@tweenjs/tween.js 20.0.0 → 20.0.2
Sign up to get free protection for your applications and to get access to all the features.
- package/README.md +109 -50
- package/dist/tween.amd.js +1 -1
- package/dist/tween.cjs.js +1 -1
- package/dist/tween.d.ts +1 -1
- package/dist/tween.esm.js +1 -1
- package/dist/tween.umd.js +1 -1
- package/package.json +1 -1
package/README.md
CHANGED
@@ -1,101 +1,159 @@
|
|
1
1
|
# tween.js
|
2
2
|
|
3
|
-
JavaScript tweening engine for easy animations, incorporating optimised Robert Penner's equations.
|
3
|
+
JavaScript (TypeScript) tweening engine for easy animations, incorporating optimised Robert Penner's equations.
|
4
4
|
|
5
5
|
[![NPM Version][npm-image]][npm-url]
|
6
6
|
[![CDNJS][cdnjs-image]][cdnjs-url]
|
7
7
|
[![NPM Downloads][downloads-image]][downloads-url]
|
8
8
|
[![Build and Tests][ci-image]][ci-url]
|
9
9
|
|
10
|
-
**Update Note** In v18 the script you should include has moved from `src/Tween.js` to `dist/tween.umd.js`. See the [installation section](#Installation) below.
|
11
|
-
|
12
10
|
---
|
13
11
|
|
14
|
-
```
|
15
|
-
|
16
|
-
|
17
|
-
box
|
18
|
-
|
19
|
-
|
20
|
-
|
21
|
-
|
22
|
-
|
12
|
+
```html
|
13
|
+
<script src="https://cdnjs.cloudflare.com/ajax/libs/tween.js/20.0.0/tween.umd.js"></script>
|
14
|
+
|
15
|
+
<div id="box"></div>
|
16
|
+
|
17
|
+
<style>
|
18
|
+
#box {
|
19
|
+
background-color: deeppink;
|
20
|
+
width: 100px;
|
21
|
+
height: 100px;
|
22
|
+
}
|
23
|
+
</style>
|
24
|
+
|
25
|
+
<script>
|
26
|
+
const box = document.getElementById('box') // Get the element we want to animate.
|
27
|
+
|
28
|
+
const coords = {x: 0, y: 0} // Start at (0, 0)
|
29
|
+
|
30
|
+
const tween = new TWEEN.Tween(coords, false) // Create a new tween that modifies 'coords'.
|
31
|
+
.to({x: 300, y: 200}, 1000) // Move to (300, 200) in 1 second.
|
32
|
+
.easing(TWEEN.Easing.Quadratic.InOut) // Use an easing function to make the animation smooth.
|
33
|
+
.onUpdate(() => {
|
34
|
+
// Called after tween.js updates 'coords'.
|
35
|
+
// Move 'box' to the position described by 'coords' with a CSS translation.
|
36
|
+
box.style.setProperty('transform', 'translate(' + coords.x + 'px, ' + coords.y + 'px)')
|
37
|
+
})
|
38
|
+
.start() // Start the tween immediately.
|
39
|
+
|
40
|
+
// Setup the animation loop.
|
41
|
+
function animate(time) {
|
42
|
+
tween.update(time)
|
43
|
+
requestAnimationFrame(animate)
|
44
|
+
}
|
23
45
|
requestAnimationFrame(animate)
|
24
|
-
|
25
|
-
|
26
|
-
|
27
|
-
|
28
|
-
|
29
|
-
|
30
|
-
|
31
|
-
|
32
|
-
|
33
|
-
|
34
|
-
|
35
|
-
|
36
|
-
|
37
|
-
|
46
|
+
</script>
|
47
|
+
```
|
48
|
+
|
49
|
+
[Try this example on CodePen](https://codepen.io/trusktr/pen/KKGaBVz?editors=1000)
|
50
|
+
|
51
|
+
# Installation
|
52
|
+
|
53
|
+
## From CDN
|
54
|
+
|
55
|
+
Install from a content-delivery network (CDN) like in the above example.
|
56
|
+
|
57
|
+
From cdnjs:
|
58
|
+
|
59
|
+
```html
|
60
|
+
<script src="https://cdnjs.cloudflare.com/ajax/libs/tween.js/20.0.0/tween.umd.js"></script>
|
61
|
+
```
|
62
|
+
|
63
|
+
Or from unpkg.com:
|
64
|
+
|
65
|
+
```html
|
66
|
+
<script src="https://unpkg.com/@tweenjs/tween.js@^20.0.0/dist/tween.umd.js"></script>
|
38
67
|
```
|
39
68
|
|
40
|
-
|
69
|
+
Note that unpkg.com supports a semver version in the URL, where the `^` in the URL tells unpkg to give you the latest version 20.x.x.
|
41
70
|
|
42
|
-
##
|
71
|
+
## Build and include in your project with script tag
|
43
72
|
|
44
73
|
Currently npm is required to build the project.
|
45
74
|
|
46
75
|
```bash
|
47
76
|
git clone https://github.com/tweenjs/tween.js
|
48
77
|
cd tween.js
|
49
|
-
npm
|
78
|
+
npm install
|
50
79
|
npm run build
|
51
80
|
```
|
52
81
|
|
53
|
-
This will create some builds in the `dist` directory. There are currently
|
82
|
+
This will create some builds in the `dist` directory. There are currently two different builds of the library:
|
54
83
|
|
55
|
-
- UMD : tween.umd.js
|
56
|
-
-
|
57
|
-
- CommonJS : tween.cjs.js
|
58
|
-
- ES6 Module : tween.es.js
|
84
|
+
- UMD : `tween.umd.js`
|
85
|
+
- ES6 Module : `tween.es.js`
|
59
86
|
|
60
87
|
You are now able to copy tween.umd.js into your project, then include it with
|
61
|
-
a script tag
|
88
|
+
a script tag, which will add TWEEN to the global scope,
|
62
89
|
|
63
90
|
```html
|
64
|
-
<script src="
|
91
|
+
<script src="path/to/tween.umd.js"></script>
|
65
92
|
```
|
66
93
|
|
67
|
-
|
94
|
+
or import TWEEN as a JavaScript module,
|
95
|
+
|
96
|
+
```html
|
97
|
+
<script type="module">
|
98
|
+
import * as TWEEN from 'path/to/tween.es.js'
|
99
|
+
</script>
|
100
|
+
```
|
101
|
+
|
102
|
+
where `path/to` is replaced with the location where you placed the file.
|
103
|
+
|
104
|
+
## With `npm install` and `import` from `node_modules`
|
68
105
|
|
69
106
|
You can add tween.js as an npm dependency:
|
70
107
|
|
71
108
|
```bash
|
72
|
-
npm
|
109
|
+
npm install @tweenjs/tween.js
|
110
|
+
```
|
111
|
+
|
112
|
+
### With a build tool
|
113
|
+
|
114
|
+
If you are using [Node.js](https://nodejs.org/), [Parcel](https://parceljs.org/), [Webpack](https://webpack.js.org/), [Rollup](https://rollupjs.org/), [Vite](https://vitejs.dev/), or another build tool, then you can now use the following to include tween.js:
|
115
|
+
|
116
|
+
```javascript
|
117
|
+
import * as TWEEN from '@tweenjs/tween.js'
|
118
|
+
```
|
119
|
+
|
120
|
+
### Without a build tool
|
121
|
+
|
122
|
+
You can import from `node_modules` if you serve node_modules as part of your website, using an `importmap` script tag. First, assuming `node_modules` is at the root of your website, you can write an import map:
|
123
|
+
|
124
|
+
```html
|
125
|
+
<script type="importmap">
|
126
|
+
{
|
127
|
+
"imports": {
|
128
|
+
"@tweenjs/tween.js": "/node_modules/@tweenjs/tween.js/dist/tween.es.js"
|
129
|
+
}
|
130
|
+
}
|
131
|
+
</script>
|
73
132
|
```
|
74
133
|
|
75
|
-
|
134
|
+
Now in any of your module scripts you can import it by its package name:
|
76
135
|
|
77
136
|
```javascript
|
78
|
-
|
137
|
+
import * as TWEEN from '@tweenjs/tween.js'
|
79
138
|
```
|
80
139
|
|
81
|
-
|
140
|
+
# Features
|
82
141
|
|
83
142
|
- Does one thing and one thing only: tween properties
|
84
143
|
- Doesn't take care of CSS units (e.g. appending `px`)
|
85
|
-
- Doesn't interpolate
|
144
|
+
- Doesn't interpolate colors
|
86
145
|
- Easing functions are reusable outside of Tween
|
87
146
|
- Can also use custom easing functions
|
88
147
|
|
89
|
-
|
148
|
+
# Documentation
|
90
149
|
|
91
150
|
- [User guide](./docs/user_guide.md)
|
92
151
|
- [Contributor guide](./docs/contributor_guide.md)
|
93
152
|
- [Tutorial](http://learningthreejs.com/blog/2011/08/17/tweenjs-for-smooth-animation/) using tween.js with three.js
|
94
153
|
- Also: [libtween](https://github.com/jsm174/libtween), a port of tween.js to C by [jsm174](https://github.com/jsm174)
|
95
|
-
- Also: [es6-tween](https://github.com/tweenjs/es6-tween), a port of tween.js to ES6/Harmony by [dalisoft](https://github.com/dalisoft)
|
96
154
|
- [Understanding tween.js](https://mikebolt.me/article/understanding-tweenjs.html)
|
97
155
|
|
98
|
-
|
156
|
+
# Examples
|
99
157
|
|
100
158
|
<table>
|
101
159
|
<tr>
|
@@ -291,9 +349,9 @@ const TWEEN = require('@tweenjs/tween.js')
|
|
291
349
|
</tr>
|
292
350
|
</table>
|
293
351
|
|
294
|
-
|
352
|
+
# Tests
|
295
353
|
|
296
|
-
You need to install `npm` first--this comes with node.js, so install that one first. Then, cd to `tween.js`'s directory and run:
|
354
|
+
You need to install `npm` first--this comes with node.js, so install that one first. Then, cd to `tween.js`'s (or wherever you cloned the repo) directory and run:
|
297
355
|
|
298
356
|
```bash
|
299
357
|
npm install
|
@@ -305,16 +363,17 @@ To run the tests run:
|
|
305
363
|
npm test
|
306
364
|
```
|
307
365
|
|
308
|
-
If you want to add any feature or change existing features, you _must_ run the tests to make sure you didn't break anything else. Any pull request (PR) needs to have updated passing tests for feature changes (or new passing tests for new features) in `src/tests.ts
|
366
|
+
If you want to add any feature or change existing features, you _must_ run the tests to make sure you didn't break anything else. Any pull request (PR) needs to have updated passing tests for feature changes (or new passing tests for new features or fixes) in `src/tests.ts` a PR to be accepted. See [contributing](CONTRIBUTING.md) for more information.
|
309
367
|
|
310
|
-
|
368
|
+
# People
|
311
369
|
|
312
370
|
Maintainers: [mikebolt](https://github.com/mikebolt), [sole](https://github.com/sole), [Joe Pea (@trusktr)](https://github.com/trusktr).
|
313
371
|
|
314
372
|
[All contributors](http://github.com/tweenjs/tween.js/contributors).
|
315
373
|
|
316
|
-
|
374
|
+
# Projects using tween.js
|
317
375
|
|
376
|
+
[<img src="./assets/projects/11_lume.jpg" width="100" alt="Lume" />](https://lume.io)
|
318
377
|
[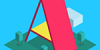](https://aframe.io)
|
319
378
|
[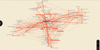](http://www.moma.org/interactives/exhibitions/2012/inventingabstraction/)
|
320
379
|
[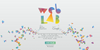](http://www.chromeweblab.com/)
|
package/dist/tween.amd.js
CHANGED
package/dist/tween.cjs.js
CHANGED
package/dist/tween.d.ts
CHANGED
package/dist/tween.esm.js
CHANGED
package/dist/tween.umd.js
CHANGED
package/package.json
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
{
|
2
2
|
"name": "@tweenjs/tween.js",
|
3
3
|
"description": "Super simple, fast and easy to use tweening engine which incorporates optimised Robert Penner's equations.",
|
4
|
-
"version": "20.0.
|
4
|
+
"version": "20.0.2",
|
5
5
|
"type": "module",
|
6
6
|
"main": "dist/tween.cjs.js",
|
7
7
|
"types": "dist/tween.d.ts",
|