@tweenjs/tween.js 19.0.0 → 20.0.1
Sign up to get free protection for your applications and to get access to all the features.
- package/README.md +218 -99
- package/dist/tween.amd.js +70 -71
- package/dist/tween.cjs.js +68 -69
- package/dist/tween.d.ts +44 -32
- package/dist/tween.esm.js +68 -70
- package/dist/tween.umd.js +70 -71
- package/package.json +7 -8
package/README.md
CHANGED
@@ -1,147 +1,265 @@
|
|
1
1
|
# tween.js
|
2
2
|
|
3
|
-
JavaScript tweening engine for easy animations, incorporating optimised Robert Penner's equations.
|
3
|
+
JavaScript (TypeScript) tweening engine for easy animations, incorporating optimised Robert Penner's equations.
|
4
4
|
|
5
5
|
[![NPM Version][npm-image]][npm-url]
|
6
6
|
[![CDNJS][cdnjs-image]][cdnjs-url]
|
7
7
|
[![NPM Downloads][downloads-image]][downloads-url]
|
8
8
|
[![Build and Tests][ci-image]][ci-url]
|
9
9
|
|
10
|
-
**Update Note** In v18 the script you should include has moved from `src/Tween.js` to `dist/tween.umd.js`. See the [installation section](#Installation) below.
|
11
|
-
|
12
10
|
---
|
13
11
|
|
14
|
-
```
|
15
|
-
|
16
|
-
|
17
|
-
box
|
18
|
-
|
19
|
-
|
20
|
-
|
21
|
-
|
22
|
-
|
12
|
+
```html
|
13
|
+
<script src="https://cdnjs.cloudflare.com/ajax/libs/tween.js/20.0.0/tween.umd.js"></script>
|
14
|
+
|
15
|
+
<div id="box"></div>
|
16
|
+
|
17
|
+
<style>
|
18
|
+
#box {
|
19
|
+
background-color: deeppink;
|
20
|
+
width: 100px;
|
21
|
+
height: 100px;
|
22
|
+
}
|
23
|
+
</style>
|
24
|
+
|
25
|
+
<script>
|
26
|
+
const box = document.getElementById('box') // Get the element we want to animate.
|
27
|
+
|
28
|
+
const coords = {x: 0, y: 0} // Start at (0, 0)
|
29
|
+
|
30
|
+
const tween = new TWEEN.Tween(coords, false) // Create a new tween that modifies 'coords'.
|
31
|
+
.to({x: 300, y: 200}, 1000) // Move to (300, 200) in 1 second.
|
32
|
+
.easing(TWEEN.Easing.Quadratic.InOut) // Use an easing function to make the animation smooth.
|
33
|
+
.onUpdate(() => {
|
34
|
+
// Called after tween.js updates 'coords'.
|
35
|
+
// Move 'box' to the position described by 'coords' with a CSS translation.
|
36
|
+
box.style.setProperty('transform', 'translate(' + coords.x + 'px, ' + coords.y + 'px)')
|
37
|
+
})
|
38
|
+
.start() // Start the tween immediately.
|
39
|
+
|
40
|
+
// Setup the animation loop.
|
41
|
+
function animate(time) {
|
42
|
+
tween.update(time)
|
43
|
+
requestAnimationFrame(animate)
|
44
|
+
}
|
23
45
|
requestAnimationFrame(animate)
|
24
|
-
|
25
|
-
|
26
|
-
|
27
|
-
|
28
|
-
|
29
|
-
|
30
|
-
|
31
|
-
|
32
|
-
|
33
|
-
|
34
|
-
|
35
|
-
|
36
|
-
|
37
|
-
|
46
|
+
</script>
|
47
|
+
```
|
48
|
+
|
49
|
+
[Try this example on CodePen](https://codepen.io/trusktr/pen/KKGaBVz?editors=1000)
|
50
|
+
|
51
|
+
# Installation
|
52
|
+
|
53
|
+
## From CDN
|
54
|
+
|
55
|
+
Install from a content-delivery network (CDN) like in the above example.
|
56
|
+
|
57
|
+
From cdnjs:
|
58
|
+
|
59
|
+
```html
|
60
|
+
<script src="https://cdnjs.cloudflare.com/ajax/libs/tween.js/20.0.0/tween.umd.js"></script>
|
38
61
|
```
|
39
62
|
|
40
|
-
|
63
|
+
Or from unpkg.com:
|
64
|
+
|
65
|
+
```html
|
66
|
+
<script src="https://unpkg.com/@tweenjs/tween.js@^20.0.0/dist/tween.umd.js"></script>
|
67
|
+
```
|
41
68
|
|
42
|
-
|
69
|
+
Note that unpkg.com supports a semver version in the URL, where the `^` in the URL tells unpkg to give you the latest version 20.x.x.
|
70
|
+
|
71
|
+
## Build and include in your project with script tag
|
43
72
|
|
44
73
|
Currently npm is required to build the project.
|
45
74
|
|
46
75
|
```bash
|
47
76
|
git clone https://github.com/tweenjs/tween.js
|
48
77
|
cd tween.js
|
49
|
-
npm
|
78
|
+
npm install
|
50
79
|
npm run build
|
51
80
|
```
|
52
81
|
|
53
|
-
This will create some builds in the `dist` directory. There are currently
|
82
|
+
This will create some builds in the `dist` directory. There are currently two different builds of the library:
|
54
83
|
|
55
|
-
- UMD : tween.umd.js
|
56
|
-
-
|
57
|
-
- CommonJS : tween.cjs.js
|
58
|
-
- ES6 Module : tween.es.js
|
84
|
+
- UMD : `tween.umd.js`
|
85
|
+
- ES6 Module : `tween.es.js`
|
59
86
|
|
60
87
|
You are now able to copy tween.umd.js into your project, then include it with
|
61
|
-
a script tag
|
88
|
+
a script tag, which will add TWEEN to the global scope,
|
62
89
|
|
63
90
|
```html
|
64
|
-
<script src="
|
91
|
+
<script src="path/to/tween.umd.js"></script>
|
65
92
|
```
|
66
93
|
|
67
|
-
|
94
|
+
or import TWEEN as a JavaScript module,
|
95
|
+
|
96
|
+
```html
|
97
|
+
<script type="module">
|
98
|
+
import * as TWEEN from 'path/to/tween.es.js'
|
99
|
+
</script>
|
100
|
+
```
|
101
|
+
|
102
|
+
where `path/to` is replaced with the location where you placed the file.
|
103
|
+
|
104
|
+
## With `npm install` and `import` from `node_modules`
|
68
105
|
|
69
106
|
You can add tween.js as an npm dependency:
|
70
107
|
|
71
108
|
```bash
|
72
|
-
npm
|
109
|
+
npm install @tweenjs/tween.js
|
73
110
|
```
|
74
111
|
|
75
|
-
|
112
|
+
### With a build tool
|
113
|
+
|
114
|
+
If you are using [Node.js](https://nodejs.org/), [Parcel](https://parceljs.org/), [Webpack](https://webpack.js.org/), [Rollup](https://rollupjs.org/), [Vite](https://vitejs.dev/), or another build tool, then you can now use the following to include tween.js:
|
76
115
|
|
77
116
|
```javascript
|
78
|
-
|
117
|
+
import * as TWEEN from '@tweenjs/tween.js'
|
118
|
+
```
|
119
|
+
|
120
|
+
### Without a build tool
|
121
|
+
|
122
|
+
You can import from `node_modules` if you serve node_modules as part of your website, using an `importmap` script tag. First, assuming `node_modules` is at the root of your website, you can write an import map:
|
123
|
+
|
124
|
+
```html
|
125
|
+
<script type="importmap">
|
126
|
+
{
|
127
|
+
"imports": {
|
128
|
+
"@tweenjs/tween.js": "/node_modules/@tweenjs/tween.js/dist/tween.es.js"
|
129
|
+
}
|
130
|
+
}
|
131
|
+
</script>
|
79
132
|
```
|
80
133
|
|
81
|
-
|
134
|
+
Now in any of your module scripts you can import it by its package name:
|
135
|
+
|
136
|
+
```javascript
|
137
|
+
import * as TWEEN from '@tweenjs/tween.js'
|
138
|
+
```
|
139
|
+
|
140
|
+
# Features
|
82
141
|
|
83
142
|
- Does one thing and one thing only: tween properties
|
84
143
|
- Doesn't take care of CSS units (e.g. appending `px`)
|
85
|
-
- Doesn't interpolate
|
144
|
+
- Doesn't interpolate colors
|
86
145
|
- Easing functions are reusable outside of Tween
|
87
146
|
- Can also use custom easing functions
|
88
147
|
|
89
|
-
|
148
|
+
# Documentation
|
90
149
|
|
91
150
|
- [User guide](./docs/user_guide.md)
|
92
151
|
- [Contributor guide](./docs/contributor_guide.md)
|
93
152
|
- [Tutorial](http://learningthreejs.com/blog/2011/08/17/tweenjs-for-smooth-animation/) using tween.js with three.js
|
94
153
|
- Also: [libtween](https://github.com/jsm174/libtween), a port of tween.js to C by [jsm174](https://github.com/jsm174)
|
95
|
-
- Also: [es6-tween](https://github.com/tweenjs/es6-tween), a port of tween.js to ES6/Harmony by [dalisoft](https://github.com/dalisoft)
|
96
154
|
- [Understanding tween.js](https://mikebolt.me/article/understanding-tweenjs.html)
|
97
155
|
|
98
|
-
|
156
|
+
# Examples
|
99
157
|
|
100
158
|
<table>
|
101
159
|
<tr>
|
102
160
|
<td>
|
103
|
-
<a href="http://tweenjs.github.io/tween.js/examples/
|
104
|
-
<img src="https://tweenjs.github.io/tween.js/assets/examples/
|
161
|
+
<a href="http://tweenjs.github.io/tween.js/examples/00_hello_world.html">
|
162
|
+
<img width="100" height="50" src="https://tweenjs.github.io/tween.js/assets/examples/00_hello_world.png" alt="hello world" />
|
105
163
|
</a>
|
106
164
|
</td>
|
107
165
|
<td>
|
108
|
-
|
109
|
-
(<a href="examples/
|
166
|
+
hello world<br />
|
167
|
+
(<a href="examples/00_hello_world.html">source</a>)
|
110
168
|
</td>
|
111
169
|
<td>
|
112
|
-
<a href="http://tweenjs.github.io/tween.js/examples/
|
113
|
-
<img src="https://tweenjs.github.io/tween.js/assets/examples/
|
170
|
+
<a href="http://tweenjs.github.io/tween.js/examples/01_bars.html">
|
171
|
+
<img width="100" height="50" src="https://tweenjs.github.io/tween.js/assets/examples/01_bars.png" alt="Bars" />
|
114
172
|
</a>
|
115
173
|
</td>
|
116
174
|
<td>
|
117
|
-
|
118
|
-
(<a href="examples/
|
175
|
+
Bars<br />
|
176
|
+
(<a href="examples/01_bars.html">source</a>)
|
177
|
+
</td>
|
178
|
+
<tr>
|
179
|
+
</tr>
|
180
|
+
<td>
|
181
|
+
<a href="http://tweenjs.github.io/tween.js/examples/02_black_and_red.html">
|
182
|
+
<img width="100" height="50" src="https://tweenjs.github.io/tween.js/assets/examples/02_black_and_red.png" alt="Black and red" />
|
183
|
+
</a>
|
184
|
+
</td>
|
185
|
+
<td>
|
186
|
+
Black and red<br />
|
187
|
+
(<a href="examples/02_black_and_red.html">source</a>)
|
188
|
+
</td>
|
189
|
+
<td>
|
190
|
+
<a href="http://tweenjs.github.io/tween.js/examples/03_graphs.html">
|
191
|
+
<img width="100" height="50" src="https://tweenjs.github.io/tween.js/assets/examples/03_graphs.png" alt="Graphs" />
|
192
|
+
</a>
|
193
|
+
</td>
|
194
|
+
<td>
|
195
|
+
Graphs<br />
|
196
|
+
(<a href="examples/03_graphs.html">source</a>)
|
119
197
|
</td>
|
120
198
|
</tr>
|
121
199
|
<tr>
|
122
200
|
<td>
|
123
|
-
<a href="http://tweenjs.github.io/tween.js/examples/
|
124
|
-
<img src="https://tweenjs.github.io/tween.js/assets/examples/
|
201
|
+
<a href="http://tweenjs.github.io/tween.js/examples/04_simplest.html">
|
202
|
+
<img width="100" height="50" src="https://tweenjs.github.io/tween.js/assets/examples/04_simplest.png" alt="Simplest possible example" />
|
125
203
|
</a>
|
126
204
|
</td>
|
127
205
|
<td>
|
128
|
-
|
129
|
-
(<a href="examples/
|
206
|
+
Simplest possible example<br />
|
207
|
+
(<a href="examples/04_simplest.html">source</a>)
|
130
208
|
</td>
|
131
209
|
<td>
|
132
|
-
<a href="http://tweenjs.github.io/tween.js/examples/
|
133
|
-
<img src="https://tweenjs.github.io/tween.js/assets/examples/
|
210
|
+
<a href="http://tweenjs.github.io/tween.js/examples/05_video_and_time.html">
|
211
|
+
<img width="100" height="50" src="https://tweenjs.github.io/tween.js/assets/examples/06_video_and_time.png" alt="Video and time" />
|
134
212
|
</a>
|
135
213
|
</td>
|
136
214
|
<td>
|
137
|
-
|
138
|
-
(<a href="examples/
|
215
|
+
Video and time<br />
|
216
|
+
(<a href="examples/05_video_and_time.html">source</a>)
|
217
|
+
</td>
|
218
|
+
</tr>
|
219
|
+
<tr>
|
220
|
+
<td>
|
221
|
+
<a href="http://tweenjs.github.io/tween.js/examples/06_array_interpolation.html">
|
222
|
+
<img width="100" height="50" src="https://tweenjs.github.io/tween.js/assets/examples/03_graphs.png" alt="Array interpolation" />
|
223
|
+
</a>
|
224
|
+
</td>
|
225
|
+
<td>
|
226
|
+
Array interpolation<br />
|
227
|
+
(<a href="examples/06_array_interpolation.html">source</a>)
|
228
|
+
</td>
|
229
|
+
<td>
|
230
|
+
<a href="http://tweenjs.github.io/tween.js/examples/07_dynamic_to.html">
|
231
|
+
<img width="100" height="50" src="https://tweenjs.github.io/tween.js/assets/examples/07_dynamic_to.png" alt="Dynamic to, object" />
|
232
|
+
</a>
|
233
|
+
</td>
|
234
|
+
<td>
|
235
|
+
Dynamic to, object<br />
|
236
|
+
(<a href="examples/07_dynamic_to.html">source</a>)
|
237
|
+
</td>
|
238
|
+
</tr>
|
239
|
+
<tr>
|
240
|
+
<td>
|
241
|
+
<a href="http://tweenjs.github.io/tween.js/examples/07a_dynamic_to_two_array_values.html">
|
242
|
+
<img width="100" height="50" src="https://tweenjs.github.io/tween.js/assets/examples/07a_dynamic_to.png" alt="Dynamic to, interpolation array" />
|
243
|
+
</a>
|
244
|
+
</td>
|
245
|
+
<td>
|
246
|
+
Dynamic to, interpolation array<br />
|
247
|
+
(<a href="examples/07a_dynamic_to_two_array_values.html">source</a>)
|
248
|
+
</td>
|
249
|
+
<td>
|
250
|
+
<a href="http://tweenjs.github.io/tween.js/examples/07b_dynamic_to_an_array_of_values.html">
|
251
|
+
<img width="100" height="50" src="https://tweenjs.github.io/tween.js/assets/examples/07b_dynamic_to.png" alt="Dynamic to, large interpolation array" />
|
252
|
+
</a>
|
253
|
+
</td>
|
254
|
+
<td>
|
255
|
+
Dynamic to, large interpolation array<br />
|
256
|
+
(<a href="examples/07b_dynamic_to_an_array_of_values.html">source</a>)
|
139
257
|
</td>
|
140
258
|
</tr>
|
141
259
|
<tr>
|
142
260
|
<td>
|
143
261
|
<a href="http://tweenjs.github.io/tween.js/examples/08_repeat.html">
|
144
|
-
<img src="https://tweenjs.github.io/tween.js/assets/examples/08_repeat.png" alt="Repeat" />
|
262
|
+
<img width="100" height="50" src="https://tweenjs.github.io/tween.js/assets/examples/08_repeat.png" alt="Repeat" />
|
145
263
|
</a>
|
146
264
|
</td>
|
147
265
|
<td>
|
@@ -149,91 +267,91 @@ const TWEEN = require('@tweenjs/tween.js')
|
|
149
267
|
(<a href="examples/08_repeat.html">source</a>)
|
150
268
|
</td>
|
151
269
|
<td>
|
152
|
-
<a href="http://tweenjs.github.io/tween.js/examples/
|
153
|
-
<img src="https://tweenjs.github.io/tween.js/assets/examples/
|
270
|
+
<a href="http://tweenjs.github.io/tween.js/examples/09_relative_values.html">
|
271
|
+
<img width="100" height="50" src="https://tweenjs.github.io/tween.js/assets/examples/09_relative.png" alt="Relative values" />
|
154
272
|
</a>
|
155
273
|
</td>
|
156
274
|
<td>
|
157
|
-
|
158
|
-
(<a href="examples/
|
275
|
+
Relative values<br />
|
276
|
+
(<a href="examples/09_relative_values.html">source</a>)
|
159
277
|
</td>
|
160
278
|
</tr>
|
161
279
|
<tr>
|
162
280
|
<td>
|
163
|
-
<a href="http://tweenjs.github.io/tween.js/examples/
|
164
|
-
<img src="https://tweenjs.github.io/tween.js/assets/examples/
|
281
|
+
<a href="http://tweenjs.github.io/tween.js/examples/10_yoyo.html">
|
282
|
+
<img width="100" height="50" src="https://tweenjs.github.io/tween.js/assets/examples/10_yoyo.png" alt="Yoyo" />
|
165
283
|
</a>
|
166
284
|
</td>
|
167
285
|
<td>
|
168
|
-
|
169
|
-
(<a href="examples/
|
286
|
+
Yoyo<br />
|
287
|
+
(<a href="examples/10_yoyo.html">source</a>)
|
170
288
|
</td>
|
171
289
|
<td>
|
172
|
-
<a href="http://tweenjs.github.io/tween.js/examples/
|
173
|
-
<img src="https://tweenjs.github.io/tween.js/assets/examples/
|
290
|
+
<a href="http://tweenjs.github.io/tween.js/examples/11_stop_all_chained_tweens.html">
|
291
|
+
<img width="100" height="50" src="https://tweenjs.github.io/tween.js/assets/examples/11_stop_all_chained_tweens.png" alt="Stop all chained tweens" />
|
174
292
|
</a>
|
175
293
|
</td>
|
176
294
|
<td>
|
177
|
-
|
178
|
-
(<a href="examples/
|
295
|
+
Stop all chained tweens<br />
|
296
|
+
(<a href="examples/11_stop_all_chained_tweens.html">source</a>)
|
179
297
|
</td>
|
180
298
|
</tr>
|
181
299
|
<tr>
|
182
300
|
<td>
|
183
|
-
<a href="http://tweenjs.github.io/tween.js/examples/
|
184
|
-
<img src="https://tweenjs.github.io/tween.js/assets/examples/
|
301
|
+
<a href="http://tweenjs.github.io/tween.js/examples/12_graphs_custom_functions.html">
|
302
|
+
<img width="100" height="50" src="https://tweenjs.github.io/tween.js/assets/examples/03_graphs.png" alt="Custom functions" />
|
185
303
|
</a>
|
186
304
|
</td>
|
187
305
|
<td>
|
188
|
-
|
189
|
-
(<a href="examples/
|
306
|
+
Custom functions<br />
|
307
|
+
(<a href="examples/12_graphs_custom_functions.html">source</a>)
|
190
308
|
</td>
|
191
309
|
<td>
|
192
|
-
<a href="http://tweenjs.github.io/tween.js/examples/
|
193
|
-
<img src="https://tweenjs.github.io/tween.js/assets/examples/
|
310
|
+
<a href="http://tweenjs.github.io/tween.js/examples/13_relative_start_time.html">
|
311
|
+
<img width="100" height="50" src="https://tweenjs.github.io/tween.js/assets/examples/13_relative_start_time.png" alt="Relative start time" />
|
194
312
|
</a>
|
195
313
|
</td>
|
196
314
|
<td>
|
197
|
-
|
198
|
-
(<a href="examples/
|
315
|
+
Relative start time<br />
|
316
|
+
(<a href="examples/13_relative_start_time.html">source</a>)
|
199
317
|
</td>
|
200
318
|
</tr>
|
201
319
|
<tr>
|
202
320
|
<td>
|
203
|
-
<a href="http://tweenjs.github.io/tween.js/examples/
|
204
|
-
<img src="https://tweenjs.github.io/tween.js/assets/examples/
|
321
|
+
<a href="http://tweenjs.github.io/tween.js/examples/14_pause_tween.html">
|
322
|
+
<img width="100" height="50" src="https://tweenjs.github.io/tween.js/assets/examples/14_pause_tween.png" alt="Pause tween" />
|
205
323
|
</a>
|
206
324
|
</td>
|
207
325
|
<td>
|
208
|
-
|
209
|
-
(<a href="examples/
|
326
|
+
Pause tween<br />
|
327
|
+
(<a href="examples/14_pause_tween.html">source</a>)
|
210
328
|
</td>
|
211
329
|
<td>
|
212
|
-
<a href="http://tweenjs.github.io/tween.js/examples/
|
213
|
-
<img src="https://tweenjs.github.io/tween.js/assets/examples/
|
330
|
+
<a href="http://tweenjs.github.io/tween.js/examples/15_complex_properties.html">
|
331
|
+
<img width="100" height="50" src="https://tweenjs.github.io/tween.js/assets/examples/15_complex_properties.png" alt="Complex properties" />
|
214
332
|
</a>
|
215
333
|
</td>
|
216
334
|
<td>
|
217
|
-
|
218
|
-
(<a href="examples/
|
335
|
+
Complex properties<br />
|
336
|
+
(<a href="examples/15_complex_properties.html">source</a>)
|
219
337
|
</td>
|
220
338
|
</tr>
|
221
339
|
<tr>
|
222
340
|
<td>
|
223
|
-
<a href="http://tweenjs.github.io/tween.js/examples/
|
224
|
-
<img src="https://tweenjs.github.io/tween.js/assets/examples/
|
341
|
+
<a href="http://tweenjs.github.io/tween.js/examples/16_animate_an_array_of_values.html">
|
342
|
+
<img width="100" height="50" src="https://tweenjs.github.io/tween.js/assets/examples/16_animate_an_array_of_values.png" alt="Animate an array of values" />
|
225
343
|
</a>
|
226
344
|
</td>
|
227
345
|
<td>
|
228
|
-
|
229
|
-
(<a href="examples/
|
346
|
+
Animate an array of values<br />
|
347
|
+
(<a href="examples/16_animate_an_array_of_values.html">source</a>)
|
230
348
|
</td>
|
231
349
|
</tr>
|
232
350
|
</table>
|
233
351
|
|
234
|
-
|
352
|
+
# Tests
|
235
353
|
|
236
|
-
You need to install `npm` first--this comes with node.js, so install that one first. Then, cd to `tween.js`'s directory and run:
|
354
|
+
You need to install `npm` first--this comes with node.js, so install that one first. Then, cd to `tween.js`'s (or wherever you cloned the repo) directory and run:
|
237
355
|
|
238
356
|
```bash
|
239
357
|
npm install
|
@@ -245,16 +363,17 @@ To run the tests run:
|
|
245
363
|
npm test
|
246
364
|
```
|
247
365
|
|
248
|
-
If you want to add any feature or change existing features, you _must_ run the tests to make sure you didn't break anything else. Any pull request (PR) needs to have updated passing tests for feature changes (or new passing tests for new features) in `src/tests.ts
|
366
|
+
If you want to add any feature or change existing features, you _must_ run the tests to make sure you didn't break anything else. Any pull request (PR) needs to have updated passing tests for feature changes (or new passing tests for new features or fixes) in `src/tests.ts` a PR to be accepted. See [contributing](CONTRIBUTING.md) for more information.
|
249
367
|
|
250
|
-
|
368
|
+
# People
|
251
369
|
|
252
370
|
Maintainers: [mikebolt](https://github.com/mikebolt), [sole](https://github.com/sole), [Joe Pea (@trusktr)](https://github.com/trusktr).
|
253
371
|
|
254
372
|
[All contributors](http://github.com/tweenjs/tween.js/contributors).
|
255
373
|
|
256
|
-
|
374
|
+
# Projects using tween.js
|
257
375
|
|
376
|
+
[](https://lume.io)
|
258
377
|
[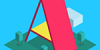](https://aframe.io)
|
259
378
|
[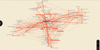](http://www.moma.org/interactives/exhibitions/2012/inventingabstraction/)
|
260
379
|
[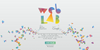](http://www.chromeweblab.com/)
|