@tsparticles/effect-trail 3.0.0-beta.4
This diff represents the content of publicly available package versions that have been released to one of the supported registries. The information contained in this diff is provided for informational purposes only and reflects changes between package versions as they appear in their respective public registries.
- package/LICENSE +21 -0
- package/README.md +75 -0
- package/browser/TrailDrawer.js +53 -0
- package/browser/index.js +4 -0
- package/browser/package.json +1 -0
- package/cjs/TrailDrawer.js +57 -0
- package/cjs/index.js +8 -0
- package/cjs/package.json +1 -0
- package/esm/TrailDrawer.js +53 -0
- package/esm/index.js +4 -0
- package/esm/package.json +1 -0
- package/package.json +108 -0
- package/report.html +39 -0
- package/tsparticles.effect.trail.js +167 -0
- package/tsparticles.effect.trail.min.js +2 -0
- package/tsparticles.effect.trail.min.js.LICENSE.txt +1 -0
- package/types/TrailDrawer.d.ts +16 -0
- package/types/index.d.ts +2 -0
- package/umd/TrailDrawer.js +67 -0
- package/umd/index.js +18 -0
package/LICENSE
ADDED
@@ -0,0 +1,21 @@
|
|
1
|
+
MIT License
|
2
|
+
|
3
|
+
Copyright (c) 2020 Matteo Bruni
|
4
|
+
|
5
|
+
Permission is hereby granted, free of charge, to any person obtaining a copy
|
6
|
+
of this software and associated documentation files (the "Software"), to deal
|
7
|
+
in the Software without restriction, including without limitation the rights
|
8
|
+
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
9
|
+
copies of the Software, and to permit persons to whom the Software is
|
10
|
+
furnished to do so, subject to the following conditions:
|
11
|
+
|
12
|
+
The above copyright notice and this permission notice shall be included in all
|
13
|
+
copies or substantial portions of the Software.
|
14
|
+
|
15
|
+
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
16
|
+
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
17
|
+
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
18
|
+
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
19
|
+
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
20
|
+
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
21
|
+
SOFTWARE.
|
package/README.md
ADDED
@@ -0,0 +1,75 @@
|
|
1
|
+
[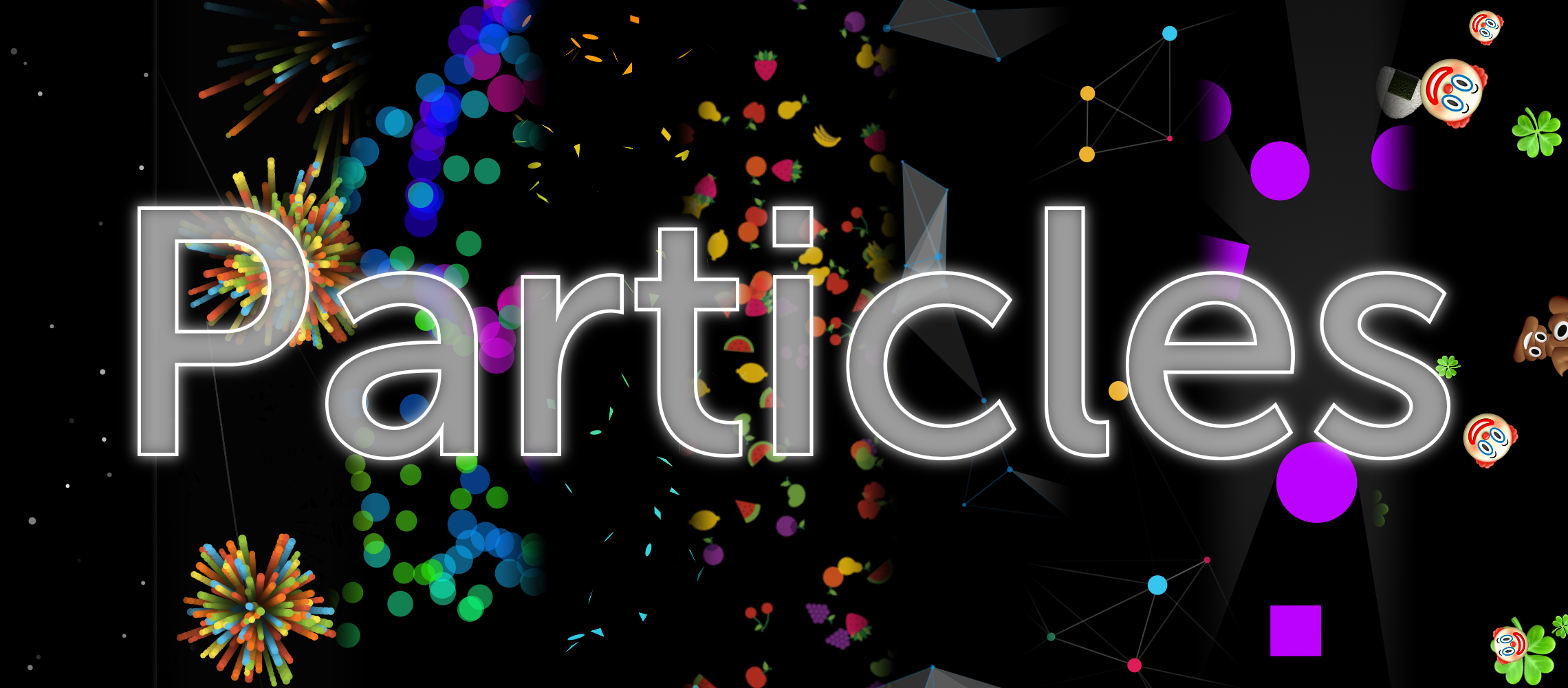](https://particles.js.org)
|
2
|
+
|
3
|
+
# tsParticles Trail Effect
|
4
|
+
|
5
|
+
[](https://www.jsdelivr.com/package/npm/@tsparticles/effect-trail)
|
6
|
+
[](https://www.npmjs.com/package/@tsparticles/effect-trail)
|
7
|
+
[](https://www.npmjs.com/package/@tsparticles/effect-trail) [](https://github.com/sponsors/matteobruni)
|
8
|
+
|
9
|
+
[tsParticles](https://github.com/tsparticles/tsparticles) additional trail effect.
|
10
|
+
|
11
|
+
## How to use it
|
12
|
+
|
13
|
+
### CDN / Vanilla JS / jQuery
|
14
|
+
|
15
|
+
The CDN/Vanilla version JS has one required file in vanilla configuration:
|
16
|
+
|
17
|
+
Including the `tsparticles.effect.trail.min.js` file will export the function to load the effect:
|
18
|
+
|
19
|
+
```text
|
20
|
+
loadTrailEffect
|
21
|
+
```
|
22
|
+
|
23
|
+
### Usage
|
24
|
+
|
25
|
+
Once the scripts are loaded you can set up `tsParticles` and the effect like this:
|
26
|
+
|
27
|
+
```javascript
|
28
|
+
(async () => {
|
29
|
+
await loadTrailEffect(tsParticles);
|
30
|
+
|
31
|
+
await tsParticles.load({
|
32
|
+
id: "tsparticles",
|
33
|
+
options: {
|
34
|
+
/* options */
|
35
|
+
/* here you can use particles.effect.type: "trail" */
|
36
|
+
},
|
37
|
+
});
|
38
|
+
})();
|
39
|
+
```
|
40
|
+
|
41
|
+
### ESM / CommonJS
|
42
|
+
|
43
|
+
This package is compatible also with ES or CommonJS modules, firstly this needs to be installed, like this:
|
44
|
+
|
45
|
+
```shell
|
46
|
+
$ npm install @tsparticles/effect-trail
|
47
|
+
```
|
48
|
+
|
49
|
+
or
|
50
|
+
|
51
|
+
```shell
|
52
|
+
$ yarn add @tsparticles/effect-trail
|
53
|
+
```
|
54
|
+
|
55
|
+
Then you need to import it in the app, like this:
|
56
|
+
|
57
|
+
```javascript
|
58
|
+
const { tsParticles } = require("@tsparticles/engine");
|
59
|
+
const { loadTrailEffect } = require("@tsparticles/effect-trail");
|
60
|
+
|
61
|
+
(async () => {
|
62
|
+
await loadTrailEffect(tsParticles);
|
63
|
+
})();
|
64
|
+
```
|
65
|
+
|
66
|
+
or
|
67
|
+
|
68
|
+
```javascript
|
69
|
+
import { tsParticles } from "@tsparticles/engine";
|
70
|
+
import { loadTrailEffect } from "@tsparticles/effect-trail";
|
71
|
+
|
72
|
+
(async () => {
|
73
|
+
await loadTrailEffect(tsParticles);
|
74
|
+
})();
|
75
|
+
```
|
@@ -0,0 +1,53 @@
|
|
1
|
+
import { getDistance, getRangeValue, } from "@tsparticles/engine";
|
2
|
+
export class TrailDrawer {
|
3
|
+
draw(data) {
|
4
|
+
const { context, radius, particle } = data, pxRatio = particle.container.retina.pixelRatio, currentPos = particle.getPosition();
|
5
|
+
if (!particle.trail || !particle.trailLength) {
|
6
|
+
return;
|
7
|
+
}
|
8
|
+
const pathLength = particle.trailLength;
|
9
|
+
particle.trail.push({
|
10
|
+
color: context.fillStyle ?? context.strokeStyle,
|
11
|
+
position: {
|
12
|
+
x: currentPos.x,
|
13
|
+
y: currentPos.y,
|
14
|
+
},
|
15
|
+
});
|
16
|
+
if (particle.trail.length < 2) {
|
17
|
+
return;
|
18
|
+
}
|
19
|
+
if (particle.trail.length > pathLength) {
|
20
|
+
particle.trail.shift();
|
21
|
+
}
|
22
|
+
const trailLength = Math.min(particle.trail.length, pathLength), offsetPos = {
|
23
|
+
x: currentPos.x,
|
24
|
+
y: currentPos.y,
|
25
|
+
};
|
26
|
+
let lastPos = particle.trail[trailLength - 1].position;
|
27
|
+
for (let i = trailLength; i > 0; i--) {
|
28
|
+
const step = particle.trail[i - 1], position = step.position;
|
29
|
+
if (getDistance(lastPos, position) > radius * 2) {
|
30
|
+
continue;
|
31
|
+
}
|
32
|
+
context.beginPath();
|
33
|
+
context.moveTo(lastPos.x - offsetPos.x, lastPos.y - offsetPos.y);
|
34
|
+
context.lineTo(position.x - offsetPos.x, position.y - offsetPos.y);
|
35
|
+
const width = Math.max((i / trailLength) * radius * 2, pxRatio, particle.trailMinWidth ?? -1);
|
36
|
+
context.lineWidth = particle.trailMaxWidth ? Math.min(width, particle.trailMaxWidth) : width;
|
37
|
+
context.strokeStyle = step.color;
|
38
|
+
context.stroke();
|
39
|
+
lastPos = position;
|
40
|
+
}
|
41
|
+
}
|
42
|
+
particleInit(container, particle) {
|
43
|
+
particle.trail = [];
|
44
|
+
const effectData = particle.effectData;
|
45
|
+
particle.trailLength = getRangeValue(effectData?.length ?? 10) * container.retina.pixelRatio;
|
46
|
+
particle.trailMaxWidth = effectData?.maxWidth
|
47
|
+
? getRangeValue(effectData.maxWidth) * container.retina.pixelRatio
|
48
|
+
: undefined;
|
49
|
+
particle.trailMinWidth = effectData?.minWidth
|
50
|
+
? getRangeValue(effectData.minWidth) * container.retina.pixelRatio
|
51
|
+
: undefined;
|
52
|
+
}
|
53
|
+
}
|
package/browser/index.js
ADDED
@@ -0,0 +1 @@
|
|
1
|
+
{ "type": "module" }
|
@@ -0,0 +1,57 @@
|
|
1
|
+
"use strict";
|
2
|
+
Object.defineProperty(exports, "__esModule", { value: true });
|
3
|
+
exports.TrailDrawer = void 0;
|
4
|
+
const engine_1 = require("@tsparticles/engine");
|
5
|
+
class TrailDrawer {
|
6
|
+
draw(data) {
|
7
|
+
const { context, radius, particle } = data, pxRatio = particle.container.retina.pixelRatio, currentPos = particle.getPosition();
|
8
|
+
if (!particle.trail || !particle.trailLength) {
|
9
|
+
return;
|
10
|
+
}
|
11
|
+
const pathLength = particle.trailLength;
|
12
|
+
particle.trail.push({
|
13
|
+
color: context.fillStyle ?? context.strokeStyle,
|
14
|
+
position: {
|
15
|
+
x: currentPos.x,
|
16
|
+
y: currentPos.y,
|
17
|
+
},
|
18
|
+
});
|
19
|
+
if (particle.trail.length < 2) {
|
20
|
+
return;
|
21
|
+
}
|
22
|
+
if (particle.trail.length > pathLength) {
|
23
|
+
particle.trail.shift();
|
24
|
+
}
|
25
|
+
const trailLength = Math.min(particle.trail.length, pathLength), offsetPos = {
|
26
|
+
x: currentPos.x,
|
27
|
+
y: currentPos.y,
|
28
|
+
};
|
29
|
+
let lastPos = particle.trail[trailLength - 1].position;
|
30
|
+
for (let i = trailLength; i > 0; i--) {
|
31
|
+
const step = particle.trail[i - 1], position = step.position;
|
32
|
+
if ((0, engine_1.getDistance)(lastPos, position) > radius * 2) {
|
33
|
+
continue;
|
34
|
+
}
|
35
|
+
context.beginPath();
|
36
|
+
context.moveTo(lastPos.x - offsetPos.x, lastPos.y - offsetPos.y);
|
37
|
+
context.lineTo(position.x - offsetPos.x, position.y - offsetPos.y);
|
38
|
+
const width = Math.max((i / trailLength) * radius * 2, pxRatio, particle.trailMinWidth ?? -1);
|
39
|
+
context.lineWidth = particle.trailMaxWidth ? Math.min(width, particle.trailMaxWidth) : width;
|
40
|
+
context.strokeStyle = step.color;
|
41
|
+
context.stroke();
|
42
|
+
lastPos = position;
|
43
|
+
}
|
44
|
+
}
|
45
|
+
particleInit(container, particle) {
|
46
|
+
particle.trail = [];
|
47
|
+
const effectData = particle.effectData;
|
48
|
+
particle.trailLength = (0, engine_1.getRangeValue)(effectData?.length ?? 10) * container.retina.pixelRatio;
|
49
|
+
particle.trailMaxWidth = effectData?.maxWidth
|
50
|
+
? (0, engine_1.getRangeValue)(effectData.maxWidth) * container.retina.pixelRatio
|
51
|
+
: undefined;
|
52
|
+
particle.trailMinWidth = effectData?.minWidth
|
53
|
+
? (0, engine_1.getRangeValue)(effectData.minWidth) * container.retina.pixelRatio
|
54
|
+
: undefined;
|
55
|
+
}
|
56
|
+
}
|
57
|
+
exports.TrailDrawer = TrailDrawer;
|
package/cjs/index.js
ADDED
@@ -0,0 +1,8 @@
|
|
1
|
+
"use strict";
|
2
|
+
Object.defineProperty(exports, "__esModule", { value: true });
|
3
|
+
exports.loadTrailEffect = void 0;
|
4
|
+
const TrailDrawer_js_1 = require("./TrailDrawer.js");
|
5
|
+
async function loadTrailEffect(engine, refresh = true) {
|
6
|
+
await engine.addEffect("trail", new TrailDrawer_js_1.TrailDrawer(), refresh);
|
7
|
+
}
|
8
|
+
exports.loadTrailEffect = loadTrailEffect;
|
package/cjs/package.json
ADDED
@@ -0,0 +1 @@
|
|
1
|
+
{ "type": "commonjs" }
|
@@ -0,0 +1,53 @@
|
|
1
|
+
import { getDistance, getRangeValue, } from "@tsparticles/engine";
|
2
|
+
export class TrailDrawer {
|
3
|
+
draw(data) {
|
4
|
+
const { context, radius, particle } = data, pxRatio = particle.container.retina.pixelRatio, currentPos = particle.getPosition();
|
5
|
+
if (!particle.trail || !particle.trailLength) {
|
6
|
+
return;
|
7
|
+
}
|
8
|
+
const pathLength = particle.trailLength;
|
9
|
+
particle.trail.push({
|
10
|
+
color: context.fillStyle ?? context.strokeStyle,
|
11
|
+
position: {
|
12
|
+
x: currentPos.x,
|
13
|
+
y: currentPos.y,
|
14
|
+
},
|
15
|
+
});
|
16
|
+
if (particle.trail.length < 2) {
|
17
|
+
return;
|
18
|
+
}
|
19
|
+
if (particle.trail.length > pathLength) {
|
20
|
+
particle.trail.shift();
|
21
|
+
}
|
22
|
+
const trailLength = Math.min(particle.trail.length, pathLength), offsetPos = {
|
23
|
+
x: currentPos.x,
|
24
|
+
y: currentPos.y,
|
25
|
+
};
|
26
|
+
let lastPos = particle.trail[trailLength - 1].position;
|
27
|
+
for (let i = trailLength; i > 0; i--) {
|
28
|
+
const step = particle.trail[i - 1], position = step.position;
|
29
|
+
if (getDistance(lastPos, position) > radius * 2) {
|
30
|
+
continue;
|
31
|
+
}
|
32
|
+
context.beginPath();
|
33
|
+
context.moveTo(lastPos.x - offsetPos.x, lastPos.y - offsetPos.y);
|
34
|
+
context.lineTo(position.x - offsetPos.x, position.y - offsetPos.y);
|
35
|
+
const width = Math.max((i / trailLength) * radius * 2, pxRatio, particle.trailMinWidth ?? -1);
|
36
|
+
context.lineWidth = particle.trailMaxWidth ? Math.min(width, particle.trailMaxWidth) : width;
|
37
|
+
context.strokeStyle = step.color;
|
38
|
+
context.stroke();
|
39
|
+
lastPos = position;
|
40
|
+
}
|
41
|
+
}
|
42
|
+
particleInit(container, particle) {
|
43
|
+
particle.trail = [];
|
44
|
+
const effectData = particle.effectData;
|
45
|
+
particle.trailLength = getRangeValue(effectData?.length ?? 10) * container.retina.pixelRatio;
|
46
|
+
particle.trailMaxWidth = effectData?.maxWidth
|
47
|
+
? getRangeValue(effectData.maxWidth) * container.retina.pixelRatio
|
48
|
+
: undefined;
|
49
|
+
particle.trailMinWidth = effectData?.minWidth
|
50
|
+
? getRangeValue(effectData.minWidth) * container.retina.pixelRatio
|
51
|
+
: undefined;
|
52
|
+
}
|
53
|
+
}
|
package/esm/index.js
ADDED
package/esm/package.json
ADDED
@@ -0,0 +1 @@
|
|
1
|
+
{ "type": "module" }
|
package/package.json
ADDED
@@ -0,0 +1,108 @@
|
|
1
|
+
{
|
2
|
+
"name": "@tsparticles/effect-trail",
|
3
|
+
"version": "3.0.0-beta.4",
|
4
|
+
"description": "tsParticles trail effect",
|
5
|
+
"homepage": "https://particles.js.org",
|
6
|
+
"repository": {
|
7
|
+
"type": "git",
|
8
|
+
"url": "git+https://github.com/tsparticles/tsparticles.git",
|
9
|
+
"directory": "effects/trail"
|
10
|
+
},
|
11
|
+
"keywords": [
|
12
|
+
"front-end",
|
13
|
+
"frontend",
|
14
|
+
"tsparticles",
|
15
|
+
"particles.js",
|
16
|
+
"particlesjs",
|
17
|
+
"particles",
|
18
|
+
"particle",
|
19
|
+
"canvas",
|
20
|
+
"jsparticles",
|
21
|
+
"xparticles",
|
22
|
+
"particles-js",
|
23
|
+
"particles-bg",
|
24
|
+
"particles-bg-vue",
|
25
|
+
"particles-ts",
|
26
|
+
"particles.ts",
|
27
|
+
"react-particles-js",
|
28
|
+
"react-particles.js",
|
29
|
+
"react-particles",
|
30
|
+
"react",
|
31
|
+
"reactjs",
|
32
|
+
"vue-particles",
|
33
|
+
"ngx-particles",
|
34
|
+
"angular-particles",
|
35
|
+
"particleground",
|
36
|
+
"vue",
|
37
|
+
"vuejs",
|
38
|
+
"preact",
|
39
|
+
"preactjs",
|
40
|
+
"jquery",
|
41
|
+
"angularjs",
|
42
|
+
"angular",
|
43
|
+
"typescript",
|
44
|
+
"javascript",
|
45
|
+
"animation",
|
46
|
+
"web",
|
47
|
+
"html5",
|
48
|
+
"web-design",
|
49
|
+
"webdesign",
|
50
|
+
"css",
|
51
|
+
"html",
|
52
|
+
"css3",
|
53
|
+
"animated",
|
54
|
+
"background",
|
55
|
+
"confetti",
|
56
|
+
"canvas",
|
57
|
+
"fireworks",
|
58
|
+
"fireworks-js",
|
59
|
+
"confetti-js",
|
60
|
+
"confettijs",
|
61
|
+
"fireworksjs",
|
62
|
+
"canvas-confetti",
|
63
|
+
"tsparticles-effect"
|
64
|
+
],
|
65
|
+
"author": "Matteo Bruni <matteo.bruni@me.com>",
|
66
|
+
"license": "MIT",
|
67
|
+
"bugs": {
|
68
|
+
"url": "https://github.com/tsparticles/tsparticles/issues"
|
69
|
+
},
|
70
|
+
"funding": [
|
71
|
+
{
|
72
|
+
"type": "github",
|
73
|
+
"url": "https://github.com/sponsors/matteobruni"
|
74
|
+
},
|
75
|
+
{
|
76
|
+
"type": "github",
|
77
|
+
"url": "https://github.com/sponsors/tsparticles"
|
78
|
+
},
|
79
|
+
{
|
80
|
+
"type": "buymeacoffee",
|
81
|
+
"url": "https://www.buymeacoffee.com/matteobruni"
|
82
|
+
}
|
83
|
+
],
|
84
|
+
"sideEffects": false,
|
85
|
+
"jsdelivr": "tsparticles.effect.trail.min.js",
|
86
|
+
"unpkg": "tsparticles.effect.trail.min.js",
|
87
|
+
"browser": "browser/index.js",
|
88
|
+
"main": "cjs/index.js",
|
89
|
+
"module": "esm/index.js",
|
90
|
+
"types": "types/index.d.ts",
|
91
|
+
"exports": {
|
92
|
+
".": {
|
93
|
+
"types": "./types/index.d.ts",
|
94
|
+
"browser": "./browser/index.js",
|
95
|
+
"import": "./esm/index.js",
|
96
|
+
"require": "./cjs/index.js",
|
97
|
+
"umd": "./umd/index.js",
|
98
|
+
"default": "./cjs/index.js"
|
99
|
+
},
|
100
|
+
"./package.json": "./package.json"
|
101
|
+
},
|
102
|
+
"dependencies": {
|
103
|
+
"@tsparticles/engine": "^3.0.0-beta.4"
|
104
|
+
},
|
105
|
+
"publishConfig": {
|
106
|
+
"access": "public"
|
107
|
+
}
|
108
|
+
}
|