sewing_kit 0.27.1 → 0.27.2
Sign up to get free protection for your applications and to get access to all the features.
- checksums.yaml +4 -4
- data/README.md +12 -1
- data/lib/sewing_kit/version.rb +1 -1
- data/lib/sewing_kit/webpack/manifest.rb +23 -0
- data/lib/sewing_kit/webpack/manifest/base.rb +3 -1
- metadata +2 -2
checksums.yaml
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
---
|
2
2
|
SHA1:
|
3
|
-
metadata.gz:
|
4
|
-
data.tar.gz:
|
3
|
+
metadata.gz: 82b56d2fde25a89c489676d3726d426d70de12bb
|
4
|
+
data.tar.gz: 45fb02c608830031960c3ae278f26048fe146412
|
5
5
|
SHA512:
|
6
|
-
metadata.gz:
|
7
|
-
data.tar.gz:
|
6
|
+
metadata.gz: 75023c4bf3810f14c5269b5e73e00cb73d2bf8262c71301ac54024408dae172333b2d71aec38bd70b43f5231fe076fb324add22206583b6a96b1cf53ccf0e99a
|
7
|
+
data.tar.gz: 5ff0c63902bae55d5ff14dbf59962d1bac7964ea5f2a163772a7b996779f7a0befa3b853fc502b018a479ca21118d6ae00e2e4523e412653222f546c8f3a88f9
|
data/README.md
CHANGED
@@ -82,8 +82,19 @@ A typical Polaris app will use React to render views and components. The follow
|
|
82
82
|
└── Home.js
|
83
83
|
```
|
84
84
|
|
85
|
+
## Transitioning from sprockets-commoner
|
86
|
+
It is currently not recommended to use `sprockets-commoner` and `sewing_kit` in the same project.
|
87
|
+
Minimally, it is required that the project does not have its own `babel-*` libraries that `sewing-kit` currently has as [dependencies](https://github.com/Shopify/sewing-kit/blob/master/package.json#L97~L102).
|
88
|
+
|
89
|
+
## Build Pipeline Gotchas
|
90
|
+
While sewing-kit is a dev dependency, it is needed for production builds. This means that the production build will break if the environment variable `YARN_INSTALL` is set to true, because it will prevent `devDependencies` from being installed.
|
91
|
+
|
92
|
+
To work around this in Buildkite, set `YARN_PRODUCTION=false` in the Environment Variables section of Pipeline Settings:
|
93
|
+
|
94
|
+
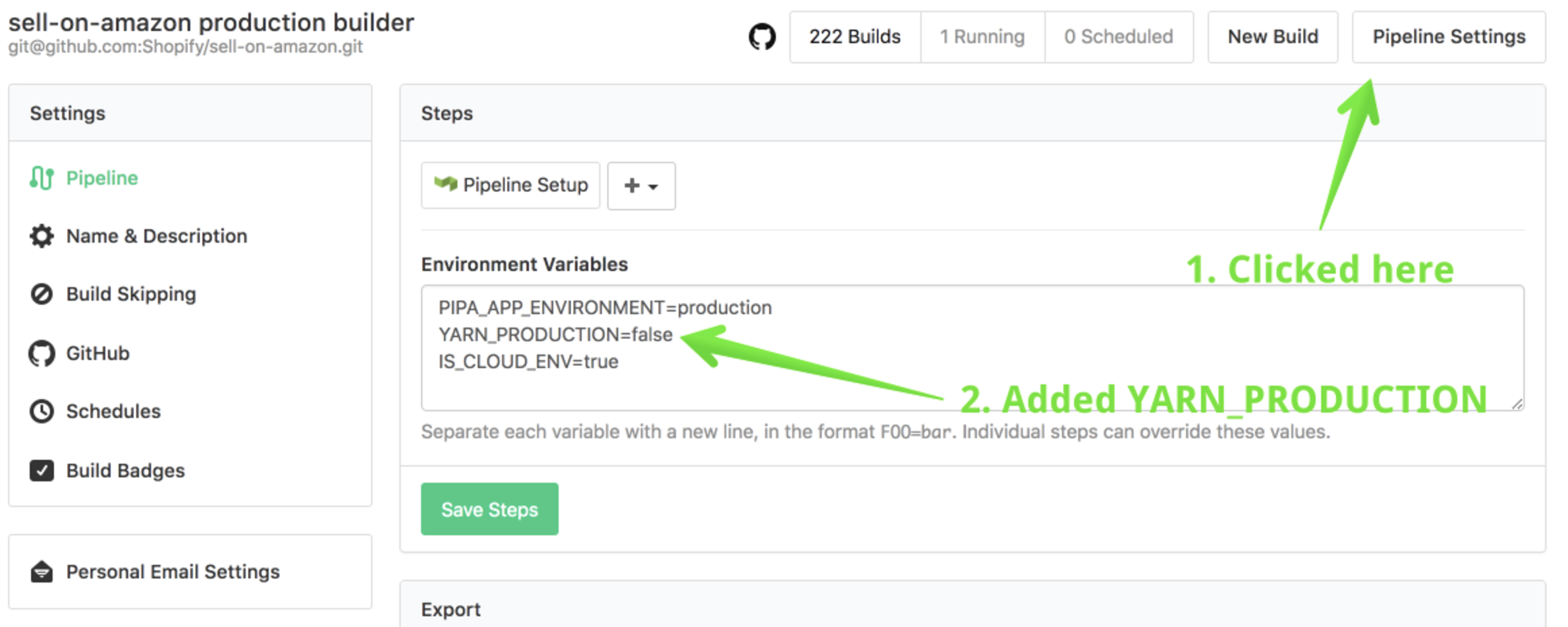
|
95
|
+
|
85
96
|
## React Boilerplate
|
86
|
-
* Create a React app in `app/ui/App.js` ([example](https://github.com/Shopify/rails_sewing_kit_example/blob/master/app/ui/App.
|
97
|
+
* Create a React app in `app/ui/App.js` ([example](https://github.com/Shopify/rails_sewing_kit_example/blob/master/app/ui/App.jsx))
|
87
98
|
* In an `erb` view, add a placeholder for React content ([example](https://github.com/Shopify/rails_sewing_kit_example/blob/master/app/views/home/index.html.erb#L4))
|
88
99
|
* In `index.js`, render a React component into the placeholder element ([example](https://github.com/Shopify/rails_sewing_kit_example/blob/master/app/ui/index.js))
|
89
100
|
* Use `sewing_kit_script_tag`/`sewing_kit_link_tag` helpers to link erb/js ([example](https://github.com/Shopify/rails_sewing_kit_example/blob/master/app/views/layouts/application.html.erb#L8))
|
data/lib/sewing_kit/version.rb
CHANGED
@@ -25,6 +25,29 @@ module SewingKit
|
|
25
25
|
end
|
26
26
|
end
|
27
27
|
|
28
|
+
class NodeSewingKitManifestMissing < StandardError
|
29
|
+
def initialize(mode, node_error_message)
|
30
|
+
env_message = if 'production' == mode
|
31
|
+
"\nIf this is a container build:\n" \
|
32
|
+
" - Verify that sewing_kit compilation succeeded before deployment\n" \
|
33
|
+
" - Verify that public/bundles/sewing-kit.manifest.json was uploaded\n" \
|
34
|
+
" - Verify that public/bundles/sewing-kit.manifest.json exists in the container\n" \
|
35
|
+
else
|
36
|
+
"\nPossible next steps:\n" \
|
37
|
+
" - If the server is still starting up, wait for a 'Compiled with latest changes' message" \
|
38
|
+
" then refresh your browser\n" \
|
39
|
+
" - Check the development console for compilation errors\n" \
|
40
|
+
" - Restart your development server\n"
|
41
|
+
end
|
42
|
+
|
43
|
+
super(
|
44
|
+
"Could not fetch the sewing-kit manifest 🙀 " \
|
45
|
+
"#{env_message}\n" \
|
46
|
+
"Original error #{node_error_message}"
|
47
|
+
)
|
48
|
+
end
|
49
|
+
end
|
50
|
+
|
28
51
|
# Raised if the node-generated manifest cannot be read from the filesystem.
|
29
52
|
class ManifestLoadError < StandardError
|
30
53
|
def initialize(path, cause)
|
@@ -22,11 +22,13 @@ module SewingKit
|
|
22
22
|
|
23
23
|
def load_metadata_from_node
|
24
24
|
begin
|
25
|
-
stdout,
|
25
|
+
stdout, stderr, status = Open3.capture3('node_modules/.bin/sewing-kit', 'manifest', "--mode=#{mode}")
|
26
26
|
rescue => e
|
27
27
|
raise NodeSewingKitNotRunnable.new(mode, e)
|
28
28
|
end
|
29
29
|
|
30
|
+
raise NodeSewingKitManifestMissing.new(mode, stderr) unless status.success?
|
31
|
+
|
30
32
|
begin
|
31
33
|
JSON.parse(stdout)
|
32
34
|
rescue => e
|
metadata
CHANGED
@@ -1,14 +1,14 @@
|
|
1
1
|
--- !ruby/object:Gem::Specification
|
2
2
|
name: sewing_kit
|
3
3
|
version: !ruby/object:Gem::Version
|
4
|
-
version: 0.27.
|
4
|
+
version: 0.27.2
|
5
5
|
platform: ruby
|
6
6
|
authors:
|
7
7
|
- Chris Sauve
|
8
8
|
autorequire:
|
9
9
|
bindir: bin
|
10
10
|
cert_chain: []
|
11
|
-
date: 2018-02-
|
11
|
+
date: 2018-02-21 00:00:00.000000000 Z
|
12
12
|
dependencies:
|
13
13
|
- !ruby/object:Gem::Dependency
|
14
14
|
name: railties
|