n8n-nodes-chat-data 1.0.0
This diff represents the content of publicly available package versions that have been released to one of the supported registries. The information contained in this diff is provided for informational purposes only and reflects changes between package versions as they appear in their respective public registries.
- package/LICENSE.md +19 -0
- package/README.md +87 -0
- package/dist/credentials/ChatDataApi.credentials.d.ts +7 -0
- package/dist/credentials/ChatDataApi.credentials.js +42 -0
- package/dist/credentials/ChatDataApi.credentials.js.map +1 -0
- package/dist/nodes/ChatData/ActionDescription.d.ts +3 -0
- package/dist/nodes/ChatData/ActionDescription.js +581 -0
- package/dist/nodes/ChatData/ActionDescription.js.map +1 -0
- package/dist/nodes/ChatData/ChatData.node.d.ts +19 -0
- package/dist/nodes/ChatData/ChatData.node.js +980 -0
- package/dist/nodes/ChatData/ChatData.node.js.map +1 -0
- package/dist/nodes/ChatData/ChatData.svg +16 -0
- package/dist/nodes/ChatData/ChatbotDescription.d.ts +3 -0
- package/dist/nodes/ChatData/ChatbotDescription.js +382 -0
- package/dist/nodes/ChatData/ChatbotDescription.js.map +1 -0
- package/dist/nodes/ChatData/TriggerDescription.d.ts +6 -0
- package/dist/nodes/ChatData/TriggerDescription.js +205 -0
- package/dist/nodes/ChatData/TriggerDescription.js.map +1 -0
- package/dist/package.json +65 -0
- package/dist/tsconfig.tsbuildinfo +1 -0
- package/index.js +0 -0
- package/package.json +65 -0
package/LICENSE.md
ADDED
@@ -0,0 +1,19 @@
|
|
1
|
+
Copyright 2022 n8n
|
2
|
+
|
3
|
+
Permission is hereby granted, free of charge, to any person obtaining a copy of
|
4
|
+
this software and associated documentation files (the "Software"), to deal in
|
5
|
+
the Software without restriction, including without limitation the rights to
|
6
|
+
use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies
|
7
|
+
of the Software, and to permit persons to whom the Software is furnished to do
|
8
|
+
so, subject to the following conditions:
|
9
|
+
|
10
|
+
The above copyright notice and this permission notice shall be included in all
|
11
|
+
copies or substantial portions of the Software.
|
12
|
+
|
13
|
+
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
14
|
+
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
15
|
+
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
16
|
+
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
17
|
+
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
18
|
+
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
19
|
+
SOFTWARE.
|
package/README.md
ADDED
@@ -0,0 +1,87 @@
|
|
1
|
+
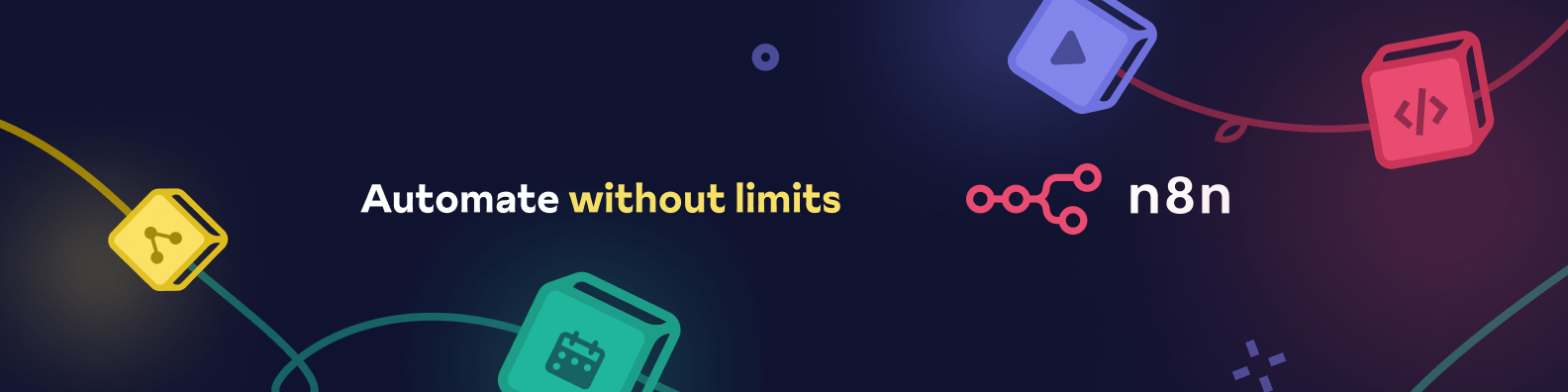
|
2
|
+
|
3
|
+
# n8n-nodes-chat-data
|
4
|
+
|
5
|
+
This is the official n8n integration for [Chat Data](https://chat-data.com). It provides nodes to interact with the ChatData platform, allowing you to manage chatbots, send messages, retrieve leads, and more.
|
6
|
+
|
7
|
+
## Prerequisites
|
8
|
+
|
9
|
+
To use the ChatData integration, you must have:
|
10
|
+
|
11
|
+
- A paid ChatData account. You can create an account at [chat-data.com](https://chat-data.com).
|
12
|
+
- Your ChatData API key, which you can obtain from your account settings.
|
13
|
+
|
14
|
+
## Installation
|
15
|
+
|
16
|
+
Follow these steps to install this node in your n8n instance:
|
17
|
+
|
18
|
+
```bash
|
19
|
+
npm install n8n-nodes-chat-data
|
20
|
+
```
|
21
|
+
|
22
|
+
For n8n desktop app/local installation:
|
23
|
+
|
24
|
+
```bash
|
25
|
+
n8n-node-dev --install n8n-nodes-chat-data
|
26
|
+
```
|
27
|
+
|
28
|
+
## Node Reference
|
29
|
+
|
30
|
+
### Chat Data
|
31
|
+
|
32
|
+
This node can be found in the 'Action' category in n8n.
|
33
|
+
|
34
|
+
#### Operations
|
35
|
+
|
36
|
+
The operations in this node are organized into the following categories:
|
37
|
+
|
38
|
+
##### Action
|
39
|
+
|
40
|
+
- **Send a Message**: Send a chat message to a chatbot
|
41
|
+
- **Get Leads**: Retrieve customer leads from a chatbot
|
42
|
+
- **Get Conversations**: Retrieve conversation history from a chatbot
|
43
|
+
|
44
|
+
##### Chatbot
|
45
|
+
|
46
|
+
- **Create Chatbot**: Set up a new AI-powered chatbot
|
47
|
+
- **Retrain Chatbot**: Update a chatbot with new data
|
48
|
+
- **Update Base Prompt**: Modify a chatbot's base prompt
|
49
|
+
- **Get Chatbot Training Status**: Check the status of a chatbot's training
|
50
|
+
|
51
|
+
##### Other
|
52
|
+
|
53
|
+
- **Make API Call**: Make a custom request to any Chat Data API endpoint
|
54
|
+
|
55
|
+
#### Triggers
|
56
|
+
|
57
|
+
Triggers in the ChatData node:
|
58
|
+
|
59
|
+
- **On New Message**: Triggered when a new chat message is received
|
60
|
+
- **On Lead Submission**: Triggered when a customer submits lead information
|
61
|
+
- **On Live Chat Escalation**: Triggered when a chat is escalated to a human agent
|
62
|
+
|
63
|
+
## Authentication
|
64
|
+
|
65
|
+
This node requires an API Key for authentication. To set this up:
|
66
|
+
|
67
|
+
1. Create an API key in your ChatData account:
|
68
|
+
- Log in to your ChatData account
|
69
|
+
- In the top right corner, click your Username > Account Settings
|
70
|
+
- In the API Keys section, click Create a new secret key
|
71
|
+
- Copy the API key value
|
72
|
+
|
73
|
+
2. In n8n, create a new credential:
|
74
|
+
- Choose "ChatDataApi" as the credential type
|
75
|
+
- Enter your API key in the API Key field
|
76
|
+
- Enter your Chat Data instance URL (usually https://app.chat-data.com)
|
77
|
+
- Save the credential
|
78
|
+
|
79
|
+
## Further Information
|
80
|
+
|
81
|
+
- [ChatData Website](https://chat-data.com)
|
82
|
+
- [ChatData API Documentation](https://docs.chat-data.com/api)
|
83
|
+
- [ChatData Pricing](https://chat-data.com/pricing)
|
84
|
+
|
85
|
+
## License
|
86
|
+
|
87
|
+
[MIT](LICENSE.md)
|
@@ -0,0 +1,42 @@
|
|
1
|
+
"use strict";
|
2
|
+
Object.defineProperty(exports, "__esModule", { value: true });
|
3
|
+
exports.ChatDataApi = void 0;
|
4
|
+
class ChatDataApi {
|
5
|
+
constructor() {
|
6
|
+
this.name = 'chatDataApi';
|
7
|
+
this.displayName = 'Chat Data API';
|
8
|
+
this.properties = [
|
9
|
+
{
|
10
|
+
displayName: 'API URL',
|
11
|
+
name: 'baseUrl',
|
12
|
+
type: 'string',
|
13
|
+
default: 'https://api.chat-data.com',
|
14
|
+
description: 'The URL to the Chat Data API',
|
15
|
+
},
|
16
|
+
{
|
17
|
+
displayName: 'API Key',
|
18
|
+
name: 'apiKey',
|
19
|
+
type: 'string',
|
20
|
+
typeOptions: {
|
21
|
+
password: true,
|
22
|
+
},
|
23
|
+
default: '',
|
24
|
+
placeholder: 'sk-...',
|
25
|
+
description: 'The API Key of your Chat Data account',
|
26
|
+
},
|
27
|
+
];
|
28
|
+
this.test = {
|
29
|
+
request: {
|
30
|
+
baseURL: '={{$credentials.baseUrl}}',
|
31
|
+
url: '/api/v2/current-plan',
|
32
|
+
method: 'GET',
|
33
|
+
headers: {
|
34
|
+
Authorization: '=Bearer {{$credentials.apiKey}}',
|
35
|
+
},
|
36
|
+
skipSslCertificateValidation: '={{$credentials.ignoreSSLErrors}}',
|
37
|
+
},
|
38
|
+
};
|
39
|
+
}
|
40
|
+
}
|
41
|
+
exports.ChatDataApi = ChatDataApi;
|
42
|
+
//# sourceMappingURL=ChatDataApi.credentials.js.map
|
@@ -0,0 +1 @@
|
|
1
|
+
{"version":3,"file":"ChatDataApi.credentials.js","sourceRoot":"","sources":["../../credentials/ChatDataApi.credentials.ts"],"names":[],"mappings":";;;AAMA,MAAa,WAAW;IAAxB;QACC,SAAI,GAAG,aAAa,CAAC;QACrB,gBAAW,GAAG,eAAe,CAAC;QAC9B,eAAU,GAAsB;YAC/B;gBACC,WAAW,EAAE,SAAS;gBACtB,IAAI,EAAE,SAAS;gBACf,IAAI,EAAE,QAAQ;gBACd,OAAO,EAAE,2BAA2B;gBACpC,WAAW,EAAE,8BAA8B;aAC3C;YACD;gBACC,WAAW,EAAE,SAAS;gBACtB,IAAI,EAAE,QAAQ;gBACd,IAAI,EAAE,QAAQ;gBACd,WAAW,EAAE;oBACZ,QAAQ,EAAE,IAAI;iBACd;gBACD,OAAO,EAAE,EAAE;gBACX,WAAW,EAAE,QAAQ;gBACrB,WAAW,EAAE,uCAAuC;aACpD;SACD,CAAC;QAGF,SAAI,GAA2B;YAC9B,OAAO,EAAE;gBACR,OAAO,EAAE,2BAA2B;gBACpC,GAAG,EAAE,sBAAsB;gBAC3B,MAAM,EAAE,KAAK;gBACb,OAAO,EAAE;oBACR,aAAa,EAAE,iCAAiC;iBAChD;gBACD,4BAA4B,EAAE,mCAAmC;aACjE;SACD,CAAC;IACH,CAAC;CAAA;AApCD,kCAoCC"}
|