html5_validators 1.1.3 → 1.2.0
Sign up to get free protection for your applications and to get access to all the features.
- checksums.yaml +4 -4
- data/.travis.yml +4 -4
- data/README.md +201 -0
- data/html5_validators.gemspec +1 -0
- data/lib/html5_validators.rb +10 -0
- data/lib/html5_validators/action_view/form_helpers.rb +9 -5
- data/lib/html5_validators/version.rb +1 -1
- data/spec/fake_app.rb +10 -0
- data/spec/features/validation_spec.rb +20 -0
- data/spec/spec_helper.rb +4 -0
- metadata +5 -4
- data/README.rdoc +0 -149
checksums.yaml
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
---
|
2
2
|
SHA1:
|
3
|
-
metadata.gz:
|
4
|
-
data.tar.gz:
|
3
|
+
metadata.gz: 53351810292bd43edac8e3b5ce7a32981d4bf2ea
|
4
|
+
data.tar.gz: 2e86f48bbb046c3d2a586330991817bf59790442
|
5
5
|
SHA512:
|
6
|
-
metadata.gz:
|
7
|
-
data.tar.gz:
|
6
|
+
metadata.gz: f945e1ad923ebd7c2de76b926365d7c892e704e536c3d82bd7fe96f01fcc8fe8d84a5e84b5182f0e63362fb035ca3fc8fa347eaa778b240bc631e52bea95b172
|
7
|
+
data.tar.gz: 201accfc19d6e505bbdb6a97a02fc9658c10be1e231c044b460ca89df1078ab767af2d2a7408aeb1c7400ca7645021ce0c76499815350d685c9ce8f988c957ca
|
data/.travis.yml
CHANGED
@@ -5,7 +5,7 @@ rvm:
|
|
5
5
|
- 1.9.3
|
6
6
|
- 2.0.0
|
7
7
|
- 2.1
|
8
|
-
- 2.2.
|
8
|
+
- 2.2.2
|
9
9
|
- ruby-head
|
10
10
|
|
11
11
|
gemfile:
|
@@ -35,11 +35,11 @@ matrix:
|
|
35
35
|
gemfile: gemfiles/Gemfile.rails-edge
|
36
36
|
- rvm: 2.1
|
37
37
|
gemfile: gemfiles/Gemfile.rails-edge
|
38
|
-
- rvm: 2.2.
|
38
|
+
- rvm: 2.2.2
|
39
39
|
gemfile: gemfiles/Gemfile.rails-3.0
|
40
|
-
- rvm: 2.2.
|
40
|
+
- rvm: 2.2.2
|
41
41
|
gemfile: gemfiles/Gemfile.rails-3.1
|
42
|
-
- rvm: 2.2.
|
42
|
+
- rvm: 2.2.2
|
43
43
|
gemfile: gemfiles/Gemfile.rails-3.2
|
44
44
|
- rvm: ruby-head
|
45
45
|
gemfile: gemfiles/Gemfile.rails-3.0
|
data/README.md
ADDED
@@ -0,0 +1,201 @@
|
|
1
|
+
# HTML5Validators
|
2
|
+
|
3
|
+
Automatic client-side validation using HTML5 Form Validation
|
4
|
+
|
5
|
+
## What is this?
|
6
|
+
|
7
|
+
html5_validators is a gem/plugin for Rails 3+ that enables automatic client-side
|
8
|
+
validation using ActiveModel + HTML5. Once you bundle this gem on your app,
|
9
|
+
the gem will automatically translate your model validation code into HTML5
|
10
|
+
validation attributes on every `form_for` invocation unless you explicitly
|
11
|
+
cancel it.
|
12
|
+
|
13
|
+
## Features
|
14
|
+
|
15
|
+
### PresenceValidator => required
|
16
|
+
|
17
|
+
* Model
|
18
|
+
```ruby
|
19
|
+
class User
|
20
|
+
include ActiveModel::Validations
|
21
|
+
validates_presence_of :name
|
22
|
+
end
|
23
|
+
```
|
24
|
+
|
25
|
+
* View
|
26
|
+
```erb
|
27
|
+
<%= f.text_field :name %>
|
28
|
+
```
|
29
|
+
other `text_field`ish helpers, `text_area`, `radio_button`, and `check_box` are also available
|
30
|
+
|
31
|
+
* HTML
|
32
|
+
```html
|
33
|
+
<input id="user_name" name="user[name]" required="required" type="text" />
|
34
|
+
```
|
35
|
+
|
36
|
+
* SPEC
|
37
|
+
|
38
|
+
http://dev.w3.org/html5/spec/Overview.html#attr-input-required
|
39
|
+
|
40
|
+
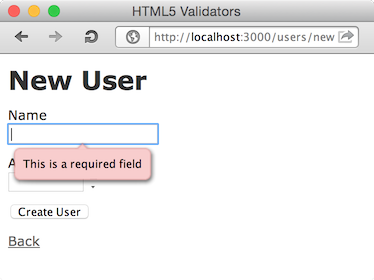
|
41
|
+
|
42
|
+
### LengthValidator => maxlength
|
43
|
+
|
44
|
+
* Model
|
45
|
+
```ruby
|
46
|
+
class User
|
47
|
+
include ActiveModel::Validations
|
48
|
+
validates_length_of :name, maximum: 10
|
49
|
+
end
|
50
|
+
```
|
51
|
+
|
52
|
+
* View
|
53
|
+
```erb
|
54
|
+
<%= f.text_field :name %>
|
55
|
+
```
|
56
|
+
`text_area` is also available
|
57
|
+
|
58
|
+
* HTML
|
59
|
+
```html
|
60
|
+
<input id="user_name" maxlength="10" name="user[name]" size="10" type="text" />
|
61
|
+
```
|
62
|
+
|
63
|
+
* SPEC
|
64
|
+
|
65
|
+
http://dev.w3.org/html5/spec/Overview.html#attr-input-maxlength
|
66
|
+
|
67
|
+
### NumericalityValidator => max, min
|
68
|
+
|
69
|
+
* Model
|
70
|
+
```ruby
|
71
|
+
class User
|
72
|
+
include ActiveModel::Validations
|
73
|
+
validates_numericality_of :age, greater_than_or_equal_to: 20
|
74
|
+
end
|
75
|
+
```
|
76
|
+
|
77
|
+
* View (be sure to use number_field)
|
78
|
+
```erb
|
79
|
+
<%= f.number_field :age %>
|
80
|
+
```
|
81
|
+
|
82
|
+
* HTML
|
83
|
+
```html
|
84
|
+
<input id="user_age" min="20" name="user[age]" size="30" type="number" />
|
85
|
+
```
|
86
|
+
|
87
|
+
* SPEC
|
88
|
+
|
89
|
+
http://dev.w3.org/html5/spec/Overview.html#attr-input-max
|
90
|
+
http://dev.w3.org/html5/spec/Overview.html#attr-input-min
|
91
|
+
|
92
|
+
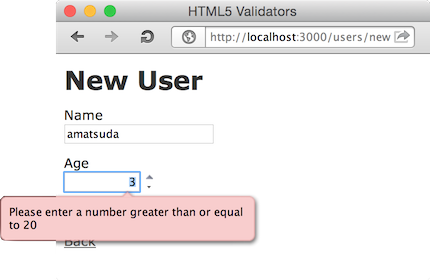
|
93
|
+
|
94
|
+
### And more (coming soon...?)
|
95
|
+
:construction:
|
96
|
+
|
97
|
+
## Disabling automatic client-side validation
|
98
|
+
|
99
|
+
There are four ways to cancel the automatic HTML5 validation.
|
100
|
+
|
101
|
+
### 1. Per form (via form_for option)
|
102
|
+
|
103
|
+
Set `auto_html5_validation: false` to `form_for` parameter.
|
104
|
+
|
105
|
+
* View
|
106
|
+
```erb
|
107
|
+
<%= form_for @user, auto_html5_validation: false do |f| %>
|
108
|
+
...
|
109
|
+
<% end %>
|
110
|
+
```
|
111
|
+
|
112
|
+
### 2. Per model instance (via model attribute)
|
113
|
+
|
114
|
+
Set `auto_html5_validation = false` attribute to ActiveModelish object.
|
115
|
+
|
116
|
+
* Controller
|
117
|
+
```ruby
|
118
|
+
@user = User.new auto_html5_validation: false
|
119
|
+
```
|
120
|
+
|
121
|
+
* View
|
122
|
+
```erb
|
123
|
+
<%= form_for @user do |f| %>
|
124
|
+
...
|
125
|
+
<% end %>
|
126
|
+
```
|
127
|
+
|
128
|
+
### 3. Per model class (via model class attribute)
|
129
|
+
|
130
|
+
Set `auto_html5_validation = false` to ActiveModelish class variable.
|
131
|
+
|
132
|
+
* Model
|
133
|
+
```ruby
|
134
|
+
class User < ActiveRecord::Base
|
135
|
+
self.auto_html5_validation = false
|
136
|
+
end
|
137
|
+
```
|
138
|
+
|
139
|
+
* Controller
|
140
|
+
```ruby
|
141
|
+
@user = User.new
|
142
|
+
```
|
143
|
+
|
144
|
+
* View
|
145
|
+
```erb
|
146
|
+
<%= form_for @user do |f| %>
|
147
|
+
...
|
148
|
+
<% end %>
|
149
|
+
```
|
150
|
+
|
151
|
+
### 4. Globally (via HTML5Validators module configuration)
|
152
|
+
|
153
|
+
Set `config.enabled = false` to Html5Validators module.
|
154
|
+
Maybe you want to put this in your test_helper, or add a controller filter as
|
155
|
+
follows for development mode.
|
156
|
+
|
157
|
+
* Controller
|
158
|
+
```ruby
|
159
|
+
# an example filter that disables the validator if the request has {h5v: 'disable'} params
|
160
|
+
around_action do |controller, block|
|
161
|
+
h5v_enabled_was = Html5Validators.enabled
|
162
|
+
Html5Validators.enabled = false if params[:h5v] == 'disable'
|
163
|
+
block.call
|
164
|
+
Html5Validators.enabled = h5v_enabled_was
|
165
|
+
end
|
166
|
+
```
|
167
|
+
|
168
|
+
## Supported versions
|
169
|
+
|
170
|
+
* Ruby 1.8.7, 1.9.2, 1.9.3, 2.0, 2.1, 2.2, 2.3 (trunk)
|
171
|
+
|
172
|
+
* Rails 3.0.x, 3.1.x, 3.2.x, 4.0.x, 4.1, 4.2, 5.0 (edge)
|
173
|
+
|
174
|
+
* HTML5 compatible browsers
|
175
|
+
|
176
|
+
|
177
|
+
## Installation
|
178
|
+
|
179
|
+
Put this line into your Gemfile:
|
180
|
+
```ruby
|
181
|
+
gem 'html5_validators'
|
182
|
+
```
|
183
|
+
|
184
|
+
Then bundle:
|
185
|
+
```
|
186
|
+
% bundle
|
187
|
+
```
|
188
|
+
|
189
|
+
## Notes
|
190
|
+
|
191
|
+
When accessed by an HTML5 incompatible lagacy browser, these extra attributes
|
192
|
+
will just be ignored.
|
193
|
+
|
194
|
+
## Todo
|
195
|
+
|
196
|
+
* more validations
|
197
|
+
|
198
|
+
|
199
|
+
## Copyright
|
200
|
+
|
201
|
+
Copyright (c) 2011 Akira Matsuda. See MIT-LICENSE for further details.
|
data/html5_validators.gemspec
CHANGED
@@ -11,6 +11,7 @@ Gem::Specification.new do |s|
|
|
11
11
|
s.homepage = 'https://github.com/amatsuda/html5_validators'
|
12
12
|
s.summary = 'Automatic client side validation using HTML5 Form Validation'
|
13
13
|
s.description = 'A gem/plugin for Rails 3+ that enables client-side validation using ActiveModel + HTML5'
|
14
|
+
s.license = 'MIT'
|
14
15
|
|
15
16
|
s.rubyforge_project = 'html5_validators'
|
16
17
|
|
data/lib/html5_validators.rb
CHANGED
@@ -1,6 +1,16 @@
|
|
1
1
|
require 'rails'
|
2
2
|
|
3
3
|
module Html5Validators
|
4
|
+
@enabled = true
|
5
|
+
|
6
|
+
def self.enabled
|
7
|
+
@enabled
|
8
|
+
end
|
9
|
+
|
10
|
+
def self.enabled=(enable)
|
11
|
+
@enabled = enable
|
12
|
+
end
|
13
|
+
|
4
14
|
class Railtie < ::Rails::Railtie #:nodoc:
|
5
15
|
initializer 'html5_validators' do |app|
|
6
16
|
ActiveSupport.on_load(:active_record) do
|
@@ -2,13 +2,13 @@ module Html5Validators
|
|
2
2
|
module ActionViewExtension
|
3
3
|
def inject_required_field
|
4
4
|
if object.class.ancestors.include?(ActiveModel::Validations) && (object.auto_html5_validation != false) && (object.class.auto_html5_validation != false)
|
5
|
-
@options["required"] ||= object.class.attribute_required?(@method_name)
|
5
|
+
@options["required"] ||= @options[:required] || object.class.attribute_required?(@method_name)
|
6
6
|
end
|
7
7
|
end
|
8
8
|
|
9
9
|
def inject_maxlength_field
|
10
10
|
if object.class.ancestors.include?(ActiveModel::Validations) && (object.auto_html5_validation != false) && (object.class.auto_html5_validation != false)
|
11
|
-
@options["maxlength"] ||= object.class.attribute_maxlength(@method_name)
|
11
|
+
@options["maxlength"] ||= @options[:maxlength] || object.class.attribute_maxlength(@method_name)
|
12
12
|
end
|
13
13
|
end
|
14
14
|
end
|
@@ -19,7 +19,11 @@ module ActionView
|
|
19
19
|
module Helpers
|
20
20
|
module FormHelper
|
21
21
|
def form_for_with_auto_html5_validation_option(record, options = {}, &proc)
|
22
|
-
|
22
|
+
if record.respond_to?(:auto_html5_validation=)
|
23
|
+
if !Html5Validators.enabled || (options[:auto_html5_validation] == false)
|
24
|
+
record.auto_html5_validation = false
|
25
|
+
end
|
26
|
+
end
|
23
27
|
form_for_without_auto_html5_validation_option record, options, &proc
|
24
28
|
end
|
25
29
|
alias_method_chain :form_for, :auto_html5_validation_option
|
@@ -37,8 +41,8 @@ module ActionView
|
|
37
41
|
inject_maxlength_field
|
38
42
|
|
39
43
|
if object.class.ancestors.include?(ActiveModel::Validations) && (object.auto_html5_validation != false) && (object.class.auto_html5_validation != false)
|
40
|
-
@options["max"] ||= object.class.attribute_max(@method_name)
|
41
|
-
@options["min"] ||= object.class.attribute_min(@method_name)
|
44
|
+
@options["max"] ||= @options["maxlength"] || @options[:maxlength] || object.class.attribute_max(@method_name)
|
45
|
+
@options["min"] ||= @options["minlength"] || @options[:minlength] || object.class.attribute_min(@method_name)
|
42
46
|
end
|
43
47
|
render_without_html5_attributes
|
44
48
|
end
|
data/spec/fake_app.rb
CHANGED
@@ -16,6 +16,7 @@ app.routes.draw do
|
|
16
16
|
resources :people, :only => [:new, :create] do
|
17
17
|
collection do
|
18
18
|
get :new_without_html5_validation
|
19
|
+
get :new_with_required_true
|
19
20
|
end
|
20
21
|
end
|
21
22
|
end
|
@@ -46,6 +47,15 @@ ERB
|
|
46
47
|
<% end %>
|
47
48
|
ERB
|
48
49
|
end
|
50
|
+
|
51
|
+
def new_with_required_true
|
52
|
+
@person = Person.new
|
53
|
+
render :inline => <<-ERB
|
54
|
+
<%= form_for @person do |f| %>
|
55
|
+
<%= f.text_field :email, :required => true %>
|
56
|
+
<% end %>
|
57
|
+
ERB
|
58
|
+
end
|
49
59
|
end
|
50
60
|
|
51
61
|
# helpers
|
@@ -33,6 +33,11 @@ feature 'person#new' do
|
|
33
33
|
|
34
34
|
find('input#person_name')[:required].should be_nil
|
35
35
|
end
|
36
|
+
scenario 'new_with_required_true form' do
|
37
|
+
visit '/people/new_with_required_true'
|
38
|
+
|
39
|
+
find('input#person_email')[:required].should == 'required'
|
40
|
+
end
|
36
41
|
|
37
42
|
context 'disabling html5_validation in class level' do
|
38
43
|
background do
|
@@ -51,6 +56,21 @@ feature 'person#new' do
|
|
51
56
|
find('input#person_name')[:required].should be_nil
|
52
57
|
end
|
53
58
|
end
|
59
|
+
|
60
|
+
context 'disabling html5_validations in gem' do
|
61
|
+
background do
|
62
|
+
Html5Validators.enabled = false
|
63
|
+
end
|
64
|
+
after do
|
65
|
+
Html5Validators.enabled = true
|
66
|
+
end
|
67
|
+
scenario 'new form' do
|
68
|
+
visit '/people/new'
|
69
|
+
|
70
|
+
find('input#person_name')[:required].should be_nil
|
71
|
+
find('textarea#person_bio')[:required].should be_nil
|
72
|
+
end
|
73
|
+
end
|
54
74
|
end
|
55
75
|
|
56
76
|
context 'with maxlength validation' do
|
data/spec/spec_helper.rb
CHANGED
@@ -11,6 +11,10 @@ require 'rspec/rails'
|
|
11
11
|
Dir["#{File.dirname(__FILE__)}/support/**/*.rb"].each {|f| require f}
|
12
12
|
|
13
13
|
RSpec.configure do |config|
|
14
|
+
if config.respond_to? :expect_with
|
15
|
+
config.expect_with(:rspec) { |c| c.syntax = :should }
|
16
|
+
end
|
17
|
+
|
14
18
|
config.before :all do
|
15
19
|
ActiveRecord::Migration.verbose = false
|
16
20
|
CreateAllTables.up unless ActiveRecord::Base.connection.table_exists? 'people'
|
metadata
CHANGED
@@ -1,14 +1,14 @@
|
|
1
1
|
--- !ruby/object:Gem::Specification
|
2
2
|
name: html5_validators
|
3
3
|
version: !ruby/object:Gem::Version
|
4
|
-
version: 1.
|
4
|
+
version: 1.2.0
|
5
5
|
platform: ruby
|
6
6
|
authors:
|
7
7
|
- Akira Matsuda
|
8
8
|
autorequire:
|
9
9
|
bindir: bin
|
10
10
|
cert_chain: []
|
11
|
-
date: 2015-
|
11
|
+
date: 2015-05-10 00:00:00.000000000 Z
|
12
12
|
dependencies:
|
13
13
|
- !ruby/object:Gem::Dependency
|
14
14
|
name: rspec-rails
|
@@ -79,7 +79,7 @@ files:
|
|
79
79
|
- ".travis.yml"
|
80
80
|
- Gemfile
|
81
81
|
- MIT-LICENSE
|
82
|
-
- README.
|
82
|
+
- README.md
|
83
83
|
- Rakefile
|
84
84
|
- gemfiles/Gemfile.rails-3.0
|
85
85
|
- gemfiles/Gemfile.rails-3.1
|
@@ -101,7 +101,8 @@ files:
|
|
101
101
|
- spec/models/active_record/inherited_spec.rb
|
102
102
|
- spec/spec_helper.rb
|
103
103
|
homepage: https://github.com/amatsuda/html5_validators
|
104
|
-
licenses:
|
104
|
+
licenses:
|
105
|
+
- MIT
|
105
106
|
metadata: {}
|
106
107
|
post_install_message:
|
107
108
|
rdoc_options: []
|
data/README.rdoc
DELETED
@@ -1,149 +0,0 @@
|
|
1
|
-
= HTML5Validators
|
2
|
-
|
3
|
-
Automatic client-side validation using HTML5 Form Validation
|
4
|
-
|
5
|
-
|
6
|
-
== What is this?
|
7
|
-
|
8
|
-
html5_validators is a gem/plugin for Rails 3 that enables client-side validation using ActiveModel + HTML5.
|
9
|
-
Once you bundle this gem on your app, the gem will automatically translate your model validation code into HTML5 validation attributes on every `form_for` invocation unless you explicitly cancel it.
|
10
|
-
|
11
|
-
|
12
|
-
== Features
|
13
|
-
|
14
|
-
=== PresenceValidator => requried
|
15
|
-
|
16
|
-
Model:
|
17
|
-
class User
|
18
|
-
include ActiveModel::Validations
|
19
|
-
validates_presence_of :name
|
20
|
-
end
|
21
|
-
|
22
|
-
View:
|
23
|
-
<%= f.text_field :name %>
|
24
|
-
other text_fieldish helpers, text_area, radio_button, and check_box are also available
|
25
|
-
|
26
|
-
HTML:
|
27
|
-
<input id="user_name" name="user[name]" required="required" type="text" />
|
28
|
-
|
29
|
-
SPEC:
|
30
|
-
http://dev.w3.org/html5/spec/Overview.html#attr-input-required
|
31
|
-
|
32
|
-
http://img.skitch.com/20110517-8sagqrkjnmkinapmcc5tduy2b8.jpg
|
33
|
-
|
34
|
-
=== LengthValidator => maxlength
|
35
|
-
|
36
|
-
Model:
|
37
|
-
class User
|
38
|
-
include ActiveModel::Validations
|
39
|
-
validates_length_of :name, :maximum => 10
|
40
|
-
end
|
41
|
-
|
42
|
-
View:
|
43
|
-
<%= f.text_field :name %>
|
44
|
-
text_area is also available
|
45
|
-
|
46
|
-
HTML:
|
47
|
-
<input id="user_name" maxlength="10" name="user[name]" size="10" type="text" />
|
48
|
-
|
49
|
-
SPEC:
|
50
|
-
http://dev.w3.org/html5/spec/Overview.html#attr-input-maxlength
|
51
|
-
|
52
|
-
=== NumericalityValidator => max, min
|
53
|
-
|
54
|
-
Model:
|
55
|
-
class User
|
56
|
-
include ActiveModel::Validations
|
57
|
-
validates_numericality_of :age, :greater_than_or_equal_to => 20
|
58
|
-
end
|
59
|
-
|
60
|
-
View: (be sure to use number_field)
|
61
|
-
<%= f.number_field :age %>
|
62
|
-
|
63
|
-
HTML:
|
64
|
-
<input id="user_age" min="20" name="user[age]" size="30" type="number" />
|
65
|
-
|
66
|
-
SPEC:
|
67
|
-
http://dev.w3.org/html5/spec/Overview.html#attr-input-max
|
68
|
-
http://dev.w3.org/html5/spec/Overview.html#attr-input-min
|
69
|
-
|
70
|
-
http://img.skitch.com/20110516-n3jhu5m4gan8iy1j8er1qb7yfa.jpg
|
71
|
-
|
72
|
-
|
73
|
-
* And more (coming soon...?)
|
74
|
-
|
75
|
-
|
76
|
-
== Disabling automatic client-side validation
|
77
|
-
|
78
|
-
There are three ways to cancel the automatic HTML5 validation
|
79
|
-
|
80
|
-
* form_for option
|
81
|
-
|
82
|
-
Set `auto_html5_validation: false` to `form_for` parameter
|
83
|
-
|
84
|
-
View:
|
85
|
-
<%= form_for @user, :auto_html5_validation => false do |f| %>
|
86
|
-
...
|
87
|
-
<% end %>
|
88
|
-
|
89
|
-
* model attribute
|
90
|
-
|
91
|
-
Set `auto_html5_validation = false` attribute to ActiveModelish object
|
92
|
-
|
93
|
-
Controller:
|
94
|
-
@user = User.new auto_html5_validation: false
|
95
|
-
|
96
|
-
View:
|
97
|
-
<%= form_for @user do |f| %>
|
98
|
-
...
|
99
|
-
<% end %>
|
100
|
-
|
101
|
-
* model class configuration
|
102
|
-
|
103
|
-
Write `auto_html5_validation = false` in ActiveModelish class
|
104
|
-
|
105
|
-
Model:
|
106
|
-
class User < ActiveRecord::Base
|
107
|
-
self.auto_html5_validation = false
|
108
|
-
end
|
109
|
-
|
110
|
-
Controller:
|
111
|
-
@user = User.new
|
112
|
-
|
113
|
-
View:
|
114
|
-
<%= form_for @user do |f| %>
|
115
|
-
...
|
116
|
-
<% end %>
|
117
|
-
|
118
|
-
|
119
|
-
== Supported versions
|
120
|
-
|
121
|
-
* Ruby 1.8.7, 1.9.2, 1.9.3, 2.0, 2.1, 2.2, 2.3 (trunk)
|
122
|
-
|
123
|
-
* Rails 3.0.x, 3.1.x, 3.2.x, 4.0.x, 4.1, 4.2, 5.0 (edge)
|
124
|
-
|
125
|
-
* HTML5 compatible browsers
|
126
|
-
|
127
|
-
|
128
|
-
== Installation
|
129
|
-
|
130
|
-
Put this line into your Gemfile:
|
131
|
-
gem 'html5_validators'
|
132
|
-
|
133
|
-
Then bundle:
|
134
|
-
% bundle
|
135
|
-
|
136
|
-
|
137
|
-
== Notes
|
138
|
-
|
139
|
-
When accessed by an HTML5 incompatible lagacy browser, these extra attributes will just be ignored.
|
140
|
-
|
141
|
-
|
142
|
-
== Todo
|
143
|
-
|
144
|
-
* more validations
|
145
|
-
|
146
|
-
|
147
|
-
== Copyright
|
148
|
-
|
149
|
-
Copyright (c) 2011 Akira Matsuda. See MIT-LICENSE for further details.
|