standard_draw_tk 0.1.1 → 0.1.2
Sign up to get free protection for your applications and to get access to all the features.
- checksums.yaml +4 -4
- data/README.md +82 -5
- data/examples/example4.rb +8 -0
- data/examples/image_example4.png +0 -0
- data/examples/right_triangle.rb +9 -0
- data/lib/standard_draw_tk.rb +8 -7
- data/lib/standard_draw_tk/version.rb +1 -1
- data/standard_draw_tk.gemspec +2 -2
- metadata +21 -17
checksums.yaml
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
---
|
2
2
|
SHA256:
|
3
|
-
metadata.gz:
|
4
|
-
data.tar.gz:
|
3
|
+
metadata.gz: d90a6bcb43272afb1a5c528dbc97ee38f4928b97952c394e444a41c35f4c4a42
|
4
|
+
data.tar.gz: b18f7a7059cd831c118fc7a3703a500362c78c80f42a55519a5d648b4ebdcb69
|
5
5
|
SHA512:
|
6
|
-
metadata.gz:
|
7
|
-
data.tar.gz:
|
6
|
+
metadata.gz: 21ecd96da9884528642f33848f6e316bc59050d5d5c8a35b4b71cb1430cf4a73230e3eb4f4556cc31662b85784ed706e54cd7a76822e4d4b3e8b6accaaba3b6f
|
7
|
+
data.tar.gz: 2e67038a30e5171e4b043d78160b297ff2e8089b4d67e5237134f7c1969d287099773839ce2cdcd51e51d4be271e9d580f5ade6d0e04f7f1a1e97d6f4a4f3682
|
data/README.md
CHANGED
@@ -1,8 +1,34 @@
|
|
1
1
|
# Standard Draw TK
|
2
2
|
|
3
|
-
|
3
|
+
The StdDraw class provides a basic capability for creating drawings with your programs. It uses a simple graphics model that allows you to create drawings consisting of points, lines, squares, circles, and other geometric shapes in a window on your computer.
|
4
|
+
|
5
|
+
I was going through the excellent [Algorithms 4th edition](https://algs4.cs.princeton.edu/home/) text and *really* wanted to use ruby instead of the default java. The book makes use of the [Princeton Standard Libraries](https://introcs.cs.princeton.edu/java/stdlib/) to help clarify the code and display useful figures.
|
6
|
+
|
7
|
+
The original graphics code uses Java Swing/AWT which works fine with Jruby but not so much with standard ruby. So I started wondering how bad it would be to rewrite it in something MRI friendly. I'm attempting to keep the original interface as much as possible while recreating the logic using the [ruby TK gem](https://github.com/ruby/tk). The graphics drawing portion works but the library is not yet fully complete. See [things to implement](https://github.com/gregors/standard_draw_tk/blob/master/README.md#things-to-implement)
|
8
|
+
|
9
|
+
If you need something not yet ported consider using the [jruby wrapper gem](https://rubygems.org/gems/princeton_standard_libraries) that lets you call the original java code in Jruby.
|
10
|
+
|
11
|
+
Most of the docs taken/modified from the [original source](https://introcs.cs.princeton.edu/java/stdlib/javadoc/StdDraw.html)
|
12
|
+
|
13
|
+
Type the following short program into your editor:
|
14
|
+
|
15
|
+
require 'standard_draw_tk'
|
16
|
+
|
17
|
+
StdDraw.pen_radius = 0.05
|
18
|
+
StdDraw.pen_color = :blue
|
19
|
+
StdDraw.point(0.5, 0.5)
|
20
|
+
StdDraw.pen_color = :magenta
|
21
|
+
StdDraw.line(0.2, 0.2, 0.8, 0.2)
|
22
|
+
StdDraw.pause
|
23
|
+
|
24
|
+
run it:
|
25
|
+
|
26
|
+
ruby examples/example4.rb
|
27
|
+
|
28
|
+
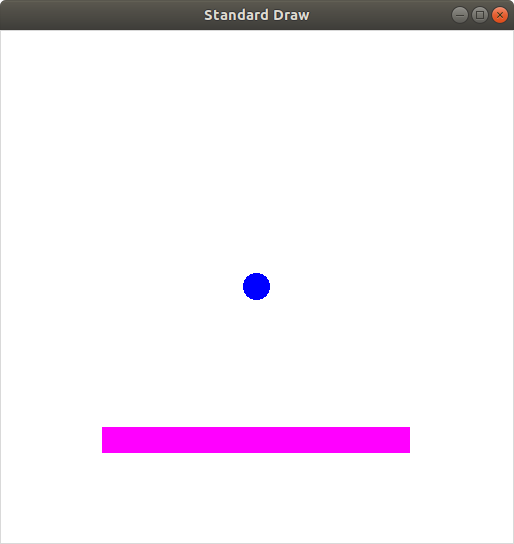
|
29
|
+
|
30
|
+
|
4
31
|
|
5
|
-
I really would like to call this code in standard ruby. So I'm attempting to keep the original interface while recreating the logic using the [ruby TK gem](https://github.com/ruby/tk).
|
6
32
|
|
7
33
|
## Installation
|
8
34
|
|
@@ -23,16 +49,67 @@ Ruby doesn't contain TK by default anymore. And installing the TK gem requires a
|
|
23
49
|
|
24
50
|
## Usage
|
25
51
|
|
26
|
-
|
52
|
+
|
53
|
+
### Note
|
54
|
+
|
55
|
+
You will need to add `StdDraw.pause` to the end of your programs as shown in the examples. I may change or alias this in the future or maybe find a work around. This deviates from the original Standar Draw lib
|
56
|
+
|
57
|
+
### Points and lines
|
58
|
+
|
59
|
+
You can draw points and line segments with the following methods:
|
60
|
+
|
61
|
+
StdDraw.point(x, y)
|
62
|
+
StdDraw.line(x1, y1, x2, y2)
|
63
|
+
|
64
|
+
The x- and y-coordinates must be in the drawing area (between 0 and 1 and by default) or the points and lines will not be visible.
|
65
|
+
|
66
|
+
### Squares, circles, rectangles, and ellipses
|
67
|
+
|
68
|
+
You can draw squares, circles, rectangles, and ellipses using the following methods:
|
69
|
+
|
70
|
+
StdDraw.circle(x, y, radius)
|
71
|
+
StdDraw.ellipse(x, y, semiMajorAxis, semiMinorAxis)
|
72
|
+
StdDraw.square(x, y, radius)
|
73
|
+
StdDraw.rectangle(x, y, halfWidth, halfHeight)
|
74
|
+
|
75
|
+
All of these methods take as arguments the location and size of the shape. The location is always specified by the x- and y-coordinates of its center. The size of a circle is specified by its radius and the size of an ellipse is specified by the lengths of its semi-major and semi-minor axes. The size of a square or rectangle is specified by its half-width or half-height. The convention for drawing squares and rectangles is parallel to those for drawing circles and ellipses, but may be unexpected to the uninitiated.
|
76
|
+
|
77
|
+
The methods above trace outlines of the given shapes. The following methods draw filled versions:
|
78
|
+
|
79
|
+
StdDraw.filledCircle(x, y, radius)
|
80
|
+
StdDraw.filledEllipse(x, y, semiMajorAxis, semiMinorAxis)
|
81
|
+
StdDraw.filledSquare(x, y, radius)
|
82
|
+
StdDraw.filledRectangle(x, y, halfWidth, halfHeight)
|
83
|
+
|
84
|
+
### Circular arcs
|
85
|
+
|
86
|
+
You can draw circular arcs with the following method:
|
87
|
+
|
88
|
+
StdDraw.arc(x, y, radius, angle1, angle2)
|
89
|
+
|
90
|
+
The arc is from the circle centered at (x, y) of the specified radius. The arc extends from angle1 to angle2. By convention, the angles are polar (counterclockwise angle from the x-axis) and represented in degrees. For example, StdDraw.arc(0.0, 0.0, 1.0, 0, 90) draws the arc of the unit circle from 3 o'clock (0 degrees) to 12 o'clock (90 degrees).
|
91
|
+
|
92
|
+
### Polygons
|
93
|
+
|
94
|
+
You can draw polygons with the following methods:
|
95
|
+
|
96
|
+
StdDraw.polygon([] x, [] y)
|
97
|
+
StdDraw.filledPolygon([] x, [] y)
|
98
|
+
|
99
|
+
### Examples
|
100
|
+
|
101
|
+
see the [examples directory](https://github.com/gregors/standard_draw_tk/tree/master/examples)
|
102
|
+
|
27
103
|
|
28
104
|
## Development
|
29
105
|
|
30
106
|
After checking out the repo, run `bin/setup` to install dependencies. You can also run `bin/console` for an interactive prompt that will allow you to experiment.
|
31
107
|
|
32
|
-
To install this gem onto your local machine, run `bundle exec rake install`. To release a new version, update the version number in `version.rb`, and then run `bundle exec rake release`, which will create a git tag for the version, push git commits and tags, and push the `.gem` file to [rubygems.org](https://rubygems.org).
|
33
|
-
|
34
108
|
## Things to implement:
|
35
109
|
|
110
|
+
### line ends bug
|
111
|
+
* [ ] ends of arcs and line aren't rounded.
|
112
|
+
|
36
113
|
### Drawing geometric shapes
|
37
114
|
* [x] line
|
38
115
|
* [x] point
|
Binary file
|
data/lib/standard_draw_tk.rb
CHANGED
@@ -52,7 +52,7 @@ class StdDraw
|
|
52
52
|
y0 = coords.scale_y(y0)
|
53
53
|
x1 = coords.scale_x(x1)
|
54
54
|
y1 = coords.scale_y(y1)
|
55
|
-
TkcLine.new(@@canvas, x0, y0, x1, y1, fill: color)
|
55
|
+
TkcLine.new(@@canvas, x0, y0, x1, y1, width: scaled_pen_radius, fill: color)
|
56
56
|
end
|
57
57
|
|
58
58
|
def self.arc(x, y, radius, angle1, angle2)
|
@@ -71,7 +71,6 @@ class StdDraw
|
|
71
71
|
y1 = ys - hs/2
|
72
72
|
x2 = x1 + ws
|
73
73
|
y2 = y1 + hs
|
74
|
-
scaled_pen_radius = @@pen_radius * StandardDrawTk::Coordinates::DEFAULT_SIZE
|
75
74
|
TkcArc.new(@@canvas, x1, y1, x2, y2, style: :arc, start: angle1, extent: angle2 - angle1, fill: color, width: scaled_pen_radius, outline: color)
|
76
75
|
end
|
77
76
|
end
|
@@ -109,11 +108,10 @@ class StdDraw
|
|
109
108
|
end
|
110
109
|
|
111
110
|
def self.point(x, y)
|
112
|
-
draw_circle(x, y, @@pen_radius, outline: color, fill: color)
|
111
|
+
draw_circle(x, y, @@pen_radius/2, outline: color, fill: color)
|
113
112
|
end
|
114
113
|
|
115
114
|
def self.pixel(x, y)
|
116
|
-
puts 'pixel'
|
117
115
|
x1 = coords.scale_x(x).round
|
118
116
|
y1 = coords.scale_y(y).round
|
119
117
|
TkcRectangle.new(@@canvas, [x1, y1, x1 + 1, y1 + 1], outline: color, fill: color)
|
@@ -133,6 +131,10 @@ class StdDraw
|
|
133
131
|
|
134
132
|
private
|
135
133
|
|
134
|
+
def self.scaled_pen_radius
|
135
|
+
@@pen_radius * StandardDrawTk::Coordinates::DEFAULT_SIZE
|
136
|
+
end
|
137
|
+
|
136
138
|
def self.draw_polygon(x, y, fill: '', outline: nil)
|
137
139
|
throw 'x-coordinate array is null' if x.nil?
|
138
140
|
throw 'y-coordinate array is null' if y.nil?
|
@@ -141,7 +143,6 @@ class StdDraw
|
|
141
143
|
x = x.map{|i| coords.scale_x(i)}
|
142
144
|
y = y.map{|i| coords.scale_y(i)}
|
143
145
|
points = x.zip(y).flatten
|
144
|
-
scaled_pen_radius = @@pen_radius * StandardDrawTk::Coordinates::DEFAULT_SIZE
|
145
146
|
TkcPolygon.new(@@canvas, *points, width: scaled_pen_radius, fill: fill, outline: outline)
|
146
147
|
end
|
147
148
|
|
@@ -167,11 +168,11 @@ class StdDraw
|
|
167
168
|
throw 'radius must be nonnegative' if radius < 0
|
168
169
|
xs = coords.scale_x(x)
|
169
170
|
ys = coords.scale_y(y)
|
170
|
-
|
171
|
+
scaled_radius = radius * StandardDrawTk::Coordinates::DEFAULT_SIZE
|
171
172
|
if scaled_pen_radius <= 1
|
172
173
|
pixel(x, y)
|
173
174
|
else
|
174
|
-
b = coords.box(xs, ys,
|
175
|
+
b = coords.box(xs, ys, scaled_radius)
|
175
176
|
TkcOval.new(@@canvas, b, outline: outline, fill: fill)
|
176
177
|
end
|
177
178
|
end
|
data/standard_draw_tk.gemspec
CHANGED
@@ -9,8 +9,8 @@ Gem::Specification.new do |spec|
|
|
9
9
|
spec.authors = ['Gregory Ostermayr']
|
10
10
|
spec.email = ['<gregory.ostermayr@gmail.com>']
|
11
11
|
|
12
|
-
spec.summary = %q{Ruby TK version of Princeton's Standard Draw
|
13
|
-
spec.description = %q{Ruby TK version of Princeton's Standard Draw library}
|
12
|
+
spec.summary = %q{Ruby TK version of Princeton's Standard Draw}
|
13
|
+
spec.description = %q{Ruby TK version of Princeton's Standard Draw. A basic graphics drawing library.}
|
14
14
|
spec.homepage = 'https://github.com/gregors/standard_draw_tk'
|
15
15
|
spec.license = 'GPLv3'
|
16
16
|
|
metadata
CHANGED
@@ -1,72 +1,73 @@
|
|
1
1
|
--- !ruby/object:Gem::Specification
|
2
2
|
name: standard_draw_tk
|
3
3
|
version: !ruby/object:Gem::Version
|
4
|
-
version: 0.1.
|
4
|
+
version: 0.1.2
|
5
5
|
platform: ruby
|
6
6
|
authors:
|
7
7
|
- Gregory Ostermayr
|
8
|
-
autorequire:
|
8
|
+
autorequire:
|
9
9
|
bindir: bin
|
10
10
|
cert_chain: []
|
11
|
-
date: 2018-12-
|
11
|
+
date: 2018-12-30 00:00:00.000000000 Z
|
12
12
|
dependencies:
|
13
13
|
- !ruby/object:Gem::Dependency
|
14
|
+
name: tk
|
14
15
|
requirement: !ruby/object:Gem::Requirement
|
15
16
|
requirements:
|
16
17
|
- - "~>"
|
17
18
|
- !ruby/object:Gem::Version
|
18
19
|
version: 0.2.0
|
19
|
-
name: tk
|
20
|
-
prerelease: false
|
21
20
|
type: :runtime
|
21
|
+
prerelease: false
|
22
22
|
version_requirements: !ruby/object:Gem::Requirement
|
23
23
|
requirements:
|
24
24
|
- - "~>"
|
25
25
|
- !ruby/object:Gem::Version
|
26
26
|
version: 0.2.0
|
27
27
|
- !ruby/object:Gem::Dependency
|
28
|
+
name: bundler
|
28
29
|
requirement: !ruby/object:Gem::Requirement
|
29
30
|
requirements:
|
30
31
|
- - "~>"
|
31
32
|
- !ruby/object:Gem::Version
|
32
33
|
version: '1.16'
|
33
|
-
name: bundler
|
34
|
-
prerelease: false
|
35
34
|
type: :development
|
35
|
+
prerelease: false
|
36
36
|
version_requirements: !ruby/object:Gem::Requirement
|
37
37
|
requirements:
|
38
38
|
- - "~>"
|
39
39
|
- !ruby/object:Gem::Version
|
40
40
|
version: '1.16'
|
41
41
|
- !ruby/object:Gem::Dependency
|
42
|
+
name: rake
|
42
43
|
requirement: !ruby/object:Gem::Requirement
|
43
44
|
requirements:
|
44
45
|
- - "~>"
|
45
46
|
- !ruby/object:Gem::Version
|
46
47
|
version: '10.0'
|
47
|
-
name: rake
|
48
|
-
prerelease: false
|
49
48
|
type: :development
|
49
|
+
prerelease: false
|
50
50
|
version_requirements: !ruby/object:Gem::Requirement
|
51
51
|
requirements:
|
52
52
|
- - "~>"
|
53
53
|
- !ruby/object:Gem::Version
|
54
54
|
version: '10.0'
|
55
55
|
- !ruby/object:Gem::Dependency
|
56
|
+
name: rspec
|
56
57
|
requirement: !ruby/object:Gem::Requirement
|
57
58
|
requirements:
|
58
59
|
- - "~>"
|
59
60
|
- !ruby/object:Gem::Version
|
60
61
|
version: '3.0'
|
61
|
-
name: rspec
|
62
|
-
prerelease: false
|
63
62
|
type: :development
|
63
|
+
prerelease: false
|
64
64
|
version_requirements: !ruby/object:Gem::Requirement
|
65
65
|
requirements:
|
66
66
|
- - "~>"
|
67
67
|
- !ruby/object:Gem::Version
|
68
68
|
version: '3.0'
|
69
|
-
description: Ruby TK version of Princeton's Standard Draw
|
69
|
+
description: Ruby TK version of Princeton's Standard Draw. A basic graphics drawing
|
70
|
+
library.
|
70
71
|
email:
|
71
72
|
- "<gregory.ostermayr@gmail.com>"
|
72
73
|
executables: []
|
@@ -87,9 +88,12 @@ files:
|
|
87
88
|
- examples/example1.rb
|
88
89
|
- examples/example2.rb
|
89
90
|
- examples/example3.rb
|
91
|
+
- examples/example4.rb
|
92
|
+
- examples/image_example4.png
|
90
93
|
- examples/points.rb
|
91
94
|
- examples/polygon.rb
|
92
95
|
- examples/rectangle.rb
|
96
|
+
- examples/right_triangle.rb
|
93
97
|
- examples/square.rb
|
94
98
|
- examples/triangle.rb
|
95
99
|
- lib/coordinates.rb
|
@@ -100,7 +104,7 @@ homepage: https://github.com/gregors/standard_draw_tk
|
|
100
104
|
licenses:
|
101
105
|
- GPLv3
|
102
106
|
metadata: {}
|
103
|
-
post_install_message:
|
107
|
+
post_install_message:
|
104
108
|
rdoc_options: []
|
105
109
|
require_paths:
|
106
110
|
- lib
|
@@ -115,9 +119,9 @@ required_rubygems_version: !ruby/object:Gem::Requirement
|
|
115
119
|
- !ruby/object:Gem::Version
|
116
120
|
version: '0'
|
117
121
|
requirements: []
|
118
|
-
rubyforge_project:
|
119
|
-
rubygems_version: 2.7.
|
120
|
-
signing_key:
|
122
|
+
rubyforge_project:
|
123
|
+
rubygems_version: 2.7.7
|
124
|
+
signing_key:
|
121
125
|
specification_version: 4
|
122
|
-
summary: Ruby TK version of Princeton's Standard Draw
|
126
|
+
summary: Ruby TK version of Princeton's Standard Draw
|
123
127
|
test_files: []
|