adama 0.1.4 → 0.1.5
This diff represents the content of publicly available package versions that have been released to one of the supported registries. The information contained in this diff is provided for informational purposes only and reflects changes between package versions as they appear in their respective public registries.
- checksums.yaml +4 -4
- data/README.md +22 -0
- data/adama.gemspec +1 -1
- data/lib/adama/command.rb +2 -3
- data/lib/adama/invoker.rb +11 -6
- data/lib/adama/version.rb +1 -1
- metadata +4 -4
checksums.yaml
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
---
|
2
2
|
SHA1:
|
3
|
-
metadata.gz:
|
4
|
-
data.tar.gz:
|
3
|
+
metadata.gz: d32465d56c5a8f938f26e1732fb75a786e22940d
|
4
|
+
data.tar.gz: fa589325c1a750016e692f0b73452b12f0b12250
|
5
5
|
SHA512:
|
6
|
-
metadata.gz:
|
7
|
-
data.tar.gz:
|
6
|
+
metadata.gz: 8c670d242b889c5ddc751e5fe9e7827401587d92c2fc50a01b3d3ef86f19b7af38aaae7c6795658bec8a051bdd45c086f3f5a7e02e3b8e5e67cbcf0ac74c0c01
|
7
|
+
data.tar.gz: 39cf0f7723a3005112c68ccb4a0ffc2b106d8a2448a426cf03f853ee002926b11623b878eab75ff3fead81a9b1c88b115d6adf469557a41c2392c2f6b91bb894
|
data/README.md
CHANGED
@@ -1,5 +1,7 @@
|
|
1
1
|
# Commander Adama
|
2
2
|
|
3
|
+
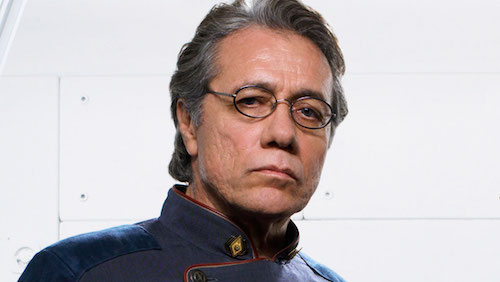
|
4
|
+
|
3
5
|
Adama is a bare bones command pattern library inspired by Collective Idea's [Interactor](https://github.com/collectiveidea/interactor) gem.
|
4
6
|
|
5
7
|
Commands are small classes that represent individual units of work. Each command is executed by a client "calling" it. An invoker is a class responsible for the execution of one or more commands.
|
@@ -86,6 +88,26 @@ Now, when you run `RebuildHumanRace.call(captain: :apollo, president: :laura)` i
|
|
86
88
|
|
87
89
|
If there is an error in any of those commands, the invoker will call `FindEarth.rollback`, then `DestroyCylons.rollback`, then `GetArrowOfApollo.rollback` leaving everything just as it was in the beginning.
|
88
90
|
|
91
|
+
#### Instance Invoker List
|
92
|
+
|
93
|
+
Typically your Invoker class takes responsibility for an immutable set of actions, however sometimes it's handy to be able to adjust the invoked commands on the fly while keeping the error handling and rollback functionality of the invoker.
|
94
|
+
|
95
|
+
e.g.
|
96
|
+
|
97
|
+
```ruby
|
98
|
+
class FightCylons
|
99
|
+
include Adama::Invoker
|
100
|
+
end
|
101
|
+
|
102
|
+
attack1 = Invoker.new(captain: :apollo, lieutenant: :starbuck).invoke(Advance, Strafe, Fire)
|
103
|
+
attack2 = Invoker.new(captain: :apollo, lieutenant: :starbuck).invoke(Advance, Fire)
|
104
|
+
|
105
|
+
attack1.run
|
106
|
+
attack2.run
|
107
|
+
```
|
108
|
+
|
109
|
+
It's important to see that we're using the `#run` instance method on the Invoker instance (as the `.call` class method would). This ensures we execute the invoker in the error / rollback handler. Calling the `#call` instance method directly would simply execute each command, without any Invoker level error handling.
|
110
|
+
|
89
111
|
### Errors
|
90
112
|
|
91
113
|
`Adama::Command#call` or `Adama::Invoker#call` will *always* raise an error of type `Adama::Errors::BaseError`.
|
data/adama.gemspec
CHANGED
@@ -30,7 +30,7 @@ Gem::Specification.new do |spec|
|
|
30
30
|
spec.executables = spec.files.grep(%r{^exe/}) { |f| File.basename(f) }
|
31
31
|
spec.require_paths = ["lib"]
|
32
32
|
|
33
|
-
spec.add_development_dependency "bundler", "~>
|
33
|
+
spec.add_development_dependency "bundler", "~> 2.0"
|
34
34
|
spec.add_development_dependency "rake", "~> 10.0"
|
35
35
|
spec.add_development_dependency "rspec", "~> 3.0"
|
36
36
|
spec.add_development_dependency "pry"
|
data/lib/adama/command.rb
CHANGED
@@ -36,13 +36,12 @@ module Adama
|
|
36
36
|
# Internal instance method. Called by both the call class method, and by
|
37
37
|
# the call method in the invoker. If it fails it raises a CommandError.
|
38
38
|
def run
|
39
|
-
|
40
|
-
call
|
39
|
+
tap(&:call)
|
41
40
|
rescue => error
|
42
41
|
raise Errors::CommandError.new(
|
43
42
|
error: error,
|
44
43
|
command: self,
|
45
|
-
backtrace: error.backtrace
|
44
|
+
backtrace: error.backtrace
|
46
45
|
)
|
47
46
|
end
|
48
47
|
|
data/lib/adama/invoker.rb
CHANGED
@@ -22,7 +22,7 @@ module Adama
|
|
22
22
|
include Command
|
23
23
|
|
24
24
|
# Our new class methods enable us to set the command list
|
25
|
-
extend
|
25
|
+
extend InvokeMethods
|
26
26
|
|
27
27
|
# We override the Command class instance methods:
|
28
28
|
#
|
@@ -30,11 +30,12 @@ module Adama
|
|
30
30
|
# call
|
31
31
|
# rollback
|
32
32
|
include InstanceMethods
|
33
|
+
include InvokeMethods
|
33
34
|
end
|
34
35
|
end
|
35
36
|
|
36
37
|
# Our new class methods enable us to set the command list
|
37
|
-
module
|
38
|
+
module InvokeMethods
|
38
39
|
|
39
40
|
# Public class method. Call invoke in your class definition to
|
40
41
|
# specify which commands will be executed.
|
@@ -49,6 +50,10 @@ module Adama
|
|
49
50
|
# )
|
50
51
|
# end
|
51
52
|
def invoke(*command_list)
|
53
|
+
if is_a?(Invoker) && self.class.commands.any?
|
54
|
+
raise(StandardError, 'Can\'t call invoke on an Invoker instance \
|
55
|
+
with a class invocation list.')
|
56
|
+
end
|
52
57
|
@commands = command_list.flatten
|
53
58
|
end
|
54
59
|
|
@@ -69,15 +74,14 @@ module Adama
|
|
69
74
|
# invoker "call" instance method, we won't have access to error's
|
70
75
|
# command so need to test for it's existence.
|
71
76
|
def run
|
72
|
-
|
73
|
-
call
|
77
|
+
tap(&:call)
|
74
78
|
rescue => error
|
75
79
|
rollback
|
76
80
|
raise Errors::InvokerError.new(
|
77
81
|
error: error,
|
78
82
|
command: error.respond_to?(:command) ? error.command : nil,
|
79
83
|
invoker: self,
|
80
|
-
backtrace: error.backtrace
|
84
|
+
backtrace: error.backtrace
|
81
85
|
)
|
82
86
|
end
|
83
87
|
|
@@ -103,7 +107,8 @@ module Adama
|
|
103
107
|
# Iterate over the commands array, instantiate each command, run it,
|
104
108
|
# and add it to the called list.
|
105
109
|
def call
|
106
|
-
self.
|
110
|
+
commands = self.commands.any? ? self.commands : self.class.commands
|
111
|
+
commands.each do |command_klass|
|
107
112
|
command = command_klass.new(kwargs)
|
108
113
|
command.run
|
109
114
|
_called << command
|
data/lib/adama/version.rb
CHANGED
metadata
CHANGED
@@ -1,14 +1,14 @@
|
|
1
1
|
--- !ruby/object:Gem::Specification
|
2
2
|
name: adama
|
3
3
|
version: !ruby/object:Gem::Version
|
4
|
-
version: 0.1.
|
4
|
+
version: 0.1.5
|
5
5
|
platform: ruby
|
6
6
|
authors:
|
7
7
|
- dradford
|
8
8
|
autorequire:
|
9
9
|
bindir: exe
|
10
10
|
cert_chain: []
|
11
|
-
date:
|
11
|
+
date: 2019-03-12 00:00:00.000000000 Z
|
12
12
|
dependencies:
|
13
13
|
- !ruby/object:Gem::Dependency
|
14
14
|
name: bundler
|
@@ -16,14 +16,14 @@ dependencies:
|
|
16
16
|
requirements:
|
17
17
|
- - "~>"
|
18
18
|
- !ruby/object:Gem::Version
|
19
|
-
version: '
|
19
|
+
version: '2.0'
|
20
20
|
type: :development
|
21
21
|
prerelease: false
|
22
22
|
version_requirements: !ruby/object:Gem::Requirement
|
23
23
|
requirements:
|
24
24
|
- - "~>"
|
25
25
|
- !ruby/object:Gem::Version
|
26
|
-
version: '
|
26
|
+
version: '2.0'
|
27
27
|
- !ruby/object:Gem::Dependency
|
28
28
|
name: rake
|
29
29
|
requirement: !ruby/object:Gem::Requirement
|