digitalocean 1.0.6 → 1.1.0
Sign up to get free protection for your applications and to get access to all the features.
- data/README.md +7 -6
- data/digitalocean.gemspec +1 -1
- data/lib/digitalocean.rb +36 -33
- data/lib/digitalocean/version.rb +1 -1
- data/spec/digitalocean/domain_spec.rb +12 -31
- data/spec/digitalocean/droplet_spec.rb +40 -75
- data/spec/digitalocean/event_spec.rb +3 -8
- data/spec/digitalocean/image_spec.rb +11 -35
- data/spec/digitalocean/integration_spec.rb +4 -4
- data/spec/digitalocean/record_spec.rb +11 -32
- data/spec/digitalocean/region_spec.rb +5 -15
- data/spec/digitalocean/size_spec.rb +5 -16
- data/spec/digitalocean/ssh_key_spec.rb +24 -19
- data/spec/digitalocean_spec.rb +17 -18
- data/spec/spec_helper.rb +0 -6
- metadata +2 -2
data/README.md
CHANGED
@@ -1,11 +1,11 @@
|
|
1
|
-
# Digitalocean Rubygem
|
1
|
+
# Digitalocean Rubygem
|
2
2
|
|
3
3
|
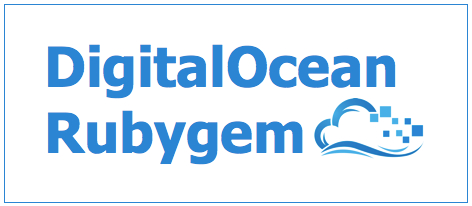
|
4
4
|
|
5
|
-
### The easiest and most complete rubygem for [DigitalOcean](https://www.digitalocean.com).
|
5
|
+
### The easiest and most complete rubygem for [DigitalOcean](https://www.digitalocean.com).
|
6
6
|
|
7
|
-
[](https://travis-ci.org/scottmotte/digitalocean)
|
8
|
+
[](http://badge.fury.io/rb/digitalocean)
|
9
9
|
|
10
10
|
```ruby
|
11
11
|
Digitalocean.client_id = "your_client_id"
|
@@ -13,7 +13,7 @@ Digitalocean.api_key = "your_api_key"
|
|
13
13
|
result = Digitalocean::Droplet.all
|
14
14
|
# =>
|
15
15
|
# <RecursiveOpenStruct status="OK", droplets=[
|
16
|
-
# {"id"=>12345, "name"=>"dev", "image_id"=>2676, "size_id"=>63, "region_id"=>3, "backups_active"=>false, "ip_address"=>"198.555.55.55", "private_ip_address"=>nil, "locked"=>false, "status"=>"active", "created_at"=>"2013-06-12T03:07:14Z"},
|
16
|
+
# {"id"=>12345, "name"=>"dev", "image_id"=>2676, "size_id"=>63, "region_id"=>3, "backups_active"=>false, "ip_address"=>"198.555.55.55", "private_ip_address"=>nil, "locked"=>false, "status"=>"active", "created_at"=>"2013-06-12T03:07:14Z"},
|
17
17
|
# {"id"=>234674, "name"=>"server2", "image_id"=>441012, "size_id"=>62, "region_id"=>1, "backups_active"=>false, "ip_address"=>"192.555.55.56", "private_ip_address"=>nil, "locked"=>false, "status"=>"active", "created_at"=>"2013-06-17T00:30:12Z"}
|
18
18
|
# ]>
|
19
19
|
#
|
@@ -92,6 +92,7 @@ Digitalocean::Droplet.power_on(id)
|
|
92
92
|
Digitalocean::Droplet.snapshot(id, {name: name})
|
93
93
|
Digitalocean::Droplet.create({name: name, size_id: size_id, image_id: image_id, region_id: region_id, ssh_key_ids: ssh_key_ids})
|
94
94
|
Digitalocean::Droplet.destroy(id)
|
95
|
+
Digitalocean::Droplet.resize(id, {size_id: size_id})
|
95
96
|
|
96
97
|
Digitalocean::Image.all
|
97
98
|
Digitalocean::Image.all({filter: "my_images"})
|
@@ -120,7 +121,7 @@ Digitalocean::Event.find(id)
|
|
120
121
|
|
121
122
|
## Example
|
122
123
|
|
123
|
-
There is a [digitalocean-rubygem-example](https://github.com/scottmotte/digitalocean-rubygem-example) to help jumpstart your development.
|
124
|
+
There is a [digitalocean-rubygem-example](https://github.com/scottmotte/digitalocean-rubygem-example) to help jumpstart your development.
|
124
125
|
|
125
126
|
## Contributing
|
126
127
|
|
data/digitalocean.gemspec
CHANGED
@@ -15,7 +15,7 @@ Gem::Specification.new do |gem|
|
|
15
15
|
gem.add_dependency "faraday", "~> 0.8.9"
|
16
16
|
gem.add_dependency "faraday_middleware", "~> 0.9.0"
|
17
17
|
gem.add_dependency "recursive-open-struct", "~> 0.4.5"
|
18
|
-
|
18
|
+
|
19
19
|
gem.add_development_dependency "foreman"
|
20
20
|
gem.add_development_dependency "pry"
|
21
21
|
gem.add_development_dependency "rake"
|
data/lib/digitalocean.rb
CHANGED
@@ -8,53 +8,56 @@ module Digitalocean
|
|
8
8
|
|
9
9
|
DEFINITIONS = {
|
10
10
|
"Domain" => {
|
11
|
-
"all" => "https://api.digitalocean.com/domains?client_id=[your_client_id]&api_key=[your_api_key]",
|
12
|
-
"find" => "https://api.digitalocean.com/domains/[domain_id]?client_id=[your_client_id]&api_key=[your_api_key]",
|
13
|
-
"create" => "https://api.digitalocean.com/domains/new?client_id=[your_client_id]&api_key=[your_api_key]&name=[domain]&ip_address=[ip_address]",
|
14
|
-
"destroy" => "https://api.digitalocean.com/domains/[domain_id]/destroy?client_id=[your_client_id]&api_key=[your_api_key]"
|
11
|
+
"all" => "https://api.digitalocean.com/v1/domains?client_id=[your_client_id]&api_key=[your_api_key]",
|
12
|
+
"find" => "https://api.digitalocean.com/v1/domains/[domain_id]?client_id=[your_client_id]&api_key=[your_api_key]",
|
13
|
+
"create" => "https://api.digitalocean.com/v1/domains/new?client_id=[your_client_id]&api_key=[your_api_key]&name=[domain]&ip_address=[ip_address]",
|
14
|
+
"destroy" => "https://api.digitalocean.com/v1/domains/[domain_id]/destroy?client_id=[your_client_id]&api_key=[your_api_key]"
|
15
15
|
},
|
16
16
|
"Droplet" => {
|
17
|
-
"all" => "https://api.digitalocean.com/droplets/?client_id=[your_client_id]&api_key=[your_api_key]",
|
18
|
-
"find" => "https://api.digitalocean.com/droplets/[droplet_id]?client_id=[your_client_id]&api_key=[your_api_key]",
|
19
|
-
"rename" => "https://api.digitalocean.com/droplets/[droplet_id]/rename/?client_id=[your_client_id]&api_key=[your_api_key]&name=[name]",
|
20
|
-
"
|
21
|
-
"
|
22
|
-
"
|
23
|
-
"
|
24
|
-
"
|
25
|
-
"
|
26
|
-
"
|
27
|
-
"
|
28
|
-
"
|
17
|
+
"all" => "https://api.digitalocean.com/v1/droplets/?client_id=[your_client_id]&api_key=[your_api_key]",
|
18
|
+
"find" => "https://api.digitalocean.com/v1/droplets/[droplet_id]?client_id=[your_client_id]&api_key=[your_api_key]",
|
19
|
+
"rename" => "https://api.digitalocean.com/v1/droplets/[droplet_id]/rename/?client_id=[your_client_id]&api_key=[your_api_key]&name=[name]",
|
20
|
+
"rebuild" => "https://api.digitalocean.com/v1/droplets/[droplet_id]/rebuild/?image_id=[image_id]&client_id=[client_id]&api_key=[api_key]",
|
21
|
+
"reboot" => "https://api.digitalocean.com/v1/droplets/[droplet_id]/reboot/?client_id=[your_client_id]&api_key=[your_api_key]",
|
22
|
+
"power_cycle" => "https://api.digitalocean.com/v1/droplets/[droplet_id]/power_cycle/?client_id=[your_client_id]&api_key=[your_api_key]",
|
23
|
+
"shutdown" => "https://api.digitalocean.com/v1/droplets/[droplet_id]/shutdown/?client_id=[your_client_id]&api_key=[your_api_key]",
|
24
|
+
"power_off" => "https://api.digitalocean.com/v1/droplets/[droplet_id]/power_off/?client_id=[your_client_id]&api_key=[your_api_key]",
|
25
|
+
"power_on" => "https://api.digitalocean.com/v1/droplets/[droplet_id]/power_on/?client_id=[your_client_id]&api_key=[your_api_key]",
|
26
|
+
"snapshot" => "https://api.digitalocean.com/v1/droplets/[droplet_id]/snapshot/?name=[snapshot_name]&client_id=[your_client_id]&api_key=[your_api_key]",
|
27
|
+
"create" => "https://api.digitalocean.com/v1/droplets/new?client_id=[your_client_id]&api_key=[your_api_key]&name=[droplet_name]&size_id=[size_id]&image_id=[image_id]®ion_id=[region_id]&ssh_key_ids=[ssh_key_ids]&private_networking=[private_networking]&backups_enabled=[backups_enabled]", # unique case that is not copy/paste
|
28
|
+
"destroy" => "https://api.digitalocean.com/v1/droplets/[droplet_id]/destroy/?client_id=[your_client_id]&api_key=[your_api_key]",
|
29
|
+
"resize" => "https://api.digitalocean.com/v1/droplets/[droplet_id]/resize/?size_id=[size_id]&client_id=[client_id]&api_key=[api_key]"
|
29
30
|
},
|
30
31
|
"Image" => {
|
31
|
-
"all" => "https://api.digitalocean.com/images/?client_id=[your_client_id]&api_key=[your_api_key]",
|
32
|
-
"find" => "https://api.digitalocean.com/images/[image_id]/?client_id=[your_client_id]&api_key=[your_api_key]",
|
33
|
-
"destroy" => "https://api.digitalocean.com/images/[image_id]/destroy/?client_id=[your_client_id]&api_key=[your_api_key]",
|
34
|
-
"transfer" => "https://api.digitalocean.com/images/[image_id]/transfer/?client_id=[your_client_id]&api_key=[your_api_key]®ion_id=[region_id]"
|
32
|
+
"all" => "https://api.digitalocean.com/v1/images/?client_id=[your_client_id]&api_key=[your_api_key]",
|
33
|
+
"find" => "https://api.digitalocean.com/v1/images/[image_id]/?client_id=[your_client_id]&api_key=[your_api_key]",
|
34
|
+
"destroy" => "https://api.digitalocean.com/v1/images/[image_id]/destroy/?client_id=[your_client_id]&api_key=[your_api_key]",
|
35
|
+
"transfer" => "https://api.digitalocean.com/v1/images/[image_id]/transfer/?client_id=[your_client_id]&api_key=[your_api_key]®ion_id=[region_id]"
|
35
36
|
},
|
36
37
|
"Record" => {
|
37
|
-
"all" => "https://api.digitalocean.com/domains/[domain_id]/records?client_id=[your_client_id]&api_key=[your_api_key]",
|
38
|
-
"find" => "https://api.digitalocean.com/domains/[domain_id]/records/[record_id]?client_id=[your_client_id]&api_key=[your_api_key]",
|
39
|
-
"create" => "https://api.digitalocean.com/domains/[domain_id]/records/new?client_id=[your_client_id]&api_key=[your_api_key]&record_type=[record_type]&data=[data]",
|
40
|
-
"edit" => "https://api.digitalocean.com/domains/[domain_id]/records/[record_id]/edit?client_id=[your_client_id]&api_key=[your_api_key]",
|
41
|
-
"destroy" => "https://api.digitalocean.com/domains/[domain_id]/records/[record_id]/destroy?client_id=[your_client_id]&api_key=[your_api_key]"
|
38
|
+
"all" => "https://api.digitalocean.com/v1/domains/[domain_id]/records?client_id=[your_client_id]&api_key=[your_api_key]",
|
39
|
+
"find" => "https://api.digitalocean.com/v1/domains/[domain_id]/records/[record_id]?client_id=[your_client_id]&api_key=[your_api_key]",
|
40
|
+
"create" => "https://api.digitalocean.com/v1/domains/[domain_id]/records/new?client_id=[your_client_id]&api_key=[your_api_key]&record_type=[record_type]&data=[data]",
|
41
|
+
"edit" => "https://api.digitalocean.com/v1/domains/[domain_id]/records/[record_id]/edit?client_id=[your_client_id]&api_key=[your_api_key]",
|
42
|
+
"destroy" => "https://api.digitalocean.com/v1/domains/[domain_id]/records/[record_id]/destroy?client_id=[your_client_id]&api_key=[your_api_key]"
|
42
43
|
},
|
43
44
|
"Region" => {
|
44
|
-
"all" => "https://api.digitalocean.com/regions/?client_id=[your_client_id]&api_key=[your_api_key]",
|
45
|
-
"find" => "https://api.digitalocean.com/regions/[region_id]?client_id=[your_client_id]&api_key=[your_api_key]"
|
45
|
+
"all" => "https://api.digitalocean.com/v1/regions/?client_id=[your_client_id]&api_key=[your_api_key]",
|
46
|
+
"find" => "https://api.digitalocean.com/v1/regions/[region_id]?client_id=[your_client_id]&api_key=[your_api_key]"
|
46
47
|
},
|
47
48
|
"Size" => {
|
48
|
-
"all" => "https://api.digitalocean.com/sizes/?client_id=[your_client_id]&api_key=[your_api_key]",
|
49
|
-
"find" => "https://api.digitalocean.com/sizes/[size_id]?client_id=[your_client_id]&api_key=[your_api_key]"
|
49
|
+
"all" => "https://api.digitalocean.com/v1/sizes/?client_id=[your_client_id]&api_key=[your_api_key]",
|
50
|
+
"find" => "https://api.digitalocean.com/v1/sizes/[size_id]?client_id=[your_client_id]&api_key=[your_api_key]"
|
50
51
|
},
|
51
52
|
"SshKey" => {
|
52
|
-
"all" => "https://api.digitalocean.com/ssh_keys/?client_id=[your_client_id]&api_key=[your_api_key]",
|
53
|
-
"find" => "https://api.digitalocean.com/ssh_keys/[ssh_key_id]/?client_id=[your_client_id]&api_key=[your_api_key]",
|
54
|
-
"
|
53
|
+
"all" => "https://api.digitalocean.com/v1/ssh_keys/?client_id=[your_client_id]&api_key=[your_api_key]",
|
54
|
+
"find" => "https://api.digitalocean.com/v1/ssh_keys/[ssh_key_id]/?client_id=[your_client_id]&api_key=[your_api_key]",
|
55
|
+
"edit" => "https://api.digitalocean.com/v1/ssh_keys/[ssh_key_id]/edit/?name=[ssh_key_name]&ssh_pub_key=[ssh_public_key]&client_id=[client_id]&api_key=[api_key]",
|
56
|
+
"create" => "https://api.digitalocean.com/v1/ssh_keys/new/?name=[ssh_key_name]&ssh_pub_key=[ssh_public_key]&client_id=[your_client_id]&api_key=[your_api_key]",
|
57
|
+
"destroy" => "https://api.digitalocean.com/v1/ssh_keys/[ssh_key_id]/destroy/?client_id=[your_client_id]&api_key=[your_api_key]"
|
55
58
|
},
|
56
59
|
"Event" => {
|
57
|
-
"find" => "https://api.digitalocean.com/events/[event_id]/?client_id=[your_client_id]&api_key=[your_api_key]"
|
60
|
+
"find" => "https://api.digitalocean.com/v1/events/[event_id]/?client_id=[your_client_id]&api_key=[your_api_key]"
|
58
61
|
}
|
59
62
|
}
|
60
63
|
|
data/lib/digitalocean/version.rb
CHANGED
@@ -1,53 +1,34 @@
|
|
1
1
|
require 'spec_helper'
|
2
2
|
|
3
3
|
describe Digitalocean::Domain do
|
4
|
-
|
4
|
+
subject(:domain) { described_class }
|
5
5
|
|
6
6
|
describe "._all" do
|
7
|
-
|
8
|
-
|
9
|
-
end
|
10
|
-
|
11
|
-
it do
|
12
|
-
@url.should eq "https://api.digitalocean.com/domains?client_id=client_id_required&api_key=api_key_required"
|
13
|
-
end
|
7
|
+
let(:url) { domain._all }
|
8
|
+
it { url.should eq "https://api.digitalocean.com/v1/domains?client_id=client_id_required&api_key=api_key_required" }
|
14
9
|
end
|
15
10
|
|
16
11
|
describe "._find" do
|
17
12
|
let(:domain_id) { "1234" }
|
13
|
+
let(:url) { domain._find(domain_id) }
|
18
14
|
|
19
|
-
|
20
|
-
@url = subject._find(domain_id)
|
21
|
-
end
|
22
|
-
|
23
|
-
it do
|
24
|
-
@url.should eq "https://api.digitalocean.com/domains/#{domain_id}?client_id=client_id_required&api_key=api_key_required"
|
25
|
-
end
|
15
|
+
it { url.should eq "https://api.digitalocean.com/v1/domains/#{domain_id}?client_id=client_id_required&api_key=api_key_required" }
|
26
16
|
end
|
27
17
|
|
28
18
|
describe "._create" do
|
29
|
-
let(:name)
|
30
|
-
let(:ip_address)
|
31
|
-
let(:args)
|
19
|
+
let(:name) { "test_domain" }
|
20
|
+
let(:ip_address) { "test_ip_address" }
|
21
|
+
let(:args) { { name: name, ip_address: ip_address } }
|
32
22
|
|
33
|
-
|
34
|
-
@url = subject._create(args)
|
35
|
-
end
|
23
|
+
let(:url) { domain._create(args) }
|
36
24
|
|
37
|
-
it
|
38
|
-
@url.should eq "https://api.digitalocean.com/domains/new?client_id=client_id_required&api_key=api_key_required&name=test_domain&ip_address=test_ip_address"
|
39
|
-
end
|
25
|
+
it { url.should eq "https://api.digitalocean.com/v1/domains/new?client_id=client_id_required&api_key=api_key_required&name=test_domain&ip_address=test_ip_address" }
|
40
26
|
end
|
41
27
|
|
42
28
|
describe "._destroy" do
|
43
29
|
let(:domain_id) { "test_domain_id" }
|
30
|
+
let(:url) { domain._destroy(domain_id) }
|
44
31
|
|
45
|
-
|
46
|
-
@url = subject._destroy(domain_id)
|
47
|
-
end
|
48
|
-
|
49
|
-
it do
|
50
|
-
@url.should eq "https://api.digitalocean.com/domains/test_domain_id/destroy?client_id=client_id_required&api_key=api_key_required"
|
51
|
-
end
|
32
|
+
it { url.should eq "https://api.digitalocean.com/v1/domains/test_domain_id/destroy?client_id=client_id_required&api_key=api_key_required" }
|
52
33
|
end
|
53
34
|
end
|
@@ -1,114 +1,79 @@
|
|
1
1
|
require 'spec_helper'
|
2
2
|
|
3
3
|
describe Digitalocean::Droplet do
|
4
|
-
|
4
|
+
subject(:droplet) { described_class }
|
5
5
|
|
6
6
|
describe "._all" do
|
7
|
-
|
8
|
-
|
9
|
-
end
|
10
|
-
|
11
|
-
it do
|
12
|
-
@url.should eq "https://api.digitalocean.com/droplets/?client_id=client_id_required&api_key=api_key_required"
|
13
|
-
end
|
7
|
+
let(:url) { droplet._all }
|
8
|
+
it { url.should eq "https://api.digitalocean.com/v1/droplets/?client_id=client_id_required&api_key=api_key_required" }
|
14
9
|
end
|
15
10
|
|
16
11
|
describe "._find" do
|
17
12
|
let(:droplet_id) { "1234" }
|
13
|
+
let(:url) { droplet._find(droplet_id) }
|
18
14
|
|
19
|
-
|
20
|
-
@url = subject._find(droplet_id)
|
21
|
-
end
|
22
|
-
|
23
|
-
it do
|
24
|
-
@url.should eq "https://api.digitalocean.com/droplets/#{droplet_id}?client_id=client_id_required&api_key=api_key_required"
|
25
|
-
end
|
15
|
+
it { url.should eq "https://api.digitalocean.com/v1/droplets/#{droplet_id}?client_id=client_id_required&api_key=api_key_required" }
|
26
16
|
end
|
27
17
|
|
28
18
|
describe "._rename" do
|
29
19
|
let(:droplet_id) { "1234" }
|
30
20
|
let(:name) { "new_name" }
|
21
|
+
let(:url) { droplet._rename(droplet_id, {name: name}) }
|
31
22
|
|
32
|
-
|
33
|
-
|
34
|
-
end
|
23
|
+
it { url.should eq "https://api.digitalocean.com/v1/droplets/#{droplet_id}/rename/?client_id=client_id_required&api_key=api_key_required&name=#{name}" }
|
24
|
+
end
|
35
25
|
|
36
|
-
|
37
|
-
|
38
|
-
|
26
|
+
describe "._rebuild" do
|
27
|
+
let(:droplet_id) { "1234" }
|
28
|
+
let(:image_id) { "11" }
|
29
|
+
|
30
|
+
let(:url) { droplet._rebuild(droplet_id, {image_id: image_id}) }
|
31
|
+
|
32
|
+
it { url.should eq "https://api.digitalocean.com/v1/droplets/#{droplet_id}/rebuild/?image_id=#{image_id}&client_id=client_id_required&api_key=api_key_required" }
|
39
33
|
end
|
40
|
-
|
34
|
+
|
41
35
|
describe "._reboot" do
|
42
36
|
let(:droplet_id) { "1234" }
|
37
|
+
let(:url) { droplet._reboot(droplet_id) }
|
43
38
|
|
44
|
-
|
45
|
-
@url = subject._reboot(droplet_id)
|
46
|
-
end
|
47
|
-
|
48
|
-
it do
|
49
|
-
@url.should eq "https://api.digitalocean.com/droplets/#{droplet_id}/reboot/?client_id=client_id_required&api_key=api_key_required"
|
50
|
-
end
|
39
|
+
it { url.should eq "https://api.digitalocean.com/v1/droplets/#{droplet_id}/reboot/?client_id=client_id_required&api_key=api_key_required" }
|
51
40
|
end
|
52
41
|
|
53
42
|
describe "._power_cycle" do
|
54
43
|
let(:droplet_id) { "1234" }
|
44
|
+
let(:url) { droplet._power_cycle(droplet_id) }
|
55
45
|
|
56
|
-
|
57
|
-
@url = subject._power_cycle(droplet_id)
|
58
|
-
end
|
59
|
-
|
60
|
-
it do
|
61
|
-
@url.should eq "https://api.digitalocean.com/droplets/#{droplet_id}/power_cycle/?client_id=client_id_required&api_key=api_key_required"
|
62
|
-
end
|
46
|
+
it { url.should eq "https://api.digitalocean.com/v1/droplets/#{droplet_id}/power_cycle/?client_id=client_id_required&api_key=api_key_required" }
|
63
47
|
end
|
64
48
|
|
65
49
|
describe "._shutdown" do
|
66
50
|
let(:droplet_id) { "1234" }
|
51
|
+
let(:url) { droplet._shutdown(droplet_id) }
|
67
52
|
|
68
|
-
|
69
|
-
@url = subject._shutdown(droplet_id)
|
70
|
-
end
|
71
|
-
|
72
|
-
it do
|
73
|
-
@url.should eq "https://api.digitalocean.com/droplets/#{droplet_id}/shutdown/?client_id=client_id_required&api_key=api_key_required"
|
74
|
-
end
|
53
|
+
it { url.should eq "https://api.digitalocean.com/v1/droplets/#{droplet_id}/shutdown/?client_id=client_id_required&api_key=api_key_required" }
|
75
54
|
end
|
76
55
|
|
77
56
|
describe "._power_off" do
|
78
57
|
let(:droplet_id) { "1234" }
|
58
|
+
let(:url) { droplet._power_off(droplet_id) }
|
79
59
|
|
80
|
-
|
81
|
-
@url = subject._power_off(droplet_id)
|
82
|
-
end
|
83
|
-
|
84
|
-
it do
|
85
|
-
@url.should eq "https://api.digitalocean.com/droplets/#{droplet_id}/power_off/?client_id=client_id_required&api_key=api_key_required"
|
86
|
-
end
|
60
|
+
it { url.should eq "https://api.digitalocean.com/v1/droplets/#{droplet_id}/power_off/?client_id=client_id_required&api_key=api_key_required" }
|
87
61
|
end
|
88
62
|
|
89
63
|
describe "._power_on" do
|
90
64
|
let(:droplet_id) { "1234" }
|
65
|
+
let(:url) { droplet._power_on(droplet_id) }
|
91
66
|
|
92
|
-
|
93
|
-
@url = subject._power_on(droplet_id)
|
94
|
-
end
|
95
|
-
|
96
|
-
it do
|
97
|
-
@url.should eq "https://api.digitalocean.com/droplets/#{droplet_id}/power_on/?client_id=client_id_required&api_key=api_key_required"
|
98
|
-
end
|
67
|
+
it { url.should eq "https://api.digitalocean.com/v1/droplets/#{droplet_id}/power_on/?client_id=client_id_required&api_key=api_key_required" }
|
99
68
|
end
|
100
69
|
|
101
70
|
describe "._snapshot" do
|
102
71
|
let(:droplet_id) { "1234" }
|
103
72
|
let(:snapshot_name) { "test_name" }
|
104
73
|
|
105
|
-
|
106
|
-
@url = subject._snapshot(droplet_id, {name: snapshot_name})
|
107
|
-
end
|
74
|
+
let(:url) { droplet._snapshot(droplet_id, {name: snapshot_name}) }
|
108
75
|
|
109
|
-
it
|
110
|
-
@url.should eq "https://api.digitalocean.com/droplets/#{droplet_id}/snapshot/?name=#{snapshot_name}&client_id=client_id_required&api_key=api_key_required"
|
111
|
-
end
|
76
|
+
it { url.should eq "https://api.digitalocean.com/v1/droplets/#{droplet_id}/snapshot/?name=#{snapshot_name}&client_id=client_id_required&api_key=api_key_required" }
|
112
77
|
end
|
113
78
|
|
114
79
|
describe "._create" do
|
@@ -118,24 +83,24 @@ describe Digitalocean::Droplet do
|
|
118
83
|
let(:region_id) { "44" }
|
119
84
|
let(:ssh_key_ids) { "12,92" }
|
120
85
|
|
121
|
-
|
122
|
-
@url = subject._create({name: name, size_id: size_id, image_id: image_id, region_id: region_id, ssh_key_ids: ssh_key_ids})
|
123
|
-
end
|
86
|
+
let(:url) { droplet._create({name: name, size_id: size_id, image_id: image_id, region_id: region_id, ssh_key_ids: ssh_key_ids}) }
|
124
87
|
|
125
|
-
it
|
126
|
-
@url.should eq "https://api.digitalocean.com/droplets/new?client_id=client_id_required&api_key=api_key_required&name=#{name}&size_id=#{size_id}&image_id=#{image_id}®ion_id=#{region_id}&ssh_key_ids=#{ssh_key_ids}&private_networking=private_networking&backups_enabled=backups_enabled"
|
127
|
-
end
|
88
|
+
it { url.should eq "https://api.digitalocean.com/v1/droplets/new?client_id=client_id_required&api_key=api_key_required&name=#{name}&size_id=#{size_id}&image_id=#{image_id}®ion_id=#{region_id}&ssh_key_ids=#{ssh_key_ids}&private_networking=private_networking&backups_enabled=backups_enabled" }
|
128
89
|
end
|
129
90
|
|
130
91
|
describe "._destroy" do
|
131
92
|
let(:droplet_id) { "1234" }
|
93
|
+
let(:url) { droplet._destroy(droplet_id) }
|
94
|
+
|
95
|
+
it { url.should eq "https://api.digitalocean.com/v1/droplets/#{droplet_id}/destroy/?client_id=client_id_required&api_key=api_key_required" }
|
96
|
+
end
|
97
|
+
|
98
|
+
describe "._resize" do
|
99
|
+
let(:droplet_id) { "1234" }
|
100
|
+
let(:size_id) { "45" }
|
132
101
|
|
133
|
-
|
134
|
-
@url = subject._destroy(droplet_id)
|
135
|
-
end
|
102
|
+
let(:url) { droplet._resize(droplet_id, {size_id: size_id}) }
|
136
103
|
|
137
|
-
it
|
138
|
-
@url.should eq "https://api.digitalocean.com/droplets/#{droplet_id}/destroy/?client_id=client_id_required&api_key=api_key_required"
|
139
|
-
end
|
104
|
+
it { url.should eq "https://api.digitalocean.com/v1/droplets/#{droplet_id}/resize/?size_id=#{size_id}&client_id=client_id_required&api_key=api_key_required" }
|
140
105
|
end
|
141
106
|
end
|
@@ -1,17 +1,12 @@
|
|
1
1
|
require 'spec_helper'
|
2
2
|
|
3
3
|
describe Digitalocean::Event do
|
4
|
-
|
4
|
+
subject(:event) { described_class }
|
5
5
|
|
6
6
|
describe "._find" do
|
7
7
|
let(:event_id) { "1234" }
|
8
|
+
let(:url) { event._find(event_id) }
|
8
9
|
|
9
|
-
|
10
|
-
@url = subject._find(event_id)
|
11
|
-
end
|
12
|
-
|
13
|
-
it do
|
14
|
-
@url.should eq "https://api.digitalocean.com/events/#{event_id}/?client_id=client_id_required&api_key=api_key_required"
|
15
|
-
end
|
10
|
+
it { url.should eq "https://api.digitalocean.com/v1/events/#{event_id}/?client_id=client_id_required&api_key=api_key_required" }
|
16
11
|
end
|
17
12
|
end
|
@@ -1,52 +1,32 @@
|
|
1
1
|
require 'spec_helper'
|
2
2
|
|
3
3
|
describe Digitalocean::Image do
|
4
|
-
|
4
|
+
subject(:image) { described_class }
|
5
5
|
|
6
6
|
describe "._all" do
|
7
|
-
|
8
|
-
|
9
|
-
end
|
10
|
-
|
11
|
-
it do
|
12
|
-
@url.should eq "https://api.digitalocean.com/images/?client_id=client_id_required&api_key=api_key_required"
|
13
|
-
end
|
7
|
+
let(:url) { image._all }
|
8
|
+
it { url.should eq "https://api.digitalocean.com/v1/images/?client_id=client_id_required&api_key=api_key_required" }
|
14
9
|
end
|
15
10
|
|
16
11
|
describe "._all with optional parameters" do
|
17
12
|
let(:args) { { filter: "my_images" } }
|
13
|
+
let(:url) { image._all(args) }
|
18
14
|
|
19
|
-
|
20
|
-
@url = subject._all(args)
|
21
|
-
end
|
22
|
-
|
23
|
-
it do
|
24
|
-
@url.should eq "https://api.digitalocean.com/images/?client_id=client_id_required&api_key=api_key_required&filter=my_images"
|
25
|
-
end
|
15
|
+
it { url.should eq "https://api.digitalocean.com/v1/images/?client_id=client_id_required&api_key=api_key_required&filter=my_images" }
|
26
16
|
end
|
27
17
|
|
28
18
|
describe "._find" do
|
29
19
|
let(:id) { "1234" }
|
20
|
+
let(:url) { image._find(id) }
|
30
21
|
|
31
|
-
|
32
|
-
@url = subject._find(id)
|
33
|
-
end
|
34
|
-
|
35
|
-
it do
|
36
|
-
@url.should eq "https://api.digitalocean.com/images/#{id}/?client_id=client_id_required&api_key=api_key_required"
|
37
|
-
end
|
22
|
+
it { url.should eq "https://api.digitalocean.com/v1/images/#{id}/?client_id=client_id_required&api_key=api_key_required" }
|
38
23
|
end
|
39
24
|
|
40
25
|
describe "._destroy" do
|
41
26
|
let(:id) { "1234" }
|
27
|
+
let(:url) { image._destroy(id) }
|
42
28
|
|
43
|
-
|
44
|
-
@url = subject._destroy(id)
|
45
|
-
end
|
46
|
-
|
47
|
-
it do
|
48
|
-
@url.should eq "https://api.digitalocean.com/images/#{id}/destroy/?client_id=client_id_required&api_key=api_key_required"
|
49
|
-
end
|
29
|
+
it { url.should eq "https://api.digitalocean.com/v1/images/#{id}/destroy/?client_id=client_id_required&api_key=api_key_required" }
|
50
30
|
end
|
51
31
|
|
52
32
|
describe "._transfer" do
|
@@ -54,12 +34,8 @@ describe Digitalocean::Image do
|
|
54
34
|
let(:region_id) { "44" }
|
55
35
|
let(:args) { {region_id: region_id } }
|
56
36
|
|
57
|
-
|
58
|
-
@url = subject._transfer(id, args)
|
59
|
-
end
|
37
|
+
let(:url) { image._transfer(id, args) }
|
60
38
|
|
61
|
-
it
|
62
|
-
@url.should eq "https://api.digitalocean.com/images/#{id}/transfer/?client_id=client_id_required&api_key=api_key_required®ion_id=44"
|
63
|
-
end
|
39
|
+
it { url.should eq "https://api.digitalocean.com/v1/images/#{id}/transfer/?client_id=client_id_required&api_key=api_key_required®ion_id=44" }
|
64
40
|
end
|
65
41
|
end
|
@@ -1,12 +1,12 @@
|
|
1
1
|
require 'spec_helper'
|
2
2
|
|
3
3
|
describe "integrations" do
|
4
|
-
|
5
|
-
|
6
|
-
|
4
|
+
subject(:region) { Digitalocean::Region }
|
5
|
+
|
6
|
+
before { Digitalocean.send(:setup_request!) }
|
7
7
|
|
8
8
|
it "makes a real call" do
|
9
|
-
regions =
|
9
|
+
regions = region.all
|
10
10
|
regions.status.should eq "ERROR"
|
11
11
|
end
|
12
12
|
end
|
@@ -1,31 +1,22 @@
|
|
1
1
|
require 'spec_helper'
|
2
2
|
|
3
3
|
describe Digitalocean::Record do
|
4
|
-
|
4
|
+
subject(:record) { described_class }
|
5
5
|
|
6
6
|
describe "._all" do
|
7
7
|
let(:domain_id) { "100" }
|
8
|
+
let(:url) { record._all(domain_id) }
|
8
9
|
|
9
|
-
|
10
|
-
@url = subject._all(domain_id)
|
11
|
-
end
|
12
|
-
|
13
|
-
it do
|
14
|
-
@url.should eq "https://api.digitalocean.com/domains/#{domain_id}/records?client_id=client_id_required&api_key=api_key_required"
|
15
|
-
end
|
10
|
+
it { url.should eq "https://api.digitalocean.com/v1/domains/#{domain_id}/records?client_id=client_id_required&api_key=api_key_required" }
|
16
11
|
end
|
17
12
|
|
18
13
|
describe "._find" do
|
19
14
|
let(:domain_id) { "100" }
|
20
15
|
let(:record_id) { "50" }
|
21
16
|
|
22
|
-
|
23
|
-
@url = subject._find(domain_id, record_id)
|
24
|
-
end
|
17
|
+
let(:url) { record._find(domain_id, record_id) }
|
25
18
|
|
26
|
-
it
|
27
|
-
@url.should eq "https://api.digitalocean.com/domains/#{domain_id}/records/#{record_id}?client_id=client_id_required&api_key=api_key_required"
|
28
|
-
end
|
19
|
+
it { url.should eq "https://api.digitalocean.com/v1/domains/#{domain_id}/records/#{record_id}?client_id=client_id_required&api_key=api_key_required" }
|
29
20
|
end
|
30
21
|
|
31
22
|
describe "._create" do
|
@@ -34,13 +25,9 @@ describe Digitalocean::Record do
|
|
34
25
|
let(:data) { "@" }
|
35
26
|
let(:attrs) { {record_type: record_type, data: data } }
|
36
27
|
|
37
|
-
|
38
|
-
@url = subject._create(domain_id, attrs)
|
39
|
-
end
|
28
|
+
let(:url) { record._create(domain_id, attrs) }
|
40
29
|
|
41
|
-
it
|
42
|
-
@url.should eq "https://api.digitalocean.com/domains/#{domain_id}/records/new?client_id=client_id_required&api_key=api_key_required&record_type=#{record_type}&data=#{data}"
|
43
|
-
end
|
30
|
+
it { url.should eq "https://api.digitalocean.com/v1/domains/#{domain_id}/records/new?client_id=client_id_required&api_key=api_key_required&record_type=#{record_type}&data=#{data}" }
|
44
31
|
end
|
45
32
|
|
46
33
|
describe "._edit" do
|
@@ -50,25 +37,17 @@ describe Digitalocean::Record do
|
|
50
37
|
let(:data) { "@" }
|
51
38
|
let(:attrs) { {record_type: record_type, data: data } }
|
52
39
|
|
53
|
-
|
54
|
-
@url = subject._edit(domain_id, record_id, attrs)
|
55
|
-
end
|
40
|
+
let(:url) { record._edit(domain_id, record_id, attrs) }
|
56
41
|
|
57
|
-
it
|
58
|
-
@url.should eq "https://api.digitalocean.com/domains/#{domain_id}/records/#{record_id}/edit?client_id=client_id_required&api_key=api_key_required&record_type=#{record_type}&data=#{data}"
|
59
|
-
end
|
42
|
+
it { url.should eq "https://api.digitalocean.com/v1/domains/#{domain_id}/records/#{record_id}/edit?client_id=client_id_required&api_key=api_key_required&record_type=#{record_type}&data=#{data}" }
|
60
43
|
end
|
61
44
|
|
62
45
|
describe "._destroy" do
|
63
46
|
let(:domain_id) { "100" }
|
64
47
|
let(:record_id) { "50" }
|
65
48
|
|
66
|
-
|
67
|
-
@url = subject._destroy(domain_id, record_id)
|
68
|
-
end
|
49
|
+
let(:url) { record._destroy(domain_id, record_id) }
|
69
50
|
|
70
|
-
it
|
71
|
-
@url.should eq "https://api.digitalocean.com/domains/#{domain_id}/records/#{record_id}/destroy?client_id=client_id_required&api_key=api_key_required"
|
72
|
-
end
|
51
|
+
it { url.should eq "https://api.digitalocean.com/v1/domains/#{domain_id}/records/#{record_id}/destroy?client_id=client_id_required&api_key=api_key_required" }
|
73
52
|
end
|
74
53
|
end
|
@@ -1,28 +1,18 @@
|
|
1
1
|
require 'spec_helper'
|
2
2
|
|
3
3
|
describe Digitalocean::Region do
|
4
|
-
|
4
|
+
subject(:region) { described_class }
|
5
5
|
|
6
6
|
describe "._all" do
|
7
|
-
|
8
|
-
@url = subject._all
|
9
|
-
end
|
7
|
+
let(:url) { region._all }
|
10
8
|
|
11
|
-
it
|
12
|
-
@url.should eq "https://api.digitalocean.com/regions/?client_id=client_id_required&api_key=api_key_required"
|
13
|
-
end
|
9
|
+
it { url.should eq "https://api.digitalocean.com/v1/regions/?client_id=client_id_required&api_key=api_key_required" }
|
14
10
|
end
|
15
11
|
|
16
12
|
describe "._find" do
|
17
13
|
let(:region_id) { "1234" }
|
14
|
+
let(:url) { region._find(region_id) }
|
18
15
|
|
19
|
-
|
20
|
-
@url = subject._find(region_id)
|
21
|
-
end
|
22
|
-
|
23
|
-
it do
|
24
|
-
@url.should eq "https://api.digitalocean.com/regions/#{region_id}?client_id=client_id_required&api_key=api_key_required"
|
25
|
-
end
|
16
|
+
it { url.should eq "https://api.digitalocean.com/v1/regions/#{region_id}?client_id=client_id_required&api_key=api_key_required" }
|
26
17
|
end
|
27
|
-
|
28
18
|
end
|
@@ -1,28 +1,17 @@
|
|
1
1
|
require 'spec_helper'
|
2
2
|
|
3
3
|
describe Digitalocean::Size do
|
4
|
-
|
4
|
+
subject(:size) { described_class }
|
5
5
|
|
6
6
|
describe "._all" do
|
7
|
-
|
8
|
-
|
9
|
-
end
|
10
|
-
|
11
|
-
it do
|
12
|
-
@url.should eq "https://api.digitalocean.com/sizes/?client_id=client_id_required&api_key=api_key_required"
|
13
|
-
end
|
7
|
+
let(:url) { size._all }
|
8
|
+
it { url.should eq "https://api.digitalocean.com/v1/sizes/?client_id=client_id_required&api_key=api_key_required" }
|
14
9
|
end
|
15
10
|
|
16
11
|
describe "._find" do
|
17
12
|
let(:size_id) { "1234" }
|
13
|
+
let(:url) { size._find(size_id) }
|
18
14
|
|
19
|
-
|
20
|
-
@url = subject._find(size_id)
|
21
|
-
end
|
22
|
-
|
23
|
-
it do
|
24
|
-
@url.should eq "https://api.digitalocean.com/sizes/#{size_id}?client_id=client_id_required&api_key=api_key_required"
|
25
|
-
end
|
15
|
+
it { url.should eq "https://api.digitalocean.com/v1/sizes/#{size_id}?client_id=client_id_required&api_key=api_key_required" }
|
26
16
|
end
|
27
|
-
|
28
17
|
end
|
@@ -1,27 +1,29 @@
|
|
1
1
|
require 'spec_helper'
|
2
2
|
|
3
3
|
describe Digitalocean::SshKey do
|
4
|
-
|
4
|
+
subject(:ssh_key) { described_class }
|
5
5
|
|
6
6
|
describe "._all" do
|
7
|
-
|
8
|
-
@url = subject._all
|
9
|
-
end
|
7
|
+
let(:url) { ssh_key._all }
|
10
8
|
|
11
|
-
it
|
12
|
-
@url.should eq "https://api.digitalocean.com/ssh_keys/?client_id=client_id_required&api_key=api_key_required"
|
13
|
-
end
|
9
|
+
it { url.should eq "https://api.digitalocean.com/v1/ssh_keys/?client_id=client_id_required&api_key=api_key_required" }
|
14
10
|
end
|
15
11
|
|
16
12
|
describe "._find" do
|
17
13
|
let(:ssh_key_id) { "test_key" }
|
18
|
-
|
19
|
-
@url = subject._find(ssh_key_id)
|
20
|
-
end
|
14
|
+
let(:url) { ssh_key._find(ssh_key_id) }
|
21
15
|
|
22
|
-
it
|
23
|
-
|
24
|
-
|
16
|
+
it { url.should eq "https://api.digitalocean.com/v1/ssh_keys/test_key/?client_id=client_id_required&api_key=api_key_required" }
|
17
|
+
end
|
18
|
+
|
19
|
+
describe "._edit" do
|
20
|
+
let(:ssh_key_id) { "test_key" }
|
21
|
+
let(:ssh_pub_key) { "test_pub_key" }
|
22
|
+
let(:name) { "test_name" }
|
23
|
+
let(:attrs) { { name: name, ssh_pub_key: ssh_pub_key } }
|
24
|
+
let(:url) { ssh_key._edit(ssh_key_id, attrs) }
|
25
|
+
|
26
|
+
it { url.should eq "https://api.digitalocean.com/v1/ssh_keys/test_key/edit/?name=#{name}&ssh_pub_key=#{ssh_pub_key}&client_id=client_id_required&api_key=api_key_required" }
|
25
27
|
end
|
26
28
|
|
27
29
|
describe "._create" do
|
@@ -29,12 +31,15 @@ describe Digitalocean::SshKey do
|
|
29
31
|
let(:ssh_pub_key) { "test_pub_key" }
|
30
32
|
let(:attrs) { { name: name, ssh_pub_key: ssh_pub_key } }
|
31
33
|
|
32
|
-
|
33
|
-
|
34
|
-
|
34
|
+
let(:url) { ssh_key._create(attrs) }
|
35
|
+
|
36
|
+
it { url.should eq "https://api.digitalocean.com/v1/ssh_keys/new/?name=#{name}&ssh_pub_key=#{ssh_pub_key}&client_id=client_id_required&api_key=api_key_required" }
|
37
|
+
end
|
38
|
+
|
39
|
+
describe "._destroy" do
|
40
|
+
let(:ssh_key_id) { "test_key" }
|
41
|
+
let(:url) { ssh_key._destroy(ssh_key_id) }
|
35
42
|
|
36
|
-
it
|
37
|
-
@url.should eq "https://api.digitalocean.com/ssh_keys/new/?name=#{name}&ssh_pub_key=#{ssh_pub_key}&client_id=client_id_required&api_key=api_key_required"
|
38
|
-
end
|
43
|
+
it { url.should eq "https://api.digitalocean.com/v1/ssh_keys/#{ssh_key_id}/destroy/?client_id=client_id_required&api_key=api_key_required" }
|
39
44
|
end
|
40
45
|
end
|
data/spec/digitalocean_spec.rb
CHANGED
@@ -1,30 +1,29 @@
|
|
1
1
|
require 'spec_helper'
|
2
2
|
|
3
3
|
describe Digitalocean do
|
4
|
-
subject {
|
4
|
+
subject(:digitalocean) { described_class }
|
5
|
+
|
6
|
+
before do
|
7
|
+
digitalocean.client_id = client_id
|
8
|
+
digitalocean.api_key = api_key
|
9
|
+
end
|
5
10
|
|
6
11
|
describe "defaults" do
|
7
|
-
|
8
|
-
|
9
|
-
subject.api_key = nil
|
10
|
-
end
|
12
|
+
let(:client_id) { nil }
|
13
|
+
let(:api_key) { nil}
|
11
14
|
|
12
|
-
|
13
|
-
|
14
|
-
|
15
|
-
|
15
|
+
its(:api_endpoint) { should eq "https://api.digitalocean.com" }
|
16
|
+
its(:client_id) { should eq "client_id_required" }
|
17
|
+
its(:api_key) { should eq "api_key_required" }
|
18
|
+
|
19
|
+
it { digitalocean::VERSION.should eq "1.1.0" }
|
16
20
|
end
|
17
21
|
|
18
22
|
describe "setting values" do
|
19
|
-
let(:client_id)
|
20
|
-
let(:api_key)
|
21
|
-
|
22
|
-
before do
|
23
|
-
subject.client_id = client_id
|
24
|
-
subject.api_key = api_key
|
25
|
-
end
|
23
|
+
let(:client_id) { "1234" }
|
24
|
+
let(:api_key) { "adf3434938492fjkdfj" }
|
26
25
|
|
27
|
-
|
28
|
-
|
26
|
+
its(:client_id) { should eq client_id }
|
27
|
+
its(:api_key) { should eq api_key }
|
29
28
|
end
|
30
29
|
end
|
data/spec/spec_helper.rb
CHANGED
metadata
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
--- !ruby/object:Gem::Specification
|
2
2
|
name: digitalocean
|
3
3
|
version: !ruby/object:Gem::Version
|
4
|
-
version: 1.0
|
4
|
+
version: 1.1.0
|
5
5
|
prerelease:
|
6
6
|
platform: ruby
|
7
7
|
authors:
|
@@ -10,7 +10,7 @@ authors:
|
|
10
10
|
autorequire:
|
11
11
|
bindir: bin
|
12
12
|
cert_chain: []
|
13
|
-
date: 2014-
|
13
|
+
date: 2014-05-29 00:00:00.000000000 Z
|
14
14
|
dependencies:
|
15
15
|
- !ruby/object:Gem::Dependency
|
16
16
|
name: faraday
|