pully 0.0.3 → 0.1.0
Sign up to get free protection for your applications and to get access to all the features.
- checksums.yaml +4 -4
- data/README.md +26 -3
- data/lib/pully/version.rb +1 -1
- data/lib/pully.rb +42 -1
- data/spec/lib_spec.rb +124 -0
- data/spec/lib_test_spec.rb +13 -0
- metadata +1 -1
checksums.yaml
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
---
|
2
2
|
SHA1:
|
3
|
-
metadata.gz:
|
4
|
-
data.tar.gz:
|
3
|
+
metadata.gz: bfac1f15dc32ea73cea429df9e08e5f6b1ce2603
|
4
|
+
data.tar.gz: a02b5a8a9b17e3cf0f2a72f897cf921711cfcb89
|
5
5
|
SHA512:
|
6
|
-
metadata.gz:
|
7
|
-
data.tar.gz:
|
6
|
+
metadata.gz: 60a3d82905a951e4a1e456340655c6a8863269a8aeaefaa84ed47b68a7a680128a57ddcb679057267dd5b886edffed9c8f023e4474d1be20d8ef7eab1c2151ca
|
7
|
+
data.tar.gz: a7e963a02497934fa619f4eb6ba2a81e42c19b7949a1e598c17682b348a1213c80361da1da2bdc464ef6a7b7f472a3a3a56f40cddc5c2ea80b673211980a2e5f
|
data/README.md
CHANGED
@@ -1,4 +1,4 @@
|
|
1
|
-
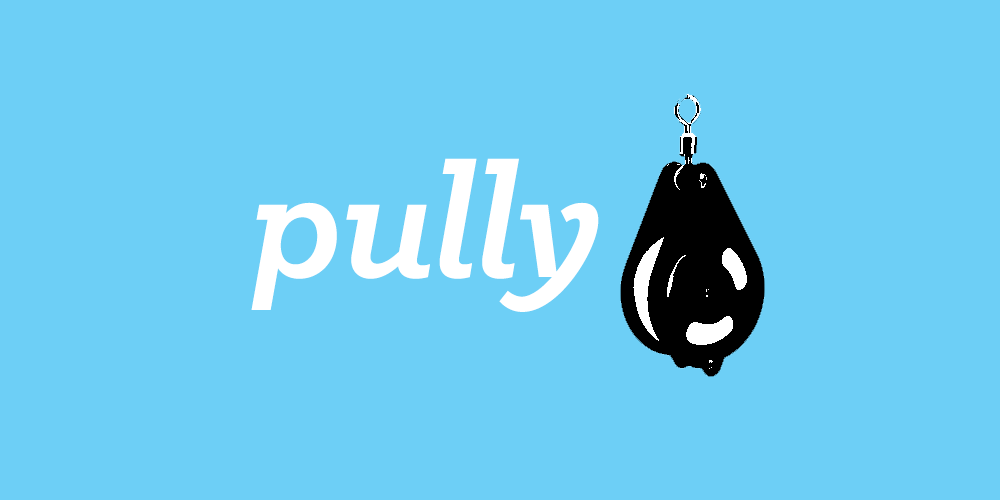
|
2
2
|
|
3
3
|
[](http://badge.fury.io/rb/pully)
|
4
4
|
[](https://travis-ci.org/sotownsend/Pully)
|
@@ -6,8 +6,12 @@
|
|
6
6
|
[](https://github.com/sotownsend/pully/blob/master/LICENSE)
|
7
7
|
|
8
8
|
# What is this?
|
9
|
+
Pully is a ruby library for managing GitHub pull requests; purpose built for our continuous integration & deployment infrastructure at [Fittr®](http://www.fittr.com).
|
9
10
|
|
10
|
-
|
11
|
+
# Selling points
|
12
|
+
1. It's easy to use
|
13
|
+
2. It has full code coverage on tests involving GitHub's API
|
14
|
+
3. While it uses the pull request interface on GitHub, **it does not use the big green button** to perform a merge.
|
11
15
|
|
12
16
|
# Basic usage
|
13
17
|
```ruby
|
@@ -24,9 +28,21 @@ pully.write_comment_to_pull_request(pull_number, "Test Comment")
|
|
24
28
|
|
25
29
|
#Get all comments
|
26
30
|
comments = pully.comments_for_pull_request(pull_number)
|
31
|
+
|
32
|
+
#Get the SHA of the 'from' branch of a certain pull request
|
33
|
+
pully.sha_for_pull_request(pull_number)
|
34
|
+
|
35
|
+
#Set the status of a pull request to pending (Other options include 'error', 'failed', and 'success')
|
36
|
+
pully.set_pull_request_status(pull_number, "pending")
|
37
|
+
|
38
|
+
#Set the status of a pull request to ready
|
39
|
+
pully.set_pull_request_status(pull_number, "success")
|
40
|
+
|
41
|
+
#Merge the request (Will NOT use GitHub's pull request merge, will merge commits into history as-is)
|
42
|
+
pully.merge_pull_request(pull_number)
|
27
43
|
```
|
28
44
|
|
29
|
-
# Organization
|
45
|
+
# Organization Repositories
|
30
46
|
If your repositories are not owner by you, i.e. they are owned by an organization or another user who has granted you permissions, you will need to
|
31
47
|
pass the `owner` field for the other individual or organization.
|
32
48
|
|
@@ -50,6 +66,13 @@ pully = Pully.new(user:"github_username", pass:"github_password", repo:"my_repos
|
|
50
66
|
|
51
67
|
Run `sudo gem install pully`
|
52
68
|
|
69
|
+
## Known issues
|
70
|
+
|
71
|
+
1. GitHub does not register commits immediately after a push is received. Things like `sha_for_pull_request` will return old values if you don't wait
|
72
|
+
several seconds
|
73
|
+
2. GitHub's status API for pull requests returns 'pending' even if the UI says 'success'. We account for this bug, but if it is fixed in the future,
|
74
|
+
then our specs will catch it
|
75
|
+
|
53
76
|
---
|
54
77
|
|
55
78
|
## FAQ
|
data/lib/pully/version.rb
CHANGED
data/lib/pully.rb
CHANGED
@@ -38,6 +38,39 @@ module Pully
|
|
38
38
|
def write_comment_to_pull_request pull_number, comment
|
39
39
|
@gh_client.add_comment(@repo_selector, pull_number, comment)
|
40
40
|
end
|
41
|
+
|
42
|
+
def sha_for_pull_request pull_number
|
43
|
+
@gh_client.pull_request(@repo_selector, pull_number).head.sha
|
44
|
+
end
|
45
|
+
|
46
|
+
def pull_request_status(pull_number)
|
47
|
+
sha = sha_for_pull_request pull_number
|
48
|
+
@gh_client.combined_status(@repo_selector, sha)["state"]
|
49
|
+
end
|
50
|
+
|
51
|
+
def set_pull_request_status(pull_number, status)
|
52
|
+
sha = sha_for_pull_request pull_number
|
53
|
+
@gh_client.create_status(@repo_selector, sha, status)
|
54
|
+
end
|
55
|
+
|
56
|
+
def merge_pull_request(pull_number)
|
57
|
+
branches = pull_request_branches(pull_number)
|
58
|
+
from_name = branches[:from]
|
59
|
+
to_name = branches[:to]
|
60
|
+
@gh_client.merge(@repo_selector, to_name, from_name)
|
61
|
+
@gh_client.close_pull_request(@repo_selector, pull_number)
|
62
|
+
end
|
63
|
+
|
64
|
+
def pull_request_branches(pull_number)
|
65
|
+
from_name = @gh_client.pull_request(@repo_selector, pull_number).head.ref
|
66
|
+
to_name = @gh_client.pull_request(@repo_selector, pull_number).base.ref
|
67
|
+
|
68
|
+
return {:from => from_name, :to => to_name}
|
69
|
+
end
|
70
|
+
|
71
|
+
def pull_request_is_open?(pull_number)
|
72
|
+
@gh_client.pull_request(@repo_selector, pull_number)["state"] == 'open'
|
73
|
+
end
|
41
74
|
end
|
42
75
|
|
43
76
|
module TestHelpers
|
@@ -122,6 +155,11 @@ module Pully
|
|
122
155
|
@git_client.branches.remote.map{|e| e.name}
|
123
156
|
end
|
124
157
|
|
158
|
+
def latest_sha branch_name
|
159
|
+
@git_client.checkout(branch_name)
|
160
|
+
@git_client.object("HEAD").sha
|
161
|
+
end
|
162
|
+
|
125
163
|
def master_branch
|
126
164
|
@repo.default_branch
|
127
165
|
end
|
@@ -130,13 +168,16 @@ module Pully
|
|
130
168
|
def commit_new_random_file(branch_name)
|
131
169
|
#Create a new file
|
132
170
|
Dir.chdir "#{@path}/pully" do
|
133
|
-
File.write branch_name, branch_name
|
171
|
+
File.write "#{branch_name}.#{SecureRandom.hex}", branch_name
|
134
172
|
end
|
135
173
|
|
136
174
|
#Commit
|
137
175
|
@git_client.add(:all => true)
|
138
176
|
@git_client.commit_all("New branch from Pully Test Suite #{SecureRandom.hex}")
|
177
|
+
local_sha = @git_client.object("HEAD").sha
|
139
178
|
@git_client.push("origin", branch_name)
|
179
|
+
|
180
|
+
return local_sha
|
140
181
|
end
|
141
182
|
end
|
142
183
|
end
|
data/spec/lib_spec.rb
CHANGED
@@ -31,6 +31,7 @@ RSpec.describe "Library" do
|
|
31
31
|
#end
|
32
32
|
|
33
33
|
it "Can call create a new pull request and returns an integer for the pull request #" do
|
34
|
+
sleep 10
|
34
35
|
#test branch creator
|
35
36
|
new_branch_name = SecureRandom.hex
|
36
37
|
th = Pully::TestHelpers::Branch.new(user: gh_info["user"], pass: gh_info["pass"], repo_selector: repo_selector(user: gh_info["user"], repo: gh_info["repo"], owner:nil), clone_url: gh_info["clone_url"])
|
@@ -45,6 +46,7 @@ RSpec.describe "Library" do
|
|
45
46
|
end
|
46
47
|
|
47
48
|
it "Can call create a new pull request for an organization repo and returns an integer for the pull request #" do
|
49
|
+
sleep 10
|
48
50
|
#test branch creator
|
49
51
|
new_branch_name = SecureRandom.hex
|
50
52
|
th = Pully::TestHelpers::Branch.new(user: gh_info["user"], pass: gh_info["pass"], repo_selector: repo_selector(user: gh_info["user"], repo: gh_info["org_repo"], owner:gh_info["org_owner"]), clone_url: gh_info["org_clone_url"])
|
@@ -95,4 +97,126 @@ RSpec.describe "Library" do
|
|
95
97
|
|
96
98
|
th.delete_branch(new_branch_name)
|
97
99
|
end
|
100
|
+
|
101
|
+
it "Can call create a new pull request, get the SHA of that pull request, and compare it to the SHA of the from branch" do
|
102
|
+
sleep 10
|
103
|
+
#test branch creator
|
104
|
+
new_branch_name = SecureRandom.hex
|
105
|
+
th = Pully::TestHelpers::Branch.new(user: gh_info["user"], pass: gh_info["pass"], repo_selector: repo_selector(user: gh_info["user"], repo: gh_info["repo"], owner:nil), clone_url: gh_info["clone_url"])
|
106
|
+
th.create_branch(new_branch_name)
|
107
|
+
local_sha = th.commit_new_random_file(new_branch_name)
|
108
|
+
|
109
|
+
pully = Pully.new(user: gh_info["user"], pass: gh_info["pass"], repo: gh_info["repo"])
|
110
|
+
pull_number = pully.create_pull_request(from:new_branch_name, to:"master", subject:"My pull request", message:"Hey XXXX, can you merge this for me?")
|
111
|
+
|
112
|
+
from_sha = pully.sha_for_pull_request(pull_number)
|
113
|
+
|
114
|
+
expect(local_sha).to eq(from_sha)
|
115
|
+
|
116
|
+
th.delete_branch(new_branch_name)
|
117
|
+
end
|
118
|
+
|
119
|
+
it "Changes the SHA when commits are added after a pull request" do
|
120
|
+
sleep 10
|
121
|
+
#test branch creator
|
122
|
+
new_branch_name = SecureRandom.hex
|
123
|
+
th = Pully::TestHelpers::Branch.new(user: gh_info["user"], pass: gh_info["pass"], repo_selector: repo_selector(user: gh_info["user"], repo: gh_info["repo"], owner:nil), clone_url: gh_info["clone_url"])
|
124
|
+
th.create_branch(new_branch_name)
|
125
|
+
local_sha_a = th.commit_new_random_file(new_branch_name)
|
126
|
+
|
127
|
+
pully = Pully.new(user: gh_info["user"], pass: gh_info["pass"], repo: gh_info["repo"])
|
128
|
+
pull_number = pully.create_pull_request(from:new_branch_name, to:"master", subject:"My pull request", message:"Hey XXXX, can you merge this for me?")
|
129
|
+
|
130
|
+
#Get the SHA from the pull request
|
131
|
+
from_sha_a = pully.sha_for_pull_request(pull_number)
|
132
|
+
expect(local_sha_a).to eq(from_sha_a)
|
133
|
+
|
134
|
+
local_sha_b = th.commit_new_random_file(new_branch_name)
|
135
|
+
sleep 5 #Wait for GitHub to update
|
136
|
+
from_sha_b = pully.sha_for_pull_request(pull_number)
|
137
|
+
expect(local_sha_b).not_to eq(local_sha_a)
|
138
|
+
expect(local_sha_b).to eq(from_sha_b)
|
139
|
+
|
140
|
+
th.delete_branch(new_branch_name)
|
141
|
+
end
|
142
|
+
|
143
|
+
it "Can set the pull request status to success, assumes BUG IN GITHUB SETS TO PENDING" do
|
144
|
+
sleep 10
|
145
|
+
#test branch creator
|
146
|
+
new_branch_name = SecureRandom.hex
|
147
|
+
th = Pully::TestHelpers::Branch.new(user: gh_info["user"], pass: gh_info["pass"], repo_selector: repo_selector(user: gh_info["user"], repo: gh_info["repo"], owner:nil), clone_url: gh_info["clone_url"])
|
148
|
+
th.create_branch(new_branch_name)
|
149
|
+
th.commit_new_random_file(new_branch_name)
|
150
|
+
|
151
|
+
pully = Pully.new(user: gh_info["user"], pass: gh_info["pass"], repo: gh_info["repo"])
|
152
|
+
pull_number = pully.create_pull_request(from:new_branch_name, to:"master", subject:"My pull request", message:"Hey XXXX, can you merge this for me?")
|
153
|
+
pully.sha_for_pull_request(pull_number)
|
154
|
+
|
155
|
+
status = pully.pull_request_status(pull_number)
|
156
|
+
expect(status).to eq("pending")
|
157
|
+
|
158
|
+
pully.set_pull_request_status(pull_number, "success")
|
159
|
+
status = pully.pull_request_status(pull_number)
|
160
|
+
expect(status).to eq("success")
|
161
|
+
|
162
|
+
th.delete_branch(new_branch_name)
|
163
|
+
end
|
164
|
+
|
165
|
+
it "Can get the from and to names of the pull request" do
|
166
|
+
sleep 10
|
167
|
+
#test branch creator
|
168
|
+
new_branch_name = SecureRandom.hex
|
169
|
+
th = Pully::TestHelpers::Branch.new(user: gh_info["user"], pass: gh_info["pass"], repo_selector: repo_selector(user: gh_info["user"], repo: gh_info["repo"], owner:nil), clone_url: gh_info["clone_url"])
|
170
|
+
th.create_branch(new_branch_name)
|
171
|
+
th.commit_new_random_file(new_branch_name)
|
172
|
+
|
173
|
+
pully = Pully.new(user: gh_info["user"], pass: gh_info["pass"], repo: gh_info["repo"])
|
174
|
+
pull_number = pully.create_pull_request(from:new_branch_name, to:"master", subject:"My pull request", message:"Hey XXXX, can you merge this for me?")
|
175
|
+
branches = pully.pull_request_branches(pull_number)
|
176
|
+
|
177
|
+
expect(branches[:from]).to eq(new_branch_name)
|
178
|
+
expect(branches[:to]).to eq("master")
|
179
|
+
|
180
|
+
th.delete_branch(new_branch_name)
|
181
|
+
end
|
182
|
+
|
183
|
+
it "Can get the from and to names of the pull request from an organization" do
|
184
|
+
sleep 10
|
185
|
+
#test branch creator
|
186
|
+
new_branch_name = SecureRandom.hex
|
187
|
+
th = Pully::TestHelpers::Branch.new(user: gh_info["user"], pass: gh_info["pass"], repo_selector: repo_selector(user: gh_info["user"], repo: gh_info["org_repo"], owner:gh_info["org_owner"]), clone_url: gh_info["org_clone_url"])
|
188
|
+
th.create_branch(new_branch_name)
|
189
|
+
th.commit_new_random_file(new_branch_name)
|
190
|
+
|
191
|
+
pully = Pully.new(user: gh_info["user"], pass: gh_info["pass"], repo: gh_info["org_repo"])
|
192
|
+
pull_number = pully.create_pull_request(from:new_branch_name, to:"master", subject:"My pull request", message:"Hey XXXX, can you merge this for me?")
|
193
|
+
branches = pully.pull_request_branches(pull_number)
|
194
|
+
|
195
|
+
expect(branches[:from]).to eq(new_branch_name)
|
196
|
+
expect(branches[:to]).to eq("master")
|
197
|
+
|
198
|
+
th.delete_branch(new_branch_name)
|
199
|
+
end
|
200
|
+
|
201
|
+
it "Can merge the pull request into master" do
|
202
|
+
sleep 10
|
203
|
+
#test branch creator
|
204
|
+
new_branch_name = SecureRandom.hex
|
205
|
+
th = Pully::TestHelpers::Branch.new(user: gh_info["user"], pass: gh_info["pass"], repo_selector: repo_selector(user: gh_info["user"], repo: gh_info["repo"], owner:nil), clone_url: gh_info["clone_url"])
|
206
|
+
th.create_branch(new_branch_name)
|
207
|
+
last_sha = th.commit_new_random_file(new_branch_name)
|
208
|
+
|
209
|
+
pully = Pully.new(user: gh_info["user"], pass: gh_info["pass"], repo: gh_info["repo"])
|
210
|
+
pull_number = pully.create_pull_request(from:new_branch_name, to:"master", subject:"My pull request", message:"Hey XXXX, can you merge this for me?")
|
211
|
+
pully.merge_pull_request(pull_number)
|
212
|
+
|
213
|
+
#Check leading sha
|
214
|
+
th = Pully::TestHelpers::Branch.new(user: gh_info["user"], pass: gh_info["pass"], repo_selector: repo_selector(user: gh_info["user"], repo: gh_info["repo"], owner:nil), clone_url: gh_info["clone_url"])
|
215
|
+
expect(th.latest_sha("master")).to eq(last_sha)
|
216
|
+
|
217
|
+
#We expect the pull request to be closed
|
218
|
+
expect(pully.pull_request_is_open?(pull_number)).to eq(false)
|
219
|
+
|
220
|
+
th.delete_branch(new_branch_name)
|
221
|
+
end
|
98
222
|
end
|
data/spec/lib_test_spec.rb
CHANGED
@@ -53,4 +53,17 @@ RSpec.describe "Test Library" do
|
|
53
53
|
th.delete_branch(new_branch_name)
|
54
54
|
expect(th.list_branches).not_to include(new_branch_name)
|
55
55
|
end
|
56
|
+
|
57
|
+
it "Can create a new branch from our repository, grab something that looks like a SHA of the commit, and delete it" do
|
58
|
+
new_branch_name = SecureRandom.hex
|
59
|
+
th = Pully::TestHelpers::Branch.new(user: gh_info["user"], pass: gh_info["pass"], repo_selector: repo_selector, clone_url: gh_info["clone_url"])
|
60
|
+
|
61
|
+
th.create_branch(new_branch_name)
|
62
|
+
sha = th.commit_new_random_file(new_branch_name)
|
63
|
+
expect(sha.class).to be(String)
|
64
|
+
expect(sha.length).to be(40)
|
65
|
+
|
66
|
+
#Delete the branch
|
67
|
+
th.delete_branch(new_branch_name)
|
68
|
+
end
|
56
69
|
end
|