copycopter_client 1.0.0.beta9 → 1.0.0.beta10
Sign up to get free protection for your applications and to get access to all the features.
- data/README.md +81 -0
- data/features/step_definitions/rails_steps.rb +1 -0
- data/lib/copycopter_client/client.rb +5 -2
- data/lib/copycopter_client/configuration.rb +9 -2
- data/lib/copycopter_client/version.rb +1 -1
- data/spec/copycopter_client/client_spec.rb +3 -1
- data/spec/copycopter_client/configuration_spec.rb +8 -2
- data/spec/spec_helper.rb +1 -1
- metadata +9 -10
- data/README.textile +0 -71
data/README.md
ADDED
@@ -0,0 +1,81 @@
|
|
1
|
+
Copycopter Client
|
2
|
+
=================
|
3
|
+
|
4
|
+
This is the client gem for integrating apps with "Copycopter":http://copycopter.com.
|
5
|
+
|
6
|
+
The client integrates with the I18n gem so that you can access copy text and translations from a Copycopter project.
|
7
|
+
|
8
|
+
Installation
|
9
|
+
------------
|
10
|
+
|
11
|
+
Just install the gem:
|
12
|
+
|
13
|
+
gem install copycopter_client
|
14
|
+
|
15
|
+
### Rails 3
|
16
|
+
|
17
|
+
Add the following line to your `Gemfile`:
|
18
|
+
|
19
|
+
gem "copycopter_client"
|
20
|
+
|
21
|
+
Then run `bundle install`.
|
22
|
+
|
23
|
+
### Rails 2
|
24
|
+
|
25
|
+
Add the following line to your `config/environment.rb`:
|
26
|
+
|
27
|
+
config.gem 'copycopter_client'
|
28
|
+
|
29
|
+
Then run `rake gems:install`. We also recommend vendoring the gem by running `rake gems:unpack:dependencies GEM=copycopter_client`.
|
30
|
+
|
31
|
+
### Configuration
|
32
|
+
|
33
|
+
Add the following to your application:
|
34
|
+
|
35
|
+
CopycopterClient.configure do |config|
|
36
|
+
config.api_key = "YOUR API KEY HERE"
|
37
|
+
end
|
38
|
+
|
39
|
+
In a Rails application, this should be saved as `config/initializers/copycopter.rb`. You can find the API key on the project page on the Copycopter website. See the CopycopterClient::Configuration class for a full list of configuration options.
|
40
|
+
|
41
|
+
Usage
|
42
|
+
-----
|
43
|
+
|
44
|
+
You can access blurbs from Copycopter by using `I18n.translate`. This is also aliased as `translate` or just `t` inside Rails controllers and views.
|
45
|
+
|
46
|
+
# In a controller
|
47
|
+
def index
|
48
|
+
flash[:success] = t("users.create.success", :default => "User created")
|
49
|
+
end
|
50
|
+
|
51
|
+
# In a view
|
52
|
+
<%= t(".welcome", :default => "Why hello there") %>
|
53
|
+
|
54
|
+
# Global scope (for example, in a Rake task)
|
55
|
+
I18n.translate("system.tasks_complete", :default => "Tasks complete")
|
56
|
+
|
57
|
+
# Interpolation
|
58
|
+
I18n.translate("mailer.welcome", :default => "Welcome, %{name}!", :name => @user.name)
|
59
|
+
|
60
|
+
See the [I18n documentation](http://rdoc.info/github/svenfuchs/i18n/master/file/README.textile) documentation for more examples.
|
61
|
+
|
62
|
+
Deploys
|
63
|
+
-------
|
64
|
+
|
65
|
+
Blurbs start out as draft copy, and won't be displayed in production environments until they're published. If you want to publish all draft copy when deploying to production, you can use the `copycopter:deploy` rake task:
|
66
|
+
|
67
|
+
rake copycopter:deploy
|
68
|
+
|
69
|
+
Credits
|
70
|
+
-------
|
71
|
+
|
72
|
+
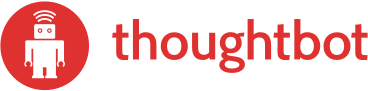
|
73
|
+
|
74
|
+
Copycopter Client is maintained and funded by [thoughtbot, inc](http://thoughtbot.com/community)
|
75
|
+
|
76
|
+
The names and logos for thoughtbot are trademarks of thoughtbot, inc.
|
77
|
+
|
78
|
+
License
|
79
|
+
-------
|
80
|
+
|
81
|
+
Copycopter Client is Copyright © 2010-2011 thoughtbot. It is free software, and may be redistributed under the terms specified in the MIT-LICENSE file.
|
@@ -23,9 +23,10 @@ module CopycopterClient
|
|
23
23
|
# @option options [Fixnum] :http_open_timeout how long to wait before timing out when opening the socket
|
24
24
|
# @option options [Boolean] :secure whether to use SSL
|
25
25
|
# @option options [Logger] :logger where to log transactions
|
26
|
+
# @option options [String] :ca_file path to root certificate file for ssl verification
|
26
27
|
def initialize(options)
|
27
28
|
[:api_key, :host, :port, :public, :http_read_timeout,
|
28
|
-
:http_open_timeout, :secure, :logger].each do |option|
|
29
|
+
:http_open_timeout, :secure, :logger, :ca_file].each do |option|
|
29
30
|
instance_variable_set("@#{option}", options[option])
|
30
31
|
end
|
31
32
|
end
|
@@ -79,7 +80,7 @@ module CopycopterClient
|
|
79
80
|
private
|
80
81
|
|
81
82
|
attr_reader :host, :port, :api_key, :http_read_timeout,
|
82
|
-
:http_open_timeout, :secure, :logger
|
83
|
+
:http_open_timeout, :secure, :logger, :ca_file
|
83
84
|
|
84
85
|
def public?
|
85
86
|
@public
|
@@ -102,6 +103,8 @@ module CopycopterClient
|
|
102
103
|
http.open_timeout = http_open_timeout
|
103
104
|
http.read_timeout = http_read_timeout
|
104
105
|
http.use_ssl = secure
|
106
|
+
http.verify_mode = OpenSSL::SSL::VERIFY_PEER
|
107
|
+
http.ca_file = ca_file
|
105
108
|
begin
|
106
109
|
yield(http)
|
107
110
|
rescue *HTTP_ERRORS => exception
|
@@ -14,7 +14,10 @@ module CopycopterClient
|
|
14
14
|
:http_open_timeout, :http_read_timeout, :client_name, :client_url,
|
15
15
|
:client_version, :port, :protocol, :proxy_host, :proxy_pass,
|
16
16
|
:proxy_port, :proxy_user, :secure, :polling_delay, :logger,
|
17
|
-
:framework, :middleware].freeze
|
17
|
+
:framework, :middleware, :ca_file].freeze
|
18
|
+
|
19
|
+
# Default root certificate used to verify ssl sessions.
|
20
|
+
CA_FILE = File.expand_path('../../../AddTrustExternalCARoot.crt', __FILE__).freeze
|
18
21
|
|
19
22
|
# @return [String] The API key for your project, found on the project edit form.
|
20
23
|
attr_accessor :api_key
|
@@ -76,11 +79,14 @@ module CopycopterClient
|
|
76
79
|
# @return the middleware stack, if any, which should respond to +use+
|
77
80
|
attr_accessor :middleware
|
78
81
|
|
82
|
+
# @return [String] the path to a root certificate file used to verify ssl sessions. Default's to the root certificate file for copycopter.com.
|
83
|
+
attr_accessor :ca_file
|
84
|
+
|
79
85
|
alias_method :secure?, :secure
|
80
86
|
|
81
87
|
# Instantiated from {CopycopterClient.configure}. Sets defaults.
|
82
88
|
def initialize
|
83
|
-
self.secure =
|
89
|
+
self.secure = true
|
84
90
|
self.host = 'copycopter.com'
|
85
91
|
self.http_open_timeout = 2
|
86
92
|
self.http_read_timeout = 5
|
@@ -91,6 +97,7 @@ module CopycopterClient
|
|
91
97
|
self.client_url = 'http://copycopter.com'
|
92
98
|
self.polling_delay = 300
|
93
99
|
self.logger = Logger.new($stdout)
|
100
|
+
self.ca_file = CA_FILE
|
94
101
|
|
95
102
|
@applied = false
|
96
103
|
end
|
@@ -40,11 +40,13 @@ describe CopycopterClient do
|
|
40
40
|
http.read_timeout.should == 4
|
41
41
|
end
|
42
42
|
|
43
|
-
it "uses ssl when secure" do
|
43
|
+
it "uses verified ssl when secure" do
|
44
44
|
project = add_project
|
45
45
|
client = build_client(:api_key => project.api_key, :secure => true)
|
46
46
|
client.download { |ignore| }
|
47
47
|
http.use_ssl.should == true
|
48
|
+
http.verify_mode.should == OpenSSL::SSL::VERIFY_PEER
|
49
|
+
http.ca_file.should == CopycopterClient::Configuration::CA_FILE
|
48
50
|
end
|
49
51
|
|
50
52
|
it "doesn't use ssl when insecure" do
|
@@ -33,7 +33,7 @@ describe CopycopterClient::Configuration do
|
|
33
33
|
it { should have_config_option(:client_version). overridable.default(CopycopterClient::VERSION) }
|
34
34
|
it { should have_config_option(:client_name). overridable.default('Copycopter Client') }
|
35
35
|
it { should have_config_option(:client_url). overridable.default('http://copycopter.com') }
|
36
|
-
it { should have_config_option(:secure). overridable.default(
|
36
|
+
it { should have_config_option(:secure). overridable.default(true) }
|
37
37
|
it { should have_config_option(:host). overridable.default('copycopter.com') }
|
38
38
|
it { should have_config_option(:http_open_timeout). overridable.default(2) }
|
39
39
|
it { should have_config_option(:http_read_timeout). overridable.default(5) }
|
@@ -44,6 +44,12 @@ describe CopycopterClient::Configuration do
|
|
44
44
|
it { should have_config_option(:framework). overridable }
|
45
45
|
it { should have_config_option(:middleware). overridable }
|
46
46
|
|
47
|
+
it "provides the root ssl certificate" do
|
48
|
+
should have_config_option(:ca_file).
|
49
|
+
overridable.
|
50
|
+
default(File.join(PROJECT_ROOT, "AddTrustExternalCARoot.crt"))
|
51
|
+
end
|
52
|
+
|
47
53
|
it "should provide default values for secure connections" do
|
48
54
|
config = CopycopterClient::Configuration.new
|
49
55
|
config.secure = true
|
@@ -72,7 +78,7 @@ describe CopycopterClient::Configuration do
|
|
72
78
|
[:api_key, :environment_name, :host, :http_open_timeout,
|
73
79
|
:http_read_timeout, :client_name, :client_url, :client_version, :port,
|
74
80
|
:protocol, :proxy_host, :proxy_pass, :proxy_port, :proxy_user, :secure,
|
75
|
-
:development_environments, :logger, :framework].each do |option|
|
81
|
+
:development_environments, :logger, :framework, :ca_file].each do |option|
|
76
82
|
hash[option].should == config[option]
|
77
83
|
end
|
78
84
|
hash[:public].should == config.public?
|
data/spec/spec_helper.rb
CHANGED
@@ -16,7 +16,7 @@ Dir.glob(File.join(PROJECT_ROOT, "spec", "support", "**", "*.rb")).each do |file
|
|
16
16
|
end
|
17
17
|
|
18
18
|
WebMock.disable_net_connect!
|
19
|
-
ShamRack.mount(FakeCopycopterApp.new, "copycopter.com")
|
19
|
+
ShamRack.mount(FakeCopycopterApp.new, "copycopter.com", 443)
|
20
20
|
|
21
21
|
Spec::Runner.configure do |config|
|
22
22
|
config.include ClientSpecHelpers
|
metadata
CHANGED
@@ -1,14 +1,14 @@
|
|
1
1
|
--- !ruby/object:Gem::Specification
|
2
2
|
name: copycopter_client
|
3
3
|
version: !ruby/object:Gem::Version
|
4
|
-
hash:
|
4
|
+
hash: 180128174
|
5
5
|
prerelease: true
|
6
6
|
segments:
|
7
7
|
- 1
|
8
8
|
- 0
|
9
9
|
- 0
|
10
|
-
-
|
11
|
-
version: 1.0.0.
|
10
|
+
- beta10
|
11
|
+
version: 1.0.0.beta10
|
12
12
|
platform: ruby
|
13
13
|
authors:
|
14
14
|
- thoughtbot
|
@@ -16,7 +16,7 @@ autorequire:
|
|
16
16
|
bindir: bin
|
17
17
|
cert_chain: []
|
18
18
|
|
19
|
-
date:
|
19
|
+
date: 2011-01-31 00:00:00 -05:00
|
20
20
|
default_executable:
|
21
21
|
dependencies:
|
22
22
|
- !ruby/object:Gem::Dependency
|
@@ -55,9 +55,10 @@ executables: []
|
|
55
55
|
|
56
56
|
extensions: []
|
57
57
|
|
58
|
-
extra_rdoc_files:
|
59
|
-
|
58
|
+
extra_rdoc_files: []
|
59
|
+
|
60
60
|
files:
|
61
|
+
- README.md
|
61
62
|
- MIT-LICENSE
|
62
63
|
- Rakefile
|
63
64
|
- init.rb
|
@@ -98,15 +99,13 @@ files:
|
|
98
99
|
- features/step_definitions/rails_steps.rb
|
99
100
|
- features/support/env.rb
|
100
101
|
- features/support/rails_server.rb
|
101
|
-
- README.textile
|
102
102
|
has_rdoc: true
|
103
103
|
homepage: http://github.com/thoughtbot/copycopter_client
|
104
104
|
licenses: []
|
105
105
|
|
106
106
|
post_install_message:
|
107
|
-
rdoc_options:
|
108
|
-
|
109
|
-
- --main
|
107
|
+
rdoc_options: []
|
108
|
+
|
110
109
|
require_paths:
|
111
110
|
- lib
|
112
111
|
required_ruby_version: !ruby/object:Gem::Requirement
|
data/README.textile
DELETED
@@ -1,71 +0,0 @@
|
|
1
|
-
h1. Copycopter Client
|
2
|
-
|
3
|
-
This is the client gem for integrating apps with "Copycopter":http://copycopter.com.
|
4
|
-
|
5
|
-
The client integrates with the I18n gem so that you can access copy text and translations from a Copycopter project.
|
6
|
-
|
7
|
-
h2. Installation
|
8
|
-
|
9
|
-
Just install the gem:
|
10
|
-
|
11
|
-
<pre>gem install copycopter_client</pre>
|
12
|
-
|
13
|
-
h3. Rails 3
|
14
|
-
|
15
|
-
Add the following line to your <code>Gemfile</code>:
|
16
|
-
|
17
|
-
<pre>gem "copycopter_client"</pre>
|
18
|
-
|
19
|
-
Then run <code>bundle install</code>.
|
20
|
-
|
21
|
-
h3. Rails 2
|
22
|
-
|
23
|
-
Add the following line to your <code>config/environment.rb</code>:
|
24
|
-
|
25
|
-
<pre>config.gem 'copycopter_client'</pre>
|
26
|
-
|
27
|
-
Then run <code>rake gems:install</code>. We also recommend vendoring the gem by running <code>rake gems:unpack:dependencies GEM=copycopter_client</code>.
|
28
|
-
|
29
|
-
h2. Configuration
|
30
|
-
|
31
|
-
Add the following to your application:
|
32
|
-
|
33
|
-
<pre>
|
34
|
-
CopycopterClient.configure do |config|
|
35
|
-
config.api_key = "YOUR API KEY HERE"
|
36
|
-
end
|
37
|
-
</pre>
|
38
|
-
|
39
|
-
In a Rails application, this should be saved as <code>config/initializers/copycopter.rb</code>. You can find the API key on the project page on the Copycopter website. See the {CopycopterClient::Configuration} class for a full list of configuration options.
|
40
|
-
|
41
|
-
h2. Usage
|
42
|
-
|
43
|
-
You can access blurbs from Copycopter by using <code>I18n.translate</code>. This is also aliased as <code>translate</code> or just <code>t</code> inside Rails controllers and views.
|
44
|
-
|
45
|
-
<pre>
|
46
|
-
# In a controller
|
47
|
-
def index
|
48
|
-
flash[:success] = t("users.create.success", :default => "User created")
|
49
|
-
end
|
50
|
-
|
51
|
-
# In a view
|
52
|
-
<%= t(".welcome", :default => "Why hello there") %>
|
53
|
-
|
54
|
-
# Global scope (for example, in a Rake task)
|
55
|
-
I18n.translate("system.tasks_complete", :default => "Tasks complete")
|
56
|
-
|
57
|
-
# Interpolation
|
58
|
-
I18n.translate("mailer.welcome", :default => "Welcome, %{name}!", :name => @user.name)
|
59
|
-
</pre>
|
60
|
-
|
61
|
-
See the "I18n documentation":http://rdoc.info/github/svenfuchs/i18n/master/file/README.textile documentation for more examples.
|
62
|
-
|
63
|
-
h2. Deploys
|
64
|
-
|
65
|
-
Blurbs start out as draft copy, and won't be displayed in production environments until they're published. If you want to publish all draft copy when deploying to production, you can use the <code>copycopter:deploy</code> rake task:
|
66
|
-
|
67
|
-
<pre>rake copycopter:deploy</pre>
|
68
|
-
|
69
|
-
h2. About
|
70
|
-
|
71
|
-
Copyright (c) 2010 "thoughtbot, inc.":http://thoughtbot.com, released under the MIT license
|