aspose_pdf_cloud 19.7.0 → 19.8.0
Sign up to get free protection for your applications and to get access to all the features.
- checksums.yaml +4 -4
- data/README.md +18 -4
- data/docs/DocMDPAccessPermissionType.md +16 -0
- data/docs/FormField.md +26 -0
- data/docs/PdfApi.md +155 -0
- data/docs/Signature.md +3 -0
- data/docs/SignatureCustomAppearance.md +20 -0
- data/docs/SignatureField.md +27 -0
- data/docs/SignatureFieldResponse.md +11 -0
- data/docs/SignatureFields.md +10 -0
- data/docs/SignatureFieldsResponse.md +11 -0
- data/docs/TimestampSettings.md +10 -0
- data/lib/aspose_pdf_cloud.rb +8 -0
- data/lib/aspose_pdf_cloud/api/pdf_api.rb +479 -8
- data/lib/aspose_pdf_cloud/models/doc_mdp_access_permission_type.rb +45 -0
- data/lib/aspose_pdf_cloud/models/form_field.rb +383 -0
- data/lib/aspose_pdf_cloud/models/signature.rb +34 -4
- data/lib/aspose_pdf_cloud/models/signature_custom_appearance.rb +329 -0
- data/lib/aspose_pdf_cloud/models/signature_field.rb +393 -0
- data/lib/aspose_pdf_cloud/models/signature_field_response.rb +224 -0
- data/lib/aspose_pdf_cloud/models/signature_fields.rb +213 -0
- data/lib/aspose_pdf_cloud/models/signature_fields_response.rb +224 -0
- data/lib/aspose_pdf_cloud/models/timestamp_settings.rb +209 -0
- data/lib/aspose_pdf_cloud/version.rb +1 -1
- data/test/pdf_tests.rb +96 -0
- data/test_data/33226.p12 +0 -0
- data/test_data/adbe.x509.rsa_sha1.valid.pdf +0 -0
- data/test_data/mixed.md +14 -0
- metadata +21 -2
@@ -0,0 +1,209 @@
|
|
1
|
+
=begin
|
2
|
+
--------------------------------------------------------------------------------------------------------------------
|
3
|
+
Copyright (c) 2019 Aspose.PDF Cloud
|
4
|
+
Permission is hereby granted, free of charge, to any person obtaining a copy
|
5
|
+
of this software and associated documentation files (the "Software"), to deal
|
6
|
+
in the Software without restriction, including without limitation the rights
|
7
|
+
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
8
|
+
copies of the Software, and to permit persons to whom the Software is
|
9
|
+
furnished to do so, subject to the following conditions:
|
10
|
+
The above copyright notice and this permission notice shall be included in all
|
11
|
+
copies or substantial portions of the Software.
|
12
|
+
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
13
|
+
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
14
|
+
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
15
|
+
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
16
|
+
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
17
|
+
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
18
|
+
SOFTWARE.
|
19
|
+
--------------------------------------------------------------------------------------------------------------------
|
20
|
+
=end
|
21
|
+
|
22
|
+
require 'date'
|
23
|
+
require 'time'
|
24
|
+
|
25
|
+
module AsposePdfCloud
|
26
|
+
# Represents the ocsp settings using during signing process.
|
27
|
+
class TimestampSettings
|
28
|
+
# Gets/sets the timestamp server url.
|
29
|
+
attr_accessor :server_url
|
30
|
+
|
31
|
+
# Gets/sets the basic authentication credentials, Username and password are combined into a string \"username:password\".
|
32
|
+
attr_accessor :basic_auth_credentials
|
33
|
+
|
34
|
+
|
35
|
+
# Attribute mapping from ruby-style variable name to JSON key.
|
36
|
+
def self.attribute_map
|
37
|
+
{
|
38
|
+
:'server_url' => :'ServerUrl',
|
39
|
+
:'basic_auth_credentials' => :'BasicAuthCredentials'
|
40
|
+
}
|
41
|
+
end
|
42
|
+
|
43
|
+
# Attribute type mapping.
|
44
|
+
def self.swagger_types
|
45
|
+
{
|
46
|
+
:'server_url' => :'String',
|
47
|
+
:'basic_auth_credentials' => :'String'
|
48
|
+
}
|
49
|
+
end
|
50
|
+
|
51
|
+
# Initializes the object
|
52
|
+
# @param [Hash] attributes Model attributes in the form of hash
|
53
|
+
def initialize(attributes = {})
|
54
|
+
return unless attributes.is_a?(Hash)
|
55
|
+
|
56
|
+
# convert string to symbol for hash key
|
57
|
+
attributes = attributes.each_with_object({}){|(k,v), h| h[k.to_sym] = v}
|
58
|
+
|
59
|
+
if attributes.has_key?(:'ServerUrl')
|
60
|
+
self.server_url = attributes[:'ServerUrl']
|
61
|
+
end
|
62
|
+
|
63
|
+
if attributes.has_key?(:'BasicAuthCredentials')
|
64
|
+
self.basic_auth_credentials = attributes[:'BasicAuthCredentials']
|
65
|
+
end
|
66
|
+
|
67
|
+
end
|
68
|
+
|
69
|
+
# Show invalid properties with the reasons. Usually used together with valid?
|
70
|
+
# @return Array for valid properies with the reasons
|
71
|
+
def list_invalid_properties
|
72
|
+
invalid_properties = Array.new
|
73
|
+
return invalid_properties
|
74
|
+
end
|
75
|
+
|
76
|
+
# Check to see if the all the properties in the model are valid
|
77
|
+
# @return true if the model is valid
|
78
|
+
def valid?
|
79
|
+
return true
|
80
|
+
end
|
81
|
+
|
82
|
+
# Checks equality by comparing each attribute.
|
83
|
+
# @param [Object] Object to be compared
|
84
|
+
def ==(o)
|
85
|
+
return true if self.equal?(o)
|
86
|
+
self.class == o.class &&
|
87
|
+
server_url == o.server_url &&
|
88
|
+
basic_auth_credentials == o.basic_auth_credentials
|
89
|
+
end
|
90
|
+
|
91
|
+
# @see the `==` method
|
92
|
+
# @param [Object] Object to be compared
|
93
|
+
def eql?(o)
|
94
|
+
self == o
|
95
|
+
end
|
96
|
+
|
97
|
+
# Calculates hash code according to all attributes.
|
98
|
+
# @return [Fixnum] Hash code
|
99
|
+
def hash
|
100
|
+
[server_url, basic_auth_credentials].hash
|
101
|
+
end
|
102
|
+
|
103
|
+
# Builds the object from hash
|
104
|
+
# @param [Hash] attributes Model attributes in the form of hash
|
105
|
+
# @return [Object] Returns the model itself
|
106
|
+
def build_from_hash(attributes)
|
107
|
+
return nil unless attributes.is_a?(Hash)
|
108
|
+
self.class.swagger_types.each_pair do |key, type|
|
109
|
+
if type =~ /\AArray<(.*)>/i
|
110
|
+
# check to ensure the input is an array given that the the attribute
|
111
|
+
# is documented as an array but the input is not
|
112
|
+
if attributes[self.class.attribute_map[key]].is_a?(Array)
|
113
|
+
self.send("#{key}=", attributes[self.class.attribute_map[key]].map{ |v| _deserialize($1, v) } )
|
114
|
+
end
|
115
|
+
elsif !attributes[self.class.attribute_map[key]].nil?
|
116
|
+
self.send("#{key}=", _deserialize(type, attributes[self.class.attribute_map[key]]))
|
117
|
+
end # or else data not found in attributes(hash), not an issue as the data can be optional
|
118
|
+
end
|
119
|
+
|
120
|
+
self
|
121
|
+
end
|
122
|
+
|
123
|
+
# Deserializes the data based on type
|
124
|
+
# @param string type Data type
|
125
|
+
# @param string value Value to be deserialized
|
126
|
+
# @return [Object] Deserialized data
|
127
|
+
def _deserialize(type, value)
|
128
|
+
case type.to_sym
|
129
|
+
when :DateTime
|
130
|
+
DateTime.parse(value)
|
131
|
+
when :Date
|
132
|
+
Date.parse(value)
|
133
|
+
when :String
|
134
|
+
value.to_s
|
135
|
+
when :Integer
|
136
|
+
value.to_i
|
137
|
+
when :Float
|
138
|
+
value.to_f
|
139
|
+
when :BOOLEAN
|
140
|
+
if value.to_s =~ /\A(true|t|yes|y|1)\z/i
|
141
|
+
true
|
142
|
+
else
|
143
|
+
false
|
144
|
+
end
|
145
|
+
when :Object
|
146
|
+
# generic object (usually a Hash), return directly
|
147
|
+
value
|
148
|
+
when /\AArray<(?<inner_type>.+)>\z/
|
149
|
+
inner_type = Regexp.last_match[:inner_type]
|
150
|
+
value.map { |v| _deserialize(inner_type, v) }
|
151
|
+
when /\AHash<(?<k_type>.+?), (?<v_type>.+)>\z/
|
152
|
+
k_type = Regexp.last_match[:k_type]
|
153
|
+
v_type = Regexp.last_match[:v_type]
|
154
|
+
{}.tap do |hash|
|
155
|
+
value.each do |k, v|
|
156
|
+
hash[_deserialize(k_type, k)] = _deserialize(v_type, v)
|
157
|
+
end
|
158
|
+
end
|
159
|
+
else # model
|
160
|
+
temp_model = AsposePdfCloud.const_get(type).new
|
161
|
+
temp_model.build_from_hash(value)
|
162
|
+
end
|
163
|
+
end
|
164
|
+
|
165
|
+
# Returns the string representation of the object
|
166
|
+
# @return [String] String presentation of the object
|
167
|
+
def to_s
|
168
|
+
to_hash.to_s
|
169
|
+
end
|
170
|
+
|
171
|
+
# to_body is an alias to to_hash (backward compatibility)
|
172
|
+
# @return [Hash] Returns the object in the form of hash
|
173
|
+
def to_body
|
174
|
+
to_hash
|
175
|
+
end
|
176
|
+
|
177
|
+
# Returns the object in the form of hash
|
178
|
+
# @return [Hash] Returns the object in the form of hash
|
179
|
+
def to_hash
|
180
|
+
hash = {}
|
181
|
+
self.class.attribute_map.each_pair do |attr, param|
|
182
|
+
value = self.send(attr)
|
183
|
+
next if value.nil?
|
184
|
+
hash[param] = _to_hash(value)
|
185
|
+
end
|
186
|
+
hash
|
187
|
+
end
|
188
|
+
|
189
|
+
# Outputs non-array value in the form of hash
|
190
|
+
# For object, use to_hash. Otherwise, just return the value
|
191
|
+
# @param [Object] value Any valid value
|
192
|
+
# @return [Hash] Returns the value in the form of hash
|
193
|
+
def _to_hash(value)
|
194
|
+
if value.is_a?(Array)
|
195
|
+
value.compact.map{ |v| _to_hash(v) }
|
196
|
+
elsif value.is_a?(Hash)
|
197
|
+
{}.tap do |hash|
|
198
|
+
value.each { |k, v| hash[k] = _to_hash(v) }
|
199
|
+
end
|
200
|
+
elsif value.respond_to? :to_hash
|
201
|
+
value.to_hash
|
202
|
+
else
|
203
|
+
value
|
204
|
+
end
|
205
|
+
end
|
206
|
+
|
207
|
+
end
|
208
|
+
|
209
|
+
end
|
data/test/pdf_tests.rb
CHANGED
@@ -3578,6 +3578,27 @@ class PdfTests < Minitest::Test
|
|
3578
3578
|
assert(response, 'Failed to convert images to pdf.')
|
3579
3579
|
end
|
3580
3580
|
|
3581
|
+
def test_get_markdown_in_storage_to_pdf
|
3582
|
+
file_name = 'mixed.md'
|
3583
|
+
upload_file(file_name)
|
3584
|
+
|
3585
|
+
src_path = @temp_folder + '/' + file_name
|
3586
|
+
response = @pdf_api.get_markdown_in_storage_to_pdf(src_path)
|
3587
|
+
assert(response, 'Failed to convert markdown to pdf.')
|
3588
|
+
end
|
3589
|
+
|
3590
|
+
def test_put_markdow_in_storage_to_pdf
|
3591
|
+
file_name = 'mixed.md'
|
3592
|
+
upload_file(file_name)
|
3593
|
+
result_name = 'fromMd.pdf'
|
3594
|
+
|
3595
|
+
src_path = @temp_folder + '/' + file_name
|
3596
|
+
opts = {
|
3597
|
+
:dst_folder => @temp_folder
|
3598
|
+
}
|
3599
|
+
response = @pdf_api.put_markdown_in_storage_to_pdf(result_name, src_path, opts)
|
3600
|
+
assert(response, 'Failed to convert markdown to pdf.')
|
3601
|
+
end
|
3581
3602
|
|
3582
3603
|
# Document Tests
|
3583
3604
|
|
@@ -3777,6 +3798,44 @@ class PdfTests < Minitest::Test
|
|
3777
3798
|
assert(response, 'Failed flatten document.')
|
3778
3799
|
end
|
3779
3800
|
|
3801
|
+
def test_get_document_gignature_fields
|
3802
|
+
file_name = 'adbe.x509.rsa_sha1.valid.pdf'
|
3803
|
+
upload_file(file_name)
|
3804
|
+
|
3805
|
+
opts = {
|
3806
|
+
:folder => @temp_folder
|
3807
|
+
}
|
3808
|
+
|
3809
|
+
response = @pdf_api.get_document_signature_fields(file_name, opts)
|
3810
|
+
assert(response, 'Failed to read document signature fields.')
|
3811
|
+
end
|
3812
|
+
|
3813
|
+
def test_get_page_gignature_fields
|
3814
|
+
file_name = 'adbe.x509.rsa_sha1.valid.pdf'
|
3815
|
+
upload_file(file_name)
|
3816
|
+
|
3817
|
+
page_number = 1
|
3818
|
+
opts = {
|
3819
|
+
:folder => @temp_folder
|
3820
|
+
}
|
3821
|
+
|
3822
|
+
response = @pdf_api.get_page_signature_fields(file_name, page_number, opts)
|
3823
|
+
assert(response, 'Failed to read page signature fields.')
|
3824
|
+
end
|
3825
|
+
|
3826
|
+
def test_get_gignature_field
|
3827
|
+
file_name = 'adbe.x509.rsa_sha1.valid.pdf'
|
3828
|
+
upload_file(file_name)
|
3829
|
+
|
3830
|
+
field_name = 'Signature1'
|
3831
|
+
opts = {
|
3832
|
+
:folder => @temp_folder
|
3833
|
+
}
|
3834
|
+
|
3835
|
+
response = @pdf_api.get_signature_field(file_name, field_name, opts)
|
3836
|
+
assert(response, 'Failed to read signature field.')
|
3837
|
+
end
|
3838
|
+
|
3780
3839
|
# Stamp Tests
|
3781
3840
|
|
3782
3841
|
def test_get_document_stamps
|
@@ -4973,6 +5032,43 @@ class PdfTests < Minitest::Test
|
|
4973
5032
|
assert(response, 'Failed to verify signature.')
|
4974
5033
|
end
|
4975
5034
|
|
5035
|
+
def test_post_page_certify
|
5036
|
+
file_name = '4pages.pdf'
|
5037
|
+
upload_file(file_name)
|
5038
|
+
|
5039
|
+
signature_file_name = '33226.p12'
|
5040
|
+
upload_file(signature_file_name)
|
5041
|
+
|
5042
|
+
page_number = 1
|
5043
|
+
|
5044
|
+
permission_type = DocMDPAccessPermissionType::NO_CHANGES
|
5045
|
+
|
5046
|
+
rectangle = Rectangle.new
|
5047
|
+
rectangle.llx = 100
|
5048
|
+
rectangle.lly = 100
|
5049
|
+
rectangle.urx = 500
|
5050
|
+
rectangle.ury = 200
|
5051
|
+
|
5052
|
+
signature = Signature.new
|
5053
|
+
signature.authority = 'Sergey Smal'
|
5054
|
+
signature.contact = 'test@mail.ru'
|
5055
|
+
signature.date = '08/01/2012 12:15:00.000 PM'
|
5056
|
+
signature.form_field_name = 'Signature1'
|
5057
|
+
signature.location = 'Ukraine'
|
5058
|
+
signature.password = 'sIikZSmz'
|
5059
|
+
signature.rectangle = rectangle
|
5060
|
+
signature.signature_path = @temp_folder + '/' + signature_file_name
|
5061
|
+
signature.signature_type = SignatureType::PKCS7
|
5062
|
+
signature.visible = true
|
5063
|
+
signature.show_properties = false
|
5064
|
+
|
5065
|
+
opts = {
|
5066
|
+
:folder => @temp_folder
|
5067
|
+
}
|
5068
|
+
|
5069
|
+
response = @pdf_api.post_page_certify(file_name, page_number, signature, permission_type, opts)
|
5070
|
+
assert(response, 'Failed to certify page.')
|
5071
|
+
end
|
4976
5072
|
|
4977
5073
|
|
4978
5074
|
# Text Replace Tests
|
data/test_data/33226.p12
ADDED
Binary file
|
Binary file
|
data/test_data/mixed.md
ADDED
@@ -0,0 +1,14 @@
|
|
1
|
+
### *Lorem Ipsum* ###
|
2
|
+
|
3
|
+
Sed ut perspiciatis, unde omnis iste natus error sit voluptatem accusantium doloremque laudantium, totam rem aperiam eaque ipsa, quae ab illo inventore veritatis et quasi architecto beatae vitae dicta sunt, explicabo.
|
4
|
+
>Nemo enim ipsam voluptatem, quia voluptas sit, aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos,
|
5
|
+
>>qui ratione voluptatem sequi nesciunt, neque porro quisquam est, qui dolorem ipsum, quia dolor sit, amet, consectetur, adipisci velit, sed quia non
|
6
|
+
numquam eius modi tempora incidunt, ut labore et dolore magnam aliquam quaerat voluptatem.
|
7
|
+
>Ut enim ad minima veniam, quis nostrum exercitationem ullam corporis suscipit laboriosam, nisi ut aliquid ex ea commodi consequatur?
|
8
|
+
----
|
9
|
+
* *One*
|
10
|
+
* **Two**
|
11
|
+
* ***Three***
|
12
|
+
****
|
13
|
+
[ 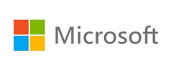 ](https://daringfireball.net/projects/markdown/syntax)
|
14
|
+
Quis autem vel eum iure reprehenderit, qui in ea voluptate velit esse, `quam nihil molestiae consequatur,` vel illum, qui dolorem eum fugiat, quo voluptas nulla pariatur? At vero eos et accusamus et iusto odio dignissimos ducimus, qui blanditiis praesentium voluptatum deleniti atque corrupti, quos dolores et quas molestias excepturi sint, obcaecati cupiditate non provident, similique sunt in culpa, qui officia deserunt mollitia animi, id est laborum et dolorum fuga. Et harum quidem rerum facilis est et expedita distinctio. Nam libero tempore, cum soluta nobis est eligendi optio, cumque nihil impedit, quo minus id, quod maxime placeat, facere possimus, omnis voluptas assumenda est, omnis dolor repellendus. Temporibus autem quibusdam et aut officiis debitis aut rerum necessitatibus saepe eveniet, ut et voluptates repudiandae sint et molestiae non recusandae. Itaque earum rerum hic tenetur a sapiente delectus, ut aut reiciendis voluptatibus maiores alias consequatur aut perferendis doloribus asperiores repellat.
|
metadata
CHANGED
@@ -1,14 +1,14 @@
|
|
1
1
|
--- !ruby/object:Gem::Specification
|
2
2
|
name: aspose_pdf_cloud
|
3
3
|
version: !ruby/object:Gem::Version
|
4
|
-
version: 19.
|
4
|
+
version: 19.8.0
|
5
5
|
platform: ruby
|
6
6
|
authors:
|
7
7
|
- Aspose PDF Cloud
|
8
8
|
autorequire:
|
9
9
|
bindir: bin
|
10
10
|
cert_chain: []
|
11
|
-
date: 2019-
|
11
|
+
date: 2019-09-05 00:00:00.000000000 Z
|
12
12
|
dependencies:
|
13
13
|
- !ruby/object:Gem::Dependency
|
14
14
|
name: json
|
@@ -110,6 +110,7 @@ files:
|
|
110
110
|
- docs/CryptoAlgorithm.md
|
111
111
|
- docs/DiscUsage.md
|
112
112
|
- docs/DocFormat.md
|
113
|
+
- docs/DocMDPAccessPermissionType.md
|
113
114
|
- docs/DocRecognitionMode.md
|
114
115
|
- docs/Document.md
|
115
116
|
- docs/DocumentPageResponse.md
|
@@ -140,6 +141,7 @@ files:
|
|
140
141
|
- docs/FontEncodingRules.md
|
141
142
|
- docs/FontSavingModes.md
|
142
143
|
- docs/FontStyles.md
|
144
|
+
- docs/FormField.md
|
143
145
|
- docs/FreeTextAnnotation.md
|
144
146
|
- docs/FreeTextAnnotationResponse.md
|
145
147
|
- docs/FreeTextAnnotations.md
|
@@ -236,6 +238,11 @@ files:
|
|
236
238
|
- docs/Segment.md
|
237
239
|
- docs/ShapeType.md
|
238
240
|
- docs/Signature.md
|
241
|
+
- docs/SignatureCustomAppearance.md
|
242
|
+
- docs/SignatureField.md
|
243
|
+
- docs/SignatureFieldResponse.md
|
244
|
+
- docs/SignatureFields.md
|
245
|
+
- docs/SignatureFieldsResponse.md
|
239
246
|
- docs/SignatureType.md
|
240
247
|
- docs/SignatureVerifyResponse.md
|
241
248
|
- docs/SoundAnnotation.md
|
@@ -296,6 +303,7 @@ files:
|
|
296
303
|
- docs/TextStamp.md
|
297
304
|
- docs/TextState.md
|
298
305
|
- docs/TextStyle.md
|
306
|
+
- docs/TimestampSettings.md
|
299
307
|
- docs/UnderlineAnnotation.md
|
300
308
|
- docs/UnderlineAnnotationResponse.md
|
301
309
|
- docs/UnderlineAnnotations.md
|
@@ -349,6 +357,7 @@ files:
|
|
349
357
|
- lib/aspose_pdf_cloud/models/crypto_algorithm.rb
|
350
358
|
- lib/aspose_pdf_cloud/models/disc_usage.rb
|
351
359
|
- lib/aspose_pdf_cloud/models/doc_format.rb
|
360
|
+
- lib/aspose_pdf_cloud/models/doc_mdp_access_permission_type.rb
|
352
361
|
- lib/aspose_pdf_cloud/models/doc_recognition_mode.rb
|
353
362
|
- lib/aspose_pdf_cloud/models/document.rb
|
354
363
|
- lib/aspose_pdf_cloud/models/document_page_response.rb
|
@@ -379,6 +388,7 @@ files:
|
|
379
388
|
- lib/aspose_pdf_cloud/models/font_encoding_rules.rb
|
380
389
|
- lib/aspose_pdf_cloud/models/font_saving_modes.rb
|
381
390
|
- lib/aspose_pdf_cloud/models/font_styles.rb
|
391
|
+
- lib/aspose_pdf_cloud/models/form_field.rb
|
382
392
|
- lib/aspose_pdf_cloud/models/free_text_annotation.rb
|
383
393
|
- lib/aspose_pdf_cloud/models/free_text_annotation_response.rb
|
384
394
|
- lib/aspose_pdf_cloud/models/free_text_annotations.rb
|
@@ -474,6 +484,11 @@ files:
|
|
474
484
|
- lib/aspose_pdf_cloud/models/segment.rb
|
475
485
|
- lib/aspose_pdf_cloud/models/shape_type.rb
|
476
486
|
- lib/aspose_pdf_cloud/models/signature.rb
|
487
|
+
- lib/aspose_pdf_cloud/models/signature_custom_appearance.rb
|
488
|
+
- lib/aspose_pdf_cloud/models/signature_field.rb
|
489
|
+
- lib/aspose_pdf_cloud/models/signature_field_response.rb
|
490
|
+
- lib/aspose_pdf_cloud/models/signature_fields.rb
|
491
|
+
- lib/aspose_pdf_cloud/models/signature_fields_response.rb
|
477
492
|
- lib/aspose_pdf_cloud/models/signature_type.rb
|
478
493
|
- lib/aspose_pdf_cloud/models/signature_verify_response.rb
|
479
494
|
- lib/aspose_pdf_cloud/models/sound_annotation.rb
|
@@ -534,6 +549,7 @@ files:
|
|
534
549
|
- lib/aspose_pdf_cloud/models/text_stamp.rb
|
535
550
|
- lib/aspose_pdf_cloud/models/text_state.rb
|
536
551
|
- lib/aspose_pdf_cloud/models/text_style.rb
|
552
|
+
- lib/aspose_pdf_cloud/models/timestamp_settings.rb
|
537
553
|
- lib/aspose_pdf_cloud/models/underline_annotation.rb
|
538
554
|
- lib/aspose_pdf_cloud/models/underline_annotation_response.rb
|
539
555
|
- lib/aspose_pdf_cloud/models/underline_annotations.rb
|
@@ -545,6 +561,7 @@ files:
|
|
545
561
|
- lib/aspose_pdf_cloud/version.rb
|
546
562
|
- test/pdf_tests.rb
|
547
563
|
- test_data/.gitattributes
|
564
|
+
- test_data/33226.p12
|
548
565
|
- test_data/33539.jpg
|
549
566
|
- test_data/44781.jpg
|
550
567
|
- test_data/4pages.epub
|
@@ -581,8 +598,10 @@ files:
|
|
581
598
|
- test_data/TexExample.tex
|
582
599
|
- test_data/Typography.PS
|
583
600
|
- test_data/XslfoExample.xslfo
|
601
|
+
- test_data/adbe.x509.rsa_sha1.valid.pdf
|
584
602
|
- test_data/butterfly.jpg
|
585
603
|
- test_data/marketing.pdf
|
604
|
+
- test_data/mixed.md
|
586
605
|
- test_data/rusdoc.pdf
|
587
606
|
- test_data/sample.tex
|
588
607
|
- test_data/template.pcl
|