stellar-erb 0.1.0
This diff represents the content of publicly available package versions that have been released to one of the supported registries. The information contained in this diff is provided for informational purposes only and reflects changes between package versions as they appear in their respective public registries.
- checksums.yaml +7 -0
- data/CHANGELOG.md +5 -0
- data/LICENSE.txt +21 -0
- data/README.md +134 -0
- data/bin/console +11 -0
- data/bin/setup +8 -0
- data/lib/stellar/erb/binding.rb +32 -0
- data/lib/stellar/erb/binding.rb.1.diff.bak +0 -0
- data/lib/stellar/erb/error.rb +63 -0
- data/lib/stellar/erb/error.rb.1.bak +47 -0
- data/lib/stellar/erb/error.rb.1.diff.bak +17 -0
- data/lib/stellar/erb/version.rb +7 -0
- data/lib/stellar/erb/view.rb +40 -0
- data/lib/stellar/erb/view.rb.1.bak +41 -0
- data/lib/stellar/erb/view.rb.1.diff.bak +65 -0
- data/lib/stellar/erb.rb +16 -0
- data/lib/stellar/erb.rb.1.bak +10 -0
- data/lib/stellar/erb.rb.1.diff.bak +16 -0
- data/lib/stellar.rb +4 -0
- data/lib/stellar.rb.1.diff.bak +0 -0
- metadata +123 -0
checksums.yaml
ADDED
@@ -0,0 +1,7 @@
|
|
1
|
+
---
|
2
|
+
SHA256:
|
3
|
+
metadata.gz: 4d36ba6cabfdabcea69f3c1eae4a85ac8a178295783bb045eddfa1fe3f466671
|
4
|
+
data.tar.gz: 2a278f0be5a4b6d19635fc5de83737b260ee0904573dcc4986d64f0c131ba6b8
|
5
|
+
SHA512:
|
6
|
+
metadata.gz: a1d708c9a15b841ac2c9ed100c6d513cffad5b3f18b0adbca3ac0ed2cb057f6f46e6b18841a6570903b3e8dff00a4f856f28fc7befd71d1bd4b5f03ab212f9b5
|
7
|
+
data.tar.gz: 835d54c01f66476e40595ec201c6b02f727d680ff6898bdb0fd9e38f83b3d749d15e7fada2656f9d56d9ca293e01ec86047c764d34b91407d4f1d758e2a27cd1
|
data/CHANGELOG.md
ADDED
data/LICENSE.txt
ADDED
@@ -0,0 +1,21 @@
|
|
1
|
+
The MIT License (MIT)
|
2
|
+
|
3
|
+
Copyright (c) 2025 David J Berube
|
4
|
+
|
5
|
+
Permission is hereby granted, free of charge, to any person obtaining a copy
|
6
|
+
of this software and associated documentation files (the "Software"), to deal
|
7
|
+
in the Software without restriction, including without limitation the rights
|
8
|
+
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
9
|
+
copies of the Software, and to permit persons to whom the Software is
|
10
|
+
furnished to do so, subject to the following conditions:
|
11
|
+
|
12
|
+
The above copyright notice and this permission notice shall be included in
|
13
|
+
all copies or substantial portions of the Software.
|
14
|
+
|
15
|
+
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
16
|
+
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
17
|
+
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
18
|
+
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
19
|
+
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
20
|
+
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
|
21
|
+
THE SOFTWARE.
|
data/README.md
ADDED
@@ -0,0 +1,134 @@
|
|
1
|
+
# Stellar::Erb
|
2
|
+
|
3
|
+

|
4
|
+
|
5
|
+
|
6
|
+
|
7
|
+
A safe, easy to use wrapper for ERB (Embedded Ruby) templates outside of Rails.
|
8
|
+
|
9
|
+
|
10
|
+
## Overview
|
11
|
+
|
12
|
+
Stellar::Erb provides a robust method for reading `.erb` files from disk and rendering them to strings, with built-in support for:
|
13
|
+
- Variable passing
|
14
|
+
- Error handling with context
|
15
|
+
- Clean backtraces
|
16
|
+
- Template reusability
|
17
|
+
|
18
|
+
|
19
|
+
## Installation
|
20
|
+
|
21
|
+
Add this line to your application's Gemfile:
|
22
|
+
|
23
|
+
```ruby
|
24
|
+
gem 'stellar-erb'
|
25
|
+
```
|
26
|
+
|
27
|
+
And then execute:
|
28
|
+
|
29
|
+
```bash
|
30
|
+
$ bundle install
|
31
|
+
```
|
32
|
+
|
33
|
+
Or install it yourself as:
|
34
|
+
|
35
|
+
```bash
|
36
|
+
$ gem install stellar-erb
|
37
|
+
```
|
38
|
+
|
39
|
+
## Usage
|
40
|
+
|
41
|
+
### Basic Template Rendering
|
42
|
+
|
43
|
+
```ruby
|
44
|
+
# template.erb
|
45
|
+
<h1>Hello, <%= name %>!</h1>
|
46
|
+
<ul>
|
47
|
+
<% items.each do |item| %>
|
48
|
+
<li><%= item %></li>
|
49
|
+
<% end %>
|
50
|
+
</ul>
|
51
|
+
|
52
|
+
# Ruby code
|
53
|
+
result = Stellar::Erb::View.render('template.erb',
|
54
|
+
name: 'John',
|
55
|
+
items: ['apple', 'banana', 'orange']
|
56
|
+
)
|
57
|
+
```
|
58
|
+
|
59
|
+
|
60
|
+
|
61
|
+
#### Creating Reusable Views
|
62
|
+
|
63
|
+
```ruby
|
64
|
+
# Create a view instance for reuse
|
65
|
+
view = Stellar::Erb::View.new('templates/header.erb',
|
66
|
+
company_name: 'Acme Corp'
|
67
|
+
)
|
68
|
+
|
69
|
+
# Render multiple times with different locals
|
70
|
+
page1 = view.render(title: 'Home')
|
71
|
+
page2 = view.render(title: 'About')
|
72
|
+
```
|
73
|
+
|
74
|
+
#### Error Handling
|
75
|
+
|
76
|
+
```ruby
|
77
|
+
begin
|
78
|
+
Stellar::Erb::View.render('template.erb', user: current_user)
|
79
|
+
rescue Stellar::Erb::Error => e
|
80
|
+
puts "Error: #{e.message}"
|
81
|
+
puts "\nContext:"
|
82
|
+
puts e.context_lines.join("\n")
|
83
|
+
puts "\nOriginal error: #{e.original_error.class}"
|
84
|
+
end
|
85
|
+
```
|
86
|
+
|
87
|
+
|
88
|
+
|
89
|
+
## Development
|
90
|
+
|
91
|
+
After checking out the repo, run `bin/setup` to install dependencies. Then, run `rake test` to run the tests. You can also run `bin/console` for an interactive prompt that will allow you to experiment.
|
92
|
+
|
93
|
+
Development workflow:
|
94
|
+
|
95
|
+
```mermaid
|
96
|
+
flowchart TD
|
97
|
+
A[Fork Repository] --> B[Clone Locally]
|
98
|
+
B --> C[Install Dependencies]
|
99
|
+
C --> D[Run Tests]
|
100
|
+
D --> E{Tests Pass?}
|
101
|
+
E -->|Yes| F[Create Feature Branch]
|
102
|
+
E -->|No| D
|
103
|
+
F --> G[Make Changes]
|
104
|
+
G --> H[Run Tests]
|
105
|
+
H --> I{Tests Pass?}
|
106
|
+
I -->|Yes| J[Submit PR]
|
107
|
+
I -->|No| G
|
108
|
+
```
|
109
|
+
|
110
|
+
## Contributing
|
111
|
+
|
112
|
+
1. Fork it
|
113
|
+
2. Create your feature branch (`git checkout -b feature/my-new-feature`)
|
114
|
+
3. Commit your changes (`git commit -am 'Add some feature'`)
|
115
|
+
4. Push to the branch (`git push origin feature/my-new-feature`)
|
116
|
+
5. Create new Pull Request
|
117
|
+
|
118
|
+
## License
|
119
|
+
|
120
|
+
The gem is available as open source under the terms of the [MIT License](https://opensource.org/licenses/MIT).
|
121
|
+
|
122
|
+
|
123
|
+
## Support
|
124
|
+
|
125
|
+
For bug reports and feature requests, please use the [GitHub Issues](https://github.com/durableprogramming/stellar-erb/issues) page.
|
126
|
+
|
127
|
+
---
|
128
|
+
|
129
|
+
Stellar::Erb is actively maintained by [Durable Programming, LLC](https://github.com/durableprogramming).
|
130
|
+
|
131
|
+
|
132
|
+
Commercial support for Stellar::Erb and related tools is available from Durable Programming, LLC. You can contact us at [durableprogramming.com](https://www.durableprogramming.com).
|
133
|
+
|
134
|
+
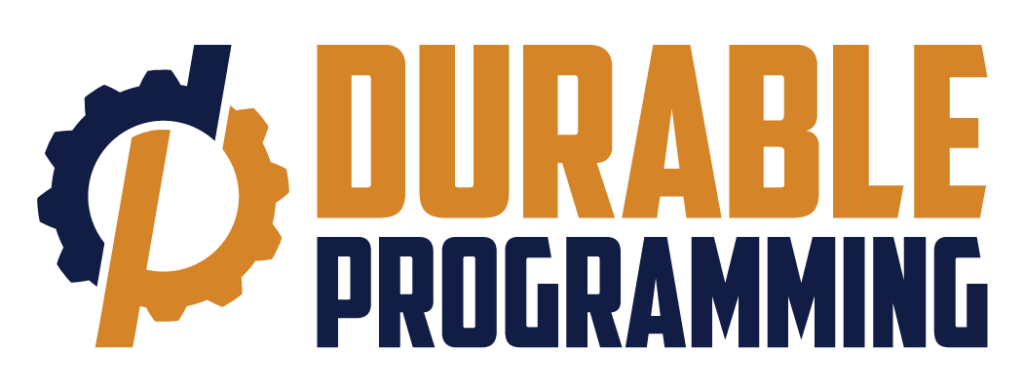
|
data/bin/console
ADDED
@@ -0,0 +1,11 @@
|
|
1
|
+
#!/usr/bin/env ruby
|
2
|
+
# frozen_string_literal: true
|
3
|
+
|
4
|
+
require "bundler/setup"
|
5
|
+
require "stellar/erb"
|
6
|
+
|
7
|
+
# You can add fixtures and/or initialization code here to make experimenting
|
8
|
+
# with your gem easier. You can also use a different console, if you like.
|
9
|
+
|
10
|
+
require "irb"
|
11
|
+
IRB.start(__FILE__)
|
data/bin/setup
ADDED
@@ -0,0 +1,32 @@
|
|
1
|
+
module Stellar
|
2
|
+
module Erb
|
3
|
+
class Binding
|
4
|
+
attr_reader :locals
|
5
|
+
|
6
|
+
def initialize(locals = {})
|
7
|
+
@locals = locals
|
8
|
+
end
|
9
|
+
|
10
|
+
def get_binding
|
11
|
+
locals.each do |key, value|
|
12
|
+
singleton_class.class_eval do
|
13
|
+
define_method(key) { value }
|
14
|
+
end
|
15
|
+
end
|
16
|
+
binding
|
17
|
+
end
|
18
|
+
|
19
|
+
def method_missing(method_name, *args, &block)
|
20
|
+
if locals.key?(method_name)
|
21
|
+
locals[method_name]
|
22
|
+
else
|
23
|
+
super
|
24
|
+
end
|
25
|
+
end
|
26
|
+
|
27
|
+
def respond_to_missing?(method_name, include_private = false)
|
28
|
+
locals.key?(method_name) || super
|
29
|
+
end
|
30
|
+
end
|
31
|
+
end
|
32
|
+
end
|
File without changes
|
@@ -0,0 +1,63 @@
|
|
1
|
+
module Stellar
|
2
|
+
module Erb
|
3
|
+
class Error < StandardError
|
4
|
+
attr_reader :template_path, :original_error, :line_number
|
5
|
+
|
6
|
+
def initialize(message = nil, template_path: nil, original_error: nil, line_number: nil)
|
7
|
+
@template_path = template_path
|
8
|
+
@original_error = original_error
|
9
|
+
@line_number = line_number
|
10
|
+
super(message)
|
11
|
+
end
|
12
|
+
|
13
|
+
def to_s
|
14
|
+
message = super
|
15
|
+
if template_path && line_number
|
16
|
+
"#{message} in '#{template_path}' on line #{line_number}"
|
17
|
+
elsif template_path
|
18
|
+
"#{message} in '#{template_path}'"
|
19
|
+
else
|
20
|
+
message
|
21
|
+
end
|
22
|
+
end
|
23
|
+
|
24
|
+
def self.wrap(error, template_path: nil)
|
25
|
+
return error if error.is_a?(self)
|
26
|
+
|
27
|
+
line_number = extract_line_number(error, template_path)
|
28
|
+
message = error.message
|
29
|
+
|
30
|
+
new(message,
|
31
|
+
template_path: template_path,
|
32
|
+
original_error: error,
|
33
|
+
line_number: line_number)
|
34
|
+
end
|
35
|
+
|
36
|
+
def self.extract_line_number(error, template_path)
|
37
|
+
return nil unless template_path && error.backtrace
|
38
|
+
|
39
|
+
backtrace_line = error.backtrace.find { |line| line.include?(template_path) }
|
40
|
+
return nil unless backtrace_line
|
41
|
+
|
42
|
+
match = backtrace_line.match(/:(\d+):/)
|
43
|
+
match ? match[1].to_i : nil
|
44
|
+
end
|
45
|
+
|
46
|
+
def context_lines(number_of_lines = 5)
|
47
|
+
return [] unless template_path && line_number && File.exist?(template_path)
|
48
|
+
|
49
|
+
lines = File.readlines(template_path)
|
50
|
+
start_line = [line_number - number_of_lines - 1, 0].max
|
51
|
+
end_line = [line_number + number_of_lines - 1, lines.length - 1].min
|
52
|
+
|
53
|
+
context = []
|
54
|
+
(start_line..end_line).each do |i|
|
55
|
+
prefix = i + 1 == line_number ? "=>" : " "
|
56
|
+
context << "#{prefix} #{i + 1}: #{lines[i].chomp}"
|
57
|
+
end
|
58
|
+
|
59
|
+
context
|
60
|
+
end
|
61
|
+
end
|
62
|
+
end
|
63
|
+
end
|
@@ -0,0 +1,47 @@
|
|
1
|
+
module Stellar
|
2
|
+
module Erb
|
3
|
+
class Error < StandardError
|
4
|
+
attr_reader :template_path, :original_error, :line_number
|
5
|
+
|
6
|
+
def initialize(message = nil, template_path: nil, original_error: nil, line_number: nil)
|
7
|
+
@template_path = template_path
|
8
|
+
@original_error = original_error
|
9
|
+
@line_number = line_number
|
10
|
+
super(message)
|
11
|
+
end
|
12
|
+
|
13
|
+
def to_s
|
14
|
+
message = super
|
15
|
+
if template_path && line_number
|
16
|
+
"#{message} in '#{template_path}' on line #{line_number}"
|
17
|
+
elsif template_path
|
18
|
+
"#{message} in '#{template_path}'"
|
19
|
+
else
|
20
|
+
message
|
21
|
+
end
|
22
|
+
end
|
23
|
+
|
24
|
+
def self.wrap(error, template_path: nil)
|
25
|
+
return error if error.is_a?(self)
|
26
|
+
|
27
|
+
line_number = extract_line_number(error, template_path)
|
28
|
+
message = error.message
|
29
|
+
|
30
|
+
new(message,
|
31
|
+
template_path: template_path,
|
32
|
+
original_error: error,
|
33
|
+
line_number: line_number)
|
34
|
+
end
|
35
|
+
|
36
|
+
def self.extract_line_number(error, template_path)
|
37
|
+
return nil unless template_path && error.backtrace
|
38
|
+
|
39
|
+
backtrace_line = error.backtrace.find { |line| line.include?(template_path) }
|
40
|
+
return nil unless backtrace_line
|
41
|
+
|
42
|
+
match = backtrace_line.match(/:(\d+):/)
|
43
|
+
match ? match[1].to_i : nil
|
44
|
+
end
|
45
|
+
end
|
46
|
+
end
|
47
|
+
end
|
@@ -0,0 +1,17 @@
|
|
1
|
+
45,60d44
|
2
|
+
<
|
3
|
+
< def context_lines(number_of_lines = 5)
|
4
|
+
< return [] unless template_path && line_number && File.exist?(template_path)
|
5
|
+
<
|
6
|
+
< lines = File.readlines(template_path)
|
7
|
+
< start_line = [line_number - number_of_lines - 1, 0].max
|
8
|
+
< end_line = [line_number + number_of_lines - 1, lines.length - 1].min
|
9
|
+
<
|
10
|
+
< context = []
|
11
|
+
< (start_line..end_line).each do |i|
|
12
|
+
< prefix = i + 1 == line_number ? "=>" : " "
|
13
|
+
< context << "#{prefix} #{i + 1}: #{lines[i].chomp}"
|
14
|
+
< end
|
15
|
+
<
|
16
|
+
< context
|
17
|
+
< end
|
@@ -0,0 +1,40 @@
|
|
1
|
+
module Stellar
|
2
|
+
module Erb
|
3
|
+
class View
|
4
|
+
attr_reader :template_path, :template_content, :locals
|
5
|
+
|
6
|
+
def initialize(template_path, locals = {})
|
7
|
+
@template_path = template_path
|
8
|
+
@template_content = File.read(template_path)
|
9
|
+
@locals = locals
|
10
|
+
end
|
11
|
+
|
12
|
+
def render(additional_locals = {})
|
13
|
+
all_locals = locals.merge(additional_locals)
|
14
|
+
binding_obj = Binding.new(all_locals)
|
15
|
+
erb = ::ERB.new(template_content)
|
16
|
+
erb.result(binding_obj.get_binding)
|
17
|
+
rescue StandardError => e
|
18
|
+
handle_error(e)
|
19
|
+
end
|
20
|
+
|
21
|
+
def self.render(template_path, locals = {})
|
22
|
+
new(template_path, locals).render
|
23
|
+
end
|
24
|
+
|
25
|
+
private
|
26
|
+
|
27
|
+
def handle_error(error)
|
28
|
+
if error.is_a?(SyntaxError)
|
29
|
+
raise Error, "Syntax error in template #{template_path}: #{error.message}"
|
30
|
+
else
|
31
|
+
backtrace = error.backtrace.select { |line| line.include?(template_path) }
|
32
|
+
message = "Error rendering template #{template_path}: #{error.message}"
|
33
|
+
error_with_context = Error.new(message)
|
34
|
+
error_with_context.set_backtrace(backtrace.empty? ? error.backtrace : backtrace)
|
35
|
+
raise error_with_context
|
36
|
+
end
|
37
|
+
end
|
38
|
+
end
|
39
|
+
end
|
40
|
+
end
|
@@ -0,0 +1,41 @@
|
|
1
|
+
module Stellar
|
2
|
+
module Erb
|
3
|
+
class View
|
4
|
+
attr_reader :template_path, :template, :context
|
5
|
+
|
6
|
+
def initialize(template_path, context = {})
|
7
|
+
@template_path = template_path
|
8
|
+
@context = context
|
9
|
+
@template = File.read(template_path)
|
10
|
+
end
|
11
|
+
|
12
|
+
def render(locals = {})
|
13
|
+
b = binding_with_context(locals)
|
14
|
+
result = ::ERB.new(template, trim_mode: '-').result(b)
|
15
|
+
result
|
16
|
+
rescue => e
|
17
|
+
handle_error(e)
|
18
|
+
end
|
19
|
+
|
20
|
+
private
|
21
|
+
|
22
|
+
def binding_with_context(locals = {})
|
23
|
+
context_binding = binding
|
24
|
+
context.merge(locals).each do |key, value|
|
25
|
+
context_binding.local_variable_set(key.to_sym, value)
|
26
|
+
end
|
27
|
+
context_binding
|
28
|
+
end
|
29
|
+
|
30
|
+
def handle_error(error)
|
31
|
+
if error.backtrace.any? { |line| line.include?(template_path) }
|
32
|
+
raise error
|
33
|
+
else
|
34
|
+
new_error = error.class.new("#{error.message} (in template: #{template_path})")
|
35
|
+
new_error.set_backtrace(error.backtrace)
|
36
|
+
raise new_error
|
37
|
+
end
|
38
|
+
end
|
39
|
+
end
|
40
|
+
end
|
41
|
+
end
|
@@ -0,0 +1,65 @@
|
|
1
|
+
4,6c4,6
|
2
|
+
< attr_reader :template_path, :template_content, :locals
|
3
|
+
<
|
4
|
+
< def initialize(template_path, locals = {})
|
5
|
+
---
|
6
|
+
> attr_reader :template_path, :template, :context
|
7
|
+
>
|
8
|
+
> def initialize(template_path, context = {})
|
9
|
+
8,9c8,9
|
10
|
+
< @template_content = File.read(template_path)
|
11
|
+
< @locals = locals
|
12
|
+
---
|
13
|
+
> @context = context
|
14
|
+
> @template = File.read(template_path)
|
15
|
+
11,17c11,16
|
16
|
+
<
|
17
|
+
< def render(additional_locals = {})
|
18
|
+
< all_locals = locals.merge(additional_locals)
|
19
|
+
< binding_obj = Binding.new(all_locals)
|
20
|
+
< erb = ::ERB.new(template_content)
|
21
|
+
< erb.result(binding_obj.get_binding)
|
22
|
+
< rescue StandardError => e
|
23
|
+
---
|
24
|
+
>
|
25
|
+
> def render(locals = {})
|
26
|
+
> b = binding_with_context(locals)
|
27
|
+
> result = ::ERB.new(template, trim_mode: '-').result(b)
|
28
|
+
> result
|
29
|
+
> rescue => e
|
30
|
+
20,24c19
|
31
|
+
<
|
32
|
+
< def self.render(template_path, locals = {})
|
33
|
+
< new(template_path, locals).render
|
34
|
+
< end
|
35
|
+
<
|
36
|
+
---
|
37
|
+
>
|
38
|
+
26c21,29
|
39
|
+
<
|
40
|
+
---
|
41
|
+
>
|
42
|
+
> def binding_with_context(locals = {})
|
43
|
+
> context_binding = binding
|
44
|
+
> context.merge(locals).each do |key, value|
|
45
|
+
> context_binding.local_variable_set(key.to_sym, value)
|
46
|
+
> end
|
47
|
+
> context_binding
|
48
|
+
> end
|
49
|
+
>
|
50
|
+
28,29c31,32
|
51
|
+
< if error.is_a?(SyntaxError)
|
52
|
+
< raise Error, "Syntax error in template #{template_path}: #{error.message}"
|
53
|
+
---
|
54
|
+
> if error.backtrace.any? { |line| line.include?(template_path) }
|
55
|
+
> raise error
|
56
|
+
31,35c34,36
|
57
|
+
< backtrace = error.backtrace.select { |line| line.include?(template_path) }
|
58
|
+
< message = "Error rendering template #{template_path}: #{error.message}"
|
59
|
+
< error_with_context = Error.new(message)
|
60
|
+
< error_with_context.set_backtrace(backtrace.empty? ? error.backtrace : backtrace)
|
61
|
+
< raise error_with_context
|
62
|
+
---
|
63
|
+
> new_error = error.class.new("#{error.message} (in template: #{template_path})")
|
64
|
+
> new_error.set_backtrace(error.backtrace)
|
65
|
+
> raise new_error
|
data/lib/stellar/erb.rb
ADDED
@@ -0,0 +1,16 @@
|
|
1
|
+
require_relative "erb/version"
|
2
|
+
require_relative "erb/binding"
|
3
|
+
require_relative "erb/error"
|
4
|
+
require_relative "erb/view"
|
5
|
+
require "erb"
|
6
|
+
|
7
|
+
module Stellar
|
8
|
+
module Erb
|
9
|
+
class Error < StandardError; end
|
10
|
+
|
11
|
+
# Shortcut method for rendering templates
|
12
|
+
def self.render(template_path, locals = {})
|
13
|
+
View.render(template_path, locals)
|
14
|
+
end
|
15
|
+
end
|
16
|
+
end
|
@@ -0,0 +1,16 @@
|
|
1
|
+
0a1,2
|
2
|
+
> # frozen_string_literal: true
|
3
|
+
>
|
4
|
+
2,5d3
|
5
|
+
< require_relative "erb/binding"
|
6
|
+
< require_relative "erb/error"
|
7
|
+
< require_relative "erb/view"
|
8
|
+
< require "erb"
|
9
|
+
10,14c8
|
10
|
+
<
|
11
|
+
< # Shortcut method for rendering templates
|
12
|
+
< def self.render(template_path, locals = {})
|
13
|
+
< View.render(template_path, locals)
|
14
|
+
< end
|
15
|
+
---
|
16
|
+
> # Your code goes here...
|
data/lib/stellar.rb
ADDED
File without changes
|
metadata
ADDED
@@ -0,0 +1,123 @@
|
|
1
|
+
--- !ruby/object:Gem::Specification
|
2
|
+
name: stellar-erb
|
3
|
+
version: !ruby/object:Gem::Version
|
4
|
+
version: 0.1.0
|
5
|
+
platform: ruby
|
6
|
+
authors:
|
7
|
+
- Durable Programming, LLC
|
8
|
+
autorequire:
|
9
|
+
bindir: exe
|
10
|
+
cert_chain: []
|
11
|
+
date: 1980-01-01 00:00:00.000000000 Z
|
12
|
+
dependencies:
|
13
|
+
- !ruby/object:Gem::Dependency
|
14
|
+
name: erb
|
15
|
+
requirement: !ruby/object:Gem::Requirement
|
16
|
+
requirements:
|
17
|
+
- - "~>"
|
18
|
+
- !ruby/object:Gem::Version
|
19
|
+
version: '4.0'
|
20
|
+
type: :runtime
|
21
|
+
prerelease: false
|
22
|
+
version_requirements: !ruby/object:Gem::Requirement
|
23
|
+
requirements:
|
24
|
+
- - "~>"
|
25
|
+
- !ruby/object:Gem::Version
|
26
|
+
version: '4.0'
|
27
|
+
- !ruby/object:Gem::Dependency
|
28
|
+
name: rake
|
29
|
+
requirement: !ruby/object:Gem::Requirement
|
30
|
+
requirements:
|
31
|
+
- - "~>"
|
32
|
+
- !ruby/object:Gem::Version
|
33
|
+
version: '13.0'
|
34
|
+
type: :development
|
35
|
+
prerelease: false
|
36
|
+
version_requirements: !ruby/object:Gem::Requirement
|
37
|
+
requirements:
|
38
|
+
- - "~>"
|
39
|
+
- !ruby/object:Gem::Version
|
40
|
+
version: '13.0'
|
41
|
+
- !ruby/object:Gem::Dependency
|
42
|
+
name: minitest
|
43
|
+
requirement: !ruby/object:Gem::Requirement
|
44
|
+
requirements:
|
45
|
+
- - "~>"
|
46
|
+
- !ruby/object:Gem::Version
|
47
|
+
version: '5.0'
|
48
|
+
type: :development
|
49
|
+
prerelease: false
|
50
|
+
version_requirements: !ruby/object:Gem::Requirement
|
51
|
+
requirements:
|
52
|
+
- - "~>"
|
53
|
+
- !ruby/object:Gem::Version
|
54
|
+
version: '5.0'
|
55
|
+
- !ruby/object:Gem::Dependency
|
56
|
+
name: rubocop
|
57
|
+
requirement: !ruby/object:Gem::Requirement
|
58
|
+
requirements:
|
59
|
+
- - "~>"
|
60
|
+
- !ruby/object:Gem::Version
|
61
|
+
version: '1.21'
|
62
|
+
type: :development
|
63
|
+
prerelease: false
|
64
|
+
version_requirements: !ruby/object:Gem::Requirement
|
65
|
+
requirements:
|
66
|
+
- - "~>"
|
67
|
+
- !ruby/object:Gem::Version
|
68
|
+
version: '1.21'
|
69
|
+
description: Stellar::Erb provides a method for reading .erb files from disk and rendering
|
70
|
+
them to strings, passing arguments, and catching errors with correct backtraces
|
71
|
+
and context.
|
72
|
+
email:
|
73
|
+
- djberube@durableprogramming.com
|
74
|
+
executables: []
|
75
|
+
extensions: []
|
76
|
+
extra_rdoc_files: []
|
77
|
+
files:
|
78
|
+
- CHANGELOG.md
|
79
|
+
- LICENSE.txt
|
80
|
+
- README.md
|
81
|
+
- bin/console
|
82
|
+
- bin/setup
|
83
|
+
- lib/stellar.rb
|
84
|
+
- lib/stellar.rb.1.diff.bak
|
85
|
+
- lib/stellar/erb.rb
|
86
|
+
- lib/stellar/erb.rb.1.bak
|
87
|
+
- lib/stellar/erb.rb.1.diff.bak
|
88
|
+
- lib/stellar/erb/binding.rb
|
89
|
+
- lib/stellar/erb/binding.rb.1.diff.bak
|
90
|
+
- lib/stellar/erb/error.rb
|
91
|
+
- lib/stellar/erb/error.rb.1.bak
|
92
|
+
- lib/stellar/erb/error.rb.1.diff.bak
|
93
|
+
- lib/stellar/erb/version.rb
|
94
|
+
- lib/stellar/erb/view.rb
|
95
|
+
- lib/stellar/erb/view.rb.1.bak
|
96
|
+
- lib/stellar/erb/view.rb.1.diff.bak
|
97
|
+
homepage: https://github.com/durableprogramming/stellar-erb
|
98
|
+
licenses:
|
99
|
+
- MIT
|
100
|
+
metadata:
|
101
|
+
homepage_uri: https://github.com/durableprogramming/stellar-erb
|
102
|
+
source_code_uri: https://github.com/durableprogramming/stellar-erb
|
103
|
+
changelog_uri: https://github.com/durableprogramming/stellar-erb/blob/main/CHANGELOG.md
|
104
|
+
post_install_message:
|
105
|
+
rdoc_options: []
|
106
|
+
require_paths:
|
107
|
+
- lib
|
108
|
+
required_ruby_version: !ruby/object:Gem::Requirement
|
109
|
+
requirements:
|
110
|
+
- - ">="
|
111
|
+
- !ruby/object:Gem::Version
|
112
|
+
version: 2.6.0
|
113
|
+
required_rubygems_version: !ruby/object:Gem::Requirement
|
114
|
+
requirements:
|
115
|
+
- - ">="
|
116
|
+
- !ruby/object:Gem::Version
|
117
|
+
version: '0'
|
118
|
+
requirements: []
|
119
|
+
rubygems_version: 3.5.22
|
120
|
+
signing_key:
|
121
|
+
specification_version: 4
|
122
|
+
summary: A safe, easy to use wrapper for ERB views outside of Rails
|
123
|
+
test_files: []
|