solana-agent 27.3.6__py3-none-any.whl → 27.3.8__py3-none-any.whl
This diff represents the content of publicly available package versions that have been released to one of the supported registries. The information contained in this diff is provided for informational purposes only and reflects changes between package versions as they appear in their respective public registries.
- solana_agent/__init__.py +1 -3
- solana_agent/adapters/mongodb_adapter.py +5 -2
- solana_agent/adapters/openai_adapter.py +32 -27
- solana_agent/adapters/pinecone_adapter.py +91 -63
- solana_agent/client/solana_agent.py +38 -23
- solana_agent/domains/agent.py +7 -13
- solana_agent/domains/routing.py +5 -5
- solana_agent/factories/agent_factory.py +49 -34
- solana_agent/interfaces/client/client.py +22 -13
- solana_agent/interfaces/plugins/plugins.py +2 -1
- solana_agent/interfaces/providers/data_storage.py +9 -2
- solana_agent/interfaces/providers/llm.py +26 -12
- solana_agent/interfaces/providers/memory.py +1 -1
- solana_agent/interfaces/providers/vector_storage.py +3 -9
- solana_agent/interfaces/services/agent.py +21 -6
- solana_agent/interfaces/services/knowledge_base.py +6 -8
- solana_agent/interfaces/services/query.py +16 -5
- solana_agent/interfaces/services/routing.py +0 -1
- solana_agent/plugins/manager.py +14 -9
- solana_agent/plugins/registry.py +13 -11
- solana_agent/plugins/tools/__init__.py +0 -5
- solana_agent/plugins/tools/auto_tool.py +1 -0
- solana_agent/repositories/memory.py +20 -22
- solana_agent/services/__init__.py +1 -1
- solana_agent/services/agent.py +119 -89
- solana_agent/services/knowledge_base.py +182 -131
- solana_agent/services/query.py +48 -24
- solana_agent/services/routing.py +30 -18
- {solana_agent-27.3.6.dist-info → solana_agent-27.3.8.dist-info}/METADATA +6 -5
- solana_agent-27.3.8.dist-info/RECORD +39 -0
- solana_agent-27.3.6.dist-info/RECORD +0 -39
- {solana_agent-27.3.6.dist-info → solana_agent-27.3.8.dist-info}/LICENSE +0 -0
- {solana_agent-27.3.6.dist-info → solana_agent-27.3.8.dist-info}/WHEEL +0 -0
solana_agent/services/query.py
CHANGED
@@ -5,10 +5,13 @@ This service orchestrates the processing of user queries, coordinating
|
|
5
5
|
other services to provide comprehensive responses while maintaining
|
6
6
|
clean separation of concerns.
|
7
7
|
"""
|
8
|
+
|
8
9
|
from typing import Any, AsyncGenerator, Dict, Literal, Optional, Union
|
9
10
|
|
10
11
|
from solana_agent.interfaces.services.query import QueryService as QueryServiceInterface
|
11
|
-
from solana_agent.interfaces.services.routing import
|
12
|
+
from solana_agent.interfaces.services.routing import (
|
13
|
+
RoutingService as RoutingServiceInterface,
|
14
|
+
)
|
12
15
|
from solana_agent.services.agent import AgentService
|
13
16
|
from solana_agent.services.routing import RoutingService
|
14
17
|
from solana_agent.services.knowledge_base import KnowledgeBaseService
|
@@ -44,11 +47,22 @@ class QueryService(QueryServiceInterface):
|
|
44
47
|
user_id: str,
|
45
48
|
query: Union[str, bytes],
|
46
49
|
output_format: Literal["text", "audio"] = "text",
|
47
|
-
audio_voice: Literal[
|
48
|
-
|
50
|
+
audio_voice: Literal[
|
51
|
+
"alloy",
|
52
|
+
"ash",
|
53
|
+
"ballad",
|
54
|
+
"coral",
|
55
|
+
"echo",
|
56
|
+
"fable",
|
57
|
+
"onyx",
|
58
|
+
"nova",
|
59
|
+
"sage",
|
60
|
+
"shimmer",
|
61
|
+
] = "nova",
|
49
62
|
audio_instructions: str = "You speak in a friendly and helpful manner.",
|
50
|
-
audio_output_format: Literal[
|
51
|
-
|
63
|
+
audio_output_format: Literal[
|
64
|
+
"mp3", "opus", "aac", "flac", "wav", "pcm"
|
65
|
+
] = "aac",
|
52
66
|
audio_input_format: Literal[
|
53
67
|
"flac", "mp3", "mp4", "mpeg", "mpga", "m4a", "ogg", "wav", "webm"
|
54
68
|
] = "mp4",
|
@@ -75,7 +89,11 @@ class QueryService(QueryServiceInterface):
|
|
75
89
|
# Handle audio input if provided
|
76
90
|
user_text = ""
|
77
91
|
if not isinstance(query, str):
|
78
|
-
async for
|
92
|
+
async for (
|
93
|
+
transcript
|
94
|
+
) in self.agent_service.llm_provider.transcribe_audio(
|
95
|
+
query, audio_input_format
|
96
|
+
):
|
79
97
|
user_text += transcript
|
80
98
|
else:
|
81
99
|
user_text = query
|
@@ -112,7 +130,7 @@ class QueryService(QueryServiceInterface):
|
|
112
130
|
query_text=user_text,
|
113
131
|
top_k=self.kb_results_count,
|
114
132
|
include_content=True,
|
115
|
-
include_metadata=False
|
133
|
+
include_metadata=False,
|
116
134
|
)
|
117
135
|
|
118
136
|
if kb_results:
|
@@ -182,7 +200,7 @@ class QueryService(QueryServiceInterface):
|
|
182
200
|
await self._store_conversation(
|
183
201
|
user_id=user_id,
|
184
202
|
user_message=user_text,
|
185
|
-
assistant_message=full_text_response
|
203
|
+
assistant_message=full_text_response,
|
186
204
|
)
|
187
205
|
|
188
206
|
except Exception as e:
|
@@ -191,7 +209,7 @@ class QueryService(QueryServiceInterface):
|
|
191
209
|
async for chunk in self.agent_service.llm_provider.tts(
|
192
210
|
text=error_msg,
|
193
211
|
voice=audio_voice,
|
194
|
-
response_format=audio_output_format
|
212
|
+
response_format=audio_output_format,
|
195
213
|
):
|
196
214
|
yield chunk
|
197
215
|
else:
|
@@ -199,6 +217,7 @@ class QueryService(QueryServiceInterface):
|
|
199
217
|
|
200
218
|
print(f"Error in query processing: {str(e)}")
|
201
219
|
import traceback
|
220
|
+
|
202
221
|
print(traceback.format_exc())
|
203
222
|
|
204
223
|
async def delete_user_history(self, user_id: str) -> None:
|
@@ -218,7 +237,7 @@ class QueryService(QueryServiceInterface):
|
|
218
237
|
user_id: str,
|
219
238
|
page_num: int = 1,
|
220
239
|
page_size: int = 20,
|
221
|
-
sort_order: str = "desc" # "asc" for oldest-first, "desc" for newest-first
|
240
|
+
sort_order: str = "desc", # "asc" for oldest-first, "desc" for newest-first
|
222
241
|
) -> Dict[str, Any]:
|
223
242
|
"""Get paginated message history for a user.
|
224
243
|
|
@@ -246,7 +265,7 @@ class QueryService(QueryServiceInterface):
|
|
246
265
|
"page": page_num,
|
247
266
|
"page_size": page_size,
|
248
267
|
"total_pages": 0,
|
249
|
-
"error": "Memory provider not available"
|
268
|
+
"error": "Memory provider not available",
|
250
269
|
}
|
251
270
|
|
252
271
|
try:
|
@@ -255,8 +274,7 @@ class QueryService(QueryServiceInterface):
|
|
255
274
|
|
256
275
|
# Get total count of documents
|
257
276
|
total = self.memory_provider.count_documents(
|
258
|
-
collection="conversations",
|
259
|
-
query={"user_id": user_id}
|
277
|
+
collection="conversations", query={"user_id": user_id}
|
260
278
|
)
|
261
279
|
|
262
280
|
# Calculate total pages
|
@@ -268,22 +286,27 @@ class QueryService(QueryServiceInterface):
|
|
268
286
|
query={"user_id": user_id},
|
269
287
|
sort=[("timestamp", 1 if sort_order == "asc" else -1)],
|
270
288
|
skip=skip,
|
271
|
-
limit=page_size
|
289
|
+
limit=page_size,
|
272
290
|
)
|
273
291
|
|
274
292
|
# Format the results
|
275
293
|
formatted_conversations = []
|
276
294
|
for conv in conversations:
|
277
295
|
# Convert datetime to Unix timestamp (seconds since epoch)
|
278
|
-
timestamp =
|
279
|
-
|
296
|
+
timestamp = (
|
297
|
+
int(conv.get("timestamp").timestamp())
|
298
|
+
if conv.get("timestamp")
|
299
|
+
else None
|
300
|
+
)
|
280
301
|
|
281
|
-
formatted_conversations.append(
|
282
|
-
|
283
|
-
|
284
|
-
|
285
|
-
|
286
|
-
|
302
|
+
formatted_conversations.append(
|
303
|
+
{
|
304
|
+
"id": str(conv.get("_id")),
|
305
|
+
"user_message": conv.get("user_message"),
|
306
|
+
"assistant_message": conv.get("assistant_message"),
|
307
|
+
"timestamp": timestamp,
|
308
|
+
}
|
309
|
+
)
|
287
310
|
|
288
311
|
return {
|
289
312
|
"data": formatted_conversations,
|
@@ -291,12 +314,13 @@ class QueryService(QueryServiceInterface):
|
|
291
314
|
"page": page_num,
|
292
315
|
"page_size": page_size,
|
293
316
|
"total_pages": total_pages,
|
294
|
-
"error": None
|
317
|
+
"error": None,
|
295
318
|
}
|
296
319
|
|
297
320
|
except Exception as e:
|
298
321
|
print(f"Error retrieving user history: {str(e)}")
|
299
322
|
import traceback
|
323
|
+
|
300
324
|
print(traceback.format_exc())
|
301
325
|
return {
|
302
326
|
"data": [],
|
@@ -304,7 +328,7 @@ class QueryService(QueryServiceInterface):
|
|
304
328
|
"page": page_num,
|
305
329
|
"page_size": page_size,
|
306
330
|
"total_pages": 0,
|
307
|
-
"error": f"Error retrieving history: {str(e)}"
|
331
|
+
"error": f"Error retrieving history: {str(e)}",
|
308
332
|
}
|
309
333
|
|
310
334
|
async def _store_conversation(
|
solana_agent/services/routing.py
CHANGED
@@ -4,8 +4,11 @@ Routing service implementation.
|
|
4
4
|
This service manages query routing to appropriate agents based on
|
5
5
|
specializations and query analysis.
|
6
6
|
"""
|
7
|
+
|
7
8
|
from typing import Dict, List, Optional, Any
|
8
|
-
from solana_agent.interfaces.services.routing import
|
9
|
+
from solana_agent.interfaces.services.routing import (
|
10
|
+
RoutingService as RoutingServiceInterface,
|
11
|
+
)
|
9
12
|
from solana_agent.interfaces.services.agent import AgentService
|
10
13
|
from solana_agent.interfaces.providers.llm import LLMProvider
|
11
14
|
from solana_agent.domains.routing import QueryAnalysis
|
@@ -48,15 +51,19 @@ class RoutingService(RoutingServiceInterface):
|
|
48
51
|
available_specializations = []
|
49
52
|
|
50
53
|
for agent_id, agent in agents.items():
|
51
|
-
available_specializations.append(
|
52
|
-
|
53
|
-
|
54
|
-
|
54
|
+
available_specializations.append(
|
55
|
+
{
|
56
|
+
"agent_name": agent_id,
|
57
|
+
"specialization": agent.specialization,
|
58
|
+
}
|
59
|
+
)
|
55
60
|
|
56
|
-
specializations_text = "\n".join(
|
57
|
-
|
58
|
-
|
59
|
-
|
61
|
+
specializations_text = "\n".join(
|
62
|
+
[
|
63
|
+
f"- {spec['agent_name']}: {spec['specialization']}"
|
64
|
+
for spec in available_specializations
|
65
|
+
]
|
66
|
+
)
|
60
67
|
|
61
68
|
prompt = f"""
|
62
69
|
Analyze this user query and determine which agent would be best suited to answer it.
|
@@ -91,17 +98,19 @@ class RoutingService(RoutingServiceInterface):
|
|
91
98
|
"secondary_specializations": analysis.secondary_specializations,
|
92
99
|
"complexity_level": analysis.complexity_level,
|
93
100
|
"topics": analysis.topics,
|
94
|
-
"confidence": analysis.confidence
|
101
|
+
"confidence": analysis.confidence,
|
95
102
|
}
|
96
103
|
except Exception as e:
|
97
104
|
print(f"Error analyzing query: {e}")
|
98
105
|
# Return default analysis on error
|
99
106
|
return {
|
100
|
-
"primary_specialization": list(agents.keys())[0]
|
107
|
+
"primary_specialization": list(agents.keys())[0]
|
108
|
+
if agents
|
109
|
+
else "general",
|
101
110
|
"secondary_specializations": [],
|
102
111
|
"complexity_level": 1,
|
103
112
|
"topics": [],
|
104
|
-
"confidence": 0.0
|
113
|
+
"confidence": 0.0,
|
105
114
|
}
|
106
115
|
|
107
116
|
async def route_query(self, query: str) -> str: # pragma: no cover
|
@@ -124,8 +133,7 @@ class RoutingService(RoutingServiceInterface):
|
|
124
133
|
|
125
134
|
# Find best agent based on analysis
|
126
135
|
best_agent = await self._find_best_ai_agent(
|
127
|
-
analysis["primary_specialization"],
|
128
|
-
analysis["secondary_specializations"]
|
136
|
+
analysis["primary_specialization"], analysis["secondary_specializations"]
|
129
137
|
)
|
130
138
|
|
131
139
|
# Return best agent
|
@@ -161,8 +169,10 @@ class RoutingService(RoutingServiceInterface):
|
|
161
169
|
score = 0
|
162
170
|
|
163
171
|
# Check for specialization match
|
164
|
-
if
|
165
|
-
|
172
|
+
if (
|
173
|
+
agent.specialization.lower() in primary_specialization.lower()
|
174
|
+
or primary_specialization.lower() in agent.specialization.lower()
|
175
|
+
):
|
166
176
|
score += 10
|
167
177
|
|
168
178
|
# Check secondary specializations
|
@@ -170,8 +180,10 @@ class RoutingService(RoutingServiceInterface):
|
|
170
180
|
if sec_spec in ai_agents: # Direct agent name match
|
171
181
|
if sec_spec == agent_id:
|
172
182
|
score += 5
|
173
|
-
elif
|
174
|
-
|
183
|
+
elif (
|
184
|
+
agent.specialization.lower() in sec_spec.lower()
|
185
|
+
or sec_spec.lower() in agent.specialization.lower()
|
186
|
+
):
|
175
187
|
score += 3
|
176
188
|
|
177
189
|
agent_scores.append((agent_id, score))
|
@@ -1,7 +1,7 @@
|
|
1
1
|
Metadata-Version: 2.3
|
2
2
|
Name: solana-agent
|
3
|
-
Version: 27.3.
|
4
|
-
Summary:
|
3
|
+
Version: 27.3.8
|
4
|
+
Summary: AI Agents for Solana
|
5
5
|
License: MIT
|
6
6
|
Keywords: ai,openai,ai agents,agi
|
7
7
|
Author: Bevan Hunt
|
@@ -36,13 +36,14 @@ Description-Content-Type: text/markdown
|
|
36
36
|
[](https://codecov.io/gh/truemagic-coder/solana-agent)
|
37
37
|
[](https://github.com/truemagic-coder/solana-agent/actions/workflows/ci.yml)
|
38
38
|
[](https://github.com/truemagic-coder/solana-agent)
|
39
|
+
[](https://github.com/astral-sh/ruff)
|
39
40
|
[](https://libraries.io/pypi/solana-agent)
|
40
41
|
|
41
42
|
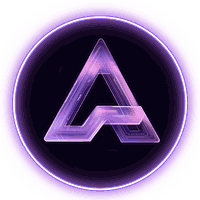
|
42
43
|
|
43
|
-
##
|
44
|
+
## AI Agents for Solana
|
44
45
|
|
45
|
-
Build your AI
|
46
|
+
Build your AI agents in three lines of code!
|
46
47
|
|
47
48
|
## Why?
|
48
49
|
* Three lines of code setup
|
@@ -56,7 +57,7 @@ Build your AI business in three lines of code!
|
|
56
57
|
* Business Alignment
|
57
58
|
* Extensible Tooling
|
58
59
|
* Simple Business Definition
|
59
|
-
* Knowledge Base
|
60
|
+
* Knowledge Base
|
60
61
|
* MCP Support
|
61
62
|
* Tested & Secure
|
62
63
|
* Built in Python
|
@@ -0,0 +1,39 @@
|
|
1
|
+
solana_agent/__init__.py,sha256=s_Dq2yqss83jp9DnHgXsxjpf9HWGRmRW2EwqroH0XKk,895
|
2
|
+
solana_agent/adapters/__init__.py,sha256=tiEEuuy0NF3ngc_tGEcRTt71zVI58v3dYY9RvMrF2Cg,204
|
3
|
+
solana_agent/adapters/mongodb_adapter.py,sha256=0KWIa6kaFbUFvtKUzuV_0p0RFlPPGKrDVIEU2McVY3k,2734
|
4
|
+
solana_agent/adapters/openai_adapter.py,sha256=NZ35mJ80yVWTbdQOAYUh7hDzOFclgfdeJ1Z8v_gfQG8,10922
|
5
|
+
solana_agent/adapters/pinecone_adapter.py,sha256=SDbf_XJMuFDKhNfF25_VXaYG3vrmYyPIo2SyhaniEwg,23048
|
6
|
+
solana_agent/client/__init__.py,sha256=47DEQpj8HBSa-_TImW-5JCeuQeRkm5NMpJWZG3hSuFU,0
|
7
|
+
solana_agent/client/solana_agent.py,sha256=jUGWxYJL9ZWxGsVX9C6FrRQyX7r6Cep0ijcfm7cbkJI,10098
|
8
|
+
solana_agent/domains/__init__.py,sha256=HiC94wVPRy-QDJSSRywCRrhrFfTBeHjfi5z-QfZv46U,168
|
9
|
+
solana_agent/domains/agent.py,sha256=3Q1wg4eIul0CPpaYBOjEthKTfcdhf1SAiWc2R-IMGO8,2561
|
10
|
+
solana_agent/domains/routing.py,sha256=1yR4IswGcmREGgbOOI6TKCfuM7gYGOhQjLkBqnZ-rNo,582
|
11
|
+
solana_agent/factories/__init__.py,sha256=47DEQpj8HBSa-_TImW-5JCeuQeRkm5NMpJWZG3hSuFU,0
|
12
|
+
solana_agent/factories/agent_factory.py,sha256=70f8QhV9bqCg2Fr3_iEejvDAAjpazOemsvI9H27jIiE,11687
|
13
|
+
solana_agent/interfaces/__init__.py,sha256=IQs1WIM1FeKP1-kY2FEfyhol_dB-I-VAe2rD6jrVF6k,355
|
14
|
+
solana_agent/interfaces/client/client.py,sha256=hsvaQiQdz3MLMNc77oD6ocvvnyl7Ez2n087ptFDA19M,3687
|
15
|
+
solana_agent/interfaces/plugins/plugins.py,sha256=Rz52cWBLdotwf4kV-2mC79tRYlN29zHSu1z9-y1HVPk,3329
|
16
|
+
solana_agent/interfaces/providers/data_storage.py,sha256=Y92Cq8BtC55VlsYLD7bo3ofqQabNnlg7Q4H1Q6CDsLU,1713
|
17
|
+
solana_agent/interfaces/providers/llm.py,sha256=SPCXsnCXj7p04E24xB0Wj1q36h2Ci4mmcNCkpHGS8LY,2417
|
18
|
+
solana_agent/interfaces/providers/memory.py,sha256=h3HEOwWCiFGIuFBX49XOv1jFaQW3NGjyKPOfmQloevk,1011
|
19
|
+
solana_agent/interfaces/providers/vector_storage.py,sha256=XPYzvoWrlDVFCS9ItBmoqCFWXXWNYY-d9I7_pvP7YYk,1561
|
20
|
+
solana_agent/interfaces/services/agent.py,sha256=YsxyvBPK3ygBEStLyL4BwmIl84NMrV3dK0PlwCFoyq0,2094
|
21
|
+
solana_agent/interfaces/services/knowledge_base.py,sha256=HsU4fAMc_oOUCqCX2z76_IbAtbTNTyvffHZ49J0ynSQ,2092
|
22
|
+
solana_agent/interfaces/services/query.py,sha256=QfpBA3hrv8pQdNbK05hbu3Vh3-53F46IFcoUjwj5J9w,1568
|
23
|
+
solana_agent/interfaces/services/routing.py,sha256=Qbn3-DQGVSQKaegHDekSFmn_XCklA0H2f0XUx9-o3wA,367
|
24
|
+
solana_agent/plugins/__init__.py,sha256=coZdgJKq1ExOaj6qB810i3rEhbjdVlrkN76ozt_Ojgo,193
|
25
|
+
solana_agent/plugins/manager.py,sha256=Rrpom5noR5XCcDDAE9C8H_ITJDfj5gdkQF1dmgi5Eu0,5040
|
26
|
+
solana_agent/plugins/registry.py,sha256=0Bx3e_4fq20FtI0vbCTQanwK0AashQOr4vsqR26Jql8,3631
|
27
|
+
solana_agent/plugins/tools/__init__.py,sha256=VDjJxvUjefIy10VztQ9WDKgIegvDbIXBQWsHLhxdZ3o,125
|
28
|
+
solana_agent/plugins/tools/auto_tool.py,sha256=uihijtlc9CCqCIaRcwPuuN7o1SHIpWL2GV3vr33GG3E,1576
|
29
|
+
solana_agent/repositories/__init__.py,sha256=fP83w83CGzXLnSdq-C5wbw9EhWTYtqE2lQTgp46-X_4,163
|
30
|
+
solana_agent/repositories/memory.py,sha256=YYpCyiDVi3a5ZOFYFkzBS6MDjo9g2TnwbEZ5KKfKbII,7204
|
31
|
+
solana_agent/services/__init__.py,sha256=iko0c2MlF8b_SA_nuBGFllr2E3g_JowOrOzGcnU9tkA,162
|
32
|
+
solana_agent/services/agent.py,sha256=2ngtuJucGsEyNPKaWu4axd1pPgKofY-H8FeSY-MPBCU,27229
|
33
|
+
solana_agent/services/knowledge_base.py,sha256=J9V8dNoCCcko3EasiGwK2JJ_A_oG_e-Ni9pgNg0T6wA,33486
|
34
|
+
solana_agent/services/query.py,sha256=J81IGpy_1xsHFR3tEQkNDNoLcX9mFBJBrUcXz0pTW2w,13279
|
35
|
+
solana_agent/services/routing.py,sha256=-0fNIKDtCn0-TLUYDFYAE4jPLMeI_jCXIpgtgWDpdf8,6986
|
36
|
+
solana_agent-27.3.8.dist-info/LICENSE,sha256=BnSRc-NSFuyF2s496l_4EyrwAP6YimvxWcjPiJ0J7g4,1057
|
37
|
+
solana_agent-27.3.8.dist-info/METADATA,sha256=NweB1BWDWg9SsE1njikM-bHRx4O1JfwYtGwVvU5_3NA,23965
|
38
|
+
solana_agent-27.3.8.dist-info/WHEEL,sha256=fGIA9gx4Qxk2KDKeNJCbOEwSrmLtjWCwzBz351GyrPQ,88
|
39
|
+
solana_agent-27.3.8.dist-info/RECORD,,
|
@@ -1,39 +0,0 @@
|
|
1
|
-
solana_agent/__init__.py,sha256=ceYeUpjIitpln8YK1r0JVJU8mzG6cRPYu-HLny3d-Tw,887
|
2
|
-
solana_agent/adapters/__init__.py,sha256=tiEEuuy0NF3ngc_tGEcRTt71zVI58v3dYY9RvMrF2Cg,204
|
3
|
-
solana_agent/adapters/mongodb_adapter.py,sha256=qqEFbY_v1XGyFXBmwd5HSXSSHnA9wWo-Hm1vGEyIG0k,2718
|
4
|
-
solana_agent/adapters/openai_adapter.py,sha256=-GS_ujZIF3OVq3LDWb30a4zEvUADvfECDo7GO1lQnqM,10997
|
5
|
-
solana_agent/adapters/pinecone_adapter.py,sha256=xn_353EoFYD8KCTckOOO4e0OKzukeBWpyhGUmDawURw,22591
|
6
|
-
solana_agent/client/__init__.py,sha256=47DEQpj8HBSa-_TImW-5JCeuQeRkm5NMpJWZG3hSuFU,0
|
7
|
-
solana_agent/client/solana_agent.py,sha256=gARyoQkWStre3bwaTrR1AoAqdCpI3sw_p0hl6z6kkmA,9982
|
8
|
-
solana_agent/domains/__init__.py,sha256=HiC94wVPRy-QDJSSRywCRrhrFfTBeHjfi5z-QfZv46U,168
|
9
|
-
solana_agent/domains/agent.py,sha256=WTo-pEc66V6D_35cpDE-kTsw1SJM-dtylPZ7em5em7Q,2659
|
10
|
-
solana_agent/domains/routing.py,sha256=UDlgTjUoC9xIBVYu_dnf9-KG_bBgdEXAv_UtDOrYo0w,650
|
11
|
-
solana_agent/factories/__init__.py,sha256=47DEQpj8HBSa-_TImW-5JCeuQeRkm5NMpJWZG3hSuFU,0
|
12
|
-
solana_agent/factories/agent_factory.py,sha256=7O1WdWA9etV2Wk8DRJUf7qTiFD3GOhBN2T278sfRegM,11536
|
13
|
-
solana_agent/interfaces/__init__.py,sha256=IQs1WIM1FeKP1-kY2FEfyhol_dB-I-VAe2rD6jrVF6k,355
|
14
|
-
solana_agent/interfaces/client/client.py,sha256=rIZQXFenEpoSWUvZrCMNE-FWwYwZMCBvXSFC8vCWksg,3610
|
15
|
-
solana_agent/interfaces/plugins/plugins.py,sha256=T8HPBsekmzVwfU_Rizp-vtzAeYkMlKMYD7U9d0Wjq9c,3338
|
16
|
-
solana_agent/interfaces/providers/data_storage.py,sha256=NqGeFvAzhz9rr-liLPRNCGjooB2EIhe-EVsMmX__b0M,1658
|
17
|
-
solana_agent/interfaces/providers/llm.py,sha256=gcZeu8ErLoSmdXWKiBVZuNl31c0KIUhGwX3wPBD_xuI,2356
|
18
|
-
solana_agent/interfaces/providers/memory.py,sha256=oNOH8WZXVW8assDigIWZAWiwkxbpDiKupxA2RB6tQvQ,1010
|
19
|
-
solana_agent/interfaces/providers/vector_storage.py,sha256=zgdlzQuXCRREVSlB-g7QKKNjVoshSe2AFoAt4CHCAB0,1606
|
20
|
-
solana_agent/interfaces/services/agent.py,sha256=tVs2piqWVfO1JiRd58e29oP1GWgYuCzberRSfaFfH4M,1979
|
21
|
-
solana_agent/interfaces/services/knowledge_base.py,sha256=J4jGeWcxpKL3XpcrqdNuV1xyd5dj2d-hyAmx4AZ272w,2103
|
22
|
-
solana_agent/interfaces/services/query.py,sha256=yo2JZPJSy2iwxtkUlMz0emm9u_27xNgnrAFJRHQoulQ,1480
|
23
|
-
solana_agent/interfaces/services/routing.py,sha256=UzJC-z-Q9puTWPFGEo2_CAhIxuxP5IRnze7S66NSrsI,397
|
24
|
-
solana_agent/plugins/__init__.py,sha256=coZdgJKq1ExOaj6qB810i3rEhbjdVlrkN76ozt_Ojgo,193
|
25
|
-
solana_agent/plugins/manager.py,sha256=Il49hXeqvu0b02pURNNp7mY8kp9_sqpi_vJIWBW5Hc0,5044
|
26
|
-
solana_agent/plugins/registry.py,sha256=5S0DlUQKogsg1zLiRUIGMHEmGYHtOovU-S-5W1Mwo1A,3639
|
27
|
-
solana_agent/plugins/tools/__init__.py,sha256=c0z7ij42gs94_VJrcn4Y8gUlTxMhsFNY6ahIsNswdLk,231
|
28
|
-
solana_agent/plugins/tools/auto_tool.py,sha256=DgES_cZ6xKSf_HJpFINpvJxrjVlk5oeqa7pZRBsR9SM,1575
|
29
|
-
solana_agent/repositories/__init__.py,sha256=fP83w83CGzXLnSdq-C5wbw9EhWTYtqE2lQTgp46-X_4,163
|
30
|
-
solana_agent/repositories/memory.py,sha256=xe6yvts31IDcv445LtRGkOpw8YWqssiSB40QAfx9zxY,7271
|
31
|
-
solana_agent/services/__init__.py,sha256=ab_NXJmwYUCmCrCzuTlZ47bJZINW0Y0F5jfQ9OovidU,163
|
32
|
-
solana_agent/services/agent.py,sha256=ZP-2fj0jbutasdul_BEQXaLLPq3Fpu2uGsVsawkVgrg,27040
|
33
|
-
solana_agent/services/knowledge_base.py,sha256=bvoLZHSrfp6BhT8i4fKhxOkaUkYGCFedLp2uTzL-7xI,32379
|
34
|
-
solana_agent/services/query.py,sha256=rAGC0Q_0Y_TQ-ONhyovQ_44myl1-not_VhD_hobmCE8,13005
|
35
|
-
solana_agent/services/routing.py,sha256=hC5t98KZPHty9kMX27KcuxcmZlwjm0g59uMkR8n7k_w,6818
|
36
|
-
solana_agent-27.3.6.dist-info/LICENSE,sha256=BnSRc-NSFuyF2s496l_4EyrwAP6YimvxWcjPiJ0J7g4,1057
|
37
|
-
solana_agent-27.3.6.dist-info/METADATA,sha256=uKDkRZikU-6xONhpfpHoOvUORdowP8_Lef0Wmarl38o,23865
|
38
|
-
solana_agent-27.3.6.dist-info/WHEEL,sha256=fGIA9gx4Qxk2KDKeNJCbOEwSrmLtjWCwzBz351GyrPQ,88
|
39
|
-
solana_agent-27.3.6.dist-info/RECORD,,
|
File without changes
|
File without changes
|