@tweenjs/tween.js 17.2.0 → 17.4.0
Sign up to get free protection for your applications and to get access to all the features.
- package/.travis.yml +1 -1
- package/README_zh-CN.md +297 -0
- package/package-lock.json +11765 -0
- package/package.json +3 -3
- package/src/Tween.js +49 -32
- package/src/.backup1.Tween.js +0 -916
package/.travis.yml
CHANGED
package/README_zh-CN.md
ADDED
@@ -0,0 +1,297 @@
|
|
1
|
+
# tween.js
|
2
|
+
|
3
|
+
tween.js是用于简单动画的JavaScript补间引擎,结合了优化的 Robert Penner 方程。
|
4
|
+
|
5
|
+
[![NPM Version][npm-image]][npm-url]
|
6
|
+
[![NPM Downloads][downloads-image]][downloads-url]
|
7
|
+
[![Travis tests][travis-image]][travis-url]
|
8
|
+
[![Flattr this][flattr-image]][flattr-url]
|
9
|
+
[![CDNJS][cdnjs-image]][cdnjs-url]
|
10
|
+
|
11
|
+
```javascript
|
12
|
+
var box = document.createElement('div');
|
13
|
+
box.style.setProperty('background-color', '#008800');
|
14
|
+
box.style.setProperty('width', '100px');
|
15
|
+
box.style.setProperty('height', '100px');
|
16
|
+
document.body.appendChild(box);
|
17
|
+
|
18
|
+
// 设置循环动画
|
19
|
+
function animate(time) {
|
20
|
+
requestAnimationFrame(animate);
|
21
|
+
TWEEN.update(time);
|
22
|
+
}
|
23
|
+
requestAnimationFrame(animate);
|
24
|
+
|
25
|
+
var coords = { x: 0, y: 0 }; // 起始点 (0, 0)
|
26
|
+
var tween = new TWEEN.Tween(coords) // 创建一个新的tween用来改变 'coords'
|
27
|
+
.to({ x: 300, y: 200 }, 1000) // 在1s内移动至 (300, 200)
|
28
|
+
.easing(TWEEN.Easing.Quadratic.Out) // 使用缓动功能使的动画更加平滑
|
29
|
+
.onUpdate(function() { // 在 tween.js 更新 'coords' 后调用
|
30
|
+
// 将 'box' 移动到 'coords' 所描述的位置,配合 CSS 过渡
|
31
|
+
box.style.setProperty('transform', 'translate(' + coords.x + 'px, ' + coords.y + 'px)');
|
32
|
+
})
|
33
|
+
.start(); // 立即开始 tween
|
34
|
+
```
|
35
|
+
|
36
|
+
[在线代码测试](https://codepen.io/mikebolt/pen/zzzvZg)
|
37
|
+
|
38
|
+
## 安装
|
39
|
+
|
40
|
+
下载 [library](https://raw.githubusercontent.com/tweenjs/tween.js/master/src/Tween.js) 并将它引入至你的代码中:
|
41
|
+
|
42
|
+
```html
|
43
|
+
<script src="js/Tween.js"></script>
|
44
|
+
```
|
45
|
+
|
46
|
+
您也可以在代码中引用 CDN 托管的版本,这要感谢 cdnjs 。例如:
|
47
|
+
|
48
|
+
```html
|
49
|
+
<script src="https://cdnjs.cloudflare.com/ajax/libs/tween.js/16.3.5/Tween.min.js"></script>
|
50
|
+
```
|
51
|
+
|
52
|
+
See [tween.js](https://cdnjs.com/libraries/tween.js/) for more versions.
|
53
|
+
|
54
|
+
查看更多 [tween.js](https://cdnjs.com/libraries/tween.js/) 版本.
|
55
|
+
|
56
|
+
### 更多高级用户想要的...
|
57
|
+
|
58
|
+
#### 使用 `npm`
|
59
|
+
|
60
|
+
```bash
|
61
|
+
npm install @tweenjs/tween.js
|
62
|
+
```
|
63
|
+
|
64
|
+
然后用标准的 node.js `require` 包含 Tween.js 模块:
|
65
|
+
|
66
|
+
```javascript
|
67
|
+
var TWEEN = require('@tweenjs/tween.js');
|
68
|
+
```
|
69
|
+
|
70
|
+
您可以像所有其他示例一样使用Tween.js,例如:
|
71
|
+
|
72
|
+
```javascript
|
73
|
+
var t = new TWEEN.Tween( /* etc */ );
|
74
|
+
t.start();
|
75
|
+
```
|
76
|
+
|
77
|
+
你将需要使用诸如`browserify`之类的工具将使用此风格的代码转换为可以在浏览器中运行的代码(浏览器无法识别 `require`)
|
78
|
+
|
79
|
+
#### Use `bower`
|
80
|
+
|
81
|
+
```bash
|
82
|
+
bower install @tweenjs/tweenjs --save
|
83
|
+
```
|
84
|
+
|
85
|
+
或者安装特定的tag.他们是git tags,如果你已经在本地克隆仓库,你可以在命令行中运行`git tag`查看tag列表,或者你可以查看下 [tween.js tags page](https://github.com/tweenjs/tween.js/tags) 列表.例如,安装 `v16.3.0`:
|
86
|
+
|
87
|
+
```bash
|
88
|
+
bower install @tweenjs/tweenjs#v16.3.0
|
89
|
+
```
|
90
|
+
|
91
|
+
然后引入库源码:
|
92
|
+
|
93
|
+
```html
|
94
|
+
<script src="bower_components/@tweenjs/tweenjs/src/Tween.js"></script>
|
95
|
+
```
|
96
|
+
|
97
|
+
## Features
|
98
|
+
|
99
|
+
* 只做一件事且仅只做一件事: 补间特性
|
100
|
+
* 不关注CSS单位 (e.g. appending `px`)
|
101
|
+
* 不插入颜色
|
102
|
+
* 缓和功能可以在Tween之外重用
|
103
|
+
* 也可以使用自定义缓动功能
|
104
|
+
|
105
|
+
## Documentation
|
106
|
+
|
107
|
+
* [使用指南](./docs/user_guide_zh-CN.md)
|
108
|
+
* [贡献者指南](./docs/contributor_guide_zh-CN.md)
|
109
|
+
* [教程](http://learningthreejs.com/blog/2011/08/17/tweenjs-for-smooth-animation/) using tween.js with three.js
|
110
|
+
* 其他: [libtween](https://github.com/jsm174/libtween), [jsm174](https://github.com/jsm174) 写的一个C语言版本的 tween.js.
|
111
|
+
* 其他: [es6-tween](https://github.com/tweenjs/es6-tween), [dalisoft](https://github.com/dalisoft) 写的一个ES6/Harmony版本的 tween.js.
|
112
|
+
* [理解 tween.js](https://mikebolt.me/article/understanding-tweenjs.html)
|
113
|
+
|
114
|
+
## 示例
|
115
|
+
|
116
|
+
<table>
|
117
|
+
<tr>
|
118
|
+
<td>
|
119
|
+
<a href="http://tweenjs.github.io/tween.js/examples/12_graphs_custom_functions.html">
|
120
|
+
<img src="./assets/examples/03_graphs.png" alt="Custom functions" />
|
121
|
+
</a>
|
122
|
+
</td>
|
123
|
+
<td>
|
124
|
+
Custom functions<br />
|
125
|
+
(<a href="examples/12_graphs_custom_functions.html">source</a>)
|
126
|
+
</td>
|
127
|
+
<td>
|
128
|
+
<a href="http://tweenjs.github.io/tween.js/examples/11_stop_all_chained_tweens.html">
|
129
|
+
<img src="./assets/examples/11_stop_all_chained_tweens.png" alt="Stop all chained tweens" />
|
130
|
+
</a>
|
131
|
+
</td>
|
132
|
+
<td>
|
133
|
+
Stop all chained tweens<br />
|
134
|
+
(<a href="examples/11_stop_all_chained_tweens.html">source</a>)
|
135
|
+
</td>
|
136
|
+
</tr>
|
137
|
+
<tr>
|
138
|
+
<td>
|
139
|
+
<a href="http://tweenjs.github.io/tween.js/examples/10_yoyo.html">
|
140
|
+
<img src="./assets/examples/10_yoyo.png" alt="Yoyo" />
|
141
|
+
</a>
|
142
|
+
</td>
|
143
|
+
<td>
|
144
|
+
Yoyo<br />
|
145
|
+
(<a href="examples/10_yoyo.html">source</a>)
|
146
|
+
</td>
|
147
|
+
<td>
|
148
|
+
<a href="http://tweenjs.github.io/tween.js/examples/09_relative_values.html">
|
149
|
+
<img src="./assets/examples/09_relative.png" alt="Relative values" />
|
150
|
+
</a>
|
151
|
+
</td>
|
152
|
+
<td>
|
153
|
+
Relative values<br />
|
154
|
+
(<a href="examples/09_relative_values.html">source</a>)
|
155
|
+
</td>
|
156
|
+
</tr>
|
157
|
+
<tr>
|
158
|
+
<td>
|
159
|
+
<a href="http://tweenjs.github.io/tween.js/examples/08_repeat.html">
|
160
|
+
<img src="./assets/examples/08_repeat.png" alt="Repeat" />
|
161
|
+
</a>
|
162
|
+
</td>
|
163
|
+
<td>
|
164
|
+
Repeat<br />
|
165
|
+
(<a href="examples/08_repeat.html">source</a>)
|
166
|
+
</td>
|
167
|
+
<td>
|
168
|
+
<a href="http://tweenjs.github.io/tween.js/examples/07_dynamic_to.html">
|
169
|
+
<img src="./assets/examples/07_dynamic_to.png" alt="Dynamic to" />
|
170
|
+
</a>
|
171
|
+
</td>
|
172
|
+
<td>
|
173
|
+
Dynamic to<br />
|
174
|
+
(<a href="examples/07_dynamic_to.html">source</a>)
|
175
|
+
</td>
|
176
|
+
</tr>
|
177
|
+
<tr>
|
178
|
+
<td>
|
179
|
+
<a href="http://tweenjs.github.io/tween.js/examples/06_array_interpolation.html">
|
180
|
+
<img src="./assets/examples/03_graphs.png" alt="Array interpolation" />
|
181
|
+
</a>
|
182
|
+
</td>
|
183
|
+
<td>
|
184
|
+
Array interpolation<br />
|
185
|
+
(<a href="examples/06_array_interpolation.html">source</a>)
|
186
|
+
</td>
|
187
|
+
<td>
|
188
|
+
<a href="http://tweenjs.github.io/tween.js/examples/05_video_and_time.html">
|
189
|
+
<img src="./assets/examples/06_video_and_time.png" alt="Video and time" />
|
190
|
+
</a>
|
191
|
+
</td>
|
192
|
+
<td>
|
193
|
+
Video and time<br />
|
194
|
+
(<a href="examples/05_video_and_time.html">source</a>)
|
195
|
+
</td>
|
196
|
+
</tr>
|
197
|
+
<tr>
|
198
|
+
<td>
|
199
|
+
<a href="http://tweenjs.github.io/tween.js/examples/04_simplest.html">
|
200
|
+
<img src="./assets/examples/04_simplest.png" alt="Simplest possible example" />
|
201
|
+
</a>
|
202
|
+
</td>
|
203
|
+
<td>
|
204
|
+
Simplest possible example<br />
|
205
|
+
(<a href="examples/04_simplest.html">source</a>)
|
206
|
+
</td>
|
207
|
+
<td>
|
208
|
+
<a href="http://tweenjs.github.io/tween.js/examples/03_graphs.html">
|
209
|
+
<img src="./assets/examples/03_graphs.png" alt="Graphs" />
|
210
|
+
</a>
|
211
|
+
</td>
|
212
|
+
<td>
|
213
|
+
Graphs<br />
|
214
|
+
(<a href="examples/03_graphs.html">source</a>)
|
215
|
+
</td>
|
216
|
+
</tr>
|
217
|
+
<tr>
|
218
|
+
<td>
|
219
|
+
<a href="http://tweenjs.github.io/tween.js/examples/02_black_and_red.html">
|
220
|
+
<img src="./assets/examples/02_black_and_red.png" alt="Black and red" />
|
221
|
+
</a>
|
222
|
+
</td>
|
223
|
+
<td>
|
224
|
+
Black and red<br />
|
225
|
+
(<a href="examples/02_black_and_red.html">source</a>)
|
226
|
+
</td>
|
227
|
+
<td>
|
228
|
+
<a href="http://tweenjs.github.io/tween.js/examples/01_bars.html">
|
229
|
+
<img src="./assets/examples/01_bars.png" alt="Bars" />
|
230
|
+
</a>
|
231
|
+
</td>
|
232
|
+
<td>
|
233
|
+
Bars<br />
|
234
|
+
(<a href="examples/01_bars.html">source</a>)
|
235
|
+
</td>
|
236
|
+
</tr>
|
237
|
+
<tr>
|
238
|
+
<td>
|
239
|
+
<a href="http://tweenjs.github.io/tween.js/examples/00_hello_world.html">
|
240
|
+
<img src="./assets/examples/00_hello_world.png" alt="hello world" />
|
241
|
+
</a>
|
242
|
+
</td>
|
243
|
+
<td>
|
244
|
+
hello world<br />
|
245
|
+
(<a href="examples/00_hello_world.html">source</a>)
|
246
|
+
</td>
|
247
|
+
</tr>
|
248
|
+
</table>
|
249
|
+
|
250
|
+
## Tests
|
251
|
+
|
252
|
+
你首先需要安装`npm`--基于node.js,所以首先安装它.然后,进入到`tween.js`的目录下并运行:
|
253
|
+
|
254
|
+
```bash
|
255
|
+
npm install
|
256
|
+
```
|
257
|
+
|
258
|
+
如果是第一次运行测试,则为运行测试安装额外的依赖,然后运行
|
259
|
+
|
260
|
+
```bash
|
261
|
+
npm test
|
262
|
+
```
|
263
|
+
|
264
|
+
每次你想运行测试.
|
265
|
+
|
266
|
+
如果你想添加任何功能或改变现有的功能,你*必须*运行测试,以确保你没有影响别的东西.如果你发一个pull request(PR)添加新的东西,它没有测试,或测试不通过,这个PR将不被接受.更详细的请看 [contributing](CONTRIBUTING.md).
|
267
|
+
|
268
|
+
## People
|
269
|
+
|
270
|
+
维护者: [mikebolt](https://github.com/mikebolt), [sole](https://github.com/sole).
|
271
|
+
|
272
|
+
[所有贡献者](http://github.com/tweenjs/tween.js/contributors).
|
273
|
+
|
274
|
+
## 使用 tween.js 的项目
|
275
|
+
|
276
|
+
[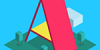](https://aframe.io)
|
277
|
+
[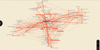](http://www.moma.org/interactives/exhibitions/2012/inventingabstraction/)
|
278
|
+
[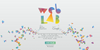](http://www.chromeweblab.com/)
|
279
|
+
[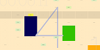](http://5013.es/toys/macchina)
|
280
|
+
[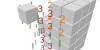](http://egraether.com/mine3d/)
|
281
|
+
[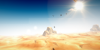](http://ro.me)
|
282
|
+
[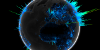](http://data-arts.appspot.com/globe)
|
283
|
+
[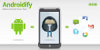](http://www.androidify.com/)
|
284
|
+
[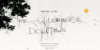](http://thewildernessdowntown.com/)
|
285
|
+
[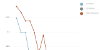](http://dejavis.org/linechart)
|
286
|
+
|
287
|
+
[npm-image]: https://img.shields.io/npm/v/@tweenjs/tween.js.svg
|
288
|
+
[npm-url]: https://npmjs.org/package/@tweenjs/tween.js
|
289
|
+
[downloads-image]: https://img.shields.io/npm/dm/@tweenjs/tween.js.svg
|
290
|
+
[downloads-url]: https://npmjs.org/package/@tweenjs/tween.js
|
291
|
+
[travis-image]: https://travis-ci.org/tweenjs/tween.js.svg?branch=master
|
292
|
+
[travis-url]: https://travis-ci.org/tweenjs/tween.js
|
293
|
+
[flattr-image]: https://api.flattr.com/button/flattr-badge-large.png
|
294
|
+
[flattr-url]: https://flattr.com/thing/45014/tween-js
|
295
|
+
[cdnjs-image]: https://img.shields.io/cdnjs/v/tween.js.svg
|
296
|
+
[cdnjs-url]: https://cdnjs.com/libraries/tween.js
|
297
|
+
|