input_calendar 0.0.4 → 0.1.0
This diff represents the content of publicly available package versions that have been released to one of the supported registries. The information contained in this diff is provided for informational purposes only and reflects changes between package versions as they appear in their respective public registries.
- data/README.md +5 -1
- data/lib/input_calendar/version.rb +2 -2
- data/src/calendar.coffee +4 -2
- data/vendor/assets/javascripts/input_calendar.js +55 -40
- metadata +6 -7
data/README.md
CHANGED
@@ -1,9 +1,13 @@
|
|
1
1
|
# input_calendar
|
2
2
|
|
3
|
-
At the current time
|
3
|
+
At the current time input_calendar is optimized for calendars that stay in place and are used in place (how I usually prefer a calendar in apps). No reason it couldn't be enhanced to hide/show dynamically and support visible date fields.
|
4
4
|
|
5
5
|
Interesting in patches to make it more configurable as long as it stays simple and elegant.
|
6
6
|
|
7
|
+
Here is what it looks like with the default CSS:
|
8
|
+
|
9
|
+
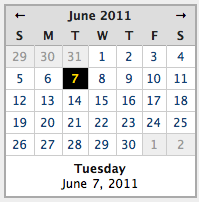
|
10
|
+
|
7
11
|
## How to use
|
8
12
|
|
9
13
|
In your view:
|
@@ -1,3 +1,3 @@
|
|
1
1
|
module InputCalendar
|
2
|
-
VERSION = "0.0
|
3
|
-
end
|
2
|
+
VERSION = "0.1.0"
|
3
|
+
end
|
data/src/calendar.coffee
CHANGED
@@ -3,7 +3,9 @@ $ = jQuery
|
|
3
3
|
class @Calendar
|
4
4
|
# only syntax takes both the id and field
|
5
5
|
constructor: (@id, @field, @options = {}) ->
|
6
|
-
|
6
|
+
# allow us to be passed in a jquery object already
|
7
|
+
unless @field.jquery
|
8
|
+
@field=$("##{@field}")
|
7
9
|
if @id?
|
8
10
|
@element=$("##{@id}")
|
9
11
|
else
|
@@ -108,7 +110,7 @@ class @Calendar
|
|
108
110
|
@field.val(@date.toLocaleDateString())
|
109
111
|
@element.find(".selected").removeClass "selected"
|
110
112
|
o.parent().addClass("selected")
|
111
|
-
@element.find(".selectedtext").html @
|
113
|
+
@element.find(".selectedtext").html @generateFooter()
|
112
114
|
if @options.onchange
|
113
115
|
@options.onchange(@field.val())
|
114
116
|
false
|
@@ -1,25 +1,25 @@
|
|
1
1
|
(function() {
|
2
2
|
var $, DateHelper, dh;
|
3
|
-
|
3
|
+
|
4
4
|
$ = jQuery;
|
5
|
+
|
5
6
|
this.Calendar = (function() {
|
7
|
+
|
6
8
|
function Calendar(id, field, options) {
|
7
9
|
var _base, _ref;
|
8
10
|
this.id = id;
|
9
11
|
this.field = field;
|
10
12
|
this.options = options != null ? options : {};
|
11
|
-
this.field = $("#" + this.field);
|
13
|
+
if (!this.field.jquery) this.field = $("#" + this.field);
|
12
14
|
if (this.id != null) {
|
13
15
|
this.element = $("#" + this.id);
|
14
16
|
} else {
|
15
17
|
this.element = $("<div>").insertAfter(this.field);
|
16
18
|
}
|
17
19
|
this.show();
|
18
|
-
|
19
|
-
_ref;
|
20
|
-
} else {
|
20
|
+
if ((_ref = (_base = this.options)["class"]) == null) {
|
21
21
|
_base["class"] = "minicalendar";
|
22
|
-
}
|
22
|
+
}
|
23
23
|
if (this.options.footer) {
|
24
24
|
this.footerTemplate = _.template(this.options.footer);
|
25
25
|
}
|
@@ -28,15 +28,16 @@
|
|
28
28
|
this.pager_date = new Date(this.date);
|
29
29
|
this.redraw();
|
30
30
|
}
|
31
|
+
|
31
32
|
Calendar.attach = function(field, options) {
|
32
33
|
return new Calendar(null, field, options);
|
33
34
|
};
|
35
|
+
|
34
36
|
Calendar.prototype.getDateFromField = function() {
|
35
|
-
if (this.field.val() === "")
|
36
|
-
return;
|
37
|
-
}
|
37
|
+
if (this.field.val() === "") return;
|
38
38
|
return this.date = this.parseIncomingDate();
|
39
39
|
};
|
40
|
+
|
40
41
|
Calendar.prototype.parseIncomingDate = function() {
|
41
42
|
var date, day, month, year, _ref;
|
42
43
|
date = this.field.val().split(" ")[0];
|
@@ -45,15 +46,19 @@
|
|
45
46
|
}), year = _ref[0], month = _ref[1], day = _ref[2];
|
46
47
|
return new Date(year, month - 1, day);
|
47
48
|
};
|
49
|
+
|
48
50
|
Calendar.prototype.show = function() {
|
49
51
|
return this.element.show();
|
50
52
|
};
|
53
|
+
|
51
54
|
Calendar.prototype.hide = function() {
|
52
55
|
return this.element.hide();
|
53
56
|
};
|
57
|
+
|
54
58
|
Calendar.prototype.label = function() {
|
55
59
|
return this.MONTHS[this.pager_date.getMonth()].label + " " + this.pager_date.getFullYear();
|
56
60
|
};
|
61
|
+
|
57
62
|
Calendar.prototype.MONTHS = [
|
58
63
|
{
|
59
64
|
label: 'January',
|
@@ -93,7 +98,9 @@
|
|
93
98
|
days: 31
|
94
99
|
}
|
95
100
|
];
|
101
|
+
|
96
102
|
Calendar.prototype.DAYS = ["Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday"];
|
103
|
+
|
97
104
|
Calendar.prototype.dayRows = function() {
|
98
105
|
var day;
|
99
106
|
return ((function() {
|
@@ -107,22 +114,26 @@
|
|
107
114
|
return _results;
|
108
115
|
}).call(this)).join("");
|
109
116
|
};
|
117
|
+
|
110
118
|
Calendar.prototype.forward = function() {
|
111
119
|
dh(this.pager_date).forward_a_month();
|
112
120
|
this.redraw();
|
113
121
|
return false;
|
114
122
|
};
|
123
|
+
|
115
124
|
Calendar.prototype.back = function() {
|
116
125
|
dh(this.pager_date).back_a_month();
|
117
126
|
this.redraw();
|
118
127
|
return false;
|
119
128
|
};
|
129
|
+
|
120
130
|
Calendar.prototype.days_in_month = function(date) {
|
121
131
|
var length, month, year, _ref;
|
122
132
|
_ref = [date.getMonth(), date.getFullYear()], month = _ref[0], year = _ref[1];
|
123
133
|
length = this.MONTHS[month].days;
|
124
134
|
return (month == 1 && (year % 4 == 0) && (year % 100 != 0)) ? 29 : length;;
|
125
135
|
};
|
136
|
+
|
126
137
|
Calendar.prototype.buildDateCells = function() {
|
127
138
|
var date, day, first_day, html, i, month, _i, _len, _ref;
|
128
139
|
date = dh(new Date(this.pager_date));
|
@@ -147,37 +158,30 @@
|
|
147
158
|
}
|
148
159
|
return html;
|
149
160
|
};
|
161
|
+
|
150
162
|
Calendar.prototype.buildDayCell = function(date, month) {
|
151
163
|
var classes;
|
152
164
|
classes = [];
|
153
165
|
classes.push("day");
|
154
|
-
if (date.getMonth() !== month)
|
155
|
-
|
156
|
-
|
157
|
-
if (date.same_as(new Date())) {
|
158
|
-
classes.push("today");
|
159
|
-
}
|
160
|
-
if (date.same_as(this.date)) {
|
161
|
-
classes.push("selected");
|
162
|
-
}
|
166
|
+
if (date.getMonth() !== month) classes.push("othermonth");
|
167
|
+
if (date.same_as(new Date())) classes.push("today");
|
168
|
+
if (date.same_as(this.date)) classes.push("selected");
|
163
169
|
return "<td class='" + (classes.join(" ")) + "'><a data-date='" + (date.toLocaleString()) + "' href='#'>" + (date.getDate()) + "</a></td>";
|
164
170
|
};
|
171
|
+
|
165
172
|
Calendar.prototype.clicked = function(event) {
|
166
173
|
var o;
|
167
174
|
o = $(event.target);
|
168
|
-
if (o[0].tagName === "TD")
|
169
|
-
o = o.children("A");
|
170
|
-
}
|
175
|
+
if (o[0].tagName === "TD") o = o.children("A");
|
171
176
|
this.date = new Date(Date.parse(o.data("date")));
|
172
177
|
this.field.val(this.date.toLocaleDateString());
|
173
178
|
this.element.find(".selected").removeClass("selected");
|
174
179
|
o.parent().addClass("selected");
|
175
|
-
this.element.find(".selectedtext").html(this.
|
176
|
-
if (this.options.onchange)
|
177
|
-
this.options.onchange(this.field.val());
|
178
|
-
}
|
180
|
+
this.element.find(".selectedtext").html(this.generateFooter());
|
181
|
+
if (this.options.onchange) this.options.onchange(this.field.val());
|
179
182
|
return false;
|
180
183
|
};
|
184
|
+
|
181
185
|
Calendar.prototype.generateFooter = function() {
|
182
186
|
var params;
|
183
187
|
if (this.footerTemplate) {
|
@@ -192,55 +196,66 @@
|
|
192
196
|
return "<b>" + this.DAYS[this.date.getDay()] + "</b><br />" + this.MONTHS[this.date.getMonth()].label + " " + (this.date.getDate()) + ", " + (this.date.getFullYear());
|
193
197
|
}
|
194
198
|
};
|
199
|
+
|
195
200
|
Calendar.prototype.redraw = function() {
|
196
201
|
var html;
|
202
|
+
var _this = this;
|
197
203
|
html = "<table class=\"" + this.options["class"] + "\" cellspacing=\"0\">\n<thead> \n <tr><th class=\"back\"><a href=\"#\">←</a></th>\n <th colspan=\"5\" class=\"month_label\">" + (this.label()) + "</th>\n <th class=\"forward\"><a href=\"#\">→</a></th></tr>\n <tr class=\"day_header\">" + (this.dayRows()) + "</tr>\n </thead>\n <tbody>" + (this.buildDateCells()) + "</tbody>\n <tfoot>\n <tr><td colspan=\"7\" class=\"selectedtext\">" + (this.generateFooter()) + "</td></tr>\n </tfoot>\n </table>";
|
198
204
|
this.element.html(html);
|
199
|
-
this.element.find("th.back").click(
|
200
|
-
return
|
201
|
-
}
|
202
|
-
this.element.find("th.forward").click(
|
203
|
-
return
|
204
|
-
}
|
205
|
-
return this.element.find("tbody").click(
|
206
|
-
return
|
207
|
-
}
|
205
|
+
this.element.find("th.back").click(function() {
|
206
|
+
return _this.back();
|
207
|
+
});
|
208
|
+
this.element.find("th.forward").click(function() {
|
209
|
+
return _this.forward();
|
210
|
+
});
|
211
|
+
return this.element.find("tbody").click(function(event) {
|
212
|
+
return _this.clicked(event);
|
213
|
+
});
|
208
214
|
};
|
215
|
+
|
209
216
|
return Calendar;
|
217
|
+
|
210
218
|
})();
|
219
|
+
|
211
220
|
dh = function(date) {
|
212
221
|
_.extend(date, DateHelper);
|
213
222
|
return date;
|
214
223
|
};
|
224
|
+
|
215
225
|
DateHelper = (function() {
|
226
|
+
|
216
227
|
function DateHelper() {}
|
228
|
+
|
217
229
|
DateHelper.forward_a_month = function(count) {
|
218
230
|
var d, month;
|
219
|
-
if (count == null)
|
220
|
-
count = 1;
|
221
|
-
}
|
231
|
+
if (count == null) count = 1;
|
222
232
|
month = this.getMonth() + count;
|
223
233
|
d = new Date(this.getFullYear(), month, 1);
|
224
234
|
return this.setTime(d);
|
225
235
|
};
|
236
|
+
|
226
237
|
DateHelper.back_a_month = function(count) {
|
227
238
|
var d, month;
|
228
|
-
if (count == null)
|
229
|
-
count = 1;
|
230
|
-
}
|
239
|
+
if (count == null) count = 1;
|
231
240
|
month = this.getMonth() - count;
|
232
241
|
d = new Date(this.getFullYear(), month, 1);
|
233
242
|
return this.setTime(d);
|
234
243
|
};
|
244
|
+
|
235
245
|
DateHelper.same_as = function(date) {
|
236
246
|
return this.getFullYear() === date.getFullYear() && this.getMonth() === date.getMonth() && this.getDate() === date.getDate();
|
237
247
|
};
|
248
|
+
|
238
249
|
DateHelper.go_tomorrow = function() {
|
239
250
|
return this.setTime(new Date(this.valueOf() + 1000 * 60 * 60 * 24));
|
240
251
|
};
|
252
|
+
|
241
253
|
DateHelper.go_yesterday = function() {
|
242
254
|
return this.setTime(new Date(this.valueOf() - 1000 * 60 * 60 * 24));
|
243
255
|
};
|
256
|
+
|
244
257
|
return DateHelper;
|
258
|
+
|
245
259
|
})();
|
260
|
+
|
246
261
|
}).call(this);
|
metadata
CHANGED
@@ -1,13 +1,13 @@
|
|
1
1
|
--- !ruby/object:Gem::Specification
|
2
2
|
name: input_calendar
|
3
3
|
version: !ruby/object:Gem::Version
|
4
|
-
hash:
|
4
|
+
hash: 27
|
5
5
|
prerelease:
|
6
6
|
segments:
|
7
7
|
- 0
|
8
|
+
- 1
|
8
9
|
- 0
|
9
|
-
|
10
|
-
version: 0.0.4
|
10
|
+
version: 0.1.0
|
11
11
|
platform: ruby
|
12
12
|
authors:
|
13
13
|
- Josh Goebel
|
@@ -15,8 +15,7 @@ autorequire:
|
|
15
15
|
bindir: bin
|
16
16
|
cert_chain: []
|
17
17
|
|
18
|
-
date:
|
19
|
-
default_executable:
|
18
|
+
date: 2012-05-09 00:00:00 Z
|
20
19
|
dependencies: []
|
21
20
|
|
22
21
|
description: Simple and clean JS calendar to enter dates on forms
|
@@ -45,7 +44,6 @@ files:
|
|
45
44
|
- vendor/assets/stylesheets/input_calendar.css
|
46
45
|
- vendor/support/jquery.js
|
47
46
|
- vendor/support/underscore.js
|
48
|
-
has_rdoc: true
|
49
47
|
homepage: http://github.com/yyyc514/input_calendar
|
50
48
|
licenses: []
|
51
49
|
|
@@ -75,9 +73,10 @@ required_rubygems_version: !ruby/object:Gem::Requirement
|
|
75
73
|
requirements: []
|
76
74
|
|
77
75
|
rubyforge_project: input_calendar
|
78
|
-
rubygems_version: 1.
|
76
|
+
rubygems_version: 1.8.15
|
79
77
|
signing_key:
|
80
78
|
specification_version: 3
|
81
79
|
summary: Simple and clean JS calendar to enter dates on forms
|
82
80
|
test_files: []
|
83
81
|
|
82
|
+
has_rdoc:
|