skippy 0.3.0.a → 0.4.0.a
Sign up to get free protection for your applications and to get access to all the features.
- checksums.yaml +4 -4
- data/README.md +26 -1
- data/app/commands/sketchup.rb +61 -0
- data/lib/skippy/os.rb +17 -0
- data/lib/skippy/os/common.rb +26 -0
- data/lib/skippy/os/mac.rb +32 -0
- data/lib/skippy/os/win.rb +56 -0
- data/lib/skippy/sketchup/app.rb +11 -0
- data/lib/skippy/version.rb +1 -1
- metadata +8 -2
checksums.yaml
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
---
|
2
2
|
SHA256:
|
3
|
-
metadata.gz:
|
4
|
-
data.tar.gz:
|
3
|
+
metadata.gz: 8911df6fd31addc62c4bc4756eb46f715eaf70ea2d2bcfb32175f104dad78f82
|
4
|
+
data.tar.gz: dbb042f7ed689a8d1a01589b4f11479014da36861e1cf578453a75eab14028b0
|
5
5
|
SHA512:
|
6
|
-
metadata.gz:
|
7
|
-
data.tar.gz:
|
6
|
+
metadata.gz: fda57b8e19f1a22ab2ad207bd938df1a5f9ba158400d5126572dc518130f48614cd30082aa4b4ac800fd33aa7c38fd32c712dfd4c7c5aac36432bc21762c0b6d
|
7
|
+
data.tar.gz: e43b4802ac2f2b0c2a60e5b8da927e9cb19cdcaee74d38c021422ebb571a7395363184f6828eccd23c66055ba2d078e8f30162849543a02d081cfcd05df853a5
|
data/README.md
CHANGED
@@ -14,7 +14,8 @@ Some of the main goals are:
|
|
14
14
|
- [ ] Add/Remove/Update user templates
|
15
15
|
- [ ] Automate common tasks
|
16
16
|
- [ ] Packaging the extension
|
17
|
-
- [
|
17
|
+
- [x] Launch SketchUp
|
18
|
+
- [x] Launch SketchUp in debug mode
|
18
19
|
- [x] Easy interface to add per-project custom commands/tasks
|
19
20
|
- [x] Library dependency management
|
20
21
|
- [x] Pull in third-party modules into extension namespace
|
@@ -201,6 +202,30 @@ For more examples, refer to:
|
|
201
202
|
* [github.com/thomthom/tt-lib](https://github.com/thomthom/tt-lib)
|
202
203
|
* [github.com/thomthom/skippy-test-lib](https://github.com/thomthom/skippy-test-lib)
|
203
204
|
|
205
|
+
### Launching SketchUp
|
206
|
+
|
207
|
+
Skippy will attempt to locate your SketchUp installations and offer commands to launch them.
|
208
|
+
|
209
|
+
These commands provides a cross platform way of launching SketchUp which can be useful in build scripts or IDE automation tasks.
|
210
|
+
|
211
|
+
(**Note:** Currently Skippy assumes that SketchUp is installed to its default installation directory.)
|
212
|
+
|
213
|
+
`skippy sketchup:list` will display a list of all known SketchUp versions on the system.
|
214
|
+
|
215
|
+
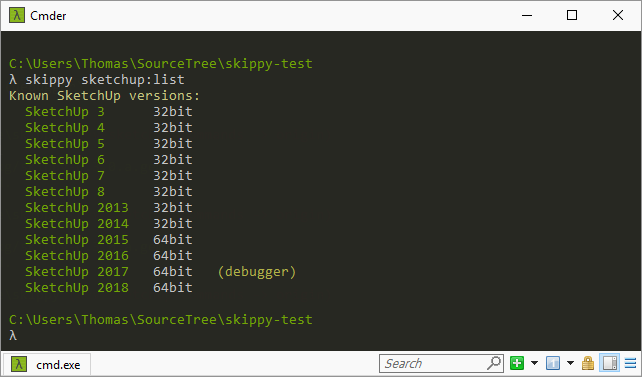
|
216
|
+
|
217
|
+
To launch one, use `skippy sketchup:launch VERSION`, for instance:
|
218
|
+
|
219
|
+
```
|
220
|
+
skippy sketchup:launch 2018
|
221
|
+
```
|
222
|
+
|
223
|
+
You can also launch SketchUp in debug mode if the [SketchUp Ruby Debugger library](https://github.com/SketchUp/sketchup-ruby-debugger) is installed.
|
224
|
+
|
225
|
+
```
|
226
|
+
skippy sketchup:debug 2018
|
227
|
+
```
|
228
|
+
|
204
229
|
## Development
|
205
230
|
|
206
231
|
After checking out the repository, run `bin/setup` to install dependencies. Then, run `rake test` to run the tests. You can also run `bin/console` for an interactive prompt that will allow you to experiment.
|
@@ -0,0 +1,61 @@
|
|
1
|
+
require 'json'
|
2
|
+
require 'stringio'
|
3
|
+
|
4
|
+
require 'skippy/error'
|
5
|
+
require 'skippy/os'
|
6
|
+
|
7
|
+
class Sketchup < Skippy::Command
|
8
|
+
|
9
|
+
include Thor::Actions
|
10
|
+
|
11
|
+
option :port, :type => :numeric, :default => 7000
|
12
|
+
desc 'debug VERSION', 'Start SketchUp with Ruby Debugger'
|
13
|
+
def debug(version)
|
14
|
+
app = find_sketchup(version)
|
15
|
+
unless app.can_debug
|
16
|
+
raise Skippy::Error, "Debug library not installed for Sketchup #{version}"
|
17
|
+
end
|
18
|
+
arguments = ['-rdebug', %("ide port=#{options.port}")]
|
19
|
+
Skippy.os.launch_app(app.executable, *arguments)
|
20
|
+
end
|
21
|
+
|
22
|
+
desc 'launch VERSION', 'Start SketchUp'
|
23
|
+
def launch(version)
|
24
|
+
app = find_sketchup(version)
|
25
|
+
Skippy.os.launch_app(app.executable)
|
26
|
+
end
|
27
|
+
|
28
|
+
desc 'list', 'List all known SketchUp versions'
|
29
|
+
def list
|
30
|
+
say shell.set_color('Known SketchUp versions:', :yellow, true)
|
31
|
+
Skippy.os.sketchup_apps.each { |sketchup|
|
32
|
+
version = sketchup.version.to_s.ljust(4)
|
33
|
+
sketchup_name = "SketchUp #{version}"
|
34
|
+
bitness = sketchup.is64bit ? '64bit' : '32bit'
|
35
|
+
debug = sketchup.can_debug ? '(debugger)' : ''
|
36
|
+
# TODO(thomthom): Use print_table ?
|
37
|
+
output = StringIO.new
|
38
|
+
output.write ' '
|
39
|
+
output.write shell.set_color(sketchup_name, :green, false)
|
40
|
+
output.write ' '
|
41
|
+
output.write bitness
|
42
|
+
output.write ' '
|
43
|
+
output.write shell.set_color(debug, :yellow, false)
|
44
|
+
say output.string
|
45
|
+
}
|
46
|
+
end
|
47
|
+
default_command(:list)
|
48
|
+
|
49
|
+
private
|
50
|
+
|
51
|
+
# @param [Integer] version
|
52
|
+
# @return [Skippy::SketchUpApp, nil]
|
53
|
+
def find_sketchup(version)
|
54
|
+
app = Skippy.os.sketchup_apps.find { |sketchup|
|
55
|
+
sketchup.version == version.to_i
|
56
|
+
}
|
57
|
+
raise Skippy::Error, "SketchUp #{version} not found." if app.nil?
|
58
|
+
app
|
59
|
+
end
|
60
|
+
|
61
|
+
end
|
data/lib/skippy/os.rb
ADDED
@@ -0,0 +1,26 @@
|
|
1
|
+
class Skippy::OSCommon
|
2
|
+
|
3
|
+
# @param [String] command
|
4
|
+
def execute_command(command)
|
5
|
+
# Something with a Thor application like skippy get the 'RUBYLIB'
|
6
|
+
# environment set which prevents SketchUp from finding its StdLib
|
7
|
+
# directories. (At least under Windows.) This relates to child processes
|
8
|
+
# inheriting the environment variables of its parent.
|
9
|
+
# To work around this we unset RUBYLIB before launching SketchUp. This
|
10
|
+
# doesn't affect skippy as it's about to exit as soon as SketchUp starts
|
11
|
+
# any way.
|
12
|
+
ENV['RUBYLIB'] = nil if ENV['RUBYLIB']
|
13
|
+
id = spawn(command)
|
14
|
+
Process.detach(id)
|
15
|
+
end
|
16
|
+
|
17
|
+
# @param [String] path
|
18
|
+
def launch_app(path, *args)
|
19
|
+
raise NotImplementedError
|
20
|
+
end
|
21
|
+
|
22
|
+
def sketchup_apps
|
23
|
+
raise NotImplementedError
|
24
|
+
end
|
25
|
+
|
26
|
+
end
|
@@ -0,0 +1,32 @@
|
|
1
|
+
require 'skippy/os/common'
|
2
|
+
require 'skippy/sketchup/app'
|
3
|
+
|
4
|
+
class Skippy::OSMac < Skippy::OSCommon
|
5
|
+
|
6
|
+
# @param [String] executable_path
|
7
|
+
def launch_app(executable_path, *args)
|
8
|
+
command = %(open -a "#{executable_path}")
|
9
|
+
unless args.empty?
|
10
|
+
command << " --args #{args.join(' ')}"
|
11
|
+
end
|
12
|
+
execute_command(command)
|
13
|
+
end
|
14
|
+
|
15
|
+
def sketchup_apps
|
16
|
+
apps = []
|
17
|
+
pattern = '/Applications/SketchUp */'
|
18
|
+
Dir.glob(pattern) { |path|
|
19
|
+
app = File.join(path, 'SketchUp.app')
|
20
|
+
debug_lib = File.join(app, 'Contents/Frameworks/SURubyDebugger.dylib')
|
21
|
+
version = File.basename(path).match(/[0-9.]+$/)[0].to_i
|
22
|
+
apps << Skippy::SketchUpApp.from_hash(
|
23
|
+
executable: app,
|
24
|
+
version: version,
|
25
|
+
can_debug: File.exist?(debug_lib),
|
26
|
+
is64bit: version > 2015,
|
27
|
+
)
|
28
|
+
}
|
29
|
+
apps.sort_by(&:version)
|
30
|
+
end
|
31
|
+
|
32
|
+
end
|
@@ -0,0 +1,56 @@
|
|
1
|
+
require 'skippy/os/common'
|
2
|
+
require 'skippy/sketchup/app'
|
3
|
+
|
4
|
+
class Skippy::OSWin < Skippy::OSCommon
|
5
|
+
|
6
|
+
# Note: This is not a good indication to 32bit bs 64bit. It's a naive
|
7
|
+
# assumption that will fail when SketchUp is installed to a
|
8
|
+
# non-standard location.
|
9
|
+
SYSTEM_32BIT = ENV['ProgramFiles(x86)'].nil? && ENV['ProgramW6432'].nil?
|
10
|
+
SYSTEM_64BIT = !SYSTEM_32BIT
|
11
|
+
|
12
|
+
PROGRAM_FILES_64BIT = File.expand_path(ENV['ProgramW6432'])
|
13
|
+
|
14
|
+
# @param [String] executable_path
|
15
|
+
def launch_app(executable_path, *args)
|
16
|
+
command = %("#{executable_path}")
|
17
|
+
unless args.empty?
|
18
|
+
command << " #{args.join(' ')}"
|
19
|
+
end
|
20
|
+
execute_command(command)
|
21
|
+
end
|
22
|
+
|
23
|
+
def sketchup_apps
|
24
|
+
# TODO(thomthom): Find by registry information.
|
25
|
+
apps = []
|
26
|
+
program_files_paths.each { |program_files|
|
27
|
+
pattern = "#{program_files}/{@Last Software,Google,SketchUp}/*SketchUp *"
|
28
|
+
Dir.glob(pattern) { |path|
|
29
|
+
exe = File.join(path, 'SketchUp.exe')
|
30
|
+
debug_dll = File.join(path, 'SURubyDebugger.dll')
|
31
|
+
version = File.basename(path).match(/[0-9.]+$/)[0].to_i
|
32
|
+
apps << Skippy::SketchUpApp.from_hash(
|
33
|
+
executable: exe,
|
34
|
+
version: version,
|
35
|
+
can_debug: File.exist?(debug_dll),
|
36
|
+
is64bit: SYSTEM_64BIT && path.start_with?("#{PROGRAM_FILES_64BIT}/"),
|
37
|
+
)
|
38
|
+
}
|
39
|
+
}
|
40
|
+
apps.sort_by(&:version)
|
41
|
+
end
|
42
|
+
|
43
|
+
private
|
44
|
+
|
45
|
+
def program_files_paths
|
46
|
+
paths = []
|
47
|
+
if SYSTEM_64BIT
|
48
|
+
paths << File.expand_path(ENV['ProgramFiles(x86)'])
|
49
|
+
paths << File.expand_path(ENV['ProgramW6432'])
|
50
|
+
else
|
51
|
+
paths << File.expand_path(ENV['ProgramFiles'])
|
52
|
+
end
|
53
|
+
paths
|
54
|
+
end
|
55
|
+
|
56
|
+
end
|
data/lib/skippy/version.rb
CHANGED
metadata
CHANGED
@@ -1,14 +1,14 @@
|
|
1
1
|
--- !ruby/object:Gem::Specification
|
2
2
|
name: skippy
|
3
3
|
version: !ruby/object:Gem::Version
|
4
|
-
version: 0.
|
4
|
+
version: 0.4.0.a
|
5
5
|
platform: ruby
|
6
6
|
authors:
|
7
7
|
- Thomas Thomassen
|
8
8
|
autorequire:
|
9
9
|
bindir: exe
|
10
10
|
cert_chain: []
|
11
|
-
date: 2017-12-
|
11
|
+
date: 2017-12-20 00:00:00.000000000 Z
|
12
12
|
dependencies:
|
13
13
|
- !ruby/object:Gem::Dependency
|
14
14
|
name: git
|
@@ -159,6 +159,7 @@ files:
|
|
159
159
|
- app/commands/install.rb
|
160
160
|
- app/commands/lib.rb
|
161
161
|
- app/commands/new.rb
|
162
|
+
- app/commands/sketchup.rb
|
162
163
|
- app/commands/template.rb
|
163
164
|
- app/resources/commands/example.rb
|
164
165
|
- app/templates/standard/%ext_name%.rb.tt
|
@@ -226,7 +227,12 @@ files:
|
|
226
227
|
- lib/skippy/library_manager.rb
|
227
228
|
- lib/skippy/module_manager.rb
|
228
229
|
- lib/skippy/namespace.rb
|
230
|
+
- lib/skippy/os.rb
|
231
|
+
- lib/skippy/os/common.rb
|
232
|
+
- lib/skippy/os/mac.rb
|
233
|
+
- lib/skippy/os/win.rb
|
229
234
|
- lib/skippy/project.rb
|
235
|
+
- lib/skippy/sketchup/app.rb
|
230
236
|
- lib/skippy/version.rb
|
231
237
|
- skippy.gemspec
|
232
238
|
homepage: https://github.com/thomthom/skippy
|