rails_ajax_formhandler 0.1.1 → 0.1.2
This diff represents the content of publicly available package versions that have been released to one of the supported registries. The information contained in this diff is provided for informational purposes only and reflects changes between package versions as they appear in their respective public registries.
- checksums.yaml +4 -4
- data/README.md +64 -22
- data/input_datepicker.png +0 -0
- data/lib/rails_ajax_formhandler/version.rb +1 -1
- data/vendor/assets/javascripts/ajax_formhandler.js +103 -1
- metadata +2 -1
checksums.yaml
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
---
|
2
2
|
SHA1:
|
3
|
-
metadata.gz:
|
4
|
-
data.tar.gz:
|
3
|
+
metadata.gz: 4980edcc055fa4f4483d16075696fdf4b67ada19
|
4
|
+
data.tar.gz: d11f00cf24ad09b51206f430b381d5d4cee5983f
|
5
5
|
SHA512:
|
6
|
-
metadata.gz:
|
7
|
-
data.tar.gz:
|
6
|
+
metadata.gz: 0b65a51e35d16001838f5f6d241126a96f93e66f1a04a6a1f379d0ee2641e125b82835aba892da354cc3647d01a7362ad0c83c3e27a2693add6da928a9f75739
|
7
|
+
data.tar.gz: 7648402f5e8429e1834da25ac3c39e31f56de7fcb3ddce6cd2600f908afba1ff40eca87574555007176aa2c3eacc0390865c8b100b8f4422bc6be8d92331ff86
|
data/README.md
CHANGED
@@ -52,6 +52,9 @@ $(document).ready(function{
|
|
52
52
|
});
|
53
53
|
|
54
54
|
```
|
55
|
+
I'll automatically detects your form and set an event-listener, so the form will be submitted with ajax.
|
56
|
+
|
57
|
+
__Please note that in order to work, use the "form_for"-helper in your view as usual!__
|
55
58
|
|
56
59
|
## Global Options
|
57
60
|
|
@@ -60,8 +63,8 @@ $(document).ready(function{
|
|
60
63
|
|
61
64
|
## HTML
|
62
65
|
|
63
|
-
html
|
64
|
-
an object
|
66
|
+
### html
|
67
|
+
accepts an object with the keys shown below
|
65
68
|
|
66
69
|
```javascript
|
67
70
|
{
|
@@ -83,23 +86,27 @@ or, if you're using Bootstrap Version 3
|
|
83
86
|
}
|
84
87
|
```
|
85
88
|
|
89
|
+
|
86
90
|
## Callbacks
|
87
91
|
|
88
|
-
|
89
|
-
|
92
|
+
all callbacks accepting only a function
|
93
|
+
|
94
|
+
### success
|
95
|
+
|
96
|
+
jqXHR as first, the form-object as second and the event as third argument
|
90
97
|
|
91
98
|
|
92
99
|
```javascript
|
93
100
|
{ ...
|
94
101
|
success: function(xhr, form, event) {
|
95
|
-
// what happens after
|
102
|
+
// what happens after success
|
96
103
|
}
|
97
104
|
}
|
98
105
|
```
|
99
106
|
|
100
107
|
|
101
|
-
error
|
102
|
-
|
108
|
+
### error
|
109
|
+
jqXHR as first, the errorThrown(string) as second, the form-object as third and the event as forth argument
|
103
110
|
|
104
111
|
|
105
112
|
```javascript
|
@@ -110,8 +117,8 @@ function with jqXHR as first, the errorThrown(string) as second, the form-object
|
|
110
117
|
}
|
111
118
|
```
|
112
119
|
|
113
|
-
send
|
114
|
-
|
120
|
+
### send
|
121
|
+
event as only argument, will be called on submit
|
115
122
|
|
116
123
|
|
117
124
|
```javascript
|
@@ -122,7 +129,7 @@ function with the event only argument, will be called on submit
|
|
122
129
|
}
|
123
130
|
```
|
124
131
|
|
125
|
-
Example
|
132
|
+
### *Example:*
|
126
133
|
|
127
134
|
|
128
135
|
```javascript
|
@@ -132,7 +139,8 @@ Example:
|
|
132
139
|
helpBlockClass: "my-help-block"
|
133
140
|
},
|
134
141
|
success: function(xhr, form, event) {
|
135
|
-
$("#
|
142
|
+
$("#success-message").html("Form successfully submitted");
|
143
|
+
form[0].reset();
|
136
144
|
}
|
137
145
|
}
|
138
146
|
```
|
@@ -147,7 +155,7 @@ def create
|
|
147
155
|
if @client.save
|
148
156
|
f.json { render :show, status: :created}
|
149
157
|
else
|
150
|
-
|
158
|
+
f.json { render json: @client.errors, status: :unprocessable_entity }
|
151
159
|
end
|
152
160
|
end
|
153
161
|
|
@@ -156,13 +164,26 @@ def update
|
|
156
164
|
if @client.update(client_params)
|
157
165
|
f.json { render :show, status: :ok}
|
158
166
|
else
|
159
|
-
|
160
|
-
end
|
161
|
-
|
167
|
+
f.json { render json: @client.errors, status: :unprocessable_entity }
|
168
|
+
end
|
162
169
|
end
|
163
170
|
|
164
171
|
```
|
165
172
|
|
173
|
+
_Note_ that the json response in case of error works only if the json-string contains only the errors-object, or, if namespacing is required, the object name as key and the errors as values.
|
174
|
+
|
175
|
+
```ruby
|
176
|
+
f.json { render _json: @client.errors_, status: :unprocessable_entity }
|
177
|
+
|
178
|
+
```
|
179
|
+
or
|
180
|
+
|
181
|
+
```ruby
|
182
|
+
f.json { render _json: { client: @client.errors, data: "some other stuff"}_, status: :unprocessable_entity }
|
183
|
+
|
184
|
+
```
|
185
|
+
That gives you the possibility, passing some other informations alongside the errors-object, if required.
|
186
|
+
|
166
187
|
## Model
|
167
188
|
|
168
189
|
Insert your validations in the model and pass your error-messages like:
|
@@ -173,17 +194,13 @@ validates :firstname, presence: { message: "We need your firstname!"}
|
|
173
194
|
|
174
195
|
```
|
175
196
|
|
176
|
-
If you're not familiar with, please refer to [guides.rubyonrails](http://guides.rubyonrails.org/active_record_validations.html).
|
197
|
+
If you're not familiar with, please refer to [http://guides.rubyonrails.org](http://guides.rubyonrails.org/active_record_validations.html).
|
177
198
|
|
178
199
|
|
179
200
|
## Multiple Forms
|
180
201
|
|
181
|
-
<<<<<<< HEAD
|
182
202
|
If you have two or more forms on one page, FormHandler detects them all and sets them up. The passed arguments will be applied to each form. For specific configuration, call the ".config_form()"
|
183
|
-
|
184
|
-
If you have two or more forms on one page, FormHandler detects them all and sets them up. The passed arguments will be applied to each for. For specific configuration, call the ".config_form()"
|
185
|
-
>>>>>>> 9d6f3ee23dfee4cef6f8454363e58dd9d7624de3
|
186
|
-
method:
|
203
|
+
method:
|
187
204
|
|
188
205
|
```javascript
|
189
206
|
|
@@ -198,8 +215,33 @@ If you have two or more forms on one page, FormHandler detects them all and sets
|
|
198
215
|
})
|
199
216
|
|
200
217
|
form_handler.init();
|
201
|
-
|
202
218
|
```
|
219
|
+
## Hidden fields
|
220
|
+
|
221
|
+
In some cases the input-field shown in the form doesn't store the actual value, but updates a hidden_field, which will be submitted.For example, a datepicker:
|
222
|
+
|
223
|
+
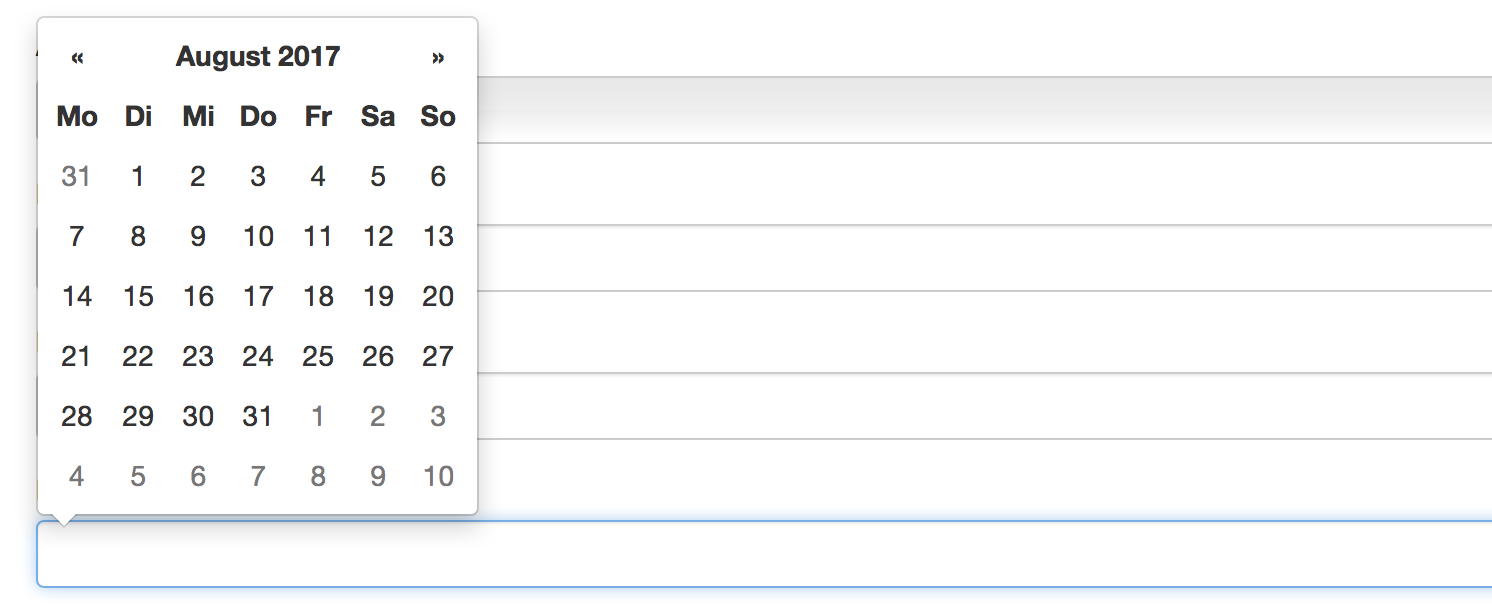
|
224
|
+
|
225
|
+
would look like
|
226
|
+
|
227
|
+
|
228
|
+
```ruby
|
229
|
+
...
|
230
|
+
<div class="field">
|
231
|
+
<input type="text" class="datepicker" id="datepicker-input">
|
232
|
+
|
233
|
+
<%= f.hidden_field :date_of_birth %>
|
234
|
+
</div>
|
235
|
+
```
|
236
|
+
|
237
|
+
In this case please make sure that the hidden-input appears _after_ the 'dummy-field', like in the example above in order to render validation errors properly!
|
238
|
+
|
239
|
+
|
240
|
+
## Support
|
241
|
+
|
242
|
+
I hope this usefull for you as it is for me. If there are any issues, pleas contact me [info@yield-in.de](info@yield-in.de)
|
243
|
+
|
244
|
+
|
203
245
|
## Development
|
204
246
|
|
205
247
|
After checking out the repo, run `bin/setup` to install dependencies. You can also run `bin/console` for an interactive prompt that will allow you to experiment.
|
Binary file
|
@@ -3,6 +3,7 @@
|
|
3
3
|
/*
|
4
4
|
Form-Handler by Davide Brancaccio
|
5
5
|
|
6
|
+
|
6
7
|
FormHandler submits your form automatically via ajax and handles validation-rendering also by adding error-styles and messages.
|
7
8
|
This works without any additional setup with standard-rails scaffold forms and controllers, just by adding validations to the model.
|
8
9
|
|
@@ -28,6 +29,18 @@ Example scaffold 'Client':
|
|
28
29
|
|
29
30
|
...
|
30
31
|
|
32
|
+
show.json.jbuilder
|
33
|
+
|
34
|
+
...
|
35
|
+
json.extract! @client, :id,:firstname,:lastname,...
|
36
|
+
|
37
|
+
<or if namespacing is required>
|
38
|
+
|
39
|
+
json.set! :client do |client|
|
40
|
+
client.extract! @client, :id,:firstname,:lastname,...
|
41
|
+
end
|
42
|
+
....
|
43
|
+
|
31
44
|
_form.html.erb
|
32
45
|
|
33
46
|
...
|
@@ -86,7 +99,97 @@ Example scaffold 'Client':
|
|
86
99
|
|
87
100
|
}
|
88
101
|
|
102
|
+
as example:
|
103
|
+
----------
|
104
|
+
var form_handler = new FormHandler({
|
105
|
+
html: {
|
106
|
+
errorClass: "my-error-class",
|
107
|
+
helpBlockClass: "error-feedback"
|
108
|
+
},
|
109
|
+
success: function(xhr, form, event) {
|
110
|
+
var response = xhr.responseJSON;
|
111
|
+
$(form)....
|
112
|
+
}
|
113
|
+
})
|
114
|
+
|
115
|
+
form_handler.init();
|
116
|
+
|
117
|
+
--------------------------------------------------------------------------------------------------------------
|
118
|
+
|
119
|
+
Multiple Form Support
|
120
|
+
----------------------
|
121
|
+
|
122
|
+
If you use more than one form on one page, FormHandler detects it automatically and applies given options to all forms.
|
123
|
+
So your settings become global. To specify the options for every single form just use the 'config_form()' method and provide
|
124
|
+
the form-name, for eexample "client" and the specified options like
|
125
|
+
|
126
|
+
form_handler.config_form('client', {
|
127
|
+
success: function(xhr, form, event) {
|
128
|
+
...do anything else
|
129
|
+
}
|
130
|
+
})
|
131
|
+
|
132
|
+
if, for some reasons, you don't want to FormHandler to take care of one or some forms, just pass ' ignore: true':
|
133
|
+
|
134
|
+
form_handler.config_form('client', {
|
135
|
+
ignore: true
|
136
|
+
}
|
137
|
+
});
|
138
|
+
|
139
|
+
|
140
|
+
Redirections
|
141
|
+
-------------
|
142
|
+
|
143
|
+
In some cases, the shown input field is not actually the field meant to be submitted, instead the value is stored in an hidden input.
|
144
|
+
For example when you use datepicker or typeahead.
|
145
|
+
|
146
|
+
Because FormHandler takes advantage of rails-naming-convention, it is looking for the hidden input-field.
|
147
|
+
To work properly, the hidden-input musst be of course inside a wrapper and must appear after the 'dummy-input'.
|
148
|
+
|
149
|
+
But if you try to edit the record, the existing value doesn't show up either, if you haven't done any workarounds.
|
150
|
+
|
151
|
+
|
152
|
+
To make sure that the validation-rendering works reliable and the value is shown also on your edit-form, use the
|
153
|
+
'apply_validation_to()' method.
|
154
|
+
|
155
|
+
Example
|
156
|
+
-------
|
157
|
+
|
158
|
+
_form.html.erb
|
159
|
+
....
|
160
|
+
<input type="text" id="date_show" class="any-datepicker">
|
161
|
+
<%= f.hidden_field :date_of_birth %>
|
162
|
+
....
|
163
|
+
|
164
|
+
client.js
|
165
|
+
var form_handler = new FormHandler({
|
166
|
+
html: {
|
167
|
+
errorClass: "my-error-class",
|
168
|
+
helpBlockClass: "error-feedback"
|
169
|
+
},
|
170
|
+
success: function(xhr, form, event) {
|
171
|
+
var response = xhr.responseJSON;
|
172
|
+
$(form)....
|
173
|
+
}
|
174
|
+
});
|
175
|
+
|
176
|
+
form_handler.apply_validation_to("client",{
|
177
|
+
"client_date_of_birth": "date_show"
|
178
|
+
})
|
179
|
+
|
180
|
+
form_handler.init();
|
181
|
+
|
182
|
+
or even easier with data-attribute:
|
183
|
+
|
184
|
+
|
185
|
+
_form.html.erb
|
186
|
+
....
|
187
|
+
<input type="text" id="date_show" class="any-datepicker">
|
188
|
+
<%= f.hidden_field :date_of_birth, data: {validation: "date_show"} %>
|
189
|
+
....
|
190
|
+
|
89
191
|
|
192
|
+
PLEASE NOTE THAT VALIDATION-RENDERING NOT WORKING WITH NESTED ATTRIBUTES, AT LEAST NOT WITHOUT ANY WORKAROUNDS!
|
90
193
|
|
91
194
|
*/
|
92
195
|
|
@@ -373,7 +476,6 @@ FormObject.prototype.get_ajax_settings = function(event,values) {
|
|
373
476
|
var field = $("#" + value);
|
374
477
|
form_object.validation_renderer.remove_error(field);
|
375
478
|
})
|
376
|
-
this.form[0].reset();
|
377
479
|
};
|
378
480
|
|
379
481
|
|
metadata
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
--- !ruby/object:Gem::Specification
|
2
2
|
name: rails_ajax_formhandler
|
3
3
|
version: !ruby/object:Gem::Version
|
4
|
-
version: 0.1.
|
4
|
+
version: 0.1.2
|
5
5
|
platform: ruby
|
6
6
|
authors:
|
7
7
|
- Davide Brancaccio
|
@@ -84,6 +84,7 @@ files:
|
|
84
84
|
- Rakefile
|
85
85
|
- bin/console
|
86
86
|
- bin/setup
|
87
|
+
- input_datepicker.png
|
87
88
|
- input_validation_example1.png
|
88
89
|
- input_validation_example2.png
|
89
90
|
- lib/.DS_Store
|