jekyll-joule 0.0.3 → 0.0.4
This diff represents the content of publicly available package versions that have been released to one of the supported registries. The information contained in this diff is provided for informational purposes only and reflects changes between package versions as they appear in their respective public registries.
- checksums.yaml +4 -4
- data/README.md +31 -0
- data/docs/api/find.md +66 -0
- data/docs/api/find_all.md +51 -0
- data/docs/api/render.md +52 -0
- data/docs/rendering.md +38 -0
- data/docs/setup.md +2 -2
- data/docs/writing-tests.md +87 -0
- data/examples/test/source/index.md +5 -0
- data/examples/test/test_example.rb +3 -3
- data/lib/jekyll/joule/site.rb +1 -2
- data/lib/jekyll/joule/version.rb +1 -1
- metadata +8 -3
- data/examples/test/source/joule.md +0 -3
checksums.yaml
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
---
|
2
2
|
SHA1:
|
3
|
-
metadata.gz:
|
4
|
-
data.tar.gz:
|
3
|
+
metadata.gz: 3d9106e4d2029a3eff12d283263d222ac4af2ddf
|
4
|
+
data.tar.gz: e71183c5bb01ae0989d99557b0780486addbe1fd
|
5
5
|
SHA512:
|
6
|
-
metadata.gz:
|
7
|
-
data.tar.gz:
|
6
|
+
metadata.gz: 6f6d471da8656f570362d52fc0997910c7410f39dab2e27b41d2ee875028703f07657278024d911bb344fd2b32d286acb4e5e7c852d1a26a67fe8b43aac3034f
|
7
|
+
data.tar.gz: 92db87cf50fb452144b5360604e29951709f6f7a663659de4701894e92dd682b33afe56081bfd592c2e648a03825160ccafc84d86c866fdb356d766bcca6bd6c
|
data/README.md
CHANGED
@@ -24,11 +24,42 @@ gem install jekyll-joule
|
|
24
24
|
```
|
25
25
|
|
26
26
|
|
27
|
+
|
28
|
+
## Basic Usage
|
29
|
+
|
30
|
+
Below is an example of how you can write a test with Joule.
|
31
|
+
|
32
|
+
```rb
|
33
|
+
class ExampleTest < JekyllUnitTest
|
34
|
+
should "render a div containing the Page title" do
|
35
|
+
@joule.render(%Q[
|
36
|
+
---
|
37
|
+
title: "Yiss"
|
38
|
+
---
|
39
|
+
<div class="aww">
|
40
|
+
{{ page.title }}
|
41
|
+
</div>
|
42
|
+
])
|
43
|
+
|
44
|
+
el = @joule.find(".aww")
|
45
|
+
|
46
|
+
assert(el)
|
47
|
+
assert(el.text.include?("Yiss"))
|
48
|
+
assert(el["class"].include?("aww"))
|
49
|
+
end
|
50
|
+
end
|
51
|
+
```
|
52
|
+
|
53
|
+
Check out the full [rendering API documentation](./docs/rendering.md)
|
54
|
+
|
55
|
+
|
56
|
+
|
27
57
|
## Documentation
|
28
58
|
|
29
59
|
**[View the docs](https://github.com/helpscout/jekyll-joule/blob/master/docs/introduction.md)** to get started with Joule!
|
30
60
|
|
31
61
|
|
62
|
+
|
32
63
|
## Examples
|
33
64
|
|
34
65
|
**[View the example](https://github.com/helpscout/jekyll-joule/tree/master/examples)** Jekyll setup + Joule test files.
|
data/docs/api/find.md
ADDED
@@ -0,0 +1,66 @@
|
|
1
|
+
# .find()
|
2
|
+
|
3
|
+
#### .find(selector)
|
4
|
+
|
5
|
+
| Argument | Type | Description |
|
6
|
+
| --- | --- | --- |
|
7
|
+
| selector | string | HTML selectors to find within your rendered Joule instance. |
|
8
|
+
|
9
|
+
|
10
|
+
#### Returns
|
11
|
+
|
12
|
+
| Type | Description |
|
13
|
+
| --- | --- |
|
14
|
+
| instance | A [Nokogiri](https://github.com/sparklemotion/nokogiri) element instance. |
|
15
|
+
|
16
|
+
|
17
|
+
## Usage
|
18
|
+
|
19
|
+
```rb
|
20
|
+
html = %Q[
|
21
|
+
<div class="pikachu">
|
22
|
+
{% include card/header/thunderbolt.html %}
|
23
|
+
<blockquote class="quote">Pika-pi</blockquote>
|
24
|
+
{% include card/footer.html %}
|
25
|
+
</div>
|
26
|
+
]
|
27
|
+
|
28
|
+
@joule.render(html)
|
29
|
+
|
30
|
+
el = @joule.find("blockquote")
|
31
|
+
```
|
32
|
+
|
33
|
+
In the above example, the variable `el` would be a Nokogiri element for:
|
34
|
+
|
35
|
+
```html
|
36
|
+
<blockquote class="quote">Pika-pi</blockquote>
|
37
|
+
```
|
38
|
+
|
39
|
+
That means that you'll be able to check the properties of this element by using methods like:
|
40
|
+
|
41
|
+
```ruby
|
42
|
+
el.text # Pika-pi
|
43
|
+
el["class"] # quote
|
44
|
+
```
|
45
|
+
|
46
|
+
### Chaining
|
47
|
+
|
48
|
+
Joule has enhanced Nokogiri's Element class by allowing you to find elements within elements.
|
49
|
+
|
50
|
+
In the example below, we're able to find the inner `.pika` selector within the outer `.pikachu` selector.
|
51
|
+
```rb
|
52
|
+
html = %Q[
|
53
|
+
<div class="pikachu">
|
54
|
+
<div class="pika">
|
55
|
+
<blockquote class="quote">
|
56
|
+
Pika-pi
|
57
|
+
</blockquote>
|
58
|
+
</div>
|
59
|
+
</div>
|
60
|
+
]
|
61
|
+
|
62
|
+
@joule.render(html)
|
63
|
+
|
64
|
+
outer = @joule.find(".pikachu")
|
65
|
+
inner = outer.find(".pika")
|
66
|
+
```
|
@@ -0,0 +1,51 @@
|
|
1
|
+
# .find_all()
|
2
|
+
|
3
|
+
#### .find_all(selector)
|
4
|
+
|
5
|
+
| Argument | Type | Description |
|
6
|
+
| --- | --- | --- |
|
7
|
+
| selector | string | HTML selectors to find within your rendered Joule instance. |
|
8
|
+
|
9
|
+
|
10
|
+
#### Returns
|
11
|
+
|
12
|
+
| Type | Description |
|
13
|
+
| --- | --- |
|
14
|
+
| array | An array of [Nokogiri](https://github.com/sparklemotion/nokogiri) element instances. |
|
15
|
+
|
16
|
+
|
17
|
+
## Usage
|
18
|
+
|
19
|
+
```rb
|
20
|
+
html = %Q[
|
21
|
+
<div class="pikachu">
|
22
|
+
<div class="spark">One</div>
|
23
|
+
<div class="spark">Two</div>
|
24
|
+
<div class="spark">Three</div>
|
25
|
+
<div class="spark">Fourteen</div>
|
26
|
+
</div>
|
27
|
+
]
|
28
|
+
|
29
|
+
@joule.render(html)
|
30
|
+
|
31
|
+
els = @joule.find_all(".spark")
|
32
|
+
```
|
33
|
+
|
34
|
+
In the above example, the variable `els` would be an Array of 4 Nokogiri elements for:
|
35
|
+
|
36
|
+
```html
|
37
|
+
<div class="spark">...</div>
|
38
|
+
```
|
39
|
+
|
40
|
+
To check the properties of an individual element from your array, you'll need to select a single element.
|
41
|
+
|
42
|
+
Example:
|
43
|
+
```ruby
|
44
|
+
els = @joule.find_all(".spark")
|
45
|
+
|
46
|
+
el = els.first
|
47
|
+
# or
|
48
|
+
el = els.last
|
49
|
+
# or
|
50
|
+
el = els[0]
|
51
|
+
```
|
data/docs/api/render.md
ADDED
@@ -0,0 +1,52 @@
|
|
1
|
+
# .render()
|
2
|
+
|
3
|
+
#### .render(content)
|
4
|
+
|
5
|
+
| Argument | Type | Description |
|
6
|
+
| --- | --- | --- |
|
7
|
+
| content | string | The HTML to be parsed and rendered by Jekyll. |
|
8
|
+
|
9
|
+
|
10
|
+
#### Returns
|
11
|
+
|
12
|
+
| Type | Description |
|
13
|
+
| --- | --- |
|
14
|
+
| instance | Joule Site instance. |
|
15
|
+
|
16
|
+
|
17
|
+
|
18
|
+
## Usage
|
19
|
+
|
20
|
+
The easiest way to pass an HTML string into `@Joule` is to use Ruby's [`%Q string interpolator](https://en.wikibooks.org/wiki/Ruby_Programming/Syntax/Literals).
|
21
|
+
|
22
|
+
Joule's render method accepts anything you'd expect Jekyll to be able to render. That includes HTML and Liquid tags, blocks, and filters (like `{% include %}`).
|
23
|
+
|
24
|
+
```rb
|
25
|
+
html = %Q[
|
26
|
+
<div class="pikachu">
|
27
|
+
{% include card/header/thunderbolt.html %}
|
28
|
+
<blockquote>Pika-pi</blockquote>
|
29
|
+
{% include card/footer.html %}
|
30
|
+
</div>
|
31
|
+
]
|
32
|
+
|
33
|
+
@joule.render(html)
|
34
|
+
```
|
35
|
+
|
36
|
+
|
37
|
+
### Markdown
|
38
|
+
|
39
|
+
Joule's `,render()` method accepts markdown as well!
|
40
|
+
|
41
|
+
```rb
|
42
|
+
markdown = %Q[
|
43
|
+
# Pikachu
|
44
|
+
{% include card/header/thunderbolt.html %}
|
45
|
+
|
46
|
+
> Pika-pi
|
47
|
+
|
48
|
+
{% include card/footer.html %}
|
49
|
+
]
|
50
|
+
|
51
|
+
@joule.render(markdown)
|
52
|
+
```
|
data/docs/rendering.md
ADDED
@@ -0,0 +1,38 @@
|
|
1
|
+
# Rendering
|
2
|
+
|
3
|
+
Joule makes it super easy to write and render HTML for Jekyll.
|
4
|
+
|
5
|
+
This allows you to inline small chunks of HTML for your unit tests. This inline technique allows you to write many tests really quickly. It also makes it really clear what you are testing.
|
6
|
+
|
7
|
+
|
8
|
+
|
9
|
+
## API
|
10
|
+
|
11
|
+
Joule comes with a handful of methods to help you render and find HTML selectors for testing:
|
12
|
+
|
13
|
+
* [.render()](./api/render.md)
|
14
|
+
* [.find()](./api/find.md)
|
15
|
+
* [.find_all()](./api/find_all.md)
|
16
|
+
|
17
|
+
|
18
|
+
|
19
|
+
## Usage
|
20
|
+
|
21
|
+
In the following example, we're going to be rendering some HTML markup along with a couple of Jekyll `{% include %}` tags. For our test, we're checking to see if the `card/header/thunderbolt.html` include does what we expect it to do.
|
22
|
+
|
23
|
+
```rb
|
24
|
+
should "render include header markup" do
|
25
|
+
html = %Q[
|
26
|
+
<div class="pikachu">
|
27
|
+
{% include card/header/thunderbolt.html %}
|
28
|
+
<blockquote class="quote">Pika-pi</blockquote>
|
29
|
+
{% include card/footer.html %}
|
30
|
+
</div>
|
31
|
+
]
|
32
|
+
@joule.render(html)
|
33
|
+
el = @joule.find(".card-header")
|
34
|
+
|
35
|
+
assert(el.text.include?("Header Yay!"))
|
36
|
+
assert(el["class"].include?("thunderbolt"))
|
37
|
+
end
|
38
|
+
```
|
data/docs/setup.md
CHANGED
@@ -4,7 +4,7 @@ The are a couple of things you need to do to get started with writing Jekyll tes
|
|
4
4
|
|
5
5
|
The following steps are based on Jekyll's own [test setup](https://github.com/jekyll/jekyll/tree/master/test). However, they've been simplified to make it easier to follow 😘.
|
6
6
|
|
7
|
-
> ##
|
7
|
+
> ## TL;DR
|
8
8
|
> You can find all of the setup code in our [examples](https://github.com/helpscout/jekyll-joule/tree/master/examples).
|
9
9
|
|
10
10
|
## Add test dependencies
|
@@ -108,4 +108,4 @@ To confirm, run the following command:
|
|
108
108
|
|
109
109
|
As long as you don't see a bunch of errors in your Terminal (😱), then you're good!
|
110
110
|
|
111
|
-
Next, let's start **[writing some tests
|
111
|
+
Next, let's start **[writing some tests](./writing-tests.md)**!
|
@@ -0,0 +1,87 @@
|
|
1
|
+
# Writing Tests
|
2
|
+
|
3
|
+
Now that we've [set up](./setup.md) our Jekyll project for testing, let's add our first test file.
|
4
|
+
|
5
|
+
> ## TL;DR
|
6
|
+
> You can find an example test in our [examples](https://github.com/helpscout/jekyll-joule/tree/master/examples/test).
|
7
|
+
|
8
|
+
## Add your test file
|
9
|
+
|
10
|
+
In your `test/` directory, add a new `.rb` with a name that describes what you're testing. Don't forget to start the file name with `test_`!
|
11
|
+
|
12
|
+
```shell
|
13
|
+
my-jekyll/
|
14
|
+
├── ...
|
15
|
+
├── test/
|
16
|
+
│ ├── helper.rb
|
17
|
+
│ └── test_yiss.rb
|
18
|
+
├── Gemfile
|
19
|
+
└── Rakefile
|
20
|
+
```
|
21
|
+
|
22
|
+
|
23
|
+
## Write your test
|
24
|
+
|
25
|
+
Copy/paste the following into your new `test_yiss.rb` file. Feel free to remove all of the Ruby comments!
|
26
|
+
|
27
|
+
```ruby
|
28
|
+
# Remember our helper.rb setup file? We'll be needing that.
|
29
|
+
require "helper"
|
30
|
+
|
31
|
+
# Name the class of your test whatever it is you'd like!
|
32
|
+
# Ideally, you'd want something that describes what you're testing.
|
33
|
+
class AwwYissTest < JekyllUnitTest
|
34
|
+
# should describes what it is you're testing
|
35
|
+
should "render a div" do
|
36
|
+
# 1. Write your markup
|
37
|
+
html = %Q[
|
38
|
+
<div class="aww">
|
39
|
+
Yiss
|
40
|
+
</div>
|
41
|
+
]
|
42
|
+
|
43
|
+
# 2. Pass it into Joule for rendering
|
44
|
+
@joule.render(html)
|
45
|
+
|
46
|
+
# 3. Find your HTML element(s)
|
47
|
+
el = @joule.find(".aww")
|
48
|
+
|
49
|
+
# 4. Write tests
|
50
|
+
assert(el)
|
51
|
+
assert(el.text.include?("Yiss"))
|
52
|
+
assert(el["class"].include?("aww"))
|
53
|
+
end
|
54
|
+
end
|
55
|
+
```
|
56
|
+
|
57
|
+
For this super simple test, all we're doing is checking if the HTML provided within the `%Q[]` tag has the text and class we expect it to have when rendered.
|
58
|
+
|
59
|
+
|
60
|
+
## Run your test
|
61
|
+
|
62
|
+
To actually run your test, run the following command (you're probably going to be doing this a lot):
|
63
|
+
|
64
|
+
`bundle exec rake test`
|
65
|
+
|
66
|
+
If all goes well, you should see something like this in your Terminal:
|
67
|
+
|
68
|
+
```
|
69
|
+
Finished in 0.05835s
|
70
|
+
1 tests, 3 assertions, 0 failures, 0 errors, 0 skips
|
71
|
+
```
|
72
|
+
|
73
|
+
And that's it! You've written your first Jekyll unit test with Minitest and Joule! 🚀
|
74
|
+
|
75
|
+
|
76
|
+
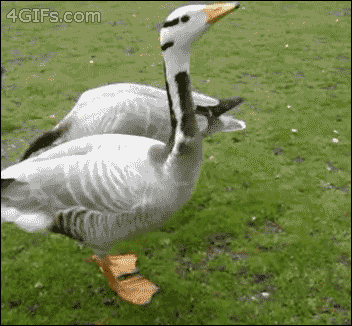<br>
|
77
|
+
_Aww yiss!_
|
78
|
+
|
79
|
+
|
80
|
+
Joule supports Jekyll plugins, `{% include %}`, Liquid filters, and all the fun things you'd expect from your Jekyll code.
|
81
|
+
|
82
|
+
Good luck and have fun testing! ✌️😎
|
83
|
+
|
84
|
+
|
85
|
+
## Wanna learn more?
|
86
|
+
|
87
|
+
To learn more about how you can use Joule, check out our **[rendering docs](./rendering.md)**.
|
@@ -13,11 +13,11 @@ class ExampleTest < JekyllUnitTest
|
|
13
13
|
@joule.render(html)
|
14
14
|
|
15
15
|
# 3. Find your HTML element(s)
|
16
|
-
el = @joule.find(".
|
16
|
+
el = @joule.find(".aww")
|
17
17
|
|
18
18
|
# 4. Write tests
|
19
19
|
assert(el)
|
20
|
-
assert(el.text.include?("
|
21
|
-
assert(el["class"].include?("
|
20
|
+
assert(el.text.include?("Yiss"))
|
21
|
+
assert(el["class"].include?("aww"))
|
22
22
|
end
|
23
23
|
end
|
data/lib/jekyll/joule/site.rb
CHANGED
@@ -36,9 +36,8 @@ module Jekyll
|
|
36
36
|
def render(content)
|
37
37
|
page = Jekyll::Joule::Page.new(@site, @site.source, "/", @test_page_name)
|
38
38
|
page.reparse(content)
|
39
|
-
page.content = %Q[<div id="#{@fixture_id}">#{page.content}</div>]
|
40
39
|
@data = generate(page)
|
41
|
-
@html = Nokogiri::HTML(@data
|
40
|
+
@html = Nokogiri::HTML(@data.content)
|
42
41
|
|
43
42
|
return self
|
44
43
|
end
|
data/lib/jekyll/joule/version.rb
CHANGED
metadata
CHANGED
@@ -1,14 +1,14 @@
|
|
1
1
|
--- !ruby/object:Gem::Specification
|
2
2
|
name: jekyll-joule
|
3
3
|
version: !ruby/object:Gem::Version
|
4
|
-
version: 0.0.
|
4
|
+
version: 0.0.4
|
5
5
|
platform: ruby
|
6
6
|
authors:
|
7
7
|
- ItsJonQ
|
8
8
|
autorequire:
|
9
9
|
bindir: exe
|
10
10
|
cert_chain: []
|
11
|
-
date: 2017-06-
|
11
|
+
date: 2017-06-05 00:00:00.000000000 Z
|
12
12
|
dependencies:
|
13
13
|
- !ruby/object:Gem::Dependency
|
14
14
|
name: jekyll
|
@@ -191,12 +191,17 @@ files:
|
|
191
191
|
- LICENSE.txt
|
192
192
|
- README.md
|
193
193
|
- Rakefile
|
194
|
+
- docs/api/find.md
|
195
|
+
- docs/api/find_all.md
|
196
|
+
- docs/api/render.md
|
194
197
|
- docs/introduction.md
|
198
|
+
- docs/rendering.md
|
195
199
|
- docs/setup.md
|
200
|
+
- docs/writing-tests.md
|
196
201
|
- examples/Gemfile
|
197
202
|
- examples/Rakefile
|
198
203
|
- examples/test/helper.rb
|
199
|
-
- examples/test/source/
|
204
|
+
- examples/test/source/index.md
|
200
205
|
- examples/test/test_example.rb
|
201
206
|
- jekyll-joule.gemspec
|
202
207
|
- lib/jekyll-joule.rb
|