sewing_kit 0.27.3 → 0.28.0
Sign up to get free protection for your applications and to get access to all the features.
- checksums.yaml +4 -4
- data/README.md +1 -9
- data/lib/sewing_kit/version.rb +1 -1
- data/lib/sewing_kit/webpack/dev.rb +1 -1
- data/lib/sewing_kit/webpack/helper.rb +9 -1
- data/lib/sewing_kit/webpack/webpack.rb +1 -1
- metadata +2 -2
checksums.yaml
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
---
|
2
2
|
SHA1:
|
3
|
-
metadata.gz:
|
4
|
-
data.tar.gz:
|
3
|
+
metadata.gz: 0bea84b065d0c7713ba5973213988b614db786e3
|
4
|
+
data.tar.gz: 2bd89efe8f95ca2c9868f211c3051854d934ac23
|
5
5
|
SHA512:
|
6
|
-
metadata.gz:
|
7
|
-
data.tar.gz:
|
6
|
+
metadata.gz: 7869086387d0f21dc8e22d49ad1863c4aefd8b57a01902bbb336c1f52910862388a60d759e27026f7d4d2b69b434d9b1dde90e07fb8abd5bd7e769bfa6f7cc23
|
7
|
+
data.tar.gz: d5d3805841c1a0d33a50cbb822d178bb2ec9f5c68239cd6feb649b3fb69af6829095c27051207f4547d27d414e41e22148c645900c896e4a9a271570b65ac17e
|
data/README.md
CHANGED
@@ -11,8 +11,7 @@ Create a Rails project using `dev init` then:
|
|
11
11
|
```sh
|
12
12
|
# Add Ruby/Node dependencies
|
13
13
|
bundle add sewing_kit
|
14
|
-
yarn add
|
15
|
-
yarn add --dev react-hot-loader@3.0.0-beta.7
|
14
|
+
yarn add @shopify/sewing-kit
|
16
15
|
|
17
16
|
# Optional - add Polaris
|
18
17
|
yarn add @shopify/polaris react react-dom
|
@@ -91,13 +90,6 @@ If sewing-kit makes a breaking change, this gem's minor version will be bumped t
|
|
91
90
|
It is currently not recommended to use `sprockets-commoner` and `sewing_kit` in the same project.
|
92
91
|
Minimally, it is required that the project does not have its own `babel-*` libraries that `sewing-kit` currently has as [dependencies](https://github.com/Shopify/sewing-kit/blob/master/package.json#L97~L102).
|
93
92
|
|
94
|
-
## Build Pipeline Gotchas
|
95
|
-
While sewing-kit is a dev dependency, it is needed for production builds. This means that the production build will break if the environment variable `YARN_INSTALL` is set to true, because it will prevent `devDependencies` from being installed.
|
96
|
-
|
97
|
-
To work around this in Buildkite, set `YARN_PRODUCTION=false` in the Environment Variables section of Pipeline Settings:
|
98
|
-
|
99
|
-
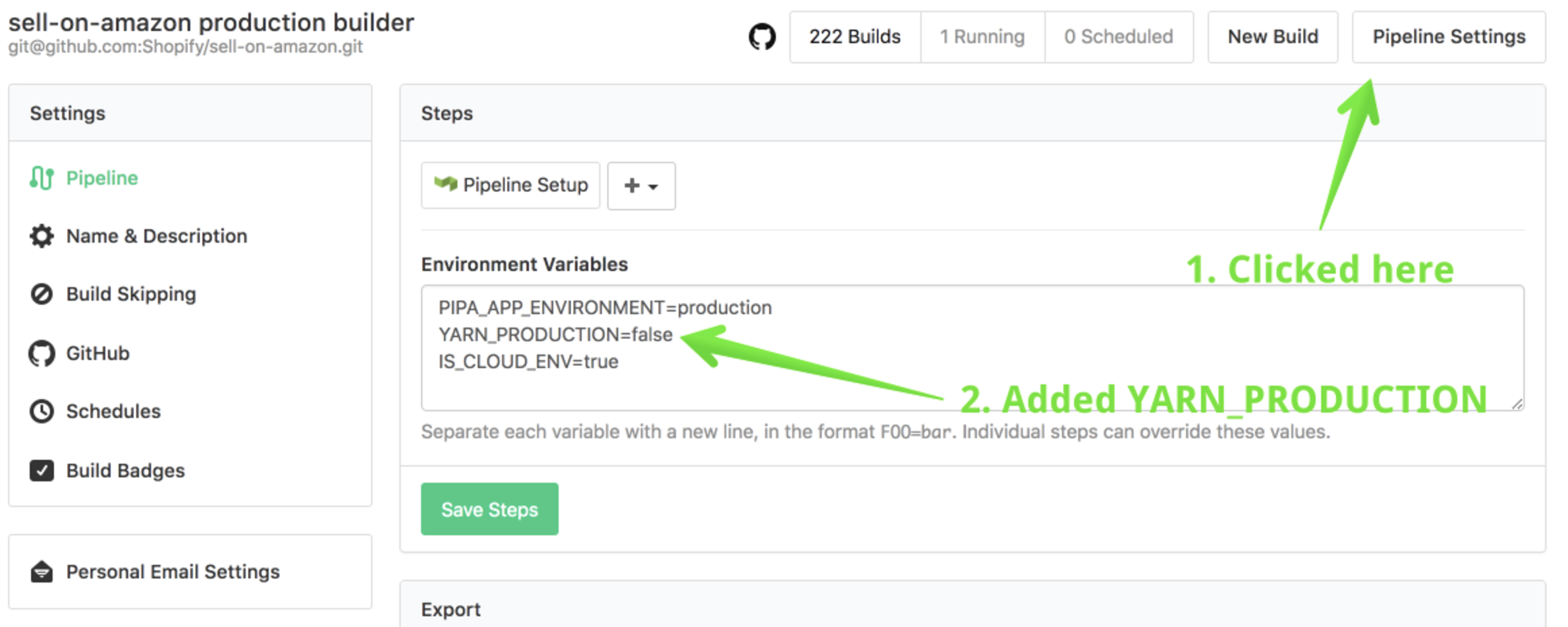
|
100
|
-
|
101
93
|
## React Boilerplate
|
102
94
|
* Create a React app in `app/ui/App.js` ([example](https://github.com/Shopify/rails_sewing_kit_example/blob/master/app/ui/App.jsx))
|
103
95
|
* In an `erb` view, add a placeholder for React content ([example](https://github.com/Shopify/rails_sewing_kit_example/blob/master/app/views/home/index.html.erb#L4))
|
data/lib/sewing_kit/version.rb
CHANGED
@@ -8,15 +8,18 @@ module SewingKit
|
|
8
8
|
module Helper
|
9
9
|
include ActionView::Helpers
|
10
10
|
|
11
|
-
class
|
11
|
+
class UnknownAssetError < StandardError
|
12
12
|
end
|
13
13
|
|
14
14
|
Asset = Struct.new(:path, :integrity)
|
15
15
|
|
16
|
+
# rubocop:disable Metrics/CyclomaticComplexity, Metrics/PerceivedComplexity
|
16
17
|
def sewing_kit_assets(entrypoint_name, extension: 'js')
|
17
18
|
return '' unless entrypoint_name.present?
|
18
19
|
|
19
20
|
assets = SewingKit::Webpack::Manifest.asset_dependencies(entrypoint_name)
|
21
|
+
|
22
|
+
raise UnknownAssetError, "#{entrypoint_name} was not found in manifest." if raise_unknown_error? assets
|
20
23
|
return [] unless assets && assets[extension]
|
21
24
|
|
22
25
|
dependencies = assets[extension]
|
@@ -25,6 +28,7 @@ module SewingKit
|
|
25
28
|
Asset.new(raw_asset['path'], raw_asset['integrity'])
|
26
29
|
end
|
27
30
|
end
|
31
|
+
# rubocop:enable Metrics/CyclomaticComplexity, Metrics/PerceivedComplexity
|
28
32
|
|
29
33
|
def sewing_kit_link_tag(*assets)
|
30
34
|
options = assets.extract_options!
|
@@ -74,6 +78,10 @@ module SewingKit
|
|
74
78
|
Rails.env.development?
|
75
79
|
end
|
76
80
|
|
81
|
+
def raise_unknown_error?(assets)
|
82
|
+
assets.nil? && serve_development_assets?
|
83
|
+
end
|
84
|
+
|
77
85
|
def dll_asset
|
78
86
|
Asset.new('/webpack/assets/dll/vendor.js')
|
79
87
|
end
|
@@ -10,7 +10,7 @@ module SewingKit
|
|
10
10
|
class NodeSewingKitNotRunnable < StandardError
|
11
11
|
def initialize(mode, cause = nil)
|
12
12
|
env_message = if 'development' == mode
|
13
|
-
"Try `yarn add
|
13
|
+
"Try `yarn add @shopify/sewing-kit`"
|
14
14
|
else
|
15
15
|
"\nIf this is a container build, try:\n" \
|
16
16
|
" - Adding #{highlight('https://github.com/heroku/heroku-buildpack-nodejs')} to your " \
|
metadata
CHANGED
@@ -1,14 +1,14 @@
|
|
1
1
|
--- !ruby/object:Gem::Specification
|
2
2
|
name: sewing_kit
|
3
3
|
version: !ruby/object:Gem::Version
|
4
|
-
version: 0.
|
4
|
+
version: 0.28.0
|
5
5
|
platform: ruby
|
6
6
|
authors:
|
7
7
|
- Chris Sauve
|
8
8
|
autorequire:
|
9
9
|
bindir: bin
|
10
10
|
cert_chain: []
|
11
|
-
date: 2018-
|
11
|
+
date: 2018-06-21 00:00:00.000000000 Z
|
12
12
|
dependencies:
|
13
13
|
- !ruby/object:Gem::Dependency
|
14
14
|
name: railties
|