doughboy 0.0.4 → 0.1.0
Sign up to get free protection for your applications and to get access to all the features.
- data/Gemfile.lock +1 -1
- data/README.markdown +13 -13
- data/lib/doughboy.rb +6 -2
- data/lib/doughboy/command.rb +25 -30
- data/lib/doughboy/version.rb +1 -1
- data/spec/doughboy/command_spec.rb +51 -70
- data/spec/doughboy_spec.rb +9 -3
- metadata +2 -2
data/Gemfile.lock
CHANGED
data/README.markdown
CHANGED
@@ -1,35 +1,35 @@
|
|
1
1
|
Doughboy
|
2
2
|
========
|
3
3
|
|
4
|
-
Doughboy is a Ruby library that strives to enable developers to
|
4
|
+
Doughboy is a Ruby library that strives to enable developers to simply make subprocessed shell calls.
|
5
5
|
|
6
6
|
Usage
|
7
7
|
--------
|
8
8
|
|
9
|
-
Doughboy provides a
|
9
|
+
Doughboy provides a composable interface to crafting shell commands. The following composition methods are available:
|
10
10
|
|
11
|
-
|
12
|
-
|
11
|
+
Doughboy.new("ruby -v")
|
12
|
+
Doughboy.new("python", "setup.py")
|
13
|
+
Doughboy.new(:executable => "python", :arguments => "setup.py")
|
13
14
|
|
14
|
-
|
15
|
+
Doing any of these things will return a command object that can be told to run:
|
15
16
|
|
16
|
-
command = Doughboy
|
17
|
+
command = Doughboy.new("ruby -v")
|
18
|
+
command.run #=> returns and Output object
|
17
19
|
|
18
|
-
The
|
20
|
+
The Output object includes the output methods: pid, stdin, stdout, stderr. For a shortcut, use the .text method. To create and run a new command, use the .with_exec shortcut:
|
21
|
+
|
22
|
+
output = Doughboy.with_exec("ruby -v") #=> returns an Output object
|
19
23
|
|
20
24
|
Command Components
|
21
25
|
------------------
|
22
26
|
|
23
|
-
Each command is composed of
|
27
|
+
Each command is composed of 2 components, executable and arguments.
|
24
28
|
|
25
29
|
### Executable
|
26
30
|
|
27
31
|
When this component is set, the full-path is captured. If no path information could be determined, the executable passed will be used.
|
28
32
|
|
29
|
-
### Options
|
30
|
-
|
31
|
-
Options are command line switches that either start w/ "-" or "--".
|
32
|
-
|
33
33
|
### Arguments
|
34
34
|
|
35
35
|
Arguments are items that are passed to the executable. They might be a list of ip addresses or files.
|
@@ -37,7 +37,7 @@ Arguments are items that are passed to the executable. They might be a list of i
|
|
37
37
|
Origin of the Name
|
38
38
|
------------------
|
39
39
|
|
40
|
-
I wish I could say that it was meant to pay homage to the fighting men of the Lost Generation, but it's not. I was watching Boyz N The Hood.
|
40
|
+
I wish I could say that it was meant to pay homage to the fighting men of the Lost Generation, but it's not. I was watching Boyz N The Hood. We got a problem here?
|
41
41
|
|
42
42
|
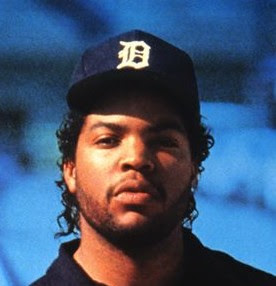
|
43
43
|
|
data/lib/doughboy.rb
CHANGED
data/lib/doughboy/command.rb
CHANGED
@@ -1,61 +1,56 @@
|
|
1
1
|
module Doughboy
|
2
2
|
class Command
|
3
|
-
attr_accessor :arguments, :executable
|
3
|
+
attr_accessor :arguments, :executable
|
4
|
+
|
5
|
+
# Command.new
|
6
|
+
# Command.new("python setup.py develop")
|
7
|
+
# Command.new("python", "setup.py develop")
|
8
|
+
# Command.new(:executable => "python", :arguments => "setup.py develop")
|
4
9
|
|
5
10
|
def initialize(*args)
|
6
|
-
if args.
|
7
|
-
|
11
|
+
return self if args.empty?
|
12
|
+
parsed_args = args.first.is_a?(Hash) ? args.first : parse_string_args(args)
|
8
13
|
|
9
|
-
|
10
|
-
|
11
|
-
end
|
12
|
-
end
|
14
|
+
self.executable = parsed_args[:executable]
|
15
|
+
self.arguments = parsed_args[:arguments]
|
13
16
|
end
|
14
17
|
|
15
|
-
def self.with_exec(
|
16
|
-
|
17
|
-
|
18
|
-
new( :executable => parsed_command[:executable],
|
19
|
-
:arguments => parsed_command[:arguments],
|
20
|
-
:options => parsed_command[:options],
|
21
|
-
:full_arguments => parsed_command[:full_arguments])
|
18
|
+
def self.with_exec(*args)
|
19
|
+
command = new(*args)
|
20
|
+
command.run
|
22
21
|
end
|
23
22
|
|
24
23
|
def command
|
25
|
-
|
26
|
-
[executable, full_arguments].join(" ")
|
27
|
-
else
|
28
|
-
[executable, options, arguments].join(" ")
|
29
|
-
end
|
24
|
+
[executable, arguments].join(" ")
|
30
25
|
end
|
31
26
|
|
32
27
|
def executable=(value)
|
33
28
|
return nil if value.nil?
|
29
|
+
|
34
30
|
full_path = `which #{value}`.strip
|
35
31
|
@executable = full_path != "" ? full_path : value
|
36
32
|
end
|
37
33
|
|
38
|
-
def
|
39
|
-
return nil if value.nil?
|
40
|
-
@options = value.is_a?(Array) ? value : value.split(" ")
|
41
|
-
end
|
42
|
-
|
43
|
-
def run!
|
34
|
+
def run
|
44
35
|
Output.new( Open4::popen4(command) )
|
45
36
|
end
|
46
37
|
|
47
38
|
private
|
48
|
-
def self.
|
39
|
+
def self.parse_executable(command)
|
49
40
|
split_command = command.split(" ")
|
50
41
|
parsed_command = { }
|
51
|
-
args_and_opts = split_command[1..(split_command.size-1)]
|
52
42
|
|
53
|
-
parsed_command[:full_arguments] = args_and_opts
|
54
43
|
parsed_command[:executable] = split_command[0]
|
55
|
-
parsed_command[:arguments]
|
56
|
-
parsed_command[:options] = args_and_opts.select { |t| t.include?("-") }
|
44
|
+
parsed_command[:arguments] = split_command[1..(split_command.size-1)]
|
57
45
|
parsed_command
|
58
46
|
end
|
59
47
|
|
48
|
+
def parse_string_args(args)
|
49
|
+
if args.size == 1
|
50
|
+
self.class.parse_executable(args.first)
|
51
|
+
else
|
52
|
+
{ :executable => args.first, :arguments => args[1]}
|
53
|
+
end
|
54
|
+
end
|
60
55
|
end
|
61
56
|
end
|
data/lib/doughboy/version.rb
CHANGED
@@ -3,30 +3,51 @@ require 'spec_helper'
|
|
3
3
|
module Doughboy
|
4
4
|
describe Command do
|
5
5
|
describe ".new" do
|
6
|
-
context "with
|
7
|
-
it "
|
6
|
+
context "with no arugments" do
|
7
|
+
it "does not error" do
|
8
|
+
lambda { Command.new }.should_not raise_exception
|
9
|
+
end
|
8
10
|
end
|
9
11
|
|
10
|
-
context "with a
|
12
|
+
context "with a single string argument" do
|
13
|
+
it "sets the executable" do
|
14
|
+
command = Command.new("python setup.py develop")
|
15
|
+
command.executable.should_not be_nil
|
16
|
+
end
|
17
|
+
|
11
18
|
it "sets the arguments" do
|
12
|
-
command = Command.new(
|
19
|
+
command = Command.new("python setup.py develop")
|
20
|
+
command.arguments.should_not be_nil
|
21
|
+
end
|
22
|
+
end
|
23
|
+
|
24
|
+
context "with a double string argument" do
|
25
|
+
it "sets the executable" do
|
26
|
+
command = Command.new("python", "setup.py develop")
|
27
|
+
command.executable.should_not be_nil
|
28
|
+
end
|
29
|
+
|
30
|
+
it "sets the arguments" do
|
31
|
+
command = Command.new("python", "setup.py develop")
|
13
32
|
command.arguments.should_not be_nil
|
14
33
|
end
|
15
34
|
|
35
|
+
end
|
36
|
+
|
37
|
+
context "with a hash argument" do
|
16
38
|
it "sets the executeable" do
|
17
39
|
command = Command.new(:executable => "python")
|
18
40
|
command.executable.should_not be_nil
|
19
41
|
end
|
20
42
|
|
21
|
-
it "sets the
|
22
|
-
|
23
|
-
command
|
24
|
-
command.options.should_not be_nil
|
43
|
+
it "sets the arguments" do
|
44
|
+
command = Command.new(:arguments => "setup.py develop")
|
45
|
+
command.arguments.should_not be_nil
|
25
46
|
end
|
26
47
|
|
27
48
|
# Command.with_exec("python setup.py develop).run
|
28
|
-
# Command.with_exec("python").
|
29
|
-
# Command.with_exec("mi").
|
49
|
+
# Command.with_exec("python").with_arg("123456").run
|
50
|
+
# Command.with_exec("mi").with_args([]).run
|
30
51
|
# Command.with_exec("python") do |input|
|
31
52
|
# input.puts("exit()")
|
32
53
|
# end
|
@@ -34,52 +55,11 @@ module Doughboy
|
|
34
55
|
end
|
35
56
|
|
36
57
|
describe ".with_exec" do
|
37
|
-
it "returns a Command" do
|
38
|
-
Command.with_exec("ruby").should be_kind_of(Command)
|
39
|
-
end
|
40
|
-
|
41
|
-
context "with a string of executable and argument" do
|
42
|
-
before(:each) do
|
43
|
-
@command_string = "ps aux"
|
44
|
-
end
|
45
|
-
|
46
|
-
it "assigns the executable" do
|
47
|
-
command = Command.with_exec(@command_string)
|
48
|
-
command.executable.should == `which ps`.strip
|
49
|
-
end
|
50
|
-
|
51
|
-
it "assigns the arguments" do
|
52
|
-
command = Command.with_exec(@command_string)
|
53
|
-
command.arguments.should == "aux"
|
54
|
-
end
|
55
|
-
end
|
56
|
-
|
57
|
-
context "with a string of executable, arguments, and options" do
|
58
|
-
before(:each) do
|
59
|
-
@command_string = "mi -r 212984"
|
60
|
-
end
|
61
58
|
|
62
|
-
|
63
|
-
|
64
|
-
command.executable.should == `which mi`.strip
|
65
|
-
end
|
66
|
-
|
67
|
-
it "assigns the arguments" do
|
68
|
-
command = Command.with_exec(@command_string)
|
69
|
-
command.arguments.should == "212984"
|
70
|
-
end
|
71
|
-
|
72
|
-
it "assigns the options" do
|
73
|
-
command = Command.with_exec(@command_string)
|
74
|
-
command.options.should == ["-r"]
|
75
|
-
end
|
59
|
+
it "returns a Output" do
|
60
|
+
Command.with_exec("ruby").should be_kind_of(Output)
|
76
61
|
end
|
77
62
|
|
78
|
-
context "with an executable" do
|
79
|
-
it "assigns the executable" do
|
80
|
-
Command.with_exec("ruby").executable.should include("ruby")
|
81
|
-
end
|
82
|
-
end
|
83
63
|
end
|
84
64
|
|
85
65
|
describe "#arguments=" do
|
@@ -92,15 +72,15 @@ module Doughboy
|
|
92
72
|
end
|
93
73
|
|
94
74
|
describe "#command" do
|
95
|
-
it "joins the executable
|
96
|
-
full_command = "/bin/ps
|
75
|
+
it "joins the executable and arguments" do
|
76
|
+
full_command = "/bin/ps aux"
|
97
77
|
command = Command.new(:executable => "ps", :options => "", :arguments => "aux")
|
98
78
|
command.command.should == full_command
|
99
79
|
end
|
100
80
|
|
101
81
|
it "returns them in the proper order" do
|
102
82
|
python = `which python`.strip
|
103
|
-
command = Command.
|
83
|
+
command = Command.new("python mbu_information.py -r 218298")
|
104
84
|
command.command.should == "#{python} mbu_information.py -r 218298"
|
105
85
|
end
|
106
86
|
end
|
@@ -119,19 +99,20 @@ module Doughboy
|
|
119
99
|
end
|
120
100
|
end
|
121
101
|
|
122
|
-
describe "#
|
123
|
-
|
124
|
-
|
125
|
-
|
126
|
-
|
127
|
-
|
128
|
-
|
102
|
+
describe "#run" do
|
103
|
+
it "runs the command" do
|
104
|
+
full_command = "/bin/ps aux"
|
105
|
+
command = Command.new()
|
106
|
+
command.stub!(:command).and_return(full_command)
|
107
|
+
|
108
|
+
Open4.should_receive(:popen4).with(full_command).
|
109
|
+
and_return([1234, StringIO.new, StringIO.new, StringIO.new])
|
110
|
+
command.run
|
129
111
|
end
|
130
112
|
|
131
|
-
|
132
|
-
|
133
|
-
command
|
134
|
-
command.options.should == ["-r", "-i"]
|
113
|
+
it "returns an output object" do
|
114
|
+
command = Command.new(:executable => "ps", :arguments => "aux")
|
115
|
+
command.run.should be_kind_of(Output)
|
135
116
|
end
|
136
117
|
end
|
137
118
|
|
@@ -143,12 +124,12 @@ module Doughboy
|
|
143
124
|
|
144
125
|
Open4.should_receive(:popen4).with(full_command).
|
145
126
|
and_return([1234, StringIO.new, StringIO.new, StringIO.new])
|
146
|
-
command.run
|
127
|
+
command.run
|
147
128
|
end
|
148
129
|
|
149
130
|
it "returns an output object" do
|
150
|
-
command = Command.new(:executable => "ps", :
|
151
|
-
command.run
|
131
|
+
command = Command.new(:executable => "ps", :arguments => "aux")
|
132
|
+
command.run.should be_kind_of(Output)
|
152
133
|
end
|
153
134
|
end
|
154
135
|
end
|
data/spec/doughboy_spec.rb
CHANGED
@@ -2,9 +2,15 @@ require 'spec_helper'
|
|
2
2
|
|
3
3
|
describe Doughboy do
|
4
4
|
|
5
|
-
describe "
|
6
|
-
it "returns a
|
7
|
-
Doughboy.
|
5
|
+
describe ".new" do
|
6
|
+
it "returns a Command" do
|
7
|
+
Doughboy.new("ruby -v").should be_kind_of(Doughboy::Command)
|
8
|
+
end
|
9
|
+
end
|
10
|
+
|
11
|
+
describe ".with_exec" do
|
12
|
+
it "returns returns the output from a command" do
|
13
|
+
Doughboy.with_exec("ruby -v").should be_kind_of(Doughboy::Output)
|
8
14
|
end
|
9
15
|
end
|
10
16
|
end
|