cloudflare-dns-update 2.1.1 → 3.0.0
Sign up to get free protection for your applications and to get access to all the features.
- checksums.yaml +5 -5
- data/bin/cloudflare-dns-update +9 -2
- data/lib/.DS_Store +0 -0
- data/lib/cloudflare/dns/update/command.rb +69 -80
- data/lib/cloudflare/dns/update/version.rb +1 -1
- metadata +57 -42
- data/.gitignore +0 -20
- data/.rspec +0 -4
- data/.travis.yml +0 -19
- data/Gemfile +0 -9
- data/README.md +0 -78
- data/Rakefile +0 -10
- data/cloudflare-dns-update.gemspec +0 -32
- data/spec/cloudflare/dns/update/command_spec.rb +0 -48
- data/spec/spec_helper.rb +0 -29
checksums.yaml
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
---
|
2
|
-
|
3
|
-
metadata.gz:
|
4
|
-
data.tar.gz:
|
2
|
+
SHA256:
|
3
|
+
metadata.gz: 84fada8eb1262bb18ed4a1e7d0392f958abe2a311f9fcba6a1703d6cea946634
|
4
|
+
data.tar.gz: b51bd44936abe2811def5ddbc0d9afcc5c74e2c447d4c36d2185904e1f8365b3
|
5
5
|
SHA512:
|
6
|
-
metadata.gz:
|
7
|
-
data.tar.gz:
|
6
|
+
metadata.gz: 1df9331da1630b9eacf84167a68b893dd2002496e70cb03e2d55d731deb91b1eeeea66441cbf2ac29adeb2853edd962d544715a9cfb4965cf79db48ad352bd87
|
7
|
+
data.tar.gz: a0d4cfadca21c79211029fd65731f54739c0ba45b45f587ed7b746cf3d8b8c5d8a5c98725656efcd62441968e21adab3e9b1966ba94dad48eeaf995c6733a00c
|
data/bin/cloudflare-dns-update
CHANGED
@@ -20,6 +20,13 @@
|
|
20
20
|
# OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
|
21
21
|
# THE SOFTWARE.
|
22
22
|
|
23
|
-
|
23
|
+
require_relative '../lib/cloudflare/dns/update/command'
|
24
24
|
|
25
|
-
|
25
|
+
begin
|
26
|
+
Cloudflare::DNS::Update::Command.call
|
27
|
+
rescue Interrupt
|
28
|
+
# Ignore.
|
29
|
+
rescue => error
|
30
|
+
Async.logger.error(Falcon::Command) {error}
|
31
|
+
exit! 1
|
32
|
+
end
|
data/lib/.DS_Store
ADDED
Binary file
|
@@ -30,8 +30,8 @@ require_relative 'version'
|
|
30
30
|
|
31
31
|
module Cloudflare::DNS::Update
|
32
32
|
module Command
|
33
|
-
def self.
|
34
|
-
Top.
|
33
|
+
def self.call(*arguments)
|
34
|
+
Top.call(*arguments)
|
35
35
|
end
|
36
36
|
|
37
37
|
# The top level utopia command.
|
@@ -39,31 +39,13 @@ module Cloudflare::DNS::Update
|
|
39
39
|
self.description = "Update remote DNS records according to locally run commands."
|
40
40
|
|
41
41
|
options do
|
42
|
-
option '-c/--configuration <path>', "Use the specified configuration file."
|
42
|
+
option '-c/--configuration <path>', "Use the specified configuration file.", default: 'dns.conf'
|
43
43
|
option '-f/--force', "Force push updates to cloudflare even if content hasn't changed.", default: false
|
44
|
-
option '--verbose | --quiet', "Verbosity of output for debugging.", key: :logging
|
45
|
-
option '-h/--help', "Print out help information."
|
46
44
|
option '-v/--version', "Print out the application version."
|
47
45
|
end
|
48
46
|
|
49
|
-
def verbose?
|
50
|
-
@options[:logging] == :verbose
|
51
|
-
end
|
52
|
-
|
53
|
-
def quiet?
|
54
|
-
@options[:logging] == :quiet
|
55
|
-
end
|
56
|
-
|
57
47
|
def logger
|
58
|
-
@logger ||=
|
59
|
-
if verbose?
|
60
|
-
logger.level = Logger::DEBUG
|
61
|
-
elsif quiet?
|
62
|
-
logger.level = Logger::WARN
|
63
|
-
else
|
64
|
-
logger.level = Logger::INFO
|
65
|
-
end
|
66
|
-
end
|
48
|
+
@logger ||= Async.logger
|
67
49
|
end
|
68
50
|
|
69
51
|
def prompt
|
@@ -78,25 +60,31 @@ module Cloudflare::DNS::Update
|
|
78
60
|
|
79
61
|
def connect!
|
80
62
|
configuration_store.transaction do |configuration|
|
81
|
-
unless configuration[:
|
82
|
-
prompt.puts "This configuration
|
83
|
-
configuration[:
|
84
|
-
configuration[:email] = prompt.ask("Cloudflare Email:")
|
63
|
+
unless configuration[:token]
|
64
|
+
prompt.puts "This configuration does not contain authorization token, we require some details."
|
65
|
+
configuration[:token] = prompt.mask("Cloudflare token:")
|
85
66
|
end
|
86
67
|
|
87
|
-
|
88
|
-
|
89
|
-
|
90
|
-
|
68
|
+
@connection = Cloudflare.connect(token: configuration[:token])
|
69
|
+
end
|
70
|
+
|
71
|
+
return @connection unless block_given?
|
72
|
+
|
73
|
+
begin
|
74
|
+
yield @connection
|
75
|
+
rescue Interrupt
|
76
|
+
# Exit gracefully
|
77
|
+
ensure
|
78
|
+
@connection.close
|
91
79
|
end
|
92
80
|
end
|
93
81
|
|
94
82
|
def initialize_zone
|
95
83
|
configuration_store.transaction do |configuration|
|
96
84
|
unless configuration[:zone]
|
97
|
-
zone = prompt.select("What zone do you want to update?", @connection.zones
|
85
|
+
zone = prompt.select("What zone do you want to update?", @connection.zones)
|
98
86
|
|
99
|
-
configuration[:zone] = zone.
|
87
|
+
configuration[:zone] = zone.value
|
100
88
|
end
|
101
89
|
end
|
102
90
|
end
|
@@ -111,9 +99,9 @@ module Cloudflare::DNS::Update
|
|
111
99
|
zone_id = configuration[:zone][:id]
|
112
100
|
zone = @connection.zones.find_by_id(zone_id)
|
113
101
|
|
114
|
-
dns_records = prompt.multi_select("What records do you want to update (select 1 or more)?", zone.dns_records
|
102
|
+
dns_records = prompt.multi_select("What records do you want to update (select 1 or more)?", zone.dns_records)
|
115
103
|
|
116
|
-
domains = configuration[:domains] = dns_records.map(&:
|
104
|
+
domains = configuration[:domains] = dns_records.map(&:value)
|
117
105
|
end
|
118
106
|
end
|
119
107
|
end
|
@@ -126,67 +114,68 @@ module Cloudflare::DNS::Update
|
|
126
114
|
end
|
127
115
|
end
|
128
116
|
|
129
|
-
def
|
130
|
-
|
131
|
-
logger.
|
132
|
-
content, status = Open3.capture2(configuration[:content_command])
|
117
|
+
def update_domain(zone, record, content)
|
118
|
+
if content != record[:content] || @options[:force]
|
119
|
+
logger.info "Content changed #{content.inspect}, updating records..."
|
133
120
|
|
134
|
-
|
135
|
-
|
136
|
-
|
137
|
-
|
138
|
-
|
139
|
-
|
121
|
+
domain = zone.dns_records.find_by_id(record[:id])
|
122
|
+
|
123
|
+
begin
|
124
|
+
domain.update_content(content)
|
125
|
+
|
126
|
+
logger.info "Updated domain: #{record[:name]} #{record[:type]} #{content}"
|
127
|
+
record[:content] = content
|
128
|
+
rescue => error
|
129
|
+
logger.warn("Failed to update domain: #{record[:name]} #{record[:type]} #{content}") {error}
|
140
130
|
end
|
141
|
-
|
142
|
-
|
143
|
-
|
144
|
-
|
145
|
-
|
146
|
-
|
131
|
+
else
|
132
|
+
logger.debug "Content hasn't changed: #{record[:name]} #{record[:type]} #{content}"
|
133
|
+
end
|
134
|
+
end
|
135
|
+
|
136
|
+
def update_domains(content = nil)
|
137
|
+
configuration_store.transaction do |configuration|
|
138
|
+
unless content
|
139
|
+
logger.debug "Executing content command: #{configuration[:content_command]}"
|
140
|
+
content, status = Open3.capture2(configuration[:content_command])
|
147
141
|
|
148
|
-
|
149
|
-
|
150
|
-
|
151
|
-
changes = {
|
152
|
-
type: domain.record[:type],
|
153
|
-
name: domain.record[:name],
|
154
|
-
content: content
|
155
|
-
}
|
156
|
-
|
157
|
-
response = domain.put(changes.to_json, content_type: 'application/json')
|
158
|
-
|
159
|
-
if response.successful?
|
160
|
-
logger.info "Updated domain content to #{content}."
|
161
|
-
record[:content] = content
|
162
|
-
else
|
163
|
-
logger.warn "Failed to update domain content to #{content}: #{response.errors.join(', ')}!"
|
164
|
-
end
|
142
|
+
unless status.success?
|
143
|
+
raise RuntimeError.new("Content command failed with non-zero output: #{status}")
|
165
144
|
end
|
166
145
|
|
167
|
-
#
|
146
|
+
# Make sure there is no trailing space:
|
147
|
+
content.chomp!
|
148
|
+
|
168
149
|
configuration[:content] = content
|
169
|
-
else
|
170
|
-
logger.debug "Content hasn't changed."
|
171
150
|
end
|
172
151
|
|
173
|
-
|
152
|
+
unless zone = @connection.zones.find_by_id(configuration[:zone][:id])
|
153
|
+
raise RuntimeError.new("Couldn't load zone #{configuration[:zone].inspect} from API!")
|
154
|
+
end
|
155
|
+
|
156
|
+
configuration[:domains].each do |record|
|
157
|
+
update_domain(zone, record, content)
|
158
|
+
end
|
174
159
|
end
|
160
|
+
|
161
|
+
return content
|
175
162
|
end
|
176
163
|
|
177
|
-
def
|
164
|
+
def call
|
178
165
|
if @options[:version]
|
179
166
|
puts VERSION
|
180
167
|
elsif @options[:help]
|
181
|
-
print_usage
|
168
|
+
print_usage
|
182
169
|
else
|
183
|
-
|
184
|
-
|
185
|
-
|
186
|
-
|
187
|
-
|
188
|
-
|
189
|
-
|
170
|
+
Sync do
|
171
|
+
connect! do
|
172
|
+
initialize_zone
|
173
|
+
initialize_domains
|
174
|
+
initialize_command
|
175
|
+
|
176
|
+
update_domains
|
177
|
+
end
|
178
|
+
end
|
190
179
|
end
|
191
180
|
end
|
192
181
|
end
|
metadata
CHANGED
@@ -1,85 +1,99 @@
|
|
1
1
|
--- !ruby/object:Gem::Specification
|
2
2
|
name: cloudflare-dns-update
|
3
3
|
version: !ruby/object:Gem::Version
|
4
|
-
version:
|
4
|
+
version: 3.0.0
|
5
5
|
platform: ruby
|
6
6
|
authors:
|
7
7
|
- Samuel Williams
|
8
|
-
autorequire:
|
8
|
+
autorequire:
|
9
9
|
bindir: bin
|
10
10
|
cert_chain: []
|
11
|
-
date:
|
11
|
+
date: 2020-07-19 00:00:00.000000000 Z
|
12
12
|
dependencies:
|
13
13
|
- !ruby/object:Gem::Dependency
|
14
|
-
name:
|
14
|
+
name: cloudflare
|
15
15
|
requirement: !ruby/object:Gem::Requirement
|
16
16
|
requirements:
|
17
17
|
- - "~>"
|
18
18
|
- !ruby/object:Gem::Version
|
19
|
-
version: '
|
19
|
+
version: '4.0'
|
20
20
|
type: :runtime
|
21
21
|
prerelease: false
|
22
22
|
version_requirements: !ruby/object:Gem::Requirement
|
23
23
|
requirements:
|
24
24
|
- - "~>"
|
25
25
|
- !ruby/object:Gem::Version
|
26
|
-
version: '
|
26
|
+
version: '4.0'
|
27
27
|
- !ruby/object:Gem::Dependency
|
28
|
-
name:
|
28
|
+
name: samovar
|
29
29
|
requirement: !ruby/object:Gem::Requirement
|
30
30
|
requirements:
|
31
31
|
- - "~>"
|
32
32
|
- !ruby/object:Gem::Version
|
33
|
-
version:
|
33
|
+
version: '2.0'
|
34
34
|
type: :runtime
|
35
35
|
prerelease: false
|
36
36
|
version_requirements: !ruby/object:Gem::Requirement
|
37
37
|
requirements:
|
38
38
|
- - "~>"
|
39
39
|
- !ruby/object:Gem::Version
|
40
|
-
version:
|
40
|
+
version: '2.0'
|
41
41
|
- !ruby/object:Gem::Dependency
|
42
|
-
name:
|
42
|
+
name: tty-prompt
|
43
43
|
requirement: !ruby/object:Gem::Requirement
|
44
44
|
requirements:
|
45
45
|
- - "~>"
|
46
46
|
- !ruby/object:Gem::Version
|
47
|
-
version: '
|
47
|
+
version: '0.21'
|
48
48
|
type: :runtime
|
49
49
|
prerelease: false
|
50
50
|
version_requirements: !ruby/object:Gem::Requirement
|
51
51
|
requirements:
|
52
52
|
- - "~>"
|
53
53
|
- !ruby/object:Gem::Version
|
54
|
-
version: '
|
54
|
+
version: '0.21'
|
55
55
|
- !ruby/object:Gem::Dependency
|
56
|
-
name: rspec
|
56
|
+
name: async-rspec
|
57
57
|
requirement: !ruby/object:Gem::Requirement
|
58
58
|
requirements:
|
59
|
-
- - "
|
59
|
+
- - ">="
|
60
60
|
- !ruby/object:Gem::Version
|
61
|
-
version: '
|
61
|
+
version: '0'
|
62
62
|
type: :development
|
63
63
|
prerelease: false
|
64
64
|
version_requirements: !ruby/object:Gem::Requirement
|
65
65
|
requirements:
|
66
|
-
- - "
|
66
|
+
- - ">="
|
67
67
|
- !ruby/object:Gem::Version
|
68
|
-
version: '
|
68
|
+
version: '0'
|
69
69
|
- !ruby/object:Gem::Dependency
|
70
70
|
name: bundler
|
71
71
|
requirement: !ruby/object:Gem::Requirement
|
72
72
|
requirements:
|
73
|
-
- - "
|
73
|
+
- - ">="
|
74
74
|
- !ruby/object:Gem::Version
|
75
|
-
version: '
|
75
|
+
version: '0'
|
76
76
|
type: :development
|
77
77
|
prerelease: false
|
78
78
|
version_requirements: !ruby/object:Gem::Requirement
|
79
79
|
requirements:
|
80
|
-
- - "
|
80
|
+
- - ">="
|
81
81
|
- !ruby/object:Gem::Version
|
82
|
-
version: '
|
82
|
+
version: '0'
|
83
|
+
- !ruby/object:Gem::Dependency
|
84
|
+
name: covered
|
85
|
+
requirement: !ruby/object:Gem::Requirement
|
86
|
+
requirements:
|
87
|
+
- - ">="
|
88
|
+
- !ruby/object:Gem::Version
|
89
|
+
version: '0'
|
90
|
+
type: :development
|
91
|
+
prerelease: false
|
92
|
+
version_requirements: !ruby/object:Gem::Requirement
|
93
|
+
requirements:
|
94
|
+
- - ">="
|
95
|
+
- !ruby/object:Gem::Version
|
96
|
+
version: '0'
|
83
97
|
- !ruby/object:Gem::Dependency
|
84
98
|
name: rake
|
85
99
|
requirement: !ruby/object:Gem::Requirement
|
@@ -94,33 +108,37 @@ dependencies:
|
|
94
108
|
- - ">="
|
95
109
|
- !ruby/object:Gem::Version
|
96
110
|
version: '0'
|
97
|
-
|
98
|
-
|
111
|
+
- !ruby/object:Gem::Dependency
|
112
|
+
name: rspec
|
113
|
+
requirement: !ruby/object:Gem::Requirement
|
114
|
+
requirements:
|
115
|
+
- - "~>"
|
116
|
+
- !ruby/object:Gem::Version
|
117
|
+
version: '3.6'
|
118
|
+
type: :development
|
119
|
+
prerelease: false
|
120
|
+
version_requirements: !ruby/object:Gem::Requirement
|
121
|
+
requirements:
|
122
|
+
- - "~>"
|
123
|
+
- !ruby/object:Gem::Version
|
124
|
+
version: '3.6'
|
125
|
+
description:
|
99
126
|
email:
|
100
|
-
- samuel.williams@oriontransfer.co.nz
|
101
127
|
executables:
|
102
128
|
- cloudflare-dns-update
|
103
129
|
extensions: []
|
104
130
|
extra_rdoc_files: []
|
105
131
|
files:
|
106
|
-
- ".gitignore"
|
107
|
-
- ".rspec"
|
108
|
-
- ".travis.yml"
|
109
|
-
- Gemfile
|
110
|
-
- README.md
|
111
|
-
- Rakefile
|
112
132
|
- bin/cloudflare-dns-update
|
113
|
-
-
|
133
|
+
- lib/.DS_Store
|
114
134
|
- lib/cloudflare/dns/update.rb
|
115
135
|
- lib/cloudflare/dns/update/command.rb
|
116
136
|
- lib/cloudflare/dns/update/version.rb
|
117
|
-
|
118
|
-
- spec/spec_helper.rb
|
119
|
-
homepage: ''
|
137
|
+
homepage:
|
120
138
|
licenses:
|
121
139
|
- MIT
|
122
140
|
metadata: {}
|
123
|
-
post_install_message:
|
141
|
+
post_install_message:
|
124
142
|
rdoc_options: []
|
125
143
|
require_paths:
|
126
144
|
- lib
|
@@ -128,18 +146,15 @@ required_ruby_version: !ruby/object:Gem::Requirement
|
|
128
146
|
requirements:
|
129
147
|
- - ">="
|
130
148
|
- !ruby/object:Gem::Version
|
131
|
-
version: '
|
149
|
+
version: '2.5'
|
132
150
|
required_rubygems_version: !ruby/object:Gem::Requirement
|
133
151
|
requirements:
|
134
152
|
- - ">="
|
135
153
|
- !ruby/object:Gem::Version
|
136
154
|
version: '0'
|
137
155
|
requirements: []
|
138
|
-
|
139
|
-
|
140
|
-
signing_key:
|
156
|
+
rubygems_version: 3.1.2
|
157
|
+
signing_key:
|
141
158
|
specification_version: 4
|
142
159
|
summary: A dyndns client for Cloudflare.
|
143
|
-
test_files:
|
144
|
-
- spec/cloudflare/dns/update/command_spec.rb
|
145
|
-
- spec/spec_helper.rb
|
160
|
+
test_files: []
|
data/.gitignore
DELETED
data/.rspec
DELETED
data/.travis.yml
DELETED
@@ -1,19 +0,0 @@
|
|
1
|
-
language: ruby
|
2
|
-
sudo: false
|
3
|
-
dist: trusty
|
4
|
-
cache: bundler
|
5
|
-
rvm:
|
6
|
-
- 2.0
|
7
|
-
- 2.1
|
8
|
-
- 2.2
|
9
|
-
- 2.3
|
10
|
-
- 2.4
|
11
|
-
- jruby-head
|
12
|
-
- ruby-head
|
13
|
-
matrix:
|
14
|
-
allow_failures:
|
15
|
-
- rvm: ruby-head
|
16
|
-
- rvm: jruby-head
|
17
|
-
env:
|
18
|
-
global:
|
19
|
-
secure: LU7mGKnIrWrnrdtq7Ug7HTxzqJoB+NbLHwT8dPmGf4GIfNosO4Jx+G4JLzUd/sCHzUR+XXrsMd7El8WkTVV28+nt6Cv2JvO4EJRIplnAL8Yauc0YSWRwA9nGYhcc2TJc5czbL0mZkJSOv4B+aWco0VWerJfgLYurIA05XrQFGGY=
|
data/Gemfile
DELETED
data/README.md
DELETED
@@ -1,78 +0,0 @@
|
|
1
|
-
# Cloudflare::DNS::Update
|
2
|
-
|
3
|
-
This gem provides a simple executable tool for managing Cloudflare records to provide dynamic DNS like functionality. You need to add it to whatever `cron` system your host system uses.
|
4
|
-
|
5
|
-
[](http://travis-ci.org/ioquatix/cloudflare-dns-update)
|
6
|
-
|
7
|
-
[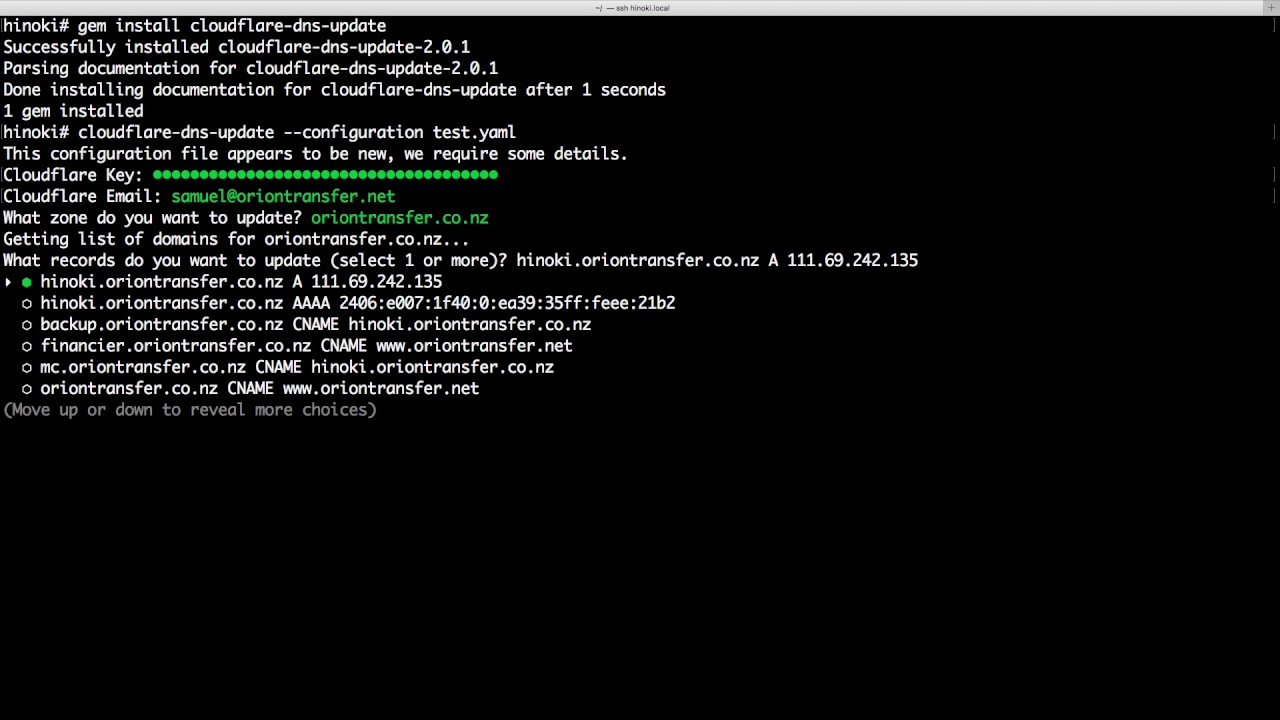](https://www.youtube.com/watch?v=lQK6bWuQllM&feature=youtu.be&hd=1 "Cloudflare DNS Update Introduction")
|
8
|
-
|
9
|
-
## Installation
|
10
|
-
|
11
|
-
Install it yourself as:
|
12
|
-
|
13
|
-
$ gem install cloudflare-dns-update
|
14
|
-
|
15
|
-
## Usage
|
16
|
-
|
17
|
-
Run the included `cloudflare-dns-update` tool and you will be walked through the configuration process. You might want to specify a specific configuration file, using the `--configuration /path/to/configuration.yml` option.
|
18
|
-
|
19
|
-
### Daily updates using CRON
|
20
|
-
|
21
|
-
Simply set up configurations for each domain you wish to update, and add to `/etc/cron.daily/dyndns`, e.g.:
|
22
|
-
|
23
|
-
#!/usr/bin/env sh
|
24
|
-
|
25
|
-
cloudflare-dns-update --configuration /srv/dyndns/example.A.yml
|
26
|
-
cloudflare-dns-update --configuration /srv/dyndns/example.AAAA.yml
|
27
|
-
|
28
|
-
Note that in case you want to update more than one domains in a zone with the same IP address, you can have multiple domains in a configuration file. Follow instructions of the configuration process. Just to note, each domain would be updated with the same content. Having both IPv4 and IPv6 records in the same configuration file is not possible nor recommended. Please create separate configuration files.
|
29
|
-
|
30
|
-
The configuration file would end up looking something like this:
|
31
|
-
|
32
|
-
---
|
33
|
-
:key: b10_NOT_A_REAL_KEY_fe5
|
34
|
-
:email: cloudflare-account@example.com
|
35
|
-
:zone: example.com
|
36
|
-
:content_command: curl ipinfo.io/ip
|
37
|
-
|
38
|
-
### IPv6 Support
|
39
|
-
|
40
|
-
It is possible to update IPv6 when you have a dynamically allocated prefix. To get your current IPv6 address, the following command can be used:
|
41
|
-
|
42
|
-
/sbin/ip -6 addr | awk -F '[ \t]+|/' '$3 == "::1" { next;} $3 ~ /^fe80::/ { next ; } /inet6/ {print $3}'
|
43
|
-
|
44
|
-
## Contributing
|
45
|
-
|
46
|
-
1. Fork it
|
47
|
-
2. Create your feature branch (`git checkout -b my-new-feature`)
|
48
|
-
3. Commit your changes (`git commit -am 'Add some feature'`)
|
49
|
-
4. Push to the branch (`git push origin my-new-feature`)
|
50
|
-
5. Create new Pull Request
|
51
|
-
|
52
|
-
## See Also
|
53
|
-
|
54
|
-
- [cloudflare](https://github.com/ioquatix/cloudflare) – Provides access to Cloudflare.
|
55
|
-
|
56
|
-
## License
|
57
|
-
|
58
|
-
Released under the MIT license.
|
59
|
-
|
60
|
-
Copyright, 2017, by [Samuel G. D. Williams](http://www.codeotaku.com/samuel-williams).
|
61
|
-
|
62
|
-
Permission is hereby granted, free of charge, to any person obtaining a copy
|
63
|
-
of this software and associated documentation files (the "Software"), to deal
|
64
|
-
in the Software without restriction, including without limitation the rights
|
65
|
-
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
66
|
-
copies of the Software, and to permit persons to whom the Software is
|
67
|
-
furnished to do so, subject to the following conditions:
|
68
|
-
|
69
|
-
The above copyright notice and this permission notice shall be included in
|
70
|
-
all copies or substantial portions of the Software.
|
71
|
-
|
72
|
-
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
73
|
-
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
74
|
-
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
75
|
-
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
76
|
-
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
77
|
-
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
|
78
|
-
THE SOFTWARE.
|
data/Rakefile
DELETED
@@ -1,32 +0,0 @@
|
|
1
|
-
# coding: utf-8
|
2
|
-
lib = File.expand_path('../lib', __FILE__)
|
3
|
-
$LOAD_PATH.unshift(lib) unless $LOAD_PATH.include?(lib)
|
4
|
-
require 'cloudflare/dns/update/version'
|
5
|
-
|
6
|
-
Gem::Specification.new do |spec|
|
7
|
-
spec.name = "cloudflare-dns-update"
|
8
|
-
spec.version = Cloudflare::DNS::Update::VERSION
|
9
|
-
spec.authors = ["Samuel Williams"]
|
10
|
-
spec.email = ["samuel.williams@oriontransfer.co.nz"]
|
11
|
-
spec.description = <<-EOF
|
12
|
-
Provides a client tool for updating Cloudflare records, with a specific
|
13
|
-
emphasis on updating IP addresses for domain records. This provides
|
14
|
-
dyndns-like functionality.
|
15
|
-
EOF
|
16
|
-
spec.summary = "A dyndns client for Cloudflare."
|
17
|
-
spec.homepage = ""
|
18
|
-
spec.license = "MIT"
|
19
|
-
|
20
|
-
spec.files = `git ls-files`.split($/)
|
21
|
-
spec.executables = spec.files.grep(%r{^bin/}) { |f| File.basename(f) }
|
22
|
-
spec.test_files = spec.files.grep(%r{^(test|spec|features)/})
|
23
|
-
spec.require_paths = ["lib"]
|
24
|
-
|
25
|
-
spec.add_dependency "samovar", "~> 1.3"
|
26
|
-
spec.add_dependency "tty-prompt", "~> 0.12.0"
|
27
|
-
spec.add_dependency "cloudflare", "~> 3.0"
|
28
|
-
|
29
|
-
spec.add_development_dependency "rspec", "~> 3.6"
|
30
|
-
spec.add_development_dependency "bundler", "~> 1.3"
|
31
|
-
spec.add_development_dependency "rake"
|
32
|
-
end
|
@@ -1,48 +0,0 @@
|
|
1
|
-
|
2
|
-
require 'cloudflare/dns/update/command'
|
3
|
-
|
4
|
-
RSpec.describe Cloudflare::DNS::Update::Command::Top, order: :defined do
|
5
|
-
include_context Cloudflare::RSpec::Connection
|
6
|
-
|
7
|
-
let(:configuration_path) {File.join(__dir__, 'test.yaml')}
|
8
|
-
|
9
|
-
subject{described_class.new(["-c", configuration_path])}
|
10
|
-
|
11
|
-
let(:zone) {connection.zones.all.first}
|
12
|
-
let!(:name) {"dyndns#{ENV['TRAVIS_JOB_ID']}"}
|
13
|
-
let!(:qualified_name) {"#{name}.#{zone.record[:name]}"}
|
14
|
-
|
15
|
-
it "should create dns record" do
|
16
|
-
response = zone.dns_records.post({
|
17
|
-
type: "A",
|
18
|
-
name: name,
|
19
|
-
content: "127.0.0.1",
|
20
|
-
ttl: 240,
|
21
|
-
proxied: false
|
22
|
-
}.to_json, content_type: 'application/json')
|
23
|
-
|
24
|
-
expect(response).to be_successful
|
25
|
-
end
|
26
|
-
|
27
|
-
let(:dns_record) {zone.dns_records.find_by_name(qualified_name)}
|
28
|
-
|
29
|
-
it "should update dns record" do
|
30
|
-
subject.connection = connection
|
31
|
-
|
32
|
-
subject.configuration_store.transaction do |configuration|
|
33
|
-
configuration[:content_command] = 'curl -s ipinfo.io/ip'
|
34
|
-
configuration[:zone] = zone.record
|
35
|
-
configuration[:domains] = [dns_record.record]
|
36
|
-
end
|
37
|
-
|
38
|
-
content = subject.update_domains
|
39
|
-
|
40
|
-
response = dns_record.get
|
41
|
-
|
42
|
-
expect(response.result[:content]).to be == content
|
43
|
-
end
|
44
|
-
|
45
|
-
it "should delete dns record" do
|
46
|
-
expect(dns_record.delete).to be_successful
|
47
|
-
end
|
48
|
-
end
|
data/spec/spec_helper.rb
DELETED
@@ -1,29 +0,0 @@
|
|
1
|
-
|
2
|
-
if ENV['COVERAGE'] || ENV['TRAVIS']
|
3
|
-
begin
|
4
|
-
require 'simplecov'
|
5
|
-
|
6
|
-
SimpleCov.start do
|
7
|
-
add_filter "/spec/"
|
8
|
-
end
|
9
|
-
|
10
|
-
if ENV['TRAVIS']
|
11
|
-
require 'coveralls'
|
12
|
-
Coveralls.wear!
|
13
|
-
end
|
14
|
-
rescue LoadError
|
15
|
-
warn "Could not load simplecov: #{$!}"
|
16
|
-
end
|
17
|
-
end
|
18
|
-
|
19
|
-
require "bundler/setup"
|
20
|
-
require "cloudflare/rspec/connection"
|
21
|
-
|
22
|
-
RSpec.configure do |config|
|
23
|
-
# Enable flags like --only-failures and --next-failure
|
24
|
-
config.example_status_persistence_file_path = ".rspec_status"
|
25
|
-
|
26
|
-
config.expect_with :rspec do |c|
|
27
|
-
c.syntax = :expect
|
28
|
-
end
|
29
|
-
end
|