zlog 0.7 → 0.8
Sign up to get free protection for your applications and to get access to all the features.
- checksums.yaml +4 -4
- data/CHANGELOG.md +6 -0
- data/Gemfile +1 -0
- data/README.md +11 -3
- data/bin/zlog +52 -0
- data/lib/zlog/cli.rb +35 -0
- data/lib/zlog/zlog.rb +54 -3
- data/test/classes/zlog_layouts_simple_spec.rb +8 -8
- data/test/classes/zlog_layouts_simplenamed_spec.rb +8 -8
- data/test/classes/zlog_spec.rb +56 -0
- metadata +6 -3
checksums.yaml
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
---
|
2
2
|
SHA1:
|
3
|
-
metadata.gz:
|
4
|
-
data.tar.gz:
|
3
|
+
metadata.gz: 95b3fd6c53f48770f2b2751940346071debc8dc4
|
4
|
+
data.tar.gz: 3954a451f924c658cb5a3a0df8b0db729a1bfc3e
|
5
5
|
SHA512:
|
6
|
-
metadata.gz:
|
7
|
-
data.tar.gz:
|
6
|
+
metadata.gz: 45b5d98da16c8d21d224ace756249d056be82d7f1c589c147879cb4ab9db9fae2b7b03ae6ccdf8e07ff46014833cc364cdb63776d12bc499a10e8fa00363dc3f
|
7
|
+
data.tar.gz: fd5cbfcfc6f24749f0adb8d2be32d9dd12daf0c040847b7355c40dc54a2d68963a7fb3f3aa3af86bf2a7baad8426172929abd352e8681fd9c14dc834d123b6b9
|
data/CHANGELOG.md
CHANGED
@@ -1,3 +1,9 @@
|
|
1
|
+
## 0.8
|
2
|
+
|
3
|
+
* feature: support reading log files for zlog formatter
|
4
|
+
* feature: added convertor from json (log file format) to logevent in zlog
|
5
|
+
* feature: get a writable logfile from a list of candidates or generate a random one
|
6
|
+
|
1
7
|
## 0.7
|
2
8
|
|
3
9
|
* improvement: correctly ordered log levels (now: ... warn ok section error ...)
|
data/Gemfile
CHANGED
data/README.md
CHANGED
@@ -8,12 +8,12 @@ Logging configuration on top of ruby's `logging` gem (see [github](https://githu
|
|
8
8
|
* supports continuous logging (eg progress indicators which you don't want to clutter your commandline)
|
9
9
|
* added section and ok log types
|
10
10
|
|
11
|
-
|
11
|
+
## requirements
|
12
12
|
|
13
13
|
* gems:
|
14
14
|
* logging
|
15
15
|
|
16
|
-
|
16
|
+
## installation
|
17
17
|
|
18
18
|
From rubygems:
|
19
19
|
|
@@ -23,7 +23,7 @@ From source:
|
|
23
23
|
|
24
24
|
gem build *.gemspec && gem install *gem
|
25
25
|
|
26
|
-
|
26
|
+
## example
|
27
27
|
|
28
28
|
Code:
|
29
29
|
|
@@ -42,4 +42,12 @@ Code:
|
|
42
42
|
|
43
43
|
See the `example` folder for more.
|
44
44
|
|
45
|
+
## utility
|
46
|
+
|
47
|
+
You can use `zlog` to read and format json-based log files:
|
48
|
+
|
49
|
+
cat file.log | zlog
|
50
|
+
|
51
|
+
It will print out a formatted representation of the log.
|
52
|
+
|
45
53
|
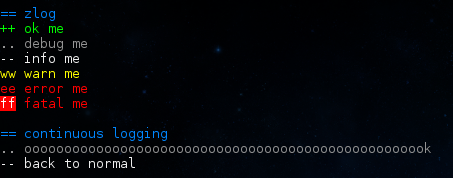
|
data/bin/zlog
ADDED
@@ -0,0 +1,52 @@
|
|
1
|
+
#!/usr/bin/env ruby
|
2
|
+
require 'zlog/cli'
|
3
|
+
require 'optparse'
|
4
|
+
|
5
|
+
Zlog.init_stdout
|
6
|
+
Log = Logging.logger['zlog']
|
7
|
+
|
8
|
+
# Define the options for commandline interaction
|
9
|
+
options = {}
|
10
|
+
opt = OptionParser.new do |opts|
|
11
|
+
opts.banner = "usage: zlog [options]\n cat my.log | zlog [options]"
|
12
|
+
|
13
|
+
opts.on("-f", "--file FILE", "Read log from file") do |file|
|
14
|
+
options[:file] = file
|
15
|
+
end
|
16
|
+
|
17
|
+
opts.on("-s", "--simple", "Print simple layout") do |v|
|
18
|
+
options[:layout] = :simple
|
19
|
+
end
|
20
|
+
|
21
|
+
opts.on("-n", "--named", "Print simple layout with names") do |v|
|
22
|
+
options[:layout] = :named
|
23
|
+
end
|
24
|
+
end
|
25
|
+
opt.parse!
|
26
|
+
|
27
|
+
# create the commandline object for zlog
|
28
|
+
cli = Zlog::CLI.new options
|
29
|
+
|
30
|
+
# tiny print helper for reuse
|
31
|
+
print_out = lambda{ |line| puts line }
|
32
|
+
|
33
|
+
# if we are part of a pipe (eg: cat file.log | zlog), read input from stdin
|
34
|
+
# otherwise just try to read from the provided file parameter
|
35
|
+
if STDIN.tty?
|
36
|
+
# check if we have a file parameter provided
|
37
|
+
if options[:file].nil?
|
38
|
+
Log.error "you must provide at least a file parameter"
|
39
|
+
puts opt
|
40
|
+
exit 0
|
41
|
+
end
|
42
|
+
# verify that the file exists
|
43
|
+
if not File::file? options[:file]
|
44
|
+
Log.abort "file '#{options[:file]}' doesn't exist"
|
45
|
+
end
|
46
|
+
# read lines from file and format them
|
47
|
+
File::readlines( options[:file] ).each do |line|
|
48
|
+
print_out.( cli.convert_line line )
|
49
|
+
end
|
50
|
+
else
|
51
|
+
cli.convert_stdin &print_out
|
52
|
+
end
|
data/lib/zlog/cli.rb
ADDED
@@ -0,0 +1,35 @@
|
|
1
|
+
require 'zlog'
|
2
|
+
|
3
|
+
class Zlog::CLI
|
4
|
+
def initialize opts = {}
|
5
|
+
@layout = case opts[:layout]
|
6
|
+
when :named
|
7
|
+
Zlog::Layouts.named
|
8
|
+
else
|
9
|
+
Zlog::Layouts.simple
|
10
|
+
end
|
11
|
+
end
|
12
|
+
|
13
|
+
def convert_line line, opts = {}
|
14
|
+
e = Zlog::json_2_event(line)
|
15
|
+
if not e.nil?
|
16
|
+
@layout.format(e)
|
17
|
+
end
|
18
|
+
end
|
19
|
+
|
20
|
+
def convert_stdin opts = {}, &handler
|
21
|
+
# collect all results to an array which will be returned
|
22
|
+
# in case that no block is given for processing
|
23
|
+
res = []
|
24
|
+
handler = lambda{|line| res.push line} if not block_given?
|
25
|
+
|
26
|
+
# process each line
|
27
|
+
STDIN.readlines.each do |line|
|
28
|
+
handler.( convert_line line, opts )
|
29
|
+
end
|
30
|
+
|
31
|
+
# return the result, in case we have any
|
32
|
+
res
|
33
|
+
end
|
34
|
+
end
|
35
|
+
|
data/lib/zlog/zlog.rb
CHANGED
@@ -1,11 +1,22 @@
|
|
1
|
+
require 'json'
|
2
|
+
|
1
3
|
module Zlog
|
2
|
-
VERSION = "0.
|
4
|
+
VERSION = "0.8"
|
5
|
+
extend self
|
6
|
+
|
7
|
+
def init_stdout opts = {layout: :simple, loglevel: nil}
|
8
|
+
# determine the layout from options
|
9
|
+
layout = case opts[:layout]
|
10
|
+
when :named
|
11
|
+
Zlog::Layouts.named
|
12
|
+
else
|
13
|
+
Zlog::Layouts.simple
|
14
|
+
end
|
3
15
|
|
4
|
-
def self.init_stdout opts = {named: false, loglevel: nil}
|
5
16
|
# configure the default stdout appender
|
6
17
|
Logging.appenders.stdout(
|
7
18
|
level: opts[:loglevel],
|
8
|
-
layout:
|
19
|
+
layout: layout
|
9
20
|
)
|
10
21
|
# find all non-stdout appenders
|
11
22
|
# this prevents duplicates of stdout appender
|
@@ -13,4 +24,44 @@ module Zlog
|
|
13
24
|
# add back all appenders + our own stdout appender
|
14
25
|
Logging.logger.root.appenders = as + ["stdout"]
|
15
26
|
end
|
27
|
+
|
28
|
+
def get_writable_logfile *candidates
|
29
|
+
ret = candidates.compact.find do |f|
|
30
|
+
# testing if something is writable, options:
|
31
|
+
# #1: discarged, returns false if the file doesn't exist;
|
32
|
+
# it would require to check the folder too... :(
|
33
|
+
# File::writable? f
|
34
|
+
#2 trial+error
|
35
|
+
begin
|
36
|
+
File.open(f,"a").close
|
37
|
+
true
|
38
|
+
rescue
|
39
|
+
false
|
40
|
+
end
|
41
|
+
end
|
42
|
+
# in case none of the provided logfiles is writable, create one
|
43
|
+
if ret.nil?
|
44
|
+
# try to get a prefix as the filename from the first provided log file
|
45
|
+
prefix = File::basename( candidates[0].to_s )
|
46
|
+
# determine a random filename
|
47
|
+
File::join( Dir::tmpdir, Dir::Tmpname.make_tmpname( prefix, nil ) )
|
48
|
+
else
|
49
|
+
# return the found good filename
|
50
|
+
ret
|
51
|
+
end
|
52
|
+
end
|
53
|
+
|
54
|
+
def json_2_event str
|
55
|
+
begin
|
56
|
+
j = JSON::load str
|
57
|
+
# LogEvent = Struct.new( :logger, :level, :data, :time, :file, :line, :method )
|
58
|
+
# from: https://github.com/TwP/logging/blob/master/lib/logging/log_event.rb
|
59
|
+
# json example:
|
60
|
+
# {"timestamp":"2013-10-10T18:37:38.513438+02:00","level":"DEBUG",
|
61
|
+
# "logger":"Log1-debug","message":"debugging info, this won't show up on console"}
|
62
|
+
Logging::LogEvent.new( j['logger'], Logging.level_num(j['level']), j['message'], j['timestamp'] )
|
63
|
+
rescue
|
64
|
+
nil
|
65
|
+
end
|
66
|
+
end
|
16
67
|
end
|
@@ -34,20 +34,20 @@ describe Zlog::Layouts::Simple do
|
|
34
34
|
format(2).must_equal (pattern_for :warning) + "\n"
|
35
35
|
end
|
36
36
|
|
37
|
-
it "must add
|
38
|
-
format(3).must_equal (pattern_for :
|
37
|
+
it "must add ok events" do
|
38
|
+
format(3).must_equal (pattern_for :ok) + "\n"
|
39
39
|
end
|
40
40
|
|
41
|
-
it "must add
|
42
|
-
format(4).must_equal (pattern_for :
|
41
|
+
it "must add section events" do
|
42
|
+
format(4).must_equal (pattern_for :section) + "\n"
|
43
43
|
end
|
44
44
|
|
45
|
-
it "must add
|
46
|
-
format(5).must_equal (pattern_for :
|
45
|
+
it "must add error events" do
|
46
|
+
format(5).must_equal (pattern_for :error) + "\n"
|
47
47
|
end
|
48
48
|
|
49
|
-
it "must add
|
50
|
-
format(6).must_equal (pattern_for :
|
49
|
+
it "must add fatal events" do
|
50
|
+
format(6).must_equal (pattern_for :fatal) + "\n"
|
51
51
|
end
|
52
52
|
|
53
53
|
it "must support continuous loggin, 1 step" do
|
@@ -35,20 +35,20 @@ describe Zlog::Layouts::SimpleNamed do
|
|
35
35
|
format(2).must_equal (pattern_for :warning) + "\n"
|
36
36
|
end
|
37
37
|
|
38
|
-
it "must add
|
39
|
-
format(3).must_equal (pattern_for :
|
38
|
+
it "must add ok events" do
|
39
|
+
format(3).must_equal (pattern_for :ok) + "\n"
|
40
40
|
end
|
41
41
|
|
42
|
-
it "must add
|
43
|
-
format(4).must_equal (pattern_for :
|
42
|
+
it "must add section events" do
|
43
|
+
format(4).must_equal (pattern_for :section) + "\n"
|
44
44
|
end
|
45
45
|
|
46
|
-
it "must add
|
47
|
-
format(5).must_equal (pattern_for :
|
46
|
+
it "must add error events" do
|
47
|
+
format(5).must_equal (pattern_for :error) + "\n"
|
48
48
|
end
|
49
49
|
|
50
|
-
it "must add
|
51
|
-
format(6).must_equal (pattern_for :
|
50
|
+
it "must add fatal events" do
|
51
|
+
format(6).must_equal (pattern_for :fatal) + "\n"
|
52
52
|
end
|
53
53
|
|
54
54
|
it "must support continuous loggin, 1 step" do
|
data/test/classes/zlog_spec.rb
CHANGED
@@ -1,7 +1,63 @@
|
|
1
1
|
require 'minitest_helper'
|
2
|
+
require 'tmpdir'
|
2
3
|
|
3
4
|
describe Zlog do
|
4
5
|
it "can initialize itself to STDOUT" do
|
5
6
|
Zlog.must_respond_to :init_stdout
|
6
7
|
end
|
8
|
+
|
9
|
+
describe 'get_writable_logfile' do
|
10
|
+
|
11
|
+
before :each do
|
12
|
+
@random_writable = File::join( Dir::tmpdir, Dir::Tmpname.make_tmpname( "", nil ) )
|
13
|
+
@random_non_writable = File::join( '/dev', Dir::Tmpname.make_tmpname("",nil) )
|
14
|
+
end
|
15
|
+
|
16
|
+
it "must give a working writable log file" do
|
17
|
+
Zlog.get_writable_logfile( @random_writable ).
|
18
|
+
must_equal @random_writable
|
19
|
+
end
|
20
|
+
|
21
|
+
it "must give return the first actually writable log file" do
|
22
|
+
Zlog.get_writable_logfile( @random_non_writable, @random_writable ).
|
23
|
+
must_equal @random_writable
|
24
|
+
end
|
25
|
+
|
26
|
+
it "must provide a random file if none of the provided candidates are writable" do
|
27
|
+
f = Zlog.get_writable_logfile( @random_non_writable, @random_writable )
|
28
|
+
f.must_be_instance_of String
|
29
|
+
f.wont_be_empty
|
30
|
+
end
|
31
|
+
|
32
|
+
end
|
33
|
+
|
34
|
+
describe 'json_2_event' do
|
35
|
+
before :each do
|
36
|
+
template = '{"timestamp":"2013-10-10T18:37:38.513438+02:00","level":"%level","logger":"Mocker%level","message":"mock message %level"}'
|
37
|
+
names = ["DEBUG","INFO","WARN","OK","SECTION","ERROR","FATAL"]
|
38
|
+
@examples = (0..(names.length-1)).
|
39
|
+
map do |level|
|
40
|
+
{
|
41
|
+
raw: template.gsub( '%level', names[level] ),
|
42
|
+
level: level,
|
43
|
+
name: names[level]
|
44
|
+
}
|
45
|
+
end
|
46
|
+
end
|
47
|
+
|
48
|
+
it "must give nil on incorrect json" do
|
49
|
+
Zlog.json_2_event( "" ).must_be_nil
|
50
|
+
end
|
51
|
+
|
52
|
+
it "must convert a logging json to a logevent" do
|
53
|
+
@examples.each do |e|
|
54
|
+
r = Zlog.json_2_event e[:raw]
|
55
|
+
r.must_be_instance_of Logging::LogEvent
|
56
|
+
r.level.must_equal e[:level]
|
57
|
+
r.logger.must_equal "Mocker#{e[:name]}"
|
58
|
+
r.data.must_equal "mock message #{e[:name]}"
|
59
|
+
end
|
60
|
+
end
|
61
|
+
|
62
|
+
end
|
7
63
|
end
|
metadata
CHANGED
@@ -1,14 +1,14 @@
|
|
1
1
|
--- !ruby/object:Gem::Specification
|
2
2
|
name: zlog
|
3
3
|
version: !ruby/object:Gem::Version
|
4
|
-
version: '0.
|
4
|
+
version: '0.8'
|
5
5
|
platform: ruby
|
6
6
|
authors:
|
7
7
|
- Dominik Richter
|
8
8
|
autorequire:
|
9
9
|
bindir: bin
|
10
10
|
cert_chain: []
|
11
|
-
date: 2013-10-
|
11
|
+
date: 2013-10-10 00:00:00.000000000 Z
|
12
12
|
dependencies:
|
13
13
|
- !ruby/object:Gem::Dependency
|
14
14
|
name: logging
|
@@ -26,7 +26,8 @@ dependencies:
|
|
26
26
|
version: 1.8.1
|
27
27
|
description: rudimentary simple logging for ruby
|
28
28
|
email: dominik.richter@googlemail.com
|
29
|
-
executables:
|
29
|
+
executables:
|
30
|
+
- zlog
|
30
31
|
extensions: []
|
31
32
|
extra_rdoc_files: []
|
32
33
|
files:
|
@@ -37,10 +38,12 @@ files:
|
|
37
38
|
- LICENSE
|
38
39
|
- README.md
|
39
40
|
- Rakefile
|
41
|
+
- bin/zlog
|
40
42
|
- example/example.file.rb
|
41
43
|
- example/example.output.png
|
42
44
|
- example/example.simple+stdout.rb
|
43
45
|
- lib/zlog.rb
|
46
|
+
- lib/zlog/cli.rb
|
44
47
|
- lib/zlog/layouts.rb
|
45
48
|
- lib/zlog/logging.rb
|
46
49
|
- lib/zlog/simple_layouts.rb
|