fca-horidai-remastered 1.0.0 → 3.0.0
Sign up to get free protection for your applications and to get access to all the features.
Potentially problematic release.
This version of fca-horidai-remastered might be problematic. Click here for more details.
- package/CountTime.json +1 -0
- package/Extra/Balancer.js +49 -0
- package/Extra/Database/index.js +4 -4
- package/Extra/ExtraGetThread.js +22 -22
- package/Extra/Security/Base/index.js +5 -5
- package/Extra/Src/Release_Memory.js +5 -5
- package/Extra/Src/Websocket.js +3 -3
- package/Language/index.json +10 -4
- package/Main.js +209 -29
- package/index.js +11 -11
- package/package.json +7 -7
- package/src/acpUsers.js +40 -0
- package/src/addFriends.js +37 -0
- package/src/changeAvatar.js +93 -0
- package/src/changeBlockedStatusMqtt.js +79 -0
- package/src/changeCover.js +73 -0
- package/src/changeName.js +79 -0
- package/src/createCommentPost.js +228 -0
- package/src/createPollMqtt.js +56 -0
- package/src/createPost.js +277 -0
- package/src/createPostGroup.js +79 -0
- package/src/forwardMessage.js +60 -0
- package/src/getAcceptList.js +38 -0
- package/src/getThreadInfoDeprecated.js +56 -0
- package/src/listenMqtt.js +38 -18
- package/src/pinMessage.js +58 -0
- package/src/refreshFb_dtsg.js +81 -0
- package/src/sendComment.js +161 -0
- package/src/setMessageReactionMqtt.js +62 -0
- package/src/setStoryReaction.js +53 -0
- package/src/setTheme.js +310 -0
- package/src/unsendMessage.js +28 -20
- package/src/unsendMessageMqtt.js +59 -0
- package/src/unsendMqttMessage.js +66 -0
- package/src/uploadAttachment.js +95 -0
- package/test/Db2.js +4 -4
- package/test/Horizon_Database/Database.sqlite +0 -0
- package/test/Horizon_Database/SyntheticDatabase.sqlite +0 -0
- package/utils.js +45 -6
- package/.gitattributes +0 -2
- package/LICENSE +0 -21
- package/README.md +0 -152
- package/SECURITY.md +0 -18
package/src/unsendMessage.js
CHANGED
@@ -1,17 +1,20 @@
|
|
1
1
|
"use strict";
|
2
2
|
|
3
|
+
const Balancer = require('../Extra/Balancer.js');
|
3
4
|
var utils = require("../utils");
|
4
5
|
var log = require("npmlog");
|
5
6
|
|
6
7
|
module.exports = function (defaultFuncs, api, ctx) {
|
7
|
-
|
8
|
+
//const BalancerInstance = new Balancer(api.unsendMessage, unsendMessage, 0.85);
|
9
|
+
|
10
|
+
function unsendMessage(messageID, threadID, callback) {
|
8
11
|
var resolveFunc = function () { };
|
9
12
|
var rejectFunc = function () { };
|
10
13
|
var returnPromise = new Promise(function (resolve, reject) {
|
11
14
|
resolveFunc = resolve;
|
12
15
|
rejectFunc = reject;
|
13
16
|
});
|
14
|
-
|
17
|
+
|
15
18
|
if (!callback) {
|
16
19
|
callback = function (err, friendList) {
|
17
20
|
if (err) return rejectFunc(err);
|
@@ -19,22 +22,27 @@ module.exports = function (defaultFuncs, api, ctx) {
|
|
19
22
|
};
|
20
23
|
}
|
21
24
|
|
22
|
-
|
23
|
-
|
24
|
-
|
25
|
-
|
26
|
-
|
27
|
-
|
28
|
-
|
29
|
-
|
30
|
-
|
31
|
-
|
32
|
-
|
33
|
-
|
34
|
-
|
35
|
-
|
36
|
-
|
25
|
+
if (threadID) return api.unsendMqttMessage(threadID, messageID, callback);
|
26
|
+
else {
|
27
|
+
var form = {
|
28
|
+
message_id: messageID
|
29
|
+
};
|
30
|
+
|
31
|
+
defaultFuncs
|
32
|
+
.post("https://www.facebook.com/messaging/unsend_message/", ctx.jar, form)
|
33
|
+
.then(utils.parseAndCheckLogin(ctx, defaultFuncs))
|
34
|
+
.then(function (resData) {
|
35
|
+
if (resData.error) throw resData;
|
36
|
+
return callback();
|
37
|
+
})
|
38
|
+
.catch(function (err) {
|
39
|
+
log.error("unsendMessage", err);
|
40
|
+
return callback(err);
|
41
|
+
});
|
42
|
+
|
43
|
+
return returnPromise;
|
44
|
+
}
|
45
|
+
}
|
37
46
|
|
38
|
-
|
39
|
-
|
40
|
-
};
|
47
|
+
return unsendMessage;
|
48
|
+
};
|
@@ -0,0 +1,59 @@
|
|
1
|
+
'use strict';
|
2
|
+
|
3
|
+
const { generateOfflineThreadingID, getCurrentTimestamp } = require('../utils');
|
4
|
+
|
5
|
+
function isCallable(func) {
|
6
|
+
try {
|
7
|
+
Reflect.apply(func, null, []);
|
8
|
+
return true;
|
9
|
+
} catch (error) {
|
10
|
+
return false;
|
11
|
+
}
|
12
|
+
}
|
13
|
+
|
14
|
+
module.exports = function (defaultFuncs, api, ctx) {
|
15
|
+
return function unsendMessageMqtt(messageID, threadID, callback) {
|
16
|
+
if (!ctx.mqttClient) {
|
17
|
+
throw new Error('Not connected to MQTT');
|
18
|
+
}
|
19
|
+
|
20
|
+
ctx.wsReqNumber += 1;
|
21
|
+
ctx.wsTaskNumber += 1;
|
22
|
+
|
23
|
+
const label = '33';
|
24
|
+
|
25
|
+
const taskPayload = {
|
26
|
+
message_id: messageID,
|
27
|
+
thread_key: threadID,
|
28
|
+
sync_group: 1,
|
29
|
+
};
|
30
|
+
|
31
|
+
const payload = JSON.stringify(taskPayload);
|
32
|
+
const version = '25393437286970779';
|
33
|
+
|
34
|
+
const task = {
|
35
|
+
failure_count: null,
|
36
|
+
label: label,
|
37
|
+
payload: payload,
|
38
|
+
queue_name: 'unsend_message',
|
39
|
+
task_id: ctx.wsTaskNumber,
|
40
|
+
};
|
41
|
+
|
42
|
+
const content = {
|
43
|
+
app_id: '2220391788200892',
|
44
|
+
payload: JSON.stringify({
|
45
|
+
tasks: [task],
|
46
|
+
epoch_id: parseInt(generateOfflineThreadingID()),
|
47
|
+
version_id: version,
|
48
|
+
}),
|
49
|
+
request_id: ctx.wsReqNumber,
|
50
|
+
type: 3,
|
51
|
+
};
|
52
|
+
|
53
|
+
if (isCallable(callback)) {
|
54
|
+
// to be implemented
|
55
|
+
}
|
56
|
+
|
57
|
+
ctx.mqttClient.publish('/ls_req', JSON.stringify(content), { qos: 1, retain: false });
|
58
|
+
};
|
59
|
+
};
|
@@ -0,0 +1,66 @@
|
|
1
|
+
/* eslint-disable linebreak-style */
|
2
|
+
"use strict";
|
3
|
+
|
4
|
+
var utils = require("../utils");
|
5
|
+
|
6
|
+
module.exports = function (defaultFuncs, api, ctx) {
|
7
|
+
return function(threadID, messageID ,callback) {
|
8
|
+
var resolveFunc = function () { };
|
9
|
+
var rejectFunc = function () { };
|
10
|
+
|
11
|
+
var returnPromise = new Promise(function (resolve, reject) {
|
12
|
+
resolveFunc = resolve;
|
13
|
+
rejectFunc = reject;
|
14
|
+
});
|
15
|
+
|
16
|
+
if (!callback && utils.getType(messageID) === "AsyncFunction" || !callback && utils.getType(messageID) === "Function") messageID = callback;
|
17
|
+
|
18
|
+
if (!callback) {
|
19
|
+
callback = function (err, data) {
|
20
|
+
if (err) return rejectFunc(err);
|
21
|
+
resolveFunc(data);
|
22
|
+
};
|
23
|
+
}
|
24
|
+
|
25
|
+
const Payload = {
|
26
|
+
message_id:messageID,
|
27
|
+
thread_key:threadID,
|
28
|
+
sync_group: 1
|
29
|
+
};
|
30
|
+
|
31
|
+
if (messageID != undefined || messageID != null) Payload.reply_metadata = {
|
32
|
+
reply_source_id: messageID,
|
33
|
+
reply_source_type: 1,
|
34
|
+
reply_type: 0
|
35
|
+
};
|
36
|
+
|
37
|
+
const Form = JSON.stringify({
|
38
|
+
app_id: "2220391788200892",
|
39
|
+
payload: JSON.stringify({
|
40
|
+
tasks: [{
|
41
|
+
label: 33,
|
42
|
+
payload: JSON.stringify(Payload),
|
43
|
+
queue_name: "unsend_message",
|
44
|
+
task_id: Math.random() * 1001 << 0,
|
45
|
+
failure_count: null,
|
46
|
+
}],
|
47
|
+
epoch_id: utils.generateOfflineThreadingID(),
|
48
|
+
version_id: '9094446350588544',
|
49
|
+
|
50
|
+
}),
|
51
|
+
request_id: ++ctx.req_ID,
|
52
|
+
type: 3
|
53
|
+
});
|
54
|
+
|
55
|
+
ctx.mqttClient.publish('/ls_req', Form,{
|
56
|
+
qos: 1,
|
57
|
+
retain: false,
|
58
|
+
});
|
59
|
+
ctx.callback_Task[ctx.req_ID] = new Object({
|
60
|
+
callback,
|
61
|
+
type: "unsendMqttMessage",
|
62
|
+
});
|
63
|
+
|
64
|
+
return returnPromise;
|
65
|
+
};
|
66
|
+
};
|
@@ -0,0 +1,95 @@
|
|
1
|
+
const utils = require("../utils");
|
2
|
+
const log = require("npmlog");
|
3
|
+
|
4
|
+
module.exports = function (defaultFuncs, api, ctx) {
|
5
|
+
function upload(attachments, callback) {
|
6
|
+
callback = callback || function () { };
|
7
|
+
const uploads = [];
|
8
|
+
|
9
|
+
// create an array of promises
|
10
|
+
for (let i = 0; i < attachments.length; i++) {
|
11
|
+
if (!utils.isReadableStream(attachments[i])) {
|
12
|
+
throw {
|
13
|
+
error:
|
14
|
+
"Attachment should be a readable stream and not " +
|
15
|
+
utils.getType(attachments[i]) +
|
16
|
+
"."
|
17
|
+
};
|
18
|
+
}
|
19
|
+
|
20
|
+
const form = {
|
21
|
+
upload_1024: attachments[i],
|
22
|
+
voice_clip: "true"
|
23
|
+
};
|
24
|
+
|
25
|
+
uploads.push(
|
26
|
+
defaultFuncs
|
27
|
+
.postFormData(
|
28
|
+
"https://upload.facebook.com/ajax/mercury/upload.php",
|
29
|
+
ctx.jar,
|
30
|
+
form,
|
31
|
+
{}
|
32
|
+
)
|
33
|
+
.then(utils.parseAndCheckLogin(ctx, defaultFuncs))
|
34
|
+
.then(function (resData) {
|
35
|
+
if (resData.error) {
|
36
|
+
throw resData;
|
37
|
+
}
|
38
|
+
|
39
|
+
// We have to return the data unformatted unless we want to change it
|
40
|
+
// back in sendMessage.
|
41
|
+
return resData.payload.metadata[0];
|
42
|
+
})
|
43
|
+
);
|
44
|
+
}
|
45
|
+
|
46
|
+
// resolve all promises
|
47
|
+
Promise
|
48
|
+
.all(uploads)
|
49
|
+
.then(function (resData) {
|
50
|
+
callback(null, resData);
|
51
|
+
})
|
52
|
+
.catch(function (err) {
|
53
|
+
log.error("uploadAttachment", err);
|
54
|
+
return callback(err);
|
55
|
+
});
|
56
|
+
}
|
57
|
+
|
58
|
+
return function uploadAttachment(attachments, callback) {
|
59
|
+
if (
|
60
|
+
!attachments &&
|
61
|
+
!utils.isReadableStream(attachments) &&
|
62
|
+
!utils.getType(attachments) === "Array" &&
|
63
|
+
(utils.getType(attachments) === "Array" && !attachments.length)
|
64
|
+
)
|
65
|
+
throw { error: "Please pass an attachment or an array of attachments." };
|
66
|
+
|
67
|
+
let resolveFunc = function () { };
|
68
|
+
let rejectFunc = function () { };
|
69
|
+
const returnPromise = new Promise(function (resolve, reject) {
|
70
|
+
resolveFunc = resolve;
|
71
|
+
rejectFunc = reject;
|
72
|
+
});
|
73
|
+
|
74
|
+
if (!callback) {
|
75
|
+
callback = function (err, info) {
|
76
|
+
if (err) {
|
77
|
+
return rejectFunc(err);
|
78
|
+
}
|
79
|
+
resolveFunc(info);
|
80
|
+
};
|
81
|
+
}
|
82
|
+
|
83
|
+
if (utils.getType(attachments) !== "Array")
|
84
|
+
attachments = [attachments];
|
85
|
+
|
86
|
+
upload(attachments, (err, info) => {
|
87
|
+
if (err) {
|
88
|
+
return callback(err);
|
89
|
+
}
|
90
|
+
callback(null, info);
|
91
|
+
});
|
92
|
+
|
93
|
+
return returnPromise;
|
94
|
+
};
|
95
|
+
};
|
package/test/Db2.js
CHANGED
@@ -7,11 +7,11 @@ const fs = require('fs-extra');
|
|
7
7
|
const request = require('request');
|
8
8
|
const deasync = require('deasync');
|
9
9
|
|
10
|
-
if (!fs.existsSync(process.cwd() + '/
|
11
|
-
fs.mkdirSync(process.cwd() + '/
|
12
|
-
fs.writeFileSync(process.cwd() + '/
|
10
|
+
if (!fs.existsSync(process.cwd() + '/main/database_fca')) {
|
11
|
+
fs.mkdirSync(process.cwd() + '/main/database_fca');
|
12
|
+
fs.writeFileSync(process.cwd() + '/main/database_fca/A_README.md', 'This folder is used by ChernobyL(NANI =)) ) to store data. Do not delete this folder or any of the files in it.', 'utf8');
|
13
13
|
}
|
14
|
-
var Database = new sqlite3.Database(process.cwd() + "/
|
14
|
+
var Database = new sqlite3.Database(process.cwd() + "/main/database_fca/SyntheticDatabase.sqlite");
|
15
15
|
|
16
16
|
Database.serialize(function() {
|
17
17
|
Database.run("CREATE TABLE IF NOT EXISTS json (ID TEXT, json TEXT)");
|
Binary file
|
Binary file
|
package/utils.js
CHANGED
@@ -2405,13 +2405,41 @@ function formatRead(event) {
|
|
2405
2405
|
function getFrom(str, startToken, endToken) {
|
2406
2406
|
var start = str.indexOf(startToken) + startToken.length;
|
2407
2407
|
if (start < startToken.length) return "";
|
2408
|
-
|
2409
2408
|
var lastHalf = str.substring(start);
|
2410
2409
|
var end = lastHalf.indexOf(endToken);
|
2411
|
-
if (end === -1) throw Error("Could not find endTime
|
2410
|
+
if (end === -1) throw Error("Could not find endTime " + endToken + " in the given string.");
|
2412
2411
|
return lastHalf.substring(0, end);
|
2413
2412
|
}
|
2414
2413
|
|
2414
|
+
|
2415
|
+
function getFroms(str, startToken, endToken) {
|
2416
|
+
//advanced search by kanzuuuuuuuuuu
|
2417
|
+
let results = [];
|
2418
|
+
let currentIndex = 0;
|
2419
|
+
|
2420
|
+
while (true) {
|
2421
|
+
let start = str.indexOf(startToken, currentIndex);
|
2422
|
+
if (start === -1) break;
|
2423
|
+
|
2424
|
+
start += startToken.length;
|
2425
|
+
|
2426
|
+
let lastHalf = str.substring(start);
|
2427
|
+
let end = lastHalf.indexOf(endToken);
|
2428
|
+
|
2429
|
+
if (end === -1) {
|
2430
|
+
if (results.length === 0) {
|
2431
|
+
throw Error("Could not find endToken `" + endToken + "` in the given string.");
|
2432
|
+
}
|
2433
|
+
break;
|
2434
|
+
}
|
2435
|
+
|
2436
|
+
results.push(lastHalf.substring(0, end));
|
2437
|
+
currentIndex = start + end + endToken.length;
|
2438
|
+
}
|
2439
|
+
|
2440
|
+
return results.length === 0 ? "" : results.length === 1 ? results[0] : results;
|
2441
|
+
}
|
2442
|
+
|
2415
2443
|
/**
|
2416
2444
|
* @param {string} html
|
2417
2445
|
*/
|
@@ -2664,7 +2692,12 @@ function parseAndCheckLogin(ctx, defaultFuncs, retryCount) {
|
|
2664
2692
|
const Check = () => new Promise((re) => {
|
2665
2693
|
defaultFuncs.get('https://facebook.com', ctx.jar).then(function(res) {
|
2666
2694
|
if (res.headers.location && res.headers.location.includes('https://www.facebook.com/checkpoint/')) {
|
2667
|
-
if (res.headers.includes('828281030927956')) return global.Fca.Action('Bypass', ctx, "956", defaultFuncs)
|
2695
|
+
if (res.headers.location.includes('828281030927956')) return global.Fca.Action('Bypass', ctx, "956", defaultFuncs)
|
2696
|
+
else if (res.request.uri && res.request.uri.href.includes("https://www.facebook.com/checkpoint/")) {
|
2697
|
+
if (res.request.uri.href.includes('601051028565049')) {
|
2698
|
+
return global.Fca.BypassAutomationNotification(undefined, ctx.jar, ctx.globalOptions, undefined ,process.env.UID)
|
2699
|
+
}
|
2700
|
+
}
|
2668
2701
|
else return global.Fca.Require.logger.Error(global.Fca.Require.Language.Index.ErrAppState);
|
2669
2702
|
}
|
2670
2703
|
else return global.Fca.Require.logger.Warning(global.Fca.Require.Language.Index.AutoLogin, function() {
|
@@ -2675,6 +2708,11 @@ function parseAndCheckLogin(ctx, defaultFuncs, retryCount) {
|
|
2675
2708
|
await Check();
|
2676
2709
|
});
|
2677
2710
|
}
|
2711
|
+
if (res.request.uri && res.request.uri.href.includes("https://www.facebook.com/checkpoint/")) {
|
2712
|
+
if (res.request.uri.href.includes('601051028565049')) {
|
2713
|
+
return global.Fca.BypassAutomationNotification(undefined, ctx.jar, ctx.globalOptions, undefined ,process.env.UID)
|
2714
|
+
}
|
2715
|
+
}
|
2678
2716
|
if (global.Fca.Require.FastConfig.AutoLogin) {
|
2679
2717
|
return global.Fca.Require.logger.Warning(global.Fca.Require.Language.Index.AutoLogin, function() {
|
2680
2718
|
return global.Fca.Action('AutoLogin');
|
@@ -2885,7 +2923,7 @@ function getAppState(jar, Encode) {
|
|
2885
2923
|
var logger = require('./logger'),languageFile = require('./Language/index.json');
|
2886
2924
|
var Language = languageFile.find(i => i.Language == globalThis.Fca.Require.FastConfig.Language).Folder.Index;
|
2887
2925
|
var data;
|
2888
|
-
switch (require(process.cwd() + "/
|
2926
|
+
switch (require(process.cwd() + "/main/json/configfca.json").EncryptFeature) {
|
2889
2927
|
case true: {
|
2890
2928
|
if (Encode == undefined) Encode = true;
|
2891
2929
|
if (process.env['FBKEY'] != undefined && Encode) {
|
@@ -2900,7 +2938,7 @@ function getAppState(jar, Encode) {
|
|
2900
2938
|
}
|
2901
2939
|
break;
|
2902
2940
|
default: {
|
2903
|
-
logger.Normal(getText(Language.IsNotABoolean,require(process.cwd() + "/
|
2941
|
+
logger.Normal(getText(Language.IsNotABoolean,require(process.cwd() + "/main/json/configfca.json").EncryptFeature));
|
2904
2942
|
data = appstate;
|
2905
2943
|
}
|
2906
2944
|
}
|
@@ -3034,5 +3072,6 @@ module.exports = {
|
|
3034
3072
|
decodeClientPayload,
|
3035
3073
|
getAppState,
|
3036
3074
|
getAdminTextMessageType,
|
3037
|
-
setProxy
|
3075
|
+
setProxy,
|
3076
|
+
getFroms
|
3038
3077
|
};
|
package/.gitattributes
DELETED
package/LICENSE
DELETED
@@ -1,21 +0,0 @@
|
|
1
|
-
MIT License
|
2
|
-
|
3
|
-
Copyright (c) 2023 KanzuWakazaki
|
4
|
-
|
5
|
-
Permission is hereby granted, free of charge, to any person obtaining a copy
|
6
|
-
of this software and associated documentation files (the "Software"), to deal
|
7
|
-
in the Software without restriction, including without limitation the rights
|
8
|
-
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
9
|
-
copies of the Software, and to permit persons to whom the Software is
|
10
|
-
furnished to do so, subject to the following conditions:
|
11
|
-
|
12
|
-
The above copyright notice and this permission notice shall be included in all
|
13
|
-
copies or substantial portions of the Software.
|
14
|
-
|
15
|
-
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
16
|
-
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
17
|
-
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
18
|
-
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
19
|
-
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
20
|
-
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
21
|
-
SOFTWARE.
|
package/README.md
DELETED
@@ -1,152 +0,0 @@
|
|
1
|
-
[](https://socket.dev/npm/package/fca-horizon-remastered)
|
2
|
-
|
3
|
-
# The following are not allowed here and a little note:
|
4
|
-
|
5
|
-
🎆
|
6
|
-
|
7
|
-
## Important !
|
8
|
-
|
9
|
-
<img width="517" alt="Reason" src="https://i.imgur.com/rD3ujmL.png">
|
10
|
-
This project is no longer being developed because the project owner lacks high security capabilities, leading to potential security vulnerabilities. Therefore, the project will be permanently suspended.
|
11
|
-
|
12
|
-
Special Thanks:
|
13
|
-
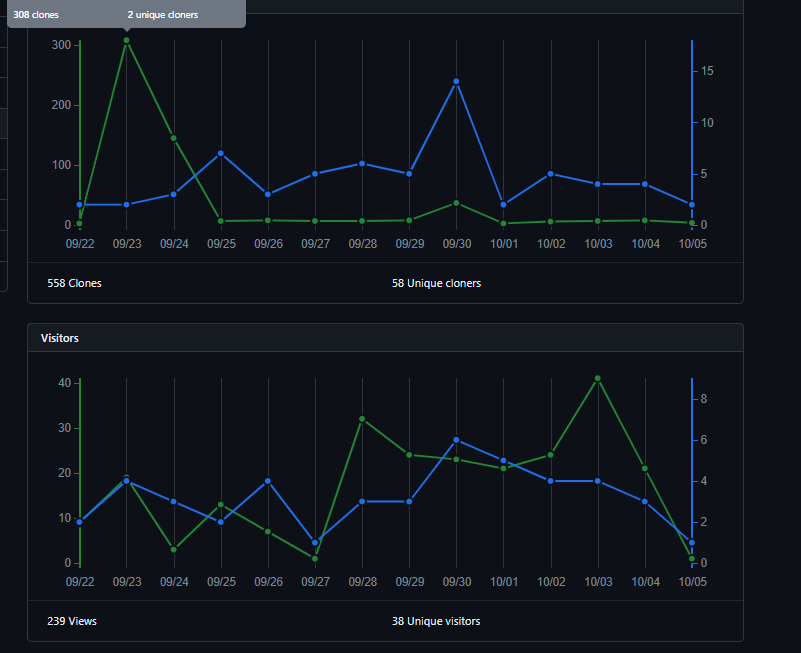
|
14
|
-
|
15
|
-
## Important !
|
16
|
-
|
17
|
-
This package require NodeJS 14.17.0 to work properly.
|
18
|
-
|
19
|
-
## Notification !
|
20
|
-
|
21
|
-
+ We will have Example Video on Channel "Nguyễn Thái Hảo Official"
|
22
|
-
|
23
|
-
Original Project(Deprecated): https://github.com/Schmavery/facebook-chat-api
|
24
|
-
|
25
|
-
Chúc các bạn một ngày tốt lành!, cảm ơn vì đã sài Sản phẩm của HZI, thân ái
|
26
|
-
|
27
|
-
KANZUWAKAZAKI(15/04/2023)
|
28
|
-
|
29
|
-
## Support For :
|
30
|
-
|
31
|
-
+ Support English, VietNamese !,
|
32
|
-
+ All bot if using listenMqtt first.
|
33
|
-
|
34
|
-
# Api Cho ChatBot Messenger
|
35
|
-
|
36
|
-
Facebook Đã Có Và Cho Người Dùng Tạo Api Cho Chatbots 😪 Tại Đey => [Đây Nè](https://developers.facebook.com/docs/messenger-platform).
|
37
|
-
|
38
|
-
### Api Này Có Thể Khiến Cho Bạn Payy Acc Như Cách Acc Bạn Chưa Từng Có, Hãy Chú Ý Nhé =))
|
39
|
-
|
40
|
-
Lưu Ý ! Nếu Bạn Muốn Sài Api Này Hãy Xem Document Tại [Đây Nè](https://github.com/Schmavery/facebook-chat-api).
|
41
|
-
|
42
|
-
## Tải Về
|
43
|
-
|
44
|
-
Nếu Bạn Muốn Sử Dụng, Hãy Tải Nó Bằng Cách:
|
45
|
-
```bash
|
46
|
-
npm i fca-horizon-remastered
|
47
|
-
```
|
48
|
-
or
|
49
|
-
```bash
|
50
|
-
npm install fca-horizon-remastered
|
51
|
-
```
|
52
|
-
|
53
|
-
Nó Sẽ Tải Vô node_modules (Lib Của Bạn) - Lưu Ý Replit Sẽ Không Hiện Đâu Mà Tìm 😪
|
54
|
-
|
55
|
-
### Tải Bản Mới Nhất Hoặc Update
|
56
|
-
|
57
|
-
Nếu Bạn Muốn Sử Dụng Phiên Bản Mới Nhất Hay Cập Nhật Thì Hãy Vô Terminal Hoặc Command Promt Nhập :
|
58
|
-
```bash
|
59
|
-
npm install fca-horizon-remastered@latest
|
60
|
-
```
|
61
|
-
Hoặc
|
62
|
-
```bash
|
63
|
-
npm i fca-horizon-remastered@latest
|
64
|
-
```
|
65
|
-
|
66
|
-
## Nếu Bạn Muốn Test Api
|
67
|
-
|
68
|
-
Lợi Ích Cho Việc Này Thì Bạn Sẽ Không Tốn Thời Gian Pay Acc Và Có Acc 😪
|
69
|
-
Hãy Sử Dụng Với Tài Khoản Thử Nghiệm => [Facebook Whitehat Accounts](https://www.facebook.com/whitehat/accounts/).
|
70
|
-
|
71
|
-
## Cách Sử Dụng
|
72
|
-
|
73
|
-
```javascript
|
74
|
-
const login = require("fca-horizon-remastered"); // lấy từ lib ra
|
75
|
-
|
76
|
-
// đăng nhập
|
77
|
-
login({email: "Gmail Account", password: "Mật Khẩu Facebook Của Bạn"}, (err, api) => {
|
78
|
-
|
79
|
-
if(err) return console.error(err); // trường hợp lỗi
|
80
|
-
|
81
|
-
// tạo bot tự động nhái theo bạn:
|
82
|
-
api.listenMqtt((err, message) => {
|
83
|
-
api.sendMessage(message.body, message.threadID);
|
84
|
-
});
|
85
|
-
|
86
|
-
});
|
87
|
-
```
|
88
|
-
|
89
|
-
Kết Quả Là Nó Sẽ Nhái Bạn Như Hình Dưới:
|
90
|
-
<img width="517" alt="screen shot 2016-11-04 at 14 36 00" src="https://cloud.githubusercontent.com/assets/4534692/20023545/f8c24130-a29d-11e6-9ef7-47568bdbc1f2.png">
|
91
|
-
|
92
|
-
Nếu Bạn Muốn Sử Dụng Nâng Cao Thì Hãy Sử Dụng Các Loại Bot Được Liệt Kê Ở Trên !
|
93
|
-
|
94
|
-
## Danh Sách
|
95
|
-
|
96
|
-
Bạn Có Thể Đọc Full Api Tại => [here](DOCS.md).
|
97
|
-
|
98
|
-
## Cài Đặt Cho Mirai:
|
99
|
-
|
100
|
-
Bạn Cần Vô File Mirai.js,Sau Đó Tìm Đến Dòng
|
101
|
-
```js
|
102
|
-
var login = require('tùy bot');
|
103
|
-
/* Có thể là :
|
104
|
-
var login = require('@maihuybao/fca-Unofficial');
|
105
|
-
var login = require('fca-xuyen-get');
|
106
|
-
var login = require('fca-unofficial-force');
|
107
|
-
...
|
108
|
-
*/
|
109
|
-
```
|
110
|
-
|
111
|
-
Và Thay Nó Bằng:
|
112
|
-
|
113
|
-
```js
|
114
|
-
var login = require('fca-horizon-remastered')
|
115
|
-
```
|
116
|
-
|
117
|
-
Sau Đó Thì Chạy Bình Thường Thôi !
|
118
|
-
|
119
|
-
## Tự Nghiên Cứu
|
120
|
-
|
121
|
-
Nếu Bạn Muốn Tự Nghiên Cứu Hoặc Tạo Bot Cho Riêng Bạn Thì Bạn Hãy Vô Cái Này Đọc Chức Năng Của Nó Và Cách Sử Dụng => [Link](https://github.com/Schmavery/facebook-chat-api#Unofficial%20Facebook%20Chat%20API)
|
122
|
-
|
123
|
-
------------------------------------
|
124
|
-
|
125
|
-
### Lưu Lại Thông Tin Đăng Nhập.
|
126
|
-
|
127
|
-
Để Lưu Lại Thì Bạn Cần 1 Apstate Kiểu (Cookie, etc,..) Để Lưu Lại Hoặc Là Sử Dụng Mã Login Như Trên Để Đăng Nhập !
|
128
|
-
|
129
|
-
Và Chế Độ Này Đã Có Sẵn Trong 1 Số Bot Việt Nam Nên Bạn Cứ Yên Tâm Nhé !
|
130
|
-
|
131
|
-
__Hướng Dẫn Với Appstate__
|
132
|
-
|
133
|
-
```js
|
134
|
-
const fs = require("fs");
|
135
|
-
const login = require("fca-horizon-remastered");
|
136
|
-
|
137
|
-
var credentials = {email: "FB_EMAIL", password: "FB_PASSWORD"}; // thông tin tk
|
138
|
-
|
139
|
-
login(credentials, (err, api) => {
|
140
|
-
if(err) return console.error(err);
|
141
|
-
// đăng nhập
|
142
|
-
fs.writeFileSync('appstate.json', JSON.stringify(api.getAppState(), null,'\t')); //tạo appstate
|
143
|
-
});
|
144
|
-
```
|
145
|
-
|
146
|
-
Hoặc Dễ Dàng Hơn ( Chuyên Nghiệp ) Bạn Có Thể Dùng => [c3c-fbstate](https://github.com/c3cbot/c3c-fbstate) Để Lấy Fbstate And Rename Lại Thành Apstate Cũng Được ! (appstate.json)
|
147
|
-
|
148
|
-
------------------------------------
|
149
|
-
|
150
|
-
## FAQS
|
151
|
-
|
152
|
-
FAQS => [Link](https://github.com/Schmavery/facebook-chat-api#FAQS)
|
package/SECURITY.md
DELETED
@@ -1,18 +0,0 @@
|
|
1
|
-
# Security Policy
|
2
|
-
|
3
|
-
+ if have any Vulnerability finded contact: Author(KanzuWakazaki.Main@proton.me) or (Facebook.com/Lazic.Kanzu). Thanks!
|
4
|
-
|
5
|
-
## Supported Versions
|
6
|
-
|
7
|
-
Use this section to tell people about which versions of your project are
|
8
|
-
currently being supported with security updates.
|
9
|
-
|
10
|
-
| Version | Supported |
|
11
|
-
| ------- | ------------------ |
|
12
|
-
| StableVersion | :white_check_mark: |
|
13
|
-
| AutoUpdate | :white_check_mark:|
|
14
|
-
| Modified | :x:
|
15
|
-
|
16
|
-
## Reporting a Vulnerability
|
17
|
-
|
18
|
-
Contact Author or create pull!
|