amazon-chime-sdk-rails 1.0.0 → 1.1.0
Sign up to get free protection for your applications and to get access to all the features.
- checksums.yaml +4 -4
- data/.github/workflows/build.yml +69 -0
- data/.gitignore +7 -1
- data/CHANGELOG.md +19 -0
- data/Dockerfile +15 -0
- data/Gemfile +5 -3
- data/README.md +132 -1005
- data/docs/Develop_Rails_API_Application.md +569 -0
- data/docs/Develop_Rails_View_Application.md +441 -0
- data/gemfiles/Gemfile.rails-5.0 +1 -1
- data/gemfiles/Gemfile.rails-5.1 +0 -1
- data/gemfiles/Gemfile.rails-5.2 +0 -1
- data/gemfiles/Gemfile.rails-6.0 +0 -1
- data/gemfiles/Gemfile.rails-6.1 +24 -0
- data/gemfiles/Gemfile.rails-7.0 +30 -0
- data/lib/chime_sdk/controller/meetings.rb +4 -3
- data/lib/chime_sdk/version.rb +1 -1
- data/lib/generators/chime_sdk/js_generator.rb +72 -9
- data/lib/generators/templates/controllers/meetings_controller.rb +1 -1
- data/spec/generators/js_generator_spec.rb +66 -6
- data/spec/rails_app/app/assets/config/manifest.js +1 -0
- data/spec/rails_app/app/assets/javascripts/application.js +3 -0
- data/spec/rails_app/app/controllers/api/public/meeting_attendees_controller.rb +11 -0
- data/spec/rails_app/app/controllers/api/public/meetings_controller.rb +15 -0
- data/spec/rails_app/app/controllers/meetings_controller.rb +1 -1
- data/spec/rails_app/app/views/layouts/_header.html.erb +1 -5
- data/spec/rails_app/app/views/layouts/application.html.erb +1 -2
- data/spec/rails_app/app/views/meetings/show.html.erb +22 -2
- data/spec/rails_app/config/application.rb +11 -2
- data/spec/rails_app/config/initializers/devise_token_auth.rb +1 -1
- data/spec/rails_app/config/routes.rb +10 -3
- data/spec/rails_app/db/seeds.rb +1 -1
- data/spec/requests/meetings_spec.rb +1 -1
- data/templates/amazon-chime-sdk-policy.yml +49 -0
- metadata +19 -57
- data/.travis.yml +0 -47
- data/spec/rails_app/app/controllers/spa_controller.rb +0 -6
- data/spec/rails_app/app/javascript/App.vue +0 -50
- data/spec/rails_app/app/javascript/channels/consumer.js +0 -6
- data/spec/rails_app/app/javascript/channels/index.js +0 -5
- data/spec/rails_app/app/javascript/components/DeviseTokenAuth.vue +0 -84
- data/spec/rails_app/app/javascript/components/meetings/Index.vue +0 -100
- data/spec/rails_app/app/javascript/components/meetings/Meeting.vue +0 -178
- data/spec/rails_app/app/javascript/components/rooms/Index.vue +0 -53
- data/spec/rails_app/app/javascript/components/rooms/Show.vue +0 -91
- data/spec/rails_app/app/javascript/packs/application.js +0 -17
- data/spec/rails_app/app/javascript/packs/spa.js +0 -14
- data/spec/rails_app/app/javascript/router/index.js +0 -74
- data/spec/rails_app/app/javascript/store/index.js +0 -37
- data/spec/rails_app/app/views/spa/index.html.erb +0 -1
- data/spec/rails_app/babel.config.js +0 -72
- data/spec/rails_app/bin/webpack +0 -18
- data/spec/rails_app/bin/webpack-dev-server +0 -18
- data/spec/rails_app/config/webpack/development.js +0 -5
- data/spec/rails_app/config/webpack/environment.js +0 -7
- data/spec/rails_app/config/webpack/loaders/vue.js +0 -6
- data/spec/rails_app/config/webpack/production.js +0 -5
- data/spec/rails_app/config/webpack/test.js +0 -5
- data/spec/rails_app/config/webpacker.yml +0 -97
- data/spec/rails_app/package.json +0 -23
- data/spec/rails_app/postcss.config.js +0 -12
@@ -0,0 +1,441 @@
|
|
1
|
+
## Develop your Rails Application with Action View
|
2
|
+
|
3
|
+
Let's start to build simple Rails application with Action View providing real-time communications in a private room.
|
4
|
+
For example, create Rails application using [Devise](https://github.com/heartcombo/devise) for user authentication.
|
5
|
+
|
6
|
+
### Prepare Rails application
|
7
|
+
|
8
|
+
At first, create new Rails application:
|
9
|
+
|
10
|
+
```bash
|
11
|
+
$ rails new chime_view_app
|
12
|
+
$ cd chime_view_app
|
13
|
+
```
|
14
|
+
|
15
|
+
Add gems to your Gemfile:
|
16
|
+
|
17
|
+
```ruby:Gemfile
|
18
|
+
# Gemfile
|
19
|
+
|
20
|
+
gem 'devise'
|
21
|
+
gem 'amazon-chime-sdk-rails'
|
22
|
+
```
|
23
|
+
|
24
|
+
Then, install *devise*:
|
25
|
+
|
26
|
+
```bash
|
27
|
+
$ bundle install
|
28
|
+
$ rails g devise:install
|
29
|
+
$ rails g devise User
|
30
|
+
$ rails g migration add_name_to_users name:string
|
31
|
+
$ rails g devise:views User
|
32
|
+
```
|
33
|
+
|
34
|
+
Update your `application_controller.rb` like this:
|
35
|
+
|
36
|
+
```ruby:app/controllers/application_controller.rb
|
37
|
+
# app/controllers/application_controller.rb
|
38
|
+
|
39
|
+
class ApplicationController < ActionController::Base
|
40
|
+
before_action :configure_permitted_parameters, if: :devise_controller?
|
41
|
+
|
42
|
+
protected
|
43
|
+
def configure_permitted_parameters
|
44
|
+
devise_parameter_sanitizer.permit(:sign_up, keys: [:name])
|
45
|
+
end
|
46
|
+
end
|
47
|
+
```
|
48
|
+
|
49
|
+
Add user name form to your `app/views/users/registrations/new.html.erb` view template like this:
|
50
|
+
|
51
|
+
```erb:app/views/users/registrations/new.html.erb
|
52
|
+
# app/views/users/registrations/new.html.erb
|
53
|
+
|
54
|
+
<div class="field">
|
55
|
+
<%= f.label :name %><br />
|
56
|
+
<%= f.text_field :name, autocomplete: "name" %>
|
57
|
+
</div>
|
58
|
+
|
59
|
+
<div class="field">
|
60
|
+
<%= f.label :email %><br />
|
61
|
+
<%= f.email_field :email, autofocus: true, autocomplete: "email" %>
|
62
|
+
</div>
|
63
|
+
```
|
64
|
+
|
65
|
+
Update *devise* configuration in `devise.rb` to use scoped views:
|
66
|
+
|
67
|
+
```ruby:config/initializers/devise.rb
|
68
|
+
# config/initializers/devise.rb
|
69
|
+
|
70
|
+
Devise.setup do |config|
|
71
|
+
# Uncomment and update
|
72
|
+
config.scoped_views = true
|
73
|
+
end
|
74
|
+
```
|
75
|
+
|
76
|
+
Add login header to your application. Create new `app/views/layouts/_header.html.erb` and update your `app/views/layouts/application.html.erb` like this:
|
77
|
+
|
78
|
+
```erb:app/views/layouts/_header.html.erb
|
79
|
+
# app/views/layouts/_header.html.erb
|
80
|
+
|
81
|
+
<header>
|
82
|
+
<div>
|
83
|
+
<div>
|
84
|
+
<strong>Rails Application for Amazon Chime SDK Meeting (Rails App with Action View)</strong>
|
85
|
+
</div>
|
86
|
+
<div>
|
87
|
+
<% if user_signed_in? %>
|
88
|
+
<%= current_user.name %>
|
89
|
+
<%= link_to 'Logout', destroy_user_session_path, method: :delete %>
|
90
|
+
<% else %>
|
91
|
+
<%= link_to "Sign up", new_user_registration_path %>
|
92
|
+
<%= link_to 'Login', new_user_session_path %>
|
93
|
+
<% end %>
|
94
|
+
</div>
|
95
|
+
</div>
|
96
|
+
</header>
|
97
|
+
```
|
98
|
+
|
99
|
+
```erb:app/views/layouts/application.html.erb
|
100
|
+
# app/views/layouts/application.html.erb
|
101
|
+
|
102
|
+
<!DOCTYPE html>
|
103
|
+
<html>
|
104
|
+
<head>
|
105
|
+
<title>Rails Application for Amazon Chime SDK Meeting</title>
|
106
|
+
<%= csrf_meta_tags %>
|
107
|
+
<%= csp_meta_tag %>
|
108
|
+
|
109
|
+
<%= stylesheet_link_tag 'application', media: 'all', 'data-turbolinks-track': 'reload' %>
|
110
|
+
<%= yield(:javascript_pack_tag) %>
|
111
|
+
<%= javascript_pack_tag 'application', 'data-turbolinks-track': 'reload' %>
|
112
|
+
</head>
|
113
|
+
|
114
|
+
<body>
|
115
|
+
<div id="app">
|
116
|
+
<%= render 'layouts/header' %>
|
117
|
+
<%= yield %>
|
118
|
+
<div>
|
119
|
+
</body>
|
120
|
+
</html>
|
121
|
+
```
|
122
|
+
|
123
|
+
### Create private room functions
|
124
|
+
|
125
|
+
Create MVC by generator:
|
126
|
+
|
127
|
+
```bash
|
128
|
+
$ rails g scaffold room name:string
|
129
|
+
$ rails g scaffold entry room:references user:references
|
130
|
+
$ rake db:migrate
|
131
|
+
```
|
132
|
+
|
133
|
+
Update your `room.rb` like this:
|
134
|
+
|
135
|
+
```ruby:app/models/room.rb
|
136
|
+
# app/models/room.rb
|
137
|
+
|
138
|
+
class Room < ApplicationRecord
|
139
|
+
has_many :entries, dependent: :destroy
|
140
|
+
has_many :members, through: :entries, source: :user
|
141
|
+
|
142
|
+
def member?(user)
|
143
|
+
members.include?(user)
|
144
|
+
end
|
145
|
+
end
|
146
|
+
```
|
147
|
+
|
148
|
+
Add uniqueness validation to your `entry.rb` like this:
|
149
|
+
|
150
|
+
```ruby:app/models/entry.rb
|
151
|
+
# app/models/entry.rb
|
152
|
+
|
153
|
+
class Entry < ApplicationRecord
|
154
|
+
belongs_to :room
|
155
|
+
belongs_to :user
|
156
|
+
# Add uniqueness validation
|
157
|
+
validates :user, uniqueness: { scope: :room }
|
158
|
+
end
|
159
|
+
```
|
160
|
+
|
161
|
+
Update *create* and *destroy* method in your `entries_controller.rb` like this:
|
162
|
+
|
163
|
+
```ruby:app/controllers/entries_controller.rb
|
164
|
+
# app/controllers/entries_controller.rb
|
165
|
+
|
166
|
+
class EntriesController < ApplicationController
|
167
|
+
before_action :authenticate_user!
|
168
|
+
before_action :set_room
|
169
|
+
before_action :set_entry, only: [:destroy]
|
170
|
+
|
171
|
+
# POST /entries
|
172
|
+
# POST /entries.json
|
173
|
+
def create
|
174
|
+
@entry = Entry.new(entry_params)
|
175
|
+
|
176
|
+
respond_to do |format|
|
177
|
+
if @entry.save
|
178
|
+
format.html { redirect_to @room, notice: 'Member was successfully added.' }
|
179
|
+
format.json { render :show, status: :created, location: @room }
|
180
|
+
else
|
181
|
+
format.html { redirect_to @room, notice: @entry.errors }
|
182
|
+
format.json { render json: @entry.errors, status: :unprocessable_entity }
|
183
|
+
end
|
184
|
+
end
|
185
|
+
end
|
186
|
+
|
187
|
+
# DELETE /entries/1
|
188
|
+
# DELETE /entries/1.json
|
189
|
+
def destroy
|
190
|
+
@entry.destroy
|
191
|
+
respond_to do |format|
|
192
|
+
format.html { redirect_to @room, notice: 'Member was successfully removed.' }
|
193
|
+
format.json { head :no_content }
|
194
|
+
end
|
195
|
+
end
|
196
|
+
|
197
|
+
private
|
198
|
+
# Use callbacks to share common setup or constraints between actions.
|
199
|
+
def set_room
|
200
|
+
@room = Room.find(params[:room_id])
|
201
|
+
end
|
202
|
+
|
203
|
+
# Use callbacks to share common setup or constraints between actions.
|
204
|
+
def set_entry
|
205
|
+
@entry = Entry.find(params[:id])
|
206
|
+
end
|
207
|
+
|
208
|
+
# Only allow a list of trusted parameters through.
|
209
|
+
def entry_params
|
210
|
+
params.require(:entry).permit(:room_id, :user_id)
|
211
|
+
end
|
212
|
+
end
|
213
|
+
```
|
214
|
+
|
215
|
+
### Develop meeting functions with amazon-chime-sdk-rails
|
216
|
+
|
217
|
+
Install *amazon-chime-sdk-rails* and generate your controllers by *Controller Generator*:
|
218
|
+
|
219
|
+
```bash
|
220
|
+
$ rails g chime_sdk:install
|
221
|
+
$ rails g chime_sdk:controllers -r room
|
222
|
+
```
|
223
|
+
|
224
|
+
Add and uncomment several functions in generated `meetings_controller.rb` and `meeting_attendees_controller.rb` for your app configurations:
|
225
|
+
|
226
|
+
```ruby:app/controllers/api/meetings_controller.rb
|
227
|
+
# app/controllers/api/meetings_controller.rb
|
228
|
+
|
229
|
+
class MeetingsController < ApplicationController
|
230
|
+
before_action :authenticate_user!
|
231
|
+
before_action :set_room
|
232
|
+
before_action :check_membership
|
233
|
+
|
234
|
+
include ChimeSdk::Controller::Meetings::Mixin
|
235
|
+
|
236
|
+
private
|
237
|
+
# Add
|
238
|
+
def set_room
|
239
|
+
@room = Room.find(params[:room_id])
|
240
|
+
end
|
241
|
+
|
242
|
+
# Add
|
243
|
+
def check_membership
|
244
|
+
unless @room.member?(current_user)
|
245
|
+
message = 'Unauthorized: you are not a member of this private room.'
|
246
|
+
redirect_to @room, notice: message
|
247
|
+
end
|
248
|
+
end
|
249
|
+
|
250
|
+
# Uncomment
|
251
|
+
def meeting_request_id
|
252
|
+
"PrivateRoom-#{@room.id}"
|
253
|
+
end
|
254
|
+
|
255
|
+
# Uncomment
|
256
|
+
def attendee_request_id
|
257
|
+
"User-#{current_user.id}"
|
258
|
+
end
|
259
|
+
|
260
|
+
# Uncomment
|
261
|
+
def application_meeting_metadata(meeting)
|
262
|
+
{
|
263
|
+
"MeetingType": "PrivateRoom",
|
264
|
+
"PrivateRoom": @room
|
265
|
+
}
|
266
|
+
end
|
267
|
+
|
268
|
+
# Uncomment
|
269
|
+
def application_attendee_metadata(attendee)
|
270
|
+
user_id = attendee[:Attendee][:ExternalUserId].split('-')[3]
|
271
|
+
{
|
272
|
+
"AttendeeType": "User",
|
273
|
+
"User": User.find_by_id(user_id)
|
274
|
+
}
|
275
|
+
end
|
276
|
+
|
277
|
+
# Uncomment
|
278
|
+
def application_attendee_metadata(attendee)
|
279
|
+
user_id = attendee[:Attendee][:ExternalUserId].split('-')[3]
|
280
|
+
{
|
281
|
+
"AttendeeType": "User",
|
282
|
+
"User": User.find_by_id(user_id)
|
283
|
+
}
|
284
|
+
end
|
285
|
+
end
|
286
|
+
```
|
287
|
+
|
288
|
+
```ruby:app/controllers/api/meeting_attendees_controller.rb
|
289
|
+
# app/controllers/api/meeting_attendees_controller.rb
|
290
|
+
|
291
|
+
class MeetingAttendeesController < ApplicationController
|
292
|
+
before_action :authenticate_user!
|
293
|
+
before_action :set_room
|
294
|
+
before_action :check_membership
|
295
|
+
|
296
|
+
include ChimeSdk::Controller::Attendees::Mixin
|
297
|
+
|
298
|
+
private
|
299
|
+
# Add
|
300
|
+
def set_room
|
301
|
+
@room = Room.find(params[:room_id])
|
302
|
+
end
|
303
|
+
|
304
|
+
# Add
|
305
|
+
def check_membership
|
306
|
+
unless @room.member?(current_user)
|
307
|
+
message = 'Unauthorized: you are not a member of this private room.'
|
308
|
+
redirect_to @room, notice: message
|
309
|
+
end
|
310
|
+
end
|
311
|
+
|
312
|
+
# Uncomment
|
313
|
+
def attendee_request_id
|
314
|
+
"User-#{current_user.id}"
|
315
|
+
end
|
316
|
+
|
317
|
+
# Uncomment
|
318
|
+
def application_attendee_metadata(attendee)
|
319
|
+
user_id = attendee[:Attendee][:ExternalUserId].split('-')[3]
|
320
|
+
{
|
321
|
+
"AttendeeType": "User",
|
322
|
+
"User": User.find_by_id(user_id)
|
323
|
+
}
|
324
|
+
end
|
325
|
+
end
|
326
|
+
```
|
327
|
+
|
328
|
+
Bundle Amazon Chime SDK into single amazon-chime-sdk.min.js file and copy it to *app/assets/javascripts* by *Single Javascript Generator* in *amazon-chime-sdk-rails*:
|
329
|
+
|
330
|
+
```bash
|
331
|
+
$ rails g chime_sdk:js
|
332
|
+
```
|
333
|
+
|
334
|
+
Add *amazon-chime-sdk.min.js* to your Asset Pipeline:
|
335
|
+
|
336
|
+
```ruby:config/initializers/assets.rb
|
337
|
+
# config/initializers/assets.rb
|
338
|
+
|
339
|
+
Rails.application.config.assets.precompile += %w( amazon-chime-sdk.min.js )
|
340
|
+
```
|
341
|
+
|
342
|
+
Then, generate meeting views by *View Generator* in *amazon-chime-sdk-rails*:
|
343
|
+
|
344
|
+
```bash
|
345
|
+
$ rails g chime_sdk:views
|
346
|
+
```
|
347
|
+
|
348
|
+
Simply customize your meeting view generated *app/views/meetings/show.html.erb*:
|
349
|
+
|
350
|
+
```javascript
|
351
|
+
// app/views/meetings/show.html.erb
|
352
|
+
|
353
|
+
function showApplicationUserName(attendee) {
|
354
|
+
// Comment
|
355
|
+
// return attendee.Attendee.AttendeeId;
|
356
|
+
// Uncomment
|
357
|
+
return `${attendee.Attendee.ApplicationMetadata.User.name} (${attendee.Attendee.AttendeeId})`;
|
358
|
+
}
|
359
|
+
```
|
360
|
+
|
361
|
+
Add member management and meeting link to your room view:
|
362
|
+
|
363
|
+
```erb:app/views/rooms/show.html.erb
|
364
|
+
# app/views/rooms/show.html.erb
|
365
|
+
|
366
|
+
<p id="notice"><%= notice %></p>
|
367
|
+
|
368
|
+
<p>
|
369
|
+
<strong>Name:</strong>
|
370
|
+
<%= @room.name %>
|
371
|
+
</p>
|
372
|
+
|
373
|
+
<p>
|
374
|
+
<strong>Private Meeting:</strong>
|
375
|
+
<p><%= link_to 'Show Meetings', room_meetings_path(@room) %></p>
|
376
|
+
<p><%= link_to 'Join the Meeting', room_meetings_path(@room), method: :post %></p>
|
377
|
+
</p>
|
378
|
+
|
379
|
+
<p>
|
380
|
+
<strong>Members:</strong>
|
381
|
+
<table>
|
382
|
+
<tbody>
|
383
|
+
<% @room.entries.each do |entry| %>
|
384
|
+
<tr>
|
385
|
+
<td><%= entry.user.name %></td>
|
386
|
+
<td><%= link_to 'Remove', [@room, entry], method: :delete, data: { confirm: 'Are you sure?' } %></td>
|
387
|
+
</tr>
|
388
|
+
<% end %>
|
389
|
+
</tbody>
|
390
|
+
</table>
|
391
|
+
</p>
|
392
|
+
|
393
|
+
<p>
|
394
|
+
<strong>Add members:</strong>
|
395
|
+
<%= form_for [@room, Entry.new] do |f| %>
|
396
|
+
<%= f.hidden_field :room_id, value: @room.id %>
|
397
|
+
<%= f.collection_select :user_id, User.all, :id, :name %>
|
398
|
+
<%= f.submit "Add" %>
|
399
|
+
<% end %>
|
400
|
+
</p>
|
401
|
+
|
402
|
+
<%= link_to 'Edit', edit_room_path(@room) %> |
|
403
|
+
<%= link_to 'Back', rooms_path %>
|
404
|
+
```
|
405
|
+
|
406
|
+
Update your `routes.rb` like this:
|
407
|
+
|
408
|
+
```ruby:config/routes.rb
|
409
|
+
# config/routes.rb
|
410
|
+
|
411
|
+
Rails.application.routes.draw do
|
412
|
+
root "rooms#index"
|
413
|
+
devise_for :users
|
414
|
+
resources :rooms do
|
415
|
+
resources :entries, only: [:create, :destroy]
|
416
|
+
resources :meetings, only: [:index, :show, :create, :destroy] do
|
417
|
+
resources :meeting_attendees, as: :attendees, path: :attendees, only: [:index, :show]
|
418
|
+
end
|
419
|
+
end
|
420
|
+
end
|
421
|
+
```
|
422
|
+
|
423
|
+
Note that you need to set up AWS credentials or IAM role for *amazon-chime-sdk-rails*. See [Set up AWS credentials](#set-up-aws-credentials) for more details.
|
424
|
+
|
425
|
+
Finally, start rails server:
|
426
|
+
|
427
|
+
```bash
|
428
|
+
$ rails s
|
429
|
+
```
|
430
|
+
|
431
|
+
Now ready to take off! Open *http://localhost:3000/* in your browser. Sign up users from *Sign up* header. For example, sign up *ichiro* as *ichiro@example.com* and *stephen* as *stephen@example.com*. Then, create a new room and add *ichiro* and *stephen* as room members.
|
432
|
+
|
433
|
+
Now you can create a new meeting from room view.
|
434
|
+
|
435
|
+
<kbd>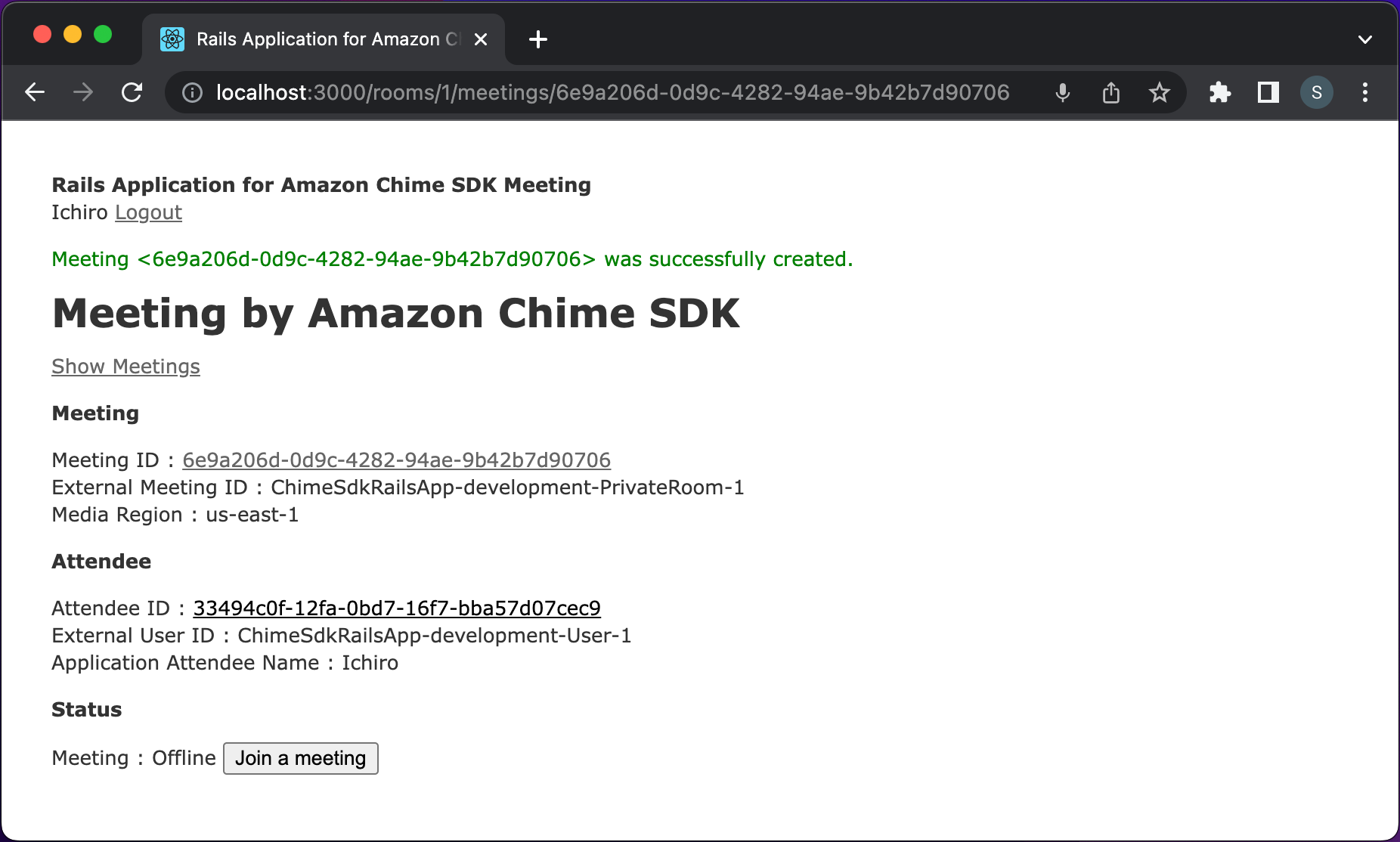</kbd>
|
436
|
+
|
437
|
+
After creating a new meeting from any private room, you can join the meeting from "*Join the Meeting*" button in your meeting view. Your rails application includes simple online meeting implementation using [Amazon Chime SDK](https://aws.amazon.com/chime/chime-sdk) as a rails view.
|
438
|
+
|
439
|
+
<kbd>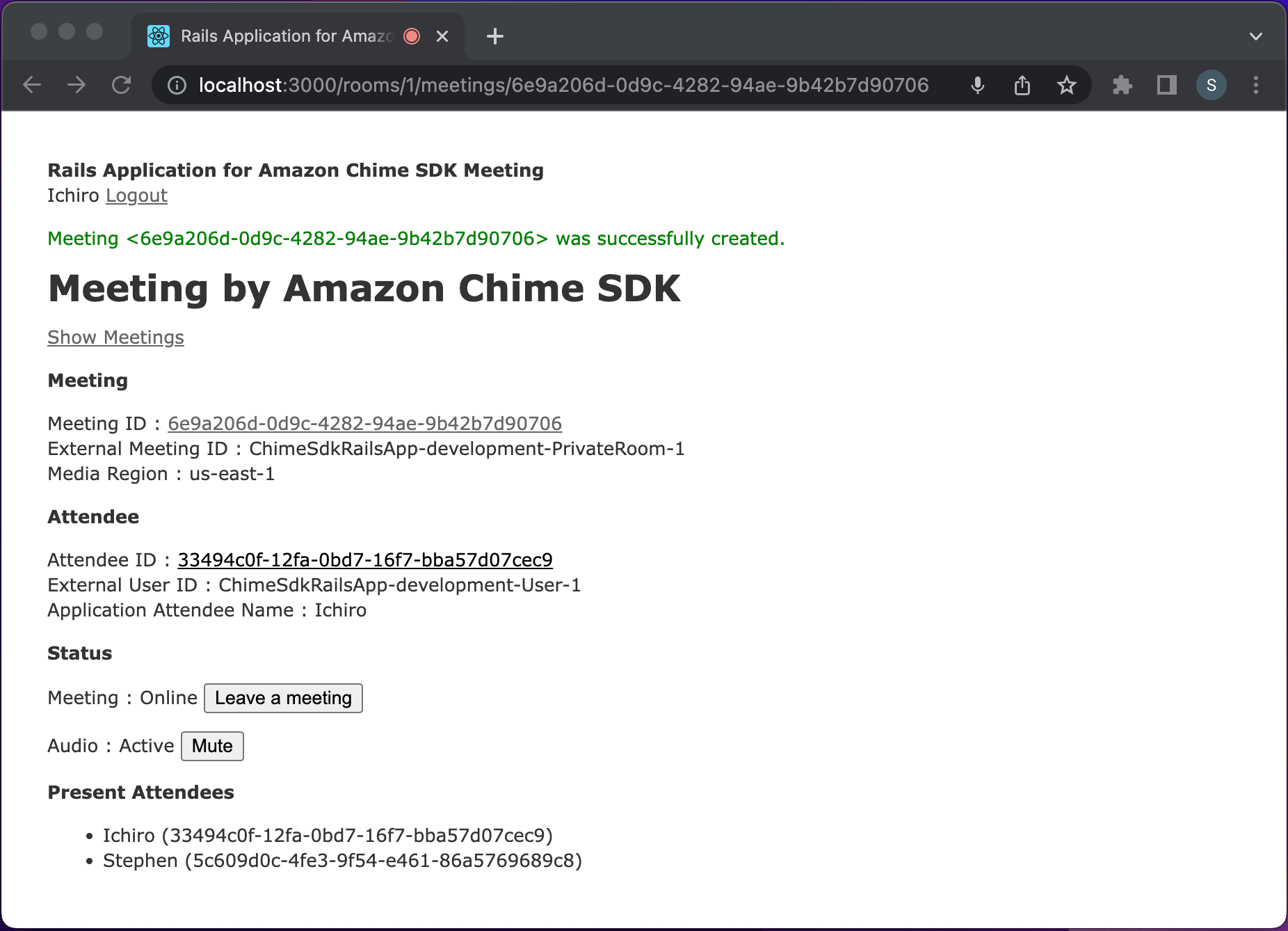</kbd>
|
440
|
+
|
441
|
+
You can also customize your meeting view using [Amazon Chime SDK for JavaScript](https://github.com/aws/amazon-chime-sdk-js). Enjoy your application development!
|
data/gemfiles/Gemfile.rails-5.0
CHANGED
data/gemfiles/Gemfile.rails-5.1
CHANGED
data/gemfiles/Gemfile.rails-5.2
CHANGED
data/gemfiles/Gemfile.rails-6.0
CHANGED
@@ -0,0 +1,24 @@
|
|
1
|
+
source 'https://rubygems.org'
|
2
|
+
|
3
|
+
gemspec path: '../'
|
4
|
+
|
5
|
+
# Bundle Rails
|
6
|
+
gem 'rails', '~> 6.1.0'
|
7
|
+
|
8
|
+
gem 'sqlite3'
|
9
|
+
gem 'puma'
|
10
|
+
gem 'sass-rails'
|
11
|
+
gem 'turbolinks'
|
12
|
+
gem 'jbuilder'
|
13
|
+
|
14
|
+
group :development do
|
15
|
+
gem 'web-console'
|
16
|
+
gem 'listen'
|
17
|
+
gem 'spring'
|
18
|
+
gem 'spring-watcher-listen'
|
19
|
+
end
|
20
|
+
|
21
|
+
group :test do
|
22
|
+
# gem 'coveralls', require: false
|
23
|
+
gem 'coveralls_reborn', require: false
|
24
|
+
end
|
@@ -0,0 +1,30 @@
|
|
1
|
+
source 'https://rubygems.org'
|
2
|
+
|
3
|
+
gemspec path: '../'
|
4
|
+
|
5
|
+
# Bundle Rails
|
6
|
+
gem 'rails', '~> 7.0.0'
|
7
|
+
# https://github.com/lynndylanhurley/devise_token_auth/pull/1517
|
8
|
+
gem 'devise_token_auth', git: 'https://github.com/lynndylanhurley/devise_token_auth.git'
|
9
|
+
# Tentative measure for 'check_for_activated_spec!' with default gem in Ruby 3.0.3
|
10
|
+
gem 'digest', '3.0.0'
|
11
|
+
gem 'io-wait', '0.2.0'
|
12
|
+
|
13
|
+
gem 'sqlite3'
|
14
|
+
gem 'puma'
|
15
|
+
gem 'sass-rails'
|
16
|
+
gem 'turbolinks'
|
17
|
+
gem 'jbuilder'
|
18
|
+
gem 'sprockets-rails'
|
19
|
+
|
20
|
+
group :development do
|
21
|
+
gem 'web-console'
|
22
|
+
gem 'listen'
|
23
|
+
gem 'spring'
|
24
|
+
gem 'spring-watcher-listen', git: 'https://github.com/rails/spring-watcher-listen.git'
|
25
|
+
end
|
26
|
+
|
27
|
+
group :test do
|
28
|
+
# gem 'coveralls', require: false
|
29
|
+
gem 'coveralls_reborn', require: false
|
30
|
+
end
|
@@ -18,7 +18,7 @@ module ChimeSdk
|
|
18
18
|
# @param [Hash] params Request parameter options
|
19
19
|
# @option params [String] :create_meeting (ChimeSdk.config.create_meeting_by_get_request) Whether the application creates meeting in this meetings#index action by HTTP GET request
|
20
20
|
def index
|
21
|
-
if params[:create_meeting].to_s.to_boolean(false) || ChimeSdk.config.create_meeting_by_get_request
|
21
|
+
if params[:create_meeting].to_s.to_boolean(false) || ChimeSdk.config.create_meeting_by_get_request && params[:create_meeting].to_s.to_boolean(true)
|
22
22
|
create
|
23
23
|
else
|
24
24
|
list_meetings
|
@@ -36,7 +36,8 @@ module ChimeSdk
|
|
36
36
|
# @option params [String] :create_attendee_from_meeting (ChimeSdk.config.create_attendee_from_meeting) Whether the application creates attendee from meeting in meetings#show action
|
37
37
|
def show
|
38
38
|
get_meeting
|
39
|
-
if params[:create_attendee_from_meeting].to_s.to_boolean(false) || ChimeSdk.config.create_attendee_from_meeting
|
39
|
+
if params[:create_attendee_from_meeting].to_s.to_boolean(false) || ChimeSdk.config.create_attendee_from_meeting && params[:create_attendee_from_meeting].to_s.to_boolean(true)
|
40
|
+
puts "create_attendee_from_meeting"
|
40
41
|
create_attendee_from_meeting
|
41
42
|
end
|
42
43
|
respond_to do |format|
|
@@ -51,7 +52,7 @@ module ChimeSdk
|
|
51
52
|
# @param [Hash] params Request parameter options
|
52
53
|
# @option params [String] :create_meeting_with_attendee (ChimeSdk.config.create_meeting_with_attendee) Whether the application creates meeting with attendee in this meetings#create action
|
53
54
|
def create
|
54
|
-
if params[:create_meeting_with_attendee].to_s.to_boolean(false) || ChimeSdk.config.create_meeting_with_attendee
|
55
|
+
if params[:create_meeting_with_attendee].to_s.to_boolean(false) || ChimeSdk.config.create_meeting_with_attendee && params[:create_meeting_with_attendee].to_s.to_boolean(true)
|
55
56
|
create_meeting_with_attendee
|
56
57
|
else
|
57
58
|
create_meeting
|
data/lib/chime_sdk/version.rb
CHANGED
@@ -4,9 +4,12 @@ module ChimeSdk
|
|
4
4
|
module Generators
|
5
5
|
# Amazon Chime SDK single .js file generator.
|
6
6
|
# Bundle Amazon Chime SDK into single amazon-chime-sdk.min.js file and copy it to app/assets/javascripts directory.
|
7
|
-
# @example Run Amazon Chime SDK single .js file generator
|
7
|
+
# @example Run Amazon Chime SDK single .js file generator with the latest version from master branch
|
8
8
|
# rails generate chime_sdk:js
|
9
|
-
# @
|
9
|
+
# @example Run Amazon Chime SDK single .js file generator with specified version
|
10
|
+
# rails generate chime_sdk:js 2.24.0
|
11
|
+
# @see https://github.com/aws-samples/amazon-chime-sdk/tree/main/utils/singlejs
|
12
|
+
# @see https://www.npmjs.com/package/amazon-chime-sdk-js
|
10
13
|
class JsGenerator < Rails::Generators::Base
|
11
14
|
desc <<-DESC.strip_heredoc
|
12
15
|
Bundle Amazon Chime SDK into single amazon-chime-sdk.min.js file and copy it to app/assets/javascripts directory.
|
@@ -15,11 +18,17 @@ module ChimeSdk
|
|
15
18
|
|
16
19
|
rails generate chime_sdk:js
|
17
20
|
|
21
|
+
You can also specify version of amazon-chime-sdk-js like this:
|
22
|
+
|
23
|
+
rails generate chime_sdk:js 2.24.0
|
24
|
+
|
18
25
|
This generator requires npm installation.
|
19
26
|
|
20
27
|
DESC
|
21
28
|
|
22
29
|
source_root File.expand_path('./')
|
30
|
+
argument :version, required: false,
|
31
|
+
desc: "Specific version of amazon-chime-sdk-js, e.g. 2.24.0"
|
23
32
|
|
24
33
|
# Build amazon-chime-sdk.min.js and copy it to app/assets/javascripts directory
|
25
34
|
def build_and_copy_chime_sdk_js
|
@@ -48,17 +57,71 @@ module ChimeSdk
|
|
48
57
|
end
|
49
58
|
# :nocov:
|
50
59
|
|
51
|
-
|
60
|
+
if version.present?
|
61
|
+
begin
|
62
|
+
sdk_version = Gem::Version.new(version)
|
63
|
+
# https://www.npmjs.com/package/amazon-chime-sdk-js
|
64
|
+
if sdk_version < Gem::Version.new("1.0.0")
|
65
|
+
puts "[Abort] Specify 1.0.0 or later as amazon-chime-sdk-js version"
|
66
|
+
exit
|
67
|
+
else
|
68
|
+
version_tag = "amazon-chime-sdk-js@#{sdk_version}"
|
69
|
+
end
|
70
|
+
rescue StandardError => e
|
71
|
+
puts "[Abort] Wrong amazon-chime-sdk-js version was specified"
|
72
|
+
exit
|
73
|
+
end
|
74
|
+
end
|
75
|
+
|
76
|
+
system "mkdir -p tmp"
|
52
77
|
puts "Cloning into 'amazon-chime-sdk-js' git repository in tmp directory ..."
|
53
|
-
|
78
|
+
system "cd tmp; git clone https://github.com/aws-samples/amazon-chime-sdk.git > /dev/null 2>&1"
|
79
|
+
repository_path = "tmp/amazon-chime-sdk"
|
80
|
+
singlejs_path = "#{repository_path}/utils/singlejs"
|
81
|
+
package_json_path = "#{singlejs_path}/package.json"
|
82
|
+
|
83
|
+
puts "Finding amazon-chime-sdk-js version ..."
|
84
|
+
chime_sdk_pattern = /\"amazon-chime-sdk-js\":[\s]*\"([\S]*)\"$/
|
85
|
+
buffer = File.open(package_json_path, "r") { |f| f.read() }
|
86
|
+
if version_tag.present?
|
87
|
+
puts " Specified \"#{sdk_version}\" as an argument"
|
88
|
+
if `npm info #{version_tag} version`.present?
|
89
|
+
puts " #{version_tag} was found as npm package"
|
90
|
+
if buffer =~ chime_sdk_pattern
|
91
|
+
buffer.gsub!(chime_sdk_pattern, "\"amazon-chime-sdk-js\": \"#{sdk_version}\"")
|
92
|
+
File.open(package_json_path, "w") { |f| f.write(buffer) }
|
93
|
+
puts " Replaced amazon-chime-sdk-js version into \"#{sdk_version}\" in package.json"
|
94
|
+
puts " amazon-chime-sdk-js \"#{sdk_version}\" will be used"
|
95
|
+
else
|
96
|
+
# :nocov:
|
97
|
+
puts "[Abort] amazon-chime-sdk-js was not found in package.json"
|
98
|
+
exit
|
99
|
+
# :nocov:
|
100
|
+
end
|
101
|
+
else
|
102
|
+
puts "[Abort] No npm package of #{version_tag} was found. Specify different amazon-chime-sdk-js version."
|
103
|
+
exit
|
104
|
+
end
|
105
|
+
else
|
106
|
+
if buffer =~ /\"amazon-chime-sdk-js\":[\s]*\"([\S]*)\"$/
|
107
|
+
sdk_version = $1
|
108
|
+
puts " amazon-chime-sdk-js \"#{sdk_version}\" was found in package.json"
|
109
|
+
puts " amazon-chime-sdk-js \"#{sdk_version}\" will be used"
|
110
|
+
else
|
111
|
+
# :nocov:
|
112
|
+
puts " No amazon-chime-sdk-js was found in package.json"
|
113
|
+
# :nocov:
|
114
|
+
end
|
115
|
+
end
|
116
|
+
|
54
117
|
puts "Running 'npm install @rollup/plugin-commonjs' in the repository ..."
|
55
|
-
|
118
|
+
system "cd #{singlejs_path}; npm install @rollup/plugin-commonjs > /dev/null 2>&1"
|
56
119
|
puts "Running 'npm run bundle' in the repository ..."
|
57
|
-
|
120
|
+
system "cd #{singlejs_path}; npm run bundle > /dev/null 2>&1"
|
58
121
|
puts "Built Amazon Chime SDK as amazon-chime-sdk.min.js"
|
59
|
-
copy_file "
|
60
|
-
copy_file "
|
61
|
-
|
122
|
+
copy_file "#{singlejs_path}/build/amazon-chime-sdk.min.js", "app/assets/javascripts/amazon-chime-sdk.min.js"
|
123
|
+
copy_file "#{singlejs_path}/build/amazon-chime-sdk.min.js.map", "app/assets/javascripts/amazon-chime-sdk.min.js.map"
|
124
|
+
system "rm -rf #{repository_path}"
|
62
125
|
puts "Cleaned up the repository in tmp directory"
|
63
126
|
puts "Completed"
|
64
127
|
end
|