taskflow-ar 0.1.0 → 0.1.1
Sign up to get free protection for your applications and to get access to all the features.
- checksums.yaml +4 -4
- data/README.md +137 -4
- data/images/flow-state.png +0 -0
- data/lib/taskflow.rb +9 -0
- data/lib/taskflow/version.rb +1 -1
- data/lib/taskflow/worker.rb +0 -1
- metadata +3 -2
checksums.yaml
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
---
|
2
2
|
SHA1:
|
3
|
-
metadata.gz:
|
4
|
-
data.tar.gz:
|
3
|
+
metadata.gz: 92e77f59dd6ec8be300d270e69189e2c68f727fa
|
4
|
+
data.tar.gz: d5913563fa164f2450f1bd680506dcadbd523c83
|
5
5
|
SHA512:
|
6
|
-
metadata.gz:
|
7
|
-
data.tar.gz:
|
6
|
+
metadata.gz: 0fb4942ce1e6cc098717a2bd3dacc6b7e436aa38fd73e1a6f100f3a8b4715714b51436a19056239d91d346e8c33836165b25568ae9580293bffb2bcdd3147b32
|
7
|
+
data.tar.gz: a28a7db985ef5ba68de88951bff811554b1e15d58805aea212d6036ea22e5e16502f3a0704ce4da59348219d6682dd5caa013f1cb845bc80f6e5d725eeb36452
|
data/README.md
CHANGED
@@ -1,8 +1,6 @@
|
|
1
1
|
# Taskflow
|
2
2
|
|
3
|
-
|
4
|
-
|
5
|
-
TODO: Delete this and the text above, and describe your gem
|
3
|
+
Taskflow is a cool rails plugin for creating and schedule task flows. NOTE: taskflow is based sidekiq, and use ActiveRecord/Mongoid as its database adapter, choose the right gem(another version taskflow is also available on my github) for your project.
|
6
4
|
|
7
5
|
## Installation
|
8
6
|
|
@@ -20,9 +18,17 @@ Or install it yourself as:
|
|
20
18
|
|
21
19
|
$ gem install taskflow-ar
|
22
20
|
|
21
|
+
Generate database migration file:
|
22
|
+
|
23
|
+
$ rails g taskflow migration
|
24
|
+
$ rake db:migrate
|
25
|
+
|
26
|
+
Tips: if you want use taskflow outside rails, you can just copy these migration files out and creat database table according to the files.
|
27
|
+
|
23
28
|
## Usage
|
24
29
|
|
25
|
-
example
|
30
|
+
Let's see an example first, the 'PlayFlow' has 7 task in total. When we start the flow, the t1(PendingTask) runs first, after t1 finishes, t2,t3,t4 would run in parallel; When t4 is done, the t5(OkTask) begins, when t2,t3,t5 are all done, the SummaryTask start to run, then run the last OkTask.
|
31
|
+
|
26
32
|
```ruby
|
27
33
|
class PlayFlow < Taskflow::Flow
|
28
34
|
NAME = "Play FLow"
|
@@ -147,6 +153,133 @@ After checking out the repo, run `bin/setup` to install dependencies. You can al
|
|
147
153
|
|
148
154
|
To install this gem onto your local machine, run `bundle exec rake install`. To release a new version, update the version number in `version.rb`, and then run `bundle exec rake release`, which will create a git tag for the version, push git commits and tags, and push the `.gem` file to [rubygems.org](https://rubygems.org).
|
149
155
|
|
156
|
+
## Documentations
|
157
|
+
### Taskflow state diagram
|
158
|
+
flow state
|
159
|
+
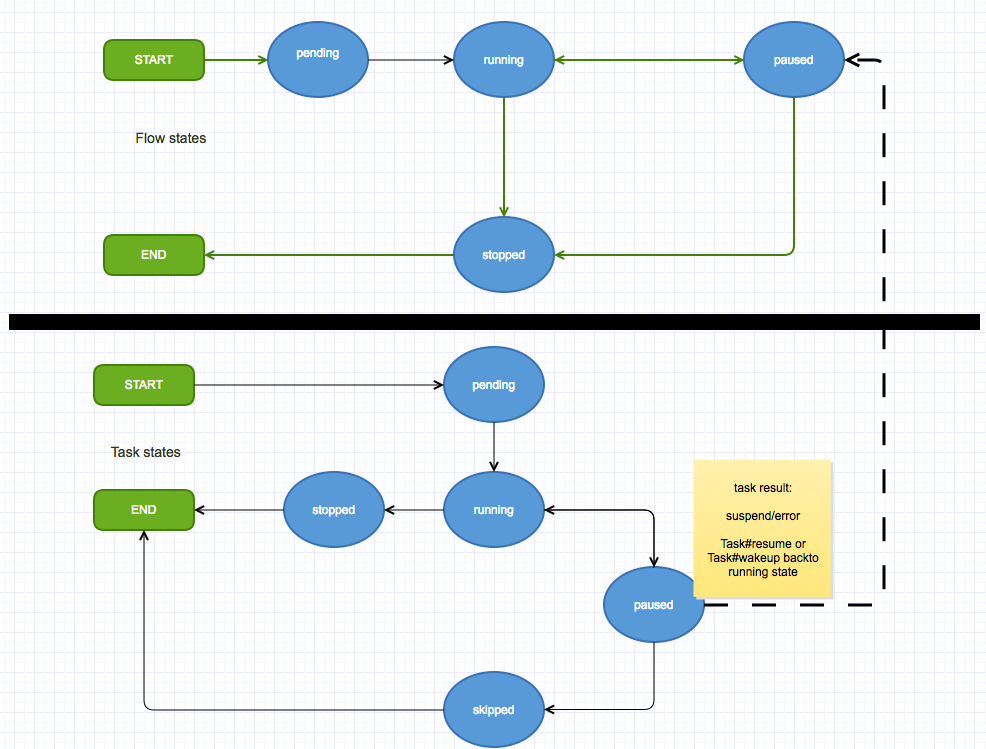
|
160
|
+
|
161
|
+
### the Taskflow::Flow
|
162
|
+
First, you should create your taskflow by inherit `Taskflow::Flow`, and you *must implement the `configure` method* to tell taskflow engine the detail info.
|
163
|
+
|
164
|
+
In `configure` method, you can use the keyword `run` to define task:
|
165
|
+
|
166
|
+
```ruby
|
167
|
+
# the keyword run
|
168
|
+
run Task_Class, name: 'task_name'
|
169
|
+
# task1 would run before another_task_obj
|
170
|
+
run Task_Class, name: 'task1',:before=>another_task_obj
|
171
|
+
# task2 would run after another_task_obj
|
172
|
+
run Task_Class, name: 'task2',:after=>another_task_obj
|
173
|
+
# the wait_task would run after task3,task4,task5 all done
|
174
|
+
run Task_Class, name: 'wait_task',:after=>[task3,task4,task5]
|
175
|
+
# pass some parameter to task, the params would set as task's input
|
176
|
+
run Task_Class, name: 'params_task',params: { :param1=>'abc' }
|
177
|
+
```
|
178
|
+
|
179
|
+
You can use `after` or `before` to specify the schedule order for certain task, if there's no after or before, the current task would just run after the previous task.
|
180
|
+
|
181
|
+
#### 0. config taskflow
|
182
|
+
You can config taskflow worker run in which sidekiq queue(default is `default`), forexample in `config/environments/production.rb`:
|
183
|
+
```ruby
|
184
|
+
Taskflow.configure do |config|
|
185
|
+
config.worker_options = { queue: 'workflow' }
|
186
|
+
end
|
187
|
+
```
|
188
|
+
|
189
|
+
#### 1. launch taskflow
|
190
|
+
```ruby
|
191
|
+
# the params would be set as taskflow's input field
|
192
|
+
Taskflow::Flow.launch 'PlayFlow',:params=>{word: 'hello'},:launched_by=>'Jason',:workflow_description=>'description'
|
193
|
+
# check whether can launch taskflow, if there's already a taskflow which has the some taskflow_klass and params, return false
|
194
|
+
Taskflow::Flow.can_launch? 'PlayFlow',:params=>{word: 'hello'},:launched_by=>'Jason',:workflow_description=>'description'
|
195
|
+
```
|
196
|
+
|
197
|
+
#### 2. taskflow control
|
198
|
+
```ruby
|
199
|
+
# Taskflow::Flow#stop! stop taskflow
|
200
|
+
flow.stop!
|
201
|
+
flow.stop! 'tom' # => stopped by tom
|
202
|
+
# Taskflow::Flow#resume, resume paused flow
|
203
|
+
flow.resume
|
204
|
+
```
|
205
|
+
|
206
|
+
### the Taskflow::Task
|
207
|
+
Define your own taskflow task. Inherit the class `Taskflow::Task`, and *implement go method*.
|
208
|
+
|
209
|
+
```ruby
|
210
|
+
def go(logger)
|
211
|
+
# write your task code here
|
212
|
+
end
|
213
|
+
```
|
214
|
+
|
215
|
+
#### 1.Sidekiq logger
|
216
|
+
the parameter `logger` of `Taskflow::Task#go` is sidekiq logger, so you can use it to log to sidekiq log file for debug.
|
217
|
+
|
218
|
+
#### 2.taskflow logger
|
219
|
+
There's another logger in `Taskflow::Task#go` : `tflogger`, `tflogger` can write log information to database. for example:
|
220
|
+
|
221
|
+
```ruby
|
222
|
+
def go(logger)
|
223
|
+
tflogger.info 'the info message would write to database'
|
224
|
+
tflogger.error 'this error message would write to database,too'
|
225
|
+
end
|
226
|
+
```
|
227
|
+
|
228
|
+
#### 3. input & output
|
229
|
+
There's a very cool feature. Every task has its own `input` and `ouput`.
|
230
|
+
```ruby
|
231
|
+
def go(logger)
|
232
|
+
puts input # => the input hash
|
233
|
+
puts input[:some_key] # => get the input value of the key 'some_key'
|
234
|
+
puts upstream.first.ouput # => get the first upstream's ouput, also is a hash
|
235
|
+
end
|
236
|
+
```
|
237
|
+
|
238
|
+
You have the `set/append_xxx` to modify the input and output.
|
239
|
+
```ruby
|
240
|
+
def go(log)
|
241
|
+
set_output :some_key=>'value' # => set the output to { :some_key=> 'value'}
|
242
|
+
append_output :some_key2=>'value2' # => add { :some_key2=>'value2' } to output
|
243
|
+
end
|
244
|
+
```
|
245
|
+
|
246
|
+
#### 4. data
|
247
|
+
Every task has its own data. After the task is done, the data would be cleanned.
|
248
|
+
```ruby
|
249
|
+
def go(log)
|
250
|
+
puts data # => print data
|
251
|
+
puts data[:key] # => access data
|
252
|
+
set_data :key=>'value'
|
253
|
+
append_data :key=>'value'
|
254
|
+
end
|
255
|
+
```
|
256
|
+
|
257
|
+
#### 5. relationship
|
258
|
+
In the task, you can access its upstream and downstream.
|
259
|
+
```ruby
|
260
|
+
def go(log)
|
261
|
+
upstream.each{|task| puts task.name }
|
262
|
+
upstream.each{|task| puts task.output }
|
263
|
+
puts downstream.first.name
|
264
|
+
end
|
265
|
+
```
|
266
|
+
|
267
|
+
#### 6. task control
|
268
|
+
```ruby
|
269
|
+
task.resume # => resume paused task
|
270
|
+
task.wakeup(hash_data) # => wakeup suspend task with some hash data
|
271
|
+
task.wakeup # => just wakeup
|
272
|
+
task.skip # => skip paused task
|
273
|
+
```
|
274
|
+
And, in `Taskflow::Task#go`, you can use keyword `suspend` to suspend current task, then the task result would convert to `suspend`,state would be `paused`.
|
275
|
+
```ruby
|
276
|
+
def go(log)
|
277
|
+
log.info 'before suspend'
|
278
|
+
suspend # => the task would be suspend right now.
|
279
|
+
log.info 'never print me'
|
280
|
+
end
|
281
|
+
```
|
282
|
+
|
150
283
|
## Contributing
|
151
284
|
|
152
285
|
Bug reports and pull requests are welcome on GitHub at https://github.com/[USERNAME]/taskflow. This project is intended to be a safe, welcoming space for collaboration, and contributors are expected to adhere to the [Contributor Covenant](contributor-covenant.org) code of conduct.
|
Binary file
|
data/lib/taskflow.rb
CHANGED
@@ -11,4 +11,13 @@ module Taskflow
|
|
11
11
|
def self.table_name_prefix
|
12
12
|
'taskflow_'
|
13
13
|
end
|
14
|
+
|
15
|
+
def self.worker_options=(opts)
|
16
|
+
orig = HashWithIndifferentAccess.new(Worker.sidekiq_options_hash || {})
|
17
|
+
Worker.sidekiq_options_hash = orig.merge(opts).merge(retry: false)
|
18
|
+
end
|
19
|
+
|
20
|
+
def self.configure
|
21
|
+
yield self
|
22
|
+
end
|
14
23
|
end
|
data/lib/taskflow/version.rb
CHANGED
data/lib/taskflow/worker.rb
CHANGED
metadata
CHANGED
@@ -1,14 +1,14 @@
|
|
1
1
|
--- !ruby/object:Gem::Specification
|
2
2
|
name: taskflow-ar
|
3
3
|
version: !ruby/object:Gem::Version
|
4
|
-
version: 0.1.
|
4
|
+
version: 0.1.1
|
5
5
|
platform: ruby
|
6
6
|
authors:
|
7
7
|
- qujianping
|
8
8
|
autorequire:
|
9
9
|
bindir: exe
|
10
10
|
cert_chain: []
|
11
|
-
date: 2015-07-
|
11
|
+
date: 2015-07-10 00:00:00.000000000 Z
|
12
12
|
dependencies:
|
13
13
|
- !ruby/object:Gem::Dependency
|
14
14
|
name: bundler
|
@@ -95,6 +95,7 @@ files:
|
|
95
95
|
- Rakefile
|
96
96
|
- bin/console
|
97
97
|
- bin/setup
|
98
|
+
- images/flow-state.png
|
98
99
|
- lib/generators/taskflow/taskflow_generator.rb
|
99
100
|
- lib/generators/templates/create_taskflow_flows.rb
|
100
101
|
- lib/generators/templates/create_taskflow_loggers.rb
|