@pdfme/common 3.1.5 → 3.2.0
This diff represents the content of publicly available package versions that have been released to one of the supported registries. The information contained in this diff is provided for informational purposes only and reflects changes between package versions as they appear in their respective public registries.
- package/README.md +303 -0
- package/dist/cjs/src/index.js.map +1 -1
- package/dist/cjs/src/schema.js +9 -2
- package/dist/cjs/src/schema.js.map +1 -1
- package/dist/esm/src/index.js.map +1 -1
- package/dist/esm/src/schema.js +8 -1
- package/dist/esm/src/schema.js.map +1 -1
- package/dist/types/src/index.d.ts +2 -2
- package/dist/types/src/schema.d.ts +110 -19
- package/dist/types/src/types.d.ts +6 -5
- package/package.json +3 -3
- package/src/index.ts +2 -0
- package/src/schema.ts +8 -1
- package/src/types.ts +22 -6
package/README.md
ADDED
@@ -0,0 +1,303 @@
|
|
1
|
+
# PDFME
|
2
|
+
|
3
|
+
<p>
|
4
|
+
<a href="https://github.com/pdfme/pdfme/blob/master/LICENSE.md">
|
5
|
+
<img src="https://img.shields.io/badge/license-MIT-blue.svg" alt="pdfme is released under the MIT license." />
|
6
|
+
</a>
|
7
|
+
<a href="https://github.com/pdfme/pdfme/actions/workflows/nodejs.yml">
|
8
|
+
<img src="https://github.com/pdfme/pdfme/workflows/Unit%20Testing/badge.svg" alt="Unit Testing status" />
|
9
|
+
</a>
|
10
|
+
<a href="https://www.npmjs.com/package/@pdfme/common">
|
11
|
+
<img src="https://img.shields.io/npm/v/@pdfme/common.svg" alt="Current npm package version." />
|
12
|
+
</a>
|
13
|
+
<a href="https://npmcharts.com/compare/@pdfme/common?minimal=true">
|
14
|
+
<img src="https://img.shields.io/npm/dm/@pdfme/common.svg" alt="Downloads per month on npm." />
|
15
|
+
</a>
|
16
|
+
<a href="https://pdfme.com/docs/development-guide#contribution">
|
17
|
+
<img src="https://img.shields.io/badge/PRs-welcome-brightgreen.svg" alt="PRs welcome!" />
|
18
|
+
</a>
|
19
|
+
<a href="https://twitter.com/intent/tweet?text=Awesome+pdf+library%21&url=https://pdfme.com">
|
20
|
+
<img src="https://img.shields.io/twitter/url/http/shields.io.svg?style=social" alt="Tweet" />
|
21
|
+
</a>
|
22
|
+
</p>
|
23
|
+
|
24
|
+
TypeScript base PDF generator and React base UI.
|
25
|
+
Open source, developed by the community, and completely free to use under the MIT license!
|
26
|
+
|
27
|
+
<p align="center">
|
28
|
+
<img src="https://raw.githubusercontent.com/pdfme/pdfme/main/website/static/img/logo.svg" width="300"/>
|
29
|
+
</p>
|
30
|
+
|
31
|
+
## Features
|
32
|
+
|
33
|
+
| Fast PDF Generator | Easy PDF template design | Simple JSON template |
|
34
|
+
| -------------------------------------------------------------------------------------------- | ----------------------------------------------------- | -------------------------------------------------------------- |
|
35
|
+
| Works on node and browser. Use templates to generate PDF, Complex operations are not needed. | Anyone can easily create templates with the designer. | Templates are JSON data that is easy to understand and handle. |
|
36
|
+
|
37
|
+
---
|
38
|
+
|
39
|
+
<!--
|
40
|
+
This is a copy of website/docs/getting-started.md from below.
|
41
|
+
- Replace the image path: /img/image.png -> /website/static/img/image.png
|
42
|
+
- Replace the link: / -> https://pdfme.com/
|
43
|
+
-->
|
44
|
+
|
45
|
+
## Introduction
|
46
|
+
|
47
|
+
pdfme was created to simplify the design and generation process of a PDF. It is especially useful for the following use cases:
|
48
|
+
|
49
|
+
- Need to create a designed PDF with short code.
|
50
|
+
- Need to integrate PDF editor features into an application.
|
51
|
+
- Need to create a large number of PDFs without compromising performance
|
52
|
+
|
53
|
+
As an example, the author's service [https://labelmake.jp/](https://labelmake.jp/) can create more than 100 varieties of PDFs and generates more than 100,000 PDF files per month. Notably, the monthly server cost, utilizing Cloud Functions For Firebase, remains below $10.
|
54
|
+
|
55
|
+
## Installation
|
56
|
+
|
57
|
+
The operating requirements should be the node environment `>=16`.
|
58
|
+
There are two packages in pdfme, generator and UI.
|
59
|
+
|
60
|
+
The package for generating PDF can be installed with the following command.
|
61
|
+
|
62
|
+
```
|
63
|
+
npm i @pdfme/generator @pdfme/common
|
64
|
+
```
|
65
|
+
|
66
|
+
The packages for using PDF designer, forms and viewers can be installed with the following commands.
|
67
|
+
|
68
|
+
```
|
69
|
+
npm i @pdfme/ui @pdfme/common
|
70
|
+
```
|
71
|
+
|
72
|
+
\*You must install `@pdfme/common` regardless of which package you use.
|
73
|
+
|
74
|
+
The following type, function and classes are available in pdfme.
|
75
|
+
|
76
|
+
`@pdfme/common`
|
77
|
+
|
78
|
+
- [Template](https://pdfme.com/docs/getting-started#template)
|
79
|
+
|
80
|
+
`@pdfme/generator`
|
81
|
+
|
82
|
+
- [generate](https://pdfme.com/docs/getting-started#generator)
|
83
|
+
|
84
|
+
`@pdfme/ui`
|
85
|
+
|
86
|
+
- [Designer](https://pdfme.com/docs/getting-started#designer)
|
87
|
+
- [Form](https://pdfme.com/docs/getting-started#form)
|
88
|
+
- [Viewer](https://pdfme.com/docs/getting-started#viewer)
|
89
|
+
|
90
|
+
If your environment uses webpack, import the necessary items as shown below.
|
91
|
+
|
92
|
+
```ts
|
93
|
+
import type { Template } from '@pdfme/common';
|
94
|
+
import { generate } from '@pdfme/generator';
|
95
|
+
```
|
96
|
+
|
97
|
+
```ts
|
98
|
+
import type { Template } from '@pdfme/common';
|
99
|
+
import { Designer, Form, Viewer } from '@pdfme/ui';
|
100
|
+
```
|
101
|
+
|
102
|
+
**All objects use `Template`, which will be briefly explained in the next section.**
|
103
|
+
|
104
|
+
## Template
|
105
|
+
|
106
|
+
The core of pdfme library are Templates.
|
107
|
+
Template Type can be imported by both `@pdfme/generator` or `@pdfme/ui`. Templates are used everywhere.
|
108
|
+
|
109
|
+
A template can be divided into two parts: a fixed part and a variable part.
|
110
|
+
We call them basePdf and schema.
|
111
|
+
The following image is a good illustration of a template.
|
112
|
+
|
113
|
+
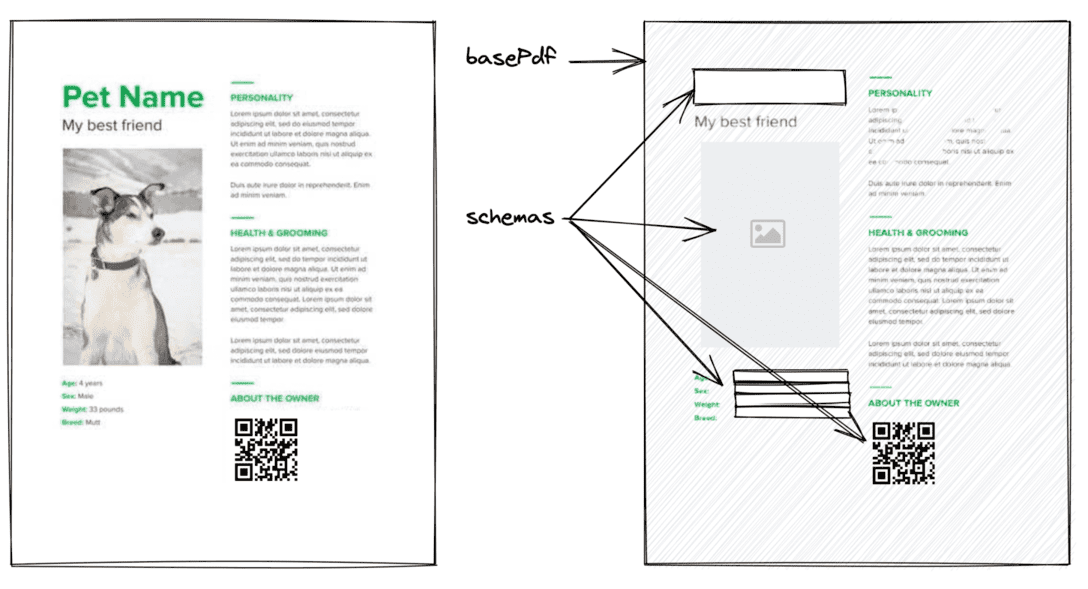
|
114
|
+
|
115
|
+
- **basePdf**: PDF data for the fixed part of the PDF to be generated.
|
116
|
+
- **schemas**: Definition data for the variable part of the PDF to be generated.
|
117
|
+
|
118
|
+
**basePdf** can be given a `string`(base64), `ArrayBuffer`, or `Uint8Array`.
|
119
|
+
A blank A4 PDF can be imported with `BLANK_PDF`. You can use it to check how it works.
|
120
|
+
|
121
|
+
**schemas** can only utilize text by default, but you can load images and various barcodes like QR codes as plugins from the `@pdfme/schemas` package.
|
122
|
+
Additionally, you can create your own schemas, allowing you to render types other than the ones mentioned above.
|
123
|
+
Check detail about [Custom Schemas](https://pdfme.com/docs/custom-schemas) from here
|
124
|
+
|
125
|
+
Let's take a look at some specific data.
|
126
|
+
(If you are using TypeScript, you can import the Template type.)
|
127
|
+
|
128
|
+
### Minimal Template
|
129
|
+
|
130
|
+
```ts
|
131
|
+
import { Template, BLANK_PDF } from '@pdfme/common';
|
132
|
+
|
133
|
+
const template: Template = {
|
134
|
+
basePdf: BLANK_PDF,
|
135
|
+
schemas: [
|
136
|
+
{
|
137
|
+
a: {
|
138
|
+
type: 'text',
|
139
|
+
position: { x: 0, y: 0 },
|
140
|
+
width: 10,
|
141
|
+
height: 10,
|
142
|
+
},
|
143
|
+
b: {
|
144
|
+
type: 'text',
|
145
|
+
position: { x: 10, y: 10 },
|
146
|
+
width: 10,
|
147
|
+
height: 10,
|
148
|
+
},
|
149
|
+
c: {
|
150
|
+
type: 'text',
|
151
|
+
position: { x: 20, y: 20 },
|
152
|
+
width: 10,
|
153
|
+
height: 10,
|
154
|
+
},
|
155
|
+
},
|
156
|
+
],
|
157
|
+
};
|
158
|
+
```
|
159
|
+
|
160
|
+
You can create a template from [Template Design page](https://pdfme.com/template-design). Or, if you want to integrate the template creation feature into your application, check out the [Designer section](https://pdfme.com/docs/getting-started#designer).
|
161
|
+
|
162
|
+
## Generator
|
163
|
+
|
164
|
+
The PDF generator function, `generate`, takes 2 arguments of `template` and `inputs` for generate a PDF. It works both in Node.js and in the browser.
|
165
|
+
|
166
|
+
The code to generate a PDF file using the [template created above](https://pdfme.com/docs/getting-started#sample-template) is shown below.
|
167
|
+
|
168
|
+
```ts
|
169
|
+
import type { Template } from '@pdfme/common';
|
170
|
+
import { generate } from '@pdfme/generator';
|
171
|
+
|
172
|
+
const template: Template = {
|
173
|
+
// skip... Check the Template section.
|
174
|
+
};
|
175
|
+
const inputs = [{ a: 'a1', b: 'b1', c: 'c1' }];
|
176
|
+
|
177
|
+
generate({ template, inputs }).then((pdf) => {
|
178
|
+
console.log(pdf);
|
179
|
+
|
180
|
+
// Browser
|
181
|
+
// const blob = new Blob([pdf.buffer], { type: 'application/pdf' });
|
182
|
+
// window.open(URL.createObjectURL(blob));
|
183
|
+
|
184
|
+
// Node.js
|
185
|
+
// fs.writeFileSync(path.join(__dirname, `test.pdf`), pdf);
|
186
|
+
});
|
187
|
+
```
|
188
|
+
|
189
|
+
You can create a PDF file like the below.
|
190
|
+
|
191
|
+
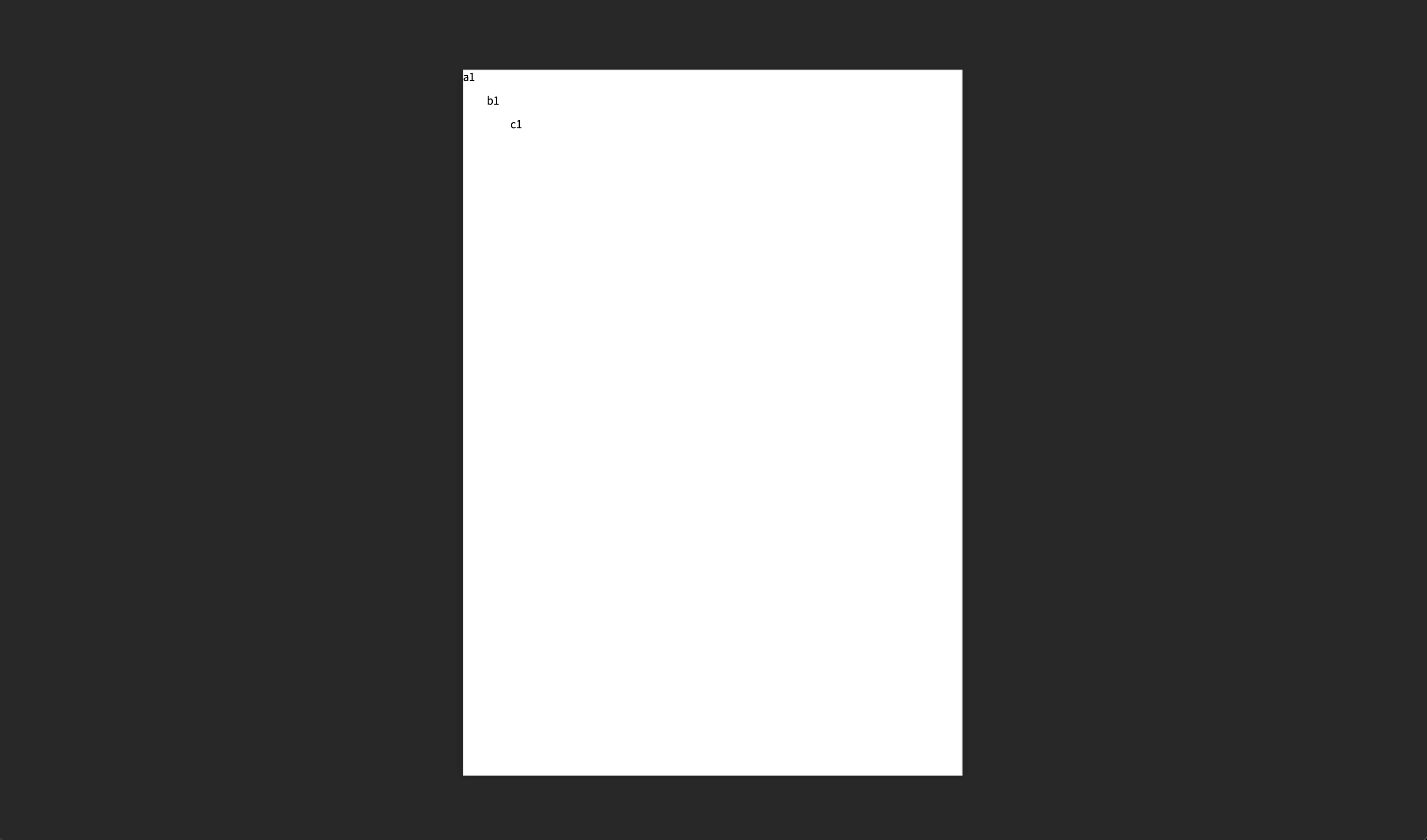
|
192
|
+
|
193
|
+
Also, each element in the inputs array corresponds to a page in the PDF, you can create a multi-page PDF file by providing multiple elements of inputs.
|
194
|
+
|
195
|
+
## UI
|
196
|
+
|
197
|
+
The UI is composed of the Designer, Form, and Viewer classes.
|
198
|
+
|
199
|
+
### Designer
|
200
|
+
|
201
|
+
The Designer allows you to edit the Template schemas, making it easy for anyone to create Template json objects.
|
202
|
+
|
203
|
+
You can design your own template from [Template Design page](https://pdfme.com/template-design), or you can integrate the designer into your application.
|
204
|
+
|
205
|
+
Let's integrate the designer using the template created above as the default template.
|
206
|
+
|
207
|
+
```ts
|
208
|
+
import type { Template } from '@pdfme/common';
|
209
|
+
import { Designer } from '@pdfme/ui';
|
210
|
+
|
211
|
+
const domContainer = document.getElementById('container');
|
212
|
+
const template: Template = {
|
213
|
+
// skip... Check the Template section.
|
214
|
+
};
|
215
|
+
|
216
|
+
const designer = new Designer({ domContainer, template });
|
217
|
+
```
|
218
|
+
|
219
|
+
The Designer class is instantiated as shown above, and the template designer is displayed in the `domContainer`.
|
220
|
+
You can edit the template as shown below. The operation is like Google Slides, etc., so you can use common keyboard shortcuts.
|
221
|
+
|
222
|
+
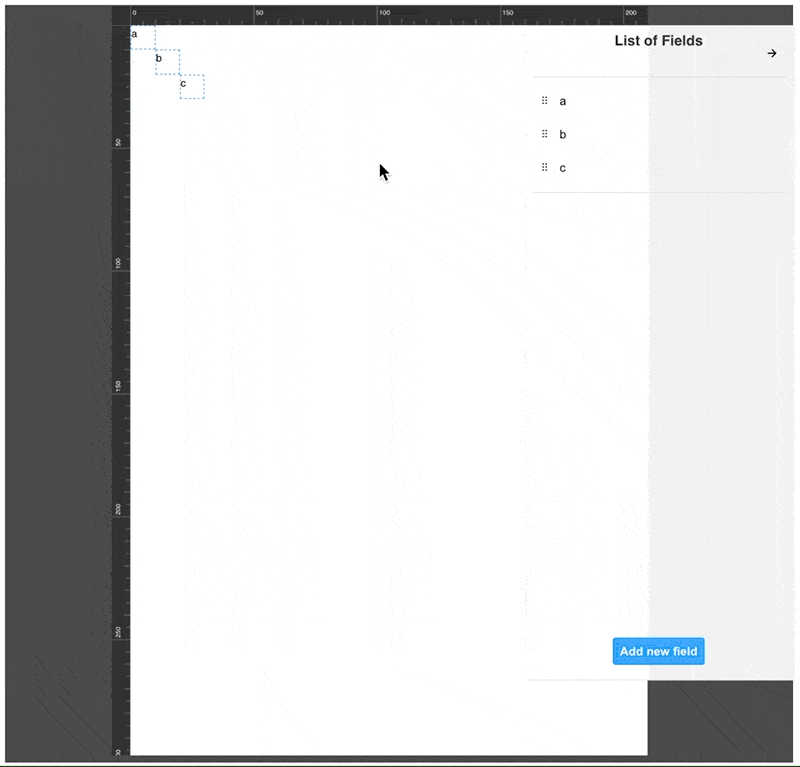
|
223
|
+
|
224
|
+
The designer instance can be manipulated with the following methods.
|
225
|
+
|
226
|
+
- `saveTemplate`
|
227
|
+
- `updateTemplate`
|
228
|
+
- `getTemplate`
|
229
|
+
- `onChangeTemplate`
|
230
|
+
- `onSaveTemplate`
|
231
|
+
- `destroy`
|
232
|
+
|
233
|
+
### Form
|
234
|
+
|
235
|
+
You can use templates to create forms and PDF viewers.
|
236
|
+
|
237
|
+
The Form creates a UI for the user to enter schemas based on the template.
|
238
|
+
|
239
|
+
```ts
|
240
|
+
import type { Template } from '@pdfme/common';
|
241
|
+
import { Form } from '@pdfme/ui';
|
242
|
+
|
243
|
+
const domContainer = document.getElementById('container');
|
244
|
+
const template: Template = {
|
245
|
+
// skip...
|
246
|
+
};
|
247
|
+
// This is initial data.
|
248
|
+
const inputs = [{ a: 'a1', b: 'b1', c: 'c1' }];
|
249
|
+
|
250
|
+
const form = new Form({ domContainer, template, inputs });
|
251
|
+
```
|
252
|
+
|
253
|
+

|
254
|
+
|
255
|
+
The form instance has a method `getInputs` to get the user's input.
|
256
|
+
|
257
|
+
You can generate a PDF file based on the user's input by passing the data you get from `getInputs` as inputs to generate, as shown in the code below.
|
258
|
+
|
259
|
+
```ts
|
260
|
+
generate({ template, inputs: form.getInputs() }).then((pdf) => {
|
261
|
+
const blob = new Blob([pdf.buffer], { type: 'application/pdf' });
|
262
|
+
window.open(URL.createObjectURL(blob));
|
263
|
+
});
|
264
|
+
```
|
265
|
+
|
266
|
+
### Viewer
|
267
|
+
|
268
|
+
Viewing a PDF file in a mobile browser is a pain, because it doesn't display well in an iframe.
|
269
|
+
|
270
|
+
The Viewer is a byproduct of the Form development process, but it allows you to show your users a preview of the PDF file you will create.
|
271
|
+
|
272
|
+
Using the Viewer is basically the same as using the Form, except that user cannot edit it.
|
273
|
+
|
274
|
+
```ts
|
275
|
+
import type { Template } from '@pdfme/common';
|
276
|
+
import { Viewer } from '@pdfme/ui';
|
277
|
+
|
278
|
+
const domContainer = document.getElementById('container');
|
279
|
+
const template: Template = {
|
280
|
+
// skip...
|
281
|
+
};
|
282
|
+
const inputs = [{ a: 'a1', b: 'b1', c: 'c1' }];
|
283
|
+
|
284
|
+
const viewer = new Viewer({ domContainer, template, inputs });
|
285
|
+
```
|
286
|
+
|
287
|
+

|
288
|
+
|
289
|
+
## Special Thanks
|
290
|
+
|
291
|
+
- [pdf-lib](https://pdf-lib.js.org/): Used in PDF generation.
|
292
|
+
- [fontkit](https://github.com/foliojs/fontkit): Used in font rendering.
|
293
|
+
- [PDF.js](https://mozilla.github.io/pdf.js/): Used in PDF viewing.
|
294
|
+
- [React](https://reactjs.org/): Used in building the UI.
|
295
|
+
- [form-render](https://xrender.fun/form-render): Used in building the UI.
|
296
|
+
- [antd](https://ant.design/): Used in building the UI.
|
297
|
+
- [react-moveable](https://daybrush.com/moveable/), [react-selecto](https://github.com/daybrush/selecto), [@scena/react-guides](https://daybrush.com/guides/): Used in Designer UI.
|
298
|
+
- [dnd-kit](https://github.com/clauderic/dnd-kit): Used in Designer UI.
|
299
|
+
|
300
|
+
I definitely could not have created pdfme without these libraries. I am grateful to the developers of these libraries.
|
301
|
+
|
302
|
+
If you want to contribute to pdfme, please check the [Development Guide](https://pdfme.com/docs/development-guide) page.
|
303
|
+
We look forward to your contribution!
|
@@ -1 +1 @@
|
|
1
|
-
{"version":3,"file":"index.js","sourceRoot":"","sources":["../../../src/index.ts"],"names":[],"mappings":";;;AAAA,iDAOwB;
|
1
|
+
{"version":3,"file":"index.js","sourceRoot":"","sources":["../../../src/index.ts"],"names":[],"mappings":";;;AAAA,iDAOwB;AA8CtB,+FApDA,6BAAc,OAoDA;AACd,+FApDA,6BAAc,OAoDA;AACd,+FApDA,6BAAc,OAoDA;AACd,0FApDA,wBAAS,OAoDA;AACT,qFApDA,mBAAI,OAoDA;AACJ,kGApDA,gCAAiB,OAoDA;AAzBnB,2CAiBqB;AASnB,oGAzBA,+BAAmB,OAyBA;AACnB,+FAzBA,0BAAc,OAyBA;AACd,8FAzBA,yBAAa,OAyBA;AACb,gGAzBA,2BAAe,OAyBA;AAKf,0FA7BA,qBAAS,OA6BA;AACT,4FA7BA,uBAAW,OA6BA;AACX,+FA7BA,0BAAc,OA6BA;AACd,8FA7BA,yBAAa,OA6BA;AACb,6FA7BA,wBAAY,OA6BA;AACZ,kGA7BA,6BAAiB,OA6BA;AACjB,mGA7BA,8BAAkB,OA6BA;AAClB,mGA7BA,8BAAkB,OA6BA;AAXlB,sFAjBA,iBAAK,OAiBA;AACL,sFAjBA,iBAAK,OAiBA;AACL,sFAjBA,iBAAK,OAiBA;AACL,2FAjBA,sBAAU,OAiBA"}
|
package/dist/cjs/src/schema.js
CHANGED
@@ -1,8 +1,8 @@
|
|
1
1
|
"use strict";
|
2
2
|
Object.defineProperty(exports, "__esModule", { value: true });
|
3
|
-
exports.DesignerProps = exports.PreviewProps = exports.UIProps = exports.UIOptions = exports.GenerateProps = exports.GeneratorOptions = exports.Inputs = exports.Template = exports.BasePdf = exports.Font = exports.SchemaForUI = exports.Schema = exports.Size = exports.Dict = exports.Lang = void 0;
|
3
|
+
exports.DesignerProps = exports.PreviewProps = exports.UIProps = exports.UIOptions = exports.GenerateProps = exports.GeneratorOptions = exports.Inputs = exports.Template = exports.BasePdf = exports.Font = exports.SchemaForUI = exports.Schema = exports.Size = exports.Mode = exports.Dict = exports.Lang = void 0;
|
4
4
|
const zod_1 = require("zod");
|
5
|
-
const langs = ['en', 'ja', 'ar', 'th', 'pl', 'it'];
|
5
|
+
const langs = ['en', 'ja', 'ar', 'th', 'pl', 'it', 'de'];
|
6
6
|
exports.Lang = zod_1.z.enum(langs);
|
7
7
|
exports.Dict = zod_1.z.object({
|
8
8
|
// -----------------used in ui-----------------
|
@@ -27,7 +27,11 @@ exports.Dict = zod_1.z.object({
|
|
27
27
|
errorBulkUpdateFieldName: zod_1.z.string(),
|
28
28
|
commitBulkUpdateFieldName: zod_1.z.string(),
|
29
29
|
bulkUpdateFieldName: zod_1.z.string(),
|
30
|
+
hexColorPrompt: zod_1.z.string(),
|
30
31
|
// -----------------used in schemas-----------------
|
32
|
+
'schemas.color': zod_1.z.string(),
|
33
|
+
'schemas.borderWidth': zod_1.z.string(),
|
34
|
+
'schemas.borderColor': zod_1.z.string(),
|
31
35
|
'schemas.textColor': zod_1.z.string(),
|
32
36
|
'schemas.bgColor': zod_1.z.string(),
|
33
37
|
'schemas.horizontal': zod_1.z.string(),
|
@@ -50,10 +54,13 @@ exports.Dict = zod_1.z.object({
|
|
50
54
|
'schemas.text.dynamicFontSize': zod_1.z.string(),
|
51
55
|
'schemas.barcodes.barColor': zod_1.z.string(),
|
52
56
|
});
|
57
|
+
exports.Mode = zod_1.z.enum(['viewer', 'form', 'designer']);
|
53
58
|
exports.Size = zod_1.z.object({ height: zod_1.z.number(), width: zod_1.z.number() });
|
54
59
|
exports.Schema = zod_1.z
|
55
60
|
.object({
|
56
61
|
type: zod_1.z.string(),
|
62
|
+
readOnly: zod_1.z.boolean().optional(),
|
63
|
+
readOnlyValue: zod_1.z.string().optional(),
|
57
64
|
position: zod_1.z.object({ x: zod_1.z.number(), y: zod_1.z.number() }),
|
58
65
|
width: zod_1.z.number(),
|
59
66
|
height: zod_1.z.number(),
|
@@ -1 +1 @@
|
|
1
|
-
{"version":3,"file":"schema.js","sourceRoot":"","sources":["../../../src/schema.ts"],"names":[],"mappings":";;;AAAA,6BAAwB;AAExB,MAAM,KAAK,GAAG,CAAC,IAAI,EAAE,IAAI,EAAE,IAAI,EAAE,IAAI,EAAE,IAAI,EAAE,IAAI,CAAU,CAAC;
|
1
|
+
{"version":3,"file":"schema.js","sourceRoot":"","sources":["../../../src/schema.ts"],"names":[],"mappings":";;;AAAA,6BAAwB;AAExB,MAAM,KAAK,GAAG,CAAC,IAAI,EAAE,IAAI,EAAE,IAAI,EAAE,IAAI,EAAE,IAAI,EAAE,IAAI,EAAE,IAAI,CAAU,CAAC;AAErD,QAAA,IAAI,GAAG,OAAC,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC;AACrB,QAAA,IAAI,GAAG,OAAC,CAAC,MAAM,CAAC;IAC3B,+CAA+C;IAC/C,MAAM,EAAE,OAAC,CAAC,MAAM,EAAE;IAClB,KAAK,EAAE,OAAC,CAAC,MAAM,EAAE;IACjB,SAAS,EAAE,OAAC,CAAC,MAAM,EAAE;IACrB,KAAK,EAAE,OAAC,CAAC,MAAM,EAAE;IACjB,KAAK,EAAE,OAAC,CAAC,MAAM,EAAE;IACjB,OAAO,EAAE,OAAC,CAAC,MAAM,EAAE;IACnB,MAAM,EAAE,OAAC,CAAC,MAAM,EAAE;IAClB,MAAM,EAAE,OAAC,CAAC,MAAM,EAAE;IAClB,IAAI,EAAE,OAAC,CAAC,MAAM,EAAE;IAChB,YAAY,EAAE,OAAC,CAAC,MAAM,EAAE;IACxB,aAAa,EAAE,OAAC,CAAC,MAAM,EAAE;IACzB,OAAO,EAAE,OAAC,CAAC,MAAM,EAAE;IACnB,SAAS,EAAE,OAAC,CAAC,MAAM,EAAE;IACrB,UAAU,EAAE,OAAC,CAAC,MAAM,EAAE;IACtB,WAAW,EAAE,OAAC,CAAC,MAAM,EAAE;IACvB,SAAS,EAAE,OAAC,CAAC,MAAM,EAAE;IACrB,IAAI,EAAE,OAAC,CAAC,MAAM,EAAE;IAChB,aAAa,EAAE,OAAC,CAAC,MAAM,EAAE;IACzB,wBAAwB,EAAE,OAAC,CAAC,MAAM,EAAE;IACpC,yBAAyB,EAAE,OAAC,CAAC,MAAM,EAAE;IACrC,mBAAmB,EAAE,OAAC,CAAC,MAAM,EAAE;IAC/B,cAAc,EAAE,OAAC,CAAC,MAAM,EAAE;IAC1B,oDAAoD;IACpD,eAAe,EAAE,OAAC,CAAC,MAAM,EAAE;IAC3B,qBAAqB,EAAE,OAAC,CAAC,MAAM,EAAE;IACjC,qBAAqB,EAAE,OAAC,CAAC,MAAM,EAAE;IACjC,mBAAmB,EAAE,OAAC,CAAC,MAAM,EAAE;IAC/B,iBAAiB,EAAE,OAAC,CAAC,MAAM,EAAE;IAC7B,oBAAoB,EAAE,OAAC,CAAC,MAAM,EAAE;IAChC,kBAAkB,EAAE,OAAC,CAAC,MAAM,EAAE;IAC9B,cAAc,EAAE,OAAC,CAAC,MAAM,EAAE;IAC1B,gBAAgB,EAAE,OAAC,CAAC,MAAM,EAAE;IAC5B,eAAe,EAAE,OAAC,CAAC,MAAM,EAAE;IAC3B,aAAa,EAAE,OAAC,CAAC,MAAM,EAAE;IACzB,gBAAgB,EAAE,OAAC,CAAC,MAAM,EAAE;IAC5B,gBAAgB,EAAE,OAAC,CAAC,MAAM,EAAE;IAE5B,uBAAuB,EAAE,OAAC,CAAC,MAAM,EAAE;IACnC,mBAAmB,EAAE,OAAC,CAAC,MAAM,EAAE;IAC/B,sBAAsB,EAAE,OAAC,CAAC,MAAM,EAAE;IAClC,wBAAwB,EAAE,OAAC,CAAC,MAAM,EAAE;IACpC,4BAA4B,EAAE,OAAC,CAAC,MAAM,EAAE;IACxC,yBAAyB,EAAE,OAAC,CAAC,MAAM,EAAE;IACrC,kBAAkB,EAAE,OAAC,CAAC,MAAM,EAAE;IAC9B,kBAAkB,EAAE,OAAC,CAAC,MAAM,EAAE;IAC9B,kBAAkB,EAAE,OAAC,CAAC,MAAM,EAAE;IAC9B,8BAA8B,EAAE,OAAC,CAAC,MAAM,EAAE;IAE1C,2BAA2B,EAAE,OAAC,CAAC,MAAM,EAAE;CACxC,CAAC,CAAC;AACU,QAAA,IAAI,GAAG,OAAC,CAAC,IAAI,CAAC,CAAC,QAAQ,EAAE,MAAM,EAAE,UAAU,CAAC,CAAC,CAAC;AAE9C,QAAA,IAAI,GAAG,OAAC,CAAC,MAAM,CAAC,EAAE,MAAM,EAAE,OAAC,CAAC,MAAM,EAAE,EAAE,KAAK,EAAE,OAAC,CAAC,MAAM,EAAE,EAAE,CAAC,CAAC;AAE3D,QAAA,MAAM,GAAG,OAAC;KACpB,MAAM,CAAC;IACN,IAAI,EAAE,OAAC,CAAC,MAAM,EAAE;IAChB,QAAQ,EAAE,OAAC,CAAC,OAAO,EAAE,CAAC,QAAQ,EAAE;IAChC,aAAa,EAAE,OAAC,CAAC,MAAM,EAAE,CAAC,QAAQ,EAAE;IACpC,QAAQ,EAAE,OAAC,CAAC,MAAM,CAAC,EAAE,CAAC,EAAE,OAAC,CAAC,MAAM,EAAE,EAAE,CAAC,EAAE,OAAC,CAAC,MAAM,EAAE,EAAE,CAAC;IACpD,KAAK,EAAE,OAAC,CAAC,MAAM,EAAE;IACjB,MAAM,EAAE,OAAC,CAAC,MAAM,EAAE;IAClB,MAAM,EAAE,OAAC,CAAC,MAAM,EAAE,CAAC,QAAQ,EAAE;IAC7B,OAAO,EAAE,OAAC,CAAC,MAAM,EAAE,CAAC,QAAQ,EAAE;CAC/B,CAAC;KACD,WAAW,EAAE,CAAC;AAEjB,MAAM,yBAAyB,GAAG,OAAC,CAAC,MAAM,CAAC;IACzC,EAAE,EAAE,OAAC,CAAC,MAAM,EAAE;IACd,GAAG,EAAE,OAAC,CAAC,MAAM,EAAE;IACf,IAAI,EAAE,OAAC,CAAC,MAAM,EAAE;CACjB,CAAC,CAAC;AACU,QAAA,WAAW,GAAG,cAAM,CAAC,KAAK,CAAC,yBAAyB,CAAC,CAAC;AAEnE,MAAM,iBAAiB,GAA6B,OAAC,CAAC,GAAG,EAAE,CAAC,MAAM,CAAC,CAAC,CAAC,EAAE,EAAE,CAAC,CAAC,YAAY,WAAW,CAAC,CAAC;AACpG,MAAM,gBAAgB,GAA4B,OAAC,CAAC,GAAG,EAAE,CAAC,MAAM,CAAC,CAAC,CAAC,EAAE,EAAE,CAAC,CAAC,YAAY,UAAU,CAAC,CAAC;AAEpF,QAAA,IAAI,GAAG,OAAC,CAAC,MAAM,CAC1B,OAAC,CAAC,MAAM,CAAC;IACP,IAAI,EAAE,OAAC,CAAC,KAAK,CAAC,CAAC,OAAC,CAAC,MAAM,EAAE,EAAE,iBAAiB,EAAE,gBAAgB,CAAC,CAAC;IAChE,QAAQ,EAAE,OAAC,CAAC,OAAO,EAAE,CAAC,QAAQ,EAAE;IAChC,MAAM,EAAE,OAAC,CAAC,OAAO,EAAE,CAAC,QAAQ,EAAE;CAC/B,CAAC,CACH,CAAC;AAEW,QAAA,OAAO,GAAG,OAAC,CAAC,KAAK,CAAC,CAAC,OAAC,CAAC,MAAM,EAAE,EAAE,iBAAiB,EAAE,gBAAgB,CAAC,CAAC,CAAC;AAErE,QAAA,QAAQ,GAAG,OAAC,CAAC,MAAM,CAAC;IAC/B,OAAO,EAAE,OAAC,CAAC,KAAK,CAAC,OAAC,CAAC,MAAM,CAAC,cAAM,CAAC,CAAC;IAClC,OAAO,EAAE,eAAO;IAChB,UAAU,EAAE,OAAC,CAAC,KAAK,CAAC,OAAC,CAAC,MAAM,CAAC,OAAC,CAAC,MAAM,EAAE,CAAC,CAAC,CAAC,MAAM,CAAC,CAAC,CAAC,CAAC,QAAQ,EAAE;IAC9D,OAAO,EAAE,OAAC,CAAC,KAAK,CAAC,OAAC,CAAC,MAAM,EAAE,CAAC,CAAC,QAAQ,EAAE;CACxC,CAAC,CAAC;AAEU,QAAA,MAAM,GAAG,OAAC,CAAC,KAAK,CAAC,OAAC,CAAC,MAAM,CAAC,OAAC,CAAC,MAAM,EAAE,CAAC,CAAC,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC;AAE3D,MAAM,aAAa,GAAG,OAAC,CAAC,MAAM,CAAC,EAAE,IAAI,EAAE,YAAI,CAAC,QAAQ,EAAE,EAAE,CAAC,CAAC,WAAW,EAAE,CAAC;AAExE,MAAM,WAAW,GAAG,OAAC,CAAC,MAAM,CAAC;IAC3B,QAAQ,EAAE,gBAAQ;IAClB,OAAO,EAAE,aAAa,CAAC,QAAQ,EAAE;IACjC,OAAO,EAAE,OAAC,CAAC,MAAM,CAAC,OAAC,CAAC,MAAM,CAAC,EAAE,EAAE,EAAE,OAAC,CAAC,GAAG,EAAE,EAAE,GAAG,EAAE,OAAC,CAAC,GAAG,EAAE,EAAE,SAAS,EAAE,OAAC,CAAC,GAAG,EAAE,EAAE,CAAC,CAAC,CAAC,QAAQ,EAAE;CAC1F,CAAC,CAAC;AAEH,iDAAiD;AAEpC,QAAA,gBAAgB,GAAG,aAAa,CAAC,MAAM,CAAC;IACnD,MAAM,EAAE,OAAC,CAAC,MAAM,EAAE,CAAC,QAAQ,EAAE;IAC7B,YAAY,EAAE,OAAC,CAAC,IAAI,EAAE,CAAC,QAAQ,EAAE;IACjC,OAAO,EAAE,OAAC,CAAC,MAAM,EAAE,CAAC,QAAQ,EAAE;IAC9B,QAAQ,EAAE,OAAC,CAAC,KAAK,CAAC,OAAC,CAAC,MAAM,EAAE,CAAC,CAAC,QAAQ,EAAE;IACxC,QAAQ,EAAE,OAAC,CAAC,MAAM,EAAE,CAAC,QAAQ,EAAE;IAC/B,gBAAgB,EAAE,OAAC,CAAC,IAAI,EAAE,CAAC,QAAQ,EAAE;IACrC,QAAQ,EAAE,OAAC,CAAC,MAAM,EAAE,CAAC,QAAQ,EAAE;IAC/B,OAAO,EAAE,OAAC,CAAC,MAAM,EAAE,CAAC,QAAQ,EAAE;IAC9B,KAAK,EAAE,OAAC,CAAC,MAAM,EAAE,CAAC,QAAQ,EAAE;CAC7B,CAAC,CAAC;AAEU,QAAA,aAAa,GAAG,WAAW,CAAC,MAAM,CAAC;IAC9C,MAAM,EAAE,cAAM;IACd,OAAO,EAAE,wBAAgB,CAAC,QAAQ,EAAE;CACrC,CAAC,CAAC,MAAM,EAAE,CAAC;AAEZ,kDAAkD;AAErC,QAAA,SAAS,GAAG,aAAa,CAAC,MAAM,CAAC;IAC5C,IAAI,EAAE,YAAI,CAAC,QAAQ,EAAE;IACrB,MAAM,EAAE,OAAC,CAAC,MAAM,CAAC,OAAC,CAAC,MAAM,EAAE,EAAE,OAAC,CAAC,MAAM,EAAE,CAAC,CAAC,QAAQ,EAAE;IACnD,KAAK,EAAE,OAAC,CAAC,MAAM,CAAC,OAAC,CAAC,MAAM,EAAE,EAAE,OAAC,CAAC,OAAO,EAAE,CAAC,CAAC,QAAQ,EAAE;CACpD,CAAC,CAAC;AAEH,MAAM,iBAAiB,GAA6B,OAAC,CAAC,GAAG,EAAE,CAAC,MAAM,CAAC,CAAC,CAAC,EAAE,EAAE,CAAC,CAAC,YAAY,WAAW,CAAC,CAAC;AAEvF,QAAA,OAAO,GAAG,WAAW,CAAC,MAAM,CAAC;IACxC,YAAY,EAAE,iBAAiB;IAC/B,OAAO,EAAE,iBAAS,CAAC,QAAQ,EAAE;CAC9B,CAAC,CAAC;AAEU,QAAA,YAAY,GAAG,eAAO,CAAC,MAAM,CAAC,EAAE,MAAM,EAAE,cAAM,EAAE,CAAC,CAAC,MAAM,EAAE,CAAC;AAE3D,QAAA,aAAa,GAAG,eAAO,CAAC,MAAM,CAAC,EAAE,CAAC,CAAC,MAAM,EAAE,CAAC"}
|
@@ -1 +1 @@
|
|
1
|
-
{"version":3,"file":"index.js","sourceRoot":"","sources":["../../../src/index.ts"],"names":[],"mappings":"AAAA,OAAO,EACL,cAAc,EACd,cAAc,EACd,cAAc,EACd,SAAS,EACT,IAAI,EACJ,iBAAiB,GAClB,MAAM,gBAAgB,CAAC;
|
1
|
+
{"version":3,"file":"index.js","sourceRoot":"","sources":["../../../src/index.ts"],"names":[],"mappings":"AAAA,OAAO,EACL,cAAc,EACd,cAAc,EACd,cAAc,EACd,SAAS,EACT,IAAI,EACJ,iBAAiB,GAClB,MAAM,gBAAgB,CAAC;AA0BxB,OAAO,EACL,mBAAmB,EACnB,cAAc,EACd,aAAa,EACb,eAAe,EACf,SAAS,EACT,WAAW,EACX,cAAc,EACd,aAAa,EACb,YAAY,EACZ,iBAAiB,EACjB,kBAAkB,EAClB,kBAAkB,EAClB,KAAK,EACL,KAAK,EACL,KAAK,EACL,UAAU,GACX,MAAM,aAAa,CAAC;AAErB,OAAO,EACL,cAAc,EACd,cAAc,EACd,cAAc,EACd,SAAS,EACT,IAAI,EACJ,iBAAiB,EACjB,mBAAmB,EACnB,cAAc,EACd,aAAa,EACb,eAAe,EACf,KAAK,EACL,KAAK,EACL,KAAK,EACL,UAAU,EACV,SAAS,EACT,WAAW,EACX,cAAc,EACd,aAAa,EACb,YAAY,EACZ,iBAAiB,EACjB,kBAAkB,EAClB,kBAAkB,GACnB,CAAC"}
|
package/dist/esm/src/schema.js
CHANGED
@@ -1,5 +1,5 @@
|
|
1
1
|
import { z } from 'zod';
|
2
|
-
const langs = ['en', 'ja', 'ar', 'th', 'pl', 'it'];
|
2
|
+
const langs = ['en', 'ja', 'ar', 'th', 'pl', 'it', 'de'];
|
3
3
|
export const Lang = z.enum(langs);
|
4
4
|
export const Dict = z.object({
|
5
5
|
// -----------------used in ui-----------------
|
@@ -24,7 +24,11 @@ export const Dict = z.object({
|
|
24
24
|
errorBulkUpdateFieldName: z.string(),
|
25
25
|
commitBulkUpdateFieldName: z.string(),
|
26
26
|
bulkUpdateFieldName: z.string(),
|
27
|
+
hexColorPrompt: z.string(),
|
27
28
|
// -----------------used in schemas-----------------
|
29
|
+
'schemas.color': z.string(),
|
30
|
+
'schemas.borderWidth': z.string(),
|
31
|
+
'schemas.borderColor': z.string(),
|
28
32
|
'schemas.textColor': z.string(),
|
29
33
|
'schemas.bgColor': z.string(),
|
30
34
|
'schemas.horizontal': z.string(),
|
@@ -47,10 +51,13 @@ export const Dict = z.object({
|
|
47
51
|
'schemas.text.dynamicFontSize': z.string(),
|
48
52
|
'schemas.barcodes.barColor': z.string(),
|
49
53
|
});
|
54
|
+
export const Mode = z.enum(['viewer', 'form', 'designer']);
|
50
55
|
export const Size = z.object({ height: z.number(), width: z.number() });
|
51
56
|
export const Schema = z
|
52
57
|
.object({
|
53
58
|
type: z.string(),
|
59
|
+
readOnly: z.boolean().optional(),
|
60
|
+
readOnlyValue: z.string().optional(),
|
54
61
|
position: z.object({ x: z.number(), y: z.number() }),
|
55
62
|
width: z.number(),
|
56
63
|
height: z.number(),
|
@@ -1 +1 @@
|
|
1
|
-
{"version":3,"file":"schema.js","sourceRoot":"","sources":["../../../src/schema.ts"],"names":[],"mappings":"AAAA,OAAO,EAAE,CAAC,EAAE,MAAM,KAAK,CAAC;AAExB,MAAM,KAAK,GAAG,CAAC,IAAI,EAAE,IAAI,EAAE,IAAI,EAAE,IAAI,EAAE,IAAI,EAAE,IAAI,CAAU,CAAC;
|
1
|
+
{"version":3,"file":"schema.js","sourceRoot":"","sources":["../../../src/schema.ts"],"names":[],"mappings":"AAAA,OAAO,EAAE,CAAC,EAAE,MAAM,KAAK,CAAC;AAExB,MAAM,KAAK,GAAG,CAAC,IAAI,EAAE,IAAI,EAAE,IAAI,EAAE,IAAI,EAAE,IAAI,EAAE,IAAI,EAAE,IAAI,CAAU,CAAC;AAElE,MAAM,CAAC,MAAM,IAAI,GAAG,CAAC,CAAC,IAAI,CAAC,KAAK,CAAC,CAAC;AAClC,MAAM,CAAC,MAAM,IAAI,GAAG,CAAC,CAAC,MAAM,CAAC;IAC3B,+CAA+C;IAC/C,MAAM,EAAE,CAAC,CAAC,MAAM,EAAE;IAClB,KAAK,EAAE,CAAC,CAAC,MAAM,EAAE;IACjB,SAAS,EAAE,CAAC,CAAC,MAAM,EAAE;IACrB,KAAK,EAAE,CAAC,CAAC,MAAM,EAAE;IACjB,KAAK,EAAE,CAAC,CAAC,MAAM,EAAE;IACjB,OAAO,EAAE,CAAC,CAAC,MAAM,EAAE;IACnB,MAAM,EAAE,CAAC,CAAC,MAAM,EAAE;IAClB,MAAM,EAAE,CAAC,CAAC,MAAM,EAAE;IAClB,IAAI,EAAE,CAAC,CAAC,MAAM,EAAE;IAChB,YAAY,EAAE,CAAC,CAAC,MAAM,EAAE;IACxB,aAAa,EAAE,CAAC,CAAC,MAAM,EAAE;IACzB,OAAO,EAAE,CAAC,CAAC,MAAM,EAAE;IACnB,SAAS,EAAE,CAAC,CAAC,MAAM,EAAE;IACrB,UAAU,EAAE,CAAC,CAAC,MAAM,EAAE;IACtB,WAAW,EAAE,CAAC,CAAC,MAAM,EAAE;IACvB,SAAS,EAAE,CAAC,CAAC,MAAM,EAAE;IACrB,IAAI,EAAE,CAAC,CAAC,MAAM,EAAE;IAChB,aAAa,EAAE,CAAC,CAAC,MAAM,EAAE;IACzB,wBAAwB,EAAE,CAAC,CAAC,MAAM,EAAE;IACpC,yBAAyB,EAAE,CAAC,CAAC,MAAM,EAAE;IACrC,mBAAmB,EAAE,CAAC,CAAC,MAAM,EAAE;IAC/B,cAAc,EAAE,CAAC,CAAC,MAAM,EAAE;IAC1B,oDAAoD;IACpD,eAAe,EAAE,CAAC,CAAC,MAAM,EAAE;IAC3B,qBAAqB,EAAE,CAAC,CAAC,MAAM,EAAE;IACjC,qBAAqB,EAAE,CAAC,CAAC,MAAM,EAAE;IACjC,mBAAmB,EAAE,CAAC,CAAC,MAAM,EAAE;IAC/B,iBAAiB,EAAE,CAAC,CAAC,MAAM,EAAE;IAC7B,oBAAoB,EAAE,CAAC,CAAC,MAAM,EAAE;IAChC,kBAAkB,EAAE,CAAC,CAAC,MAAM,EAAE;IAC9B,cAAc,EAAE,CAAC,CAAC,MAAM,EAAE;IAC1B,gBAAgB,EAAE,CAAC,CAAC,MAAM,EAAE;IAC5B,eAAe,EAAE,CAAC,CAAC,MAAM,EAAE;IAC3B,aAAa,EAAE,CAAC,CAAC,MAAM,EAAE;IACzB,gBAAgB,EAAE,CAAC,CAAC,MAAM,EAAE;IAC5B,gBAAgB,EAAE,CAAC,CAAC,MAAM,EAAE;IAE5B,uBAAuB,EAAE,CAAC,CAAC,MAAM,EAAE;IACnC,mBAAmB,EAAE,CAAC,CAAC,MAAM,EAAE;IAC/B,sBAAsB,EAAE,CAAC,CAAC,MAAM,EAAE;IAClC,wBAAwB,EAAE,CAAC,CAAC,MAAM,EAAE;IACpC,4BAA4B,EAAE,CAAC,CAAC,MAAM,EAAE;IACxC,yBAAyB,EAAE,CAAC,CAAC,MAAM,EAAE;IACrC,kBAAkB,EAAE,CAAC,CAAC,MAAM,EAAE;IAC9B,kBAAkB,EAAE,CAAC,CAAC,MAAM,EAAE;IAC9B,kBAAkB,EAAE,CAAC,CAAC,MAAM,EAAE;IAC9B,8BAA8B,EAAE,CAAC,CAAC,MAAM,EAAE;IAE1C,2BAA2B,EAAE,CAAC,CAAC,MAAM,EAAE;CACxC,CAAC,CAAC;AACH,MAAM,CAAC,MAAM,IAAI,GAAG,CAAC,CAAC,IAAI,CAAC,CAAC,QAAQ,EAAE,MAAM,EAAE,UAAU,CAAC,CAAC,CAAC;AAE3D,MAAM,CAAC,MAAM,IAAI,GAAG,CAAC,CAAC,MAAM,CAAC,EAAE,MAAM,EAAE,CAAC,CAAC,MAAM,EAAE,EAAE,KAAK,EAAE,CAAC,CAAC,MAAM,EAAE,EAAE,CAAC,CAAC;AAExE,MAAM,CAAC,MAAM,MAAM,GAAG,CAAC;KACpB,MAAM,CAAC;IACN,IAAI,EAAE,CAAC,CAAC,MAAM,EAAE;IAChB,QAAQ,EAAE,CAAC,CAAC,OAAO,EAAE,CAAC,QAAQ,EAAE;IAChC,aAAa,EAAE,CAAC,CAAC,MAAM,EAAE,CAAC,QAAQ,EAAE;IACpC,QAAQ,EAAE,CAAC,CAAC,MAAM,CAAC,EAAE,CAAC,EAAE,CAAC,CAAC,MAAM,EAAE,EAAE,CAAC,EAAE,CAAC,CAAC,MAAM,EAAE,EAAE,CAAC;IACpD,KAAK,EAAE,CAAC,CAAC,MAAM,EAAE;IACjB,MAAM,EAAE,CAAC,CAAC,MAAM,EAAE;IAClB,MAAM,EAAE,CAAC,CAAC,MAAM,EAAE,CAAC,QAAQ,EAAE;IAC7B,OAAO,EAAE,CAAC,CAAC,MAAM,EAAE,CAAC,QAAQ,EAAE;CAC/B,CAAC;KACD,WAAW,EAAE,CAAC;AAEjB,MAAM,yBAAyB,GAAG,CAAC,CAAC,MAAM,CAAC;IACzC,EAAE,EAAE,CAAC,CAAC,MAAM,EAAE;IACd,GAAG,EAAE,CAAC,CAAC,MAAM,EAAE;IACf,IAAI,EAAE,CAAC,CAAC,MAAM,EAAE;CACjB,CAAC,CAAC;AACH,MAAM,CAAC,MAAM,WAAW,GAAG,MAAM,CAAC,KAAK,CAAC,yBAAyB,CAAC,CAAC;AAEnE,MAAM,iBAAiB,GAA6B,CAAC,CAAC,GAAG,EAAE,CAAC,MAAM,CAAC,CAAC,CAAC,EAAE,EAAE,CAAC,CAAC,YAAY,WAAW,CAAC,CAAC;AACpG,MAAM,gBAAgB,GAA4B,CAAC,CAAC,GAAG,EAAE,CAAC,MAAM,CAAC,CAAC,CAAC,EAAE,EAAE,CAAC,CAAC,YAAY,UAAU,CAAC,CAAC;AAEjG,MAAM,CAAC,MAAM,IAAI,GAAG,CAAC,CAAC,MAAM,CAC1B,CAAC,CAAC,MAAM,CAAC;IACP,IAAI,EAAE,CAAC,CAAC,KAAK,CAAC,CAAC,CAAC,CAAC,MAAM,EAAE,EAAE,iBAAiB,EAAE,gBAAgB,CAAC,CAAC;IAChE,QAAQ,EAAE,CAAC,CAAC,OAAO,EAAE,CAAC,QAAQ,EAAE;IAChC,MAAM,EAAE,CAAC,CAAC,OAAO,EAAE,CAAC,QAAQ,EAAE;CAC/B,CAAC,CACH,CAAC;AAEF,MAAM,CAAC,MAAM,OAAO,GAAG,CAAC,CAAC,KAAK,CAAC,CAAC,CAAC,CAAC,MAAM,EAAE,EAAE,iBAAiB,EAAE,gBAAgB,CAAC,CAAC,CAAC;AAElF,MAAM,CAAC,MAAM,QAAQ,GAAG,CAAC,CAAC,MAAM,CAAC;IAC/B,OAAO,EAAE,CAAC,CAAC,KAAK,CAAC,CAAC,CAAC,MAAM,CAAC,MAAM,CAAC,CAAC;IAClC,OAAO,EAAE,OAAO;IAChB,UAAU,EAAE,CAAC,CAAC,KAAK,CAAC,CAAC,CAAC,MAAM,CAAC,CAAC,CAAC,MAAM,EAAE,CAAC,CAAC,CAAC,MAAM,CAAC,CAAC,CAAC,CAAC,QAAQ,EAAE;IAC9D,OAAO,EAAE,CAAC,CAAC,KAAK,CAAC,CAAC,CAAC,MAAM,EAAE,CAAC,CAAC,QAAQ,EAAE;CACxC,CAAC,CAAC;AAEH,MAAM,CAAC,MAAM,MAAM,GAAG,CAAC,CAAC,KAAK,CAAC,CAAC,CAAC,MAAM,CAAC,CAAC,CAAC,MAAM,EAAE,CAAC,CAAC,CAAC,GAAG,CAAC,CAAC,CAAC,CAAC;AAE3D,MAAM,aAAa,GAAG,CAAC,CAAC,MAAM,CAAC,EAAE,IAAI,EAAE,IAAI,CAAC,QAAQ,EAAE,EAAE,CAAC,CAAC,WAAW,EAAE,CAAC;AAExE,MAAM,WAAW,GAAG,CAAC,CAAC,MAAM,CAAC;IAC3B,QAAQ,EAAE,QAAQ;IAClB,OAAO,EAAE,aAAa,CAAC,QAAQ,EAAE;IACjC,OAAO,EAAE,CAAC,CAAC,MAAM,CAAC,CAAC,CAAC,MAAM,CAAC,EAAE,EAAE,EAAE,CAAC,CAAC,GAAG,EAAE,EAAE,GAAG,EAAE,CAAC,CAAC,GAAG,EAAE,EAAE,SAAS,EAAE,CAAC,CAAC,GAAG,EAAE,EAAE,CAAC,CAAC,CAAC,QAAQ,EAAE;CAC1F,CAAC,CAAC;AAEH,iDAAiD;AAEjD,MAAM,CAAC,MAAM,gBAAgB,GAAG,aAAa,CAAC,MAAM,CAAC;IACnD,MAAM,EAAE,CAAC,CAAC,MAAM,EAAE,CAAC,QAAQ,EAAE;IAC7B,YAAY,EAAE,CAAC,CAAC,IAAI,EAAE,CAAC,QAAQ,EAAE;IACjC,OAAO,EAAE,CAAC,CAAC,MAAM,EAAE,CAAC,QAAQ,EAAE;IAC9B,QAAQ,EAAE,CAAC,CAAC,KAAK,CAAC,CAAC,CAAC,MAAM,EAAE,CAAC,CAAC,QAAQ,EAAE;IACxC,QAAQ,EAAE,CAAC,CAAC,MAAM,EAAE,CAAC,QAAQ,EAAE;IAC/B,gBAAgB,EAAE,CAAC,CAAC,IAAI,EAAE,CAAC,QAAQ,EAAE;IACrC,QAAQ,EAAE,CAAC,CAAC,MAAM,EAAE,CAAC,QAAQ,EAAE;IAC/B,OAAO,EAAE,CAAC,CAAC,MAAM,EAAE,CAAC,QAAQ,EAAE;IAC9B,KAAK,EAAE,CAAC,CAAC,MAAM,EAAE,CAAC,QAAQ,EAAE;CAC7B,CAAC,CAAC;AAEH,MAAM,CAAC,MAAM,aAAa,GAAG,WAAW,CAAC,MAAM,CAAC;IAC9C,MAAM,EAAE,MAAM;IACd,OAAO,EAAE,gBAAgB,CAAC,QAAQ,EAAE;CACrC,CAAC,CAAC,MAAM,EAAE,CAAC;AAEZ,kDAAkD;AAElD,MAAM,CAAC,MAAM,SAAS,GAAG,aAAa,CAAC,MAAM,CAAC;IAC5C,IAAI,EAAE,IAAI,CAAC,QAAQ,EAAE;IACrB,MAAM,EAAE,CAAC,CAAC,MAAM,CAAC,CAAC,CAAC,MAAM,EAAE,EAAE,CAAC,CAAC,MAAM,EAAE,CAAC,CAAC,QAAQ,EAAE;IACnD,KAAK,EAAE,CAAC,CAAC,MAAM,CAAC,CAAC,CAAC,MAAM,EAAE,EAAE,CAAC,CAAC,OAAO,EAAE,CAAC,CAAC,QAAQ,EAAE;CACpD,CAAC,CAAC;AAEH,MAAM,iBAAiB,GAA6B,CAAC,CAAC,GAAG,EAAE,CAAC,MAAM,CAAC,CAAC,CAAC,EAAE,EAAE,CAAC,CAAC,YAAY,WAAW,CAAC,CAAC;AAEpG,MAAM,CAAC,MAAM,OAAO,GAAG,WAAW,CAAC,MAAM,CAAC;IACxC,YAAY,EAAE,iBAAiB;IAC/B,OAAO,EAAE,SAAS,CAAC,QAAQ,EAAE;CAC9B,CAAC,CAAC;AAEH,MAAM,CAAC,MAAM,YAAY,GAAG,OAAO,CAAC,MAAM,CAAC,EAAE,MAAM,EAAE,MAAM,EAAE,CAAC,CAAC,MAAM,EAAE,CAAC;AAExE,MAAM,CAAC,MAAM,aAAa,GAAG,OAAO,CAAC,MAAM,CAAC,EAAE,CAAC,CAAC,MAAM,EAAE,CAAC"}
|
@@ -1,5 +1,5 @@
|
|
1
1
|
import { MM_TO_PT_RATIO, PT_TO_MM_RATIO, PT_TO_PX_RATIO, BLANK_PDF, ZOOM, DEFAULT_FONT_NAME } from './constants.js';
|
2
|
-
import type { ChangeSchemas, PropPanel, PropPanelSchema, PropPanelWidgetProps, PDFRenderProps, UIRenderProps, Plugin, Lang, Dict, Size, Schema, SchemaForUI, Font, BasePdf, Template, GeneratorOptions, Plugins, GenerateProps, UIOptions, UIProps, PreviewProps, DesignerProps } from './types.js';
|
2
|
+
import type { ChangeSchemas, PropPanel, PropPanelSchema, PropPanelWidgetProps, PDFRenderProps, Mode, UIRenderProps, Plugin, Lang, Dict, Size, Schema, SchemaForUI, Font, BasePdf, Template, GeneratorOptions, Plugins, GenerateProps, UIOptions, UIProps, PreviewProps, DesignerProps } from './types.js';
|
3
3
|
import { getFallbackFontName, getDefaultFont, getB64BasePdf, b64toUint8Array, checkFont, checkInputs, checkUIOptions, checkTemplate, checkUIProps, checkPreviewProps, checkDesignerProps, checkGenerateProps, mm2pt, pt2mm, pt2px, isHexValid } from './helper.js';
|
4
4
|
export { MM_TO_PT_RATIO, PT_TO_MM_RATIO, PT_TO_PX_RATIO, BLANK_PDF, ZOOM, DEFAULT_FONT_NAME, getFallbackFontName, getDefaultFont, getB64BasePdf, b64toUint8Array, mm2pt, pt2mm, pt2px, isHexValid, checkFont, checkInputs, checkUIOptions, checkTemplate, checkUIProps, checkPreviewProps, checkDesignerProps, checkGenerateProps, };
|
5
|
-
export type { Lang, Dict, Size, Schema, SchemaForUI, Font, BasePdf, Template, GeneratorOptions, Plugins, GenerateProps, UIOptions, UIProps, PreviewProps, DesignerProps, ChangeSchemas, PropPanel, PropPanelSchema, PropPanelWidgetProps, PDFRenderProps, UIRenderProps, Plugin, };
|
5
|
+
export type { Lang, Dict, Size, Schema, SchemaForUI, Font, BasePdf, Template, GeneratorOptions, Plugins, GenerateProps, UIOptions, UIProps, PreviewProps, DesignerProps, ChangeSchemas, PropPanel, PropPanelSchema, PropPanelWidgetProps, PDFRenderProps, UIRenderProps, Mode, Plugin, };
|
@@ -1,5 +1,5 @@
|
|
1
1
|
import { z } from 'zod';
|
2
|
-
export declare const Lang: z.ZodEnum<["en", "ja", "ar", "th", "pl", "it"]>;
|
2
|
+
export declare const Lang: z.ZodEnum<["en", "ja", "ar", "th", "pl", "it", "de"]>;
|
3
3
|
export declare const Dict: z.ZodObject<{
|
4
4
|
cancel: z.ZodString;
|
5
5
|
field: z.ZodString;
|
@@ -22,6 +22,10 @@ export declare const Dict: z.ZodObject<{
|
|
22
22
|
errorBulkUpdateFieldName: z.ZodString;
|
23
23
|
commitBulkUpdateFieldName: z.ZodString;
|
24
24
|
bulkUpdateFieldName: z.ZodString;
|
25
|
+
hexColorPrompt: z.ZodString;
|
26
|
+
'schemas.color': z.ZodString;
|
27
|
+
'schemas.borderWidth': z.ZodString;
|
28
|
+
'schemas.borderColor': z.ZodString;
|
25
29
|
'schemas.textColor': z.ZodString;
|
26
30
|
'schemas.bgColor': z.ZodString;
|
27
31
|
'schemas.horizontal': z.ZodString;
|
@@ -65,6 +69,10 @@ export declare const Dict: z.ZodObject<{
|
|
65
69
|
errorBulkUpdateFieldName: string;
|
66
70
|
commitBulkUpdateFieldName: string;
|
67
71
|
bulkUpdateFieldName: string;
|
72
|
+
hexColorPrompt: string;
|
73
|
+
'schemas.color': string;
|
74
|
+
'schemas.borderWidth': string;
|
75
|
+
'schemas.borderColor': string;
|
68
76
|
'schemas.textColor': string;
|
69
77
|
'schemas.bgColor': string;
|
70
78
|
'schemas.horizontal': string;
|
@@ -108,6 +116,10 @@ export declare const Dict: z.ZodObject<{
|
|
108
116
|
errorBulkUpdateFieldName: string;
|
109
117
|
commitBulkUpdateFieldName: string;
|
110
118
|
bulkUpdateFieldName: string;
|
119
|
+
hexColorPrompt: string;
|
120
|
+
'schemas.color': string;
|
121
|
+
'schemas.borderWidth': string;
|
122
|
+
'schemas.borderColor': string;
|
111
123
|
'schemas.textColor': string;
|
112
124
|
'schemas.bgColor': string;
|
113
125
|
'schemas.horizontal': string;
|
@@ -130,6 +142,7 @@ export declare const Dict: z.ZodObject<{
|
|
130
142
|
'schemas.text.dynamicFontSize': string;
|
131
143
|
'schemas.barcodes.barColor': string;
|
132
144
|
}>;
|
145
|
+
export declare const Mode: z.ZodEnum<["viewer", "form", "designer"]>;
|
133
146
|
export declare const Size: z.ZodObject<{
|
134
147
|
height: z.ZodNumber;
|
135
148
|
width: z.ZodNumber;
|
@@ -142,6 +155,8 @@ export declare const Size: z.ZodObject<{
|
|
142
155
|
}>;
|
143
156
|
export declare const Schema: z.ZodObject<{
|
144
157
|
type: z.ZodString;
|
158
|
+
readOnly: z.ZodOptional<z.ZodBoolean>;
|
159
|
+
readOnlyValue: z.ZodOptional<z.ZodString>;
|
145
160
|
position: z.ZodObject<{
|
146
161
|
x: z.ZodNumber;
|
147
162
|
y: z.ZodNumber;
|
@@ -158,6 +173,8 @@ export declare const Schema: z.ZodObject<{
|
|
158
173
|
opacity: z.ZodOptional<z.ZodNumber>;
|
159
174
|
}, "passthrough", z.ZodTypeAny, z.objectOutputType<{
|
160
175
|
type: z.ZodString;
|
176
|
+
readOnly: z.ZodOptional<z.ZodBoolean>;
|
177
|
+
readOnlyValue: z.ZodOptional<z.ZodString>;
|
161
178
|
position: z.ZodObject<{
|
162
179
|
x: z.ZodNumber;
|
163
180
|
y: z.ZodNumber;
|
@@ -174,6 +191,8 @@ export declare const Schema: z.ZodObject<{
|
|
174
191
|
opacity: z.ZodOptional<z.ZodNumber>;
|
175
192
|
}, z.ZodTypeAny, "passthrough">, z.objectInputType<{
|
176
193
|
type: z.ZodString;
|
194
|
+
readOnly: z.ZodOptional<z.ZodBoolean>;
|
195
|
+
readOnlyValue: z.ZodOptional<z.ZodString>;
|
177
196
|
position: z.ZodObject<{
|
178
197
|
x: z.ZodNumber;
|
179
198
|
y: z.ZodNumber;
|
@@ -195,6 +214,8 @@ export declare const SchemaForUI: z.ZodObject<{
|
|
195
214
|
height: z.ZodNumber;
|
196
215
|
rotate: z.ZodOptional<z.ZodNumber>;
|
197
216
|
type: z.ZodString;
|
217
|
+
readOnly: z.ZodOptional<z.ZodBoolean>;
|
218
|
+
readOnlyValue: z.ZodOptional<z.ZodString>;
|
198
219
|
position: z.ZodObject<{
|
199
220
|
x: z.ZodNumber;
|
200
221
|
y: z.ZodNumber;
|
@@ -221,6 +242,8 @@ export declare const SchemaForUI: z.ZodObject<{
|
|
221
242
|
key: string;
|
222
243
|
opacity?: number | undefined;
|
223
244
|
rotate?: number | undefined;
|
245
|
+
readOnly?: boolean | undefined;
|
246
|
+
readOnlyValue?: string | undefined;
|
224
247
|
}, {
|
225
248
|
width: number;
|
226
249
|
height: number;
|
@@ -234,6 +257,8 @@ export declare const SchemaForUI: z.ZodObject<{
|
|
234
257
|
key: string;
|
235
258
|
opacity?: number | undefined;
|
236
259
|
rotate?: number | undefined;
|
260
|
+
readOnly?: boolean | undefined;
|
261
|
+
readOnlyValue?: string | undefined;
|
237
262
|
}>;
|
238
263
|
export declare const Font: z.ZodRecord<z.ZodString, z.ZodObject<{
|
239
264
|
data: z.ZodUnion<[z.ZodString, z.ZodType<ArrayBuffer, z.ZodTypeDef, ArrayBuffer>, z.ZodType<Uint8Array, z.ZodTypeDef, Uint8Array>]>;
|
@@ -252,6 +277,8 @@ export declare const BasePdf: z.ZodUnion<[z.ZodString, z.ZodType<ArrayBuffer, z.
|
|
252
277
|
export declare const Template: z.ZodObject<{
|
253
278
|
schemas: z.ZodArray<z.ZodRecord<z.ZodString, z.ZodObject<{
|
254
279
|
type: z.ZodString;
|
280
|
+
readOnly: z.ZodOptional<z.ZodBoolean>;
|
281
|
+
readOnlyValue: z.ZodOptional<z.ZodString>;
|
255
282
|
position: z.ZodObject<{
|
256
283
|
x: z.ZodNumber;
|
257
284
|
y: z.ZodNumber;
|
@@ -268,6 +295,8 @@ export declare const Template: z.ZodObject<{
|
|
268
295
|
opacity: z.ZodOptional<z.ZodNumber>;
|
269
296
|
}, "passthrough", z.ZodTypeAny, z.objectOutputType<{
|
270
297
|
type: z.ZodString;
|
298
|
+
readOnly: z.ZodOptional<z.ZodBoolean>;
|
299
|
+
readOnlyValue: z.ZodOptional<z.ZodString>;
|
271
300
|
position: z.ZodObject<{
|
272
301
|
x: z.ZodNumber;
|
273
302
|
y: z.ZodNumber;
|
@@ -284,6 +313,8 @@ export declare const Template: z.ZodObject<{
|
|
284
313
|
opacity: z.ZodOptional<z.ZodNumber>;
|
285
314
|
}, z.ZodTypeAny, "passthrough">, z.objectInputType<{
|
286
315
|
type: z.ZodString;
|
316
|
+
readOnly: z.ZodOptional<z.ZodBoolean>;
|
317
|
+
readOnlyValue: z.ZodOptional<z.ZodString>;
|
287
318
|
position: z.ZodObject<{
|
288
319
|
x: z.ZodNumber;
|
289
320
|
y: z.ZodNumber;
|
@@ -305,6 +336,8 @@ export declare const Template: z.ZodObject<{
|
|
305
336
|
}, "strip", z.ZodTypeAny, {
|
306
337
|
schemas: Record<string, z.objectOutputType<{
|
307
338
|
type: z.ZodString;
|
339
|
+
readOnly: z.ZodOptional<z.ZodBoolean>;
|
340
|
+
readOnlyValue: z.ZodOptional<z.ZodString>;
|
308
341
|
position: z.ZodObject<{
|
309
342
|
x: z.ZodNumber;
|
310
343
|
y: z.ZodNumber;
|
@@ -326,6 +359,8 @@ export declare const Template: z.ZodObject<{
|
|
326
359
|
}, {
|
327
360
|
schemas: Record<string, z.objectInputType<{
|
328
361
|
type: z.ZodString;
|
362
|
+
readOnly: z.ZodOptional<z.ZodBoolean>;
|
363
|
+
readOnlyValue: z.ZodOptional<z.ZodString>;
|
329
364
|
position: z.ZodObject<{
|
330
365
|
x: z.ZodNumber;
|
331
366
|
y: z.ZodNumber;
|
@@ -420,6 +455,8 @@ export declare const GenerateProps: z.ZodObject<{
|
|
420
455
|
template: z.ZodObject<{
|
421
456
|
schemas: z.ZodArray<z.ZodRecord<z.ZodString, z.ZodObject<{
|
422
457
|
type: z.ZodString;
|
458
|
+
readOnly: z.ZodOptional<z.ZodBoolean>;
|
459
|
+
readOnlyValue: z.ZodOptional<z.ZodString>;
|
423
460
|
position: z.ZodObject<{
|
424
461
|
x: z.ZodNumber;
|
425
462
|
y: z.ZodNumber;
|
@@ -436,6 +473,8 @@ export declare const GenerateProps: z.ZodObject<{
|
|
436
473
|
opacity: z.ZodOptional<z.ZodNumber>;
|
437
474
|
}, "passthrough", z.ZodTypeAny, z.objectOutputType<{
|
438
475
|
type: z.ZodString;
|
476
|
+
readOnly: z.ZodOptional<z.ZodBoolean>;
|
477
|
+
readOnlyValue: z.ZodOptional<z.ZodString>;
|
439
478
|
position: z.ZodObject<{
|
440
479
|
x: z.ZodNumber;
|
441
480
|
y: z.ZodNumber;
|
@@ -452,6 +491,8 @@ export declare const GenerateProps: z.ZodObject<{
|
|
452
491
|
opacity: z.ZodOptional<z.ZodNumber>;
|
453
492
|
}, z.ZodTypeAny, "passthrough">, z.objectInputType<{
|
454
493
|
type: z.ZodString;
|
494
|
+
readOnly: z.ZodOptional<z.ZodBoolean>;
|
495
|
+
readOnlyValue: z.ZodOptional<z.ZodString>;
|
455
496
|
position: z.ZodObject<{
|
456
497
|
x: z.ZodNumber;
|
457
498
|
y: z.ZodNumber;
|
@@ -473,6 +514,8 @@ export declare const GenerateProps: z.ZodObject<{
|
|
473
514
|
}, "strip", z.ZodTypeAny, {
|
474
515
|
schemas: Record<string, z.objectOutputType<{
|
475
516
|
type: z.ZodString;
|
517
|
+
readOnly: z.ZodOptional<z.ZodBoolean>;
|
518
|
+
readOnlyValue: z.ZodOptional<z.ZodString>;
|
476
519
|
position: z.ZodObject<{
|
477
520
|
x: z.ZodNumber;
|
478
521
|
y: z.ZodNumber;
|
@@ -494,6 +537,8 @@ export declare const GenerateProps: z.ZodObject<{
|
|
494
537
|
}, {
|
495
538
|
schemas: Record<string, z.objectInputType<{
|
496
539
|
type: z.ZodString;
|
540
|
+
readOnly: z.ZodOptional<z.ZodBoolean>;
|
541
|
+
readOnlyValue: z.ZodOptional<z.ZodString>;
|
497
542
|
position: z.ZodObject<{
|
498
543
|
x: z.ZodNumber;
|
499
544
|
y: z.ZodNumber;
|
@@ -601,6 +646,8 @@ export declare const GenerateProps: z.ZodObject<{
|
|
601
646
|
template: {
|
602
647
|
schemas: Record<string, z.objectOutputType<{
|
603
648
|
type: z.ZodString;
|
649
|
+
readOnly: z.ZodOptional<z.ZodBoolean>;
|
650
|
+
readOnlyValue: z.ZodOptional<z.ZodString>;
|
604
651
|
position: z.ZodObject<{
|
605
652
|
x: z.ZodNumber;
|
606
653
|
y: z.ZodNumber;
|
@@ -654,6 +701,8 @@ export declare const GenerateProps: z.ZodObject<{
|
|
654
701
|
template: {
|
655
702
|
schemas: Record<string, z.objectInputType<{
|
656
703
|
type: z.ZodString;
|
704
|
+
readOnly: z.ZodOptional<z.ZodBoolean>;
|
705
|
+
readOnlyValue: z.ZodOptional<z.ZodString>;
|
657
706
|
position: z.ZodObject<{
|
658
707
|
x: z.ZodNumber;
|
659
708
|
y: z.ZodNumber;
|
@@ -718,7 +767,7 @@ export declare const UIOptions: z.ZodObject<{
|
|
718
767
|
fallback?: boolean | undefined;
|
719
768
|
subset?: boolean | undefined;
|
720
769
|
}>>>;
|
721
|
-
lang: z.ZodOptional<z.ZodEnum<["en", "ja", "ar", "th", "pl", "it"]>>;
|
770
|
+
lang: z.ZodOptional<z.ZodEnum<["en", "ja", "ar", "th", "pl", "it", "de"]>>;
|
722
771
|
labels: z.ZodOptional<z.ZodRecord<z.ZodString, z.ZodString>>;
|
723
772
|
theme: z.ZodOptional<z.ZodRecord<z.ZodString, z.ZodUnknown>>;
|
724
773
|
}, "passthrough", z.ZodTypeAny, z.objectOutputType<{
|
@@ -735,7 +784,7 @@ export declare const UIOptions: z.ZodObject<{
|
|
735
784
|
fallback?: boolean | undefined;
|
736
785
|
subset?: boolean | undefined;
|
737
786
|
}>>>;
|
738
|
-
lang: z.ZodOptional<z.ZodEnum<["en", "ja", "ar", "th", "pl", "it"]>>;
|
787
|
+
lang: z.ZodOptional<z.ZodEnum<["en", "ja", "ar", "th", "pl", "it", "de"]>>;
|
739
788
|
labels: z.ZodOptional<z.ZodRecord<z.ZodString, z.ZodString>>;
|
740
789
|
theme: z.ZodOptional<z.ZodRecord<z.ZodString, z.ZodUnknown>>;
|
741
790
|
}, z.ZodTypeAny, "passthrough">, z.objectInputType<{
|
@@ -752,7 +801,7 @@ export declare const UIOptions: z.ZodObject<{
|
|
752
801
|
fallback?: boolean | undefined;
|
753
802
|
subset?: boolean | undefined;
|
754
803
|
}>>>;
|
755
|
-
lang: z.ZodOptional<z.ZodEnum<["en", "ja", "ar", "th", "pl", "it"]>>;
|
804
|
+
lang: z.ZodOptional<z.ZodEnum<["en", "ja", "ar", "th", "pl", "it", "de"]>>;
|
756
805
|
labels: z.ZodOptional<z.ZodRecord<z.ZodString, z.ZodString>>;
|
757
806
|
theme: z.ZodOptional<z.ZodRecord<z.ZodString, z.ZodUnknown>>;
|
758
807
|
}, z.ZodTypeAny, "passthrough">>;
|
@@ -760,6 +809,8 @@ export declare const UIProps: z.ZodObject<{
|
|
760
809
|
template: z.ZodObject<{
|
761
810
|
schemas: z.ZodArray<z.ZodRecord<z.ZodString, z.ZodObject<{
|
762
811
|
type: z.ZodString;
|
812
|
+
readOnly: z.ZodOptional<z.ZodBoolean>;
|
813
|
+
readOnlyValue: z.ZodOptional<z.ZodString>;
|
763
814
|
position: z.ZodObject<{
|
764
815
|
x: z.ZodNumber;
|
765
816
|
y: z.ZodNumber;
|
@@ -776,6 +827,8 @@ export declare const UIProps: z.ZodObject<{
|
|
776
827
|
opacity: z.ZodOptional<z.ZodNumber>;
|
777
828
|
}, "passthrough", z.ZodTypeAny, z.objectOutputType<{
|
778
829
|
type: z.ZodString;
|
830
|
+
readOnly: z.ZodOptional<z.ZodBoolean>;
|
831
|
+
readOnlyValue: z.ZodOptional<z.ZodString>;
|
779
832
|
position: z.ZodObject<{
|
780
833
|
x: z.ZodNumber;
|
781
834
|
y: z.ZodNumber;
|
@@ -792,6 +845,8 @@ export declare const UIProps: z.ZodObject<{
|
|
792
845
|
opacity: z.ZodOptional<z.ZodNumber>;
|
793
846
|
}, z.ZodTypeAny, "passthrough">, z.objectInputType<{
|
794
847
|
type: z.ZodString;
|
848
|
+
readOnly: z.ZodOptional<z.ZodBoolean>;
|
849
|
+
readOnlyValue: z.ZodOptional<z.ZodString>;
|
795
850
|
position: z.ZodObject<{
|
796
851
|
x: z.ZodNumber;
|
797
852
|
y: z.ZodNumber;
|
@@ -813,6 +868,8 @@ export declare const UIProps: z.ZodObject<{
|
|
813
868
|
}, "strip", z.ZodTypeAny, {
|
814
869
|
schemas: Record<string, z.objectOutputType<{
|
815
870
|
type: z.ZodString;
|
871
|
+
readOnly: z.ZodOptional<z.ZodBoolean>;
|
872
|
+
readOnlyValue: z.ZodOptional<z.ZodString>;
|
816
873
|
position: z.ZodObject<{
|
817
874
|
x: z.ZodNumber;
|
818
875
|
y: z.ZodNumber;
|
@@ -834,6 +891,8 @@ export declare const UIProps: z.ZodObject<{
|
|
834
891
|
}, {
|
835
892
|
schemas: Record<string, z.objectInputType<{
|
836
893
|
type: z.ZodString;
|
894
|
+
readOnly: z.ZodOptional<z.ZodBoolean>;
|
895
|
+
readOnlyValue: z.ZodOptional<z.ZodString>;
|
837
896
|
position: z.ZodObject<{
|
838
897
|
x: z.ZodNumber;
|
839
898
|
y: z.ZodNumber;
|
@@ -881,7 +940,7 @@ export declare const UIProps: z.ZodObject<{
|
|
881
940
|
fallback?: boolean | undefined;
|
882
941
|
subset?: boolean | undefined;
|
883
942
|
}>>>;
|
884
|
-
lang: z.ZodOptional<z.ZodEnum<["en", "ja", "ar", "th", "pl", "it"]>>;
|
943
|
+
lang: z.ZodOptional<z.ZodEnum<["en", "ja", "ar", "th", "pl", "it", "de"]>>;
|
885
944
|
labels: z.ZodOptional<z.ZodRecord<z.ZodString, z.ZodString>>;
|
886
945
|
theme: z.ZodOptional<z.ZodRecord<z.ZodString, z.ZodUnknown>>;
|
887
946
|
}, "passthrough", z.ZodTypeAny, z.objectOutputType<{
|
@@ -898,7 +957,7 @@ export declare const UIProps: z.ZodObject<{
|
|
898
957
|
fallback?: boolean | undefined;
|
899
958
|
subset?: boolean | undefined;
|
900
959
|
}>>>;
|
901
|
-
lang: z.ZodOptional<z.ZodEnum<["en", "ja", "ar", "th", "pl", "it"]>>;
|
960
|
+
lang: z.ZodOptional<z.ZodEnum<["en", "ja", "ar", "th", "pl", "it", "de"]>>;
|
902
961
|
labels: z.ZodOptional<z.ZodRecord<z.ZodString, z.ZodString>>;
|
903
962
|
theme: z.ZodOptional<z.ZodRecord<z.ZodString, z.ZodUnknown>>;
|
904
963
|
}, z.ZodTypeAny, "passthrough">, z.objectInputType<{
|
@@ -915,7 +974,7 @@ export declare const UIProps: z.ZodObject<{
|
|
915
974
|
fallback?: boolean | undefined;
|
916
975
|
subset?: boolean | undefined;
|
917
976
|
}>>>;
|
918
|
-
lang: z.ZodOptional<z.ZodEnum<["en", "ja", "ar", "th", "pl", "it"]>>;
|
977
|
+
lang: z.ZodOptional<z.ZodEnum<["en", "ja", "ar", "th", "pl", "it", "de"]>>;
|
919
978
|
labels: z.ZodOptional<z.ZodRecord<z.ZodString, z.ZodString>>;
|
920
979
|
theme: z.ZodOptional<z.ZodRecord<z.ZodString, z.ZodUnknown>>;
|
921
980
|
}, z.ZodTypeAny, "passthrough">>>;
|
@@ -923,6 +982,8 @@ export declare const UIProps: z.ZodObject<{
|
|
923
982
|
template: {
|
924
983
|
schemas: Record<string, z.objectOutputType<{
|
925
984
|
type: z.ZodString;
|
985
|
+
readOnly: z.ZodOptional<z.ZodBoolean>;
|
986
|
+
readOnlyValue: z.ZodOptional<z.ZodString>;
|
926
987
|
position: z.ZodObject<{
|
927
988
|
x: z.ZodNumber;
|
928
989
|
y: z.ZodNumber;
|
@@ -962,7 +1023,7 @@ export declare const UIProps: z.ZodObject<{
|
|
962
1023
|
fallback?: boolean | undefined;
|
963
1024
|
subset?: boolean | undefined;
|
964
1025
|
}>>>;
|
965
|
-
lang: z.ZodOptional<z.ZodEnum<["en", "ja", "ar", "th", "pl", "it"]>>;
|
1026
|
+
lang: z.ZodOptional<z.ZodEnum<["en", "ja", "ar", "th", "pl", "it", "de"]>>;
|
966
1027
|
labels: z.ZodOptional<z.ZodRecord<z.ZodString, z.ZodString>>;
|
967
1028
|
theme: z.ZodOptional<z.ZodRecord<z.ZodString, z.ZodUnknown>>;
|
968
1029
|
}, z.ZodTypeAny, "passthrough"> | undefined;
|
@@ -970,6 +1031,8 @@ export declare const UIProps: z.ZodObject<{
|
|
970
1031
|
template: {
|
971
1032
|
schemas: Record<string, z.objectInputType<{
|
972
1033
|
type: z.ZodString;
|
1034
|
+
readOnly: z.ZodOptional<z.ZodBoolean>;
|
1035
|
+
readOnlyValue: z.ZodOptional<z.ZodString>;
|
973
1036
|
position: z.ZodObject<{
|
974
1037
|
x: z.ZodNumber;
|
975
1038
|
y: z.ZodNumber;
|
@@ -1009,7 +1072,7 @@ export declare const UIProps: z.ZodObject<{
|
|
1009
1072
|
fallback?: boolean | undefined;
|
1010
1073
|
subset?: boolean | undefined;
|
1011
1074
|
}>>>;
|
1012
|
-
lang: z.ZodOptional<z.ZodEnum<["en", "ja", "ar", "th", "pl", "it"]>>;
|
1075
|
+
lang: z.ZodOptional<z.ZodEnum<["en", "ja", "ar", "th", "pl", "it", "de"]>>;
|
1013
1076
|
labels: z.ZodOptional<z.ZodRecord<z.ZodString, z.ZodString>>;
|
1014
1077
|
theme: z.ZodOptional<z.ZodRecord<z.ZodString, z.ZodUnknown>>;
|
1015
1078
|
}, z.ZodTypeAny, "passthrough"> | undefined;
|
@@ -1029,7 +1092,7 @@ export declare const PreviewProps: z.ZodObject<{
|
|
1029
1092
|
fallback?: boolean | undefined;
|
1030
1093
|
subset?: boolean | undefined;
|
1031
1094
|
}>>>;
|
1032
|
-
lang: z.ZodOptional<z.ZodEnum<["en", "ja", "ar", "th", "pl", "it"]>>;
|
1095
|
+
lang: z.ZodOptional<z.ZodEnum<["en", "ja", "ar", "th", "pl", "it", "de"]>>;
|
1033
1096
|
labels: z.ZodOptional<z.ZodRecord<z.ZodString, z.ZodString>>;
|
1034
1097
|
theme: z.ZodOptional<z.ZodRecord<z.ZodString, z.ZodUnknown>>;
|
1035
1098
|
}, "passthrough", z.ZodTypeAny, z.objectOutputType<{
|
@@ -1046,7 +1109,7 @@ export declare const PreviewProps: z.ZodObject<{
|
|
1046
1109
|
fallback?: boolean | undefined;
|
1047
1110
|
subset?: boolean | undefined;
|
1048
1111
|
}>>>;
|
1049
|
-
lang: z.ZodOptional<z.ZodEnum<["en", "ja", "ar", "th", "pl", "it"]>>;
|
1112
|
+
lang: z.ZodOptional<z.ZodEnum<["en", "ja", "ar", "th", "pl", "it", "de"]>>;
|
1050
1113
|
labels: z.ZodOptional<z.ZodRecord<z.ZodString, z.ZodString>>;
|
1051
1114
|
theme: z.ZodOptional<z.ZodRecord<z.ZodString, z.ZodUnknown>>;
|
1052
1115
|
}, z.ZodTypeAny, "passthrough">, z.objectInputType<{
|
@@ -1063,13 +1126,15 @@ export declare const PreviewProps: z.ZodObject<{
|
|
1063
1126
|
fallback?: boolean | undefined;
|
1064
1127
|
subset?: boolean | undefined;
|
1065
1128
|
}>>>;
|
1066
|
-
lang: z.ZodOptional<z.ZodEnum<["en", "ja", "ar", "th", "pl", "it"]>>;
|
1129
|
+
lang: z.ZodOptional<z.ZodEnum<["en", "ja", "ar", "th", "pl", "it", "de"]>>;
|
1067
1130
|
labels: z.ZodOptional<z.ZodRecord<z.ZodString, z.ZodString>>;
|
1068
1131
|
theme: z.ZodOptional<z.ZodRecord<z.ZodString, z.ZodUnknown>>;
|
1069
1132
|
}, z.ZodTypeAny, "passthrough">>>;
|
1070
1133
|
template: z.ZodObject<{
|
1071
1134
|
schemas: z.ZodArray<z.ZodRecord<z.ZodString, z.ZodObject<{
|
1072
1135
|
type: z.ZodString;
|
1136
|
+
readOnly: z.ZodOptional<z.ZodBoolean>;
|
1137
|
+
readOnlyValue: z.ZodOptional<z.ZodString>;
|
1073
1138
|
position: z.ZodObject<{
|
1074
1139
|
x: z.ZodNumber;
|
1075
1140
|
y: z.ZodNumber;
|
@@ -1086,6 +1151,8 @@ export declare const PreviewProps: z.ZodObject<{
|
|
1086
1151
|
opacity: z.ZodOptional<z.ZodNumber>;
|
1087
1152
|
}, "passthrough", z.ZodTypeAny, z.objectOutputType<{
|
1088
1153
|
type: z.ZodString;
|
1154
|
+
readOnly: z.ZodOptional<z.ZodBoolean>;
|
1155
|
+
readOnlyValue: z.ZodOptional<z.ZodString>;
|
1089
1156
|
position: z.ZodObject<{
|
1090
1157
|
x: z.ZodNumber;
|
1091
1158
|
y: z.ZodNumber;
|
@@ -1102,6 +1169,8 @@ export declare const PreviewProps: z.ZodObject<{
|
|
1102
1169
|
opacity: z.ZodOptional<z.ZodNumber>;
|
1103
1170
|
}, z.ZodTypeAny, "passthrough">, z.objectInputType<{
|
1104
1171
|
type: z.ZodString;
|
1172
|
+
readOnly: z.ZodOptional<z.ZodBoolean>;
|
1173
|
+
readOnlyValue: z.ZodOptional<z.ZodString>;
|
1105
1174
|
position: z.ZodObject<{
|
1106
1175
|
x: z.ZodNumber;
|
1107
1176
|
y: z.ZodNumber;
|
@@ -1123,6 +1192,8 @@ export declare const PreviewProps: z.ZodObject<{
|
|
1123
1192
|
}, "strip", z.ZodTypeAny, {
|
1124
1193
|
schemas: Record<string, z.objectOutputType<{
|
1125
1194
|
type: z.ZodString;
|
1195
|
+
readOnly: z.ZodOptional<z.ZodBoolean>;
|
1196
|
+
readOnlyValue: z.ZodOptional<z.ZodString>;
|
1126
1197
|
position: z.ZodObject<{
|
1127
1198
|
x: z.ZodNumber;
|
1128
1199
|
y: z.ZodNumber;
|
@@ -1144,6 +1215,8 @@ export declare const PreviewProps: z.ZodObject<{
|
|
1144
1215
|
}, {
|
1145
1216
|
schemas: Record<string, z.objectInputType<{
|
1146
1217
|
type: z.ZodString;
|
1218
|
+
readOnly: z.ZodOptional<z.ZodBoolean>;
|
1219
|
+
readOnlyValue: z.ZodOptional<z.ZodString>;
|
1147
1220
|
position: z.ZodObject<{
|
1148
1221
|
x: z.ZodNumber;
|
1149
1222
|
y: z.ZodNumber;
|
@@ -1182,6 +1255,8 @@ export declare const PreviewProps: z.ZodObject<{
|
|
1182
1255
|
template: {
|
1183
1256
|
schemas: Record<string, z.objectOutputType<{
|
1184
1257
|
type: z.ZodString;
|
1258
|
+
readOnly: z.ZodOptional<z.ZodBoolean>;
|
1259
|
+
readOnlyValue: z.ZodOptional<z.ZodString>;
|
1185
1260
|
position: z.ZodObject<{
|
1186
1261
|
x: z.ZodNumber;
|
1187
1262
|
y: z.ZodNumber;
|
@@ -1217,7 +1292,7 @@ export declare const PreviewProps: z.ZodObject<{
|
|
1217
1292
|
fallback?: boolean | undefined;
|
1218
1293
|
subset?: boolean | undefined;
|
1219
1294
|
}>>>;
|
1220
|
-
lang: z.ZodOptional<z.ZodEnum<["en", "ja", "ar", "th", "pl", "it"]>>;
|
1295
|
+
lang: z.ZodOptional<z.ZodEnum<["en", "ja", "ar", "th", "pl", "it", "de"]>>;
|
1221
1296
|
labels: z.ZodOptional<z.ZodRecord<z.ZodString, z.ZodString>>;
|
1222
1297
|
theme: z.ZodOptional<z.ZodRecord<z.ZodString, z.ZodUnknown>>;
|
1223
1298
|
}, z.ZodTypeAny, "passthrough"> | undefined;
|
@@ -1230,6 +1305,8 @@ export declare const PreviewProps: z.ZodObject<{
|
|
1230
1305
|
template: {
|
1231
1306
|
schemas: Record<string, z.objectInputType<{
|
1232
1307
|
type: z.ZodString;
|
1308
|
+
readOnly: z.ZodOptional<z.ZodBoolean>;
|
1309
|
+
readOnlyValue: z.ZodOptional<z.ZodString>;
|
1233
1310
|
position: z.ZodObject<{
|
1234
1311
|
x: z.ZodNumber;
|
1235
1312
|
y: z.ZodNumber;
|
@@ -1265,7 +1342,7 @@ export declare const PreviewProps: z.ZodObject<{
|
|
1265
1342
|
fallback?: boolean | undefined;
|
1266
1343
|
subset?: boolean | undefined;
|
1267
1344
|
}>>>;
|
1268
|
-
lang: z.ZodOptional<z.ZodEnum<["en", "ja", "ar", "th", "pl", "it"]>>;
|
1345
|
+
lang: z.ZodOptional<z.ZodEnum<["en", "ja", "ar", "th", "pl", "it", "de"]>>;
|
1269
1346
|
labels: z.ZodOptional<z.ZodRecord<z.ZodString, z.ZodString>>;
|
1270
1347
|
theme: z.ZodOptional<z.ZodRecord<z.ZodString, z.ZodUnknown>>;
|
1271
1348
|
}, z.ZodTypeAny, "passthrough"> | undefined;
|
@@ -1290,7 +1367,7 @@ export declare const DesignerProps: z.ZodObject<{
|
|
1290
1367
|
fallback?: boolean | undefined;
|
1291
1368
|
subset?: boolean | undefined;
|
1292
1369
|
}>>>;
|
1293
|
-
lang: z.ZodOptional<z.ZodEnum<["en", "ja", "ar", "th", "pl", "it"]>>;
|
1370
|
+
lang: z.ZodOptional<z.ZodEnum<["en", "ja", "ar", "th", "pl", "it", "de"]>>;
|
1294
1371
|
labels: z.ZodOptional<z.ZodRecord<z.ZodString, z.ZodString>>;
|
1295
1372
|
theme: z.ZodOptional<z.ZodRecord<z.ZodString, z.ZodUnknown>>;
|
1296
1373
|
}, "passthrough", z.ZodTypeAny, z.objectOutputType<{
|
@@ -1307,7 +1384,7 @@ export declare const DesignerProps: z.ZodObject<{
|
|
1307
1384
|
fallback?: boolean | undefined;
|
1308
1385
|
subset?: boolean | undefined;
|
1309
1386
|
}>>>;
|
1310
|
-
lang: z.ZodOptional<z.ZodEnum<["en", "ja", "ar", "th", "pl", "it"]>>;
|
1387
|
+
lang: z.ZodOptional<z.ZodEnum<["en", "ja", "ar", "th", "pl", "it", "de"]>>;
|
1311
1388
|
labels: z.ZodOptional<z.ZodRecord<z.ZodString, z.ZodString>>;
|
1312
1389
|
theme: z.ZodOptional<z.ZodRecord<z.ZodString, z.ZodUnknown>>;
|
1313
1390
|
}, z.ZodTypeAny, "passthrough">, z.objectInputType<{
|
@@ -1324,13 +1401,15 @@ export declare const DesignerProps: z.ZodObject<{
|
|
1324
1401
|
fallback?: boolean | undefined;
|
1325
1402
|
subset?: boolean | undefined;
|
1326
1403
|
}>>>;
|
1327
|
-
lang: z.ZodOptional<z.ZodEnum<["en", "ja", "ar", "th", "pl", "it"]>>;
|
1404
|
+
lang: z.ZodOptional<z.ZodEnum<["en", "ja", "ar", "th", "pl", "it", "de"]>>;
|
1328
1405
|
labels: z.ZodOptional<z.ZodRecord<z.ZodString, z.ZodString>>;
|
1329
1406
|
theme: z.ZodOptional<z.ZodRecord<z.ZodString, z.ZodUnknown>>;
|
1330
1407
|
}, z.ZodTypeAny, "passthrough">>>;
|
1331
1408
|
template: z.ZodObject<{
|
1332
1409
|
schemas: z.ZodArray<z.ZodRecord<z.ZodString, z.ZodObject<{
|
1333
1410
|
type: z.ZodString;
|
1411
|
+
readOnly: z.ZodOptional<z.ZodBoolean>;
|
1412
|
+
readOnlyValue: z.ZodOptional<z.ZodString>;
|
1334
1413
|
position: z.ZodObject<{
|
1335
1414
|
x: z.ZodNumber;
|
1336
1415
|
y: z.ZodNumber;
|
@@ -1347,6 +1426,8 @@ export declare const DesignerProps: z.ZodObject<{
|
|
1347
1426
|
opacity: z.ZodOptional<z.ZodNumber>;
|
1348
1427
|
}, "passthrough", z.ZodTypeAny, z.objectOutputType<{
|
1349
1428
|
type: z.ZodString;
|
1429
|
+
readOnly: z.ZodOptional<z.ZodBoolean>;
|
1430
|
+
readOnlyValue: z.ZodOptional<z.ZodString>;
|
1350
1431
|
position: z.ZodObject<{
|
1351
1432
|
x: z.ZodNumber;
|
1352
1433
|
y: z.ZodNumber;
|
@@ -1363,6 +1444,8 @@ export declare const DesignerProps: z.ZodObject<{
|
|
1363
1444
|
opacity: z.ZodOptional<z.ZodNumber>;
|
1364
1445
|
}, z.ZodTypeAny, "passthrough">, z.objectInputType<{
|
1365
1446
|
type: z.ZodString;
|
1447
|
+
readOnly: z.ZodOptional<z.ZodBoolean>;
|
1448
|
+
readOnlyValue: z.ZodOptional<z.ZodString>;
|
1366
1449
|
position: z.ZodObject<{
|
1367
1450
|
x: z.ZodNumber;
|
1368
1451
|
y: z.ZodNumber;
|
@@ -1384,6 +1467,8 @@ export declare const DesignerProps: z.ZodObject<{
|
|
1384
1467
|
}, "strip", z.ZodTypeAny, {
|
1385
1468
|
schemas: Record<string, z.objectOutputType<{
|
1386
1469
|
type: z.ZodString;
|
1470
|
+
readOnly: z.ZodOptional<z.ZodBoolean>;
|
1471
|
+
readOnlyValue: z.ZodOptional<z.ZodString>;
|
1387
1472
|
position: z.ZodObject<{
|
1388
1473
|
x: z.ZodNumber;
|
1389
1474
|
y: z.ZodNumber;
|
@@ -1405,6 +1490,8 @@ export declare const DesignerProps: z.ZodObject<{
|
|
1405
1490
|
}, {
|
1406
1491
|
schemas: Record<string, z.objectInputType<{
|
1407
1492
|
type: z.ZodString;
|
1493
|
+
readOnly: z.ZodOptional<z.ZodBoolean>;
|
1494
|
+
readOnlyValue: z.ZodOptional<z.ZodString>;
|
1408
1495
|
position: z.ZodObject<{
|
1409
1496
|
x: z.ZodNumber;
|
1410
1497
|
y: z.ZodNumber;
|
@@ -1442,6 +1529,8 @@ export declare const DesignerProps: z.ZodObject<{
|
|
1442
1529
|
template: {
|
1443
1530
|
schemas: Record<string, z.objectOutputType<{
|
1444
1531
|
type: z.ZodString;
|
1532
|
+
readOnly: z.ZodOptional<z.ZodBoolean>;
|
1533
|
+
readOnlyValue: z.ZodOptional<z.ZodString>;
|
1445
1534
|
position: z.ZodObject<{
|
1446
1535
|
x: z.ZodNumber;
|
1447
1536
|
y: z.ZodNumber;
|
@@ -1476,7 +1565,7 @@ export declare const DesignerProps: z.ZodObject<{
|
|
1476
1565
|
fallback?: boolean | undefined;
|
1477
1566
|
subset?: boolean | undefined;
|
1478
1567
|
}>>>;
|
1479
|
-
lang: z.ZodOptional<z.ZodEnum<["en", "ja", "ar", "th", "pl", "it"]>>;
|
1568
|
+
lang: z.ZodOptional<z.ZodEnum<["en", "ja", "ar", "th", "pl", "it", "de"]>>;
|
1480
1569
|
labels: z.ZodOptional<z.ZodRecord<z.ZodString, z.ZodString>>;
|
1481
1570
|
theme: z.ZodOptional<z.ZodRecord<z.ZodString, z.ZodUnknown>>;
|
1482
1571
|
}, z.ZodTypeAny, "passthrough"> | undefined;
|
@@ -1489,6 +1578,8 @@ export declare const DesignerProps: z.ZodObject<{
|
|
1489
1578
|
template: {
|
1490
1579
|
schemas: Record<string, z.objectInputType<{
|
1491
1580
|
type: z.ZodString;
|
1581
|
+
readOnly: z.ZodOptional<z.ZodBoolean>;
|
1582
|
+
readOnlyValue: z.ZodOptional<z.ZodString>;
|
1492
1583
|
position: z.ZodObject<{
|
1493
1584
|
x: z.ZodNumber;
|
1494
1585
|
y: z.ZodNumber;
|
@@ -1523,7 +1614,7 @@ export declare const DesignerProps: z.ZodObject<{
|
|
1523
1614
|
fallback?: boolean | undefined;
|
1524
1615
|
subset?: boolean | undefined;
|
1525
1616
|
}>>>;
|
1526
|
-
lang: z.ZodOptional<z.ZodEnum<["en", "ja", "ar", "th", "pl", "it"]>>;
|
1617
|
+
lang: z.ZodOptional<z.ZodEnum<["en", "ja", "ar", "th", "pl", "it", "de"]>>;
|
1527
1618
|
labels: z.ZodOptional<z.ZodRecord<z.ZodString, z.ZodString>>;
|
1528
1619
|
theme: z.ZodOptional<z.ZodRecord<z.ZodString, z.ZodUnknown>>;
|
1529
1620
|
}, z.ZodTypeAny, "passthrough"> | undefined;
|
@@ -2,7 +2,7 @@ import { z } from 'zod';
|
|
2
2
|
import type { PDFPage, PDFDocument } from '@pdfme/pdf-lib';
|
3
3
|
import type { ThemeConfig, GlobalToken } from 'antd';
|
4
4
|
import type { WidgetProps as _PropPanelWidgetProps, Schema as _PropPanelSchema } from 'form-render';
|
5
|
-
import { Lang, Dict, Size, Schema, Font, SchemaForUI, BasePdf, Template, GeneratorOptions, GenerateProps, UIOptions, UIProps, PreviewProps, DesignerProps } from './schema.js';
|
5
|
+
import { Lang, Dict, Mode, Size, Schema, Font, SchemaForUI, BasePdf, Template, GeneratorOptions, GenerateProps, UIOptions, UIProps, PreviewProps, DesignerProps } from './schema.js';
|
6
6
|
export type PropPanelSchema = _PropPanelSchema;
|
7
7
|
export type ChangeSchemas = (objs: {
|
8
8
|
key: string;
|
@@ -36,7 +36,7 @@ export interface PDFRenderProps<T extends Schema> {
|
|
36
36
|
*
|
37
37
|
* @template T - Type of the extended Schema object.
|
38
38
|
* @property {T} schema - Extended Schema object for rendering.
|
39
|
-
* @property {
|
39
|
+
* @property {Mode} mode - String indicating the rendering state. 'designer' is only used when the field is in edit mode in the Designer.
|
40
40
|
* @property {number} [tabIndex] - Tab index for Form.
|
41
41
|
* @property {string} [placeholder] - Placeholder text for Form.
|
42
42
|
* @property {() => void} [stopEditing] - Stops editing mode, can be used when the mode is 'designer'.
|
@@ -51,7 +51,7 @@ export interface PDFRenderProps<T extends Schema> {
|
|
51
51
|
*/
|
52
52
|
export type UIRenderProps<T extends Schema> = {
|
53
53
|
schema: T;
|
54
|
-
mode:
|
54
|
+
mode: Mode;
|
55
55
|
tabIndex?: number;
|
56
56
|
placeholder?: string;
|
57
57
|
stopEditing?: () => void;
|
@@ -115,8 +115,8 @@ export interface PropPanel<T extends Schema> {
|
|
115
115
|
export type Plugin<T extends Schema & {
|
116
116
|
[key: string]: any;
|
117
117
|
}> = {
|
118
|
-
pdf: (arg: PDFRenderProps<T>) => Promise<void
|
119
|
-
ui: (arg: UIRenderProps<T>) => Promise<void
|
118
|
+
pdf: (arg: PDFRenderProps<T>) => Promise<void> | void;
|
119
|
+
ui: (arg: UIRenderProps<T>) => Promise<void> | void;
|
120
120
|
propPanel: PropPanel<T>;
|
121
121
|
};
|
122
122
|
export type Plugins = {
|
@@ -124,6 +124,7 @@ export type Plugins = {
|
|
124
124
|
};
|
125
125
|
export type Lang = z.infer<typeof Lang>;
|
126
126
|
export type Dict = z.infer<typeof Dict>;
|
127
|
+
export type Mode = z.infer<typeof Mode>;
|
127
128
|
export type Size = z.infer<typeof Size>;
|
128
129
|
export type Schema = z.infer<typeof Schema>;
|
129
130
|
export type SchemaForUI = z.infer<typeof SchemaForUI>;
|
package/package.json
CHANGED
@@ -1,6 +1,6 @@
|
|
1
1
|
{
|
2
2
|
"name": "@pdfme/common",
|
3
|
-
"version": "3.
|
3
|
+
"version": "3.2.0",
|
4
4
|
"sideEffects": false,
|
5
5
|
"author": "hand-dot",
|
6
6
|
"license": "MIT",
|
@@ -32,7 +32,7 @@
|
|
32
32
|
}
|
33
33
|
},
|
34
34
|
"scripts": {
|
35
|
-
"
|
35
|
+
"dev": "tsc -p tsconfig.esm.json -w",
|
36
36
|
"build": "npm-run-all --parallel build:cjs build:esm",
|
37
37
|
"build:cjs": "tsc -p tsconfig.cjs.json",
|
38
38
|
"build:esm": "tsc -p tsconfig.esm.json",
|
@@ -43,7 +43,7 @@
|
|
43
43
|
"prettier": "prettier --write 'src/**/*.ts'"
|
44
44
|
},
|
45
45
|
"dependencies": {
|
46
|
-
"@pdfme/pdf-lib": "^1.
|
46
|
+
"@pdfme/pdf-lib": "^1.18.3",
|
47
47
|
"antd": "^5.11.2",
|
48
48
|
"buffer": "^6.0.3",
|
49
49
|
"form-render": "^2.2.20",
|
package/src/index.ts
CHANGED
@@ -12,6 +12,7 @@ import type {
|
|
12
12
|
PropPanelSchema,
|
13
13
|
PropPanelWidgetProps,
|
14
14
|
PDFRenderProps,
|
15
|
+
Mode,
|
15
16
|
UIRenderProps,
|
16
17
|
Plugin,
|
17
18
|
Lang,
|
@@ -96,5 +97,6 @@ export type {
|
|
96
97
|
PropPanelWidgetProps,
|
97
98
|
PDFRenderProps,
|
98
99
|
UIRenderProps,
|
100
|
+
Mode,
|
99
101
|
Plugin,
|
100
102
|
};
|
package/src/schema.ts
CHANGED
@@ -1,6 +1,6 @@
|
|
1
1
|
import { z } from 'zod';
|
2
2
|
|
3
|
-
const langs = ['en', 'ja', 'ar', 'th', 'pl', 'it'] as const;
|
3
|
+
const langs = ['en', 'ja', 'ar', 'th', 'pl', 'it', 'de'] as const;
|
4
4
|
|
5
5
|
export const Lang = z.enum(langs);
|
6
6
|
export const Dict = z.object({
|
@@ -26,7 +26,11 @@ export const Dict = z.object({
|
|
26
26
|
errorBulkUpdateFieldName: z.string(),
|
27
27
|
commitBulkUpdateFieldName: z.string(),
|
28
28
|
bulkUpdateFieldName: z.string(),
|
29
|
+
hexColorPrompt: z.string(),
|
29
30
|
// -----------------used in schemas-----------------
|
31
|
+
'schemas.color': z.string(),
|
32
|
+
'schemas.borderWidth': z.string(),
|
33
|
+
'schemas.borderColor': z.string(),
|
30
34
|
'schemas.textColor': z.string(),
|
31
35
|
'schemas.bgColor': z.string(),
|
32
36
|
'schemas.horizontal': z.string(),
|
@@ -51,12 +55,15 @@ export const Dict = z.object({
|
|
51
55
|
|
52
56
|
'schemas.barcodes.barColor': z.string(),
|
53
57
|
});
|
58
|
+
export const Mode = z.enum(['viewer', 'form', 'designer']);
|
54
59
|
|
55
60
|
export const Size = z.object({ height: z.number(), width: z.number() });
|
56
61
|
|
57
62
|
export const Schema = z
|
58
63
|
.object({
|
59
64
|
type: z.string(),
|
65
|
+
readOnly: z.boolean().optional(),
|
66
|
+
readOnlyValue: z.string().optional(),
|
60
67
|
position: z.object({ x: z.number(), y: z.number() }),
|
61
68
|
width: z.number(),
|
62
69
|
height: z.number(),
|
package/src/types.ts
CHANGED
@@ -2,8 +2,23 @@ import { z } from 'zod';
|
|
2
2
|
import type { PDFPage, PDFDocument } from '@pdfme/pdf-lib';
|
3
3
|
import type { ThemeConfig, GlobalToken } from 'antd';
|
4
4
|
import type { WidgetProps as _PropPanelWidgetProps, Schema as _PropPanelSchema } from 'form-render';
|
5
|
-
|
6
|
-
|
5
|
+
import {
|
6
|
+
Lang,
|
7
|
+
Dict,
|
8
|
+
Mode,
|
9
|
+
Size,
|
10
|
+
Schema,
|
11
|
+
Font,
|
12
|
+
SchemaForUI,
|
13
|
+
BasePdf,
|
14
|
+
Template,
|
15
|
+
GeneratorOptions,
|
16
|
+
GenerateProps,
|
17
|
+
UIOptions,
|
18
|
+
UIProps,
|
19
|
+
PreviewProps,
|
20
|
+
DesignerProps,
|
21
|
+
} from './schema.js';
|
7
22
|
|
8
23
|
export type PropPanelSchema = _PropPanelSchema;
|
9
24
|
export type ChangeSchemas = (objs: { key: string; value: any; schemaId: string }[]) => void;
|
@@ -37,7 +52,7 @@ export interface PDFRenderProps<T extends Schema> {
|
|
37
52
|
*
|
38
53
|
* @template T - Type of the extended Schema object.
|
39
54
|
* @property {T} schema - Extended Schema object for rendering.
|
40
|
-
* @property {
|
55
|
+
* @property {Mode} mode - String indicating the rendering state. 'designer' is only used when the field is in edit mode in the Designer.
|
41
56
|
* @property {number} [tabIndex] - Tab index for Form.
|
42
57
|
* @property {string} [placeholder] - Placeholder text for Form.
|
43
58
|
* @property {() => void} [stopEditing] - Stops editing mode, can be used when the mode is 'designer'.
|
@@ -52,7 +67,7 @@ export interface PDFRenderProps<T extends Schema> {
|
|
52
67
|
*/
|
53
68
|
export type UIRenderProps<T extends Schema> = {
|
54
69
|
schema: T;
|
55
|
-
mode:
|
70
|
+
mode: Mode;
|
56
71
|
tabIndex?: number;
|
57
72
|
placeholder?: string;
|
58
73
|
stopEditing?: () => void;
|
@@ -121,8 +136,8 @@ export interface PropPanel<T extends Schema> {
|
|
121
136
|
* @property {PropPanel} propPanel Object for defining the property panel.
|
122
137
|
*/
|
123
138
|
export type Plugin<T extends Schema & { [key: string]: any }> = {
|
124
|
-
pdf: (arg: PDFRenderProps<T>) => Promise<void
|
125
|
-
ui: (arg: UIRenderProps<T>) => Promise<void
|
139
|
+
pdf: (arg: PDFRenderProps<T>) => Promise<void> | void;
|
140
|
+
ui: (arg: UIRenderProps<T>) => Promise<void> | void;
|
126
141
|
propPanel: PropPanel<T>;
|
127
142
|
};
|
128
143
|
|
@@ -130,6 +145,7 @@ export type Plugins = { [key: string]: Plugin<any> | undefined };
|
|
130
145
|
|
131
146
|
export type Lang = z.infer<typeof Lang>;
|
132
147
|
export type Dict = z.infer<typeof Dict>;
|
148
|
+
export type Mode = z.infer<typeof Mode>;
|
133
149
|
export type Size = z.infer<typeof Size>;
|
134
150
|
export type Schema = z.infer<typeof Schema>;
|
135
151
|
export type SchemaForUI = z.infer<typeof SchemaForUI>;
|