gerry 0.0.3
This diff represents the content of publicly available package versions that have been released to one of the supported registries. The information contained in this diff is provided for informational purposes only and reflects changes between package versions as they appear in their respective public registries.
- checksums.yaml +7 -0
- data/README.md +60 -0
- data/Rakefile +7 -0
- data/lib/gerry/client/accounts.rb +29 -0
- data/lib/gerry/client/changes.rb +20 -0
- data/lib/gerry/client/groups.rb +57 -0
- data/lib/gerry/client/projects.rb +21 -0
- data/lib/gerry/client/request.rb +86 -0
- data/lib/gerry/client.rb +30 -0
- data/lib/gerry/version.rb +5 -0
- data/lib/gerry.rb +12 -0
- data/spec/accounts_spec.rb +36 -0
- data/spec/changes_spec.rb +32 -0
- data/spec/fixtures/account_groups.json +33 -0
- data/spec/fixtures/capabilities.json +7 -0
- data/spec/fixtures/changes.json +31 -0
- data/spec/fixtures/group_members.json +15 -0
- data/spec/fixtures/groups.json +69 -0
- data/spec/fixtures/open_changes.json +17 -0
- data/spec/fixtures/projects.json +9 -0
- data/spec/fixtures/query_capabilities.json +5 -0
- data/spec/groups_spec.rb +92 -0
- data/spec/projects_spec.rb +26 -0
- data/spec/request_spec.rb +42 -0
- data/spec/spec_helper.rb +50 -0
- metadata +195 -0
checksums.yaml
ADDED
@@ -0,0 +1,7 @@
|
|
1
|
+
---
|
2
|
+
SHA1:
|
3
|
+
metadata.gz: 702aaae3f3f3ee526dbf3e7e4cc992abe99b7a31
|
4
|
+
data.tar.gz: 80dbe7f9b6e0bb8bfb26fa730cec895024dd4b4a
|
5
|
+
SHA512:
|
6
|
+
metadata.gz: c16e1ffe1e8cc8d9096b5ec7d7413c25e273436aa61203355dc86e48283a8e5cfd0388f08fce1657989c055f721068361aff111b123d21b0605cf62d7a3c58b4
|
7
|
+
data.tar.gz: a721f594ca1c4cc1a09c9854d180b9755e70d48c0d3ae9936308502efb7047def937325fb40c629855e2c87366de9352a9a4bae20a62b7c9f5efa92348fc2ada
|
data/README.md
ADDED
@@ -0,0 +1,60 @@
|
|
1
|
+
# gerry[][travis]
|
2
|
+
|
3
|
+
Simple Ruby wrapper for the Gerrit Code Review REST-API.
|
4
|
+
|
5
|
+
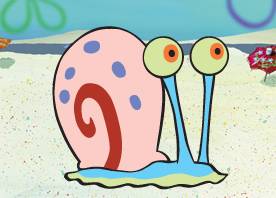
|
6
|
+
|
7
|
+
[travis]: https://travis-ci.org/trumant/gerry
|
8
|
+
|
9
|
+
## Documentation
|
10
|
+
[http://rdoc.info/github/trumant/gerry][documentation]
|
11
|
+
|
12
|
+
[documentation]: http://rdoc.info/github/trumant/gerry
|
13
|
+
|
14
|
+
## Install
|
15
|
+
```
|
16
|
+
bundle
|
17
|
+
bundle exec rake install
|
18
|
+
```
|
19
|
+
|
20
|
+
## Examples
|
21
|
+
### Get the global capabilities
|
22
|
+
```ruby
|
23
|
+
client = Gerry.new('https://review')
|
24
|
+
client.account_capabilities
|
25
|
+
=> {"queryLimit"=>{"min"=>0, "max"=>250}}
|
26
|
+
```
|
27
|
+
|
28
|
+
### List projects
|
29
|
+
```ruby
|
30
|
+
client = Gerry.new('https://review')
|
31
|
+
client.projects
|
32
|
+
=> { "awesome"=>{ "description"=>"Awesome project"}}
|
33
|
+
```
|
34
|
+
|
35
|
+
### List open changes
|
36
|
+
```ruby
|
37
|
+
client = Gerry.new('https://review')
|
38
|
+
client.changes(['q=is:open'])
|
39
|
+
=> [{"project"=>"awesome", "branch"=>"master", "id"=>"Ibfedd978...."}]
|
40
|
+
```
|
41
|
+
|
42
|
+
## Licence
|
43
|
+
The MIT Licence
|
44
|
+
|
45
|
+
Copyright (c) Fabian Mettler, Andrew Erickson, Travis Truman
|
46
|
+
|
47
|
+
Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
|
48
|
+
|
49
|
+
The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.
|
50
|
+
|
51
|
+
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
52
|
+
|
53
|
+
## References
|
54
|
+
[http://code.google.com/p/gerrit/][gerrit]
|
55
|
+
|
56
|
+
[gerrit]: http://code.google.com/p/gerrit/
|
57
|
+
|
58
|
+
[https://gerrit-review.googlesource.com/Documentation/rest-api.html][apidocumentation]
|
59
|
+
|
60
|
+
[apidocumentation]: https://gerrit-review.googlesource.com/Documentation/rest-api.html
|
data/Rakefile
ADDED
@@ -0,0 +1,29 @@
|
|
1
|
+
module Gerry
|
2
|
+
class Client
|
3
|
+
module Accounts
|
4
|
+
# Get the global capabilities that are enabled for the calling user.
|
5
|
+
#
|
6
|
+
# @param [Array] options the query parameters.
|
7
|
+
# @return [Hash] the account capabilities.
|
8
|
+
def account_capabilities(options = [])
|
9
|
+
url = '/accounts/self/capabilities'
|
10
|
+
|
11
|
+
if options.empty?
|
12
|
+
return get(url)
|
13
|
+
end
|
14
|
+
|
15
|
+
options = map_options(options)
|
16
|
+
get("#{url}?#{options}")
|
17
|
+
end
|
18
|
+
|
19
|
+
# Get all groups that contain the specified account as a member
|
20
|
+
#
|
21
|
+
# @param [String] account_id the account
|
22
|
+
# @return [Enumberable] the groups
|
23
|
+
def groups_for_account(account_id)
|
24
|
+
url = "/accounts/#{account_id}/groups/"
|
25
|
+
get(url)
|
26
|
+
end
|
27
|
+
end
|
28
|
+
end
|
29
|
+
end
|
@@ -0,0 +1,20 @@
|
|
1
|
+
module Gerry
|
2
|
+
class Client
|
3
|
+
module Changes
|
4
|
+
# Get changes visible to the caller.
|
5
|
+
#
|
6
|
+
# @param [Array] options the query parameters.
|
7
|
+
# @return [Hash] the changes.
|
8
|
+
def changes(options = [])
|
9
|
+
url = '/changes/'
|
10
|
+
|
11
|
+
if options.empty?
|
12
|
+
return get(url)
|
13
|
+
end
|
14
|
+
|
15
|
+
options = map_options(options)
|
16
|
+
get("#{url}?#{options}")
|
17
|
+
end
|
18
|
+
end
|
19
|
+
end
|
20
|
+
end
|
@@ -0,0 +1,57 @@
|
|
1
|
+
require 'json'
|
2
|
+
|
3
|
+
module Gerry
|
4
|
+
class Client
|
5
|
+
module Groups
|
6
|
+
# Get all groups
|
7
|
+
#
|
8
|
+
# @return [Hash] the groups
|
9
|
+
def groups
|
10
|
+
url = '/groups/'
|
11
|
+
get(url)
|
12
|
+
end
|
13
|
+
|
14
|
+
# Get all members for a group
|
15
|
+
#
|
16
|
+
# @return [Array] the members
|
17
|
+
def group_members(group_id)
|
18
|
+
url = "/groups/#{group_id}/members/"
|
19
|
+
get(url)
|
20
|
+
end
|
21
|
+
|
22
|
+
# Create a new group
|
23
|
+
#
|
24
|
+
# @return [Hash] the group details
|
25
|
+
def create_group(name, description, visible, owner_id=nil)
|
26
|
+
url = "/groups/#{name}"
|
27
|
+
body = {
|
28
|
+
description: description,
|
29
|
+
visible_to_all: visible,
|
30
|
+
}
|
31
|
+
body[:owner_id] = owner_id unless owner_id.nil? || owner_id.empty?
|
32
|
+
put(url, body)
|
33
|
+
end
|
34
|
+
|
35
|
+
# Adds one or more users to a group
|
36
|
+
#
|
37
|
+
# @param [String] group_id the group id
|
38
|
+
# @param [Enumberable] users the list of users identified by email address
|
39
|
+
# @return [Hash] the account info details for each user added
|
40
|
+
def add_to_group(group_id, users)
|
41
|
+
url = "/groups/#{group_id}/members"
|
42
|
+
body = {
|
43
|
+
members: users
|
44
|
+
}
|
45
|
+
post(url, body)
|
46
|
+
end
|
47
|
+
|
48
|
+
def remove_from_group(group_id, users)
|
49
|
+
url = "/groups/#{group_id}/members.delete"
|
50
|
+
body = {
|
51
|
+
members: users
|
52
|
+
}
|
53
|
+
post(url, body)
|
54
|
+
end
|
55
|
+
end
|
56
|
+
end
|
57
|
+
end
|
@@ -0,0 +1,21 @@
|
|
1
|
+
module Gerry
|
2
|
+
class Client
|
3
|
+
module Projects
|
4
|
+
# Get the projects accessible by the caller.
|
5
|
+
#
|
6
|
+
# @return [Hash] the projects.
|
7
|
+
def projects
|
8
|
+
get('/projects/')
|
9
|
+
end
|
10
|
+
|
11
|
+
# Get the projects that start with the specified prefix
|
12
|
+
# and accessible by the caller.
|
13
|
+
#
|
14
|
+
# @param [String] name the project name.
|
15
|
+
# @return [Hash] the projects.
|
16
|
+
def find_project(name)
|
17
|
+
get("/projects/#{name}")
|
18
|
+
end
|
19
|
+
end
|
20
|
+
end
|
21
|
+
end
|
@@ -0,0 +1,86 @@
|
|
1
|
+
module Gerry
|
2
|
+
class Client
|
3
|
+
module Request
|
4
|
+
# Get the mapped options.
|
5
|
+
#
|
6
|
+
# @param [Array] options the query parameters.
|
7
|
+
# @return [String] the mapped options.
|
8
|
+
def map_options(options)
|
9
|
+
options.map{|v| "#{v}"}.join('&')
|
10
|
+
end
|
11
|
+
|
12
|
+
private
|
13
|
+
class RequestError < StandardError
|
14
|
+
end
|
15
|
+
|
16
|
+
def get(url)
|
17
|
+
if @username && @password
|
18
|
+
auth = { username: @username, password: @password }
|
19
|
+
response = self.class.get("/a#{url}", digest_auth: auth)
|
20
|
+
parse(response)
|
21
|
+
else
|
22
|
+
response = self.class.get(url)
|
23
|
+
parse(response)
|
24
|
+
end
|
25
|
+
end
|
26
|
+
|
27
|
+
def put(url, body)
|
28
|
+
if @username && @password
|
29
|
+
auth = { username: @username, password: @password }
|
30
|
+
response = self.class.put("/a#{url}",
|
31
|
+
body: body.to_json,
|
32
|
+
headers: { 'Content-Type' => 'application/json' },
|
33
|
+
digest_auth: auth
|
34
|
+
)
|
35
|
+
parse(response)
|
36
|
+
else
|
37
|
+
response = self.class.put(url,
|
38
|
+
body: body.to_json,
|
39
|
+
headers: { 'Content-Type' => 'application/json' }
|
40
|
+
)
|
41
|
+
parse(response)
|
42
|
+
end
|
43
|
+
end
|
44
|
+
|
45
|
+
def post(url, body)
|
46
|
+
if @username && @password
|
47
|
+
auth = { username: @username, password: @password }
|
48
|
+
response = self.class.post("/a#{url}",
|
49
|
+
body: body.to_json,
|
50
|
+
headers: { 'Content-Type' => 'application/json' },
|
51
|
+
digest_auth: auth
|
52
|
+
)
|
53
|
+
parse(response)
|
54
|
+
else
|
55
|
+
response = self.class.post(url,
|
56
|
+
body: body.to_json,
|
57
|
+
headers: { 'Content-Type' => 'application/json' }
|
58
|
+
)
|
59
|
+
parse(response)
|
60
|
+
end
|
61
|
+
end
|
62
|
+
|
63
|
+
def parse(response)
|
64
|
+
unless /2[0-9][0-9]/.match(response.code.to_s)
|
65
|
+
raise_request_error(response)
|
66
|
+
end
|
67
|
+
if response.body
|
68
|
+
JSON.parse(remove_magic_prefix(response.body))
|
69
|
+
else
|
70
|
+
nil
|
71
|
+
end
|
72
|
+
end
|
73
|
+
|
74
|
+
def raise_request_error(response)
|
75
|
+
body = response.body
|
76
|
+
raise RequestError.new("There was a request error! Response was: #{body}")
|
77
|
+
end
|
78
|
+
|
79
|
+
def remove_magic_prefix(response_body)
|
80
|
+
# We need to strip the magic prefix from the first line of the response, see
|
81
|
+
# https://gerrit-review.googlesource.com/Documentation/rest-api.html#output.
|
82
|
+
response_body.sub(/^\)\]\}'$/, '')
|
83
|
+
end
|
84
|
+
end
|
85
|
+
end
|
86
|
+
end
|
data/lib/gerry/client.rb
ADDED
@@ -0,0 +1,30 @@
|
|
1
|
+
require 'httparty'
|
2
|
+
require 'json'
|
3
|
+
|
4
|
+
module Gerry
|
5
|
+
class Client
|
6
|
+
include HTTParty
|
7
|
+
headers 'Accept' => 'application/json'
|
8
|
+
|
9
|
+
require_relative 'client/accounts'
|
10
|
+
require_relative 'client/changes'
|
11
|
+
require_relative 'client/groups'
|
12
|
+
require_relative 'client/projects'
|
13
|
+
require_relative 'client/request'
|
14
|
+
|
15
|
+
include Accounts
|
16
|
+
include Changes
|
17
|
+
include Groups
|
18
|
+
include Projects
|
19
|
+
include Request
|
20
|
+
|
21
|
+
def initialize(url, username = nil, password = nil)
|
22
|
+
self.class.base_uri(url)
|
23
|
+
|
24
|
+
if username && password
|
25
|
+
@username = username
|
26
|
+
@password = password
|
27
|
+
end
|
28
|
+
end
|
29
|
+
end
|
30
|
+
end
|
data/lib/gerry.rb
ADDED
@@ -0,0 +1,36 @@
|
|
1
|
+
require 'spec_helper'
|
2
|
+
|
3
|
+
describe '.account_capabilities' do
|
4
|
+
it 'should fetch all account capabilities' do
|
5
|
+
stub = stub_get('/accounts/self/capabilities', 'capabilities.json')
|
6
|
+
|
7
|
+
client = MockGerry.new
|
8
|
+
capabilities = client.account_capabilities
|
9
|
+
|
10
|
+
expect(capabilities['queryLimit']['min']).to eq(0)
|
11
|
+
expect(capabilities['queryLimit']['max']).to eq(500)
|
12
|
+
end
|
13
|
+
|
14
|
+
it 'should fetch some account capabilities' do
|
15
|
+
stub = stub_get('/accounts/self/capabilities?q=createAccount&q=createGroup', 'query_capabilities.json')
|
16
|
+
|
17
|
+
client = MockGerry.new
|
18
|
+
capabilities = client.account_capabilities(['q=createAccount', 'q=createGroup'])
|
19
|
+
expect(stub).to have_been_requested
|
20
|
+
|
21
|
+
expect(capabilities['createAccount']).to eq(true)
|
22
|
+
expect(capabilities['createGroup']).to eq(true)
|
23
|
+
end
|
24
|
+
end
|
25
|
+
|
26
|
+
describe '.groups_for_account' do
|
27
|
+
it "fetches all groups for which the account is a member" do
|
28
|
+
user = "jane.roe@example.com"
|
29
|
+
|
30
|
+
stub = stub_get("/accounts/#{user}/groups/", "account_groups.json")
|
31
|
+
|
32
|
+
client = MockGerry.new
|
33
|
+
new_group = client.groups_for_account(user)
|
34
|
+
expect(stub).to have_been_requested
|
35
|
+
end
|
36
|
+
end
|
@@ -0,0 +1,32 @@
|
|
1
|
+
require 'spec_helper'
|
2
|
+
|
3
|
+
describe '.changes' do
|
4
|
+
it 'should fetch all changes' do
|
5
|
+
stub = stub_get('/changes/', 'changes.json')
|
6
|
+
|
7
|
+
client = MockGerry.new
|
8
|
+
changes = client.changes
|
9
|
+
|
10
|
+
expect(stub).to have_been_requested
|
11
|
+
|
12
|
+
expect(changes[0]['project']).to eq('awesome')
|
13
|
+
expect(changes[0]['branch']).to eq('master')
|
14
|
+
|
15
|
+
expect(changes[1]['project']).to eq('clean')
|
16
|
+
expect(changes[1]['subject']).to eq('Refactor code')
|
17
|
+
expect(changes[1]['owner']['name']).to eq('Batman')
|
18
|
+
end
|
19
|
+
|
20
|
+
it 'should fetch all open changes' do
|
21
|
+
|
22
|
+
stub = stub_get('/changes/?q=is:open+owner:self', 'open_changes.json')
|
23
|
+
|
24
|
+
client = MockGerry.new
|
25
|
+
changes = client.changes(['q=is:open+owner:self'])
|
26
|
+
|
27
|
+
expect(stub).to have_been_requested
|
28
|
+
|
29
|
+
expect(changes[0]['status']).to eq('OPEN')
|
30
|
+
expect(changes[0]['owner']['name']).to eq('The Duke')
|
31
|
+
end
|
32
|
+
end
|
@@ -0,0 +1,33 @@
|
|
1
|
+
)]}'
|
2
|
+
[
|
3
|
+
{
|
4
|
+
"kind": "gerritcodereview#group",
|
5
|
+
"id": "global%3AAnonymous-Users",
|
6
|
+
"url": "#/admin/groups/uuid-global%3AAnonymous-Users",
|
7
|
+
"options": {
|
8
|
+
},
|
9
|
+
"description": "Any user, signed-in or not",
|
10
|
+
"group_id": 2,
|
11
|
+
"owner_id": "6a1e70e1a88782771a91808c8af9bbb7a9871389"
|
12
|
+
},
|
13
|
+
{
|
14
|
+
"kind": "gerritcodereview#group",
|
15
|
+
"id": "834ec36dd5e0ed21a2ff5d7e2255da082d63bbd7",
|
16
|
+
"url": "#/admin/groups/uuid-834ec36dd5e0ed21a2ff5d7e2255da082d63bbd7",
|
17
|
+
"options": {
|
18
|
+
"visible_to_all": true
|
19
|
+
},
|
20
|
+
"group_id": 6,
|
21
|
+
"owner_id": "834ec36dd5e0ed21a2ff5d7e2255da082d63bbd7"
|
22
|
+
},
|
23
|
+
{
|
24
|
+
"kind": "gerritcodereview#group",
|
25
|
+
"id": "global%3ARegistered-Users",
|
26
|
+
"url": "#/admin/groups/uuid-global%3ARegistered-Users",
|
27
|
+
"options": {
|
28
|
+
},
|
29
|
+
"description": "Any signed-in user",
|
30
|
+
"group_id": 3,
|
31
|
+
"owner_id": "6a1e70e1a88782771a91808c8af9bbb7a9871389"
|
32
|
+
}
|
33
|
+
]
|
@@ -0,0 +1,31 @@
|
|
1
|
+
)]}'
|
2
|
+
[
|
3
|
+
{
|
4
|
+
"project": "awesome",
|
5
|
+
"branch": "master",
|
6
|
+
"id": "Idd8747a5ea93bb9bddf7695bab25670be135f777",
|
7
|
+
"subject": "Add an awesome feature",
|
8
|
+
"status": "NEW",
|
9
|
+
"created": "2012-09-12 15:54:21.531000000",
|
10
|
+
"updated": "2012-10-13 01:34:40.511000000",
|
11
|
+
"_sortkey": "00115dfe00009334",
|
12
|
+
"_number": 3,
|
13
|
+
"owner": {
|
14
|
+
"name": "The Duke"
|
15
|
+
}
|
16
|
+
},
|
17
|
+
{
|
18
|
+
"project": "clean",
|
19
|
+
"branch": "master",
|
20
|
+
"id": "Idac803deea68faa1294b3fa5bf814277e899f075",
|
21
|
+
"subject": "Refactor code",
|
22
|
+
"status": "NEW",
|
23
|
+
"created": "2012-09-12 15:51:30.605000000",
|
24
|
+
"updated": "2012-10-13 00:45:26.431000000",
|
25
|
+
"_sortkey": "00205dcddd00937d",
|
26
|
+
"_number": 42,
|
27
|
+
"owner": {
|
28
|
+
"name": "Batman"
|
29
|
+
}
|
30
|
+
}
|
31
|
+
]
|
@@ -0,0 +1,69 @@
|
|
1
|
+
)]}'
|
2
|
+
{
|
3
|
+
"Administrators": {
|
4
|
+
"kind": "gerritcodereview#group",
|
5
|
+
"id": "6a1e70e1a88782771a91808c8af9bbb7a9871389",
|
6
|
+
"url": "#/admin/groups/uuid-6a1e70e1a88782771a91808c8af9bbb7a9871389",
|
7
|
+
"options": {
|
8
|
+
},
|
9
|
+
"description": "Gerrit Site Administrators",
|
10
|
+
"group_id": 1,
|
11
|
+
"owner": "Administrators",
|
12
|
+
"owner_id": "6a1e70e1a88782771a91808c8af9bbb7a9871389"
|
13
|
+
},
|
14
|
+
"Anonymous Users": {
|
15
|
+
"kind": "gerritcodereview#group",
|
16
|
+
"id": "global%3AAnonymous-Users",
|
17
|
+
"url": "#/admin/groups/uuid-global%3AAnonymous-Users",
|
18
|
+
"options": {
|
19
|
+
},
|
20
|
+
"description": "Any user, signed-in or not",
|
21
|
+
"group_id": 2,
|
22
|
+
"owner": "Administrators",
|
23
|
+
"owner_id": "6a1e70e1a88782771a91808c8af9bbb7a9871389"
|
24
|
+
},
|
25
|
+
"MyProject_Committers": {
|
26
|
+
"kind": "gerritcodereview#group",
|
27
|
+
"id": "834ec36dd5e0ed21a2ff5d7e2255da082d63bbd7",
|
28
|
+
"url": "#/admin/groups/uuid-834ec36dd5e0ed21a2ff5d7e2255da082d63bbd7",
|
29
|
+
"options": {
|
30
|
+
"visible_to_all": true
|
31
|
+
},
|
32
|
+
"group_id": 6,
|
33
|
+
"owner": "MyProject_Committers",
|
34
|
+
"owner_id": "834ec36dd5e0ed21a2ff5d7e2255da082d63bbd7"
|
35
|
+
},
|
36
|
+
"Non-Interactive Users": {
|
37
|
+
"kind": "gerritcodereview#group",
|
38
|
+
"id": "5057f3cbd3519d6ab69364429a89ffdffba50f73",
|
39
|
+
"url": "#/admin/groups/uuid-5057f3cbd3519d6ab69364429a89ffdffba50f73",
|
40
|
+
"options": {
|
41
|
+
},
|
42
|
+
"description": "Users who perform batch actions on Gerrit",
|
43
|
+
"group_id": 4,
|
44
|
+
"owner": "Administrators",
|
45
|
+
"owner_id": "6a1e70e1a88782771a91808c8af9bbb7a9871389"
|
46
|
+
},
|
47
|
+
"Project Owners": {
|
48
|
+
"kind": "gerritcodereview#group",
|
49
|
+
"id": "global%3AProject-Owners",
|
50
|
+
"url": "#/admin/groups/uuid-global%3AProject-Owners",
|
51
|
+
"options": {
|
52
|
+
},
|
53
|
+
"description": "Any owner of the project",
|
54
|
+
"group_id": 5,
|
55
|
+
"owner": "Administrators",
|
56
|
+
"owner_id": "6a1e70e1a88782771a91808c8af9bbb7a9871389"
|
57
|
+
},
|
58
|
+
"Registered Users": {
|
59
|
+
"kind": "gerritcodereview#group",
|
60
|
+
"id": "global%3ARegistered-Users",
|
61
|
+
"url": "#/admin/groups/uuid-global%3ARegistered-Users",
|
62
|
+
"options": {
|
63
|
+
},
|
64
|
+
"description": "Any signed-in user",
|
65
|
+
"group_id": 3,
|
66
|
+
"owner": "Administrators",
|
67
|
+
"owner_id": "6a1e70e1a88782771a91808c8af9bbb7a9871389"
|
68
|
+
}
|
69
|
+
}
|
@@ -0,0 +1,17 @@
|
|
1
|
+
)]}'
|
2
|
+
[
|
3
|
+
{
|
4
|
+
"project": "awesome",
|
5
|
+
"branch": "master",
|
6
|
+
"id": "Idd8747a5ea93bb9bddf7695bab25670be135f777",
|
7
|
+
"subject": "Add an awesome feature",
|
8
|
+
"status": "OPEN",
|
9
|
+
"created": "2012-09-12 15:54:21.531000000",
|
10
|
+
"updated": "2012-10-13 01:34:40.511000000",
|
11
|
+
"_sortkey": "00115dfe00009334",
|
12
|
+
"_number": 3,
|
13
|
+
"owner": {
|
14
|
+
"name": "The Duke"
|
15
|
+
}
|
16
|
+
}
|
17
|
+
]
|
data/spec/groups_spec.rb
ADDED
@@ -0,0 +1,92 @@
|
|
1
|
+
require 'spec_helper'
|
2
|
+
|
3
|
+
describe '.list_groups' do
|
4
|
+
it 'fetchs all groups' do
|
5
|
+
stub = stub_get('/groups/', 'groups.json')
|
6
|
+
|
7
|
+
client = MockGerry.new
|
8
|
+
groups = client.groups
|
9
|
+
expect(stub).to have_been_requested
|
10
|
+
|
11
|
+
expect(groups.size).to eq(6)
|
12
|
+
expect(groups).to include('Project Owners')
|
13
|
+
end
|
14
|
+
end
|
15
|
+
|
16
|
+
describe '.group_members' do
|
17
|
+
it "fetchs all members of the specified group" do
|
18
|
+
stub = stub_get('/groups/834ec36dd5e0ed21a2ff5d7e2255da082d63bbd7/members/', 'group_members.json')
|
19
|
+
|
20
|
+
client = MockGerry.new
|
21
|
+
group_members = client.group_members('834ec36dd5e0ed21a2ff5d7e2255da082d63bbd7')
|
22
|
+
expect(stub).to have_been_requested
|
23
|
+
|
24
|
+
expect(group_members.size).to eq(2)
|
25
|
+
expect(group_members[1]['email']).to eq('john.doe@example.com')
|
26
|
+
end
|
27
|
+
end
|
28
|
+
|
29
|
+
describe '.create_group' do
|
30
|
+
let(:name) { "my_new_group_name" }
|
31
|
+
let(:description) { "group description" }
|
32
|
+
let(:visible) { true }
|
33
|
+
let(:owner_id) { nil }
|
34
|
+
|
35
|
+
it "creates a group" do
|
36
|
+
input = {
|
37
|
+
description: description,
|
38
|
+
visible_to_all: visible
|
39
|
+
}
|
40
|
+
|
41
|
+
response = %Q<)]}'
|
42
|
+
{
|
43
|
+
"kind": "gerritcodereview#group",
|
44
|
+
"id": "9999c971bb4ab872aab759d8c49833ee6b9ff320",
|
45
|
+
"name": "#{name}",
|
46
|
+
"url": "#/admin/groups/uuid-9999c971bb4ab872aab759d8c49833ee6b9ff320",
|
47
|
+
"options": {
|
48
|
+
"visible_to_all": true
|
49
|
+
},
|
50
|
+
"description":"#{description}",
|
51
|
+
"group_id": 551,
|
52
|
+
"owner": "#{name}",
|
53
|
+
"owner_id": "9999c971bb4ab872aab759d8c49833ee6b9ff320"
|
54
|
+
}
|
55
|
+
>
|
56
|
+
stub = stub_put('/groups/my_new_group_name', input.to_json, response)
|
57
|
+
|
58
|
+
client = MockGerry.new
|
59
|
+
new_group = client.create_group(name, description, visible, owner_id)
|
60
|
+
expect(stub).to have_been_requested
|
61
|
+
end
|
62
|
+
end
|
63
|
+
|
64
|
+
describe '.add_to_group' do
|
65
|
+
it "adds users to a group" do
|
66
|
+
users = %w(jane.roe@example.com john.doe@example.com)
|
67
|
+
group_id = "9999c971bb4ab872aab759d8c49833ee6b9ff320"
|
68
|
+
input = {
|
69
|
+
members: users
|
70
|
+
}
|
71
|
+
stub = stub_post("/groups/#{group_id}/members", input.to_json, get_fixture('group_members.json'))
|
72
|
+
|
73
|
+
client = MockGerry.new
|
74
|
+
new_group = client.add_to_group(group_id, users)
|
75
|
+
expect(stub).to have_been_requested
|
76
|
+
end
|
77
|
+
end
|
78
|
+
|
79
|
+
describe '.remove_from_group' do
|
80
|
+
it "removes users from a group" do
|
81
|
+
users = %w(jane.roe@example.com john.doe@example.com)
|
82
|
+
group_id = "9999c971bb4ab872aab759d8c49833ee6b9ff320"
|
83
|
+
input = {
|
84
|
+
members: users
|
85
|
+
}
|
86
|
+
stub = stub_post("/groups/#{group_id}/members.delete", input.to_json, "")
|
87
|
+
|
88
|
+
client = MockGerry.new
|
89
|
+
new_group = client.remove_from_group(group_id, users)
|
90
|
+
expect(stub).to have_been_requested
|
91
|
+
end
|
92
|
+
end
|
@@ -0,0 +1,26 @@
|
|
1
|
+
require 'spec_helper'
|
2
|
+
|
3
|
+
describe '.projects' do
|
4
|
+
it 'should fetch all projects' do
|
5
|
+
stub = stub_get('/projects/', 'projects.json')
|
6
|
+
|
7
|
+
client = MockGerry.new
|
8
|
+
projects = client.projects
|
9
|
+
|
10
|
+
expect(stub).to have_been_requested
|
11
|
+
|
12
|
+
expect(projects['awesome']['description']).to eq('Awesome project')
|
13
|
+
expect(projects['clean']['description']).to eq('Clean code!')
|
14
|
+
end
|
15
|
+
|
16
|
+
it 'should fetch a project' do
|
17
|
+
stub = stub_get('/projects/awesome', 'projects.json')
|
18
|
+
|
19
|
+
client = MockGerry.new
|
20
|
+
projects = client.find_project('awesome')
|
21
|
+
|
22
|
+
expect(stub).to have_been_requested
|
23
|
+
|
24
|
+
expect(projects['awesome']['description']).to eq('Awesome project')
|
25
|
+
end
|
26
|
+
end
|
@@ -0,0 +1,42 @@
|
|
1
|
+
require 'spec_helper'
|
2
|
+
|
3
|
+
describe '.map_options' do
|
4
|
+
it 'should map the query options' do
|
5
|
+
|
6
|
+
client = MockGerry.new
|
7
|
+
options = client.map_options(['q=createAccount', 'q=createGroup'])
|
8
|
+
|
9
|
+
expect(options).to eq('q=createAccount&q=createGroup')
|
10
|
+
end
|
11
|
+
end
|
12
|
+
|
13
|
+
describe '.get' do
|
14
|
+
it 'should request projects as anoymous' do
|
15
|
+
stub = stub_get('/projects/', 'projects.json')
|
16
|
+
|
17
|
+
client = MockGerry.new
|
18
|
+
client.projects
|
19
|
+
|
20
|
+
expect(stub).to have_been_requested
|
21
|
+
end
|
22
|
+
|
23
|
+
it 'should request projects as user' do
|
24
|
+
username = 'gerry'
|
25
|
+
password = 'whoop'
|
26
|
+
|
27
|
+
body = get_fixture('projects.json')
|
28
|
+
|
29
|
+
stub = stub_request(:get, "http://localhost/a/projects/").
|
30
|
+
with(:headers => {'Accept'=>'application/json'}).
|
31
|
+
to_return(:status => 200, :body => body, :headers => {})
|
32
|
+
|
33
|
+
client = Gerry.new(MockGerry::URL, 'gerry', 'whoop')
|
34
|
+
projects = client.projects
|
35
|
+
|
36
|
+
# twice because the first is the auth challenge and then the actual request
|
37
|
+
expect(stub).to have_been_requested.twice
|
38
|
+
|
39
|
+
expect(projects['awesome']['description']).to eq('Awesome project')
|
40
|
+
expect(projects['clean']['description']).to eq('Clean code!')
|
41
|
+
end
|
42
|
+
end
|
data/spec/spec_helper.rb
ADDED
@@ -0,0 +1,50 @@
|
|
1
|
+
require "rspec/expectations"
|
2
|
+
require 'webmock/rspec'
|
3
|
+
|
4
|
+
require_relative '../lib/gerry'
|
5
|
+
|
6
|
+
class MockGerry < Gerry::Client
|
7
|
+
URL = 'http://localhost'
|
8
|
+
|
9
|
+
def initialize
|
10
|
+
super(URL)
|
11
|
+
end
|
12
|
+
end
|
13
|
+
|
14
|
+
def get_fixture(filename)
|
15
|
+
return '' if filename.empty?
|
16
|
+
file_path = File.expand_path(File.dirname(__FILE__) + '/fixtures/' + filename)
|
17
|
+
File.read(file_path)
|
18
|
+
end
|
19
|
+
|
20
|
+
def stub_get(url, filename)
|
21
|
+
body = get_fixture(filename)
|
22
|
+
stub_request(:get, "#{MockGerry::URL}#{url}").
|
23
|
+
to_return(:status => 200, :body => "#{body}", :headers => {'Content-Type' => 'application/json'})
|
24
|
+
end
|
25
|
+
|
26
|
+
def stub_put(url, body, response_body=nil)
|
27
|
+
response = {
|
28
|
+
status: 200,
|
29
|
+
headers: {
|
30
|
+
'Content-Type' => 'application/json'
|
31
|
+
},
|
32
|
+
body: response_body
|
33
|
+
}
|
34
|
+
stub_request(:put, "#{MockGerry::URL}#{url}").
|
35
|
+
with(:body => body, :headers => { 'Content-Type' => 'application/json' }).
|
36
|
+
to_return(response)
|
37
|
+
end
|
38
|
+
|
39
|
+
def stub_post(url, body, response_body=nil)
|
40
|
+
response = {
|
41
|
+
status: 200,
|
42
|
+
headers: {
|
43
|
+
'Content-Type' => 'application/json'
|
44
|
+
},
|
45
|
+
body: response_body
|
46
|
+
}
|
47
|
+
stub_request(:post, "#{MockGerry::URL}#{url}").
|
48
|
+
with(:body => body, :headers => { 'Content-Type' => 'application/json' }).
|
49
|
+
to_return(response)
|
50
|
+
end
|
metadata
ADDED
@@ -0,0 +1,195 @@
|
|
1
|
+
--- !ruby/object:Gem::Specification
|
2
|
+
name: gerry
|
3
|
+
version: !ruby/object:Gem::Version
|
4
|
+
version: 0.0.3
|
5
|
+
platform: ruby
|
6
|
+
authors:
|
7
|
+
- Fabian Mettler
|
8
|
+
- Andrew Erickson
|
9
|
+
- Travis Truman
|
10
|
+
autorequire:
|
11
|
+
bindir: bin
|
12
|
+
cert_chain: []
|
13
|
+
date: 2015-06-12 00:00:00.000000000 Z
|
14
|
+
dependencies:
|
15
|
+
- !ruby/object:Gem::Dependency
|
16
|
+
name: httparty
|
17
|
+
requirement: !ruby/object:Gem::Requirement
|
18
|
+
requirements:
|
19
|
+
- - ">="
|
20
|
+
- !ruby/object:Gem::Version
|
21
|
+
version: '0'
|
22
|
+
type: :runtime
|
23
|
+
prerelease: false
|
24
|
+
version_requirements: !ruby/object:Gem::Requirement
|
25
|
+
requirements:
|
26
|
+
- - ">="
|
27
|
+
- !ruby/object:Gem::Version
|
28
|
+
version: '0'
|
29
|
+
- !ruby/object:Gem::Dependency
|
30
|
+
name: rake
|
31
|
+
requirement: !ruby/object:Gem::Requirement
|
32
|
+
requirements:
|
33
|
+
- - ">="
|
34
|
+
- !ruby/object:Gem::Version
|
35
|
+
version: '0'
|
36
|
+
type: :development
|
37
|
+
prerelease: false
|
38
|
+
version_requirements: !ruby/object:Gem::Requirement
|
39
|
+
requirements:
|
40
|
+
- - ">="
|
41
|
+
- !ruby/object:Gem::Version
|
42
|
+
version: '0'
|
43
|
+
- !ruby/object:Gem::Dependency
|
44
|
+
name: rspec
|
45
|
+
requirement: !ruby/object:Gem::Requirement
|
46
|
+
requirements:
|
47
|
+
- - "~>"
|
48
|
+
- !ruby/object:Gem::Version
|
49
|
+
version: 3.1.0
|
50
|
+
type: :development
|
51
|
+
prerelease: false
|
52
|
+
version_requirements: !ruby/object:Gem::Requirement
|
53
|
+
requirements:
|
54
|
+
- - "~>"
|
55
|
+
- !ruby/object:Gem::Version
|
56
|
+
version: 3.1.0
|
57
|
+
- !ruby/object:Gem::Dependency
|
58
|
+
name: rack-test
|
59
|
+
requirement: !ruby/object:Gem::Requirement
|
60
|
+
requirements:
|
61
|
+
- - ">="
|
62
|
+
- !ruby/object:Gem::Version
|
63
|
+
version: '0'
|
64
|
+
type: :development
|
65
|
+
prerelease: false
|
66
|
+
version_requirements: !ruby/object:Gem::Requirement
|
67
|
+
requirements:
|
68
|
+
- - ">="
|
69
|
+
- !ruby/object:Gem::Version
|
70
|
+
version: '0'
|
71
|
+
- !ruby/object:Gem::Dependency
|
72
|
+
name: webmock
|
73
|
+
requirement: !ruby/object:Gem::Requirement
|
74
|
+
requirements:
|
75
|
+
- - ">="
|
76
|
+
- !ruby/object:Gem::Version
|
77
|
+
version: '0'
|
78
|
+
type: :development
|
79
|
+
prerelease: false
|
80
|
+
version_requirements: !ruby/object:Gem::Requirement
|
81
|
+
requirements:
|
82
|
+
- - ">="
|
83
|
+
- !ruby/object:Gem::Version
|
84
|
+
version: '0'
|
85
|
+
- !ruby/object:Gem::Dependency
|
86
|
+
name: guard
|
87
|
+
requirement: !ruby/object:Gem::Requirement
|
88
|
+
requirements:
|
89
|
+
- - ">="
|
90
|
+
- !ruby/object:Gem::Version
|
91
|
+
version: '0'
|
92
|
+
type: :development
|
93
|
+
prerelease: false
|
94
|
+
version_requirements: !ruby/object:Gem::Requirement
|
95
|
+
requirements:
|
96
|
+
- - ">="
|
97
|
+
- !ruby/object:Gem::Version
|
98
|
+
version: '0'
|
99
|
+
- !ruby/object:Gem::Dependency
|
100
|
+
name: guard-rspec
|
101
|
+
requirement: !ruby/object:Gem::Requirement
|
102
|
+
requirements:
|
103
|
+
- - ">="
|
104
|
+
- !ruby/object:Gem::Version
|
105
|
+
version: '0'
|
106
|
+
type: :development
|
107
|
+
prerelease: false
|
108
|
+
version_requirements: !ruby/object:Gem::Requirement
|
109
|
+
requirements:
|
110
|
+
- - ">="
|
111
|
+
- !ruby/object:Gem::Version
|
112
|
+
version: '0'
|
113
|
+
- !ruby/object:Gem::Dependency
|
114
|
+
name: rb-readline
|
115
|
+
requirement: !ruby/object:Gem::Requirement
|
116
|
+
requirements:
|
117
|
+
- - ">="
|
118
|
+
- !ruby/object:Gem::Version
|
119
|
+
version: '0'
|
120
|
+
type: :development
|
121
|
+
prerelease: false
|
122
|
+
version_requirements: !ruby/object:Gem::Requirement
|
123
|
+
requirements:
|
124
|
+
- - ">="
|
125
|
+
- !ruby/object:Gem::Version
|
126
|
+
version: '0'
|
127
|
+
description: |2
|
128
|
+
Simple Ruby wrapper for the Gerrit Code Review REST-API.
|
129
|
+
email: trumant@gmail.com
|
130
|
+
executables: []
|
131
|
+
extensions: []
|
132
|
+
extra_rdoc_files: []
|
133
|
+
files:
|
134
|
+
- README.md
|
135
|
+
- Rakefile
|
136
|
+
- lib/gerry.rb
|
137
|
+
- lib/gerry/client.rb
|
138
|
+
- lib/gerry/client/accounts.rb
|
139
|
+
- lib/gerry/client/changes.rb
|
140
|
+
- lib/gerry/client/groups.rb
|
141
|
+
- lib/gerry/client/projects.rb
|
142
|
+
- lib/gerry/client/request.rb
|
143
|
+
- lib/gerry/version.rb
|
144
|
+
- spec/accounts_spec.rb
|
145
|
+
- spec/changes_spec.rb
|
146
|
+
- spec/fixtures/account_groups.json
|
147
|
+
- spec/fixtures/capabilities.json
|
148
|
+
- spec/fixtures/changes.json
|
149
|
+
- spec/fixtures/group_members.json
|
150
|
+
- spec/fixtures/groups.json
|
151
|
+
- spec/fixtures/open_changes.json
|
152
|
+
- spec/fixtures/projects.json
|
153
|
+
- spec/fixtures/query_capabilities.json
|
154
|
+
- spec/groups_spec.rb
|
155
|
+
- spec/projects_spec.rb
|
156
|
+
- spec/request_spec.rb
|
157
|
+
- spec/spec_helper.rb
|
158
|
+
homepage: http://github.com/trumant/gerry
|
159
|
+
licenses: []
|
160
|
+
metadata: {}
|
161
|
+
post_install_message:
|
162
|
+
rdoc_options: []
|
163
|
+
require_paths:
|
164
|
+
- lib
|
165
|
+
required_ruby_version: !ruby/object:Gem::Requirement
|
166
|
+
requirements:
|
167
|
+
- - ">="
|
168
|
+
- !ruby/object:Gem::Version
|
169
|
+
version: '0'
|
170
|
+
required_rubygems_version: !ruby/object:Gem::Requirement
|
171
|
+
requirements:
|
172
|
+
- - ">="
|
173
|
+
- !ruby/object:Gem::Version
|
174
|
+
version: '0'
|
175
|
+
requirements: []
|
176
|
+
rubyforge_project:
|
177
|
+
rubygems_version: 2.2.2
|
178
|
+
signing_key:
|
179
|
+
specification_version: 4
|
180
|
+
summary: Simple Ruby wrapper for the Gerrit Code Review REST-API.
|
181
|
+
test_files:
|
182
|
+
- spec/accounts_spec.rb
|
183
|
+
- spec/changes_spec.rb
|
184
|
+
- spec/fixtures/account_groups.json
|
185
|
+
- spec/fixtures/capabilities.json
|
186
|
+
- spec/fixtures/changes.json
|
187
|
+
- spec/fixtures/group_members.json
|
188
|
+
- spec/fixtures/groups.json
|
189
|
+
- spec/fixtures/open_changes.json
|
190
|
+
- spec/fixtures/projects.json
|
191
|
+
- spec/fixtures/query_capabilities.json
|
192
|
+
- spec/groups_spec.rb
|
193
|
+
- spec/projects_spec.rb
|
194
|
+
- spec/request_spec.rb
|
195
|
+
- spec/spec_helper.rb
|