tccli 3.0.1171.1__py2.py3-none-any.whl → 3.0.1172.1__py2.py3-none-any.whl
This diff represents the content of publicly available package versions that have been released to one of the supported registries. The information contained in this diff is provided for informational purposes only and reflects changes between package versions as they appear in their respective public registries.
- tccli/__init__.py +1 -1
- tccli/services/billing/v20180709/api.json +125 -5
- tccli/services/es/v20180416/api.json +89 -2
- tccli/services/ess/ess_client.py +53 -0
- tccli/services/ess/v20201111/api.json +101 -0
- tccli/services/ess/v20201111/examples.json +8 -0
- tccli/services/essbasic/essbasic_client.py +67 -14
- tccli/services/essbasic/v20210526/api.json +92 -0
- tccli/services/essbasic/v20210526/examples.json +8 -0
- tccli/services/faceid/v20180301/api.json +2 -2
- tccli/services/mongodb/v20190725/api.json +57 -57
- tccli/services/mongodb/v20190725/examples.json +2 -2
- tccli/services/ms/v20180408/api.json +46 -46
- tccli/services/ocr/v20181119/api.json +1 -1
- tccli/services/organization/organization_client.py +2837 -293
- tccli/services/organization/v20210331/api.json +7182 -1735
- tccli/services/organization/v20210331/examples.json +384 -0
- tccli/services/tat/tat_client.py +53 -0
- tccli/services/tat/v20201028/api.json +149 -5
- tccli/services/tat/v20201028/examples.json +8 -0
- tccli/services/trocket/v20230308/api.json +65 -12
- {tccli-3.0.1171.1.dist-info → tccli-3.0.1172.1.dist-info}/METADATA +2 -2
- {tccli-3.0.1171.1.dist-info → tccli-3.0.1172.1.dist-info}/RECORD +26 -26
- {tccli-3.0.1171.1.dist-info → tccli-3.0.1172.1.dist-info}/WHEEL +0 -0
- {tccli-3.0.1171.1.dist-info → tccli-3.0.1172.1.dist-info}/entry_points.txt +0 -0
- {tccli-3.0.1171.1.dist-info → tccli-3.0.1172.1.dist-info}/license_files/LICENSE +0 -0
@@ -2723,6 +2723,58 @@ def doDescribeChannelOrganizations(args, parsed_globals):
|
|
2723
2723
|
FormatOutput.output("action", json_obj, g_param[OptionsDefine.Output], g_param[OptionsDefine.Filter])
|
2724
2724
|
|
2725
2725
|
|
2726
|
+
def doCreateBatchInitOrganizationUrl(args, parsed_globals):
|
2727
|
+
g_param = parse_global_arg(parsed_globals)
|
2728
|
+
|
2729
|
+
if g_param[OptionsDefine.UseCVMRole.replace('-', '_')]:
|
2730
|
+
cred = credential.CVMRoleCredential()
|
2731
|
+
elif g_param[OptionsDefine.RoleArn.replace('-', '_')] and g_param[OptionsDefine.RoleSessionName.replace('-', '_')]:
|
2732
|
+
cred = credential.STSAssumeRoleCredential(
|
2733
|
+
g_param[OptionsDefine.SecretId], g_param[OptionsDefine.SecretKey], g_param[OptionsDefine.RoleArn.replace('-', '_')],
|
2734
|
+
g_param[OptionsDefine.RoleSessionName.replace('-', '_')], endpoint=g_param["sts_cred_endpoint"]
|
2735
|
+
)
|
2736
|
+
elif os.getenv(OptionsDefine.ENV_TKE_REGION) and os.getenv(OptionsDefine.ENV_TKE_PROVIDER_ID) and os.getenv(OptionsDefine.ENV_TKE_WEB_IDENTITY_TOKEN_FILE) and os.getenv(OptionsDefine.ENV_TKE_ROLE_ARN):
|
2737
|
+
cred = credential.DefaultTkeOIDCRoleArnProvider().get_credentials()
|
2738
|
+
else:
|
2739
|
+
cred = credential.Credential(
|
2740
|
+
g_param[OptionsDefine.SecretId], g_param[OptionsDefine.SecretKey], g_param[OptionsDefine.Token]
|
2741
|
+
)
|
2742
|
+
http_profile = HttpProfile(
|
2743
|
+
reqTimeout=60 if g_param[OptionsDefine.Timeout] is None else int(g_param[OptionsDefine.Timeout]),
|
2744
|
+
reqMethod="POST",
|
2745
|
+
endpoint=g_param[OptionsDefine.Endpoint],
|
2746
|
+
proxy=g_param[OptionsDefine.HttpsProxy.replace('-', '_')]
|
2747
|
+
)
|
2748
|
+
profile = ClientProfile(httpProfile=http_profile, signMethod="HmacSHA256")
|
2749
|
+
if g_param[OptionsDefine.Language]:
|
2750
|
+
profile.language = g_param[OptionsDefine.Language]
|
2751
|
+
mod = CLIENT_MAP[g_param[OptionsDefine.Version]]
|
2752
|
+
client = mod.EssbasicClient(cred, g_param[OptionsDefine.Region], profile)
|
2753
|
+
client._sdkVersion += ("_CLI_" + __version__)
|
2754
|
+
models = MODELS_MAP[g_param[OptionsDefine.Version]]
|
2755
|
+
model = models.CreateBatchInitOrganizationUrlRequest()
|
2756
|
+
model.from_json_string(json.dumps(args))
|
2757
|
+
start_time = time.time()
|
2758
|
+
while True:
|
2759
|
+
rsp = client.CreateBatchInitOrganizationUrl(model)
|
2760
|
+
result = rsp.to_json_string()
|
2761
|
+
try:
|
2762
|
+
json_obj = json.loads(result)
|
2763
|
+
except TypeError as e:
|
2764
|
+
json_obj = json.loads(result.decode('utf-8')) # python3.3
|
2765
|
+
if not g_param[OptionsDefine.Waiter] or search(g_param['OptionsDefine.WaiterInfo']['expr'], json_obj) == g_param['OptionsDefine.WaiterInfo']['to']:
|
2766
|
+
break
|
2767
|
+
cur_time = time.time()
|
2768
|
+
if cur_time - start_time >= g_param['OptionsDefine.WaiterInfo']['timeout']:
|
2769
|
+
raise ClientError('Request timeout, wait `%s` to `%s` timeout, last request is %s' %
|
2770
|
+
(g_param['OptionsDefine.WaiterInfo']['expr'], g_param['OptionsDefine.WaiterInfo']['to'],
|
2771
|
+
search(g_param['OptionsDefine.WaiterInfo']['expr'], json_obj)))
|
2772
|
+
else:
|
2773
|
+
print('Inquiry result is %s.' % search(g_param['OptionsDefine.WaiterInfo']['expr'], json_obj))
|
2774
|
+
time.sleep(g_param['OptionsDefine.WaiterInfo']['interval'])
|
2775
|
+
FormatOutput.output("action", json_obj, g_param[OptionsDefine.Output], g_param[OptionsDefine.Filter])
|
2776
|
+
|
2777
|
+
|
2726
2778
|
def doSendFlow(args, parsed_globals):
|
2727
2779
|
g_param = parse_global_arg(parsed_globals)
|
2728
2780
|
|
@@ -4231,7 +4283,7 @@ def doDescribeCustomFlowIdsByFlowId(args, parsed_globals):
|
|
4231
4283
|
FormatOutput.output("action", json_obj, g_param[OptionsDefine.Output], g_param[OptionsDefine.Filter])
|
4232
4284
|
|
4233
4285
|
|
4234
|
-
def
|
4286
|
+
def doDescribeFaceIdResults(args, parsed_globals):
|
4235
4287
|
g_param = parse_global_arg(parsed_globals)
|
4236
4288
|
|
4237
4289
|
if g_param[OptionsDefine.UseCVMRole.replace('-', '_')]:
|
@@ -4260,11 +4312,11 @@ def doCreateH5FaceIdUrl(args, parsed_globals):
|
|
4260
4312
|
client = mod.EssbasicClient(cred, g_param[OptionsDefine.Region], profile)
|
4261
4313
|
client._sdkVersion += ("_CLI_" + __version__)
|
4262
4314
|
models = MODELS_MAP[g_param[OptionsDefine.Version]]
|
4263
|
-
model = models.
|
4315
|
+
model = models.DescribeFaceIdResultsRequest()
|
4264
4316
|
model.from_json_string(json.dumps(args))
|
4265
4317
|
start_time = time.time()
|
4266
4318
|
while True:
|
4267
|
-
rsp = client.
|
4319
|
+
rsp = client.DescribeFaceIdResults(model)
|
4268
4320
|
result = rsp.to_json_string()
|
4269
4321
|
try:
|
4270
4322
|
json_obj = json.loads(result)
|
@@ -4283,7 +4335,7 @@ def doCreateH5FaceIdUrl(args, parsed_globals):
|
|
4283
4335
|
FormatOutput.output("action", json_obj, g_param[OptionsDefine.Output], g_param[OptionsDefine.Filter])
|
4284
4336
|
|
4285
4337
|
|
4286
|
-
def
|
4338
|
+
def doCreateChannelOrganizationInfoChangeUrl(args, parsed_globals):
|
4287
4339
|
g_param = parse_global_arg(parsed_globals)
|
4288
4340
|
|
4289
4341
|
if g_param[OptionsDefine.UseCVMRole.replace('-', '_')]:
|
@@ -4312,11 +4364,11 @@ def doDescribeFaceIdResults(args, parsed_globals):
|
|
4312
4364
|
client = mod.EssbasicClient(cred, g_param[OptionsDefine.Region], profile)
|
4313
4365
|
client._sdkVersion += ("_CLI_" + __version__)
|
4314
4366
|
models = MODELS_MAP[g_param[OptionsDefine.Version]]
|
4315
|
-
model = models.
|
4367
|
+
model = models.CreateChannelOrganizationInfoChangeUrlRequest()
|
4316
4368
|
model.from_json_string(json.dumps(args))
|
4317
4369
|
start_time = time.time()
|
4318
4370
|
while True:
|
4319
|
-
rsp = client.
|
4371
|
+
rsp = client.CreateChannelOrganizationInfoChangeUrl(model)
|
4320
4372
|
result = rsp.to_json_string()
|
4321
4373
|
try:
|
4322
4374
|
json_obj = json.loads(result)
|
@@ -4335,7 +4387,7 @@ def doDescribeFaceIdResults(args, parsed_globals):
|
|
4335
4387
|
FormatOutput.output("action", json_obj, g_param[OptionsDefine.Output], g_param[OptionsDefine.Filter])
|
4336
4388
|
|
4337
4389
|
|
4338
|
-
def
|
4390
|
+
def doDescribeUsers(args, parsed_globals):
|
4339
4391
|
g_param = parse_global_arg(parsed_globals)
|
4340
4392
|
|
4341
4393
|
if g_param[OptionsDefine.UseCVMRole.replace('-', '_')]:
|
@@ -4364,11 +4416,11 @@ def doCreateChannelOrganizationInfoChangeUrl(args, parsed_globals):
|
|
4364
4416
|
client = mod.EssbasicClient(cred, g_param[OptionsDefine.Region], profile)
|
4365
4417
|
client._sdkVersion += ("_CLI_" + __version__)
|
4366
4418
|
models = MODELS_MAP[g_param[OptionsDefine.Version]]
|
4367
|
-
model = models.
|
4419
|
+
model = models.DescribeUsersRequest()
|
4368
4420
|
model.from_json_string(json.dumps(args))
|
4369
4421
|
start_time = time.time()
|
4370
4422
|
while True:
|
4371
|
-
rsp = client.
|
4423
|
+
rsp = client.DescribeUsers(model)
|
4372
4424
|
result = rsp.to_json_string()
|
4373
4425
|
try:
|
4374
4426
|
json_obj = json.loads(result)
|
@@ -4751,7 +4803,7 @@ def doCancelFlow(args, parsed_globals):
|
|
4751
4803
|
FormatOutput.output("action", json_obj, g_param[OptionsDefine.Output], g_param[OptionsDefine.Filter])
|
4752
4804
|
|
4753
4805
|
|
4754
|
-
def
|
4806
|
+
def doCreateH5FaceIdUrl(args, parsed_globals):
|
4755
4807
|
g_param = parse_global_arg(parsed_globals)
|
4756
4808
|
|
4757
4809
|
if g_param[OptionsDefine.UseCVMRole.replace('-', '_')]:
|
@@ -4780,11 +4832,11 @@ def doDescribeUsers(args, parsed_globals):
|
|
4780
4832
|
client = mod.EssbasicClient(cred, g_param[OptionsDefine.Region], profile)
|
4781
4833
|
client._sdkVersion += ("_CLI_" + __version__)
|
4782
4834
|
models = MODELS_MAP[g_param[OptionsDefine.Version]]
|
4783
|
-
model = models.
|
4835
|
+
model = models.CreateH5FaceIdUrlRequest()
|
4784
4836
|
model.from_json_string(json.dumps(args))
|
4785
4837
|
start_time = time.time()
|
4786
4838
|
while True:
|
4787
|
-
rsp = client.
|
4839
|
+
rsp = client.CreateH5FaceIdUrl(model)
|
4788
4840
|
result = rsp.to_json_string()
|
4789
4841
|
try:
|
4790
4842
|
json_obj = json.loads(result)
|
@@ -6740,6 +6792,7 @@ ACTION_MAP = {
|
|
6740
6792
|
"ChannelDescribeRoles": doChannelDescribeRoles,
|
6741
6793
|
"DescribeResourceUrlsByFlows": doDescribeResourceUrlsByFlows,
|
6742
6794
|
"DescribeChannelOrganizations": doDescribeChannelOrganizations,
|
6795
|
+
"CreateBatchInitOrganizationUrl": doCreateBatchInitOrganizationUrl,
|
6743
6796
|
"SendFlow": doSendFlow,
|
6744
6797
|
"DescribeFaceIdPhotos": doDescribeFaceIdPhotos,
|
6745
6798
|
"ChannelDescribeFlowComponents": doChannelDescribeFlowComponents,
|
@@ -6769,9 +6822,9 @@ ACTION_MAP = {
|
|
6769
6822
|
"VerifySubOrganization": doVerifySubOrganization,
|
6770
6823
|
"ArchiveFlow": doArchiveFlow,
|
6771
6824
|
"DescribeCustomFlowIdsByFlowId": doDescribeCustomFlowIdsByFlowId,
|
6772
|
-
"CreateH5FaceIdUrl": doCreateH5FaceIdUrl,
|
6773
6825
|
"DescribeFaceIdResults": doDescribeFaceIdResults,
|
6774
6826
|
"CreateChannelOrganizationInfoChangeUrl": doCreateChannelOrganizationInfoChangeUrl,
|
6827
|
+
"DescribeUsers": doDescribeUsers,
|
6775
6828
|
"CreateFaceIdSign": doCreateFaceIdSign,
|
6776
6829
|
"CheckVerifyCodeMatchFlowId": doCheckVerifyCodeMatchFlowId,
|
6777
6830
|
"ChannelCreateFlowGroupByFiles": doChannelCreateFlowGroupByFiles,
|
@@ -6779,7 +6832,7 @@ ACTION_MAP = {
|
|
6779
6832
|
"DescribeFlowDetailInfo": doDescribeFlowDetailInfo,
|
6780
6833
|
"ChannelDescribeBillUsageDetail": doChannelDescribeBillUsageDetail,
|
6781
6834
|
"CancelFlow": doCancelFlow,
|
6782
|
-
"
|
6835
|
+
"CreateH5FaceIdUrl": doCreateH5FaceIdUrl,
|
6783
6836
|
"DescribeFlowApprovers": doDescribeFlowApprovers,
|
6784
6837
|
"DeleteSeal": doDeleteSeal,
|
6785
6838
|
"ModifyFlowDeadline": doModifyFlowDeadline,
|
@@ -315,6 +315,13 @@
|
|
315
315
|
"output": "ChannelVerifyPdfResponse",
|
316
316
|
"status": "online"
|
317
317
|
},
|
318
|
+
"CreateBatchInitOrganizationUrl": {
|
319
|
+
"document": "支持企业进行批量初始化操作:\n\n此接口存在以下限制:\n1. 批量操作的企业需要已经完成电子签的认证流程。\n2. 通过此接口生成的链接在小程序端进行操作时,操作人需要是<font color=\"red\">所有企业的超管或法人</font>。\n3. 批量操作的企业,需要是本方第三方应用下的企业。\n4. <font color=\"red\">操作链接过期时间默认为生成链接后7天。</font>",
|
320
|
+
"input": "CreateBatchInitOrganizationUrlRequest",
|
321
|
+
"name": "批量操作企业初始化",
|
322
|
+
"output": "CreateBatchInitOrganizationUrlResponse",
|
323
|
+
"status": "online"
|
324
|
+
},
|
318
325
|
"CreateBatchOrganizationRegistrationTasks": {
|
319
326
|
"document": "该接口用于批量创建企业认证链接, 可以支持PC浏览器,H5和小程序三种途径。\n此接口为异步提交任务接口,需要与[查询子企业批量认证链接](https://qcloudimg.tencent-cloud.cn/raw/1d3737991b2a3be78002bd78a47d6917.png)配合使用,整体流程如下图。\n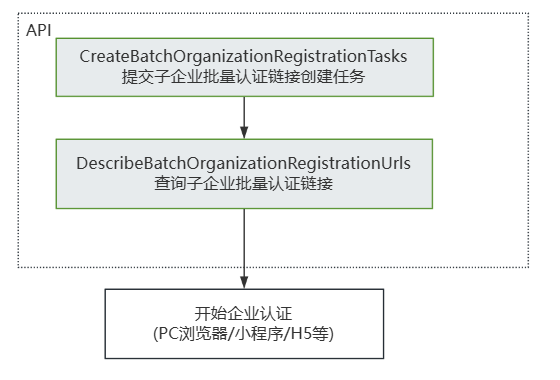\n\n\n\n**注意**\n\n1. 单次最多创建10个子企业。\n2. 一天内,同一家企业最多创建8000个子企业。\n3. 同一批创建的子客户不能重复,包括企业名称、企业统一信用代码和子客户经办人openId。\n4. 跳转到小程序的实现,请参考微信官方文档(分为<a href=\"https://developers.weixin.qq.com/miniprogram/dev/api/navigate/wx.navigateToMiniProgram.html\">全屏</a>、<a href=\"https://developers.weixin.qq.com/miniprogram/dev/framework/open-ability/openEmbeddedMiniProgram.html\">半屏</a>两种方式)。如何配置跳转电子签小程序,可参考:<a href=\"https://qian.tencent.com/developers/company/openwxminiprogram\">跳转电子签小程序配置</a>。\n\n\n\n**腾讯电子签小程序的AppID 和 原始Id如下:**\n\n| 小程序 | AppID | 原始ID |\n| ------------ | ------------ | ------------ |\n| 腾讯电子签(正式版) | wxa023b292fd19d41d | gh_da88f6188665 |\n| 腾讯电子签Demo | wx371151823f6f3edf | gh_39a5d3de69fa |",
|
320
327
|
"input": "CreateBatchOrganizationRegistrationTasksRequest",
|
@@ -5841,6 +5848,91 @@
|
|
5841
5848
|
],
|
5842
5849
|
"usage": "in"
|
5843
5850
|
},
|
5851
|
+
"CreateBatchInitOrganizationUrlRequest": {
|
5852
|
+
"document": "CreateBatchInitOrganizationUrl请求参数结构体",
|
5853
|
+
"members": [
|
5854
|
+
{
|
5855
|
+
"disabled": false,
|
5856
|
+
"document": "应用相关信息。 此接口Agent.AppId 必填。",
|
5857
|
+
"example": "无",
|
5858
|
+
"member": "Agent",
|
5859
|
+
"name": "Agent",
|
5860
|
+
"required": true,
|
5861
|
+
"type": "object"
|
5862
|
+
},
|
5863
|
+
{
|
5864
|
+
"disabled": false,
|
5865
|
+
"document": "初始化操作类型\n<ul><li>CREATE_SEAL : 创建印章</li>\n<li>OPEN_AUTO_SIGN :开通企业自动签署</li></ul>",
|
5866
|
+
"example": "无",
|
5867
|
+
"member": "string",
|
5868
|
+
"name": "OperateTypes",
|
5869
|
+
"required": true,
|
5870
|
+
"type": "list"
|
5871
|
+
},
|
5872
|
+
{
|
5873
|
+
"disabled": false,
|
5874
|
+
"document": "批量操作的企业列表在第三方平台的企业Id列表,即ProxyOrganizationOpenId列表,最大支持50个",
|
5875
|
+
"example": "无",
|
5876
|
+
"member": "string",
|
5877
|
+
"name": "ProxyOrganizationOpenIds",
|
5878
|
+
"required": true,
|
5879
|
+
"type": "list"
|
5880
|
+
}
|
5881
|
+
],
|
5882
|
+
"type": "object"
|
5883
|
+
},
|
5884
|
+
"CreateBatchInitOrganizationUrlResponse": {
|
5885
|
+
"document": "CreateBatchInitOrganizationUrl返回参数结构体",
|
5886
|
+
"members": [
|
5887
|
+
{
|
5888
|
+
"disabled": false,
|
5889
|
+
"document": "小程序路径",
|
5890
|
+
"example": "无",
|
5891
|
+
"member": "string",
|
5892
|
+
"name": "MiniAppPath",
|
5893
|
+
"output_required": true,
|
5894
|
+
"type": "string",
|
5895
|
+
"value_allowed_null": false
|
5896
|
+
},
|
5897
|
+
{
|
5898
|
+
"disabled": false,
|
5899
|
+
"document": "操作长链",
|
5900
|
+
"example": "无",
|
5901
|
+
"member": "string",
|
5902
|
+
"name": "OperateLongUrl",
|
5903
|
+
"output_required": true,
|
5904
|
+
"type": "string",
|
5905
|
+
"value_allowed_null": false
|
5906
|
+
},
|
5907
|
+
{
|
5908
|
+
"disabled": false,
|
5909
|
+
"document": "操作短链",
|
5910
|
+
"example": "无",
|
5911
|
+
"member": "string",
|
5912
|
+
"name": "OperateShortUrl",
|
5913
|
+
"output_required": true,
|
5914
|
+
"type": "string",
|
5915
|
+
"value_allowed_null": false
|
5916
|
+
},
|
5917
|
+
{
|
5918
|
+
"disabled": false,
|
5919
|
+
"document": "操作二维码",
|
5920
|
+
"example": "无",
|
5921
|
+
"member": "string",
|
5922
|
+
"name": "QRCodeUrl",
|
5923
|
+
"output_required": true,
|
5924
|
+
"type": "string",
|
5925
|
+
"value_allowed_null": false
|
5926
|
+
},
|
5927
|
+
{
|
5928
|
+
"document": "唯一请求 ID,由服务端生成,每次请求都会返回(若请求因其他原因未能抵达服务端,则该次请求不会获得 RequestId)。定位问题时需要提供该次请求的 RequestId。",
|
5929
|
+
"member": "string",
|
5930
|
+
"name": "RequestId",
|
5931
|
+
"type": "string"
|
5932
|
+
}
|
5933
|
+
],
|
5934
|
+
"type": "object"
|
5935
|
+
},
|
5844
5936
|
"CreateBatchOrganizationRegistrationTasksRequest": {
|
5845
5937
|
"document": "CreateBatchOrganizationRegistrationTasks请求参数结构体",
|
5846
5938
|
"members": [
|
@@ -672,6 +672,14 @@
|
|
672
672
|
"title": "验证不通过"
|
673
673
|
}
|
674
674
|
],
|
675
|
+
"CreateBatchInitOrganizationUrl": [
|
676
|
+
{
|
677
|
+
"document": "",
|
678
|
+
"input": "POST / HTTP/1.1\nHost: essbasic.tencentcloudapi.com\nContent-Type: application/json\nX-TC-Action: CreateBatchInitOrganizationUrl\n<公共请求参数>\n\n{\n \"Agent\": {\n \"ProxyOrganizationOpenId\": \"\",\n \"AppId\": \"yDKHHUUckpacc9ijUuXGmVcuzACNH5pF\"\n },\n \"ProxyOrganizationOpenIds\": [\n \"tencent-testorg-cd\",\n \"tencent-testorg-ca\",\n \"tencent-testorg-zhangy\",\n \"tencent-testorg-tianjing\",\n \"tencent-testorg-hongkong\"\n ],\n \"OperateTypes\": [\n \"OPEN_AUTO_SIGN\",\n \"CREATE_SEAL\"\n ]\n}",
|
679
|
+
"output": "{\n \"Response\": {\n \"MiniAppPath\": \"pages/guide/index?to=BATCH_INITIALIZATION_ORGANIZATIONS&shortKey=yDAHpURDfyLc5LgBhXb6\",\n \"OperateLongUrl\": \"https://res.ess.tencent.cn/cdn/h5-activity-dev/jump-mp.html?to=BATCH_INITIALIZATION_ORGANIZATIONS&shortKey=yDCHpURDfyLc5LgBhXb6\",\n \"OperateShortUrl\": \"https://essurl.cn/zahbUBHxW3\",\n \"QRCodeUrl\": \"https://dyn.ess.tencent.cn/imgs/qrcode_urls/batch_initialization_organizations/yDCHHUUckpacc9ijUuXGmVcuzZCNH5pF.png\",\n \"RequestId\": \"s1723520103899037060\"\n }\n}",
|
680
|
+
"title": "创建批量操作企业初始化链接"
|
681
|
+
}
|
682
|
+
],
|
675
683
|
"CreateBatchOrganizationRegistrationTasks": [
|
676
684
|
{
|
677
685
|
"document": "用户同时创建3个企业, 创建链接成功",
|
@@ -413,7 +413,7 @@
|
|
413
413
|
"members": [
|
414
414
|
{
|
415
415
|
"disabled": false,
|
416
|
-
"document": "认证结果码\n收费结果码:\n'0': '认证通过'\n'-1': '认证未通过'\n'-6': '持卡人信息有误'\n'-7': '未开通无卡支付'\n'-8': '此卡被没收'\n'-9': '无效卡号'\n'-10': '此卡无对应发卡行'\n'-11': '该卡未初始化或睡眠卡'\n'-12': '作弊卡、吞卡'\n'-13': '此卡已挂失'\n'-14': '该卡已过期'\n'-15': '受限制的卡'\n'-16': '密码错误次数超限'\n'-17': '发卡行不支持此交易'\n不收费结果码:\n'-2': '姓名校验不通过'\n'-3': '身份证号码有误'\n'-4': '银行卡号码有误'\n'-5': '手机号码不合法'\n'-18': '验证中心服务繁忙'\n'-19': '验证次数超限,请次日重试'",
|
416
|
+
"document": "认证结果码\n收费结果码:\n'0': '认证通过'\n'-1': '认证未通过'\n'-6': '持卡人信息有误'\n'-7': '未开通无卡支付'\n'-8': '此卡被没收'\n'-9': '无效卡号'\n'-10': '此卡无对应发卡行'\n'-11': '该卡未初始化或睡眠卡'\n'-12': '作弊卡、吞卡'\n'-13': '此卡已挂失'\n'-14': '该卡已过期'\n'-15': '受限制的卡'\n'-16': '密码错误次数超限'\n'-17': '发卡行不支持此交易'\n不收费结果码:\n'-2': '姓名校验不通过'\n'-3': '身份证号码有误'\n'-4': '银行卡号码有误'\n'-5': '手机号码不合法'\n'-18': '验证中心服务繁忙'\n'-19': '验证次数超限,请次日重试'\n'-20': '该证件号暂不支持核验,当前仅支持二代身份证'",
|
417
417
|
"example": "0",
|
418
418
|
"member": "string",
|
419
419
|
"name": "Result",
|
@@ -496,7 +496,7 @@
|
|
496
496
|
"members": [
|
497
497
|
{
|
498
498
|
"disabled": false,
|
499
|
-
"document": "认证结果码\n收费结果码:\n'0': '认证通过'\n'-1': '认证未通过'\n'-5': '持卡人信息有误'\n'-6': '未开通无卡支付'\n'-7': '此卡被没收'\n'-8': '无效卡号'\n'-9': '此卡无对应发卡行'\n'-10': '该卡未初始化或睡眠卡'\n'-11': '作弊卡、吞卡'\n'-12': '此卡已挂失'\n'-13': '该卡已过期'\n'-14': '受限制的卡'\n'-15': '密码错误次数超限'\n'-16': '发卡行不支持此交易'\n不收费结果码:\n'-2': '姓名校验不通过'\n'-3': '身份证号码有误'\n'-4': '银行卡号码有误'\n'-17': '验证中心服务繁忙'\n'-18': '验证次数超限,请次日重试'",
|
499
|
+
"document": "认证结果码\n收费结果码:\n'0': '认证通过'\n'-1': '认证未通过'\n'-5': '持卡人信息有误'\n'-6': '未开通无卡支付'\n'-7': '此卡被没收'\n'-8': '无效卡号'\n'-9': '此卡无对应发卡行'\n'-10': '该卡未初始化或睡眠卡'\n'-11': '作弊卡、吞卡'\n'-12': '此卡已挂失'\n'-13': '该卡已过期'\n'-14': '受限制的卡'\n'-15': '密码错误次数超限'\n'-16': '发卡行不支持此交易'\n不收费结果码:\n'-2': '姓名校验不通过'\n'-3': '身份证号码有误'\n'-4': '银行卡号码有误'\n'-17': '验证中心服务繁忙'\n'-18': '验证次数超限,请次日重试'\n'-19': '该证件号暂不支持核验,当前仅支持二代身份证'\t",
|
500
500
|
"example": "0",
|
501
501
|
"member": "string",
|
502
502
|
"name": "Result",
|