turd 0.0.6 → 0.0.7
Sign up to get free protection for your applications and to get access to all the features.
- checksums.yaml +7 -0
- data/README.md +3 -1
- data/lib/turd.rb +4 -1
- data/lib/turd/assert.rb +19 -0
- data/lib/turd/ssh.rb +46 -0
- data/lib/turd/version.rb +1 -1
- metadata +25 -14
checksums.yaml
ADDED
@@ -0,0 +1,7 @@
|
|
1
|
+
---
|
2
|
+
SHA1:
|
3
|
+
metadata.gz: cd226a56c5325a59851958937786cbba3683d903
|
4
|
+
data.tar.gz: e99a1f8d96a0bf8d9322b5cb2ab266d3b57da750
|
5
|
+
SHA512:
|
6
|
+
metadata.gz: 4cb659f2563423e5ef001ee061cf006ea6ccf9cca4ea6fbb1b104db9e9185469b1c26a5e1193c954f3eea3800a0b6558e932d5463ffbe04c62d59352386ecefc
|
7
|
+
data.tar.gz: 8224c5b4c247350792abbe88379fde40417e3bf7a21bb2b8ecd33063a0d1da0528bb87b0ef805e520900ab042a1989c198009cd01396bb550214a9794a06af01
|
data/README.md
CHANGED
@@ -1,3 +1,5 @@
|
|
1
|
+
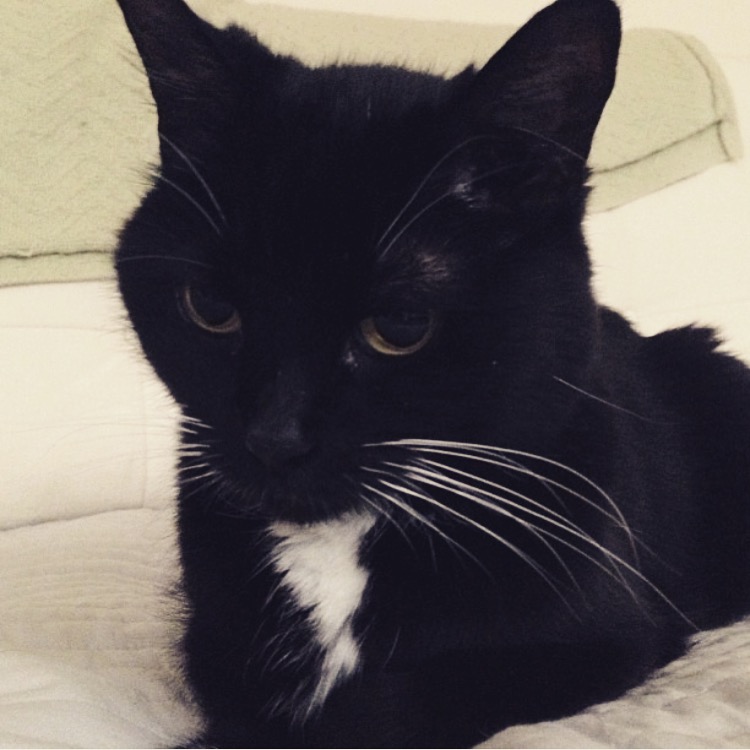
|
2
|
+
|
1
3
|
### turd
|
2
4
|
|
3
5
|
Turd is a little wrapper around typhoeus which is a wrapper around curb which is a wrapper around libcurl which is a wrapper around tcp which is a wrapper around electrons and shit. You can use turd to test http requests and responses. We use it at GitHub to validate responses from various services and alert us if they do not conform to the expected responses. http integration tests if you will. It can be also used for basic telnet-like plain text request validations. Lastly, it also produces some basic diagnostic data like various timings from libcurl.
|
@@ -10,4 +12,4 @@ Check out the examples directory for usage details. The basic idea is you define
|
|
10
12
|
|
11
13
|
#### License
|
12
14
|
|
13
|
-
turd is open source software available under the MIT License
|
15
|
+
turd is open source software available under the MIT License
|
data/lib/turd.rb
CHANGED
@@ -12,6 +12,7 @@ $LOAD_PATH.unshift __DIR__ unless
|
|
12
12
|
|
13
13
|
require 'turd/assert'
|
14
14
|
require 'turd/http'
|
15
|
+
require 'turd/ssh'
|
15
16
|
require 'turd/tcp'
|
16
17
|
require 'turd/version'
|
17
18
|
|
@@ -25,6 +26,8 @@ module Turd
|
|
25
26
|
response = Turd::Http.request(request_definition)
|
26
27
|
when "tcp"
|
27
28
|
response = Turd::Tcp.connect(request_definition)
|
29
|
+
when "ssh"
|
30
|
+
response = Turd::SSH.connect(request_definition)
|
28
31
|
else
|
29
32
|
raise "No request type defined!"
|
30
33
|
end
|
@@ -33,4 +36,4 @@ module Turd
|
|
33
36
|
end
|
34
37
|
|
35
38
|
end
|
36
|
-
end
|
39
|
+
end
|
data/lib/turd/assert.rb
CHANGED
@@ -48,6 +48,25 @@ module Turd
|
|
48
48
|
end
|
49
49
|
end
|
50
50
|
end
|
51
|
+
|
52
|
+
when "ssh"
|
53
|
+
response_definition.each do |option, value|
|
54
|
+
case option
|
55
|
+
when :exit_status
|
56
|
+
if response[:exit_status] != value
|
57
|
+
response.store(:failed, option)
|
58
|
+
raise AssertionFailure.new(response), "Expected exit status of #{value} but got #{response[:exit_status].inspect}"
|
59
|
+
end
|
60
|
+
when :stdout
|
61
|
+
value.each do |v|
|
62
|
+
if !response[:stdout].include?(v)
|
63
|
+
response.store(:failed, option)
|
64
|
+
raise AssertionFailure.new(response), "Expected stdout to include #{v.inspect}"
|
65
|
+
end
|
66
|
+
end
|
67
|
+
end
|
68
|
+
end
|
69
|
+
return response
|
51
70
|
end
|
52
71
|
|
53
72
|
return return_http_response(response)
|
data/lib/turd/ssh.rb
ADDED
@@ -0,0 +1,46 @@
|
|
1
|
+
require 'net/ssh'
|
2
|
+
|
3
|
+
module Turd
|
4
|
+
class SSH
|
5
|
+
def self.connect(request_definition)
|
6
|
+
options = request_definition.fetch(:options)
|
7
|
+
host = options.fetch(:server)
|
8
|
+
username = options.fetch(:username)
|
9
|
+
command = options.fetch(:command)
|
10
|
+
stdin = options.fetch(:stdin, nil)
|
11
|
+
key = options.fetch(:key, nil)
|
12
|
+
|
13
|
+
response = {:stdout => "", :stderr => ""}
|
14
|
+
ssh_options = key ? {:keys_only => true, :key_data => [key], :keys => [], :use_agent => false} : {}
|
15
|
+
ssh_options[:non_interactive] = true
|
16
|
+
|
17
|
+
started = Time.now.to_f
|
18
|
+
connected = exited = stdout_started = nil
|
19
|
+
Net::SSH.start(host, username, ssh_options) do |ssh|
|
20
|
+
connected = Time.now.to_f
|
21
|
+
ssh.open_channel do |channel|
|
22
|
+
channel.exec(command) do |ch, success|
|
23
|
+
if success
|
24
|
+
channel.send_data(stdin) if stdin
|
25
|
+
channel.eof!
|
26
|
+
channel.on_request("exit-status") do |_, data|
|
27
|
+
exited = Time.now.to_f
|
28
|
+
response[:exit_status] = data.read_long
|
29
|
+
end
|
30
|
+
channel.on_data do |_, data|
|
31
|
+
stdout_started = Time.now.to_f
|
32
|
+
response[:stdout] << data
|
33
|
+
end
|
34
|
+
end
|
35
|
+
end
|
36
|
+
end
|
37
|
+
ssh.loop
|
38
|
+
end
|
39
|
+
finished = Time.now.to_f
|
40
|
+
response[:total_time] = finished - started
|
41
|
+
response[:read_time] = exited - connected if exited and connected
|
42
|
+
response[:sshauth_time] = connected - started if connected
|
43
|
+
response
|
44
|
+
end
|
45
|
+
end
|
46
|
+
end
|
data/lib/turd/version.rb
CHANGED
metadata
CHANGED
@@ -1,30 +1,41 @@
|
|
1
1
|
--- !ruby/object:Gem::Specification
|
2
2
|
name: turd
|
3
3
|
version: !ruby/object:Gem::Version
|
4
|
-
version: 0.0.
|
5
|
-
prerelease:
|
4
|
+
version: 0.0.7
|
6
5
|
platform: ruby
|
7
6
|
authors:
|
8
7
|
- joe williams
|
9
8
|
autorequire:
|
10
9
|
bindir: bin
|
11
10
|
cert_chain: []
|
12
|
-
date:
|
11
|
+
date: 2017-03-23 00:00:00.000000000 Z
|
13
12
|
dependencies:
|
14
13
|
- !ruby/object:Gem::Dependency
|
15
14
|
name: typhoeus
|
16
15
|
requirement: !ruby/object:Gem::Requirement
|
17
|
-
none: false
|
18
16
|
requirements:
|
19
|
-
- -
|
17
|
+
- - ">="
|
20
18
|
- !ruby/object:Gem::Version
|
21
19
|
version: '0'
|
22
20
|
type: :runtime
|
23
21
|
prerelease: false
|
24
22
|
version_requirements: !ruby/object:Gem::Requirement
|
25
|
-
none: false
|
26
23
|
requirements:
|
27
|
-
- -
|
24
|
+
- - ">="
|
25
|
+
- !ruby/object:Gem::Version
|
26
|
+
version: '0'
|
27
|
+
- !ruby/object:Gem::Dependency
|
28
|
+
name: net-ssh
|
29
|
+
requirement: !ruby/object:Gem::Requirement
|
30
|
+
requirements:
|
31
|
+
- - ">="
|
32
|
+
- !ruby/object:Gem::Version
|
33
|
+
version: '0'
|
34
|
+
type: :runtime
|
35
|
+
prerelease: false
|
36
|
+
version_requirements: !ruby/object:Gem::Requirement
|
37
|
+
requirements:
|
38
|
+
- - ">="
|
28
39
|
- !ruby/object:Gem::Version
|
29
40
|
version: '0'
|
30
41
|
description:
|
@@ -34,34 +45,34 @@ extensions: []
|
|
34
45
|
extra_rdoc_files:
|
35
46
|
- README.md
|
36
47
|
files:
|
48
|
+
- README.md
|
37
49
|
- lib/turd.rb
|
38
50
|
- lib/turd/assert.rb
|
39
51
|
- lib/turd/http.rb
|
52
|
+
- lib/turd/ssh.rb
|
40
53
|
- lib/turd/tcp.rb
|
41
54
|
- lib/turd/version.rb
|
42
|
-
- README.md
|
43
55
|
homepage: http://github.com/joewilliams/turd
|
44
56
|
licenses: []
|
57
|
+
metadata: {}
|
45
58
|
post_install_message:
|
46
59
|
rdoc_options: []
|
47
60
|
require_paths:
|
48
61
|
- lib
|
49
62
|
required_ruby_version: !ruby/object:Gem::Requirement
|
50
|
-
none: false
|
51
63
|
requirements:
|
52
|
-
- -
|
64
|
+
- - ">="
|
53
65
|
- !ruby/object:Gem::Version
|
54
66
|
version: '0'
|
55
67
|
required_rubygems_version: !ruby/object:Gem::Requirement
|
56
|
-
none: false
|
57
68
|
requirements:
|
58
|
-
- -
|
69
|
+
- - ">="
|
59
70
|
- !ruby/object:Gem::Version
|
60
71
|
version: '0'
|
61
72
|
requirements: []
|
62
73
|
rubyforge_project:
|
63
|
-
rubygems_version:
|
74
|
+
rubygems_version: 2.4.5.1
|
64
75
|
signing_key:
|
65
|
-
specification_version:
|
76
|
+
specification_version: 4
|
66
77
|
summary: a http and tcp testing lib
|
67
78
|
test_files: []
|