credible 0.1.1 → 0.1.2
This diff represents the content of publicly available package versions that have been released to one of the supported registries. The information contained in this diff is provided for informational purposes only and reflects changes between package versions as they appear in their respective public registries.
- checksums.yaml +4 -4
- data/README.md +110 -6
- data/lib/credible/version.rb +1 -1
- metadata +6 -8
- data/MIT-LICENSE +0 -20
- data/app/assets/config/credible_manifest.js +0 -1
- data/app/assets/stylesheets/credible/application.css +0 -15
checksums.yaml
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
---
|
2
2
|
SHA256:
|
3
|
-
metadata.gz:
|
4
|
-
data.tar.gz:
|
3
|
+
metadata.gz: 78167188c05b03d8745a6895582115a8bc9a7e907746d363d853c0b66007642e
|
4
|
+
data.tar.gz: 216308cfe275a979d556b611fab6df86e54344f50799112b9c694c18af9e2b33
|
5
5
|
SHA512:
|
6
|
-
metadata.gz:
|
7
|
-
data.tar.gz:
|
6
|
+
metadata.gz: e4a3329ad3a8f108cb8e5a90849ef215dea4d628891c4bbf8535087511ae89e71f6509210153df363a16074a6480b40d00c8a19dffcab1494db6d2ba299d2299
|
7
|
+
data.tar.gz: f3de667f740c74484648a7d2a658c64c0f37c8aeb33d8fbf947d5a21fa6ab38d9534f755e4694c7592d64423ea0fc73c2a38891b3038f57585a0ce12fd304a23
|
data/README.md
CHANGED
@@ -1,10 +1,15 @@
|
|
1
1
|
# Credible
|
2
|
-
Short description and motivation.
|
3
2
|
|
4
|
-
|
5
|
-
|
3
|
+
[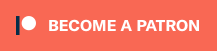](https://www.patreon.com/thombruce)
|
4
|
+
|
5
|
+
[](https://rubygems.org/gems/credible)
|
6
|
+
|
7
|
+
[](MIT-LICENSE)
|
8
|
+
|
9
|
+
Credible was extracted from [thombruce/helvellyn](https://github.com/thombruce/helvellyn) and is still a work in progress. The goal is to provide JWT and API token based authentication using [Warden](https://github.com/wardencommunity/warden/) for Rails API applications.
|
6
10
|
|
7
11
|
## Installation
|
12
|
+
|
8
13
|
Add this line to your application's Gemfile:
|
9
14
|
|
10
15
|
```ruby
|
@@ -16,13 +21,112 @@ And then execute:
|
|
16
21
|
$ bundle
|
17
22
|
```
|
18
23
|
|
19
|
-
|
24
|
+
Mount Credible's authentication routes in your `config/routes.rb` file:
|
25
|
+
|
26
|
+
```ruby
|
27
|
+
Rails.application.routes.draw do
|
28
|
+
mount Credible::Engine => "/auth"
|
29
|
+
end
|
30
|
+
```
|
31
|
+
|
32
|
+
Credible is a work in progress and requires a complicated setup for now. It was extracted from [thombruce/helvellyn](https://github.com/thombruce/helvellyn). You'll need to add models and configure them manually. In future, I'll had helpers to handle this for you.
|
33
|
+
|
34
|
+
## Add Models
|
35
|
+
|
36
|
+
To get Credible working, your app will need at least a User model and a Session model.
|
37
|
+
|
38
|
+
### User model
|
39
|
+
|
20
40
|
```bash
|
21
|
-
|
41
|
+
rails g model User email:string:uniq password_digest:string confirmation_token:token confirmed_at:datetime
|
42
|
+
```
|
43
|
+
|
44
|
+
```ruby
|
45
|
+
class User < ApplicationRecord
|
46
|
+
has_secure_password validations: false
|
47
|
+
has_secure_token :confirmation_token
|
48
|
+
|
49
|
+
has_many :sessions, dependent: :destroy
|
50
|
+
|
51
|
+
validates :email, presence: true
|
52
|
+
validates :email, format: { with: URI::MailTo::EMAIL_REGEXP }
|
53
|
+
|
54
|
+
# Custom password validation
|
55
|
+
validate do |record|
|
56
|
+
record.errors.add(:password, :blank) unless record.password_digest.present? || record.confirmation_token.present?
|
57
|
+
end
|
58
|
+
|
59
|
+
validates_length_of :password, maximum: ActiveModel::SecurePassword::MAX_PASSWORD_LENGTH_ALLOWED
|
60
|
+
validates_confirmation_of :password, allow_blank: true
|
61
|
+
# End custom password validation
|
62
|
+
|
63
|
+
def self.authenticate(params)
|
64
|
+
user = find_by_login(params[:login])
|
65
|
+
user&.authenticate(params[:password])
|
66
|
+
end
|
67
|
+
|
68
|
+
def confirm
|
69
|
+
self.confirmation_token = nil
|
70
|
+
self.password = SecureRandom.hex(8) unless password_digest.present?
|
71
|
+
self.confirmed_at = Time.now.utc
|
72
|
+
end
|
73
|
+
|
74
|
+
def confirmed?
|
75
|
+
confirmed_at.present?
|
76
|
+
end
|
77
|
+
|
78
|
+
def reset_password
|
79
|
+
self.password_digest = nil
|
80
|
+
self.confirmed_at = nil
|
81
|
+
regenerate_confirmation_token
|
82
|
+
end
|
83
|
+
end
|
84
|
+
```
|
85
|
+
|
86
|
+
### Session model
|
87
|
+
|
88
|
+
```bash
|
89
|
+
rails g model Session user:references token:token
|
90
|
+
```
|
91
|
+
|
92
|
+
```ruby
|
93
|
+
class Session < ApplicationRecord
|
94
|
+
belongs_to :user
|
95
|
+
|
96
|
+
has_secure_token
|
97
|
+
|
98
|
+
def self.authenticate(params)
|
99
|
+
user = User.authenticate(params)
|
100
|
+
new(user: user)
|
101
|
+
end
|
102
|
+
|
103
|
+
def jwt
|
104
|
+
payload = {
|
105
|
+
data: jwt_data,
|
106
|
+
iss: 'Helvellyn'
|
107
|
+
}
|
108
|
+
JWT.encode payload, Rails.application.secrets.secret_key_base, 'HS256' # [1]
|
109
|
+
end
|
110
|
+
|
111
|
+
private
|
112
|
+
|
113
|
+
def jwt_data
|
114
|
+
{
|
115
|
+
session_id: id,
|
116
|
+
user_id: user.id,
|
117
|
+
user: {
|
118
|
+
id: user.id,
|
119
|
+
email: user.email
|
120
|
+
}
|
121
|
+
}
|
122
|
+
end
|
123
|
+
end
|
22
124
|
```
|
23
125
|
|
24
126
|
## Contributing
|
25
|
-
|
127
|
+
|
128
|
+
Credible is not yet accepting contributions. Watch this space.
|
26
129
|
|
27
130
|
## License
|
131
|
+
|
28
132
|
The gem is available as open source under the terms of the [MIT License](https://opensource.org/licenses/MIT).
|
data/lib/credible/version.rb
CHANGED
metadata
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
--- !ruby/object:Gem::Specification
|
2
2
|
name: credible
|
3
3
|
version: !ruby/object:Gem::Version
|
4
|
-
version: 0.1.
|
4
|
+
version: 0.1.2
|
5
5
|
platform: ruby
|
6
6
|
authors:
|
7
7
|
- Thom Bruce
|
@@ -78,14 +78,14 @@ dependencies:
|
|
78
78
|
requirements:
|
79
79
|
- - "~>"
|
80
80
|
- !ruby/object:Gem::Version
|
81
|
-
version:
|
81
|
+
version: 3.9.0
|
82
82
|
type: :development
|
83
83
|
prerelease: false
|
84
84
|
version_requirements: !ruby/object:Gem::Requirement
|
85
85
|
requirements:
|
86
86
|
- - "~>"
|
87
87
|
- !ruby/object:Gem::Version
|
88
|
-
version:
|
88
|
+
version: 3.9.0
|
89
89
|
description: Provides token-based authentication for Rails API apps.
|
90
90
|
email:
|
91
91
|
- thom@thombruce.com
|
@@ -93,11 +93,8 @@ executables: []
|
|
93
93
|
extensions: []
|
94
94
|
extra_rdoc_files: []
|
95
95
|
files:
|
96
|
-
- MIT-LICENSE
|
97
96
|
- README.md
|
98
97
|
- Rakefile
|
99
|
-
- app/assets/config/credible_manifest.js
|
100
|
-
- app/assets/stylesheets/credible/application.css
|
101
98
|
- app/controllers/credible/application_controller.rb
|
102
99
|
- app/controllers/credible/authentication/sessions_controller.rb
|
103
100
|
- app/controllers/credible/authentication/users_controller.rb
|
@@ -125,10 +122,11 @@ files:
|
|
125
122
|
- lib/credible/engine.rb
|
126
123
|
- lib/credible/version.rb
|
127
124
|
- lib/tasks/credible_tasks.rake
|
128
|
-
homepage: https://
|
125
|
+
homepage: https://github.com/thombruce/credible
|
129
126
|
licenses:
|
130
127
|
- MIT
|
131
|
-
metadata:
|
128
|
+
metadata:
|
129
|
+
source_code_uri: https://github.com/example/example
|
132
130
|
post_install_message:
|
133
131
|
rdoc_options: []
|
134
132
|
require_paths:
|
data/MIT-LICENSE
DELETED
@@ -1,20 +0,0 @@
|
|
1
|
-
Copyright 2020 Thom Bruce
|
2
|
-
|
3
|
-
Permission is hereby granted, free of charge, to any person obtaining
|
4
|
-
a copy of this software and associated documentation files (the
|
5
|
-
"Software"), to deal in the Software without restriction, including
|
6
|
-
without limitation the rights to use, copy, modify, merge, publish,
|
7
|
-
distribute, sublicense, and/or sell copies of the Software, and to
|
8
|
-
permit persons to whom the Software is furnished to do so, subject to
|
9
|
-
the following conditions:
|
10
|
-
|
11
|
-
The above copyright notice and this permission notice shall be
|
12
|
-
included in all copies or substantial portions of the Software.
|
13
|
-
|
14
|
-
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
15
|
-
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
16
|
-
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
17
|
-
NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
18
|
-
LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
19
|
-
OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
20
|
-
WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
@@ -1 +0,0 @@
|
|
1
|
-
//= link_directory ../stylesheets/credible .css
|
@@ -1,15 +0,0 @@
|
|
1
|
-
/*
|
2
|
-
* This is a manifest file that'll be compiled into application.css, which will include all the files
|
3
|
-
* listed below.
|
4
|
-
*
|
5
|
-
* Any CSS and SCSS file within this directory, lib/assets/stylesheets, vendor/assets/stylesheets,
|
6
|
-
* or any plugin's vendor/assets/stylesheets directory can be referenced here using a relative path.
|
7
|
-
*
|
8
|
-
* You're free to add application-wide styles to this file and they'll appear at the bottom of the
|
9
|
-
* compiled file so the styles you add here take precedence over styles defined in any other CSS/SCSS
|
10
|
-
* files in this directory. Styles in this file should be added after the last require_* statement.
|
11
|
-
* It is generally better to create a new file per style scope.
|
12
|
-
*
|
13
|
-
*= require_tree .
|
14
|
-
*= require_self
|
15
|
-
*/
|