dns_mock 1.5.5 → 1.5.6
This diff represents the content of publicly available package versions that have been released to one of the supported registries. The information contained in this diff is provided for informational purposes only and reflects changes between package versions as they appear in their respective public registries.
- checksums.yaml +4 -4
- data/dns_mock.gemspec +3 -2
- data/lib/dns_mock/version.rb +1 -1
- metadata +4 -28
- data/.circleci/config.yml +0 -139
- data/.circleci/gemspec_compatible +0 -38
- data/.circleci/gemspec_latest +0 -48
- data/.codeclimate.yml +0 -13
- data/.github/BRANCH_NAMING_CONVENTION.md +0 -36
- data/.github/DEVELOPMENT_ENVIRONMENT_GUIDE.md +0 -26
- data/.github/FUNDING.yml +0 -1
- data/.github/ISSUE_TEMPLATE/bug_report.md +0 -28
- data/.github/ISSUE_TEMPLATE/feature_request.md +0 -27
- data/.github/ISSUE_TEMPLATE/issue_report.md +0 -28
- data/.github/ISSUE_TEMPLATE/question.md +0 -22
- data/.github/PULL_REQUEST_TEMPLATE.md +0 -49
- data/.gitignore +0 -11
- data/.overcommit.yml +0 -32
- data/.reek.yml +0 -65
- data/.rspec +0 -2
- data/.rubocop.yml +0 -123
- data/.ruby-gemset +0 -1
- data/CHANGELOG.md +0 -381
- data/CODE_OF_CONDUCT.md +0 -74
- data/CONTRIBUTING.md +0 -48
- data/Gemfile +0 -5
- data/README.md +0 -201
- data/Rakefile +0 -8
data/README.md
DELETED
@@ -1,201 +0,0 @@
|
|
1
|
-
# 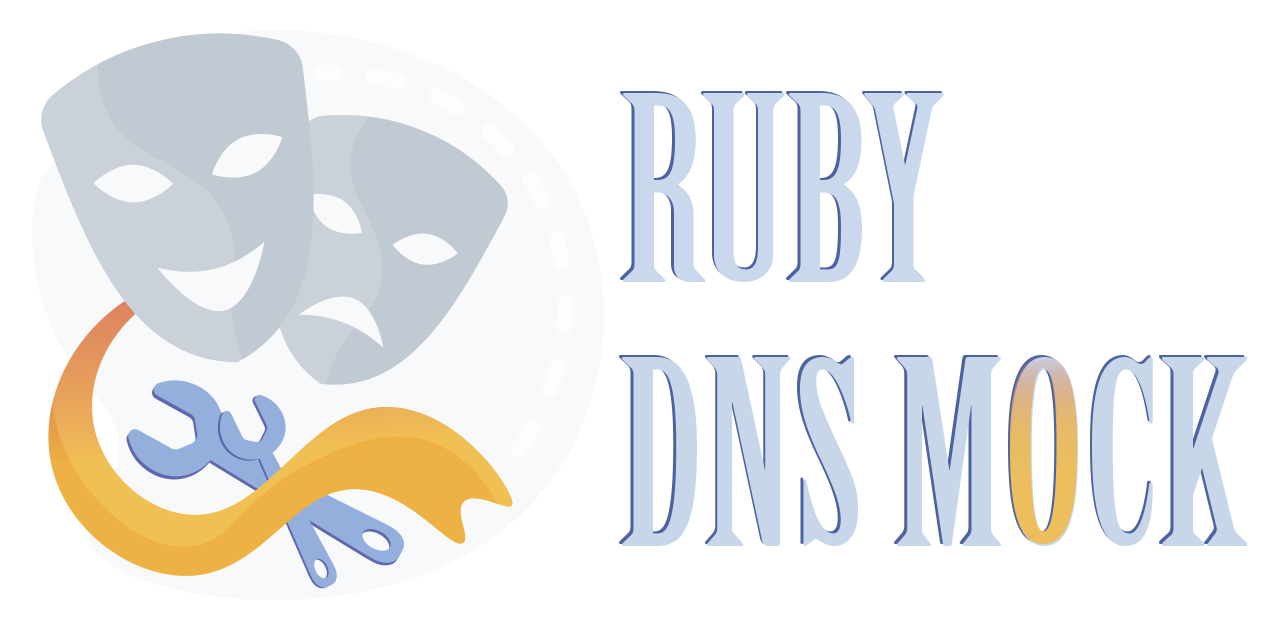
|
2
|
-
|
3
|
-
[](https://codeclimate.com/github/mocktools/ruby-dns-mock/maintainability)
|
4
|
-
[](https://codeclimate.com/github/mocktools/ruby-dns-mock/test_coverage)
|
5
|
-
[](https://circleci.com/gh/mocktools/ruby-dns-mock/tree/master)
|
6
|
-
[](https://badge.fury.io/rb/dns_mock)
|
7
|
-
[](https://rubygems.org/gems/dns_mock)
|
8
|
-
[](https://github.com/markets/awesome-ruby)
|
9
|
-
[](LICENSE.txt)
|
10
|
-
[](CODE_OF_CONDUCT.md)
|
11
|
-
|
12
|
-
💎 Ruby DNS mock. Mimic any DNS records for your test environment and even more.
|
13
|
-
|
14
|
-
## Table of Contents
|
15
|
-
|
16
|
-
- [Features](#features)
|
17
|
-
- [Requirements](#requirements)
|
18
|
-
- [Installation](#installation)
|
19
|
-
- [Usage](#usage)
|
20
|
-
- [RSpec](#rspec)
|
21
|
-
- [DnsMock RSpec helper](#dnsmock-rspec-helper)
|
22
|
-
- [DnsMock RSpec interface](#dnsmock-rspec-interface)
|
23
|
-
- [Contributing](#contributing)
|
24
|
-
- [License](#license)
|
25
|
-
- [Code of Conduct](#code-of-conduct)
|
26
|
-
- [Credits](#credits)
|
27
|
-
- [Versioning](#versioning)
|
28
|
-
- [Changelog](CHANGELOG.md)
|
29
|
-
|
30
|
-
## Features
|
31
|
-
|
32
|
-
- Ability to mimic any DNS records (`A`, `AAAA`, `CNAME`, `MX`, `NS`, `PTR`, `SOA` and `TXT`)
|
33
|
-
- Automatically converts hostnames to RDNS/[Punycode](https://en.wikipedia.org/wiki/Punycode) representation
|
34
|
-
- Lightweight UDP DNS mock server with dynamic/manual port assignment
|
35
|
-
- Test framework agnostic (it's PORO, so you can use it outside of `RSpec`, `Test::Unit` or `MiniTest`)
|
36
|
-
- Simple and intuitive DSL
|
37
|
-
- Only one runtime dependency
|
38
|
-
|
39
|
-
## Requirements
|
40
|
-
|
41
|
-
Ruby MRI 2.5.0+
|
42
|
-
|
43
|
-
## Installation
|
44
|
-
|
45
|
-
Add this line to your application's `Gemfile`:
|
46
|
-
|
47
|
-
```ruby
|
48
|
-
group :development, :test do
|
49
|
-
gem 'dns_mock', require: false
|
50
|
-
end
|
51
|
-
```
|
52
|
-
|
53
|
-
And then execute:
|
54
|
-
|
55
|
-
$ bundle
|
56
|
-
|
57
|
-
Or install it yourself as:
|
58
|
-
|
59
|
-
$ gem install dns_mock
|
60
|
-
|
61
|
-
## Usage
|
62
|
-
|
63
|
-
```ruby
|
64
|
-
# Example of mocked DNS records, please follow this data structure
|
65
|
-
records = {
|
66
|
-
'example.com' => {
|
67
|
-
a: %w[1.1.1.1 2.2.2.2],
|
68
|
-
aaaa: %w[2a00:1450:4001:81e::200e],
|
69
|
-
ns: %w[ns1.domain.com ns2.domain.com],
|
70
|
-
mx: %w[mx1.domain.com mx2.domain.com:50], # you can specify host(s) or host(s) with priority, use '.:0' for definition null MX record
|
71
|
-
txt: %w[txt_record_1 txt_record_2],
|
72
|
-
cname: 'mañana.com', # you can specify hostname in UTF-8. It will be converted to xn--maana-pta.com automatically
|
73
|
-
soa: [
|
74
|
-
{
|
75
|
-
mname: 'dns1.domain.com',
|
76
|
-
rname: 'dns2.domain.com',
|
77
|
-
serial: 2_035_971_683,
|
78
|
-
refresh: 10_000,
|
79
|
-
retry: 2_400,
|
80
|
-
expire: 604_800,
|
81
|
-
minimum: 3_600
|
82
|
-
}
|
83
|
-
]
|
84
|
-
},
|
85
|
-
'1.2.3.4' => { # You can define RDNS host address without lookup prefix. It will be converted to 4.3.2.1.in-addr.arpa automatically
|
86
|
-
ptr: %w[domain_1.com domain_2.com]
|
87
|
-
}
|
88
|
-
}
|
89
|
-
|
90
|
-
# Public DnsMock interface
|
91
|
-
# records:Hash, port:Integer, exception_if_not_found:Boolean
|
92
|
-
# are optional params. By default creates dns mock server with
|
93
|
-
# empty records. A free port for server will be randomly assigned
|
94
|
-
# in the range from 49152 to 65535, if record not found exception
|
95
|
-
# won't raises. Please note if you specify zero port number,
|
96
|
-
# free port number will be randomly assigned as a server port too.
|
97
|
-
# Returns current dns mock server
|
98
|
-
dns_mock_server = DnsMock.start_server(records: records) # => DnsMock::Server instance
|
99
|
-
|
100
|
-
# returns current dns mock server port
|
101
|
-
dns_mock_server.port # => 49322
|
102
|
-
|
103
|
-
# interface to setup mock records.
|
104
|
-
# Available only in case when server mocked records is empty
|
105
|
-
dns_mock_server.assign_mocks(records) # => true/nil
|
106
|
-
|
107
|
-
# interface to reset current mocked records
|
108
|
-
dns_mock_server.reset_mocks! # => true
|
109
|
-
|
110
|
-
# interface to stop current dns mock server
|
111
|
-
dns_mock_server.stop! # => true
|
112
|
-
|
113
|
-
# returns list of running dns mock servers
|
114
|
-
DnsMock.running_servers # => [DnsMock::Server instance]
|
115
|
-
|
116
|
-
# interface to stop all running dns mock servers
|
117
|
-
DnsMock.stop_running_servers! # => true
|
118
|
-
```
|
119
|
-
|
120
|
-
### RSpec
|
121
|
-
|
122
|
-
Require this either in your Gemfile or in RSpec's support scripts. So either:
|
123
|
-
|
124
|
-
```ruby
|
125
|
-
# Gemfile
|
126
|
-
group :test do
|
127
|
-
gem 'rspec'
|
128
|
-
gem 'dns_mock', require: 'dns_mock/test_framework/rspec'
|
129
|
-
end
|
130
|
-
```
|
131
|
-
|
132
|
-
or
|
133
|
-
|
134
|
-
```ruby
|
135
|
-
# spec/support/config/dns_mock.rb
|
136
|
-
require 'dns_mock/test_framework/rspec'
|
137
|
-
```
|
138
|
-
|
139
|
-
#### DnsMock RSpec helper
|
140
|
-
|
141
|
-
Just add `DnsMock::TestFramework::RSpec::Helper` if you wanna use shortcut `dns_mock_server` for DnsMock server instance inside of your `RSpec.describe` blocks:
|
142
|
-
|
143
|
-
```ruby
|
144
|
-
# spec/support/config/dns_mock.rb
|
145
|
-
RSpec.configure do |config|
|
146
|
-
config.include DnsMock::TestFramework::RSpec::Helper
|
147
|
-
end
|
148
|
-
```
|
149
|
-
|
150
|
-
```ruby
|
151
|
-
# your awesome first_a_record_spec.rb
|
152
|
-
RSpec.describe FirstARecord do
|
153
|
-
subject(:service) do
|
154
|
-
described_class.call(
|
155
|
-
hostname,
|
156
|
-
dns_gateway_host: 'localhost',
|
157
|
-
dns_gateway_port: dns_mock_server.port
|
158
|
-
)
|
159
|
-
end
|
160
|
-
|
161
|
-
let(:hostname) { 'example.com' }
|
162
|
-
let(:first_a_record) { '1.2.3.4' }
|
163
|
-
let(:records) { { hostname => { a: [first_a_record] } } }
|
164
|
-
|
165
|
-
before { dns_mock_server.assign_mocks(records) }
|
166
|
-
|
167
|
-
it { is_expected.to eq(first_a_record) }
|
168
|
-
end
|
169
|
-
```
|
170
|
-
|
171
|
-
#### DnsMock RSpec interface
|
172
|
-
|
173
|
-
If you won't use `DnsMock::TestFramework::RSpec::Helper` you can use `DnsMock::TestFramework::RSpec::Interface` directly instead:
|
174
|
-
|
175
|
-
```ruby
|
176
|
-
DnsMock::TestFramework::RSpec::Interface.start_server # creates and runs DnsMock server instance
|
177
|
-
DnsMock::TestFramework::RSpec::Interface.stop_server! # stops current DnsMock server instance
|
178
|
-
DnsMock::TestFramework::RSpec::Interface.reset_mocks! # resets mocks in current DnsMock server instance
|
179
|
-
DnsMock::TestFramework::RSpec::Interface.clear_server! # stops and clears current DnsMock server instance
|
180
|
-
```
|
181
|
-
|
182
|
-
## Contributing
|
183
|
-
|
184
|
-
Bug reports and pull requests are welcome on GitHub at https://github.com/mocktools/ruby-dns-mock. This project is intended to be a safe, welcoming space for collaboration, and contributors are expected to adhere to the [Contributor Covenant](http://contributor-covenant.org) code of conduct. Please check the [open tickets](https://github.com/mocktools/ruby-dns-mock/issues). Be sure to follow Contributor Code of Conduct below and our [Contributing Guidelines](CONTRIBUTING.md).
|
185
|
-
|
186
|
-
## License
|
187
|
-
|
188
|
-
The gem is available as open source under the terms of the [MIT License](https://opensource.org/licenses/MIT).
|
189
|
-
|
190
|
-
## Code of Conduct
|
191
|
-
|
192
|
-
Everyone interacting in the DnsMock project’s codebases, issue trackers, chat rooms and mailing lists is expected to follow the [code of conduct](CODE_OF_CONDUCT.md).
|
193
|
-
|
194
|
-
## Credits
|
195
|
-
|
196
|
-
- [The Contributors](https://github.com/mocktools/ruby-dns-mock/graphs/contributors) for code and awesome suggestions
|
197
|
-
- [The Stargazers](https://github.com/mocktools/ruby-dns-mock/stargazers) for showing their support
|
198
|
-
|
199
|
-
## Versioning
|
200
|
-
|
201
|
-
DnsMock uses [Semantic Versioning 2.0.0](https://semver.org)
|