rspec-check-auth 0.5.0
This diff represents the content of publicly available package versions that have been released to one of the supported registries. The information contained in this diff is provided for informational purposes only and reflects changes between package versions as they appear in their respective public registries.
- data/.gitignore +5 -0
- data/CHANGELOG +1 -0
- data/LICENSE +9 -0
- data/README.md +74 -0
- data/Rakefile +50 -0
- data/VERSION +1 -0
- data/lib/rspec_check_auth/check_auth/output.rb +22 -0
- data/lib/rspec_check_auth/check_auth/request.rb +37 -0
- data/lib/rspec_check_auth/check_auth.rb +64 -0
- data/lib/rspec_check_auth/extend_hash.rb +9 -0
- data/lib/rspec_check_auth/extend_object.rb +11 -0
- data/lib/rspec_check_auth.rb +10 -0
- data/rspec-check-auth.gemspec +59 -0
- data/spec/rspec_check_auth_spec.rb +150 -0
- data/spec/spec_helper.rb +9 -0
- metadata +80 -0
data/CHANGELOG
ADDED
@@ -0,0 +1 @@
|
|
1
|
+
v0.5 - Public Release
|
data/LICENSE
ADDED
@@ -0,0 +1,9 @@
|
|
1
|
+
Copyright (c) 2009 Brightbox Systems Ltd
|
2
|
+
|
3
|
+
Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
|
4
|
+
|
5
|
+
The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.
|
6
|
+
|
7
|
+
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
8
|
+
|
9
|
+
See http://www.brightbox.co.uk/ for contact details.
|
data/README.md
ADDED
@@ -0,0 +1,74 @@
|
|
1
|
+
# RSpec Check-Auth
|
2
|
+
|
3
|
+
Check-Auth makes it easier to test your controller actions require authentication.
|
4
|
+
|
5
|
+
## Installation
|
6
|
+
|
7
|
+
gem install rspec-check-auth
|
8
|
+
|
9
|
+
To use check-auth you need to give it a block. This block returns the right bit of code (as a string to be eval'd) for that format. For instance, to check a HTML request it needs to be redirected, but for an XML request it should just be a 403 response.
|
10
|
+
|
11
|
+
Here's an example block that expects HTML to be redirected to login_url and XML/JSON requests to be given a 403 response:
|
12
|
+
|
13
|
+
CheckAuth.checking_block do |format|
|
14
|
+
case format
|
15
|
+
when :html
|
16
|
+
a = <<-EOF
|
17
|
+
response.should be_redirect
|
18
|
+
response.location.should == login_url
|
19
|
+
EOF
|
20
|
+
when :xml
|
21
|
+
"response.should be_unauthorised"
|
22
|
+
when :json
|
23
|
+
"response.should be_unauthorised"
|
24
|
+
else
|
25
|
+
%Q{raise "#{format} not defined in checking_block"}
|
26
|
+
end
|
27
|
+
end
|
28
|
+
|
29
|
+
## Usage
|
30
|
+
|
31
|
+
CheckAuth knows about different HTTP methods, and can figure out the usual crowd (POST create, DELETE destroy, etc.) You can override the method by passing a `:method` argument.
|
32
|
+
|
33
|
+
check_auth_for do |c|
|
34
|
+
c.index
|
35
|
+
c.index :method => :post
|
36
|
+
end
|
37
|
+
|
38
|
+
You can test your resourceful controller in one fell swoop too.
|
39
|
+
|
40
|
+
check_auth_for {|c| c.resource_actions }
|
41
|
+
|
42
|
+
Feel free to add any additional params for the resources, or any normal action.
|
43
|
+
|
44
|
+
check_auth_for do |c|
|
45
|
+
c.resource_actions :parent_id => "some_id"
|
46
|
+
c.parent_info :parent_id => "some_id"
|
47
|
+
end
|
48
|
+
|
49
|
+
By default it tests via HTMl and XML, but adding more or less formats is no issue. Just pass the `:format` arg.
|
50
|
+
|
51
|
+
check_auth_for do |c|
|
52
|
+
c.resource_actions :format => :html
|
53
|
+
c.parent_info :format => [:html, :json]
|
54
|
+
end
|
55
|
+
|
56
|
+
Only want some of the usual crowd? No problem, just pass the `:except` param and `resource_actions` will shun those specified.
|
57
|
+
|
58
|
+
check_auth_for {|c| c.resource_actions :except => [:new, :create, :edit, :update] }
|
59
|
+
|
60
|
+
And finally here's a proper example from a Brightbox controller spec.
|
61
|
+
|
62
|
+
check_auth_for do |c|
|
63
|
+
c.resource_actions
|
64
|
+
c.index :format => :json # above line does html/xml
|
65
|
+
c.find_available_items :parent_id => 0, :size => 0, :format => :xml
|
66
|
+
c.mailer :id => "some_id"
|
67
|
+
c.mailer :method => :post, :id => "some_id"
|
68
|
+
end
|
69
|
+
|
70
|
+
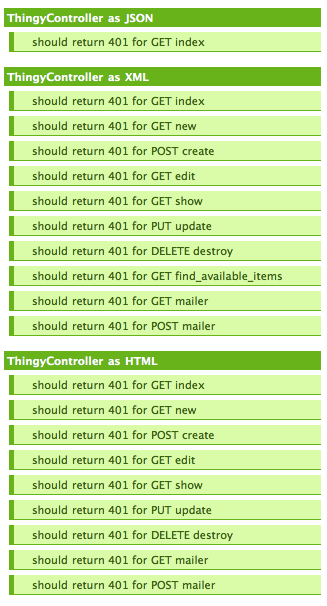
|
71
|
+
|
72
|
+
## Licence
|
73
|
+
|
74
|
+
See LICENCE
|
data/Rakefile
ADDED
@@ -0,0 +1,50 @@
|
|
1
|
+
require 'rubygems'
|
2
|
+
require 'rake'
|
3
|
+
|
4
|
+
begin
|
5
|
+
require 'jeweler'
|
6
|
+
Jeweler::Tasks.new do |gem|
|
7
|
+
gem.name = "rspec-check-auth"
|
8
|
+
gem.description = %Q{Quickly check your rails controller actions require authentication}
|
9
|
+
gem.summary = %Q{Quickly check your rails controller actions require authentication}
|
10
|
+
gem.email = "hello@brightbox.co.uk"
|
11
|
+
gem.homepage = "http://github.com/brightbox/rspec-check-auth"
|
12
|
+
gem.authors = ["Caius Durling"]
|
13
|
+
gem.add_development_dependency "rspec"
|
14
|
+
# gem is a Gem::Specification... see http://www.rubygems.org/read/chapter/20 for additional settings
|
15
|
+
end
|
16
|
+
rescue LoadError
|
17
|
+
puts "Jeweler (or a dependency) not available. Install it with: sudo gem install jeweler"
|
18
|
+
end
|
19
|
+
|
20
|
+
require 'spec/rake/spectask'
|
21
|
+
Spec::Rake::SpecTask.new(:spec) do |spec|
|
22
|
+
spec.libs << 'lib' << 'spec'
|
23
|
+
spec.spec_files = FileList['spec/**/*_spec.rb']
|
24
|
+
end
|
25
|
+
|
26
|
+
Spec::Rake::SpecTask.new(:rcov) do |spec|
|
27
|
+
spec.libs << 'lib' << 'spec'
|
28
|
+
spec.pattern = 'spec/**/*_spec.rb'
|
29
|
+
spec.rcov = true
|
30
|
+
end
|
31
|
+
|
32
|
+
task :spec => :check_dependencies
|
33
|
+
|
34
|
+
task :default => :spec
|
35
|
+
|
36
|
+
task :build => :gemspec
|
37
|
+
|
38
|
+
require 'rake/rdoctask'
|
39
|
+
Rake::RDocTask.new do |rdoc|
|
40
|
+
if File.exist?('VERSION')
|
41
|
+
version = File.read('VERSION')
|
42
|
+
else
|
43
|
+
version = ""
|
44
|
+
end
|
45
|
+
|
46
|
+
rdoc.rdoc_dir = 'rdoc'
|
47
|
+
rdoc.title = "rspec-check-auth #{version}"
|
48
|
+
rdoc.rdoc_files.include('README*')
|
49
|
+
rdoc.rdoc_files.include('lib/**/*.rb')
|
50
|
+
end
|
data/VERSION
ADDED
@@ -0,0 +1 @@
|
|
1
|
+
0.5.0
|
@@ -0,0 +1,22 @@
|
|
1
|
+
class CheckAuth
|
2
|
+
# Handles generating the code to eval from all the request objects
|
3
|
+
class Output
|
4
|
+
|
5
|
+
def self.generate_steps_for format, objects
|
6
|
+
# Wrap each format in a new describe block
|
7
|
+
o = [%Q{describe "as #{format.to_s.upcase}" do}]
|
8
|
+
# Run through each step
|
9
|
+
objects.each do |request|
|
10
|
+
o << <<-EOF
|
11
|
+
it "should return 401 for #{request.method.to_s.upcase} #{request.action}" do
|
12
|
+
#{request.method}(#{request.action.inspect}#{", " + request.params.inspect})
|
13
|
+
|
14
|
+
#{CheckAuth.checking_block(format)}
|
15
|
+
end
|
16
|
+
EOF
|
17
|
+
end
|
18
|
+
o << "end"
|
19
|
+
o.join("\n")
|
20
|
+
end
|
21
|
+
end
|
22
|
+
end
|
@@ -0,0 +1,37 @@
|
|
1
|
+
class CheckAuth
|
2
|
+
# Represents a single request spec
|
3
|
+
#
|
4
|
+
# Format and Method should be a single symbol
|
5
|
+
class Request
|
6
|
+
attr_reader :action, :method, :params, :format
|
7
|
+
|
8
|
+
def initialize action, format, params
|
9
|
+
@action = action
|
10
|
+
@format = format
|
11
|
+
@method = params[:method] || default_method
|
12
|
+
@params = params.except(:method)
|
13
|
+
end
|
14
|
+
|
15
|
+
# Adds the format into the params
|
16
|
+
# unless it's HTML
|
17
|
+
def params
|
18
|
+
return @params.merge(:format => @format.to_s) unless @format == :html
|
19
|
+
@params
|
20
|
+
end
|
21
|
+
|
22
|
+
# Works out the default method
|
23
|
+
# for certain controller actions
|
24
|
+
def default_method
|
25
|
+
case @action.to_s
|
26
|
+
when /create/
|
27
|
+
:post
|
28
|
+
when /update/
|
29
|
+
:put
|
30
|
+
when /destroy/
|
31
|
+
:delete
|
32
|
+
else# and when /(index|show|new|edit)/
|
33
|
+
:get
|
34
|
+
end
|
35
|
+
end
|
36
|
+
end
|
37
|
+
end
|
@@ -0,0 +1,64 @@
|
|
1
|
+
class CheckAuth
|
2
|
+
|
3
|
+
def initialize
|
4
|
+
@store = {}
|
5
|
+
end
|
6
|
+
|
7
|
+
# The main method
|
8
|
+
def self.for &block
|
9
|
+
instance = self.new
|
10
|
+
block.call(instance)
|
11
|
+
instance.output
|
12
|
+
end
|
13
|
+
|
14
|
+
# Handles checking
|
15
|
+
@@block = nil
|
16
|
+
def self.checking_block format=nil, &block
|
17
|
+
if block
|
18
|
+
@@block = block
|
19
|
+
else
|
20
|
+
raise "CheckAuth#checking_block needs defining" unless @@block
|
21
|
+
@@block.call(format)
|
22
|
+
end
|
23
|
+
end
|
24
|
+
|
25
|
+
def add action, params={}
|
26
|
+
params[:format] = [:html, :xml] unless params.has_key?(:format)
|
27
|
+
params[:format].arrayize.each do |format|
|
28
|
+
@store[format] ||= []
|
29
|
+
r = Request.new(action, format, params.except(:format))
|
30
|
+
@store[format] << r
|
31
|
+
end
|
32
|
+
end
|
33
|
+
|
34
|
+
#
|
35
|
+
# Tests all the standard methods a resources route has.
|
36
|
+
# Any params given (other than :except) are passed to each action as params,
|
37
|
+
# and actions that require an id (edit, show, update) get passed "some_id"
|
38
|
+
#
|
39
|
+
# Accepts :except => [:action, :names] for actions to be skipped in testing
|
40
|
+
#
|
41
|
+
def resource_actions params={}
|
42
|
+
skip = params.delete(:except).arrayize || {}
|
43
|
+
params[:format] = params.has_key?(:format) ? params[:format].arrayize : [:html,:xml]
|
44
|
+
# Add each action, unless they should be skipped
|
45
|
+
add :index, params unless skip.include?(:index)
|
46
|
+
add :new, params unless skip.include?(:new)
|
47
|
+
add :create, params unless skip.include?(:create)
|
48
|
+
add :edit, {:id => "some_id"}.merge(params) unless skip.include?(:edit)
|
49
|
+
add :show, {:id => "some_id"}.merge(params) unless skip.include?(:show)
|
50
|
+
add :update, {:id => "some_id"}.merge(params) unless skip.include?(:update)
|
51
|
+
add :destroy, {:id => "some_id"}.merge(params) unless skip.include?(:destroy)
|
52
|
+
end
|
53
|
+
|
54
|
+
def output
|
55
|
+
@store.map do |format, requests|
|
56
|
+
Output.generate_steps_for format, requests
|
57
|
+
end.join("\n\n")
|
58
|
+
end
|
59
|
+
|
60
|
+
def method_missing method, *args
|
61
|
+
add(method, *args)
|
62
|
+
end
|
63
|
+
|
64
|
+
end
|
@@ -0,0 +1,10 @@
|
|
1
|
+
require "rspec_check_auth/extend_hash"
|
2
|
+
require "rspec_check_auth/extend_object"
|
3
|
+
require "rspec_check_auth/check_auth"
|
4
|
+
require "rspec_check_auth/check_auth/request"
|
5
|
+
require "rspec_check_auth/check_auth/output"
|
6
|
+
|
7
|
+
def check_auth_for &block
|
8
|
+
return unless block
|
9
|
+
eval(CheckAuth.for &block)
|
10
|
+
end
|
@@ -0,0 +1,59 @@
|
|
1
|
+
# Generated by jeweler
|
2
|
+
# DO NOT EDIT THIS FILE DIRECTLY
|
3
|
+
# Instead, edit Jeweler::Tasks in Rakefile, and run the gemspec command
|
4
|
+
# -*- encoding: utf-8 -*-
|
5
|
+
|
6
|
+
Gem::Specification.new do |s|
|
7
|
+
s.name = %q{rspec-check-auth}
|
8
|
+
s.version = "0.5.0"
|
9
|
+
|
10
|
+
s.required_rubygems_version = Gem::Requirement.new(">= 0") if s.respond_to? :required_rubygems_version=
|
11
|
+
s.authors = ["Caius Durling"]
|
12
|
+
s.date = %q{2009-12-03}
|
13
|
+
s.description = %q{Quickly check your rails controller actions require authentication}
|
14
|
+
s.email = %q{hello@brightbox.co.uk}
|
15
|
+
s.extra_rdoc_files = [
|
16
|
+
"LICENSE",
|
17
|
+
"README.md"
|
18
|
+
]
|
19
|
+
s.files = [
|
20
|
+
".gitignore",
|
21
|
+
"CHANGELOG",
|
22
|
+
"LICENSE",
|
23
|
+
"README.md",
|
24
|
+
"Rakefile",
|
25
|
+
"VERSION",
|
26
|
+
"lib/rspec_check_auth.rb",
|
27
|
+
"lib/rspec_check_auth/check_auth.rb",
|
28
|
+
"lib/rspec_check_auth/check_auth/output.rb",
|
29
|
+
"lib/rspec_check_auth/check_auth/request.rb",
|
30
|
+
"lib/rspec_check_auth/extend_hash.rb",
|
31
|
+
"lib/rspec_check_auth/extend_object.rb",
|
32
|
+
"rspec-check-auth.gemspec",
|
33
|
+
"spec/rspec_check_auth_spec.rb",
|
34
|
+
"spec/spec_helper.rb"
|
35
|
+
]
|
36
|
+
s.homepage = %q{http://github.com/brightbox/rspec-check-auth}
|
37
|
+
s.rdoc_options = ["--charset=UTF-8"]
|
38
|
+
s.require_paths = ["lib"]
|
39
|
+
s.rubygems_version = %q{1.3.5}
|
40
|
+
s.summary = %q{Quickly check your rails controller actions require authentication}
|
41
|
+
s.test_files = [
|
42
|
+
"spec/rspec_check_auth_spec.rb",
|
43
|
+
"spec/spec_helper.rb"
|
44
|
+
]
|
45
|
+
|
46
|
+
if s.respond_to? :specification_version then
|
47
|
+
current_version = Gem::Specification::CURRENT_SPECIFICATION_VERSION
|
48
|
+
s.specification_version = 3
|
49
|
+
|
50
|
+
if Gem::Version.new(Gem::RubyGemsVersion) >= Gem::Version.new('1.2.0') then
|
51
|
+
s.add_development_dependency(%q<rspec>, [">= 0"])
|
52
|
+
else
|
53
|
+
s.add_dependency(%q<rspec>, [">= 0"])
|
54
|
+
end
|
55
|
+
else
|
56
|
+
s.add_dependency(%q<rspec>, [">= 0"])
|
57
|
+
end
|
58
|
+
end
|
59
|
+
|
@@ -0,0 +1,150 @@
|
|
1
|
+
require File.expand_path(File.dirname(__FILE__) + '/spec_helper')
|
2
|
+
|
3
|
+
describe "RspecCheckAuth" do
|
4
|
+
|
5
|
+
it "should require a checking block" do
|
6
|
+
lambda { CheckAuth.checking_block }.should raise_error("CheckAuth#checking_block needs defining")
|
7
|
+
end
|
8
|
+
|
9
|
+
it "should call the checking block when one is set" do
|
10
|
+
CheckAuth.checking_block do
|
11
|
+
raise "I'm in the block!"
|
12
|
+
end
|
13
|
+
|
14
|
+
lambda { CheckAuth.checking_block }.should raise_error("I'm in the block!")
|
15
|
+
end
|
16
|
+
|
17
|
+
it "should add an action with default formats" do
|
18
|
+
expect_request_for(:route, :html)
|
19
|
+
expect_request_for(:route, :xml)
|
20
|
+
|
21
|
+
expect_output_for(:html, [:"route_html"])
|
22
|
+
expect_output_for(:xml, [:"route_xml"])
|
23
|
+
|
24
|
+
CheckAuth.for do |c|
|
25
|
+
c.add :route
|
26
|
+
end
|
27
|
+
end
|
28
|
+
|
29
|
+
it "should add an action with custom params" do
|
30
|
+
expect_request_for :route, :html, :some => :fields
|
31
|
+
expect_request_for :route, :xml, :some => :fields
|
32
|
+
|
33
|
+
expect_output_for :html, [:route_html]
|
34
|
+
expect_output_for :xml, [:route_xml]
|
35
|
+
|
36
|
+
CheckAuth.for do |c|
|
37
|
+
c.add :route, :some => :fields
|
38
|
+
end
|
39
|
+
end
|
40
|
+
|
41
|
+
it "should add an action with custom formats" do
|
42
|
+
expect_request_for :route, :html
|
43
|
+
expect_request_for :route, :json
|
44
|
+
expect_request_for :route, :fart
|
45
|
+
|
46
|
+
expect_output_for :html, [:route_html]
|
47
|
+
expect_output_for :json, [:route_json]
|
48
|
+
expect_output_for :fart, [:route_fart]
|
49
|
+
|
50
|
+
CheckAuth.for do |c|
|
51
|
+
c.add :route, :format => [:html, :json, :fart]
|
52
|
+
end
|
53
|
+
end
|
54
|
+
|
55
|
+
it "should add an action with custom params and custom formats" do
|
56
|
+
expect_request_for :route, :html, :some => :fields
|
57
|
+
expect_request_for :route, :json, :some => :fields
|
58
|
+
expect_request_for :route, :fart, :some => :fields
|
59
|
+
|
60
|
+
expect_output_for :html, [:route_html]
|
61
|
+
expect_output_for :json, [:route_json]
|
62
|
+
expect_output_for :fart, [:route_fart]
|
63
|
+
|
64
|
+
CheckAuth.for do |c|
|
65
|
+
c.add :route, :format => [:html, :json, :fart], :some => :fields
|
66
|
+
end
|
67
|
+
end
|
68
|
+
|
69
|
+
it "should add standard resource routes in one line" do
|
70
|
+
expect_requests_for_resource_actions
|
71
|
+
|
72
|
+
expect_output_for(:html, [:index_html, :new_html, :create_html, :edit_html, :show_html, :update_html, :destroy_html])
|
73
|
+
expect_output_for(:xml, [:index_xml, :new_xml, :create_xml, :edit_xml, :show_xml, :update_xml, :destroy_xml])
|
74
|
+
|
75
|
+
CheckAuth.for do |c|
|
76
|
+
c.resource_actions
|
77
|
+
end
|
78
|
+
end
|
79
|
+
|
80
|
+
it "should ignore resources it's been told to" do
|
81
|
+
expect_request_for :index, :html
|
82
|
+
expect_request_for :index, :xml
|
83
|
+
|
84
|
+
expect_output_for(:html, [:index_html])
|
85
|
+
expect_output_for(:xml, [:index_xml])
|
86
|
+
|
87
|
+
CheckAuth.for do |c|
|
88
|
+
c.resource_actions :except => [:new, :show, :edit, :update, :create, :destroy]
|
89
|
+
end
|
90
|
+
end
|
91
|
+
|
92
|
+
it "should add standard resource routes with extra params" do
|
93
|
+
expect_requests_for_resource_actions :some => :fields
|
94
|
+
|
95
|
+
expect_output_for(:html, [:index_html, :new_html, :create_html, :edit_html, :show_html, :update_html, :destroy_html])
|
96
|
+
expect_output_for(:xml, [:index_xml, :new_xml, :create_xml, :edit_xml, :show_xml, :update_xml, :destroy_xml])
|
97
|
+
|
98
|
+
CheckAuth.for do |c|
|
99
|
+
c.resource_actions :some => :fields
|
100
|
+
end
|
101
|
+
end
|
102
|
+
|
103
|
+
it "should add standard resource routes with extra formats" do
|
104
|
+
expect_requests_for_resource_actions({}, [:html, :json, :fart])
|
105
|
+
|
106
|
+
expect_output_for(:html, [:index_html, :new_html, :create_html, :edit_html, :show_html, :update_html, :destroy_html])
|
107
|
+
expect_output_for(:json, [:index_json, :new_json, :create_json, :edit_json, :show_json, :update_json, :destroy_json])
|
108
|
+
expect_output_for(:fart, [:index_fart, :new_fart, :create_fart, :edit_fart, :show_fart, :update_fart, :destroy_fart])
|
109
|
+
|
110
|
+
CheckAuth.for do |c|
|
111
|
+
c.resource_actions :format => [:html, :json, :fart]
|
112
|
+
end
|
113
|
+
end
|
114
|
+
|
115
|
+
it "should add standard resource routes with extra params and extra formats" do
|
116
|
+
expect_requests_for_resource_actions({:some => :fields}, [:html, :json, :fart])
|
117
|
+
|
118
|
+
expect_output_for(:html, [:index_html, :new_html, :create_html, :edit_html, :show_html, :update_html, :destroy_html])
|
119
|
+
expect_output_for(:json, [:index_json, :new_json, :create_json, :edit_json, :show_json, :update_json, :destroy_json])
|
120
|
+
expect_output_for(:fart, [:index_fart, :new_fart, :create_fart, :edit_fart, :show_fart, :update_fart, :destroy_fart])
|
121
|
+
|
122
|
+
CheckAuth.for do |c|
|
123
|
+
c.resource_actions :format => [:html, :json, :fart], :some => :fields
|
124
|
+
end
|
125
|
+
end
|
126
|
+
|
127
|
+
|
128
|
+
|
129
|
+
private
|
130
|
+
|
131
|
+
def expect_requests_for_resource_actions options={}, formats=[:html, :xml]
|
132
|
+
formats.each do |f|
|
133
|
+
expect_request_for(:index, f, options)
|
134
|
+
expect_request_for(:new, f, options)
|
135
|
+
expect_request_for(:create, f, options)
|
136
|
+
expect_request_for(:edit, f, options.merge(:id => "some_id"))
|
137
|
+
expect_request_for(:show, f, options.merge(:id => "some_id"))
|
138
|
+
expect_request_for(:update, f, options.merge(:id => "some_id"))
|
139
|
+
expect_request_for(:destroy, f, options.merge(:id => "some_id"))
|
140
|
+
end
|
141
|
+
end
|
142
|
+
|
143
|
+
def expect_request_for action, format, options={}
|
144
|
+
CheckAuth::Request.should_receive(:new).with(action, format, options).and_return(:"#{action}_#{format}")
|
145
|
+
end
|
146
|
+
|
147
|
+
def expect_output_for format, actions=[]
|
148
|
+
CheckAuth::Output.should_receive(:generate_steps_for).with(format, actions)
|
149
|
+
end
|
150
|
+
end
|
data/spec/spec_helper.rb
ADDED
metadata
ADDED
@@ -0,0 +1,80 @@
|
|
1
|
+
--- !ruby/object:Gem::Specification
|
2
|
+
name: rspec-check-auth
|
3
|
+
version: !ruby/object:Gem::Version
|
4
|
+
version: 0.5.0
|
5
|
+
platform: ruby
|
6
|
+
authors:
|
7
|
+
- Caius Durling
|
8
|
+
autorequire:
|
9
|
+
bindir: bin
|
10
|
+
cert_chain: []
|
11
|
+
|
12
|
+
date: 2009-12-03 00:00:00 +00:00
|
13
|
+
default_executable:
|
14
|
+
dependencies:
|
15
|
+
- !ruby/object:Gem::Dependency
|
16
|
+
name: rspec
|
17
|
+
type: :development
|
18
|
+
version_requirement:
|
19
|
+
version_requirements: !ruby/object:Gem::Requirement
|
20
|
+
requirements:
|
21
|
+
- - ">="
|
22
|
+
- !ruby/object:Gem::Version
|
23
|
+
version: "0"
|
24
|
+
version:
|
25
|
+
description: Quickly check your rails controller actions require authentication
|
26
|
+
email: hello@brightbox.co.uk
|
27
|
+
executables: []
|
28
|
+
|
29
|
+
extensions: []
|
30
|
+
|
31
|
+
extra_rdoc_files:
|
32
|
+
- LICENSE
|
33
|
+
- README.md
|
34
|
+
files:
|
35
|
+
- .gitignore
|
36
|
+
- CHANGELOG
|
37
|
+
- LICENSE
|
38
|
+
- README.md
|
39
|
+
- Rakefile
|
40
|
+
- VERSION
|
41
|
+
- lib/rspec_check_auth.rb
|
42
|
+
- lib/rspec_check_auth/check_auth.rb
|
43
|
+
- lib/rspec_check_auth/check_auth/output.rb
|
44
|
+
- lib/rspec_check_auth/check_auth/request.rb
|
45
|
+
- lib/rspec_check_auth/extend_hash.rb
|
46
|
+
- lib/rspec_check_auth/extend_object.rb
|
47
|
+
- rspec-check-auth.gemspec
|
48
|
+
- spec/rspec_check_auth_spec.rb
|
49
|
+
- spec/spec_helper.rb
|
50
|
+
has_rdoc: true
|
51
|
+
homepage: http://github.com/brightbox/rspec-check-auth
|
52
|
+
licenses: []
|
53
|
+
|
54
|
+
post_install_message:
|
55
|
+
rdoc_options:
|
56
|
+
- --charset=UTF-8
|
57
|
+
require_paths:
|
58
|
+
- lib
|
59
|
+
required_ruby_version: !ruby/object:Gem::Requirement
|
60
|
+
requirements:
|
61
|
+
- - ">="
|
62
|
+
- !ruby/object:Gem::Version
|
63
|
+
version: "0"
|
64
|
+
version:
|
65
|
+
required_rubygems_version: !ruby/object:Gem::Requirement
|
66
|
+
requirements:
|
67
|
+
- - ">="
|
68
|
+
- !ruby/object:Gem::Version
|
69
|
+
version: "0"
|
70
|
+
version:
|
71
|
+
requirements: []
|
72
|
+
|
73
|
+
rubyforge_project:
|
74
|
+
rubygems_version: 1.3.5
|
75
|
+
signing_key:
|
76
|
+
specification_version: 3
|
77
|
+
summary: Quickly check your rails controller actions require authentication
|
78
|
+
test_files:
|
79
|
+
- spec/rspec_check_auth_spec.rb
|
80
|
+
- spec/spec_helper.rb
|