clearance 1.8.0 → 1.8.1
This diff represents the content of publicly available package versions that have been released to one of the supported registries. The information contained in this diff is provided for informational purposes only and reflects changes between package versions as they appear in their respective public registries.
Potentially problematic release.
This version of clearance might be problematic. Click here for more details.
- checksums.yaml +4 -4
- data/.gitignore +2 -0
- data/.yardopts +3 -0
- data/Gemfile +1 -1
- data/Gemfile.lock +25 -24
- data/NEWS.md +586 -311
- data/README.md +143 -334
- data/app/controllers/clearance/sessions_controller.rb +0 -1
- data/gemfiles/rails4.0.gemfile +1 -1
- data/gemfiles/rails4.1.gemfile +1 -1
- data/gemfiles/rails4.2.gemfile +1 -1
- data/lib/clearance.rb +2 -0
- data/lib/clearance/authentication.rb +2 -0
- data/lib/clearance/configuration.rb +106 -14
- data/lib/clearance/version.rb +1 -1
- data/spec/app_templates/testapp/config/initializers/action_mailer.rb +1 -3
- data/spec/clearance/contoller_spec.rb +11 -0
- data/spec/clearance/controller_spec.rb +11 -0
- data/spec/clearance/rack_session_spec.rb +5 -5
- data/spec/controllers/sessions_controller_spec.rb +2 -2
- metadata +5 -2
data/README.md
CHANGED
@@ -1,14 +1,12 @@
|
|
1
|
-
Clearance
|
2
|
-
=========
|
1
|
+
# Clearance
|
3
2
|
|
4
3
|
[](http://travis-ci.org/thoughtbot/clearance?branch=master)
|
5
4
|
[](https://codeclimate.com/github/thoughtbot/clearance)
|
6
5
|
|
7
6
|
Rails authentication with email & password.
|
8
7
|
|
9
|
-
Clearance
|
10
|
-
|
11
|
-
defaults.
|
8
|
+
Clearance is intended to be small, simple, and well-tested. It has opinionated
|
9
|
+
defaults but is intended to be easy to override.
|
12
10
|
|
13
11
|
Please use [GitHub Issues] to report bugs. If you have a question about the
|
14
12
|
library, please use the `clearance` tag on [Stack Overflow]. This tag is
|
@@ -17,45 +15,25 @@ monitored by contributors.
|
|
17
15
|
[GitHub Issues]: https://github.com/thoughtbot/clearance/issues
|
18
16
|
[Stack Overflow]: http://stackoverflow.com/questions/tagged/clearance
|
19
17
|
|
20
|
-
|
21
|
-
|
22
|
-
Install
|
23
|
-
-------
|
18
|
+
## Install
|
24
19
|
|
25
20
|
Clearance is a Rails engine tested against Rails `>= 3.2` and Ruby `>= 1.9.3`.
|
26
|
-
|
27
|
-
|
28
|
-
Include the gem in your Gemfile:
|
21
|
+
To get started, add Clearance to your `Gemfile`, `bundle install`, and run the
|
22
|
+
`install generator`:
|
29
23
|
|
30
|
-
```
|
31
|
-
|
24
|
+
```sh
|
25
|
+
$ rails generate clearance:install
|
32
26
|
```
|
33
27
|
|
34
|
-
Bundle:
|
35
|
-
|
36
|
-
bundle install
|
37
|
-
|
38
|
-
Make sure the development database exists. Then, run the generator:
|
39
|
-
|
40
|
-
rails generate clearance:install
|
41
|
-
|
42
28
|
The generator:
|
43
29
|
|
44
|
-
*
|
45
|
-
*
|
46
|
-
*
|
47
|
-
|
48
|
-
|
49
|
-
Then, follow the instructions output from the generator.
|
50
|
-
|
51
|
-
Use Clearance [0.8.8](https://github.com/thoughtbot/clearance/tree/v0.8.8) for
|
52
|
-
Rails 2 apps.
|
53
|
-
|
54
|
-
Use Clearance [0.16.3](http://rubygems.org/gems/clearance/versions/0.16.3) for
|
55
|
-
Ruby 1.8.7 apps.
|
30
|
+
* Inserts `Clearance::User` into your `User` model
|
31
|
+
* Inserts `Clearance::Controller` into your `ApplicationController`
|
32
|
+
* Creates an initializer to allow further configuration.
|
33
|
+
* Creates a migration that either creates a users table or adds any necessary
|
34
|
+
columns to the existing table.
|
56
35
|
|
57
|
-
Configure
|
58
|
-
---------
|
36
|
+
## Configure
|
59
37
|
|
60
38
|
Override any of these defaults in `config/initializers/clearance.rb`:
|
61
39
|
|
@@ -77,40 +55,15 @@ Clearance.configure do |config|
|
|
77
55
|
end
|
78
56
|
```
|
79
57
|
|
80
|
-
Use
|
81
|
-
---
|
58
|
+
## Use
|
82
59
|
|
83
|
-
|
84
|
-
helpers. For example:
|
85
|
-
|
86
|
-
```haml
|
87
|
-
- if signed_in?
|
88
|
-
= current_user.email
|
89
|
-
= button_to 'Sign out', sign_out_path, method: :delete
|
90
|
-
- else
|
91
|
-
= link_to 'Sign in', sign_in_path
|
92
|
-
```
|
93
|
-
|
94
|
-
To authenticate a user elsewhere than `sessions/new` (like in an API):
|
60
|
+
### Access Control
|
95
61
|
|
96
|
-
|
97
|
-
User.authenticate 'email@example.com', 'password'
|
98
|
-
```
|
99
|
-
|
100
|
-
When a user resets their password, Clearance delivers them an email. So, you
|
101
|
-
should change the `mailer_sender` default, used in the email's "from" header:
|
102
|
-
|
103
|
-
```ruby
|
104
|
-
Clearance.configure do |config|
|
105
|
-
config.mailer_sender = 'reply@example.com'
|
106
|
-
end
|
107
|
-
```
|
108
|
-
|
109
|
-
Use `require_login` to control access in controllers:
|
62
|
+
Use the `require_login` filter to control access to controller actions.
|
110
63
|
|
111
64
|
```ruby
|
112
65
|
class ArticlesController < ApplicationController
|
113
|
-
|
66
|
+
before_action :require_login
|
114
67
|
|
115
68
|
def index
|
116
69
|
current_user.articles
|
@@ -118,7 +71,8 @@ class ArticlesController < ApplicationController
|
|
118
71
|
end
|
119
72
|
```
|
120
73
|
|
121
|
-
|
74
|
+
Clearance also provides routing constraints that can be used to control access
|
75
|
+
at the routing layer:
|
122
76
|
|
123
77
|
```ruby
|
124
78
|
Blog::Application.routes.draw do
|
@@ -136,6 +90,33 @@ Blog::Application.routes.draw do
|
|
136
90
|
end
|
137
91
|
```
|
138
92
|
|
93
|
+
### Helper Methods
|
94
|
+
|
95
|
+
Use `current_user`, `signed_in?`, and `signed_out?` in controllers, views, and
|
96
|
+
helpers. For example:
|
97
|
+
|
98
|
+
```erb
|
99
|
+
<% if signed_in? %>
|
100
|
+
<%= current_user.email %>
|
101
|
+
<%= button_to "Sign out", sign_out_path, method: :delete %>
|
102
|
+
<% else %>
|
103
|
+
<%= link_to "Sign in", sign_in_path %>
|
104
|
+
<% end %>
|
105
|
+
```
|
106
|
+
|
107
|
+
### Password Resets
|
108
|
+
|
109
|
+
When a user resets their password, Clearance delivers them an email. You
|
110
|
+
should change the `mailer_sender` default, used in the email's "from" header:
|
111
|
+
|
112
|
+
```ruby
|
113
|
+
Clearance.configure do |config|
|
114
|
+
config.mailer_sender = 'reply@example.com'
|
115
|
+
end
|
116
|
+
```
|
117
|
+
|
118
|
+
### Integrating with Rack Applications
|
119
|
+
|
139
120
|
Clearance adds its session to the Rack environment hash so middleware and other
|
140
121
|
Rack applications can interact with it:
|
141
122
|
|
@@ -155,26 +136,24 @@ class Bubblegum::Middleware
|
|
155
136
|
end
|
156
137
|
```
|
157
138
|
|
158
|
-
Overriding
|
159
|
-
|
139
|
+
## Overriding Clearance
|
140
|
+
|
141
|
+
### Routes
|
160
142
|
|
161
143
|
See [config/routes.rb](/config/routes.rb) for the default set of routes.
|
162
144
|
|
163
|
-
|
164
|
-
|
165
|
-
all clearance routes, giving the user full control over routing and URL design.
|
145
|
+
As of Clearance 1.5 it is recommended that you disable Clearance routes and take
|
146
|
+
full control over routing and URL design.
|
166
147
|
|
167
148
|
To disable the routes, set `config.routes = false`. You can optionally run
|
168
149
|
`rails generate clearance:routes` to dump a copy of the default routes into your
|
169
150
|
application for modification.
|
170
151
|
|
171
|
-
|
172
|
-
|
173
|
-
Overriding controllers
|
174
|
-
----------------------
|
152
|
+
### Controllers
|
175
153
|
|
176
154
|
See [app/controllers/clearance](/app/controllers/clearance) for the default
|
177
|
-
behavior.
|
155
|
+
behavior. Many protected methods were extracted in these controllers in an
|
156
|
+
attempt to make overrides and hooks simpler.
|
178
157
|
|
179
158
|
To override a Clearance controller, subclass it:
|
180
159
|
|
@@ -184,203 +163,79 @@ class SessionsController < Clearance::SessionsController
|
|
184
163
|
class UsersController < Clearance::UsersController
|
185
164
|
```
|
186
165
|
|
187
|
-
|
188
|
-
|
189
|
-
Then, override public methods:
|
190
|
-
|
191
|
-
passwords#create
|
192
|
-
passwords#edit
|
193
|
-
passwords#new
|
194
|
-
passwords#update
|
195
|
-
sessions#create
|
196
|
-
sessions#destroy
|
197
|
-
sessions#new
|
198
|
-
users#create
|
199
|
-
users#new
|
200
|
-
|
201
|
-
Or, override private methods:
|
202
|
-
|
203
|
-
passwords#find_user_by_id_and_confirmation_token
|
204
|
-
passwords#find_user_for_create
|
205
|
-
passwords#find_user_for_edit
|
206
|
-
passwords#find_user_for_update
|
207
|
-
passwords#flash_failure_after_create
|
208
|
-
passwords#flash_failure_after_update
|
209
|
-
passwords#flash_failure_when_forbidden
|
210
|
-
passwords#forbid_missing_token
|
211
|
-
passwords#forbid_non_existent_user
|
212
|
-
passwords#url_after_create
|
213
|
-
passwords#url_after_update
|
214
|
-
sessions#url_after_create
|
215
|
-
sessions#url_after_destroy
|
216
|
-
users#flash_failure_after_create
|
217
|
-
users#url_after_create
|
218
|
-
users#user_from_params
|
219
|
-
users#user_params
|
220
|
-
|
166
|
+
### Redirects
|
221
167
|
All of these controller methods redirect to `'/'` by default:
|
222
168
|
|
223
|
-
passwords#url_after_update
|
224
|
-
sessions#url_after_create
|
225
|
-
sessions#url_for_signed_in_users
|
226
|
-
users#url_after_create
|
227
|
-
application#url_after_denied_access_when_signed_in
|
228
|
-
|
229
|
-
To override them all at once, change the global configuration:
|
230
|
-
|
231
|
-
```ruby
|
232
|
-
Clearance.configure do |config|
|
233
|
-
config.redirect_url = '/overridden'
|
234
|
-
end
|
235
169
|
```
|
236
|
-
|
237
|
-
|
238
|
-
|
239
|
-
|
240
|
-
|
241
|
-
[i18n translations](http://guides.rubyonrails.org/i18n.html).
|
242
|
-
Override them like any other translation.
|
243
|
-
|
244
|
-
See [config/locales/clearance.en.yml](/config/locales/clearance.en.yml) for the
|
245
|
-
default behavior.
|
246
|
-
|
247
|
-
Overriding layouts
|
248
|
-
----------------
|
249
|
-
|
250
|
-
By default, Clearance uses your application's default layout. If you would like
|
251
|
-
to change the layout that Clearance uses when rendering its views, simply specify
|
252
|
-
the layout in an initializer.
|
253
|
-
|
254
|
-
```ruby
|
255
|
-
Clearance::PasswordsController.layout 'my_passwords_layout'
|
256
|
-
Clearance::SessionsController.layout 'my_sessions_layout'
|
257
|
-
Clearance::UsersController.layout 'my_admin_layout'
|
170
|
+
passwords#url_after_update
|
171
|
+
sessions#url_after_create
|
172
|
+
sessions#url_for_signed_in_users
|
173
|
+
users#url_after_create
|
174
|
+
application#url_after_denied_access_when_signed_in
|
258
175
|
```
|
259
176
|
|
260
|
-
|
261
|
-
----------------
|
262
|
-
|
263
|
-
See [app/views](/app/views) for the default behavior.
|
264
|
-
|
265
|
-
To override a view, create your own:
|
266
|
-
|
267
|
-
app/views/clearance_mailer/change_password.html.erb
|
268
|
-
app/views/passwords/create.html.erb
|
269
|
-
app/views/passwords/edit.html.erb
|
270
|
-
app/views/passwords/new.html.erb
|
271
|
-
app/views/sessions/_form.html.erb
|
272
|
-
app/views/sessions/new.html.erb
|
273
|
-
app/views/users/_form.html.erb
|
274
|
-
app/views/users/new.html.erb
|
275
|
-
|
276
|
-
There is a shortcut to copy all Clearance views into your app:
|
277
|
-
|
278
|
-
rails generate clearance:views
|
177
|
+
To override them all at once, change the global configuration of `redirect_url`.
|
279
178
|
|
280
|
-
|
281
|
-
--------------------
|
179
|
+
### Views
|
282
180
|
|
283
|
-
See [
|
284
|
-
|
285
|
-
To override the model, redefine public methods:
|
286
|
-
|
287
|
-
User.authenticate(email, password)
|
288
|
-
User#forgot_password!
|
289
|
-
User#reset_remember_token!
|
290
|
-
User#update_password(new_password)
|
291
|
-
|
292
|
-
Or, redefine private methods:
|
293
|
-
|
294
|
-
User#email_optional?
|
295
|
-
User#generate_confirmation_token
|
296
|
-
User#generate_remember_token
|
297
|
-
User#normalize_email
|
298
|
-
User#password_optional?
|
299
|
-
|
300
|
-
Overriding the password strategy
|
301
|
-
--------------------------------
|
302
|
-
|
303
|
-
By default, Clearance uses BCrypt encryption of the user's password.
|
304
|
-
|
305
|
-
See
|
306
|
-
[lib/clearance/password_strategies/bcrypt.rb](/lib/clearance/password_strategies/bcrypt.rb)
|
307
|
-
for the default behavior.
|
181
|
+
See [app/views](/app/views) for the default behavior.
|
308
182
|
|
309
|
-
|
183
|
+
To override a view, create your own copy of it:
|
310
184
|
|
311
|
-
```ruby
|
312
|
-
Clearance.configure do |config|
|
313
|
-
config.password_strategy = Clearance::PasswordStrategies::SHA1
|
314
|
-
end
|
315
185
|
```
|
316
|
-
|
317
|
-
|
318
|
-
|
319
|
-
|
320
|
-
|
321
|
-
|
322
|
-
|
323
|
-
|
186
|
+
app/views/clearance_mailer/change_password.html.erb
|
187
|
+
app/views/passwords/create.html.erb
|
188
|
+
app/views/passwords/edit.html.erb
|
189
|
+
app/views/passwords/new.html.erb
|
190
|
+
app/views/sessions/_form.html.erb
|
191
|
+
app/views/sessions/new.html.erb
|
192
|
+
app/views/users/_form.html.erb
|
193
|
+
app/views/users/new.html.erb
|
324
194
|
```
|
325
195
|
|
326
|
-
|
327
|
-
|
328
|
-
Switching password strategies may cause your existing users to not be able to sign in.
|
196
|
+
You can use the Clearance views generator to copy the default views to your
|
197
|
+
application for modification.
|
329
198
|
|
330
|
-
|
331
|
-
|
332
|
-
|
199
|
+
```shell
|
200
|
+
$ rails generate clearance:views
|
201
|
+
```
|
333
202
|
|
334
|
-
|
335
|
-
switch to BCrypt transparently, use
|
336
|
-
[Clearance::PasswordStrategies::BCryptMigrationFromSHA1](/lib/clearance/password_strategies/bcrypt_migration_from_sha1.rb).
|
203
|
+
### Layouts
|
337
204
|
|
338
|
-
|
339
|
-
|
205
|
+
By default, Clearance uses your application's default layout. If you would like
|
206
|
+
to change the layout that Clearance uses when rendering its views, simply
|
207
|
+
specify the layout in an initializer.
|
340
208
|
|
341
209
|
```ruby
|
342
|
-
|
343
|
-
|
344
|
-
|
345
|
-
end
|
346
|
-
end
|
210
|
+
Clearance::PasswordsController.layout 'my_passwords_layout'
|
211
|
+
Clearance::SessionsController.layout 'my_sessions_layout'
|
212
|
+
Clearance::UsersController.layout 'my_admin_layout'
|
347
213
|
```
|
348
214
|
|
349
|
-
|
350
|
-
In your `authenticated?` method, encrypt the password with your desired
|
351
|
-
strategy, and then compare it to the `encrypted_password` that is provided by
|
352
|
-
Clearance.
|
215
|
+
### Translations
|
353
216
|
|
354
|
-
|
355
|
-
|
356
|
-
|
357
|
-
encrypted_password == encrypt(password)
|
358
|
-
end
|
217
|
+
All flash messages and email subject lines are stored in [i18n translations]
|
218
|
+
(http://guides.rubyonrails.org/i18n.html). Override them like any other
|
219
|
+
translation.
|
359
220
|
|
360
|
-
|
361
|
-
|
221
|
+
See [config/locales/clearance.en.yml](/config/locales/clearance.en.yml) for the
|
222
|
+
default behavior.
|
362
223
|
|
363
|
-
private
|
364
224
|
|
365
|
-
|
366
|
-
# your encryption strategy
|
367
|
-
end
|
368
|
-
end
|
225
|
+
### User Model
|
369
226
|
|
370
|
-
|
371
|
-
|
372
|
-
end
|
373
|
-
```
|
227
|
+
See [lib/clearance/user.rb](/lib/clearance/user.rb) for the default behavior.
|
228
|
+
You can override those methods as needed.
|
374
229
|
|
375
|
-
Deliver
|
376
|
-
------------------------------------------------
|
230
|
+
### Deliver Email in Background Job
|
377
231
|
|
378
|
-
Clearance has
|
232
|
+
Clearance has a password reset mailer. If you are using Rails 4.2 and Clearance
|
233
|
+
1.6 or greater, Clearance will use ActiveJob's `deliver_later` method to
|
234
|
+
automatically take advantage of your configured queue.
|
379
235
|
|
380
|
-
|
381
|
-
|
382
|
-
`
|
383
|
-
`deliver_email` method:
|
236
|
+
If you are using an earlier version of Rails, you can override the
|
237
|
+
`Clearance::Passwords` controller and define the behavior you need in the
|
238
|
+
`deliver_email` method.
|
384
239
|
|
385
240
|
```ruby
|
386
241
|
class PasswordsController < Clearance::PasswordsController
|
@@ -390,14 +245,13 @@ class PasswordsController < Clearance::PasswordsController
|
|
390
245
|
end
|
391
246
|
```
|
392
247
|
|
393
|
-
|
248
|
+
## Extending Sign In
|
394
249
|
|
395
|
-
|
396
|
-
|
397
|
-
|
398
|
-
|
399
|
-
|
400
|
-
-------------------
|
250
|
+
By default, Clearance will sign in any user with valid credentials. If you need
|
251
|
+
to support additional checks during the sign in process then you can use the
|
252
|
+
SignInGuard stack. For example, using the SignInGuard stack, you could prevent
|
253
|
+
suspended users from signing in, or require that users confirm their email
|
254
|
+
address before accessing the site.
|
401
255
|
|
402
256
|
`SignInGuard`s offer fine-grained control over the process of
|
403
257
|
signing in a user. Each guard is run in order and hands the session off to
|
@@ -441,39 +295,52 @@ class EmailConfirmationGuard < Clearance::SignInGuard
|
|
441
295
|
end
|
442
296
|
```
|
443
297
|
|
444
|
-
|
445
|
-
----------------------
|
298
|
+
## Testing
|
446
299
|
|
447
|
-
|
448
|
-
integration with your Rails app over time.
|
300
|
+
### Fast Feature Specs
|
449
301
|
|
450
|
-
|
302
|
+
Clearance includes middleware that avoids wasting time spent visiting, loading,
|
303
|
+
and submitting the sign in form. It instead signs in the designated user
|
304
|
+
directly. The speed increase can be [substantial][backdoor].
|
305
|
+
|
306
|
+
[backdoor]: http://robots.thoughtbot.com/post/37907699673/faster-tests-sign-in-through-the-back-door
|
307
|
+
|
308
|
+
Enable the Middleware in Test:
|
451
309
|
|
452
310
|
```ruby
|
453
|
-
|
454
|
-
|
455
|
-
|
311
|
+
# config/environments/test.rb
|
312
|
+
MyRailsApp::Application.configure do
|
313
|
+
# ...
|
314
|
+
config.middleware.use Clearance::BackDoor
|
315
|
+
# ...
|
316
|
+
end
|
456
317
|
```
|
457
318
|
|
458
|
-
|
319
|
+
Usage:
|
459
320
|
|
460
|
-
|
321
|
+
```ruby
|
322
|
+
visit root_path(as: user)
|
323
|
+
```
|
461
324
|
|
462
|
-
|
325
|
+
### Ready Made Feature Specs
|
463
326
|
|
464
|
-
|
327
|
+
If you're using RSpec, you can generate feature specs to help prevent
|
328
|
+
regressions in Clearance's integration with your Rails app over time. These
|
329
|
+
feature specs, will also require `factory_girl_rails`.
|
465
330
|
|
466
|
-
|
331
|
+
To Generate the clearance specs, run:
|
467
332
|
|
468
|
-
|
333
|
+
```shell
|
334
|
+
$ rails generate clearance:specs
|
335
|
+
```
|
469
336
|
|
470
|
-
|
471
|
-
---------------------------------------------
|
337
|
+
### Controller Test Helpers
|
472
338
|
|
473
339
|
To test controller actions that are protected by `before_filter :require_login`,
|
474
|
-
require Clearance's test helpers
|
340
|
+
require Clearance's test helpers in your test suite.
|
475
341
|
|
476
|
-
For `rspec`, add
|
342
|
+
For `rspec`, add the following line to your `spec/rails_helper.rb` or
|
343
|
+
`spec/spec_helper` if `rails_helper` does not exist:
|
477
344
|
|
478
345
|
```ruby
|
479
346
|
require 'clearance/rspec'
|
@@ -488,70 +355,13 @@ require 'clearance/test_unit'
|
|
488
355
|
This will make `Clearance::Controller` methods work in your controllers
|
489
356
|
during functional tests and provide access to helper methods like:
|
490
357
|
|
491
|
-
sign_in
|
492
|
-
sign_in_as(user)
|
493
|
-
sign_out
|
494
|
-
|
495
|
-
And matchers like:
|
496
|
-
|
497
|
-
deny_access
|
498
|
-
|
499
|
-
Example:
|
500
|
-
|
501
|
-
```ruby
|
502
|
-
context 'a guest' do
|
503
|
-
before do
|
504
|
-
get :show
|
505
|
-
end
|
506
|
-
|
507
|
-
it { should deny_access }
|
508
|
-
end
|
509
|
-
|
510
|
-
context 'a user' do
|
511
|
-
before do
|
512
|
-
sign_in
|
513
|
-
get :show
|
514
|
-
end
|
515
|
-
|
516
|
-
it { should respond_with(:success) }
|
517
|
-
end
|
518
|
-
```
|
519
|
-
|
520
|
-
You may want to customize the tests:
|
521
|
-
|
522
358
|
```ruby
|
523
|
-
|
524
|
-
|
525
|
-
|
526
|
-
```
|
527
|
-
|
528
|
-
Faster tests
|
529
|
-
------------
|
530
|
-
|
531
|
-
Clearance includes middleware that avoids wasting time spent visiting, loading,
|
532
|
-
and submitting the sign in form. It instead signs in the designated
|
533
|
-
user directly. The speed increase can be
|
534
|
-
[substantial](http://robots.thoughtbot.com/post/37907699673/faster-tests-sign-in-through-the-back-door).
|
535
|
-
|
536
|
-
Configuration:
|
537
|
-
|
538
|
-
```ruby
|
539
|
-
# config/environments/test.rb
|
540
|
-
MyRailsApp::Application.configure do
|
541
|
-
# ...
|
542
|
-
config.middleware.use Clearance::BackDoor
|
543
|
-
# ...
|
544
|
-
end
|
545
|
-
```
|
546
|
-
|
547
|
-
Usage:
|
548
|
-
|
549
|
-
```ruby
|
550
|
-
visit root_path(as: user)
|
359
|
+
sign_in
|
360
|
+
sign_in_as(user)
|
361
|
+
sign_out
|
551
362
|
```
|
552
363
|
|
553
|
-
Credits
|
554
|
-
-------
|
364
|
+
## Credits
|
555
365
|
|
556
366
|
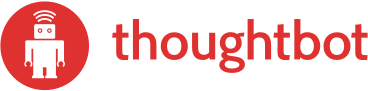
|
557
367
|
|
@@ -559,8 +369,7 @@ Clearance is maintained by [thoughtbot, inc](http://thoughtbot.com/community)
|
|
559
369
|
and [contributors](https://github.com/thoughtbot/clearance/contributors) like
|
560
370
|
you. Thank you!
|
561
371
|
|
562
|
-
License
|
563
|
-
-------
|
372
|
+
## License
|
564
373
|
|
565
374
|
Clearance is copyright © 2009 thoughtbot. It is free software, and may be
|
566
375
|
redistributed under the terms specified in the `LICENSE` file.
|