api_analytics 1.0.5 → 1.1.0
Sign up to get free protection for your applications and to get access to all the features.
- checksums.yaml +4 -4
- data/README.md +56 -3
- data/api_analytics.gemspec +0 -1
- data/lib/api_analytics/version.rb +1 -1
- data/lib/api_analytics.rb +30 -12
- metadata +2 -2
checksums.yaml
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
---
|
2
2
|
SHA256:
|
3
|
-
metadata.gz:
|
4
|
-
data.tar.gz:
|
3
|
+
metadata.gz: 3e645b37969365ab4362e1638745aa8cd4620eee12faf229e0f7a80ea4487ceb
|
4
|
+
data.tar.gz: 2dda631a1982214b4d1928b5af24ee79a067dfde0b214ecfdd4e7410a6dd1067
|
5
5
|
SHA512:
|
6
|
-
metadata.gz:
|
7
|
-
data.tar.gz:
|
6
|
+
metadata.gz: c345b31b23c7e66a499a30e1cb49db591880186fc82af7aa5caa1b7ea690b0a54ed655e73c5e01d25c36ee5bff2f891813e5ffe0f62d12c946d4a0b0caa41980
|
7
|
+
data.tar.gz: 0c9374687dd5588b5cf8e735d4d4c27f0dfc42e10392ec4d45d6dcc84cff00eac97ad3e10e64f306ef06af89157b667f087c23cb97045efceacbacf0d2601e18
|
data/README.md
CHANGED
@@ -31,7 +31,7 @@ module RailsMiddleware
|
|
31
31
|
config.load_defaults 6.1
|
32
32
|
config.api_only = true
|
33
33
|
|
34
|
-
config.middleware.use ::Analytics::Rails, <
|
34
|
+
config.middleware.use ::Analytics::Rails, <API-KEY> # Add middleware
|
35
35
|
end
|
36
36
|
end
|
37
37
|
```
|
@@ -42,7 +42,7 @@ end
|
|
42
42
|
require 'sinatra'
|
43
43
|
require 'api_analytics'
|
44
44
|
|
45
|
-
use Analytics::Sinatra, <
|
45
|
+
use Analytics::Sinatra, <API-KEY> # Add middleware
|
46
46
|
|
47
47
|
before do
|
48
48
|
content_type 'application/json'
|
@@ -55,4 +55,57 @@ end
|
|
55
55
|
|
56
56
|
### 3. View your analytics
|
57
57
|
|
58
|
-
Your API will log
|
58
|
+
Your API will now log and store incoming request data on all valid routes. Your logged data can be viewed using two methods: through visualizations and stats on our dashboard, or accessed directly via our data API.
|
59
|
+
|
60
|
+
You can use the same API key across multiple APIs, but all your data will appear in the same dashboard. We recommend generating a new API key for each additional API you want analytics for.
|
61
|
+
|
62
|
+
#### Dashboard
|
63
|
+
|
64
|
+
Head to https://my-api-analytics.vercel.app/dashboard and paste in your API key to access your dashboard.
|
65
|
+
|
66
|
+
Demo: https://my-api-analytics.vercel.app/dashboard/demo
|
67
|
+
|
68
|
+
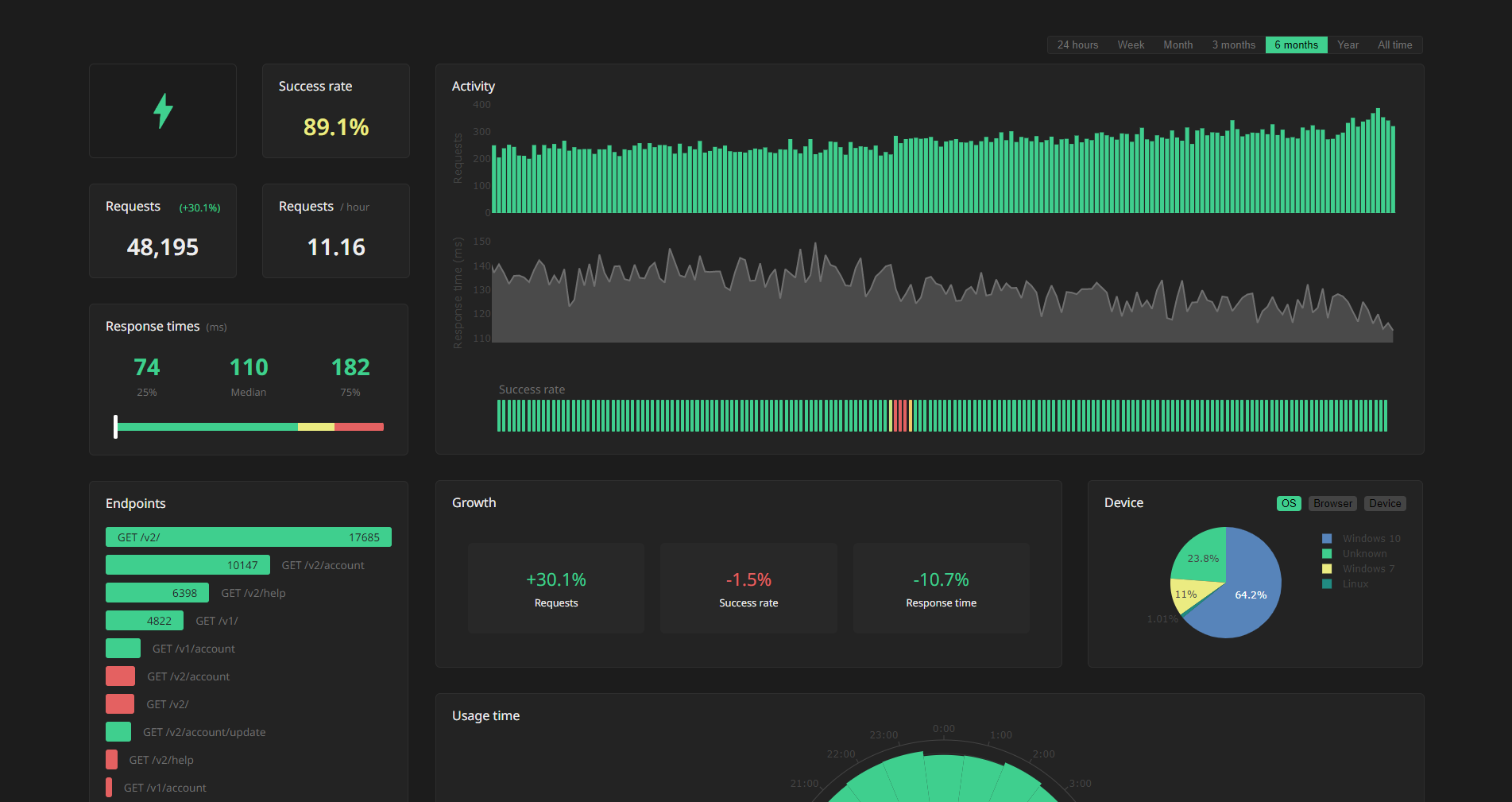
|
69
|
+
|
70
|
+
#### Data API
|
71
|
+
|
72
|
+
Logged data for all requests can be accessed via our API. Simply send a GET request to `https://api-analytics-server.vercel.app/api/data` with your API key set as `API-Key` in headers.
|
73
|
+
|
74
|
+
```py
|
75
|
+
import requests
|
76
|
+
|
77
|
+
headers = {
|
78
|
+
"API-Key": <API-KEY>
|
79
|
+
}
|
80
|
+
|
81
|
+
response = requests.get("https://api-analytics-server.vercel.app/api/data", headers=headers)
|
82
|
+
print(response.json())
|
83
|
+
```
|
84
|
+
|
85
|
+
## Monitoring (coming soon)
|
86
|
+
|
87
|
+
Opt-in active API monitoring is coming soon. Our servers will regularly ping your API endpoints to monitor uptime and response time. Optional email alerts to notify you when your endpoints are down can be subscribed to.
|
88
|
+
|
89
|
+
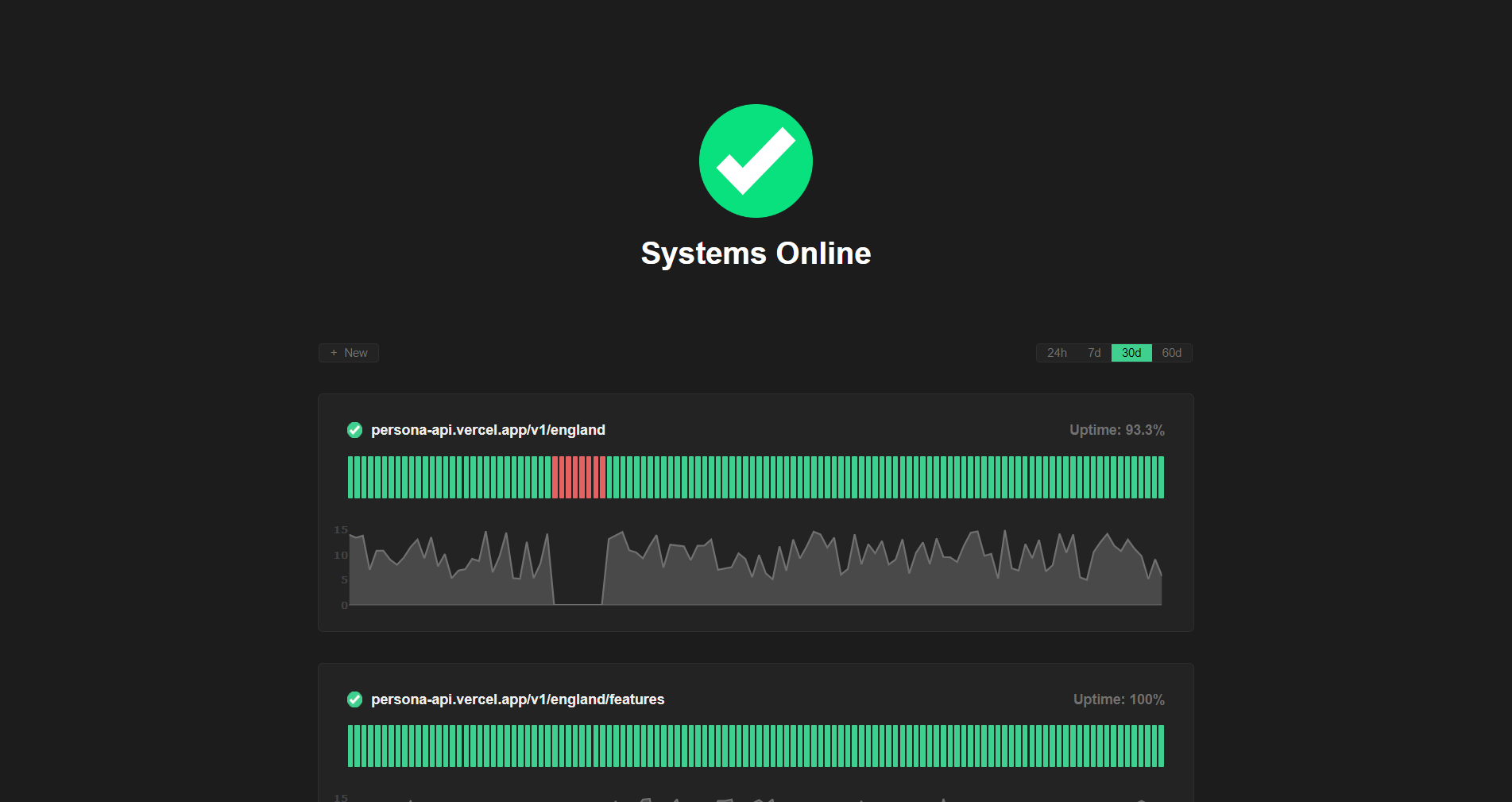
|
90
|
+
|
91
|
+
## Data and Security
|
92
|
+
|
93
|
+
All data is stored securely in compliance with The EU General Data Protection Regulation (GDPR).
|
94
|
+
|
95
|
+
For any given request to your API, data recorded is limited to:
|
96
|
+
- Path requested by client
|
97
|
+
- Client IP address
|
98
|
+
- Client operating system
|
99
|
+
- Client browser
|
100
|
+
- Request method (GET, POST, PUT, etc.)
|
101
|
+
- Time of request
|
102
|
+
- Status code
|
103
|
+
- Response time
|
104
|
+
- API hostname
|
105
|
+
- API framework (FastAPI, Flask, Express etc.)
|
106
|
+
|
107
|
+
Data collected is only ever used to populate your analytics dashboard. Your data is anonymous, with the API key the only link between you and you API's analytics. Should you lose your API key, you will have no method to access your API analytics. Inactive API keys (> 1 year) and its associated API request data may be deleted.
|
108
|
+
|
109
|
+
### Delete Data
|
110
|
+
|
111
|
+
At any time, you can delete all stored data associated with your API key by going to https://my-api-analytics.vercel.app/delete and entering your API key.
|
data/api_analytics.gemspec
CHANGED
@@ -7,7 +7,6 @@ Gem::Specification.new do |spec|
|
|
7
7
|
spec.version = Analytics::VERSION
|
8
8
|
spec.authors = ["Tom Draper"]
|
9
9
|
spec.email = ["tomjdraper1@gmail.com"]
|
10
|
-
|
11
10
|
spec.summary = "Monitoring and analytics for API applications."
|
12
11
|
spec.description = "Monitoring and analytics for API applications."
|
13
12
|
spec.homepage = "https://github.com/tom-draper/api-analytics"
|
data/lib/api_analytics.rb
CHANGED
@@ -9,36 +9,56 @@ module Analytics
|
|
9
9
|
def initialize(app, api_key)
|
10
10
|
@app = app
|
11
11
|
@api_key = api_key
|
12
|
+
@requests = Array.new
|
13
|
+
@last_posted = Time.now
|
12
14
|
end
|
13
15
|
|
14
16
|
def call(env)
|
15
17
|
start = Time.now
|
16
18
|
status, headers, response = @app.call(env)
|
17
19
|
|
18
|
-
|
19
|
-
api_key: @api_key,
|
20
|
+
request_data = {
|
20
21
|
hostname: env['HTTP_HOST'],
|
21
22
|
ip_address: env['REMOTE_ADDR'],
|
22
23
|
path: env['REQUEST_PATH'],
|
23
24
|
user_agent: env['HTTP_USER_AGENT'],
|
24
25
|
method: env['REQUEST_METHOD'],
|
25
26
|
status: status,
|
26
|
-
framework: @framework,
|
27
27
|
response_time: (Time.now - start).to_f.round,
|
28
|
+
created_at: Time.now.utc.iso8601
|
28
29
|
}
|
29
30
|
|
30
|
-
|
31
|
-
log_request(data)
|
32
|
-
}
|
31
|
+
log_request(request_data)
|
33
32
|
|
34
33
|
[status, headers, response]
|
35
34
|
end
|
36
|
-
|
35
|
+
|
37
36
|
private
|
38
37
|
|
39
|
-
def
|
40
|
-
|
41
|
-
|
38
|
+
def post_requests(api_key, requests, framework)
|
39
|
+
payload = {
|
40
|
+
api_key: api_key,
|
41
|
+
requests: requests,
|
42
|
+
framework: framework
|
43
|
+
}
|
44
|
+
uri = URI('http://213.168.248.206/api/log-request')
|
45
|
+
res = Net::HTTP.post(uri, payload.to_json)
|
46
|
+
end
|
47
|
+
|
48
|
+
def log_request(request_data)
|
49
|
+
if @api_key.empty?
|
50
|
+
return
|
51
|
+
end
|
52
|
+
now = Time.now
|
53
|
+
@requests.push(request_data)
|
54
|
+
if (now - @last_posted) > 5
|
55
|
+
requests = @requests.dup
|
56
|
+
Thread.new {
|
57
|
+
post_requests(@api_key, requests, @framework)
|
58
|
+
}
|
59
|
+
@requests = Array.new
|
60
|
+
@last_posted = now
|
61
|
+
end
|
42
62
|
end
|
43
63
|
end
|
44
64
|
|
@@ -59,5 +79,3 @@ module Analytics
|
|
59
79
|
end
|
60
80
|
end
|
61
81
|
|
62
|
-
|
63
|
-
|
metadata
CHANGED
@@ -1,14 +1,14 @@
|
|
1
1
|
--- !ruby/object:Gem::Specification
|
2
2
|
name: api_analytics
|
3
3
|
version: !ruby/object:Gem::Version
|
4
|
-
version: 1.0
|
4
|
+
version: 1.1.0
|
5
5
|
platform: ruby
|
6
6
|
authors:
|
7
7
|
- Tom Draper
|
8
8
|
autorequire:
|
9
9
|
bindir: exe
|
10
10
|
cert_chain: []
|
11
|
-
date: 2023-
|
11
|
+
date: 2023-04-03 00:00:00.000000000 Z
|
12
12
|
dependencies: []
|
13
13
|
description: Monitoring and analytics for API applications.
|
14
14
|
email:
|