lecture 1.0.0
Sign up to get free protection for your applications and to get access to all the features.
- checksums.yaml +7 -0
- data/.gitignore +9 -0
- data/.rubocop.yml +866 -0
- data/.ruby-version +1 -0
- data/Gemfile +7 -0
- data/LICENSE.txt +21 -0
- data/README.md +145 -0
- data/Rakefile +4 -0
- data/bin/console +18 -0
- data/bin/lecture +29 -0
- data/bin/setup +6 -0
- data/examples/example.rb +35 -0
- data/lecture.gemspec +34 -0
- data/lib/lecture.rb +89 -0
- data/lib/lecture/config.rb +23 -0
- data/lib/lecture/runner.rb +89 -0
- data/lib/lecture/slide.rb +23 -0
- data/lib/lecture/slide/base.rb +43 -0
- data/lib/lecture/slide/block.rb +25 -0
- data/lib/lecture/slide/center.rb +21 -0
- data/lib/lecture/slide/code.rb +21 -0
- data/lib/lecture/slide/section.rb +29 -0
- data/lib/lecture/terminal.rb +64 -0
- data/lib/lecture/version.rb +5 -0
- data/screenshots/block.png +0 -0
- data/screenshots/center.png +0 -0
- data/screenshots/code.png +0 -0
- data/screenshots/section.png +0 -0
- metadata +184 -0
data/.ruby-version
ADDED
@@ -0,0 +1 @@
|
|
1
|
+
2.4.1
|
data/Gemfile
ADDED
data/LICENSE.txt
ADDED
@@ -0,0 +1,21 @@
|
|
1
|
+
The MIT License (MIT)
|
2
|
+
|
3
|
+
Copyright (c) 2017 npezza93
|
4
|
+
|
5
|
+
Permission is hereby granted, free of charge, to any person obtaining a copy
|
6
|
+
of this software and associated documentation files (the "Software"), to deal
|
7
|
+
in the Software without restriction, including without limitation the rights
|
8
|
+
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
9
|
+
copies of the Software, and to permit persons to whom the Software is
|
10
|
+
furnished to do so, subject to the following conditions:
|
11
|
+
|
12
|
+
The above copyright notice and this permission notice shall be included in
|
13
|
+
all copies or substantial portions of the Software.
|
14
|
+
|
15
|
+
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
16
|
+
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
17
|
+
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
18
|
+
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
19
|
+
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
20
|
+
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
|
21
|
+
THE SOFTWARE.
|
data/README.md
ADDED
@@ -0,0 +1,145 @@
|
|
1
|
+
# Lecture
|
2
|
+
|
3
|
+
Lecture is a way to present a slideshow through the terminal. All slides are written
|
4
|
+
in Ruby. There is an example in the examples folder, found [here](https://github.com/npezza93/lecture/tree/master/examples).
|
5
|
+
|
6
|
+
## Installation
|
7
|
+
|
8
|
+
```bash
|
9
|
+
❯ gem install lecture
|
10
|
+
```
|
11
|
+
|
12
|
+
## Usage
|
13
|
+
|
14
|
+
```bash
|
15
|
+
❯ lecture #{slides.rb}
|
16
|
+
```
|
17
|
+
|
18
|
+
### Configuration
|
19
|
+
|
20
|
+
At the top of your slides.rb file you can use the `configure` method to configure
|
21
|
+
settings within Lecture.
|
22
|
+
|
23
|
+
```ruby
|
24
|
+
configure do |lecture|
|
25
|
+
lecture.section_footer_text = "───"
|
26
|
+
end
|
27
|
+
```
|
28
|
+
|
29
|
+
Configurable settings include:
|
30
|
+
- character_print_delay
|
31
|
+
- transition_time
|
32
|
+
- pygment_style
|
33
|
+
- section_header_text
|
34
|
+
- section_footer_text
|
35
|
+
|
36
|
+
### Slide Types
|
37
|
+
|
38
|
+
When writing your slides.rb file you have four slide types available.
|
39
|
+
|
40
|
+
#### center
|
41
|
+
|
42
|
+
A center slide centers every line on the screen horizontally and vertically.
|
43
|
+
|
44
|
+
```ruby
|
45
|
+
center <<~SLIDE
|
46
|
+
Hello
|
47
|
+
|
48
|
+
|
49
|
+
This is a slide
|
50
|
+
SLIDE
|
51
|
+
```
|
52
|
+
|
53
|
+
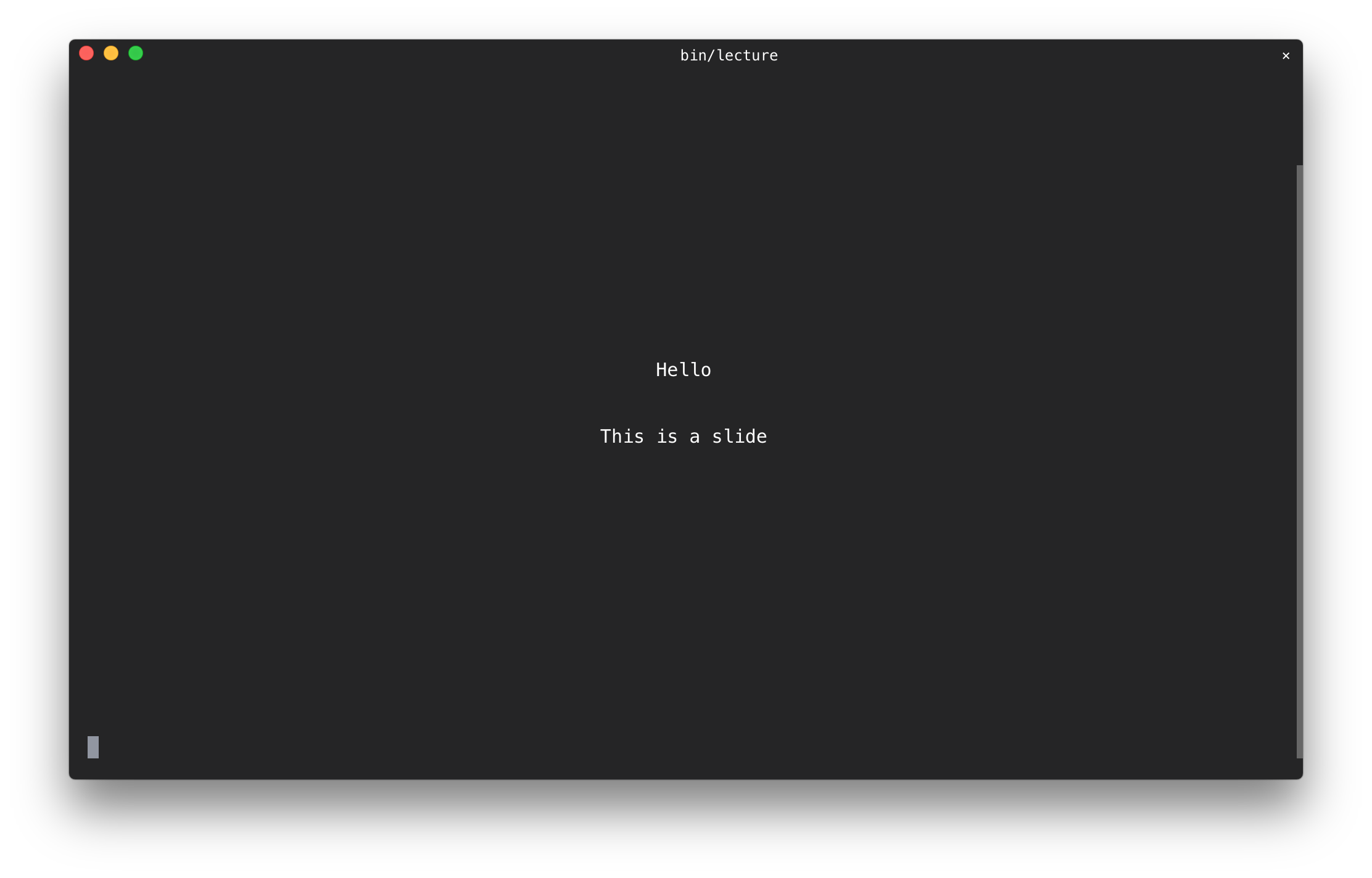
|
54
|
+
|
55
|
+
#### block
|
56
|
+
|
57
|
+
A block slide preserves the text formatting, but centers the slide as a whole on the screen.
|
58
|
+
|
59
|
+
|
60
|
+
```ruby
|
61
|
+
block <<~SLIDE
|
62
|
+
The lines in this block of text are
|
63
|
+
not centered
|
64
|
+
SLIDE
|
65
|
+
```
|
66
|
+
|
67
|
+
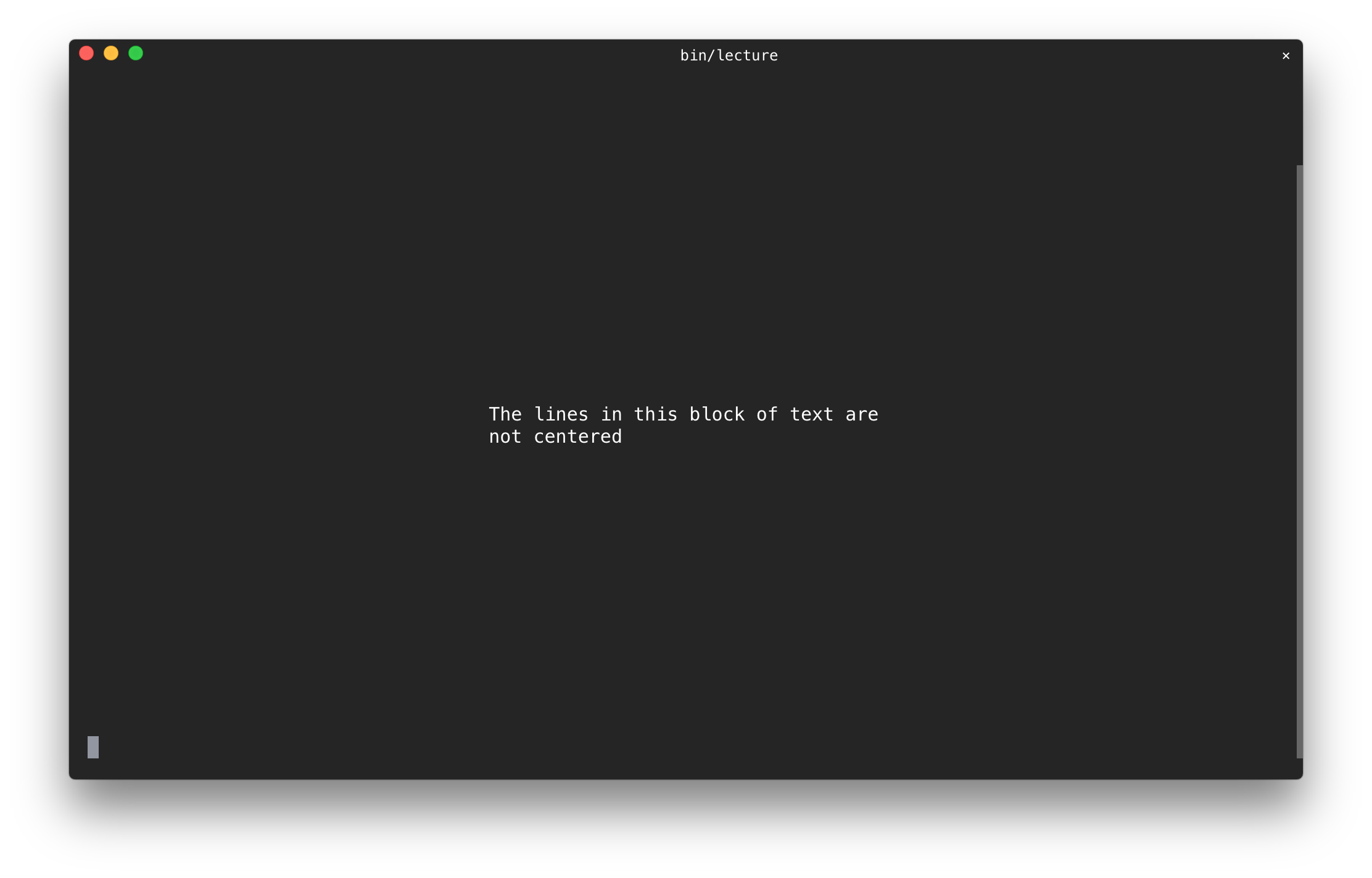
|
68
|
+
|
69
|
+
#### section
|
70
|
+
|
71
|
+
A section slide has a title and draws a centered string of text above and below the title (default: " § ").
|
72
|
+
It can be configured with the `section_header_text` and `section_footer_text` keys.
|
73
|
+
|
74
|
+
Sections allow you to group slides in your Ruby slide deck, and can yield to a block to promote slide organization.
|
75
|
+
|
76
|
+
```ruby
|
77
|
+
section("This is a section") do
|
78
|
+
center("This is a centered slide inside a section")
|
79
|
+
end
|
80
|
+
```
|
81
|
+
|
82
|
+

|
83
|
+
|
84
|
+
#### code
|
85
|
+
|
86
|
+
A code slide contains source code and has syntax highlighting.
|
87
|
+
|
88
|
+
The code method requires a second keyword argument with the name of the programming language.
|
89
|
+
|
90
|
+
The syntax highlighting style can be configured with the `pygment_style` key. Styles can be found [here](https://github.com/tmm1/pygments.rb), default is `paraiso-dark`.
|
91
|
+
|
92
|
+
```ruby
|
93
|
+
code(%(
|
94
|
+
class PostsController < ApplicationController
|
95
|
+
def index
|
96
|
+
@posts = Post.all
|
97
|
+
end
|
98
|
+
end
|
99
|
+
), lexer: :ruby)
|
100
|
+
```
|
101
|
+
|
102
|
+
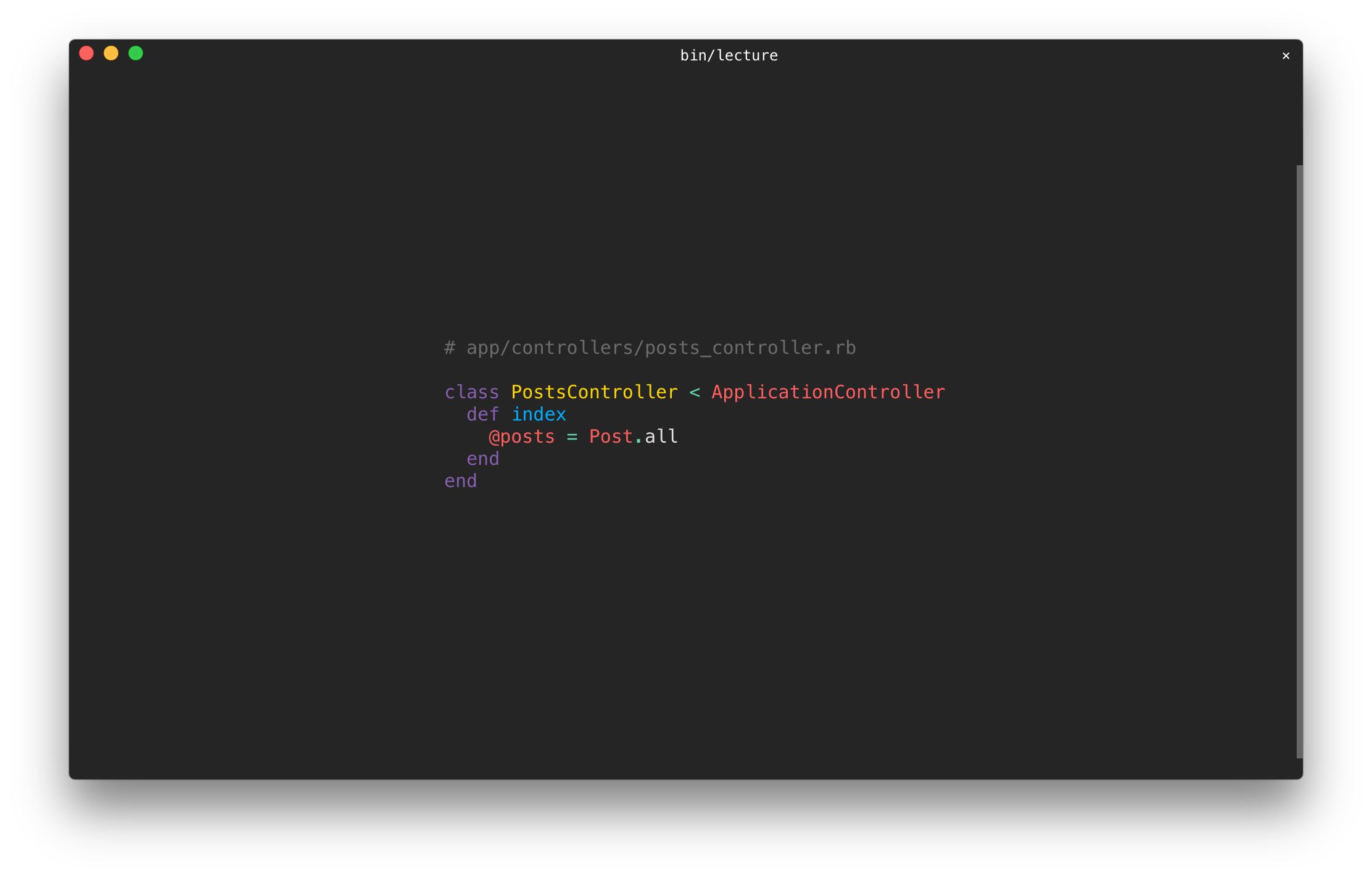
|
103
|
+
|
104
|
+
### Keyboard Controls
|
105
|
+
|
106
|
+
- <kbd>SPACE</kbd> or <kbd>→</kbd> go to the next slide
|
107
|
+
- <kbd>←</kbd> go to the previous slide
|
108
|
+
- <kbd>a</kbd> jumps to the first slide of the deck
|
109
|
+
- <kbd>d</kbd> jumps to the last slide of the deck
|
110
|
+
- <kbd>j</kbd> followed by a slide number(0 index) jumps to the given slide
|
111
|
+
- <kbd>w</kbd> redraws the slide
|
112
|
+
- <kbd>q</kbd> or <kbd>ESC</kbd> exits the slideshow
|
113
|
+
|
114
|
+
### String Utilities
|
115
|
+
|
116
|
+
You have a variety of string colors and modes available when creating slides. To get a list of colors and modes you can use: `Lecture.available_colors` and `Lecture.available_modes`.
|
117
|
+
|
118
|
+
```ruby
|
119
|
+
center <<~SLIDE
|
120
|
+
#{'Slide'.bold}
|
121
|
+
|
122
|
+
|
123
|
+
This is a #{'slide'.green}
|
124
|
+
SLIDE
|
125
|
+
```
|
126
|
+
|
127
|
+
You can also use ANSI escape sequences and unicode characters to spice up your slides.
|
128
|
+
|
129
|
+
## Development
|
130
|
+
|
131
|
+
After checking out the repo, run `bin/setup` to install dependencies. You can also run `bin/console` for an interactive prompt that will allow you to experiment.
|
132
|
+
|
133
|
+
To install this gem onto your local machine, run `bundle exec rake install`. To release a new version, update the version number in `version.rb`, and then run `bundle exec rake release`, which will create a git tag for the version, push git commits and tags, and push the `.gem` file to [rubygems.org](https://rubygems.org).
|
134
|
+
|
135
|
+
## Contributing
|
136
|
+
|
137
|
+
Bug reports and pull requests are welcome on GitHub at https://github.com/npezza93/lecture.
|
138
|
+
|
139
|
+
## License
|
140
|
+
|
141
|
+
The gem is available as open source under the terms of the [MIT License](http://opensource.org/licenses/MIT).
|
142
|
+
|
143
|
+
---
|
144
|
+
|
145
|
+
Inspired by [fxn/tkn](https://github.com/fxn/tkn)
|
data/Rakefile
ADDED
data/bin/console
ADDED
@@ -0,0 +1,18 @@
|
|
1
|
+
#!/usr/bin/env ruby
|
2
|
+
# frozen_string_literal: true
|
3
|
+
|
4
|
+
require "bundler/setup"
|
5
|
+
require "lecture"
|
6
|
+
|
7
|
+
# You can add fixtures and/or initialization code here to make experimenting
|
8
|
+
def reload!(print = true)
|
9
|
+
puts "Reloading..." if print
|
10
|
+
files = $LOADED_FEATURES.select do |feat|
|
11
|
+
feat =~ /\/lecture\//
|
12
|
+
end
|
13
|
+
files.each { |file| load file }
|
14
|
+
true
|
15
|
+
end
|
16
|
+
|
17
|
+
require "pry"
|
18
|
+
Pry.start
|
data/bin/lecture
ADDED
@@ -0,0 +1,29 @@
|
|
1
|
+
#!/usr/bin/env ruby
|
2
|
+
# frozen_string_literal: true
|
3
|
+
|
4
|
+
$LOAD_PATH.unshift(File.dirname(File.realpath(__FILE__)) + "/../lib")
|
5
|
+
|
6
|
+
require "bundler/setup"
|
7
|
+
require "lecture"
|
8
|
+
require "getoptlong"
|
9
|
+
|
10
|
+
opts = GetoptLong.new(["--help", "-h", GetoptLong::NO_ARGUMENT])
|
11
|
+
opts.each do |opt, _|
|
12
|
+
next unless opt == "--help"
|
13
|
+
|
14
|
+
puts Lecture::HELP
|
15
|
+
exit 0
|
16
|
+
end
|
17
|
+
|
18
|
+
if ARGV.empty?
|
19
|
+
puts "lecture: A file must be supplied\n\n"
|
20
|
+
puts Lecture::HELP
|
21
|
+
exit 1
|
22
|
+
end
|
23
|
+
|
24
|
+
unless File.exist?(slide_deck = ARGV[0])
|
25
|
+
puts "lecture: Can't find slide deck '#{slide_deck}'"
|
26
|
+
exit 1
|
27
|
+
end
|
28
|
+
|
29
|
+
Lecture.execute(slide_deck)
|
data/bin/setup
ADDED
data/examples/example.rb
ADDED
@@ -0,0 +1,35 @@
|
|
1
|
+
# frozen_string_literal: true
|
2
|
+
|
3
|
+
configure do |lecture|
|
4
|
+
lecture.section_footer_text = "───"
|
5
|
+
end
|
6
|
+
|
7
|
+
center <<~SLIDE
|
8
|
+
Hello
|
9
|
+
|
10
|
+
|
11
|
+
This is a slide
|
12
|
+
SLIDE
|
13
|
+
|
14
|
+
center <<~SLIDE
|
15
|
+
#{'Slide 2'.bold}
|
16
|
+
|
17
|
+
|
18
|
+
This is the #{'second'.green} slide
|
19
|
+
SLIDE
|
20
|
+
|
21
|
+
code(%(
|
22
|
+
# app/controllers/posts_controller.rb
|
23
|
+
|
24
|
+
class PostsController < ApplicationController
|
25
|
+
def index
|
26
|
+
@posts = Post.all
|
27
|
+
end
|
28
|
+
end
|
29
|
+
), lexer: :ruby)
|
30
|
+
|
31
|
+
section("This is a section") do
|
32
|
+
center("This is a centered slide inside a section")
|
33
|
+
end
|
34
|
+
|
35
|
+
block("The lines in this block of text are\nnot centered")
|
data/lecture.gemspec
ADDED
@@ -0,0 +1,34 @@
|
|
1
|
+
# coding: utf-8
|
2
|
+
# frozen_string_literal: true
|
3
|
+
|
4
|
+
lib = File.expand_path("../lib", __FILE__)
|
5
|
+
$LOAD_PATH.unshift(lib) unless $LOAD_PATH.include?(lib)
|
6
|
+
|
7
|
+
require "lecture/version"
|
8
|
+
|
9
|
+
Gem::Specification.new do |spec|
|
10
|
+
spec.name = "lecture"
|
11
|
+
spec.version = Lecture::VERSION
|
12
|
+
spec.authors = ["npezza93"]
|
13
|
+
spec.email = ["npezza93@gmail.com"]
|
14
|
+
|
15
|
+
spec.summary = "Terminal Slideshow"
|
16
|
+
# spec.homepage = "TODO: Put your gem's website or public repo URL here."
|
17
|
+
spec.license = "MIT"
|
18
|
+
|
19
|
+
spec.files = `git ls-files -z`.split("\x0").reject do |f|
|
20
|
+
f.match(%r{^(test|spec|features)/})
|
21
|
+
end
|
22
|
+
spec.executables = ["lecture"]
|
23
|
+
spec.require_paths = ["lib"]
|
24
|
+
|
25
|
+
spec.add_dependency "pygments.rb"
|
26
|
+
spec.add_dependency "colorize"
|
27
|
+
spec.add_dependency "activesupport"
|
28
|
+
|
29
|
+
spec.add_development_dependency "bundler", "~> 1.15"
|
30
|
+
spec.add_development_dependency "rake", "~> 10.0"
|
31
|
+
spec.add_development_dependency "rubocop"
|
32
|
+
spec.add_development_dependency "pry"
|
33
|
+
spec.add_development_dependency "minitest"
|
34
|
+
end
|
data/lib/lecture.rb
ADDED
@@ -0,0 +1,89 @@
|
|
1
|
+
# frozen_string_literal: true
|
2
|
+
|
3
|
+
require "pygments.rb"
|
4
|
+
require "colorize"
|
5
|
+
require "io/console"
|
6
|
+
require "active_support/all"
|
7
|
+
|
8
|
+
require "lecture/version"
|
9
|
+
require "lecture/config"
|
10
|
+
require "lecture/terminal"
|
11
|
+
require "lecture/slide"
|
12
|
+
|
13
|
+
module Lecture
|
14
|
+
mattr_writer :pygment_style, :character_print_delay, :slide_types,
|
15
|
+
:transition_time, :section_header_text, :section_footer_text
|
16
|
+
|
17
|
+
def self.character_print_delay
|
18
|
+
@@character_print_delay || 0.018
|
19
|
+
end
|
20
|
+
|
21
|
+
def self.transition_time
|
22
|
+
@@transition_time || 0.3
|
23
|
+
end
|
24
|
+
|
25
|
+
def self.pygment_style
|
26
|
+
@@pygment_style || "paraiso-dark"
|
27
|
+
end
|
28
|
+
|
29
|
+
def self.slide_types
|
30
|
+
@@slide_types ||= Set.new
|
31
|
+
end
|
32
|
+
|
33
|
+
def self.section_header_text
|
34
|
+
@@section_header_text ||= " § "
|
35
|
+
end
|
36
|
+
|
37
|
+
def self.section_footer_text
|
38
|
+
@@section_footer_text ||= " § "
|
39
|
+
end
|
40
|
+
|
41
|
+
def self.available_colors
|
42
|
+
String.colors
|
43
|
+
end
|
44
|
+
|
45
|
+
def self.available_modes
|
46
|
+
String.modes
|
47
|
+
end
|
48
|
+
|
49
|
+
def self.execute(deck)
|
50
|
+
Runner.new(deck).execute
|
51
|
+
end
|
52
|
+
|
53
|
+
HELP = <<~HELP
|
54
|
+
#{'VERSION'.bold} #{Lecture::VERSION}
|
55
|
+
|
56
|
+
#{'NAME'.bold}
|
57
|
+
lecture - Terminal Slideshow
|
58
|
+
|
59
|
+
#{'SYNOPSIS'.bold}
|
60
|
+
lecture [--help] <slides>.rb
|
61
|
+
|
62
|
+
#{'OPTIONS'.bold}
|
63
|
+
-h, --help
|
64
|
+
Print this help
|
65
|
+
|
66
|
+
#{'COMMANDS'.bold}
|
67
|
+
Next slide: SPC, Right Arrow
|
68
|
+
Previous slide: Left Arrow
|
69
|
+
First slide: a
|
70
|
+
Last slide: d
|
71
|
+
Jump to slide: j
|
72
|
+
Reload slide: w
|
73
|
+
Quit: q
|
74
|
+
|
75
|
+
#{'SLIDE TYPES'.bold}
|
76
|
+
code Highlighted block of code
|
77
|
+
center All lines are centered on the screen
|
78
|
+
block The section of text is centered as whole
|
79
|
+
section A stylized section identifier slide
|
80
|
+
HELP
|
81
|
+
end
|
82
|
+
|
83
|
+
require "lecture/slide/base"
|
84
|
+
require "lecture/slide/center"
|
85
|
+
require "lecture/slide/code"
|
86
|
+
require "lecture/slide/section"
|
87
|
+
require "lecture/slide/block"
|
88
|
+
|
89
|
+
require "lecture/runner"
|
@@ -0,0 +1,23 @@
|
|
1
|
+
module Lecture
|
2
|
+
class Config
|
3
|
+
def character_print_delay=(value)
|
4
|
+
Lecture.character_print_delay = value
|
5
|
+
end
|
6
|
+
|
7
|
+
def transition_time=(value)
|
8
|
+
Lecture.transition_time = value
|
9
|
+
end
|
10
|
+
|
11
|
+
def pygment_style=(value)
|
12
|
+
Lecture.pygment_style = value
|
13
|
+
end
|
14
|
+
|
15
|
+
def section_header_text=(value)
|
16
|
+
Lecture.section_header_text = value
|
17
|
+
end
|
18
|
+
|
19
|
+
def section_footer_text=(value)
|
20
|
+
Lecture.section_footer_text = value
|
21
|
+
end
|
22
|
+
end
|
23
|
+
end
|
@@ -0,0 +1,89 @@
|
|
1
|
+
# frozen_string_literal: true
|
2
|
+
|
3
|
+
module Lecture
|
4
|
+
class Runner
|
5
|
+
include Terminal
|
6
|
+
|
7
|
+
Lecture.slide_types.each do |format|
|
8
|
+
define_method(format) do |slide_content, **options, &block|
|
9
|
+
slides.push(
|
10
|
+
Slide.new(content: slide_content, format: format, **options)
|
11
|
+
)
|
12
|
+
block.call if block.present?
|
13
|
+
end
|
14
|
+
end
|
15
|
+
|
16
|
+
attr_accessor :current_slide, :slides, :deck
|
17
|
+
|
18
|
+
def initialize(deck)
|
19
|
+
@current_slide = 0
|
20
|
+
@slides = []
|
21
|
+
@deck = deck
|
22
|
+
end
|
23
|
+
|
24
|
+
def execute
|
25
|
+
instance_eval(File.read(deck))
|
26
|
+
first_slide
|
27
|
+
|
28
|
+
loop do
|
29
|
+
command_handler
|
30
|
+
end
|
31
|
+
end
|
32
|
+
|
33
|
+
def configure
|
34
|
+
yield(Config.new)
|
35
|
+
end
|
36
|
+
|
37
|
+
private
|
38
|
+
|
39
|
+
def command_handler
|
40
|
+
case getch
|
41
|
+
when " ", "\e[C" then next_slide
|
42
|
+
when "\e[D" then prev_slide
|
43
|
+
when "a" then first_slide
|
44
|
+
when "d" then last_slide
|
45
|
+
when "j" then jump_to
|
46
|
+
when "w" then wipe
|
47
|
+
when "q", "\e" then clear_and_exit
|
48
|
+
end
|
49
|
+
end
|
50
|
+
|
51
|
+
def display(slide_number)
|
52
|
+
clear
|
53
|
+
self.current_slide = slide_number
|
54
|
+
|
55
|
+
clear_and_exit if slides.length - 1 < current_slide
|
56
|
+
|
57
|
+
slides.at(current_slide).display
|
58
|
+
|
59
|
+
move_to(0, lines)
|
60
|
+
end
|
61
|
+
|
62
|
+
def jump_to
|
63
|
+
move_to(0, lines)
|
64
|
+
print "Jump to: "
|
65
|
+
|
66
|
+
display(STDIN.gets.strip.to_i)
|
67
|
+
end
|
68
|
+
|
69
|
+
def next_slide
|
70
|
+
display(current_slide + 1)
|
71
|
+
end
|
72
|
+
|
73
|
+
def prev_slide
|
74
|
+
display(current_slide - 1)
|
75
|
+
end
|
76
|
+
|
77
|
+
def first_slide
|
78
|
+
display(0)
|
79
|
+
end
|
80
|
+
|
81
|
+
def last_slide
|
82
|
+
display(slides.size - 1)
|
83
|
+
end
|
84
|
+
|
85
|
+
def wipe
|
86
|
+
display(current_slide)
|
87
|
+
end
|
88
|
+
end
|
89
|
+
end
|