6W2Yg1fvg943VyuOUF8WMFpJA5A,859
|
|
332
332
|
zrb/util/git.py,sha256=TShnMxPAk20Tglp25d_XPVZX-q0mvKeqdprVMeXQ5f0,4787
|
333
333
|
zrb/util/git_subtree.py,sha256=zyWl0aUEZJyUJKjfw1uglozB4R1kF9pWtfKjhu8DN44,2658
|
334
334
|
zrb/util/group.py,sha256=Bg7HrSycoK110U5s_Tca6-uUQuZ5CMgb8wxZSrvDQ98,2790
|
335
|
-
zrb/util/load.py,sha256=
|
335
|
+
zrb/util/load.py,sha256=Aeyh1EWtp-oJGVAhcjZn-VSB9innoOe8ZkUawha_ddk,1339
|
336
336
|
zrb/util/run.py,sha256=DGHUP9x1Q8V8UF3FbpmjLGuhVVCCLfjTH2teT8qXlNI,207
|
337
337
|
zrb/util/string/__init__.py,sha256=47DEQpj8HBSa-_TImW-5JCeuQeRkm5NMpJWZG3hSuFU,0
|
338
338
|
zrb/util/string/conversion.py,sha256=636sfF1a3_bpzXNw5bdSzbJzwakyAoo70KT3_ItgZEo,4333
|
@@ -341,7 +341,7 @@ zrb/util/string/name.py,sha256=8picJfUBXNpdh64GNaHv3om23QHhUZux7DguFLrXHp8,1163
|
|
341
341
|
zrb/util/todo.py,sha256=1nDdwPc22oFoK_1ZTXyf3638Bg6sqE2yp_U4_-frHoc,16015
|
342
342
|
zrb/xcom/__init__.py,sha256=47DEQpj8HBSa-_TImW-5JCeuQeRkm5NMpJWZG3hSuFU,0
|
343
343
|
zrb/xcom/xcom.py,sha256=o79rxR9wphnShrcIushA0Qt71d_p3ZTxjNf7x9hJB78,1571
|
344
|
-
zrb-1.4.
|
345
|
-
zrb-1.4.
|
346
|
-
zrb-1.4.
|
347
|
-
zrb-1.4.
|
344
|
+
zrb-1.4.2.dist-info/METADATA,sha256=P5W8EKRiKGR4QNCpW0dtC-NJEXxoMfuTz9uHCwgY2WU,8096
|
345
|
+
zrb-1.4.2.dist-info/WHEEL,sha256=sP946D7jFCHeNz5Iq4fL4Lu-PrWrFsgfLXbbkciIZwg,88
|
346
|
+
zrb-1.4.2.dist-info/entry_points.txt,sha256=-Pg3ElWPfnaSM-XvXqCxEAa-wfVI6BEgcs386s8C8v8,46
|
347
|
+
zrb-1.4.2.dist-info/RECORD,,
|
File without changes
|
File without changes
|