ollama-ai 1.0.0 → 1.2.0
This diff represents the content of publicly available package versions that have been released to one of the supported registries. The information contained in this diff is provided for informational purposes only and reflects changes between package versions as they appear in their respective public registries.
- checksums.yaml +4 -4
- data/Gemfile +1 -1
- data/Gemfile.lock +23 -14
- data/README.md +264 -183
- data/controllers/client.rb +9 -3
- data/ollama-ai.gemspec +2 -1
- data/static/gem.rb +1 -1
- data/tasks/generate-readme.clj +1 -1
- data/template.md +231 -154
- metadata +22 -8
data/template.md
CHANGED
@@ -1,6 +1,6 @@
|
|
1
1
|
# Ollama AI
|
2
2
|
|
3
|
-
A Ruby gem for interacting with [Ollama](https://
|
3
|
+
A Ruby gem for interacting with [Ollama](https://ollama.ai)'s API that allows you to run open source AI LLMs (Large Language Models) locally.
|
4
4
|
|
5
5
|
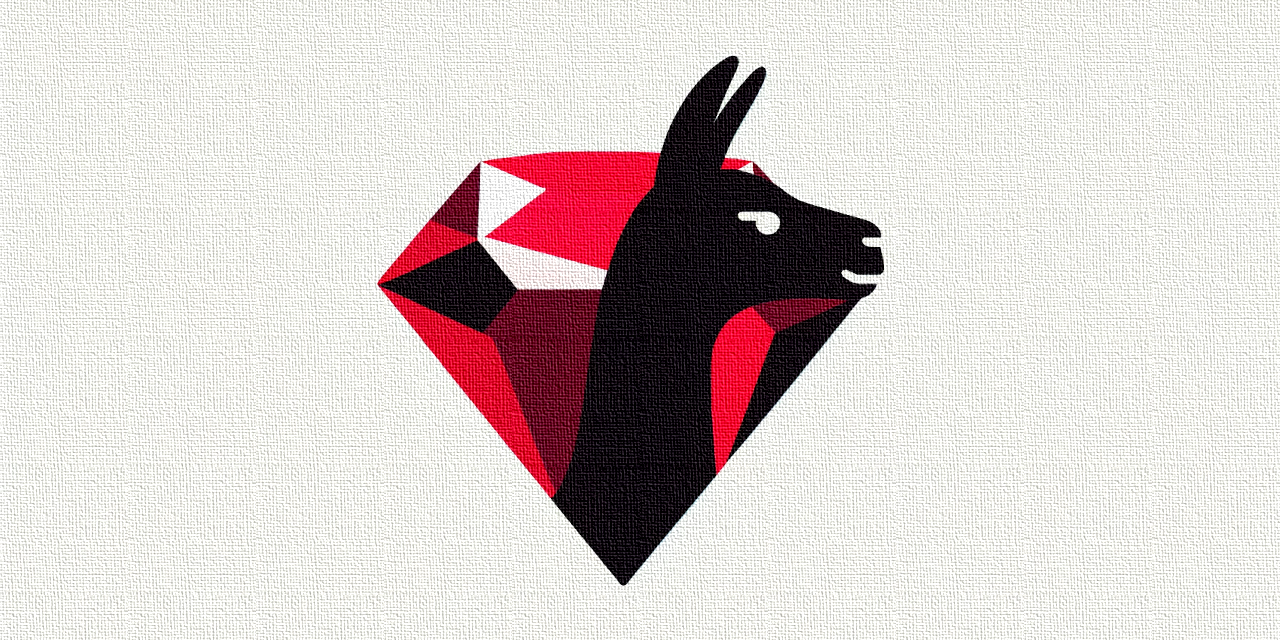
|
6
6
|
|
@@ -9,7 +9,7 @@ A Ruby gem for interacting with [Ollama](https://github.com/jmorganca/ollama)'s
|
|
9
9
|
## TL;DR and Quick Start
|
10
10
|
|
11
11
|
```ruby
|
12
|
-
gem 'ollama-ai', '~> 1.
|
12
|
+
gem 'ollama-ai', '~> 1.2.0'
|
13
13
|
```
|
14
14
|
|
15
15
|
```ruby
|
@@ -21,40 +21,40 @@ client = Ollama.new(
|
|
21
21
|
)
|
22
22
|
|
23
23
|
result = client.generate(
|
24
|
-
{ model: '
|
24
|
+
{ model: 'llama2',
|
25
25
|
prompt: 'Hi!' }
|
26
26
|
)
|
27
27
|
```
|
28
28
|
|
29
29
|
Result:
|
30
30
|
```ruby
|
31
|
-
[{ 'model' => '
|
32
|
-
'created_at' => '2024-01-
|
31
|
+
[{ 'model' => 'llama2',
|
32
|
+
'created_at' => '2024-01-07T01:34:02.088810408Z',
|
33
33
|
'response' => 'Hello',
|
34
34
|
'done' => false },
|
35
|
-
{ 'model' => '
|
36
|
-
'created_at' => '2024-01-
|
35
|
+
{ 'model' => 'llama2',
|
36
|
+
'created_at' => '2024-01-07T01:34:02.419045606Z',
|
37
37
|
'response' => '!',
|
38
38
|
'done' => false },
|
39
|
-
#
|
40
|
-
{ 'model' => '
|
41
|
-
'created_at' => '2024-01-
|
42
|
-
'response' => '
|
39
|
+
# ..
|
40
|
+
{ 'model' => 'llama2',
|
41
|
+
'created_at' => '2024-01-07T01:34:07.680049831Z',
|
42
|
+
'response' => '?',
|
43
43
|
'done' => false },
|
44
|
-
{ 'model' => '
|
45
|
-
'created_at' => '2024-01-
|
44
|
+
{ 'model' => 'llama2',
|
45
|
+
'created_at' => '2024-01-07T01:34:07.872170352Z',
|
46
46
|
'response' => '',
|
47
47
|
'done' => true,
|
48
48
|
'context' =>
|
49
|
-
[
|
49
|
+
[518, 25_580,
|
50
50
|
# ...
|
51
|
-
|
52
|
-
'total_duration' =>
|
53
|
-
'load_duration' =>
|
54
|
-
'prompt_eval_count' =>
|
55
|
-
'prompt_eval_duration' =>
|
56
|
-
'eval_count' =>
|
57
|
-
'eval_duration' =>
|
51
|
+
13_563, 29_973],
|
52
|
+
'total_duration' => 11_653_781_127,
|
53
|
+
'load_duration' => 1_186_200_439,
|
54
|
+
'prompt_eval_count' => 22,
|
55
|
+
'prompt_eval_duration' => 5_006_751_000,
|
56
|
+
'eval_count' => 25,
|
57
|
+
'eval_duration' => 5_453_058_000 }]
|
58
58
|
```
|
59
59
|
|
60
60
|
## Index
|
@@ -66,11 +66,11 @@ Result:
|
|
66
66
|
### Installing
|
67
67
|
|
68
68
|
```sh
|
69
|
-
gem install ollama-ai -v 1.
|
69
|
+
gem install ollama-ai -v 1.2.0
|
70
70
|
```
|
71
71
|
|
72
72
|
```sh
|
73
|
-
gem 'ollama-ai', '~> 1.
|
73
|
+
gem 'ollama-ai', '~> 1.2.0'
|
74
74
|
```
|
75
75
|
|
76
76
|
## Usage
|
@@ -113,7 +113,7 @@ API Documentation: https://github.com/jmorganca/ollama/blob/main/docs/api.md#gen
|
|
113
113
|
|
114
114
|
```ruby
|
115
115
|
result = client.generate(
|
116
|
-
{ model: '
|
116
|
+
{ model: 'llama2',
|
117
117
|
prompt: 'Hi!',
|
118
118
|
stream: false }
|
119
119
|
)
|
@@ -121,21 +121,20 @@ result = client.generate(
|
|
121
121
|
|
122
122
|
Result:
|
123
123
|
```ruby
|
124
|
-
[{ 'model' => '
|
125
|
-
'created_at' => '2024-01-
|
126
|
-
'response' =>
|
127
|
-
"Hello! How can I assist you today? Do you have any questions or problems that you'd like help with?",
|
124
|
+
[{ 'model' => 'llama2',
|
125
|
+
'created_at' => '2024-01-07T01:35:41.951371247Z',
|
126
|
+
'response' => "Hi there! It's nice to meet you. How are you today?",
|
128
127
|
'done' => true,
|
129
128
|
'context' =>
|
130
|
-
[
|
129
|
+
[518, 25_580,
|
131
130
|
# ...
|
132
|
-
|
133
|
-
'total_duration' =>
|
134
|
-
'load_duration' =>
|
135
|
-
'prompt_eval_count' =>
|
136
|
-
'prompt_eval_duration' =>
|
137
|
-
'eval_count' =>
|
138
|
-
'eval_duration' =>
|
131
|
+
9826, 29_973],
|
132
|
+
'total_duration' => 6_981_097_576,
|
133
|
+
'load_duration' => 625_053,
|
134
|
+
'prompt_eval_count' => 22,
|
135
|
+
'prompt_eval_duration' => 4_075_171_000,
|
136
|
+
'eval_count' => 16,
|
137
|
+
'eval_duration' => 2_900_325_000 }]
|
139
138
|
```
|
140
139
|
|
141
140
|
##### Receiving Stream Events
|
@@ -146,7 +145,7 @@ Ensure that you have enabled [Server-Sent Events](#streaming-and-server-sent-eve
|
|
146
145
|
|
147
146
|
```ruby
|
148
147
|
client.generate(
|
149
|
-
{ model: '
|
148
|
+
{ model: 'llama2',
|
150
149
|
prompt: 'Hi!' }
|
151
150
|
) do |event, raw|
|
152
151
|
puts event
|
@@ -155,8 +154,8 @@ end
|
|
155
154
|
|
156
155
|
Event:
|
157
156
|
```ruby
|
158
|
-
{ 'model' => '
|
159
|
-
'created_at' => '2024-01-
|
157
|
+
{ 'model' => 'llama2',
|
158
|
+
'created_at' => '2024-01-07T01:36:30.665245712Z',
|
160
159
|
'response' => 'Hello',
|
161
160
|
'done' => false }
|
162
161
|
```
|
@@ -164,46 +163,46 @@ Event:
|
|
164
163
|
You can get all the receive events at once as an array:
|
165
164
|
```ruby
|
166
165
|
result = client.generate(
|
167
|
-
{ model: '
|
166
|
+
{ model: 'llama2',
|
168
167
|
prompt: 'Hi!' }
|
169
168
|
)
|
170
169
|
```
|
171
170
|
|
172
171
|
Result:
|
173
172
|
```ruby
|
174
|
-
[{ 'model' => '
|
175
|
-
'created_at' => '2024-01-
|
173
|
+
[{ 'model' => 'llama2',
|
174
|
+
'created_at' => '2024-01-07T01:36:30.665245712Z',
|
176
175
|
'response' => 'Hello',
|
177
176
|
'done' => false },
|
178
|
-
{ 'model' => '
|
179
|
-
'created_at' => '2024-01-
|
177
|
+
{ 'model' => 'llama2',
|
178
|
+
'created_at' => '2024-01-07T01:36:30.927337136Z',
|
180
179
|
'response' => '!',
|
181
180
|
'done' => false },
|
182
181
|
# ...
|
183
|
-
{ 'model' => '
|
184
|
-
'created_at' => '2024-01-
|
185
|
-
'response' => '
|
182
|
+
{ 'model' => 'llama2',
|
183
|
+
'created_at' => '2024-01-07T01:36:37.249416767Z',
|
184
|
+
'response' => '?',
|
186
185
|
'done' => false },
|
187
|
-
{ 'model' => '
|
188
|
-
'created_at' => '2024-01-
|
186
|
+
{ 'model' => 'llama2',
|
187
|
+
'created_at' => '2024-01-07T01:36:37.44041283Z',
|
189
188
|
'response' => '',
|
190
189
|
'done' => true,
|
191
190
|
'context' =>
|
192
|
-
[
|
191
|
+
[518, 25_580,
|
193
192
|
# ...
|
194
|
-
|
195
|
-
'total_duration' =>
|
196
|
-
'load_duration' =>
|
197
|
-
'prompt_eval_count' =>
|
198
|
-
'prompt_eval_duration' =>
|
199
|
-
'eval_count' =>
|
200
|
-
'eval_duration' =>
|
193
|
+
13_563, 29_973],
|
194
|
+
'total_duration' => 10_551_395_645,
|
195
|
+
'load_duration' => 966_631,
|
196
|
+
'prompt_eval_count' => 22,
|
197
|
+
'prompt_eval_duration' => 4_034_990_000,
|
198
|
+
'eval_count' => 25,
|
199
|
+
'eval_duration' => 6_512_954_000 }]
|
201
200
|
```
|
202
201
|
|
203
202
|
You can mix both as well:
|
204
203
|
```ruby
|
205
204
|
result = client.generate(
|
206
|
-
{ model: '
|
205
|
+
{ model: 'llama2',
|
207
206
|
prompt: 'Hi!' }
|
208
207
|
) do |event, raw|
|
209
208
|
puts event
|
@@ -216,7 +215,7 @@ API Documentation: https://github.com/jmorganca/ollama/blob/main/docs/api.md#gen
|
|
216
215
|
|
217
216
|
```ruby
|
218
217
|
result = client.chat(
|
219
|
-
{ model: '
|
218
|
+
{ model: 'llama2',
|
220
219
|
messages: [
|
221
220
|
{ role: 'user', content: 'Hi! My name is Purple.' }
|
222
221
|
] }
|
@@ -227,37 +226,37 @@ end
|
|
227
226
|
|
228
227
|
Event:
|
229
228
|
```ruby
|
230
|
-
{ 'model' => '
|
231
|
-
'created_at' => '2024-01-
|
232
|
-
'message' => { 'role' => 'assistant', 'content' =>
|
229
|
+
{ 'model' => 'llama2',
|
230
|
+
'created_at' => '2024-01-07T01:38:01.729897311Z',
|
231
|
+
'message' => { 'role' => 'assistant', 'content' => "\n" },
|
233
232
|
'done' => false }
|
234
233
|
```
|
235
234
|
|
236
235
|
Result:
|
237
236
|
```ruby
|
238
|
-
[{ 'model' => '
|
239
|
-
'created_at' => '2024-01-
|
240
|
-
'message' => { 'role' => 'assistant', 'content' =>
|
237
|
+
[{ 'model' => 'llama2',
|
238
|
+
'created_at' => '2024-01-07T01:38:01.729897311Z',
|
239
|
+
'message' => { 'role' => 'assistant', 'content' => "\n" },
|
241
240
|
'done' => false },
|
242
|
-
{ 'model' => '
|
243
|
-
'created_at' => '2024-01-
|
244
|
-
'message' => { 'role' => 'assistant', 'content' => '
|
241
|
+
{ 'model' => 'llama2',
|
242
|
+
'created_at' => '2024-01-07T01:38:02.081494506Z',
|
243
|
+
'message' => { 'role' => 'assistant', 'content' => '*' },
|
245
244
|
'done' => false },
|
246
245
|
# ...
|
247
|
-
{ 'model' => '
|
248
|
-
'created_at' => '2024-01-
|
246
|
+
{ 'model' => 'llama2',
|
247
|
+
'created_at' => '2024-01-07T01:38:17.855905499Z',
|
249
248
|
'message' => { 'role' => 'assistant', 'content' => '?' },
|
250
249
|
'done' => false },
|
251
|
-
{ 'model' => '
|
252
|
-
'created_at' => '2024-01-
|
250
|
+
{ 'model' => 'llama2',
|
251
|
+
'created_at' => '2024-01-07T01:38:18.07331245Z',
|
253
252
|
'message' => { 'role' => 'assistant', 'content' => '' },
|
254
253
|
'done' => true,
|
255
|
-
'total_duration' =>
|
256
|
-
'load_duration' =>
|
257
|
-
'prompt_eval_count' =>
|
258
|
-
'prompt_eval_duration' =>
|
259
|
-
'eval_count' =>
|
260
|
-
'eval_duration' =>
|
254
|
+
'total_duration' => 22_494_544_502,
|
255
|
+
'load_duration' => 4_224_600,
|
256
|
+
'prompt_eval_count' => 28,
|
257
|
+
'prompt_eval_duration' => 6_496_583_000,
|
258
|
+
'eval_count' => 61,
|
259
|
+
'eval_duration' => 15_991_728_000 }]
|
261
260
|
```
|
262
261
|
|
263
262
|
##### Back-and-Forth Conversations
|
@@ -268,11 +267,11 @@ To maintain a back-and-forth conversation, you need to append the received respo
|
|
268
267
|
|
269
268
|
```ruby
|
270
269
|
result = client.chat(
|
271
|
-
{ model: '
|
270
|
+
{ model: 'llama2',
|
272
271
|
messages: [
|
273
272
|
{ role: 'user', content: 'Hi! My name is Purple.' },
|
274
273
|
{ role: 'assistant',
|
275
|
-
content:
|
274
|
+
content: 'Hi, Purple!' },
|
276
275
|
{ role: 'user', content: "What's my name?" }
|
277
276
|
] }
|
278
277
|
) do |event, raw|
|
@@ -283,37 +282,41 @@ end
|
|
283
282
|
Event:
|
284
283
|
|
285
284
|
```ruby
|
286
|
-
{ 'model' => '
|
287
|
-
'created_at' => '2024-01-
|
288
|
-
'message' => { 'role' => 'assistant', 'content' => '
|
285
|
+
{ 'model' => 'llama2',
|
286
|
+
'created_at' => '2024-01-07T01:40:07.352998498Z',
|
287
|
+
'message' => { 'role' => 'assistant', 'content' => ' Pur' },
|
289
288
|
'done' => false }
|
290
289
|
```
|
291
290
|
|
292
291
|
Result:
|
293
292
|
```ruby
|
294
|
-
[{ 'model' => '
|
295
|
-
'created_at' => '2024-01-
|
293
|
+
[{ 'model' => 'llama2',
|
294
|
+
'created_at' => '2024-01-07T01:40:06.562939469Z',
|
296
295
|
'message' => { 'role' => 'assistant', 'content' => 'Your' },
|
297
296
|
'done' => false },
|
298
|
-
{ 'model' => 'dolphin-phi',
|
299
|
-
'created_at' => '2024-01-06T19:07:51.184476541Z',
|
300
|
-
'message' => { 'role' => 'assistant', 'content' => ' name' },
|
301
|
-
'done' => false },
|
302
297
|
# ...
|
303
|
-
{ 'model' => '
|
304
|
-
'created_at' => '2024-01-
|
305
|
-
'message' => { 'role' => 'assistant', 'content' => '
|
298
|
+
{ 'model' => 'llama2',
|
299
|
+
'created_at' => '2024-01-07T01:40:07.352998498Z',
|
300
|
+
'message' => { 'role' => 'assistant', 'content' => ' Pur' },
|
301
|
+
'done' => false },
|
302
|
+
{ 'model' => 'llama2',
|
303
|
+
'created_at' => '2024-01-07T01:40:07.545323584Z',
|
304
|
+
'message' => { 'role' => 'assistant', 'content' => 'ple' },
|
305
|
+
'done' => false },
|
306
|
+
{ 'model' => 'llama2',
|
307
|
+
'created_at' => '2024-01-07T01:40:07.77769408Z',
|
308
|
+
'message' => { 'role' => 'assistant', 'content' => '!' },
|
306
309
|
'done' => false },
|
307
|
-
{ 'model' => '
|
308
|
-
'created_at' => '2024-01-
|
310
|
+
{ 'model' => 'llama2',
|
311
|
+
'created_at' => '2024-01-07T01:40:07.974165849Z',
|
309
312
|
'message' => { 'role' => 'assistant', 'content' => '' },
|
310
313
|
'done' => true,
|
311
|
-
'total_duration' =>
|
312
|
-
'load_duration' =>
|
313
|
-
'prompt_eval_count' =>
|
314
|
-
'prompt_eval_duration' =>
|
315
|
-
'eval_count' =>
|
316
|
-
'eval_duration' =>
|
314
|
+
'total_duration' => 11_482_012_681,
|
315
|
+
'load_duration' => 4_246_882,
|
316
|
+
'prompt_eval_count' => 57,
|
317
|
+
'prompt_eval_duration' => 10_387_150_000,
|
318
|
+
'eval_count' => 6,
|
319
|
+
'eval_duration' => 1_089_249_000 }]
|
317
320
|
```
|
318
321
|
|
319
322
|
#### embeddings: Generate Embeddings
|
@@ -322,7 +325,7 @@ API Documentation: https://github.com/jmorganca/ollama/blob/main/docs/api.md#gen
|
|
322
325
|
|
323
326
|
```ruby
|
324
327
|
result = client.embeddings(
|
325
|
-
{ model: '
|
328
|
+
{ model: 'llama2',
|
326
329
|
prompt: 'Hi!' }
|
327
330
|
)
|
328
331
|
```
|
@@ -330,11 +333,9 @@ result = client.embeddings(
|
|
330
333
|
Result:
|
331
334
|
```ruby
|
332
335
|
[{ 'embedding' =>
|
333
|
-
[
|
334
|
-
1.0635842084884644,
|
336
|
+
[0.6970467567443848, -2.248202085494995,
|
335
337
|
# ...
|
336
|
-
-0.
|
337
|
-
0.051569778472185135] }]
|
338
|
+
-1.5994540452957153, -0.3464218080043793] }]
|
338
339
|
```
|
339
340
|
|
340
341
|
#### Models
|
@@ -346,7 +347,7 @@ API Documentation: https://github.com/jmorganca/ollama/blob/main/docs/api.md#cre
|
|
346
347
|
```ruby
|
347
348
|
result = client.create(
|
348
349
|
{ name: 'mario',
|
349
|
-
modelfile: "FROM
|
350
|
+
modelfile: "FROM llama2\nSYSTEM You are mario from Super Mario Bros." }
|
350
351
|
) do |event, raw|
|
351
352
|
puts event
|
352
353
|
end
|
@@ -387,9 +388,7 @@ client.generate(
|
|
387
388
|
end
|
388
389
|
```
|
389
390
|
|
390
|
-
>
|
391
|
-
>
|
392
|
-
> _What brings you here? How can I help you on your journey?_
|
391
|
+
> _Woah! *adjusts sunglasses* It's-a me, Mario! *winks* You must be a new friend I've-a met here in the Mushroom Kingdom. *tips top hat* What brings you to this neck of the woods? Maybe you're looking for-a some help on your adventure? *nods* Just let me know, and I'll do my best to-a assist ya! 😃_
|
393
392
|
|
394
393
|
##### tags: List Local Models
|
395
394
|
|
@@ -402,28 +401,28 @@ result = client.tags
|
|
402
401
|
Result:
|
403
402
|
```ruby
|
404
403
|
[{ 'models' =>
|
405
|
-
[{ 'name' => '
|
406
|
-
'modified_at' => '2024-01-
|
407
|
-
'size' =>
|
404
|
+
[{ 'name' => 'llama2:latest',
|
405
|
+
'modified_at' => '2024-01-06T15:06:23.6349195-03:00',
|
406
|
+
'size' => 3_826_793_677,
|
408
407
|
'digest' =>
|
409
|
-
|
408
|
+
'78e26419b4469263f75331927a00a0284ef6544c1975b826b15abdaef17bb962',
|
410
409
|
'details' =>
|
411
|
-
|
412
|
-
|
413
|
-
|
414
|
-
|
415
|
-
|
410
|
+
{ 'format' => 'gguf',
|
411
|
+
'family' => 'llama',
|
412
|
+
'families' => ['llama'],
|
413
|
+
'parameter_size' => '7B',
|
414
|
+
'quantization_level' => 'Q4_0' } },
|
416
415
|
{ 'name' => 'mario:latest',
|
417
|
-
'modified_at' => '2024-01-
|
418
|
-
'size' =>
|
416
|
+
'modified_at' => '2024-01-06T22:41:59.495298101-03:00',
|
417
|
+
'size' => 3_826_793_787,
|
419
418
|
'digest' =>
|
420
|
-
|
419
|
+
'291f46d2fa687dfaff45de96a8cb6e32707bc16ec1e1dfe8d65e9634c34c660c',
|
421
420
|
'details' =>
|
422
|
-
|
423
|
-
|
424
|
-
|
425
|
-
|
426
|
-
|
421
|
+
{ 'format' => 'gguf',
|
422
|
+
'family' => 'llama',
|
423
|
+
'families' => ['llama'],
|
424
|
+
'parameter_size' => '7B',
|
425
|
+
'quantization_level' => 'Q4_0' } }] }]
|
427
426
|
```
|
428
427
|
|
429
428
|
##### show: Show Model Information
|
@@ -432,35 +431,33 @@ API Documentation: https://github.com/jmorganca/ollama/blob/main/docs/api.md#sho
|
|
432
431
|
|
433
432
|
```ruby
|
434
433
|
result = client.show(
|
435
|
-
{ name: '
|
434
|
+
{ name: 'llama2' }
|
436
435
|
)
|
437
436
|
```
|
438
437
|
|
439
438
|
Result:
|
440
439
|
```ruby
|
441
440
|
[{ 'license' =>
|
442
|
-
"
|
441
|
+
"LLAMA 2 COMMUNITY LICENSE AGREEMENT\t\n" \
|
443
442
|
# ...
|
444
|
-
|
443
|
+
"* Reporting violations of the Acceptable Use Policy or unlicensed uses of Llama..." \
|
444
|
+
"\n",
|
445
445
|
'modelfile' =>
|
446
446
|
"# Modelfile generated by \"ollama show\"\n" \
|
447
447
|
# ...
|
448
|
-
'PARAMETER stop "
|
448
|
+
'PARAMETER stop "<</SYS>>"',
|
449
449
|
'parameters' =>
|
450
|
-
"stop
|
451
|
-
|
452
|
-
|
453
|
-
|
454
|
-
|
455
|
-
"
|
456
|
-
"{{ .Prompt }}<|im_end|>\n" \
|
457
|
-
"<|im_start|>assistant\n",
|
458
|
-
'system' => 'You are Dolphin, a helpful AI assistant.',
|
450
|
+
"stop [INST]\n" \
|
451
|
+
"stop [/INST]\n" \
|
452
|
+
"stop <<SYS>>\n" \
|
453
|
+
'stop <</SYS>>',
|
454
|
+
'template' =>
|
455
|
+
"[INST] <<SYS>>{{ .System }}<</SYS>>\n\n{{ .Prompt }} [/INST]\n",
|
459
456
|
'details' =>
|
460
457
|
{ 'format' => 'gguf',
|
461
|
-
'family' => '
|
462
|
-
'families' => ['
|
463
|
-
'parameter_size' => '
|
458
|
+
'family' => 'llama',
|
459
|
+
'families' => ['llama'],
|
460
|
+
'parameter_size' => '7B',
|
464
461
|
'quantization_level' => 'Q4_0' } }]
|
465
462
|
```
|
466
463
|
|
@@ -470,8 +467,8 @@ API Documentation: https://github.com/jmorganca/ollama/blob/main/docs/api.md#cop
|
|
470
467
|
|
471
468
|
```ruby
|
472
469
|
result = client.copy(
|
473
|
-
{ source: '
|
474
|
-
destination: '
|
470
|
+
{ source: 'llama2',
|
471
|
+
destination: 'llama2-backup' }
|
475
472
|
)
|
476
473
|
```
|
477
474
|
|
@@ -508,7 +505,7 @@ API Documentation: https://github.com/jmorganca/ollama/blob/main/docs/api.md#del
|
|
508
505
|
|
509
506
|
```ruby
|
510
507
|
result = client.delete(
|
511
|
-
{ name: '
|
508
|
+
{ name: 'llama2' }
|
512
509
|
)
|
513
510
|
```
|
514
511
|
|
@@ -521,14 +518,14 @@ If the model does not exist:
|
|
521
518
|
```ruby
|
522
519
|
begin
|
523
520
|
result = client.delete(
|
524
|
-
{ name: '
|
521
|
+
{ name: 'llama2' }
|
525
522
|
)
|
526
523
|
rescue Ollama::Errors::OllamaError => error
|
527
524
|
puts error.class # Ollama::Errors::RequestError
|
528
525
|
puts error.message # 'the server responded with status 404'
|
529
526
|
|
530
527
|
puts error.payload
|
531
|
-
# { name: '
|
528
|
+
# { name: 'llama2',
|
532
529
|
# ...
|
533
530
|
# }
|
534
531
|
|
@@ -543,7 +540,7 @@ API Documentation: https://github.com/jmorganca/ollama/blob/main/docs/api.md#pul
|
|
543
540
|
|
544
541
|
```ruby
|
545
542
|
result = client.pull(
|
546
|
-
{ name: '
|
543
|
+
{ name: 'llama2' }
|
547
544
|
) do |event, raw|
|
548
545
|
puts event
|
549
546
|
end
|
@@ -625,6 +622,69 @@ Result:
|
|
625
622
|
{ 'status' => 'success' }]
|
626
623
|
```
|
627
624
|
|
625
|
+
### Modes
|
626
|
+
|
627
|
+
#### Text
|
628
|
+
|
629
|
+
You can use the [generate](#generate-generate-a-completion) or [chat](#chat-generate-a-chat-completion) methods for text.
|
630
|
+
|
631
|
+
#### Image
|
632
|
+
|
633
|
+
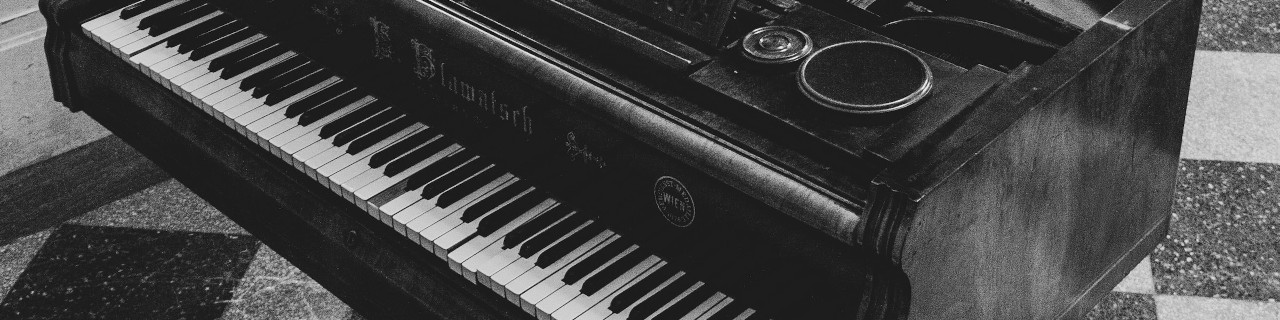
|
634
|
+
|
635
|
+
> _Courtesy of [Unsplash](https://unsplash.com/photos/greyscale-photo-of-grand-piano-czPs0z3-Ggg)_
|
636
|
+
|
637
|
+
You need to choose a model that supports images, like [LLaVA](https://ollama.ai/library/llava) or [bakllava](https://ollama.ai/library/bakllava), and encode the image as [Base64](https://en.wikipedia.org/wiki/Base64).
|
638
|
+
|
639
|
+
Depending on your hardware, some models that support images can be slow, so you may want to increase the client [timeout](#timeout):
|
640
|
+
|
641
|
+
```ruby
|
642
|
+
client = Ollama.new(
|
643
|
+
credentials: { address: 'http://localhost:11434' },
|
644
|
+
options: {
|
645
|
+
server_sent_events: true,
|
646
|
+
connection: { request: { timeout: 120, read_timeout: 120 } } }
|
647
|
+
)
|
648
|
+
```
|
649
|
+
|
650
|
+
Using the `generate` method:
|
651
|
+
|
652
|
+
```ruby
|
653
|
+
require 'base64'
|
654
|
+
|
655
|
+
client.generate(
|
656
|
+
{ model: 'llava',
|
657
|
+
prompt: 'Please describe this image.',
|
658
|
+
images: [Base64.strict_encode64(File.read('piano.jpg'))] }
|
659
|
+
) do |event, raw|
|
660
|
+
print event['response']
|
661
|
+
end
|
662
|
+
```
|
663
|
+
|
664
|
+
Output:
|
665
|
+
> _The image is a black and white photo of an old piano, which appears to be in need of maintenance. A chair is situated right next to the piano. Apart from that, there are no other objects or people visible in the scene._
|
666
|
+
|
667
|
+
Using the `chat` method:
|
668
|
+
```ruby
|
669
|
+
require 'base64'
|
670
|
+
|
671
|
+
result = client.chat(
|
672
|
+
{ model: 'llava',
|
673
|
+
messages: [
|
674
|
+
{ role: 'user',
|
675
|
+
content: 'Please describe this image.',
|
676
|
+
images: [Base64.strict_encode64(File.read('piano.jpg'))] }
|
677
|
+
] }
|
678
|
+
) do |event, raw|
|
679
|
+
puts event
|
680
|
+
end
|
681
|
+
```
|
682
|
+
|
683
|
+
Output:
|
684
|
+
> _The image displays an old piano, sitting on a wooden floor with black keys. Next to the piano, there is another keyboard in the scene, possibly used for playing music._
|
685
|
+
>
|
686
|
+
> _On top of the piano, there are two mice placed in different locations within its frame. These mice might be meant for controlling the music being played or simply as decorative items. The overall atmosphere seems to be focused on artistic expression through this unique instrument._
|
687
|
+
|
628
688
|
### Streaming and Server-Sent Events (SSE)
|
629
689
|
|
630
690
|
[Server-Sent Events (SSE)](https://en.wikipedia.org/wiki/Server-sent_events) is a technology that allows certain endpoints to offer streaming capabilities, such as creating the impression that "the model is typing along with you," rather than delivering the entire answer all at once.
|
@@ -640,7 +700,7 @@ client = Ollama.new(
|
|
640
700
|
Or, you can decide on a request basis:
|
641
701
|
```ruby
|
642
702
|
result = client.generate(
|
643
|
-
{ model: '
|
703
|
+
{ model: 'llama2',
|
644
704
|
prompt: 'Hi!' },
|
645
705
|
server_sent_events: true
|
646
706
|
) do |event, raw|
|
@@ -661,7 +721,7 @@ Ollama may launch a new endpoint that we haven't covered in the Gem yet. If that
|
|
661
721
|
```ruby
|
662
722
|
result = client.request(
|
663
723
|
'api/generate',
|
664
|
-
{ model: '
|
724
|
+
{ model: 'llama2',
|
665
725
|
prompt: 'Hi!' },
|
666
726
|
request_method: 'POST', server_sent_events: true
|
667
727
|
)
|
@@ -669,6 +729,21 @@ result = client.request(
|
|
669
729
|
|
670
730
|
### Request Options
|
671
731
|
|
732
|
+
#### Adapter
|
733
|
+
|
734
|
+
The gem uses [Faraday](https://github.com/lostisland/faraday) with the [Typhoeus](https://github.com/typhoeus/typhoeus) adapter by default.
|
735
|
+
|
736
|
+
You can use a different adapter if you want:
|
737
|
+
|
738
|
+
```ruby
|
739
|
+
require 'faraday/net_http'
|
740
|
+
|
741
|
+
client = Ollama.new(
|
742
|
+
credentials: { address: 'http://localhost:11434' },
|
743
|
+
options: { connection: { adapter: :net_http } }
|
744
|
+
)
|
745
|
+
```
|
746
|
+
|
672
747
|
#### Timeout
|
673
748
|
|
674
749
|
You can set the maximum number of seconds to wait for the request to complete with the `timeout` option:
|
@@ -707,7 +782,7 @@ require 'ollama-ai'
|
|
707
782
|
|
708
783
|
begin
|
709
784
|
client.chat_completions(
|
710
|
-
{ model: '
|
785
|
+
{ model: 'llama2',
|
711
786
|
prompt: 'Hi!' }
|
712
787
|
)
|
713
788
|
rescue Ollama::Errors::OllamaError => error
|
@@ -715,7 +790,7 @@ rescue Ollama::Errors::OllamaError => error
|
|
715
790
|
puts error.message # 'the server responded with status 500'
|
716
791
|
|
717
792
|
puts error.payload
|
718
|
-
# { model: '
|
793
|
+
# { model: 'llama2',
|
719
794
|
# prompt: 'Hi!',
|
720
795
|
# ...
|
721
796
|
# }
|
@@ -732,7 +807,7 @@ require 'ollama-ai/errors'
|
|
732
807
|
|
733
808
|
begin
|
734
809
|
client.chat_completions(
|
735
|
-
{ model: '
|
810
|
+
{ model: 'llama2',
|
736
811
|
prompt: 'Hi!' }
|
737
812
|
)
|
738
813
|
rescue OllamaError => error
|
@@ -755,6 +830,8 @@ RequestError
|
|
755
830
|
```bash
|
756
831
|
bundle
|
757
832
|
rubocop -A
|
833
|
+
|
834
|
+
bundle exec ruby spec/tasks/run-client.rb
|
758
835
|
```
|
759
836
|
|
760
837
|
### Purpose
|
@@ -768,7 +845,7 @@ gem build ollama-ai.gemspec
|
|
768
845
|
|
769
846
|
gem signin
|
770
847
|
|
771
|
-
gem push ollama-ai-1.
|
848
|
+
gem push ollama-ai-1.2.0.gem
|
772
849
|
```
|
773
850
|
|
774
851
|
### Updating the README
|