bar-of-progress 0.1.0 → 0.1.1
Sign up to get free protection for your applications and to get access to all the features.
- checksums.yaml +4 -4
- data/.travis.yml +6 -0
- data/README.md +7 -0
- data/lib/bar-of-progress.rb +53 -7
- data/lib/bar_of_progress/version.rb +1 -1
- data/spec/lib/bar_of_progress_spec.rb +2 -0
- metadata +2 -1
checksums.yaml
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
---
|
2
2
|
SHA1:
|
3
|
-
metadata.gz:
|
4
|
-
data.tar.gz:
|
3
|
+
metadata.gz: e4e60372a78682438217bb7b7568a84de5f2e24d
|
4
|
+
data.tar.gz: 5edf32dbd4518fe1b4da85353b047c7380e1512b
|
5
5
|
SHA512:
|
6
|
-
metadata.gz:
|
7
|
-
data.tar.gz:
|
6
|
+
metadata.gz: 1e68553503a3d9df8c8dad7e853588ae8dc8211b7527a2c1b0d5e8d8a34733009adae2c115073fa584c7a85f2c64446fdd36b871f4b9cb7f84c4a8d199acb3a2
|
7
|
+
data.tar.gz: 55a24fa8675e6298ea52c68261f26d2314ee75ce9928d00dba118aa2932a11989ebabd2dfe0131473c4dd30bd901fd5bc86109f40c179d90f680bd2ad34b15ed
|
data/.travis.yml
ADDED
data/README.md
CHANGED
@@ -1,5 +1,8 @@
|
|
1
1
|
# bar-of-progress
|
2
2
|
|
3
|
+
[](https://travis-ci.org/searls/bar-of-progress) [](https://codeclimate.com/github/searls/bar-of-progress)
|
4
|
+
|
5
|
+
|
3
6
|
Everyone knows that progress bars are one of the hard, unsolved problems of computer science, so I decided to give it a shot.
|
4
7
|
|
5
8
|
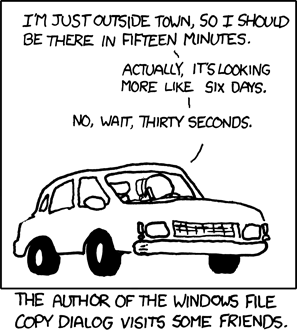
|
@@ -9,6 +12,8 @@ Everyone knows that progress bars are one of the hard, unsolved problems of comp
|
|
9
12
|
### Default progress bars
|
10
13
|
|
11
14
|
``` ruby
|
15
|
+
require 'bar-of-progress'
|
16
|
+
|
12
17
|
bar = BarOfProgress.new #=> defaults to completeness == "100"
|
13
18
|
|
14
19
|
bar.progress #=> "[◌◌◌◌◌◌◌◌◌◌]"
|
@@ -21,6 +26,8 @@ bar.progress(49) #=> "[●●●●◍◌◌◌◌◌]"
|
|
21
26
|
### Custom progress bars
|
22
27
|
|
23
28
|
``` ruby
|
29
|
+
require 'bar-of-progress'
|
30
|
+
|
24
31
|
bar = BarOfProgress.new(
|
25
32
|
:total => 115.5, #=> default (will be converted to BigDecimal): 100
|
26
33
|
:length => 14, #=> default (will be converted to Fixnum): 10
|
data/lib/bar-of-progress.rb
CHANGED
@@ -1,3 +1,5 @@
|
|
1
|
+
# encoding: utf-8
|
2
|
+
|
1
3
|
require "bar_of_progress/version"
|
2
4
|
|
3
5
|
require 'bigdecimal'
|
@@ -23,21 +25,65 @@ class BarOfProgress
|
|
23
25
|
end
|
24
26
|
|
25
27
|
def progress(amount = 0)
|
26
|
-
bubbles =
|
27
|
-
|
28
|
-
partial_bubbles = bubbles.truncate(@options[:precision]) % 1 == 0 ? 0 : 1
|
29
|
-
"#{@options[:braces][0]}#{chars(@options[:complete_indicator], full_bubbles)}#{chars(@options[:partial_indicator], partial_bubbles)}#{chars(@options[:incomplete_indicator], (@options[:length] - full_bubbles - partial_bubbles))}#{@options[:braces][1]}"
|
28
|
+
bubbles = clamped_bubbles_for(amount)
|
29
|
+
Output.new(bubbles.floor, partial_bubbles_for(bubbles), @options).to_s
|
30
30
|
end
|
31
31
|
|
32
32
|
private
|
33
33
|
|
34
|
+
def clamped_bubbles_for(amount)
|
35
|
+
clamp(bubbles_for(amount), 0, @options[:length]).to_d
|
36
|
+
end
|
37
|
+
|
38
|
+
def partial_bubbles_for(bubbles)
|
39
|
+
bubbles.truncate(@options[:precision]) % 1 == 0 ? 0 : 1
|
40
|
+
end
|
41
|
+
|
42
|
+
def bubbles_for(amount)
|
43
|
+
(amount.to_d / @options[:total]) * @options[:length]
|
44
|
+
end
|
45
|
+
|
34
46
|
# This method is amazing.
|
35
47
|
def clamp(n, min, max)
|
36
48
|
[[n, min].max, max].min
|
37
49
|
end
|
38
50
|
|
39
|
-
|
40
|
-
|
41
|
-
|
51
|
+
class Output
|
52
|
+
def initialize(complete, partial, options)
|
53
|
+
@complete = complete
|
54
|
+
@partial = partial
|
55
|
+
@options = options
|
56
|
+
end
|
57
|
+
|
58
|
+
def to_s
|
59
|
+
"#{left_bracket}#{complete}#{partial}#{incomplete}#{right_bracket}"
|
60
|
+
end
|
61
|
+
|
62
|
+
private
|
63
|
+
|
64
|
+
def left_bracket
|
65
|
+
@options[:braces][0]
|
66
|
+
end
|
67
|
+
|
68
|
+
def complete
|
69
|
+
chars(@options[:complete_indicator], @complete)
|
70
|
+
end
|
71
|
+
|
72
|
+
def partial
|
73
|
+
@options[:partial_indicator] if @partial > 0
|
74
|
+
end
|
75
|
+
|
76
|
+
def incomplete
|
77
|
+
chars(@options[:incomplete_indicator], (@options[:length] - @complete - @partial))
|
78
|
+
end
|
79
|
+
|
80
|
+
def right_bracket
|
81
|
+
@options[:braces][1]
|
82
|
+
end
|
83
|
+
|
84
|
+
def chars(char, n)
|
85
|
+
return "" if n <= 0
|
86
|
+
char * n
|
87
|
+
end
|
42
88
|
end
|
43
89
|
end
|
metadata
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
--- !ruby/object:Gem::Specification
|
2
2
|
name: bar-of-progress
|
3
3
|
version: !ruby/object:Gem::Version
|
4
|
-
version: 0.1.
|
4
|
+
version: 0.1.1
|
5
5
|
platform: ruby
|
6
6
|
authors:
|
7
7
|
- Justin Searls
|
@@ -47,6 +47,7 @@ extra_rdoc_files: []
|
|
47
47
|
files:
|
48
48
|
- .gitignore
|
49
49
|
- .rspec
|
50
|
+
- .travis.yml
|
50
51
|
- Gemfile
|
51
52
|
- LICENSE.txt
|
52
53
|
- README.md
|