ollama-ai 1.0.0 → 1.0.1
Sign up to get free protection for your applications and to get access to all the features.
- checksums.yaml +4 -4
- data/Gemfile.lock +11 -11
- data/README.md +219 -154
- data/controllers/client.rb +3 -3
- data/ollama-ai.gemspec +1 -1
- data/static/gem.rb +1 -1
- data/template.md +216 -154
- metadata +4 -4
data/README.md
CHANGED
@@ -1,6 +1,6 @@
|
|
1
1
|
# Ollama AI
|
2
2
|
|
3
|
-
A Ruby gem for interacting with [Ollama](https://
|
3
|
+
A Ruby gem for interacting with [Ollama](https://ollama.ai)'s API that allows you to run open source AI LLMs (Large Language Models) locally.
|
4
4
|
|
5
5
|
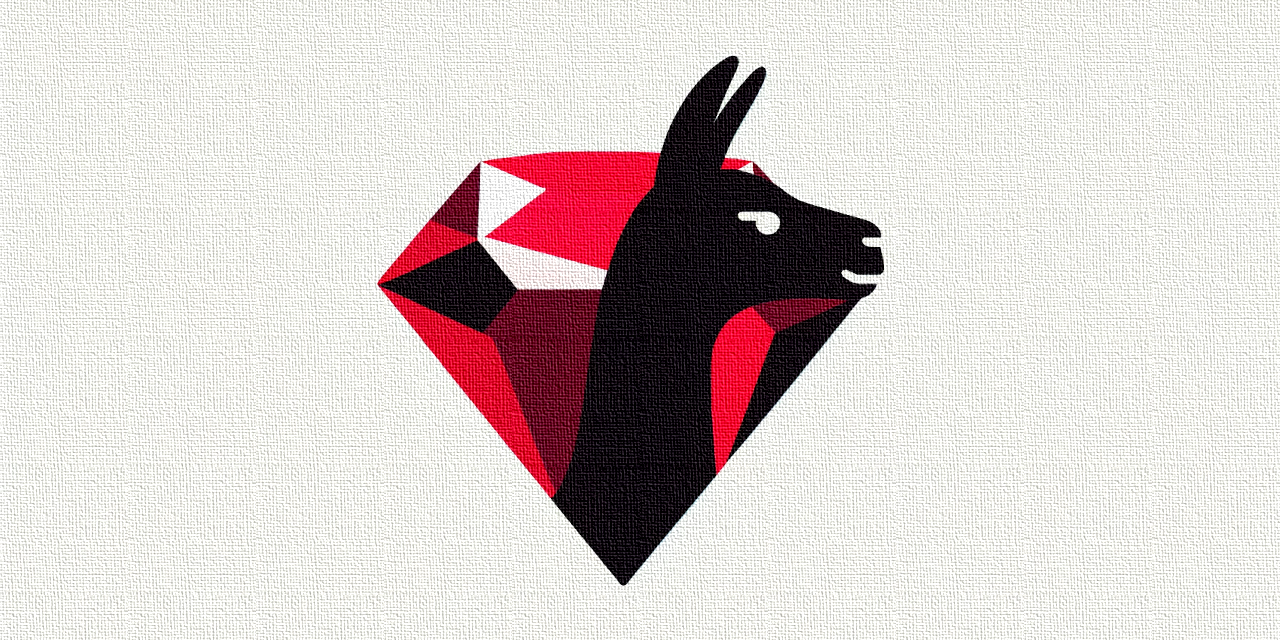
|
6
6
|
|
@@ -9,7 +9,7 @@ A Ruby gem for interacting with [Ollama](https://github.com/jmorganca/ollama)'s
|
|
9
9
|
## TL;DR and Quick Start
|
10
10
|
|
11
11
|
```ruby
|
12
|
-
gem 'ollama-ai', '~> 1.0.
|
12
|
+
gem 'ollama-ai', '~> 1.0.1'
|
13
13
|
```
|
14
14
|
|
15
15
|
```ruby
|
@@ -21,40 +21,40 @@ client = Ollama.new(
|
|
21
21
|
)
|
22
22
|
|
23
23
|
result = client.generate(
|
24
|
-
{ model: '
|
24
|
+
{ model: 'llama2',
|
25
25
|
prompt: 'Hi!' }
|
26
26
|
)
|
27
27
|
```
|
28
28
|
|
29
29
|
Result:
|
30
30
|
```ruby
|
31
|
-
[{ 'model' => '
|
32
|
-
'created_at' => '2024-01-
|
31
|
+
[{ 'model' => 'llama2',
|
32
|
+
'created_at' => '2024-01-07T01:34:02.088810408Z',
|
33
33
|
'response' => 'Hello',
|
34
34
|
'done' => false },
|
35
|
-
{ 'model' => '
|
36
|
-
'created_at' => '2024-01-
|
35
|
+
{ 'model' => 'llama2',
|
36
|
+
'created_at' => '2024-01-07T01:34:02.419045606Z',
|
37
37
|
'response' => '!',
|
38
38
|
'done' => false },
|
39
|
-
#
|
40
|
-
{ 'model' => '
|
41
|
-
'created_at' => '2024-01-
|
42
|
-
'response' => '
|
39
|
+
# ..
|
40
|
+
{ 'model' => 'llama2',
|
41
|
+
'created_at' => '2024-01-07T01:34:07.680049831Z',
|
42
|
+
'response' => '?',
|
43
43
|
'done' => false },
|
44
|
-
{ 'model' => '
|
45
|
-
'created_at' => '2024-01-
|
44
|
+
{ 'model' => 'llama2',
|
45
|
+
'created_at' => '2024-01-07T01:34:07.872170352Z',
|
46
46
|
'response' => '',
|
47
47
|
'done' => true,
|
48
48
|
'context' =>
|
49
|
-
[
|
49
|
+
[518, 25_580,
|
50
50
|
# ...
|
51
|
-
|
52
|
-
'total_duration' =>
|
53
|
-
'load_duration' =>
|
54
|
-
'prompt_eval_count' =>
|
55
|
-
'prompt_eval_duration' =>
|
56
|
-
'eval_count' =>
|
57
|
-
'eval_duration' =>
|
51
|
+
13_563, 29_973],
|
52
|
+
'total_duration' => 11_653_781_127,
|
53
|
+
'load_duration' => 1_186_200_439,
|
54
|
+
'prompt_eval_count' => 22,
|
55
|
+
'prompt_eval_duration' => 5_006_751_000,
|
56
|
+
'eval_count' => 25,
|
57
|
+
'eval_duration' => 5_453_058_000 }]
|
58
58
|
```
|
59
59
|
|
60
60
|
## Index
|
@@ -80,6 +80,9 @@ Result:
|
|
80
80
|
- [delete: Delete a Model](#delete-delete-a-model)
|
81
81
|
- [pull: Pull a Model](#pull-pull-a-model)
|
82
82
|
- [push: Push a Model](#push-push-a-model)
|
83
|
+
- [Modes](#modes)
|
84
|
+
- [Text](#text)
|
85
|
+
- [Image](#image)
|
83
86
|
- [Streaming and Server-Sent Events (SSE)](#streaming-and-server-sent-events-sse)
|
84
87
|
- [Server-Sent Events (SSE) Hang](#server-sent-events-sse-hang)
|
85
88
|
- [New Functionalities and APIs](#new-functionalities-and-apis)
|
@@ -101,11 +104,11 @@ Result:
|
|
101
104
|
### Installing
|
102
105
|
|
103
106
|
```sh
|
104
|
-
gem install ollama-ai -v 1.0.
|
107
|
+
gem install ollama-ai -v 1.0.1
|
105
108
|
```
|
106
109
|
|
107
110
|
```sh
|
108
|
-
gem 'ollama-ai', '~> 1.0.
|
111
|
+
gem 'ollama-ai', '~> 1.0.1'
|
109
112
|
```
|
110
113
|
|
111
114
|
## Usage
|
@@ -148,7 +151,7 @@ API Documentation: https://github.com/jmorganca/ollama/blob/main/docs/api.md#gen
|
|
148
151
|
|
149
152
|
```ruby
|
150
153
|
result = client.generate(
|
151
|
-
{ model: '
|
154
|
+
{ model: 'llama2',
|
152
155
|
prompt: 'Hi!',
|
153
156
|
stream: false }
|
154
157
|
)
|
@@ -156,21 +159,20 @@ result = client.generate(
|
|
156
159
|
|
157
160
|
Result:
|
158
161
|
```ruby
|
159
|
-
[{ 'model' => '
|
160
|
-
'created_at' => '2024-01-
|
161
|
-
'response' =>
|
162
|
-
"Hello! How can I assist you today? Do you have any questions or problems that you'd like help with?",
|
162
|
+
[{ 'model' => 'llama2',
|
163
|
+
'created_at' => '2024-01-07T01:35:41.951371247Z',
|
164
|
+
'response' => "Hi there! It's nice to meet you. How are you today?",
|
163
165
|
'done' => true,
|
164
166
|
'context' =>
|
165
|
-
[
|
167
|
+
[518, 25_580,
|
166
168
|
# ...
|
167
|
-
|
168
|
-
'total_duration' =>
|
169
|
-
'load_duration' =>
|
170
|
-
'prompt_eval_count' =>
|
171
|
-
'prompt_eval_duration' =>
|
172
|
-
'eval_count' =>
|
173
|
-
'eval_duration' =>
|
169
|
+
9826, 29_973],
|
170
|
+
'total_duration' => 6_981_097_576,
|
171
|
+
'load_duration' => 625_053,
|
172
|
+
'prompt_eval_count' => 22,
|
173
|
+
'prompt_eval_duration' => 4_075_171_000,
|
174
|
+
'eval_count' => 16,
|
175
|
+
'eval_duration' => 2_900_325_000 }]
|
174
176
|
```
|
175
177
|
|
176
178
|
##### Receiving Stream Events
|
@@ -181,7 +183,7 @@ Ensure that you have enabled [Server-Sent Events](#streaming-and-server-sent-eve
|
|
181
183
|
|
182
184
|
```ruby
|
183
185
|
client.generate(
|
184
|
-
{ model: '
|
186
|
+
{ model: 'llama2',
|
185
187
|
prompt: 'Hi!' }
|
186
188
|
) do |event, raw|
|
187
189
|
puts event
|
@@ -190,8 +192,8 @@ end
|
|
190
192
|
|
191
193
|
Event:
|
192
194
|
```ruby
|
193
|
-
{ 'model' => '
|
194
|
-
'created_at' => '2024-01-
|
195
|
+
{ 'model' => 'llama2',
|
196
|
+
'created_at' => '2024-01-07T01:36:30.665245712Z',
|
195
197
|
'response' => 'Hello',
|
196
198
|
'done' => false }
|
197
199
|
```
|
@@ -199,46 +201,46 @@ Event:
|
|
199
201
|
You can get all the receive events at once as an array:
|
200
202
|
```ruby
|
201
203
|
result = client.generate(
|
202
|
-
{ model: '
|
204
|
+
{ model: 'llama2',
|
203
205
|
prompt: 'Hi!' }
|
204
206
|
)
|
205
207
|
```
|
206
208
|
|
207
209
|
Result:
|
208
210
|
```ruby
|
209
|
-
[{ 'model' => '
|
210
|
-
'created_at' => '2024-01-
|
211
|
+
[{ 'model' => 'llama2',
|
212
|
+
'created_at' => '2024-01-07T01:36:30.665245712Z',
|
211
213
|
'response' => 'Hello',
|
212
214
|
'done' => false },
|
213
|
-
{ 'model' => '
|
214
|
-
'created_at' => '2024-01-
|
215
|
+
{ 'model' => 'llama2',
|
216
|
+
'created_at' => '2024-01-07T01:36:30.927337136Z',
|
215
217
|
'response' => '!',
|
216
218
|
'done' => false },
|
217
219
|
# ...
|
218
|
-
{ 'model' => '
|
219
|
-
'created_at' => '2024-01-
|
220
|
-
'response' => '
|
220
|
+
{ 'model' => 'llama2',
|
221
|
+
'created_at' => '2024-01-07T01:36:37.249416767Z',
|
222
|
+
'response' => '?',
|
221
223
|
'done' => false },
|
222
|
-
{ 'model' => '
|
223
|
-
'created_at' => '2024-01-
|
224
|
+
{ 'model' => 'llama2',
|
225
|
+
'created_at' => '2024-01-07T01:36:37.44041283Z',
|
224
226
|
'response' => '',
|
225
227
|
'done' => true,
|
226
228
|
'context' =>
|
227
|
-
[
|
229
|
+
[518, 25_580,
|
228
230
|
# ...
|
229
|
-
|
230
|
-
'total_duration' =>
|
231
|
-
'load_duration' =>
|
232
|
-
'prompt_eval_count' =>
|
233
|
-
'prompt_eval_duration' =>
|
234
|
-
'eval_count' =>
|
235
|
-
'eval_duration' =>
|
231
|
+
13_563, 29_973],
|
232
|
+
'total_duration' => 10_551_395_645,
|
233
|
+
'load_duration' => 966_631,
|
234
|
+
'prompt_eval_count' => 22,
|
235
|
+
'prompt_eval_duration' => 4_034_990_000,
|
236
|
+
'eval_count' => 25,
|
237
|
+
'eval_duration' => 6_512_954_000 }]
|
236
238
|
```
|
237
239
|
|
238
240
|
You can mix both as well:
|
239
241
|
```ruby
|
240
242
|
result = client.generate(
|
241
|
-
{ model: '
|
243
|
+
{ model: 'llama2',
|
242
244
|
prompt: 'Hi!' }
|
243
245
|
) do |event, raw|
|
244
246
|
puts event
|
@@ -251,7 +253,7 @@ API Documentation: https://github.com/jmorganca/ollama/blob/main/docs/api.md#gen
|
|
251
253
|
|
252
254
|
```ruby
|
253
255
|
result = client.chat(
|
254
|
-
{ model: '
|
256
|
+
{ model: 'llama2',
|
255
257
|
messages: [
|
256
258
|
{ role: 'user', content: 'Hi! My name is Purple.' }
|
257
259
|
] }
|
@@ -262,37 +264,37 @@ end
|
|
262
264
|
|
263
265
|
Event:
|
264
266
|
```ruby
|
265
|
-
{ 'model' => '
|
266
|
-
'created_at' => '2024-01-
|
267
|
-
'message' => { 'role' => 'assistant', 'content' =>
|
267
|
+
{ 'model' => 'llama2',
|
268
|
+
'created_at' => '2024-01-07T01:38:01.729897311Z',
|
269
|
+
'message' => { 'role' => 'assistant', 'content' => "\n" },
|
268
270
|
'done' => false }
|
269
271
|
```
|
270
272
|
|
271
273
|
Result:
|
272
274
|
```ruby
|
273
|
-
[{ 'model' => '
|
274
|
-
'created_at' => '2024-01-
|
275
|
-
'message' => { 'role' => 'assistant', 'content' =>
|
275
|
+
[{ 'model' => 'llama2',
|
276
|
+
'created_at' => '2024-01-07T01:38:01.729897311Z',
|
277
|
+
'message' => { 'role' => 'assistant', 'content' => "\n" },
|
276
278
|
'done' => false },
|
277
|
-
{ 'model' => '
|
278
|
-
'created_at' => '2024-01-
|
279
|
-
'message' => { 'role' => 'assistant', 'content' => '
|
279
|
+
{ 'model' => 'llama2',
|
280
|
+
'created_at' => '2024-01-07T01:38:02.081494506Z',
|
281
|
+
'message' => { 'role' => 'assistant', 'content' => '*' },
|
280
282
|
'done' => false },
|
281
283
|
# ...
|
282
|
-
{ 'model' => '
|
283
|
-
'created_at' => '2024-01-
|
284
|
+
{ 'model' => 'llama2',
|
285
|
+
'created_at' => '2024-01-07T01:38:17.855905499Z',
|
284
286
|
'message' => { 'role' => 'assistant', 'content' => '?' },
|
285
287
|
'done' => false },
|
286
|
-
{ 'model' => '
|
287
|
-
'created_at' => '2024-01-
|
288
|
+
{ 'model' => 'llama2',
|
289
|
+
'created_at' => '2024-01-07T01:38:18.07331245Z',
|
288
290
|
'message' => { 'role' => 'assistant', 'content' => '' },
|
289
291
|
'done' => true,
|
290
|
-
'total_duration' =>
|
291
|
-
'load_duration' =>
|
292
|
-
'prompt_eval_count' =>
|
293
|
-
'prompt_eval_duration' =>
|
294
|
-
'eval_count' =>
|
295
|
-
'eval_duration' =>
|
292
|
+
'total_duration' => 22_494_544_502,
|
293
|
+
'load_duration' => 4_224_600,
|
294
|
+
'prompt_eval_count' => 28,
|
295
|
+
'prompt_eval_duration' => 6_496_583_000,
|
296
|
+
'eval_count' => 61,
|
297
|
+
'eval_duration' => 15_991_728_000 }]
|
296
298
|
```
|
297
299
|
|
298
300
|
##### Back-and-Forth Conversations
|
@@ -303,11 +305,11 @@ To maintain a back-and-forth conversation, you need to append the received respo
|
|
303
305
|
|
304
306
|
```ruby
|
305
307
|
result = client.chat(
|
306
|
-
{ model: '
|
308
|
+
{ model: 'llama2',
|
307
309
|
messages: [
|
308
310
|
{ role: 'user', content: 'Hi! My name is Purple.' },
|
309
311
|
{ role: 'assistant',
|
310
|
-
content:
|
312
|
+
content: 'Hi, Purple!' },
|
311
313
|
{ role: 'user', content: "What's my name?" }
|
312
314
|
] }
|
313
315
|
) do |event, raw|
|
@@ -318,37 +320,41 @@ end
|
|
318
320
|
Event:
|
319
321
|
|
320
322
|
```ruby
|
321
|
-
{ 'model' => '
|
322
|
-
'created_at' => '2024-01-
|
323
|
-
'message' => { 'role' => 'assistant', 'content' => '
|
323
|
+
{ 'model' => 'llama2',
|
324
|
+
'created_at' => '2024-01-07T01:40:07.352998498Z',
|
325
|
+
'message' => { 'role' => 'assistant', 'content' => ' Pur' },
|
324
326
|
'done' => false }
|
325
327
|
```
|
326
328
|
|
327
329
|
Result:
|
328
330
|
```ruby
|
329
|
-
[{ 'model' => '
|
330
|
-
'created_at' => '2024-01-
|
331
|
+
[{ 'model' => 'llama2',
|
332
|
+
'created_at' => '2024-01-07T01:40:06.562939469Z',
|
331
333
|
'message' => { 'role' => 'assistant', 'content' => 'Your' },
|
332
334
|
'done' => false },
|
333
|
-
{ 'model' => 'dolphin-phi',
|
334
|
-
'created_at' => '2024-01-06T19:07:51.184476541Z',
|
335
|
-
'message' => { 'role' => 'assistant', 'content' => ' name' },
|
336
|
-
'done' => false },
|
337
335
|
# ...
|
338
|
-
{ 'model' => '
|
339
|
-
'created_at' => '2024-01-
|
340
|
-
'message' => { 'role' => 'assistant', 'content' => '
|
336
|
+
{ 'model' => 'llama2',
|
337
|
+
'created_at' => '2024-01-07T01:40:07.352998498Z',
|
338
|
+
'message' => { 'role' => 'assistant', 'content' => ' Pur' },
|
339
|
+
'done' => false },
|
340
|
+
{ 'model' => 'llama2',
|
341
|
+
'created_at' => '2024-01-07T01:40:07.545323584Z',
|
342
|
+
'message' => { 'role' => 'assistant', 'content' => 'ple' },
|
341
343
|
'done' => false },
|
342
|
-
{ 'model' => '
|
343
|
-
'created_at' => '2024-01-
|
344
|
+
{ 'model' => 'llama2',
|
345
|
+
'created_at' => '2024-01-07T01:40:07.77769408Z',
|
346
|
+
'message' => { 'role' => 'assistant', 'content' => '!' },
|
347
|
+
'done' => false },
|
348
|
+
{ 'model' => 'llama2',
|
349
|
+
'created_at' => '2024-01-07T01:40:07.974165849Z',
|
344
350
|
'message' => { 'role' => 'assistant', 'content' => '' },
|
345
351
|
'done' => true,
|
346
|
-
'total_duration' =>
|
347
|
-
'load_duration' =>
|
348
|
-
'prompt_eval_count' =>
|
349
|
-
'prompt_eval_duration' =>
|
350
|
-
'eval_count' =>
|
351
|
-
'eval_duration' =>
|
352
|
+
'total_duration' => 11_482_012_681,
|
353
|
+
'load_duration' => 4_246_882,
|
354
|
+
'prompt_eval_count' => 57,
|
355
|
+
'prompt_eval_duration' => 10_387_150_000,
|
356
|
+
'eval_count' => 6,
|
357
|
+
'eval_duration' => 1_089_249_000 }]
|
352
358
|
```
|
353
359
|
|
354
360
|
#### embeddings: Generate Embeddings
|
@@ -357,7 +363,7 @@ API Documentation: https://github.com/jmorganca/ollama/blob/main/docs/api.md#gen
|
|
357
363
|
|
358
364
|
```ruby
|
359
365
|
result = client.embeddings(
|
360
|
-
{ model: '
|
366
|
+
{ model: 'llama2',
|
361
367
|
prompt: 'Hi!' }
|
362
368
|
)
|
363
369
|
```
|
@@ -365,11 +371,9 @@ result = client.embeddings(
|
|
365
371
|
Result:
|
366
372
|
```ruby
|
367
373
|
[{ 'embedding' =>
|
368
|
-
[
|
369
|
-
1.0635842084884644,
|
374
|
+
[0.6970467567443848, -2.248202085494995,
|
370
375
|
# ...
|
371
|
-
-0.
|
372
|
-
0.051569778472185135] }]
|
376
|
+
-1.5994540452957153, -0.3464218080043793] }]
|
373
377
|
```
|
374
378
|
|
375
379
|
#### Models
|
@@ -381,7 +385,7 @@ API Documentation: https://github.com/jmorganca/ollama/blob/main/docs/api.md#cre
|
|
381
385
|
```ruby
|
382
386
|
result = client.create(
|
383
387
|
{ name: 'mario',
|
384
|
-
modelfile: "FROM
|
388
|
+
modelfile: "FROM llama2\nSYSTEM You are mario from Super Mario Bros." }
|
385
389
|
) do |event, raw|
|
386
390
|
puts event
|
387
391
|
end
|
@@ -422,9 +426,7 @@ client.generate(
|
|
422
426
|
end
|
423
427
|
```
|
424
428
|
|
425
|
-
>
|
426
|
-
>
|
427
|
-
> _What brings you here? How can I help you on your journey?_
|
429
|
+
> _Woah! *adjusts sunglasses* It's-a me, Mario! *winks* You must be a new friend I've-a met here in the Mushroom Kingdom. *tips top hat* What brings you to this neck of the woods? Maybe you're looking for-a some help on your adventure? *nods* Just let me know, and I'll do my best to-a assist ya! 😃_
|
428
430
|
|
429
431
|
##### tags: List Local Models
|
430
432
|
|
@@ -437,28 +439,28 @@ result = client.tags
|
|
437
439
|
Result:
|
438
440
|
```ruby
|
439
441
|
[{ 'models' =>
|
440
|
-
[{ 'name' => '
|
441
|
-
'modified_at' => '2024-01-
|
442
|
-
'size' =>
|
442
|
+
[{ 'name' => 'llama2:latest',
|
443
|
+
'modified_at' => '2024-01-06T15:06:23.6349195-03:00',
|
444
|
+
'size' => 3_826_793_677,
|
443
445
|
'digest' =>
|
444
|
-
|
446
|
+
'78e26419b4469263f75331927a00a0284ef6544c1975b826b15abdaef17bb962',
|
445
447
|
'details' =>
|
446
|
-
|
447
|
-
|
448
|
-
|
449
|
-
|
450
|
-
|
448
|
+
{ 'format' => 'gguf',
|
449
|
+
'family' => 'llama',
|
450
|
+
'families' => ['llama'],
|
451
|
+
'parameter_size' => '7B',
|
452
|
+
'quantization_level' => 'Q4_0' } },
|
451
453
|
{ 'name' => 'mario:latest',
|
452
|
-
'modified_at' => '2024-01-
|
453
|
-
'size' =>
|
454
|
+
'modified_at' => '2024-01-06T22:41:59.495298101-03:00',
|
455
|
+
'size' => 3_826_793_787,
|
454
456
|
'digest' =>
|
455
|
-
|
457
|
+
'291f46d2fa687dfaff45de96a8cb6e32707bc16ec1e1dfe8d65e9634c34c660c',
|
456
458
|
'details' =>
|
457
|
-
|
458
|
-
|
459
|
-
|
460
|
-
|
461
|
-
|
459
|
+
{ 'format' => 'gguf',
|
460
|
+
'family' => 'llama',
|
461
|
+
'families' => ['llama'],
|
462
|
+
'parameter_size' => '7B',
|
463
|
+
'quantization_level' => 'Q4_0' } }] }]
|
462
464
|
```
|
463
465
|
|
464
466
|
##### show: Show Model Information
|
@@ -467,35 +469,33 @@ API Documentation: https://github.com/jmorganca/ollama/blob/main/docs/api.md#sho
|
|
467
469
|
|
468
470
|
```ruby
|
469
471
|
result = client.show(
|
470
|
-
{ name: '
|
472
|
+
{ name: 'llama2' }
|
471
473
|
)
|
472
474
|
```
|
473
475
|
|
474
476
|
Result:
|
475
477
|
```ruby
|
476
478
|
[{ 'license' =>
|
477
|
-
"
|
479
|
+
"LLAMA 2 COMMUNITY LICENSE AGREEMENT\t\n" \
|
478
480
|
# ...
|
479
|
-
|
481
|
+
"* Reporting violations of the Acceptable Use Policy or unlicensed uses of Llama..." \
|
482
|
+
"\n",
|
480
483
|
'modelfile' =>
|
481
484
|
"# Modelfile generated by \"ollama show\"\n" \
|
482
485
|
# ...
|
483
|
-
'PARAMETER stop "
|
486
|
+
'PARAMETER stop "<</SYS>>"',
|
484
487
|
'parameters' =>
|
485
|
-
"stop
|
486
|
-
|
487
|
-
|
488
|
-
|
489
|
-
|
490
|
-
"
|
491
|
-
"{{ .Prompt }}<|im_end|>\n" \
|
492
|
-
"<|im_start|>assistant\n",
|
493
|
-
'system' => 'You are Dolphin, a helpful AI assistant.',
|
488
|
+
"stop [INST]\n" \
|
489
|
+
"stop [/INST]\n" \
|
490
|
+
"stop <<SYS>>\n" \
|
491
|
+
'stop <</SYS>>',
|
492
|
+
'template' =>
|
493
|
+
"[INST] <<SYS>>{{ .System }}<</SYS>>\n\n{{ .Prompt }} [/INST]\n",
|
494
494
|
'details' =>
|
495
495
|
{ 'format' => 'gguf',
|
496
|
-
'family' => '
|
497
|
-
'families' => ['
|
498
|
-
'parameter_size' => '
|
496
|
+
'family' => 'llama',
|
497
|
+
'families' => ['llama'],
|
498
|
+
'parameter_size' => '7B',
|
499
499
|
'quantization_level' => 'Q4_0' } }]
|
500
500
|
```
|
501
501
|
|
@@ -505,8 +505,8 @@ API Documentation: https://github.com/jmorganca/ollama/blob/main/docs/api.md#cop
|
|
505
505
|
|
506
506
|
```ruby
|
507
507
|
result = client.copy(
|
508
|
-
{ source: '
|
509
|
-
destination: '
|
508
|
+
{ source: 'llama2',
|
509
|
+
destination: 'llama2-backup' }
|
510
510
|
)
|
511
511
|
```
|
512
512
|
|
@@ -543,7 +543,7 @@ API Documentation: https://github.com/jmorganca/ollama/blob/main/docs/api.md#del
|
|
543
543
|
|
544
544
|
```ruby
|
545
545
|
result = client.delete(
|
546
|
-
{ name: '
|
546
|
+
{ name: 'llama2' }
|
547
547
|
)
|
548
548
|
```
|
549
549
|
|
@@ -556,14 +556,14 @@ If the model does not exist:
|
|
556
556
|
```ruby
|
557
557
|
begin
|
558
558
|
result = client.delete(
|
559
|
-
{ name: '
|
559
|
+
{ name: 'llama2' }
|
560
560
|
)
|
561
561
|
rescue Ollama::Errors::OllamaError => error
|
562
562
|
puts error.class # Ollama::Errors::RequestError
|
563
563
|
puts error.message # 'the server responded with status 404'
|
564
564
|
|
565
565
|
puts error.payload
|
566
|
-
# { name: '
|
566
|
+
# { name: 'llama2',
|
567
567
|
# ...
|
568
568
|
# }
|
569
569
|
|
@@ -578,7 +578,7 @@ API Documentation: https://github.com/jmorganca/ollama/blob/main/docs/api.md#pul
|
|
578
578
|
|
579
579
|
```ruby
|
580
580
|
result = client.pull(
|
581
|
-
{ name: '
|
581
|
+
{ name: 'llama2' }
|
582
582
|
) do |event, raw|
|
583
583
|
puts event
|
584
584
|
end
|
@@ -660,6 +660,69 @@ Result:
|
|
660
660
|
{ 'status' => 'success' }]
|
661
661
|
```
|
662
662
|
|
663
|
+
### Modes
|
664
|
+
|
665
|
+
#### Text
|
666
|
+
|
667
|
+
You can use the [generate](#generate-generate-a-completion) or [chat](#chat-generate-a-chat-completion) methods for text.
|
668
|
+
|
669
|
+
#### Image
|
670
|
+
|
671
|
+
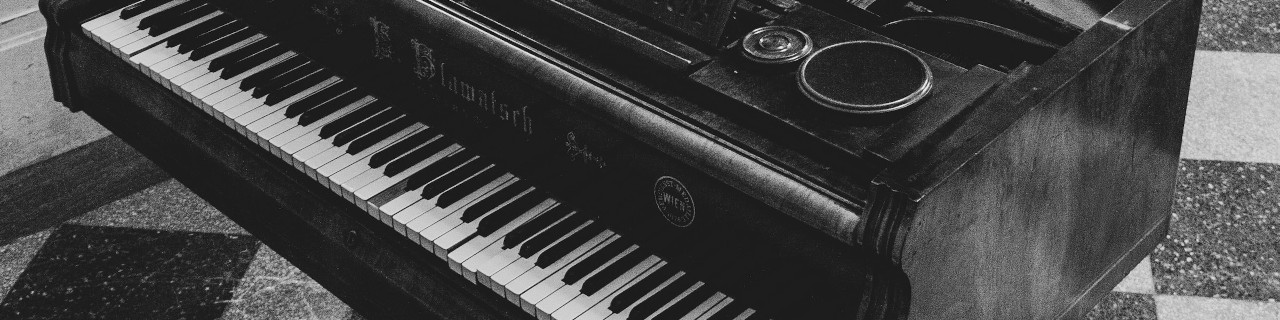
|
672
|
+
|
673
|
+
> _Courtesy of [Unsplash](https://unsplash.com/photos/greyscale-photo-of-grand-piano-czPs0z3-Ggg)_
|
674
|
+
|
675
|
+
You need to choose a model that supports images, like [LLaVA](https://ollama.ai/library/llava) or [bakllava](https://ollama.ai/library/bakllava), and encode the image as [Base64](https://en.wikipedia.org/wiki/Base64).
|
676
|
+
|
677
|
+
Depending on your hardware, some models that support images can be slow, so you may want to increase the client [timeout](#timeout):
|
678
|
+
|
679
|
+
```ruby
|
680
|
+
client = Ollama.new(
|
681
|
+
credentials: { address: 'http://localhost:11434' },
|
682
|
+
options: {
|
683
|
+
server_sent_events: true,
|
684
|
+
connection: { request: { timeout: 120, read_timeout: 120 } } }
|
685
|
+
)
|
686
|
+
```
|
687
|
+
|
688
|
+
Using the `generate` method:
|
689
|
+
|
690
|
+
```ruby
|
691
|
+
require 'base64'
|
692
|
+
|
693
|
+
client.generate(
|
694
|
+
{ model: 'llava',
|
695
|
+
prompt: 'Please describe this image.',
|
696
|
+
images: [Base64.strict_encode64(File.read('piano.jpg'))] }
|
697
|
+
) do |event, raw|
|
698
|
+
print event['response']
|
699
|
+
end
|
700
|
+
```
|
701
|
+
|
702
|
+
Output:
|
703
|
+
> _The image is a black and white photo of an old piano, which appears to be in need of maintenance. A chair is situated right next to the piano. Apart from that, there are no other objects or people visible in the scene._
|
704
|
+
|
705
|
+
Using the `chat` method:
|
706
|
+
```ruby
|
707
|
+
require 'base64'
|
708
|
+
|
709
|
+
result = client.chat(
|
710
|
+
{ model: 'llava',
|
711
|
+
messages: [
|
712
|
+
{ role: 'user',
|
713
|
+
content: 'Please describe this image.',
|
714
|
+
images: [Base64.strict_encode64(File.read('piano.jpg'))] }
|
715
|
+
] }
|
716
|
+
) do |event, raw|
|
717
|
+
puts event
|
718
|
+
end
|
719
|
+
```
|
720
|
+
|
721
|
+
Output:
|
722
|
+
> _The image displays an old piano, sitting on a wooden floor with black keys. Next to the piano, there is another keyboard in the scene, possibly used for playing music._
|
723
|
+
>
|
724
|
+
> _On top of the piano, there are two mice placed in different locations within its frame. These mice might be meant for controlling the music being played or simply as decorative items. The overall atmosphere seems to be focused on artistic expression through this unique instrument._
|
725
|
+
|
663
726
|
### Streaming and Server-Sent Events (SSE)
|
664
727
|
|
665
728
|
[Server-Sent Events (SSE)](https://en.wikipedia.org/wiki/Server-sent_events) is a technology that allows certain endpoints to offer streaming capabilities, such as creating the impression that "the model is typing along with you," rather than delivering the entire answer all at once.
|
@@ -675,7 +738,7 @@ client = Ollama.new(
|
|
675
738
|
Or, you can decide on a request basis:
|
676
739
|
```ruby
|
677
740
|
result = client.generate(
|
678
|
-
{ model: '
|
741
|
+
{ model: 'llama2',
|
679
742
|
prompt: 'Hi!' },
|
680
743
|
server_sent_events: true
|
681
744
|
) do |event, raw|
|
@@ -696,7 +759,7 @@ Ollama may launch a new endpoint that we haven't covered in the Gem yet. If that
|
|
696
759
|
```ruby
|
697
760
|
result = client.request(
|
698
761
|
'api/generate',
|
699
|
-
{ model: '
|
762
|
+
{ model: 'llama2',
|
700
763
|
prompt: 'Hi!' },
|
701
764
|
request_method: 'POST', server_sent_events: true
|
702
765
|
)
|
@@ -742,7 +805,7 @@ require 'ollama-ai'
|
|
742
805
|
|
743
806
|
begin
|
744
807
|
client.chat_completions(
|
745
|
-
{ model: '
|
808
|
+
{ model: 'llama2',
|
746
809
|
prompt: 'Hi!' }
|
747
810
|
)
|
748
811
|
rescue Ollama::Errors::OllamaError => error
|
@@ -750,7 +813,7 @@ rescue Ollama::Errors::OllamaError => error
|
|
750
813
|
puts error.message # 'the server responded with status 500'
|
751
814
|
|
752
815
|
puts error.payload
|
753
|
-
# { model: '
|
816
|
+
# { model: 'llama2',
|
754
817
|
# prompt: 'Hi!',
|
755
818
|
# ...
|
756
819
|
# }
|
@@ -767,7 +830,7 @@ require 'ollama-ai/errors'
|
|
767
830
|
|
768
831
|
begin
|
769
832
|
client.chat_completions(
|
770
|
-
{ model: '
|
833
|
+
{ model: 'llama2',
|
771
834
|
prompt: 'Hi!' }
|
772
835
|
)
|
773
836
|
rescue OllamaError => error
|
@@ -790,6 +853,8 @@ RequestError
|
|
790
853
|
```bash
|
791
854
|
bundle
|
792
855
|
rubocop -A
|
856
|
+
|
857
|
+
bundle exec ruby spec/tasks/run-client.rb
|
793
858
|
```
|
794
859
|
|
795
860
|
### Purpose
|
@@ -803,7 +868,7 @@ gem build ollama-ai.gemspec
|
|
803
868
|
|
804
869
|
gem signin
|
805
870
|
|
806
|
-
gem push ollama-ai-1.0.
|
871
|
+
gem push ollama-ai-1.0.1.gem
|
807
872
|
```
|
808
873
|
|
809
874
|
### Updating the README
|