motion-takeoff 0.0.5 → 0.0.6
Sign up to get free protection for your applications and to get access to all the features.
- checksums.yaml +4 -4
- data/README.md +11 -4
- data/lib/project/reminders.rb +59 -48
- data/lib/project/version.rb +1 -1
- metadata +9 -10
checksums.yaml
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
---
|
2
2
|
SHA1:
|
3
|
-
metadata.gz:
|
4
|
-
data.tar.gz:
|
3
|
+
metadata.gz: 02db886ae966ee9fa7985a23a2780157faad50c8
|
4
|
+
data.tar.gz: 4c4ca37df252faa76ef86fc066855af92b565896
|
5
5
|
SHA512:
|
6
|
-
metadata.gz:
|
7
|
-
data.tar.gz:
|
6
|
+
metadata.gz: ba5d2fae0185482be6827a3def58f881271c42fe7eaabc7ab39358ec6e072864294cdb7a8ec031a81dd6df3ce7484fd026009a247c476dcb180235cc8bec38ff
|
7
|
+
data.tar.gz: bf1083b3e406ee797c652c73be453e2a03c85cdf5c1210d9cd7ac6645de4514b8eb6310c53e2770a4efd3aec26a87bd6d49fddf9f5d60431cc60b4993deb36b0
|
data/README.md
CHANGED
@@ -1,5 +1,7 @@
|
|
1
1
|
# motion-takeoff [](https://travis-ci.org/MohawkApps/motion-takeoff) [](https://codeclimate.com/github/MohawkApps/motion-takeoff) [](http://badge.fury.io/rb/motion-takeoff)
|
2
2
|
|
3
|
+
[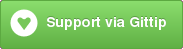](https://www.gittip.com/markrickert/)
|
4
|
+
|
3
5
|
A RubyMotion specific iOS gem for scheduling stuff. You can use motion-takeoff to display messages at certain launch counts and schedule local notifications. Currently, there are two modules in this gem: `Messages` & `Reminders`.
|
4
6
|
|
5
7
|
The `Messages` module will allow you to schedule alerts to users at certain launch counts.
|
@@ -53,6 +55,14 @@ end
|
|
53
55
|
|
54
56
|
The reminders module is a nice wrapper on `UILocalNotification` and makes it easy to schedule reminders to come back and use your app!
|
55
57
|
|
58
|
+
iOS 8 requires that you ask the user for permission to send notifications:
|
59
|
+
|
60
|
+
```ruby
|
61
|
+
def application(application, didFinishLaunchingWithOptions:launchOptions)
|
62
|
+
Takeoff::Reminders.setup
|
63
|
+
end
|
64
|
+
```
|
65
|
+
|
56
66
|
You should always reset all local notifications when your app becomes active:
|
57
67
|
|
58
68
|
```ruby
|
@@ -71,7 +81,7 @@ def applicationDidEnterBackground(application)
|
|
71
81
|
)
|
72
82
|
|
73
83
|
Takeoff::Reminders.schedule(
|
74
|
-
body: "Fires 10 seconds
|
84
|
+
body: "Fires 10 seconds after the first notification.",
|
75
85
|
fire_date: Time.now + 30 #Time object in the future
|
76
86
|
)
|
77
87
|
end
|
@@ -110,6 +120,3 @@ I'd like it to be able to be a multi-purpose tool for doing things at launch oth
|
|
110
120
|
3. Commit your changes (`git commit -am 'Add some feature'`)
|
111
121
|
4. Push to the branch (`git push origin my-new-feature`)
|
112
122
|
5. Create new Pull Request
|
113
|
-
|
114
|
-
---
|
115
|
-
[](https://bitdeli.com/free "Bitdeli Badge")
|
data/lib/project/reminders.rb
CHANGED
@@ -1,60 +1,71 @@
|
|
1
1
|
module Takeoff
|
2
2
|
class Reminders
|
3
3
|
|
4
|
-
|
5
|
-
|
6
|
-
|
7
|
-
|
8
|
-
|
9
|
-
|
10
|
-
|
11
|
-
|
12
|
-
|
13
|
-
repeat: {
|
14
|
-
calendar: nil,
|
15
|
-
interval: 0
|
16
|
-
},
|
17
|
-
time_zone: NSTimeZone.defaultTimeZone,
|
18
|
-
sound: UILocalNotificationDefaultSoundName,
|
19
|
-
user_info: {}
|
20
|
-
|
21
|
-
}.merge(opts)
|
22
|
-
|
23
|
-
# Fix the repeat if they just send the interval.
|
24
|
-
unless opts[:repeat].is_a? Hash
|
25
|
-
opts[:repeat] = {
|
26
|
-
calendar: NSCalendar.currentCalendar,
|
27
|
-
interval: opts[:repeat]
|
28
|
-
}
|
29
|
-
end
|
30
|
-
# Interpret the fire date to an NSDate class it they specified a number of seconds
|
31
|
-
if opts[:fire_date].is_a? Fixnum
|
32
|
-
opts[:fire_date] = NSDate.dateWithTimeIntervalSinceNow(opts[:fire_date])
|
4
|
+
class << self
|
5
|
+
def setup
|
6
|
+
if Device.ios_version >= "8.0"
|
7
|
+
types = UIUserNotificationTypeSound | UIUserNotificationTypeBadge | UIUserNotificationTypeAlert
|
8
|
+
notificationSettings = UIUserNotificationSettings.settingsForTypes(types, categories:nil)
|
9
|
+
UIApplication.sharedApplication.registerUserNotificationSettings(notificationSettings)
|
10
|
+
else
|
11
|
+
UIApplication.sharedApplication.registerForRemoteNotificationTypes((UIRemoteNotificationTypeBadge | UIRemoteNotificationTypeSound | UIRemoteNotificationTypeAlert))
|
12
|
+
end
|
33
13
|
end
|
34
14
|
|
35
|
-
|
36
|
-
|
37
|
-
|
38
|
-
|
39
|
-
|
40
|
-
|
41
|
-
|
42
|
-
|
43
|
-
|
44
|
-
|
45
|
-
|
46
|
-
|
15
|
+
def schedule (opts)
|
16
|
+
raise "You must specify a :body" unless opts[:body]
|
17
|
+
raise "You must specify a :fire_date" unless opts[:fire_date]
|
18
|
+
|
19
|
+
opts = {
|
20
|
+
action: nil,
|
21
|
+
launch_image: nil,
|
22
|
+
badge_number: 0,
|
23
|
+
has_action: true,
|
24
|
+
repeat: {
|
25
|
+
calendar: nil,
|
26
|
+
interval: 0
|
27
|
+
},
|
28
|
+
time_zone: NSTimeZone.defaultTimeZone,
|
29
|
+
sound: UILocalNotificationDefaultSoundName,
|
30
|
+
user_info: {}
|
31
|
+
|
32
|
+
}.merge(opts)
|
33
|
+
|
34
|
+
# Fix the repeat if they just send the interval.
|
35
|
+
unless opts[:repeat].is_a? Hash
|
36
|
+
opts[:repeat] = {
|
37
|
+
calendar: NSCalendar.currentCalendar,
|
38
|
+
interval: opts[:repeat]
|
39
|
+
}
|
40
|
+
end
|
41
|
+
# Interpret the fire date to an NSDate class it they specified a number of seconds
|
42
|
+
if opts[:fire_date].is_a? Fixnum
|
43
|
+
opts[:fire_date] = NSDate.dateWithTimeIntervalSinceNow(opts[:fire_date])
|
44
|
+
end
|
45
|
+
|
46
|
+
notification = UILocalNotification.new.tap do |notif|
|
47
|
+
notif.alertAction = opts[:action]
|
48
|
+
notif.alertBody = opts[:body]
|
49
|
+
notif.alertLaunchImage = opts[:launch_image]
|
50
|
+
notif.applicationIconBadgeNumber = opts[:badge_number]
|
51
|
+
notif.timeZone = opts[:time_zone]
|
52
|
+
notif.fireDate = opts[:fire_date]
|
53
|
+
notif.hasAction = opts[:has_action]
|
54
|
+
notif.repeatCalendar = opts[:repeat][:calendar]
|
55
|
+
notif.repeatInterval = opts[:repeat][:interval]
|
56
|
+
notif.soundName = opts[:sound]
|
57
|
+
notif.userInfo = opts[:user_info]
|
58
|
+
end
|
59
|
+
UIApplication.sharedApplication.scheduleLocalNotification notification
|
47
60
|
end
|
48
|
-
UIApplication.sharedApplication.scheduleLocalNotification notification
|
49
|
-
end
|
50
61
|
|
51
|
-
|
52
|
-
|
53
|
-
|
54
|
-
|
62
|
+
def reset
|
63
|
+
UIApplication.sharedApplication.tap do |app|
|
64
|
+
app.applicationIconBadgeNumber = 0
|
65
|
+
app.cancelAllLocalNotifications
|
66
|
+
end
|
55
67
|
end
|
56
68
|
end
|
57
|
-
|
58
69
|
end
|
59
70
|
end
|
60
71
|
|
data/lib/project/version.rb
CHANGED
metadata
CHANGED
@@ -1,41 +1,41 @@
|
|
1
1
|
--- !ruby/object:Gem::Specification
|
2
2
|
name: motion-takeoff
|
3
3
|
version: !ruby/object:Gem::Version
|
4
|
-
version: 0.0.
|
4
|
+
version: 0.0.6
|
5
5
|
platform: ruby
|
6
6
|
authors:
|
7
7
|
- Mark Rickert
|
8
8
|
autorequire:
|
9
9
|
bindir: bin
|
10
10
|
cert_chain: []
|
11
|
-
date:
|
11
|
+
date: 2014-11-11 00:00:00.000000000 Z
|
12
12
|
dependencies:
|
13
13
|
- !ruby/object:Gem::Dependency
|
14
14
|
name: rake
|
15
15
|
requirement: !ruby/object:Gem::Requirement
|
16
16
|
requirements:
|
17
|
-
- -
|
17
|
+
- - ">="
|
18
18
|
- !ruby/object:Gem::Version
|
19
19
|
version: '0'
|
20
20
|
type: :development
|
21
21
|
prerelease: false
|
22
22
|
version_requirements: !ruby/object:Gem::Requirement
|
23
23
|
requirements:
|
24
|
-
- -
|
24
|
+
- - ">="
|
25
25
|
- !ruby/object:Gem::Version
|
26
26
|
version: '0'
|
27
27
|
- !ruby/object:Gem::Dependency
|
28
28
|
name: bubble-wrap
|
29
29
|
requirement: !ruby/object:Gem::Requirement
|
30
30
|
requirements:
|
31
|
-
- -
|
31
|
+
- - ">"
|
32
32
|
- !ruby/object:Gem::Version
|
33
33
|
version: 1.0.0
|
34
34
|
type: :runtime
|
35
35
|
prerelease: false
|
36
36
|
version_requirements: !ruby/object:Gem::Requirement
|
37
37
|
requirements:
|
38
|
-
- -
|
38
|
+
- - ">"
|
39
39
|
- !ruby/object:Gem::Version
|
40
40
|
version: 1.0.0
|
41
41
|
description: A RubyMotion specific iOS gem for scheduling stuff.
|
@@ -60,20 +60,19 @@ require_paths:
|
|
60
60
|
- lib
|
61
61
|
required_ruby_version: !ruby/object:Gem::Requirement
|
62
62
|
requirements:
|
63
|
-
- -
|
63
|
+
- - ">="
|
64
64
|
- !ruby/object:Gem::Version
|
65
65
|
version: '0'
|
66
66
|
required_rubygems_version: !ruby/object:Gem::Requirement
|
67
67
|
requirements:
|
68
|
-
- -
|
68
|
+
- - ">="
|
69
69
|
- !ruby/object:Gem::Version
|
70
70
|
version: '0'
|
71
71
|
requirements: []
|
72
72
|
rubyforge_project:
|
73
|
-
rubygems_version: 2.0
|
73
|
+
rubygems_version: 2.2.0
|
74
74
|
signing_key:
|
75
75
|
specification_version: 4
|
76
76
|
summary: A RubyMotion specific iOS gem for scheduling stuff. You can use motion-takeoff
|
77
77
|
to display messages at certain launch counts and schedule local notifications.
|
78
78
|
test_files: []
|
79
|
-
has_rdoc:
|