gollum_editor 0.1
Sign up to get free protection for your applications and to get access to all the features.
- data/lib/assets/images/gollum_editor/icon-sprite.png +0 -0
- data/lib/assets/javascripts/gollum_editor/gollum.dialog.js +263 -0
- data/lib/assets/javascripts/gollum_editor/gollum.editor.js +1072 -0
- data/lib/assets/javascripts/gollum_editor/gollum.js +10 -0
- data/lib/assets/javascripts/gollum_editor/gollum.placeholder.js +54 -0
- data/lib/assets/javascripts/gollum_editor/jquery.color.js +123 -0
- data/lib/assets/javascripts/gollum_editor/langs/markdown.js +211 -0
- data/lib/assets/stylesheets/gollum_editor/dialog.css +141 -0
- data/lib/assets/stylesheets/gollum_editor/editor.css +537 -0
- data/lib/assets/stylesheets/gollum_editor/gollum.css +4 -0
- data/lib/generators/gollum_editor/install_generator.rb +13 -0
- data/lib/gollum_editor.rb +3 -0
- data/lib/gollum_editor/form_builder.rb +5 -0
- data/lib/gollum_editor/form_helper.rb +74 -0
- data/lib/gollum_editor/version.rb +3 -0
- metadata +60 -0
@@ -0,0 +1,10 @@
|
|
1
|
+
//
|
2
|
+
//= require gollum_editor/jquery.color
|
3
|
+
//= require gollum_editor/gollum.placeholder
|
4
|
+
//= require gollum_editor/gollum.dialog
|
5
|
+
//= require gollum_editor/gollum.editor
|
6
|
+
//= require gollum_editor/langs/markdown
|
7
|
+
|
8
|
+
$(document).ready(function() {
|
9
|
+
$.GollumEditor();
|
10
|
+
});
|
@@ -0,0 +1,54 @@
|
|
1
|
+
(function($) {
|
2
|
+
|
3
|
+
var Placeholder = {
|
4
|
+
|
5
|
+
_PLACEHOLDERS : [],
|
6
|
+
|
7
|
+
_p : function( $field ) {
|
8
|
+
|
9
|
+
this.fieldObject = $field;
|
10
|
+
this.placeholderText = $field.val();
|
11
|
+
var placeholderText = $field.val();
|
12
|
+
|
13
|
+
$field.addClass('ph');
|
14
|
+
|
15
|
+
$field.blur(function() {
|
16
|
+
if ( $(this).val() == '' ) {
|
17
|
+
$(this).val( placeholderText );
|
18
|
+
$(this).addClass('ph');
|
19
|
+
}
|
20
|
+
});
|
21
|
+
|
22
|
+
$field.focus(function() {
|
23
|
+
$(this).removeClass('ph');
|
24
|
+
if ( $(this).val() == placeholderText ) {
|
25
|
+
$(this).val('');
|
26
|
+
} else {
|
27
|
+
$(this)[0].select();
|
28
|
+
}
|
29
|
+
});
|
30
|
+
|
31
|
+
},
|
32
|
+
|
33
|
+
add : function( $field ) {
|
34
|
+
Placeholder._PLACEHOLDERS.push( new Placeholder._p( $field ) );
|
35
|
+
},
|
36
|
+
|
37
|
+
clearAll: function() {
|
38
|
+
for ( var i=0; i < Placeholder._PLACEHOLDERS.length; i++ ) {
|
39
|
+
if ( Placeholder._PLACEHOLDERS[i].fieldObject.val() ==
|
40
|
+
Placeholder._PLACEHOLDERS[i].placeholderText ) {
|
41
|
+
Placeholder._PLACEHOLDERS[i].fieldObject.val('');
|
42
|
+
}
|
43
|
+
}
|
44
|
+
},
|
45
|
+
|
46
|
+
exists : function() {
|
47
|
+
return ( _PLACEHOLDERS.length );
|
48
|
+
}
|
49
|
+
|
50
|
+
};
|
51
|
+
|
52
|
+
$.GollumPlaceholder = Placeholder;
|
53
|
+
|
54
|
+
})(jQuery);
|
@@ -0,0 +1,123 @@
|
|
1
|
+
/*
|
2
|
+
* jQuery Color Animations
|
3
|
+
* Copyright 2007 John Resig
|
4
|
+
* Released under the MIT and GPL licenses.
|
5
|
+
*/
|
6
|
+
|
7
|
+
(function(jQuery){
|
8
|
+
|
9
|
+
// We override the animation for all of these color styles
|
10
|
+
jQuery.each(['backgroundColor', 'borderBottomColor', 'borderLeftColor', 'borderRightColor', 'borderTopColor', 'color', 'outlineColor'], function(i,attr){
|
11
|
+
jQuery.fx.step[attr] = function(fx){
|
12
|
+
if ( fx.state == 0 ) {
|
13
|
+
fx.start = getColor( fx.elem, attr );
|
14
|
+
fx.end = getRGB( fx.end );
|
15
|
+
}
|
16
|
+
|
17
|
+
fx.elem.style[attr] = "rgb(" + [
|
18
|
+
Math.max(Math.min( parseInt((fx.pos * (fx.end[0] - fx.start[0])) + fx.start[0]), 255), 0),
|
19
|
+
Math.max(Math.min( parseInt((fx.pos * (fx.end[1] - fx.start[1])) + fx.start[1]), 255), 0),
|
20
|
+
Math.max(Math.min( parseInt((fx.pos * (fx.end[2] - fx.start[2])) + fx.start[2]), 255), 0)
|
21
|
+
].join(",") + ")";
|
22
|
+
}
|
23
|
+
});
|
24
|
+
|
25
|
+
// Color Conversion functions from highlightFade
|
26
|
+
// By Blair Mitchelmore
|
27
|
+
// http://jquery.offput.ca/highlightFade/
|
28
|
+
|
29
|
+
// Parse strings looking for color tuples [255,255,255]
|
30
|
+
function getRGB(color) {
|
31
|
+
var result;
|
32
|
+
|
33
|
+
// Check if we're already dealing with an array of colors
|
34
|
+
if ( color && color.constructor == Array && color.length == 3 )
|
35
|
+
return color;
|
36
|
+
|
37
|
+
// Look for rgb(num,num,num)
|
38
|
+
if (result = /rgb\(\s*([0-9]{1,3})\s*,\s*([0-9]{1,3})\s*,\s*([0-9]{1,3})\s*\)/.exec(color))
|
39
|
+
return [parseInt(result[1]), parseInt(result[2]), parseInt(result[3])];
|
40
|
+
|
41
|
+
// Look for rgb(num%,num%,num%)
|
42
|
+
if (result = /rgb\(\s*([0-9]+(?:\.[0-9]+)?)\%\s*,\s*([0-9]+(?:\.[0-9]+)?)\%\s*,\s*([0-9]+(?:\.[0-9]+)?)\%\s*\)/.exec(color))
|
43
|
+
return [parseFloat(result[1])*2.55, parseFloat(result[2])*2.55, parseFloat(result[3])*2.55];
|
44
|
+
|
45
|
+
// Look for #a0b1c2
|
46
|
+
if (result = /#([a-fA-F0-9]{2})([a-fA-F0-9]{2})([a-fA-F0-9]{2})/.exec(color))
|
47
|
+
return [parseInt(result[1],16), parseInt(result[2],16), parseInt(result[3],16)];
|
48
|
+
|
49
|
+
// Look for #fff
|
50
|
+
if (result = /#([a-fA-F0-9])([a-fA-F0-9])([a-fA-F0-9])/.exec(color))
|
51
|
+
return [parseInt(result[1]+result[1],16), parseInt(result[2]+result[2],16), parseInt(result[3]+result[3],16)];
|
52
|
+
|
53
|
+
// Otherwise, we're most likely dealing with a named color
|
54
|
+
return colors[jQuery.trim(color).toLowerCase()];
|
55
|
+
}
|
56
|
+
|
57
|
+
function getColor(elem, attr) {
|
58
|
+
var color;
|
59
|
+
|
60
|
+
do {
|
61
|
+
color = jQuery.curCSS(elem, attr);
|
62
|
+
|
63
|
+
// Keep going until we find an element that has color, or we hit the body
|
64
|
+
if ( color != '' && color != 'transparent' || jQuery.nodeName(elem, "body") )
|
65
|
+
break;
|
66
|
+
|
67
|
+
attr = "backgroundColor";
|
68
|
+
} while ( elem = elem.parentNode );
|
69
|
+
|
70
|
+
return getRGB(color);
|
71
|
+
};
|
72
|
+
|
73
|
+
// Some named colors to work with
|
74
|
+
// From Interface by Stefan Petre
|
75
|
+
// http://interface.eyecon.ro/
|
76
|
+
|
77
|
+
var colors = {
|
78
|
+
aqua:[0,255,255],
|
79
|
+
azure:[240,255,255],
|
80
|
+
beige:[245,245,220],
|
81
|
+
black:[0,0,0],
|
82
|
+
blue:[0,0,255],
|
83
|
+
brown:[165,42,42],
|
84
|
+
cyan:[0,255,255],
|
85
|
+
darkblue:[0,0,139],
|
86
|
+
darkcyan:[0,139,139],
|
87
|
+
darkgrey:[169,169,169],
|
88
|
+
darkgreen:[0,100,0],
|
89
|
+
darkkhaki:[189,183,107],
|
90
|
+
darkmagenta:[139,0,139],
|
91
|
+
darkolivegreen:[85,107,47],
|
92
|
+
darkorange:[255,140,0],
|
93
|
+
darkorchid:[153,50,204],
|
94
|
+
darkred:[139,0,0],
|
95
|
+
darksalmon:[233,150,122],
|
96
|
+
darkviolet:[148,0,211],
|
97
|
+
fuchsia:[255,0,255],
|
98
|
+
gold:[255,215,0],
|
99
|
+
green:[0,128,0],
|
100
|
+
indigo:[75,0,130],
|
101
|
+
khaki:[240,230,140],
|
102
|
+
lightblue:[173,216,230],
|
103
|
+
lightcyan:[224,255,255],
|
104
|
+
lightgreen:[144,238,144],
|
105
|
+
lightgrey:[211,211,211],
|
106
|
+
lightpink:[255,182,193],
|
107
|
+
lightyellow:[255,255,224],
|
108
|
+
lime:[0,255,0],
|
109
|
+
magenta:[255,0,255],
|
110
|
+
maroon:[128,0,0],
|
111
|
+
navy:[0,0,128],
|
112
|
+
olive:[128,128,0],
|
113
|
+
orange:[255,165,0],
|
114
|
+
pink:[255,192,203],
|
115
|
+
purple:[128,0,128],
|
116
|
+
violet:[128,0,128],
|
117
|
+
red:[255,0,0],
|
118
|
+
silver:[192,192,192],
|
119
|
+
white:[255,255,255],
|
120
|
+
yellow:[255,255,0]
|
121
|
+
};
|
122
|
+
|
123
|
+
})(jQuery);
|
@@ -0,0 +1,211 @@
|
|
1
|
+
/**
|
2
|
+
* Markdown Language Definition
|
3
|
+
*
|
4
|
+
* A language definition for string manipulation operations, in this case
|
5
|
+
* for the Markdown, uh, markup language. Uses regexes for various functions
|
6
|
+
* by default. If regexes won't do and you need to do some serious
|
7
|
+
* manipulation, you can declare a function in the object instead.
|
8
|
+
*
|
9
|
+
* Code example:
|
10
|
+
* 'functionbar-id' : {
|
11
|
+
* exec: function(text, selectedText) {
|
12
|
+
* functionStuffHere();
|
13
|
+
* },
|
14
|
+
* search: /somesearchregex/gi,
|
15
|
+
* replace: 'replace text for RegExp.replace',
|
16
|
+
* append: "just add this where the cursor is"
|
17
|
+
* }
|
18
|
+
*
|
19
|
+
**/
|
20
|
+
(function($) {
|
21
|
+
|
22
|
+
var MarkDown = {
|
23
|
+
|
24
|
+
'function-bold' : {
|
25
|
+
search: /([^\n]+)([\n\s]*)/g,
|
26
|
+
replace: "**$1**$2"
|
27
|
+
},
|
28
|
+
|
29
|
+
'function-italic' : {
|
30
|
+
search: /([^\n]+)([\n\s]*)/g,
|
31
|
+
replace: "_$1_$2"
|
32
|
+
},
|
33
|
+
|
34
|
+
'function-code' : {
|
35
|
+
search: /(^[\n]+)([\n\s]*)/g,
|
36
|
+
replace: "`$1`$2"
|
37
|
+
},
|
38
|
+
|
39
|
+
'function-hr' : {
|
40
|
+
append: "\n***\n"
|
41
|
+
},
|
42
|
+
|
43
|
+
'function-ul' : {
|
44
|
+
search: /(.+)([\n]?)/g,
|
45
|
+
replace: "* $1$2"
|
46
|
+
},
|
47
|
+
|
48
|
+
/* This looks silly but is completely valid Markdown */
|
49
|
+
'function-ol' : {
|
50
|
+
search: /(.+)([\n]?)/g,
|
51
|
+
replace: "1. $1$2"
|
52
|
+
},
|
53
|
+
|
54
|
+
'function-blockquote' : {
|
55
|
+
search: /(.+)([\n]?)/g,
|
56
|
+
replace: "> $1$2"
|
57
|
+
},
|
58
|
+
|
59
|
+
'function-h1' : {
|
60
|
+
search: /(.+)([\n]?)/g,
|
61
|
+
replace: "# $1$2"
|
62
|
+
},
|
63
|
+
|
64
|
+
'function-h2' : {
|
65
|
+
search: /(.+)([\n]?)/g,
|
66
|
+
replace: "## $1$2"
|
67
|
+
},
|
68
|
+
|
69
|
+
'function-h3' : {
|
70
|
+
search: /(.+)([\n]?)/g,
|
71
|
+
replace: "### $1$2"
|
72
|
+
},
|
73
|
+
|
74
|
+
'function-link' : {
|
75
|
+
exec: function( txt, selText, $field ) {
|
76
|
+
var results = null;
|
77
|
+
$.GollumEditor.Dialog.init({
|
78
|
+
title: 'Insert Link',
|
79
|
+
fields: [
|
80
|
+
{
|
81
|
+
id: 'text',
|
82
|
+
name: 'Link Text',
|
83
|
+
type: 'text'
|
84
|
+
},
|
85
|
+
{
|
86
|
+
id: 'href',
|
87
|
+
name: 'URL',
|
88
|
+
type: 'text'
|
89
|
+
}
|
90
|
+
],
|
91
|
+
OK: function( res ) {
|
92
|
+
var rep = '';
|
93
|
+
if ( res['text'] && res['href'] ) {
|
94
|
+
rep = '[' + res['text'] + '](' +
|
95
|
+
res['href'] + ')';
|
96
|
+
}
|
97
|
+
$.GollumEditor.replaceSelection( rep );
|
98
|
+
}
|
99
|
+
});
|
100
|
+
}
|
101
|
+
},
|
102
|
+
|
103
|
+
'function-image' : {
|
104
|
+
exec: function( txt, selText, $field ) {
|
105
|
+
var results = null;
|
106
|
+
$.GollumEditor.Dialog.init({
|
107
|
+
title: 'Insert Image',
|
108
|
+
fields: [
|
109
|
+
{
|
110
|
+
id: 'url',
|
111
|
+
name: 'Image URL',
|
112
|
+
type: 'text'
|
113
|
+
},
|
114
|
+
{
|
115
|
+
id: 'alt',
|
116
|
+
name: 'Alt Text',
|
117
|
+
type: 'text'
|
118
|
+
}
|
119
|
+
],
|
120
|
+
OK: function( res ) {
|
121
|
+
var rep = '';
|
122
|
+
if ( res['url'] && res['alt'] ) {
|
123
|
+
rep = '![' + res['alt'] + ']' +
|
124
|
+
'(' + res['url'] + ')';
|
125
|
+
}
|
126
|
+
$.GollumEditor.replaceSelection( rep );
|
127
|
+
}
|
128
|
+
});
|
129
|
+
}
|
130
|
+
}
|
131
|
+
|
132
|
+
};
|
133
|
+
|
134
|
+
var MarkDownHelp = [
|
135
|
+
|
136
|
+
{
|
137
|
+
menuName: 'Block Elements',
|
138
|
+
content: [
|
139
|
+
{
|
140
|
+
menuName: 'Paragraphs & Breaks',
|
141
|
+
data: '<p>To create a paragraph, simply create a block of text that is not separated by one or more blank lines. Blocks of text separated by one or more blank lines will be parsed as paragraphs.</p><p>If you want to create a line break, end a line with two or more spaces, then hit Return/Enter.</p>'
|
142
|
+
},
|
143
|
+
{
|
144
|
+
menuName: 'Headers',
|
145
|
+
data: '<p>Markdown supports two header formats. The wiki editor uses the “atx’-style headers. Simply prefix your header text with the number of <code>#</code> characters to specify heading depth. For example: <code># Header 1</code>, <code>## Header 2</code> and <code>### Header 3</code> will be progressively smaller headers. You may end your headers with any number of hashes.</p>'
|
146
|
+
},
|
147
|
+
{
|
148
|
+
menuName: 'Blockquotes',
|
149
|
+
data: '<p>Markdown creates blockquotes email-style by prefixing each line with the <code>></code>. This looks best if you decide to hard-wrap text and prefix each line with a <code>></code> character, but Markdown supports just putting <code>></code> before your paragraph.</p>'
|
150
|
+
},
|
151
|
+
{
|
152
|
+
menuName: 'Lists',
|
153
|
+
data: '<p>Markdown supports both ordered and unordered lists. To create an ordered list, simply prefix each line with a number (any number will do — this is why the editor only uses one number.) To create an unordered list, you can prefix each line with <code>*</code>, <code>+</code> or <code>-</code>.</p> List items can contain multiple paragraphs, however each paragraph must be indented by at least 4 spaces or a tab.'
|
154
|
+
},
|
155
|
+
{
|
156
|
+
menuName: 'Code Blocks',
|
157
|
+
data: '<p>Markdown wraps code blocks in pre-formatted tags to preserve indentation in your code blocks. To create a code block, indent the entire block by at least 4 spaces or one tab. Markdown will strip the extra indentation you’ve added to the code block.</p>'
|
158
|
+
},
|
159
|
+
{
|
160
|
+
menuName: 'Horizontal Rules',
|
161
|
+
data: 'Horizontal rules are created by placing three or more hyphens, asterisks or underscores on a line by themselves. Spaces are allowed between the hyphens, asterisks or underscores.'
|
162
|
+
}
|
163
|
+
]
|
164
|
+
},
|
165
|
+
|
166
|
+
{
|
167
|
+
menuName: 'Span Elements',
|
168
|
+
content: [
|
169
|
+
{
|
170
|
+
menuName: 'Links',
|
171
|
+
data: '<p>Markdown has two types of links: <strong>inline</strong> and <strong>reference</strong>. For both types of links, the text you want to display to the user is placed in square brackets. For example, if you want your link to display the text “GitHub”, you write <code>[GitHub]</code>.</p><p>To create an inline link, create a set of parentheses immediately after the brackets and write your URL within the parentheses. (e.g., <code>[GitHub](http://github.com/)</code>). Relative paths are allowed in inline links.</p><p>To create a reference link, use two sets of square brackets. <code>[my internal link][internal-ref]</code> will link to the internal reference <code>internal-ref</code>.</p>'
|
172
|
+
},
|
173
|
+
|
174
|
+
{
|
175
|
+
menuName: 'Emphasis',
|
176
|
+
data: '<p>Asterisks (<code>*</code>) and underscores (<code>_</code>) are treated as emphasis and are wrapped with an <code><em></code> tag, which usually displays as italics in most browsers. Double asterisks (<code>**</code>) or double underscores (<code>__</code>) are treated as bold using the <code><strong></code> tag. To create italic or bold text, simply wrap your words in single/double asterisks/underscores. For example, <code>**My double emphasis text**</code> becomes <strong>My double emphasis text</strong>, and <code>*My single emphasis text*</code> becomes <em>My single emphasis text</em>.</p>'
|
177
|
+
},
|
178
|
+
|
179
|
+
{
|
180
|
+
menuName: 'Code',
|
181
|
+
data: '<p>To create inline spans of code, simply wrap the code in backticks (<code>`</code>). Markdown will turn <code>`myFunction`</code> into <code>myFunction</code>.</p>'
|
182
|
+
},
|
183
|
+
|
184
|
+
{
|
185
|
+
menuName: 'Images',
|
186
|
+
data: '<p>Markdown image syntax looks a lot like the syntax for links; it is essentially the same syntax preceded by an exclamation point (<code>!</code>). For example, if you want to link to an image at <code>http://github.com/unicorn.png</code> with the alternate text <code>My Unicorn</code>, you would write <code>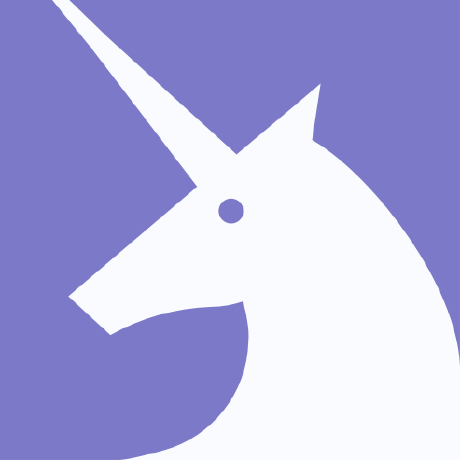</code>.</p>'
|
187
|
+
}
|
188
|
+
]
|
189
|
+
},
|
190
|
+
|
191
|
+
{
|
192
|
+
menuName: 'Miscellaneous',
|
193
|
+
content: [
|
194
|
+
{
|
195
|
+
menuName: 'Automatic Links',
|
196
|
+
data: '<p>If you want to create a link that displays the actual URL, markdown allows you to quickly wrap the URL in <code><</code> and <code>></code> to do so. For example, the link <a href="javascript:void(0);">http://github.com/</a> is easily produced by writing <code><http://github.com/></code>.</p>'
|
197
|
+
},
|
198
|
+
|
199
|
+
{
|
200
|
+
menuName: 'Escaping',
|
201
|
+
data: '<p>If you want to use a special Markdown character in your document (such as displaying literal asterisks), you can escape the character with the backslash (<code>\\</code>). Markdown will ignore the character directly after a backslash.'
|
202
|
+
}
|
203
|
+
]
|
204
|
+
}
|
205
|
+
];
|
206
|
+
|
207
|
+
|
208
|
+
$.GollumEditor.defineLanguage('markdown', MarkDown);
|
209
|
+
$.GollumEditor.defineHelp('markdown', MarkDownHelp);
|
210
|
+
|
211
|
+
})(jQuery);
|
@@ -0,0 +1,141 @@
|
|
1
|
+
/* @control dialog */
|
2
|
+
|
3
|
+
#gollum-dialog-dialog {
|
4
|
+
display: block;
|
5
|
+
overflow: visible;
|
6
|
+
position: absolute;
|
7
|
+
top: 50%;
|
8
|
+
left: 50%;
|
9
|
+
}
|
10
|
+
|
11
|
+
#gollum-dialog-dialog.active {
|
12
|
+
display: block;
|
13
|
+
}
|
14
|
+
|
15
|
+
#gollum-dialog-dialog-inner {
|
16
|
+
margin: 0 0 0 -225px;
|
17
|
+
position: relative;
|
18
|
+
width: 450px;
|
19
|
+
|
20
|
+
border: 7px solid #999;
|
21
|
+
border: 7px solid rgba(0, 0, 0, 0.3);
|
22
|
+
border-radius: 5px;
|
23
|
+
-moz-border-radius: 5px;
|
24
|
+
-webkit-border-radius: 5px;
|
25
|
+
}
|
26
|
+
|
27
|
+
#gollum-dialog-dialog-bg {
|
28
|
+
background-color: #fff;
|
29
|
+
overflow: hidden;
|
30
|
+
padding: 1em;
|
31
|
+
|
32
|
+
background: -webkit-gradient(linear, left top, left bottom, from(#f7f7f7), to(#ffffff));
|
33
|
+
background: -moz-linear-gradient(top, #f7f7f7, #ffffff);
|
34
|
+
}
|
35
|
+
|
36
|
+
#gollum-dialog-dialog-inner h4 {
|
37
|
+
border-bottom: 1px solid #ddd;
|
38
|
+
color: #000;
|
39
|
+
font-size: 1.8em;
|
40
|
+
line-height: normal;
|
41
|
+
font-weight: bold;
|
42
|
+
margin: 0 0 0.75em 0;
|
43
|
+
padding: 0 0 0.3em 0;
|
44
|
+
}
|
45
|
+
|
46
|
+
#gollum-dialog-dialog-body {
|
47
|
+
font-size: 1.2em;
|
48
|
+
line-height: 1.6em;
|
49
|
+
}
|
50
|
+
|
51
|
+
#gollum-dialog-dialog-body fieldset {
|
52
|
+
display: block;
|
53
|
+
border: 0;
|
54
|
+
margin: 0;
|
55
|
+
overflow: hidden;
|
56
|
+
padding: 0;
|
57
|
+
}
|
58
|
+
|
59
|
+
#gollum-dialog-dialog-body fieldset .field {
|
60
|
+
margin: 0 0 1.5em 0;
|
61
|
+
padding: 0;
|
62
|
+
}
|
63
|
+
|
64
|
+
#gollum-dialog-dialog-body fieldset .field label {
|
65
|
+
color: #000;
|
66
|
+
display: block;
|
67
|
+
font-size: 1.2em;
|
68
|
+
font-weight: bold;
|
69
|
+
line-height: 1.6em;
|
70
|
+
margin: 0;
|
71
|
+
padding: 0;
|
72
|
+
min-width: 80px;
|
73
|
+
}
|
74
|
+
|
75
|
+
#gollum-dialog-dialog-body fieldset .field input[type="text"] {
|
76
|
+
border: 1px solid #ddd;
|
77
|
+
display: block;
|
78
|
+
font-family: 'Helvetica Neue', Helvetica, Arial, sans-serif;
|
79
|
+
font-size: 1.2em;
|
80
|
+
line-height: 1.6em;
|
81
|
+
margin: 0.3em 0 0 0;
|
82
|
+
padding: 0.3em 0.5em;
|
83
|
+
width: 96.5%;
|
84
|
+
}
|
85
|
+
|
86
|
+
#gollum-dialog-dialog-body fieldset .field input.code {
|
87
|
+
font-family: 'Monaco', 'Courier New', Courier, monospace;
|
88
|
+
}
|
89
|
+
|
90
|
+
#gollum-dialog-dialog-body fieldset .field:last-child {
|
91
|
+
margin: 0 0 1em 0;
|
92
|
+
}
|
93
|
+
|
94
|
+
#gollum-dialog-dialog-buttons {
|
95
|
+
border-top: 1px solid #ddd;
|
96
|
+
overflow: hidden;
|
97
|
+
margin: 1.5em 0 0 0;
|
98
|
+
padding: 1em 0 0 0;
|
99
|
+
}
|
100
|
+
|
101
|
+
#gollum-dialog-dialog a.minibutton {
|
102
|
+
float: right;
|
103
|
+
margin-right: 0.5em;
|
104
|
+
width: auto;
|
105
|
+
}
|
106
|
+
|
107
|
+
#gollum-dialog-dialog a.minibutton,
|
108
|
+
#gollum-dialog-dialog a.minibutton:visited {
|
109
|
+
background-color: #f7f7f7;
|
110
|
+
border: 1px solid #d4d4d4;
|
111
|
+
color: #333;
|
112
|
+
cursor: pointer;
|
113
|
+
display: block;
|
114
|
+
font-size: 1.2em;
|
115
|
+
font-family: 'Helvetica Neue', Helvetica, Arial, sans-serif;
|
116
|
+
font-weight: bold;
|
117
|
+
margin: 0 0 0 0.8em;
|
118
|
+
padding: 0.4em 1em;
|
119
|
+
|
120
|
+
text-shadow: 0 1px 0 #fff;
|
121
|
+
|
122
|
+
filter:progid:DXImageTransform.Microsoft.gradient(GradientType=0, startColorstr='#f4f4f4', endColorstr='#ececec');
|
123
|
+
background: -webkit-gradient(linear, left top, left bottom, from(#f4f4f4), to(#ececec));
|
124
|
+
background: -moz-linear-gradient(top, #f4f4f4, #ececec);
|
125
|
+
|
126
|
+
border-radius: 3px;
|
127
|
+
-moz-border-radius: 3px;
|
128
|
+
-webkit-border-radius: 3px;
|
129
|
+
}
|
130
|
+
|
131
|
+
#gollum-dialog-dialog a.minibutton:hover {
|
132
|
+
background: #3072b3;
|
133
|
+
border-color: #518cc6 #518cc6 #2a65a0;
|
134
|
+
color: #fff;
|
135
|
+
text-shadow: 0 -1px 0 rgba(0, 0, 0, 0.3);
|
136
|
+
text-decoration: none;
|
137
|
+
|
138
|
+
filter:progid:DXImageTransform.Microsoft.gradient(GradientType=0, startColorstr='#599bdc', endColorstr='#3072b3');
|
139
|
+
background: -webkit-gradient(linear, left top, left bottom, from(#599bdc), to(#3072b3));
|
140
|
+
background: -moz-linear-gradient(top, #599bdc, #3072b3);
|
141
|
+
}
|