eye 0.2.3 → 0.2.4
This diff represents the content of publicly available package versions that have been released to one of the supported registries. The information contained in this diff is provided for informational purposes only and reflects changes between package versions as they appear in their respective public registries.
- checksums.yaml +4 -4
- data/.travis.yml +3 -0
- data/README.md +5 -0
- data/bin/eye +7 -0
- data/lib/eye.rb +1 -1
- data/lib/eye/application.rb +4 -0
- data/lib/eye/checker.rb +6 -1
- data/lib/eye/checker/cpu.rb +1 -1
- data/lib/eye/checker/file_ctime.rb +0 -4
- data/lib/eye/checker/file_size.rb +0 -4
- data/lib/eye/checker/http.rb +2 -10
- data/lib/eye/checker/memory.rb +1 -1
- data/lib/eye/checker/socket.rb +2 -10
- data/lib/eye/controller.rb +2 -0
- data/lib/eye/controller/commands.rb +9 -2
- data/lib/eye/controller/load.rb +2 -4
- data/lib/eye/controller/show_history.rb +62 -0
- data/lib/eye/controller/status.rb +0 -7
- data/lib/eye/dsl/child_process_opts.rb +2 -1
- data/lib/eye/process/controller.rb +4 -0
- data/lib/eye/process/data.rb +2 -2
- data/lib/eye/process/monitor.rb +1 -1
- data/lib/eye/process/scheduler.rb +6 -0
- data/lib/eye/process/states_history.rb +7 -3
- data/lib/eye/system.rb +14 -20
- data/lib/eye/system_resources.rb +9 -6
- data/lib/eye/utils/alive_array.rb +1 -1
- data/spec/controller/controller_spec.rb +16 -0
- data/spec/controller/intergration_spec.rb +1 -1
- data/spec/process/restart_spec.rb +13 -1
- data/spec/process/scheduler_spec.rb +11 -1
- data/spec/spec_helper.rb +0 -1
- data/spec/support/rr_celluloid.rb +9 -30
- data/spec/system_resources_spec.rb +16 -18
- data/spec/system_spec.rb +2 -2
- metadata +4 -4
- data/spec/support/scheduler_hack.rb +0 -16
checksums.yaml
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
---
|
2
2
|
SHA1:
|
3
|
-
metadata.gz:
|
4
|
-
data.tar.gz:
|
3
|
+
metadata.gz: 7f123c5b720394e6881f617954469fac8a054e5b
|
4
|
+
data.tar.gz: d717bad68e6f059afd86ea9b05975b59c5f8e36d
|
5
5
|
SHA512:
|
6
|
-
metadata.gz:
|
7
|
-
data.tar.gz:
|
6
|
+
metadata.gz: b56a789a1a18fd4acdda18ea67943bf1c4861e9fe46e8dce7c4b815ca17a1c2367a013e91fa3006669af4c61d470b3b8425792d1c4154a63090023799cf34416
|
7
|
+
data.tar.gz: fa7ef643b765bfc27bb4c2bab36d73f9277c82d1ca22df3dd857b9597e98f00e5896811f77fdc26b24785e504153bfd17a77b883bec693662e904ac50a836347
|
data/.travis.yml
ADDED
data/README.md
CHANGED
@@ -1,8 +1,13 @@
|
|
1
1
|
Eye
|
2
2
|
===
|
3
3
|
|
4
|
+
[](http://travis-ci.org/kostya/eye)
|
5
|
+
|
4
6
|
Process monitoring tool. An alternative to God and Bluepill. With Bluepill like config syntax. Requires MRI Ruby >= 1.9.2. Uses Celluloid and Celluloid::IO.
|
5
7
|
|
8
|
+
Little demo, shows general commands and how chain works:
|
9
|
+
|
10
|
+
[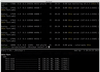](https://raw.github.com/kostya/stuff/master/eye/eye.gif)
|
6
11
|
|
7
12
|
Recommended installation on the server (system wide):
|
8
13
|
|
data/bin/eye
CHANGED
@@ -83,6 +83,13 @@ class Cli < Thor
|
|
83
83
|
send_command(:break_chain, *targets)
|
84
84
|
end
|
85
85
|
|
86
|
+
desc "history TARGET[,...]", "show process states history"
|
87
|
+
def history(*targets)
|
88
|
+
res = cmd(:history, *targets)
|
89
|
+
puts res if res && !res.empty?
|
90
|
+
puts
|
91
|
+
end
|
92
|
+
|
86
93
|
desc "trace [TARGET]", "tracing log for app,group or process"
|
87
94
|
def trace(target = "")
|
88
95
|
log_trace(target)
|
data/lib/eye.rb
CHANGED
data/lib/eye/application.rb
CHANGED
data/lib/eye/checker.rb
CHANGED
@@ -78,7 +78,7 @@ class Eye::Checker
|
|
78
78
|
end
|
79
79
|
|
80
80
|
def check_name
|
81
|
-
|
81
|
+
@check_name ||= @type.to_s
|
82
82
|
end
|
83
83
|
|
84
84
|
def max_tries
|
@@ -114,4 +114,9 @@ class Eye::Checker
|
|
114
114
|
param :times, [Fixnum, Array]
|
115
115
|
param :fire, Symbol, nil, nil, [:stop, :restart, :unmonitor, :nothing]
|
116
116
|
|
117
|
+
class Defer < Eye::Checker
|
118
|
+
def get_value
|
119
|
+
Celluloid::Future.new{ get_value_deferred }.value
|
120
|
+
end
|
121
|
+
end
|
117
122
|
end
|
data/lib/eye/checker/cpu.rb
CHANGED
data/lib/eye/checker/http.rb
CHANGED
@@ -1,6 +1,6 @@
|
|
1
1
|
require 'net/http'
|
2
2
|
|
3
|
-
class Eye::Checker::Http < Eye::Checker
|
3
|
+
class Eye::Checker::Http < Eye::Checker::Defer
|
4
4
|
|
5
5
|
# checks :http, :every => 5.seconds, :times => 1,
|
6
6
|
# :url => "http://127.0.0.1:3000/", :kind => :success, :pattern => /OK/, :timeout => 3.seconds
|
@@ -14,10 +14,6 @@ class Eye::Checker::Http < Eye::Checker
|
|
14
14
|
|
15
15
|
attr_reader :uri
|
16
16
|
|
17
|
-
def check_name
|
18
|
-
'http'
|
19
|
-
end
|
20
|
-
|
21
17
|
def initialize(*args)
|
22
18
|
super
|
23
19
|
|
@@ -33,11 +29,7 @@ class Eye::Checker::Http < Eye::Checker
|
|
33
29
|
@read_timeout = (read_timeout || timeout || 15).to_f
|
34
30
|
end
|
35
31
|
|
36
|
-
def
|
37
|
-
Celluloid::Future.new{ get_value_sync }.value
|
38
|
-
end
|
39
|
-
|
40
|
-
def get_value_sync
|
32
|
+
def get_value_deferred
|
41
33
|
res = session.start{ |http| http.get(@uri.request_uri) }
|
42
34
|
{:result => res}
|
43
35
|
|
data/lib/eye/checker/memory.rb
CHANGED
data/lib/eye/checker/socket.rb
CHANGED
@@ -1,4 +1,4 @@
|
|
1
|
-
class Eye::Checker::Socket < Eye::Checker
|
1
|
+
class Eye::Checker::Socket < Eye::Checker::Defer
|
2
2
|
|
3
3
|
# checks :socket, :every => 5.seconds, :times => 1,
|
4
4
|
# :addr => "unix:/var/run/daemon.sock", :timeout => 3.seconds,
|
@@ -20,10 +20,6 @@ class Eye::Checker::Socket < Eye::Checker
|
|
20
20
|
param :expect_data, [String, Regexp, Proc]
|
21
21
|
param :protocol, [Symbol], nil, nil, [:default, :em_object]
|
22
22
|
|
23
|
-
def check_name
|
24
|
-
'socket'
|
25
|
-
end
|
26
|
-
|
27
23
|
def initialize(*args)
|
28
24
|
super
|
29
25
|
@open_timeout = (open_timeout || 1).to_f
|
@@ -39,11 +35,7 @@ class Eye::Checker::Socket < Eye::Checker
|
|
39
35
|
end
|
40
36
|
end
|
41
37
|
|
42
|
-
def
|
43
|
-
Celluloid::Future.new{ get_value_sync }.value
|
44
|
-
end
|
45
|
-
|
46
|
-
def get_value_sync
|
38
|
+
def get_value_deferred
|
47
39
|
sock = begin
|
48
40
|
Timeout::timeout(@open_timeout){ open_socket }
|
49
41
|
rescue Timeout::Error
|
data/lib/eye/controller.rb
CHANGED
@@ -19,6 +19,7 @@ class Eye::Controller
|
|
19
19
|
autoload :Commands, 'eye/controller/commands'
|
20
20
|
autoload :Status, 'eye/controller/status'
|
21
21
|
autoload :SendCommand, 'eye/controller/send_command'
|
22
|
+
autoload :ShowHistory, 'eye/controller/show_history'
|
22
23
|
|
23
24
|
include Eye::Logger::Helpers
|
24
25
|
include Eye::Controller::Load
|
@@ -26,6 +27,7 @@ class Eye::Controller
|
|
26
27
|
include Eye::Controller::Commands
|
27
28
|
include Eye::Controller::Status
|
28
29
|
include Eye::Controller::SendCommand
|
30
|
+
include Eye::Controller::ShowHistory
|
29
31
|
|
30
32
|
attr_reader :applications, :current_config
|
31
33
|
|
@@ -20,12 +20,12 @@ module Eye::Controller::Commands
|
|
20
20
|
exclusive{ load(*args) }
|
21
21
|
when :info
|
22
22
|
info_string(*args)
|
23
|
-
when :object_info
|
24
|
-
info_data(*args)
|
25
23
|
when :xinfo
|
26
24
|
info_string_debug(*args)
|
27
25
|
when :oinfo
|
28
26
|
info_string_short
|
27
|
+
when :history
|
28
|
+
history_string(*args)
|
29
29
|
when :quit
|
30
30
|
quit
|
31
31
|
when :check
|
@@ -38,6 +38,13 @@ module Eye::Controller::Commands
|
|
38
38
|
:pong
|
39
39
|
when :logger_dev
|
40
40
|
Eye::Logger.dev
|
41
|
+
|
42
|
+
# object commands, for api
|
43
|
+
when :raw_info
|
44
|
+
info_data(*args)
|
45
|
+
when :raw_history
|
46
|
+
history_data(*args)
|
47
|
+
|
41
48
|
else
|
42
49
|
:unknown_command
|
43
50
|
end
|
data/lib/eye/controller/load.rb
CHANGED
@@ -27,9 +27,8 @@ private
|
|
27
27
|
BT_REGX = %r[/lib/eye/|lib/celluloid|internal:prelude|logger.rb:|active_support/core_ext|shellwords.rb].freeze
|
28
28
|
|
29
29
|
def catch_load_error(filename, &block)
|
30
|
-
|
30
|
+
{:error => false, :config => yield }
|
31
31
|
|
32
|
-
{:error => false, :config => res}
|
33
32
|
rescue Eye::Dsl::Error, Exception, NoMethodError => ex
|
34
33
|
error "load: config error <#{filename}>: #{ex.message}"
|
35
34
|
|
@@ -81,12 +80,11 @@ private
|
|
81
80
|
end
|
82
81
|
|
83
82
|
def _load(filename)
|
83
|
+
info "load: #{filename} in #{Eye::VERSION}"
|
84
84
|
new_cfg = parse_set_of_configs(filename)
|
85
85
|
|
86
86
|
load_config(new_cfg, @loaded_config[:applications].keys)
|
87
87
|
@loaded_config = nil
|
88
|
-
|
89
|
-
GC.start
|
90
88
|
end
|
91
89
|
|
92
90
|
def load_config(new_config, changed_apps = [])
|
@@ -0,0 +1,62 @@
|
|
1
|
+
module Eye::Controller::ShowHistory
|
2
|
+
|
3
|
+
def history_string(*obj_strs)
|
4
|
+
data = history_data(*obj_strs)
|
5
|
+
|
6
|
+
res = []
|
7
|
+
data.each do |name, data|
|
8
|
+
res << detail_process_info(name, data)
|
9
|
+
end
|
10
|
+
|
11
|
+
res * "\n"
|
12
|
+
end
|
13
|
+
|
14
|
+
def history_data(*obj_strs)
|
15
|
+
res = {}
|
16
|
+
get_processes_for_history(*obj_strs).each do |process|
|
17
|
+
res[process.full_name] = process.schedule_history.reject{|c| c[:state] == :check_crash }
|
18
|
+
end
|
19
|
+
res
|
20
|
+
end
|
21
|
+
|
22
|
+
private
|
23
|
+
|
24
|
+
def get_processes_for_history(*obj_strs)
|
25
|
+
res = []
|
26
|
+
matched_objects(*obj_strs) do |obj|
|
27
|
+
if (obj.is_a?(Eye::Process) || obj.is_a?(Eye::ChildProcess))
|
28
|
+
res << obj
|
29
|
+
else
|
30
|
+
res += obj.processes.to_a
|
31
|
+
end
|
32
|
+
end
|
33
|
+
Eye::Utils::AliveArray.new(res)
|
34
|
+
end
|
35
|
+
|
36
|
+
def detail_process_info(name, history)
|
37
|
+
return if history.empty?
|
38
|
+
|
39
|
+
res = "\033[1m#{name}\033[0m:\n"
|
40
|
+
history = history.reverse
|
41
|
+
|
42
|
+
history.chunk{|h| [h[:state], h[:reason]] }.each do |_, hist|
|
43
|
+
if hist.size >= 3
|
44
|
+
res << detail_process_info_string(hist[0])
|
45
|
+
res << detail_process_info_string(:state => "... #{hist.size - 2} times", :reason => '...', :at => hist[-1][:at])
|
46
|
+
res << detail_process_info_string(hist[-1])
|
47
|
+
else
|
48
|
+
hist.each do |h|
|
49
|
+
res << detail_process_info_string(h)
|
50
|
+
end
|
51
|
+
end
|
52
|
+
end
|
53
|
+
|
54
|
+
res
|
55
|
+
end
|
56
|
+
|
57
|
+
def detail_process_info_string(h)
|
58
|
+
state = h[:state].to_s.ljust(14)
|
59
|
+
"#{Time.at(h[:at]).to_s(:db)} - #{state} (#{h[:reason]})\n"
|
60
|
+
end
|
61
|
+
|
62
|
+
end
|
@@ -97,8 +97,6 @@ private
|
|
97
97
|
end
|
98
98
|
end
|
99
99
|
|
100
|
-
REV_REGX = /r:([a-z0-9]{5,})/i
|
101
|
-
|
102
100
|
def resources_str(r, mb = true)
|
103
101
|
return '' if r.blank?
|
104
102
|
|
@@ -109,11 +107,6 @@ private
|
|
109
107
|
res += ", #{mem}"
|
110
108
|
end
|
111
109
|
|
112
|
-
# show process revision, as parse from procline part: 'r:12345678'
|
113
|
-
if r[:cmd] && r[:cmd].to_s =~ REV_REGX
|
114
|
-
res += ", #{$1.to_s[0..5]}"
|
115
|
-
end
|
116
|
-
|
117
110
|
res += ", <#{r[:pid]}>"
|
118
111
|
|
119
112
|
res
|
@@ -1,7 +1,8 @@
|
|
1
1
|
class Eye::Dsl::ChildProcessOpts < Eye::Dsl::Opts
|
2
2
|
|
3
3
|
def allow_options
|
4
|
-
[:stop_command, :restart_command, :childs_update_period
|
4
|
+
[:stop_command, :restart_command, :childs_update_period,
|
5
|
+
:stop_signals, :stop_grace, :stop_timeout, :restart_timeout]
|
5
6
|
end
|
6
7
|
|
7
8
|
end
|
data/lib/eye/process/data.rb
CHANGED
@@ -24,8 +24,8 @@ module Eye::Process::Data
|
|
24
24
|
resources: Eye::SystemResources.resources(pid) }
|
25
25
|
|
26
26
|
if @states_history
|
27
|
-
h.merge!( state_changed_at: @states_history.
|
28
|
-
state_reason: @states_history.
|
27
|
+
h.merge!( state_changed_at: @states_history.last_state_changed_at,
|
28
|
+
state_reason: @states_history.last_reason )
|
29
29
|
end
|
30
30
|
|
31
31
|
h.merge!(debug: debug_data) if debug
|
data/lib/eye/process/monitor.rb
CHANGED
@@ -37,6 +37,8 @@ module Eye::Process::Scheduler
|
|
37
37
|
send(command, *h[:args], &block)
|
38
38
|
@current_scheduled_command = nil
|
39
39
|
info "<= #{command}"
|
40
|
+
|
41
|
+
schedule_history.push(command, reason, @last_scheduled_at.to_i)
|
40
42
|
end
|
41
43
|
|
42
44
|
def scheduler_actions_list
|
@@ -50,6 +52,10 @@ module Eye::Process::Scheduler
|
|
50
52
|
attr_accessor :current_scheduled_command
|
51
53
|
attr_accessor :last_scheduled_command, :last_scheduled_reason, :last_scheduled_at
|
52
54
|
|
55
|
+
def schedule_history
|
56
|
+
@schedule_history ||= Eye::Process::StatesHistory.new(50)
|
57
|
+
end
|
58
|
+
|
53
59
|
private
|
54
60
|
|
55
61
|
def remove_scheduler
|
@@ -1,7 +1,7 @@
|
|
1
1
|
class Eye::Process::StatesHistory < Eye::Utils::Tail
|
2
2
|
|
3
3
|
def push(state, reason = nil, tm = Time.now)
|
4
|
-
super(state: state, at: tm, reason: reason)
|
4
|
+
super(state: state, at: tm.to_i, reason: reason)
|
5
5
|
end
|
6
6
|
|
7
7
|
def states
|
@@ -11,7 +11,7 @@ class Eye::Process::StatesHistory < Eye::Utils::Tail
|
|
11
11
|
def states_for_period(period, from_time = nil)
|
12
12
|
tm = Time.now - period
|
13
13
|
tm = [tm, from_time].max if from_time
|
14
|
-
|
14
|
+
tm = tm.to_f
|
15
15
|
self.select{|s| s[:at] >= tm }.map{|c| c[:state] }
|
16
16
|
end
|
17
17
|
|
@@ -19,8 +19,12 @@ class Eye::Process::StatesHistory < Eye::Utils::Tail
|
|
19
19
|
last[:state]
|
20
20
|
end
|
21
21
|
|
22
|
+
def last_reason
|
23
|
+
last[:reason]
|
24
|
+
end
|
25
|
+
|
22
26
|
def last_state_changed_at
|
23
|
-
last[:at]
|
27
|
+
Time.at(last[:at])
|
24
28
|
end
|
25
29
|
|
26
30
|
def seq?(*seq)
|
data/lib/eye/system.rb
CHANGED
@@ -95,29 +95,17 @@ module Eye::System
|
|
95
95
|
end
|
96
96
|
|
97
97
|
# get table
|
98
|
-
# {pid => {:rss =>, :cpu =>, :ppid => , :
|
98
|
+
# {pid => {:rss =>, :cpu =>, :ppid => , :start_time => }}
|
99
99
|
# slow
|
100
100
|
def ps_aux
|
101
|
-
|
102
|
-
|
103
|
-
|
104
|
-
|
105
|
-
|
106
|
-
|
107
|
-
str = Process.send('`', cmd).force_encoding('binary')
|
108
|
-
lines = str.split("\n")
|
109
|
-
lines.shift # remove first line
|
110
|
-
lines.inject(Hash.new) do |mem, line|
|
111
|
-
chunk = line.strip.split(/\s+/).map(&:strip)
|
112
|
-
mem[chunk[0].to_i] = {
|
113
|
-
:rss => chunk[3].to_i,
|
114
|
-
:cpu => chunk[2].to_i,
|
115
|
-
:ppid => chunk[1].to_i,
|
116
|
-
:start_time => chunk[4],
|
117
|
-
:cmd => chunk[5..-1].join(' ')
|
118
|
-
}
|
119
|
-
mem
|
101
|
+
str = Process.send('`', PS_AUX_CMD).force_encoding('binary')
|
102
|
+
h = {}
|
103
|
+
str.each_line do |line|
|
104
|
+
chunk = line.strip.split(/\s+/)
|
105
|
+
h[chunk[0].to_i] = { :ppid => chunk[1].to_i, :cpu => chunk[2].to_i,
|
106
|
+
:rss => chunk[3].to_i, :start_time => chunk[4] }
|
120
107
|
end
|
108
|
+
h
|
121
109
|
end
|
122
110
|
|
123
111
|
# normalize file
|
@@ -136,6 +124,12 @@ module Eye::System
|
|
136
124
|
|
137
125
|
private
|
138
126
|
|
127
|
+
PS_AUX_CMD = if RUBY_PLATFORM.include?('darwin')
|
128
|
+
'ps axo pid,ppid,pcpu,rss,start'
|
129
|
+
else
|
130
|
+
'ps axo pid,ppid,pcpu,rss,start_time'
|
131
|
+
end
|
132
|
+
|
139
133
|
def spawn_options(config = {})
|
140
134
|
o = {}
|
141
135
|
o = {chdir: config[:working_dir]} if config[:working_dir]
|
data/lib/eye/system_resources.rb
CHANGED
@@ -24,10 +24,6 @@ class Eye::SystemResources
|
|
24
24
|
childs
|
25
25
|
end
|
26
26
|
|
27
|
-
def cmd(pid)
|
28
|
-
ps_aux[pid].try :[], :cmd
|
29
|
-
end
|
30
|
-
|
31
27
|
def start_time(pid)
|
32
28
|
ps_aux[pid].try :[], :start_time
|
33
29
|
end
|
@@ -38,7 +34,6 @@ class Eye::SystemResources
|
|
38
34
|
{ :memory => memory(pid),
|
39
35
|
:cpu => cpu(pid),
|
40
36
|
:start_time => start_time(pid),
|
41
|
-
:cmd => cmd(pid),
|
42
37
|
:pid => pid
|
43
38
|
}
|
44
39
|
end
|
@@ -49,6 +44,11 @@ class Eye::SystemResources
|
|
49
44
|
end
|
50
45
|
|
51
46
|
private
|
47
|
+
|
48
|
+
def reset!
|
49
|
+
setup.terminate
|
50
|
+
@actor = nil
|
51
|
+
end
|
52
52
|
|
53
53
|
def ps_aux
|
54
54
|
setup
|
@@ -67,7 +67,10 @@ class Eye::SystemResources
|
|
67
67
|
end
|
68
68
|
|
69
69
|
def get
|
70
|
-
|
70
|
+
if @at + UPDATE_INTERVAL < Time.now
|
71
|
+
@at = Time.now # for minimize races
|
72
|
+
set!
|
73
|
+
end
|
71
74
|
@ps_aux
|
72
75
|
end
|
73
76
|
|
@@ -38,6 +38,8 @@ describe "Eye::Controller" do
|
|
38
38
|
|
39
39
|
app1 = apps.first
|
40
40
|
app_check(app1, 'app1', 3)
|
41
|
+
app1.processes.map(&:name).sort.should == ["g4", "g5", "p1", "p2", "q3"]
|
42
|
+
|
41
43
|
app2 = apps.last
|
42
44
|
app_check(app2, 'app2', 1)
|
43
45
|
|
@@ -104,6 +106,20 @@ S
|
|
104
106
|
subject.info_string_short.split("\n").size.should == 2
|
105
107
|
end
|
106
108
|
|
109
|
+
it "history_string" do
|
110
|
+
subject.load(fixture("dsl/load.eye"))
|
111
|
+
str = subject.history_string('*')
|
112
|
+
str.should be_a(String)
|
113
|
+
str.size.should > 100
|
114
|
+
end
|
115
|
+
|
116
|
+
it "history_data" do
|
117
|
+
subject.load(fixture("dsl/load.eye"))
|
118
|
+
h = subject.history_data('app1')
|
119
|
+
h.size.should == 5
|
120
|
+
h.keys.sort.should == ["app1:g4", "app1:g5", "app1:gr1:p1", "app1:gr1:p2", "app1:gr2:q3"]
|
121
|
+
end
|
122
|
+
|
107
123
|
it "should delete all apps" do
|
108
124
|
subject.load(fixture("dsl/load.eye")).should include(error: false)
|
109
125
|
subject.send_command(:delete, 'all')
|
@@ -124,7 +124,7 @@ describe "Intergration" do
|
|
124
124
|
r2 = @p2.states_history.detect{|c| c[:state] == :restarting}[:at]
|
125
125
|
|
126
126
|
# >8 because, grace start, and grace stop added
|
127
|
-
(r2 - r1).should
|
127
|
+
(r2 - r1).should >= 8
|
128
128
|
end
|
129
129
|
|
130
130
|
it "restart group with chain async" do
|
@@ -127,6 +127,19 @@ describe "Process Restart" do
|
|
127
127
|
end
|
128
128
|
end
|
129
129
|
|
130
|
+
it "restart eye-daemonized lock-process from unmonitored status, and process realy running (WAS a problem)" do
|
131
|
+
start_ok_process(C.p4)
|
132
|
+
@pid = @process.pid
|
133
|
+
@process.unmonitor
|
134
|
+
Eye::System.pid_alive?(@pid).should == true
|
135
|
+
|
136
|
+
@process.restart
|
137
|
+
@process.state_name.should == :up
|
138
|
+
|
139
|
+
Eye::System.pid_alive?(@pid).should == false
|
140
|
+
@process.load_pid_from_file.should_not == @pid
|
141
|
+
end
|
142
|
+
|
130
143
|
[:down, :unmonitored, :up].each do |st|
|
131
144
|
it "ok restart from #{st}" do
|
132
145
|
start_ok_process(C.p1)
|
@@ -170,5 +183,4 @@ describe "Process Restart" do
|
|
170
183
|
@process.unmonitored?.should == true
|
171
184
|
end
|
172
185
|
|
173
|
-
|
174
186
|
end
|
@@ -154,6 +154,16 @@ describe "Scheduler" do
|
|
154
154
|
@process.last_scheduled_reason.should == 'reason'
|
155
155
|
@process.test3.should == [1, :bla, 3]
|
156
156
|
end
|
157
|
+
|
158
|
+
it "save history" do
|
159
|
+
@process.schedule :scheduler_test3, 1, :bla, 3, "reason"
|
160
|
+
sleep 0.1
|
161
|
+
h = @process.schedule_history
|
162
|
+
h.size.should == 1
|
163
|
+
h = h[0]
|
164
|
+
h[:state].should == :scheduler_test3
|
165
|
+
h[:reason].should == "reason"
|
166
|
+
end
|
157
167
|
end
|
158
168
|
|
159
169
|
describe "schedule_in" do
|
@@ -161,7 +171,7 @@ describe "Scheduler" do
|
|
161
171
|
@process.schedule_in(1.second, :scheduler_test3, 1, 2, 3)
|
162
172
|
sleep 0.5
|
163
173
|
@process.test3.should == nil
|
164
|
-
sleep 0.
|
174
|
+
sleep 0.7
|
165
175
|
@process.test3.should == [1,2,3]
|
166
176
|
end
|
167
177
|
end
|
data/spec/spec_helper.rb
CHANGED
@@ -1,36 +1,15 @@
|
|
1
1
|
require 'rr'
|
2
2
|
require 'rspec/core/mocking/with_rr'
|
3
3
|
|
4
|
-
module RR
|
5
|
-
|
6
|
-
|
7
|
-
|
8
|
-
|
9
|
-
|
10
|
-
|
11
|
-
|
12
|
-
def stub(subject=DoubleDefinitions::DoubleDefinitionCreate::NO_SUBJECT, method_name=nil, &definition_eval_block)
|
13
|
-
s = subject.respond_to?(:wrapped_object) ? subject.wrapped_object : subject
|
14
|
-
super(s, method_name, &definition_eval_block)
|
15
|
-
end
|
16
|
-
|
17
|
-
def dont_allow(subject=DoubleDefinitions::DoubleDefinitionCreate::NO_SUBJECT, method_name=nil, &definition_eval_block)
|
18
|
-
s = subject.respond_to?(:wrapped_object) ? subject.wrapped_object : subject
|
19
|
-
super(s, method_name, &definition_eval_block)
|
20
|
-
end
|
21
|
-
|
22
|
-
def proxy(subject=DoubleDefinitions::DoubleDefinitionCreate::NO_SUBJECT, method_name=nil, &definition_eval_block)
|
23
|
-
s = subject.respond_to?(:wrapped_object) ? subject.wrapped_object : subject
|
24
|
-
super(s, method_name, &definition_eval_block)
|
25
|
-
end
|
26
|
-
|
27
|
-
def strong(subject=DoubleDefinitions::DoubleDefinitionCreate::NO_SUBJECT, method_name=nil, &definition_eval_block)
|
28
|
-
s = subject.respond_to?(:wrapped_object) ? subject.wrapped_object : subject
|
29
|
-
super(s, method_name, &definition_eval_block)
|
30
|
-
end
|
31
|
-
|
4
|
+
module RR::CelluloidExt
|
5
|
+
%w{mock stub dont_allow proxy strong}.each do |_method|
|
6
|
+
module_eval <<-Q
|
7
|
+
def #{_method}(*args)
|
8
|
+
args[0] = args[0].wrapped_object if args[0].respond_to?(:wrapped_object)
|
9
|
+
super
|
10
|
+
end
|
11
|
+
Q
|
32
12
|
end
|
33
|
-
|
34
13
|
end
|
35
14
|
|
36
|
-
RSpec::Core::MockFrameworkAdapter.send(:include, RR::
|
15
|
+
RSpec::Core::MockFrameworkAdapter.send(:include, RR::CelluloidExt)
|
@@ -11,12 +11,12 @@ describe "Eye::SystemResources" do
|
|
11
11
|
it "should get cpu" do
|
12
12
|
x = Eye::SystemResources.cpu($$)
|
13
13
|
x.should >= 0
|
14
|
-
x.should
|
14
|
+
x.should <= 150
|
15
15
|
end
|
16
16
|
|
17
17
|
it "should get start time" do
|
18
18
|
x = Eye::SystemResources.start_time($$)
|
19
|
-
x.length.should >=
|
19
|
+
x.length.should >= 4
|
20
20
|
end
|
21
21
|
|
22
22
|
it "should get childs" do
|
@@ -27,31 +27,29 @@ describe "Eye::SystemResources" do
|
|
27
27
|
x.all?{|c| c > 0 }.should == true
|
28
28
|
end
|
29
29
|
|
30
|
-
it "should get cmd" do
|
31
|
-
Eye::Control.set_proc_line
|
32
|
-
x = Eye::SystemResources.cmd($$)
|
33
|
-
x.should match(/eye/)
|
34
|
-
end
|
35
|
-
|
36
30
|
it "should cache and update when interval" do
|
37
|
-
|
31
|
+
Eye::SystemResources.send :reset!
|
32
|
+
stub(Eye::System).ps_aux{ {$$ => {:rss => 123}} }
|
38
33
|
|
39
|
-
|
40
|
-
|
34
|
+
silence_warnings{ Eye::SystemResources::PsAxActor::UPDATE_INTERVAL = 1 }
|
35
|
+
|
36
|
+
x1 = Eye::SystemResources.memory($$)
|
37
|
+
x2 = Eye::SystemResources.memory($$)
|
41
38
|
x1.should == x2
|
42
39
|
|
43
|
-
sleep
|
44
|
-
x3 = Eye::SystemResources.
|
40
|
+
sleep 0.5
|
41
|
+
x3 = Eye::SystemResources.memory($$)
|
45
42
|
x1.should == x3
|
46
43
|
|
47
|
-
|
48
|
-
$0 = "ruby rspec ..."
|
44
|
+
stub(Eye::System).ps_aux{ {$$ => {:rss => 124}} }
|
49
45
|
|
50
|
-
sleep
|
51
|
-
x4 = Eye::SystemResources.
|
46
|
+
sleep 0.7
|
47
|
+
x4 = Eye::SystemResources.memory($$)
|
52
48
|
x1.should == x4 # first value is old
|
53
49
|
|
54
|
-
|
50
|
+
sleep 0.1
|
51
|
+
|
52
|
+
x5 = Eye::SystemResources.memory($$)
|
55
53
|
x1.should_not == x5 # seconds is new
|
56
54
|
end
|
57
55
|
|
data/spec/system_spec.rb
CHANGED
@@ -12,7 +12,7 @@ describe "Eye::System" do
|
|
12
12
|
it "check_pid_alive" do
|
13
13
|
Eye::System.check_pid_alive($$).should == {:result => true}
|
14
14
|
Eye::System.check_pid_alive(123456)[:error].class.should == Errno::ESRCH
|
15
|
-
Eye::System.check_pid_alive(-122)[:error].
|
15
|
+
Eye::System.check_pid_alive(-122)[:error].should be
|
16
16
|
Eye::System.check_pid_alive(nil).should == {:result => false}
|
17
17
|
end
|
18
18
|
|
@@ -25,7 +25,7 @@ describe "Eye::System" do
|
|
25
25
|
x[:ppid].should > 1 # parent pid
|
26
26
|
x[:cpu].should >= 0 # proc
|
27
27
|
x[:rss].should > 1000 # memory
|
28
|
-
x[:start_time].length.should >=
|
28
|
+
x[:start_time].length.should >= 4
|
29
29
|
end
|
30
30
|
|
31
31
|
it "prepare env" do
|
metadata
CHANGED
@@ -1,14 +1,14 @@
|
|
1
1
|
--- !ruby/object:Gem::Specification
|
2
2
|
name: eye
|
3
3
|
version: !ruby/object:Gem::Version
|
4
|
-
version: 0.2.
|
4
|
+
version: 0.2.4
|
5
5
|
platform: ruby
|
6
6
|
authors:
|
7
7
|
- Konstantin Makarchev
|
8
8
|
autorequire:
|
9
9
|
bindir: bin
|
10
10
|
cert_chain: []
|
11
|
-
date: 2013-
|
11
|
+
date: 2013-05-09 00:00:00.000000000 Z
|
12
12
|
dependencies:
|
13
13
|
- !ruby/object:Gem::Dependency
|
14
14
|
name: celluloid
|
@@ -245,6 +245,7 @@ extra_rdoc_files: []
|
|
245
245
|
files:
|
246
246
|
- .gitignore
|
247
247
|
- .rspec
|
248
|
+
- .travis.yml
|
248
249
|
- Gemfile
|
249
250
|
- LICENSE
|
250
251
|
- README.md
|
@@ -281,6 +282,7 @@ files:
|
|
281
282
|
- lib/eye/controller/helpers.rb
|
282
283
|
- lib/eye/controller/load.rb
|
283
284
|
- lib/eye/controller/send_command.rb
|
285
|
+
- lib/eye/controller/show_history.rb
|
284
286
|
- lib/eye/controller/status.rb
|
285
287
|
- lib/eye/dsl.rb
|
286
288
|
- lib/eye/dsl/application_opts.rb
|
@@ -440,7 +442,6 @@ files:
|
|
440
442
|
- spec/process/update_config_spec.rb
|
441
443
|
- spec/spec_helper.rb
|
442
444
|
- spec/support/rr_celluloid.rb
|
443
|
-
- spec/support/scheduler_hack.rb
|
444
445
|
- spec/support/spec_support.rb
|
445
446
|
- spec/system_resources_spec.rb
|
446
447
|
- spec/system_spec.rb
|
@@ -583,7 +584,6 @@ test_files:
|
|
583
584
|
- spec/process/update_config_spec.rb
|
584
585
|
- spec/spec_helper.rb
|
585
586
|
- spec/support/rr_celluloid.rb
|
586
|
-
- spec/support/scheduler_hack.rb
|
587
587
|
- spec/support/spec_support.rb
|
588
588
|
- spec/system_resources_spec.rb
|
589
589
|
- spec/system_spec.rb
|
@@ -1,16 +0,0 @@
|
|
1
|
-
module Eye::Process::SchedulerHack
|
2
|
-
|
3
|
-
def scheduled_action(command, *args, &block)
|
4
|
-
super
|
5
|
-
|
6
|
-
schedule_history << command
|
7
|
-
end
|
8
|
-
|
9
|
-
def schedule_history
|
10
|
-
@schedule_history ||= Eye::Process::StatesHistory.new(100)
|
11
|
-
end
|
12
|
-
|
13
|
-
end
|
14
|
-
|
15
|
-
Eye::Process.send :include, Eye::Process::SchedulerHack
|
16
|
-
Eye::Group.send :include, Eye::Process::SchedulerHack
|