multirb 0.0.3 → 0.0.5
Sign up to get free protection for your applications and to get access to all the features.
- data/README.md +7 -4
- data/lib/multirb.rb +42 -5
- data/lib/multirb/version.rb +1 -1
- data/multirb.gemspec +2 -2
- data/test/test_multirb.rb +1 -0
- metadata +6 -6
data/README.md
CHANGED
@@ -1,6 +1,6 @@
|
|
1
1
|
# multirb
|
2
2
|
|
3
|
-
Runs an IRB-esque prompt (but it's NOT really IRB!) over multiple Rubies using RVM.
|
3
|
+
Runs an IRB-esque prompt (but it's NOT really IRB!) over multiple Rubies using RVM or RBENV.
|
4
4
|
|
5
5
|
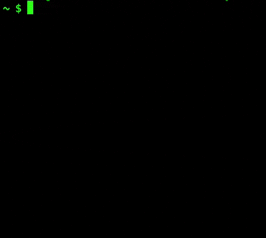
|
6
6
|
|
@@ -18,7 +18,7 @@ Install with:
|
|
18
18
|
|
19
19
|
$ gem install multirb
|
20
20
|
|
21
|
-
You also need a complete, working [RVM](https://rvm.io/) installation. Feel free to submit pull requests to make this auto detect other Ruby version managers
|
21
|
+
You also need a complete, working [RVM](https://rvm.io/) or [RBENV](https://github.com/sstephenson/rbenv/) installation. Feel free to submit pull requests to make this auto detect other Ruby version managers.
|
22
22
|
|
23
23
|
Note: multirb works fine from MRI Ruby 1.9 and JRuby 1.7.x but has not been tested elsewhere yet.
|
24
24
|
|
@@ -44,10 +44,13 @@ Currently specifies jruby, 1.8.7, 1.9.2, 1.9.3, and 2.0.0 as 'all versions'; 1.8
|
|
44
44
|
|
45
45
|
## Versions
|
46
46
|
|
47
|
-
0.0.
|
47
|
+
0.0.5 (current gem release):
|
48
|
+
* fixes a Ruby version detection bug triggered by interpolation (found by dalton)
|
49
|
+
|
50
|
+
0.0.4:
|
48
51
|
* rbenv support
|
49
52
|
|
50
|
-
0.0.3
|
53
|
+
0.0.3:
|
51
54
|
* acts nicely when using Ctrl+D to exit rather than raising a needless error
|
52
55
|
* uses Ruby 2.0.0-rc2 as a default
|
53
56
|
|
data/lib/multirb.rb
CHANGED
@@ -5,8 +5,10 @@ require 'readline'
|
|
5
5
|
require 'tempfile'
|
6
6
|
|
7
7
|
module Multirb
|
8
|
-
ALL_VERSIONS = %w{
|
9
|
-
DEFAULT_VERSIONS = %w{1.8.7 1.9.3 2.0.0
|
8
|
+
ALL_VERSIONS = %w{1.8.7 1.9.2 1.9.3 2.0.0 jruby}
|
9
|
+
DEFAULT_VERSIONS = %w{1.8.7 1.9.3 2.0.0}
|
10
|
+
|
11
|
+
RBENV_INSTALLED_VERSIONS = `rbenv versions`.split("\n").map { |version| version.delete('*').strip.split.first } if ENV['PATH']['.rbenv']
|
10
12
|
|
11
13
|
def read_lines
|
12
14
|
lines = []
|
@@ -27,7 +29,7 @@ module Multirb
|
|
27
29
|
case lines.last
|
28
30
|
when /\#\s?all$/
|
29
31
|
ALL_VERSIONS
|
30
|
-
when /\#\s?(
|
32
|
+
when /\#\s?([^"']+)$/
|
31
33
|
[*$1.strip.split(',').map(&:strip)]
|
32
34
|
else
|
33
35
|
defaults
|
@@ -60,7 +62,42 @@ module Multirb
|
|
60
62
|
f
|
61
63
|
end
|
62
64
|
|
63
|
-
def run_code(
|
65
|
+
def run_code(*args)
|
66
|
+
case installed_ruby_version_manager
|
67
|
+
when :rvm
|
68
|
+
run_rvm(*args)
|
69
|
+
when :rbenv
|
70
|
+
run_rbenv(*args)
|
71
|
+
when :none
|
72
|
+
raise "You don't have either RVM or RBENV installed"
|
73
|
+
end
|
74
|
+
end
|
75
|
+
|
76
|
+
private
|
77
|
+
|
78
|
+
def installed_ruby_version_manager
|
79
|
+
@installed_version ||= begin
|
80
|
+
return :rvm if ENV['PATH']['.rvm']
|
81
|
+
return :rbenv if ENV['PATH']['.rbenv']
|
82
|
+
:none
|
83
|
+
end
|
84
|
+
@installed_manager
|
85
|
+
end
|
86
|
+
|
87
|
+
def run_rvm(filename, version)
|
64
88
|
`rvm #{version} exec ruby #{filename}`
|
65
89
|
end
|
66
|
-
|
90
|
+
|
91
|
+
def run_rbenv(filename, version)
|
92
|
+
`/bin/sh -c "RBENV_VERSION=#{rbenv_version(version)} ~/.rbenv/shims/ruby #{filename}"`
|
93
|
+
rescue ArgumentError => e # Rescue when version is not installed and print a message
|
94
|
+
e.message
|
95
|
+
end
|
96
|
+
|
97
|
+
def rbenv_version(wanted_version)
|
98
|
+
rbenv_version = RBENV_INSTALLED_VERSIONS.select {|version| version.match(/^#{wanted_version}/) }.last
|
99
|
+
# raise error if there is not such version installed
|
100
|
+
raise ArgumentError.new("version not installed") unless rbenv_version
|
101
|
+
rbenv_version
|
102
|
+
end
|
103
|
+
end
|
data/lib/multirb/version.rb
CHANGED
data/multirb.gemspec
CHANGED
@@ -8,8 +8,8 @@ Gem::Specification.new do |gem|
|
|
8
8
|
gem.version = Multirb::VERSION
|
9
9
|
gem.authors = ["Peter Cooper"]
|
10
10
|
gem.email = ["git@peterc.org"]
|
11
|
-
gem.description = %{Run Ruby code over multiple implementations/versions using RVM from a IRB-esque prompt}
|
12
|
-
gem.summary = %{Run Ruby code over multiple implementations/versions using RVM from a IRB-esque prompt}
|
11
|
+
gem.description = %{Run Ruby code over multiple implementations/versions using RVM or RBENV from a IRB-esque prompt}
|
12
|
+
gem.summary = %{Run Ruby code over multiple implementations/versions using RVM or RBENV from a IRB-esque prompt}
|
13
13
|
gem.homepage = "https://github.com/peterc/multirb"
|
14
14
|
|
15
15
|
gem.files = `git ls-files`.split($/)
|
data/test/test_multirb.rb
CHANGED
@@ -25,5 +25,6 @@ describe Multirb do
|
|
25
25
|
determine_versions(['2 + 2 #all', '5 + 5'], %w{1.9.2}).must_equal %w{1.9.2}
|
26
26
|
determine_versions(['2 + 2 # 1.9.2,1.9.3,2.0.0']).must_equal %w{1.9.2 1.9.3 2.0.0}
|
27
27
|
determine_versions(['2 + 2 # 1.9.2, 1.9.3, 2.0.0']).must_equal %w{1.9.2 1.9.3 2.0.0}
|
28
|
+
determine_versions(['2 + 2 "#{1.9}" blah blah']).must_equal DEFAULT_VERSIONS
|
28
29
|
end
|
29
30
|
end
|
metadata
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
--- !ruby/object:Gem::Specification
|
2
2
|
name: multirb
|
3
3
|
version: !ruby/object:Gem::Version
|
4
|
-
version: 0.0.
|
4
|
+
version: 0.0.5
|
5
5
|
prerelease:
|
6
6
|
platform: ruby
|
7
7
|
authors:
|
@@ -9,10 +9,10 @@ authors:
|
|
9
9
|
autorequire:
|
10
10
|
bindir: bin
|
11
11
|
cert_chain: []
|
12
|
-
date: 2013-
|
12
|
+
date: 2013-03-03 00:00:00.000000000 Z
|
13
13
|
dependencies: []
|
14
|
-
description: Run Ruby code over multiple implementations/versions using RVM
|
15
|
-
IRB-esque prompt
|
14
|
+
description: Run Ruby code over multiple implementations/versions using RVM or RBENV
|
15
|
+
from a IRB-esque prompt
|
16
16
|
email:
|
17
17
|
- git@peterc.org
|
18
18
|
executables:
|
@@ -53,7 +53,7 @@ rubyforge_project:
|
|
53
53
|
rubygems_version: 1.8.24
|
54
54
|
signing_key:
|
55
55
|
specification_version: 3
|
56
|
-
summary: Run Ruby code over multiple implementations/versions using RVM
|
57
|
-
prompt
|
56
|
+
summary: Run Ruby code over multiple implementations/versions using RVM or RBENV from
|
57
|
+
a IRB-esque prompt
|
58
58
|
test_files:
|
59
59
|
- test/test_multirb.rb
|