prism-remote 1.0.0
This diff represents the content of publicly available package versions that have been released to one of the supported registries. The information contained in this diff is provided for informational purposes only and reflects changes between package versions as they appear in their respective public registries.
- package/LICENSE +21 -0
- package/README.md +36 -0
- package/example.html +24 -0
- package/package.json +26 -0
- package/prism-remote.js +80 -0
package/LICENSE
ADDED
@@ -0,0 +1,21 @@
|
|
1
|
+
MIT License
|
2
|
+
|
3
|
+
Copyright (c) 2024 Austin Riba
|
4
|
+
|
5
|
+
Permission is hereby granted, free of charge, to any person obtaining a copy
|
6
|
+
of this software and associated documentation files (the "Software"), to deal
|
7
|
+
in the Software without restriction, including without limitation the rights
|
8
|
+
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
9
|
+
copies of the Software, and to permit persons to whom the Software is
|
10
|
+
furnished to do so, subject to the following conditions:
|
11
|
+
|
12
|
+
The above copyright notice and this permission notice shall be included in all
|
13
|
+
copies or substantial portions of the Software.
|
14
|
+
|
15
|
+
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
16
|
+
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
17
|
+
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
18
|
+
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
19
|
+
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
20
|
+
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
21
|
+
SOFTWARE.
|
package/README.md
ADDED
@@ -0,0 +1,36 @@
|
|
1
|
+
# prism-remote
|
2
|
+
Easily embed remote code with syntax highlighting provided by [Prism](https://prismjs.com/) using a custom HTML
|
3
|
+
element. No other dependencies other than Prism itself.
|
4
|
+
|
5
|
+
Example:
|
6
|
+
|
7
|
+
```html
|
8
|
+
<prism-remote
|
9
|
+
src="https://github.com/Fingel/prism-remote/blob/main/prism-remote.js"
|
10
|
+
start="1"
|
11
|
+
end="10"
|
12
|
+
lang="javascript"
|
13
|
+
>
|
14
|
+
</prism-remote>
|
15
|
+
```
|
16
|
+
Would result in:
|
17
|
+
|
18
|
+
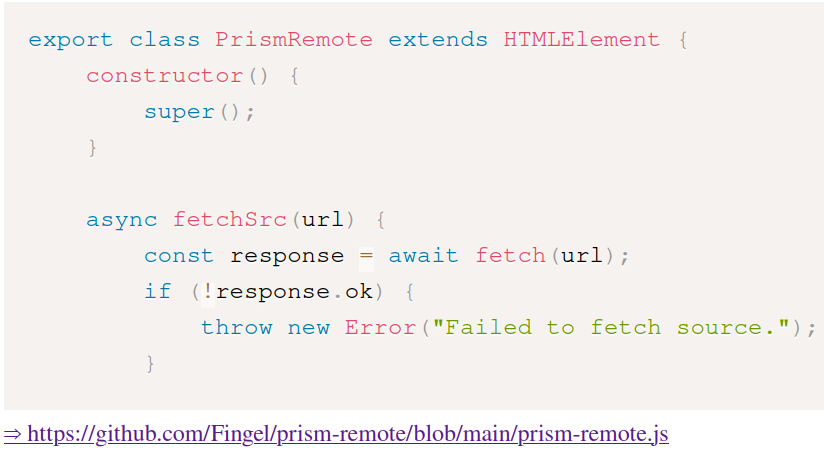
|
19
|
+
|
20
|
+
### Usage
|
21
|
+
Provide the following attributes to the `<prism-remote>` tag:
|
22
|
+
- `src` (required) The URL to the text you want to display.
|
23
|
+
- `lang` (required) The language for highlighting.
|
24
|
+
- `start` The first line to display (1 indexed).
|
25
|
+
- `end` The last line to display.
|
26
|
+
|
27
|
+
If you are using Github it's possible to provide the natural URL instead of the raw URL. For
|
28
|
+
example: https://github.com/Fingel/prism-remote/blob/main/prism-remote.js instead of https://raw.githubusercontent.com/Fingel/prism-remote/main/prism-remote.js
|
29
|
+
. The attribution link at the bottom will point to the natural URL.
|
30
|
+
|
31
|
+
The attribution link has a class of `prism-remote-attribution` so you can style it (or hide it alltogether).
|
32
|
+
|
33
|
+
### Installation
|
34
|
+
Make sure you have [Prism](https://prismjs.com/) available.
|
35
|
+
|
36
|
+
Include [prism-remote.js](prism-remote.js) on your page.
|
package/example.html
ADDED
@@ -0,0 +1,24 @@
|
|
1
|
+
<!DOCTYPE html>
|
2
|
+
<html>
|
3
|
+
<head>
|
4
|
+
<meta charset="utf-8">
|
5
|
+
<meta name="viewport" content="width=device-width, initial-scale=1">
|
6
|
+
<title></title>
|
7
|
+
<link href="https://unpkg.com/prismjs@1.29.0/themes/prism.min.css" rel="stylesheet">
|
8
|
+
</head>
|
9
|
+
<body>
|
10
|
+
<h2>Github source with limited line range</h2>
|
11
|
+
<prism-remote src="https://github.com/Fingel/prism-remote/blob/main/prism-remote.js"
|
12
|
+
start="1"
|
13
|
+
end="10"
|
14
|
+
lang="javascript"
|
15
|
+
></prism-remote>
|
16
|
+
|
17
|
+
<h2>Raw source with all lines</h2>
|
18
|
+
<prism-remote src="https://raw.githubusercontent.com/Fingel/prism-remote/main/prism-remote.js" lang="javascript"></prism-remote>
|
19
|
+
|
20
|
+
<script src="prism-remote.js" type="module"></script>
|
21
|
+
<script src="https://unpkg.com/prismjs@1.29.0/components/prism-core.min.js"></script>
|
22
|
+
<script src="https://unpkg.com/prismjs@1.29.0/plugins/autoloader/prism-autoloader.min.js"></script>
|
23
|
+
</body>
|
24
|
+
</html>
|
package/package.json
ADDED
@@ -0,0 +1,26 @@
|
|
1
|
+
{
|
2
|
+
"name": "prism-remote",
|
3
|
+
"version": "1.0.0",
|
4
|
+
"type": "module",
|
5
|
+
"description": "A custom element for displaying remote code blocks with syntax highlighting provided by Prism",
|
6
|
+
"main": "prism-remote.js",
|
7
|
+
"module": "prism-remote.js",
|
8
|
+
"scripts": {
|
9
|
+
"test": "echo \"Error: no test specified\" && exit 1"
|
10
|
+
},
|
11
|
+
"repository": {
|
12
|
+
"type": "git",
|
13
|
+
"url": "git+ssh://git@github.com/Fingel/prism-remote.git"
|
14
|
+
},
|
15
|
+
"keywords": [
|
16
|
+
"prism",
|
17
|
+
"web components",
|
18
|
+
"custom elements"
|
19
|
+
],
|
20
|
+
"author": "Austin Riba <austin@m51.io>",
|
21
|
+
"license": "MIT",
|
22
|
+
"bugs": {
|
23
|
+
"url": "https://github.com/Fingel/prism-remote/issues"
|
24
|
+
},
|
25
|
+
"homepage": "https://github.com/Fingel/prism-remote#readme"
|
26
|
+
}
|
package/prism-remote.js
ADDED
@@ -0,0 +1,80 @@
|
|
1
|
+
export class PrismRemote extends HTMLElement {
|
2
|
+
constructor() {
|
3
|
+
super();
|
4
|
+
}
|
5
|
+
|
6
|
+
async fetchSrc(url) {
|
7
|
+
const response = await fetch(url);
|
8
|
+
if (!response.ok) {
|
9
|
+
throw new Error("Failed to fetch source.");
|
10
|
+
}
|
11
|
+
const result = await response.text();
|
12
|
+
return result;
|
13
|
+
}
|
14
|
+
|
15
|
+
async connectedCallback() {
|
16
|
+
let srcUrl;
|
17
|
+
if (this.hasAttribute("src")) {
|
18
|
+
srcUrl = this.getAttribute("src");
|
19
|
+
} else {
|
20
|
+
console.error("src attribute must be set");
|
21
|
+
return;
|
22
|
+
}
|
23
|
+
|
24
|
+
let rawUrl;
|
25
|
+
if (srcUrl.includes("github.com")) {
|
26
|
+
rawUrl = srcUrl
|
27
|
+
.replace("github.com", "raw.githubusercontent.com")
|
28
|
+
.replace("/blob/", "/");
|
29
|
+
} else {
|
30
|
+
rawUrl = srcUrl;
|
31
|
+
}
|
32
|
+
|
33
|
+
let lang;
|
34
|
+
if (this.hasAttribute("lang")) {
|
35
|
+
lang = this.getAttribute("lang");
|
36
|
+
} else {
|
37
|
+
console.error("lang attribute must be set");
|
38
|
+
return;
|
39
|
+
}
|
40
|
+
|
41
|
+
let code = await this.fetchSrc(rawUrl);
|
42
|
+
let codeLines = code.split("\n");
|
43
|
+
const start = this.hasAttribute("start")
|
44
|
+
? this.getAttribute("start")
|
45
|
+
: 1;
|
46
|
+
const end = this.hasAttribute("end")
|
47
|
+
? this.getAttribute("end")
|
48
|
+
: codeLines.length;
|
49
|
+
codeLines = codeLines.splice(start - 1, end);
|
50
|
+
code = codeLines.join("\n");
|
51
|
+
|
52
|
+
const codePre = document.createElement("pre");
|
53
|
+
const codeBlock = document.createElement("code");
|
54
|
+
codeBlock.setAttribute("class", `language-${lang}`);
|
55
|
+
codeBlock.textContent = code;
|
56
|
+
codePre.appendChild(codeBlock);
|
57
|
+
|
58
|
+
const attribution = document.createElement("div");
|
59
|
+
attribution.setAttribute("class", "prism-remote-attribution");
|
60
|
+
const attributionLink = document.createElement("a");
|
61
|
+
attributionLink.setAttribute("href", srcUrl);
|
62
|
+
attributionLink.textContent = srcUrl;
|
63
|
+
attribution.appendChild(attributionLink);
|
64
|
+
|
65
|
+
const style = document.createElement("style");
|
66
|
+
style.textContent = `
|
67
|
+
.prism-remote-attribution a::before {
|
68
|
+
content: "⇒ ";
|
69
|
+
}
|
70
|
+
`;
|
71
|
+
|
72
|
+
this.appendChild(style);
|
73
|
+
this.appendChild(codePre);
|
74
|
+
this.appendChild(attribution);
|
75
|
+
|
76
|
+
Prism.highlightAllUnder(this);
|
77
|
+
}
|
78
|
+
}
|
79
|
+
|
80
|
+
customElements.define("prism-remote", PrismRemote);
|