moran 0.0.1 → 0.0.2
Sign up to get free protection for your applications and to get access to all the features.
- checksums.yaml +4 -4
- data/README.md +6 -1
- data/motion/moran/java_to_ruby.rb +43 -8
- data/motion/moran/version.rb +1 -1
- metadata +2 -2
checksums.yaml
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
---
|
2
2
|
SHA1:
|
3
|
-
metadata.gz:
|
4
|
-
data.tar.gz:
|
3
|
+
metadata.gz: 70ddd5afef0ee4b3beef8724628259b09ba16051
|
4
|
+
data.tar.gz: d22f30c8b0fe1ef20e5844b707ce57f9899eff07
|
5
5
|
SHA512:
|
6
|
-
metadata.gz:
|
7
|
-
data.tar.gz:
|
6
|
+
metadata.gz: fdf5541c3bfb2795037b6ce93e68ef1b64b679ee002670df53acc9eae66e638d2252f0d53227b59ab93ffdf4025081f20726176eef7981ade0c830410e699f33
|
7
|
+
data.tar.gz: 1fe77e79f5e070badd17cbe2e1b04f0aaf1a45c9ecca9244770ddc417b010fe7c93fd75d6e92742ee6b74fca136de0849a19d2b9d1d44945952a35a553baa06a
|
data/README.md
CHANGED
@@ -1,6 +1,6 @@
|
|
1
1
|
# moran
|
2
2
|
|
3
|
-
moran is a simple JSON parser and generator for [RubyMotion Android](http://rubymotion.com). It provides a Ruby wrapper around the [Jackson JSON parser for Java.](https://github.com/FasterXML/jackson)
|
3
|
+
moran (mo-RAN) is a simple JSON parser and generator for [RubyMotion Android](http://rubymotion.com). It provides a Ruby wrapper around the [Jackson JSON parser for Java.](https://github.com/FasterXML/jackson)
|
4
4
|
|
5
5
|
moran emulates the standard Ruby JSON API, providing `parse` and `generate` methods:
|
6
6
|
|
@@ -87,4 +87,9 @@ or
|
|
87
87
|
rake spec:device
|
88
88
|
```
|
89
89
|
|
90
|
+
## What's in a name?
|
91
|
+
|
92
|
+
Moran was named after jazz pianist and composer [Jason Moran](http://www.jasonmoran.com/)
|
93
|
+
|
94
|
+
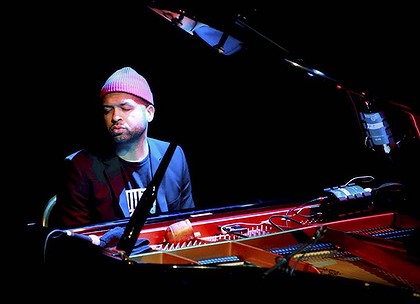
|
90
95
|
|
@@ -6,11 +6,11 @@
|
|
6
6
|
# JavaToRuby.convert_hashmap(hashmap)
|
7
7
|
#
|
8
8
|
# This supports nested hashes and should assure that all Java-based values are properly converted
|
9
|
-
# to their Ruby counterparts (i.e. java.lang.String => String).
|
9
|
+
# to their Ruby counterparts (i.e. java.lang.String => String).
|
10
10
|
#
|
11
11
|
# This has not been extensively tested, or optimized for performance. It should work well enough
|
12
|
-
# for simple cases, but may well fall over with a large HashMap.
|
13
|
-
#
|
12
|
+
# for simple cases, but may well fall over with a large HashMap.
|
13
|
+
#
|
14
14
|
class JavaToRuby
|
15
15
|
|
16
16
|
class << self
|
@@ -34,9 +34,9 @@ class JavaToRuby
|
|
34
34
|
end
|
35
35
|
end
|
36
36
|
end
|
37
|
-
|
37
|
+
|
38
38
|
def json_object_to_hash(json_obj)
|
39
|
-
return nil if json_obj.nil?
|
39
|
+
return nil if (json_obj.nil? || json_obj.toString == "null")
|
40
40
|
Hash.new.tap do |h|
|
41
41
|
it = json_obj.keys()
|
42
42
|
while it.hasNext() do
|
@@ -46,14 +46,30 @@ class JavaToRuby
|
|
46
46
|
end
|
47
47
|
end
|
48
48
|
end
|
49
|
+
|
50
|
+
def json_array_to_array(json_array)
|
51
|
+
return nil if json_array.nil?
|
52
|
+
Array.new.tap do |a|
|
53
|
+
inx = 0
|
54
|
+
while inx < json_array.length() do
|
55
|
+
a << convert_value(json_array.get(inx))
|
56
|
+
inx += 1
|
57
|
+
end
|
58
|
+
end
|
59
|
+
end
|
49
60
|
private
|
50
61
|
|
51
62
|
def convert_value(value)
|
52
63
|
new_value ||= hashmap_to_hash(value) if hashmap?(value)
|
53
64
|
new_value ||= to_array(value) if array?(value)
|
54
65
|
new_value ||= to_boolean(value) if boolean?(value)
|
55
|
-
new_value ||=
|
56
|
-
new_value ||= value
|
66
|
+
new_value ||= to_float(value) if double?(value)
|
67
|
+
new_value ||= json_object_to_hash(value) if json_object?(value)
|
68
|
+
new_value ||= json_array_to_array(value) if json_array?(value)
|
69
|
+
|
70
|
+
# Using ternary here so the null-turned-JSONObjects return nil
|
71
|
+
# instead of the original value
|
72
|
+
new_value ||= null?(value) ? nil : value
|
57
73
|
end
|
58
74
|
|
59
75
|
def hashmap?(value)
|
@@ -68,8 +84,23 @@ class JavaToRuby
|
|
68
84
|
["true","false"].include?(value.to_s.downcase)
|
69
85
|
end
|
70
86
|
|
87
|
+
def json_object?(value)
|
88
|
+
# If value is a null-turned-JSONObject, the class is java.lang.JSONObject$1
|
89
|
+
# so we use include here
|
90
|
+
value.class.to_s.include?("JSONObject")
|
91
|
+
end
|
92
|
+
|
93
|
+
def json_array?(value)
|
94
|
+
value.class.to_s.end_with?("JSONArray")
|
95
|
+
end
|
96
|
+
|
97
|
+
def double?(value)
|
98
|
+
value.class.to_s.end_with?("Double")
|
99
|
+
end
|
100
|
+
|
71
101
|
def null?(value)
|
72
|
-
value
|
102
|
+
# If value is a null-turned-JSONObject, value.toString returns "null"
|
103
|
+
value.nil? || value.to_s.downcase == "null" || (value.respond_to?("toString") && value.toString == "null")
|
73
104
|
end
|
74
105
|
|
75
106
|
def to_array(array)
|
@@ -78,6 +109,10 @@ class JavaToRuby
|
|
78
109
|
array.map { |value| convert_value(value) }
|
79
110
|
end
|
80
111
|
|
112
|
+
def to_float(value)
|
113
|
+
value.to_s.to_f
|
114
|
+
end
|
115
|
+
|
81
116
|
def to_boolean(value)
|
82
117
|
value.to_s.downcase == "true"
|
83
118
|
end
|
data/motion/moran/version.rb
CHANGED
metadata
CHANGED
@@ -1,14 +1,14 @@
|
|
1
1
|
--- !ruby/object:Gem::Specification
|
2
2
|
name: moran
|
3
3
|
version: !ruby/object:Gem::Version
|
4
|
-
version: 0.0.
|
4
|
+
version: 0.0.2
|
5
5
|
platform: ruby
|
6
6
|
authors:
|
7
7
|
- Darin Wilson
|
8
8
|
autorequire:
|
9
9
|
bindir: bin
|
10
10
|
cert_chain: []
|
11
|
-
date: 2015-
|
11
|
+
date: 2015-05-09 00:00:00.000000000 Z
|
12
12
|
dependencies:
|
13
13
|
- !ruby/object:Gem::Dependency
|
14
14
|
name: motion-gradle
|